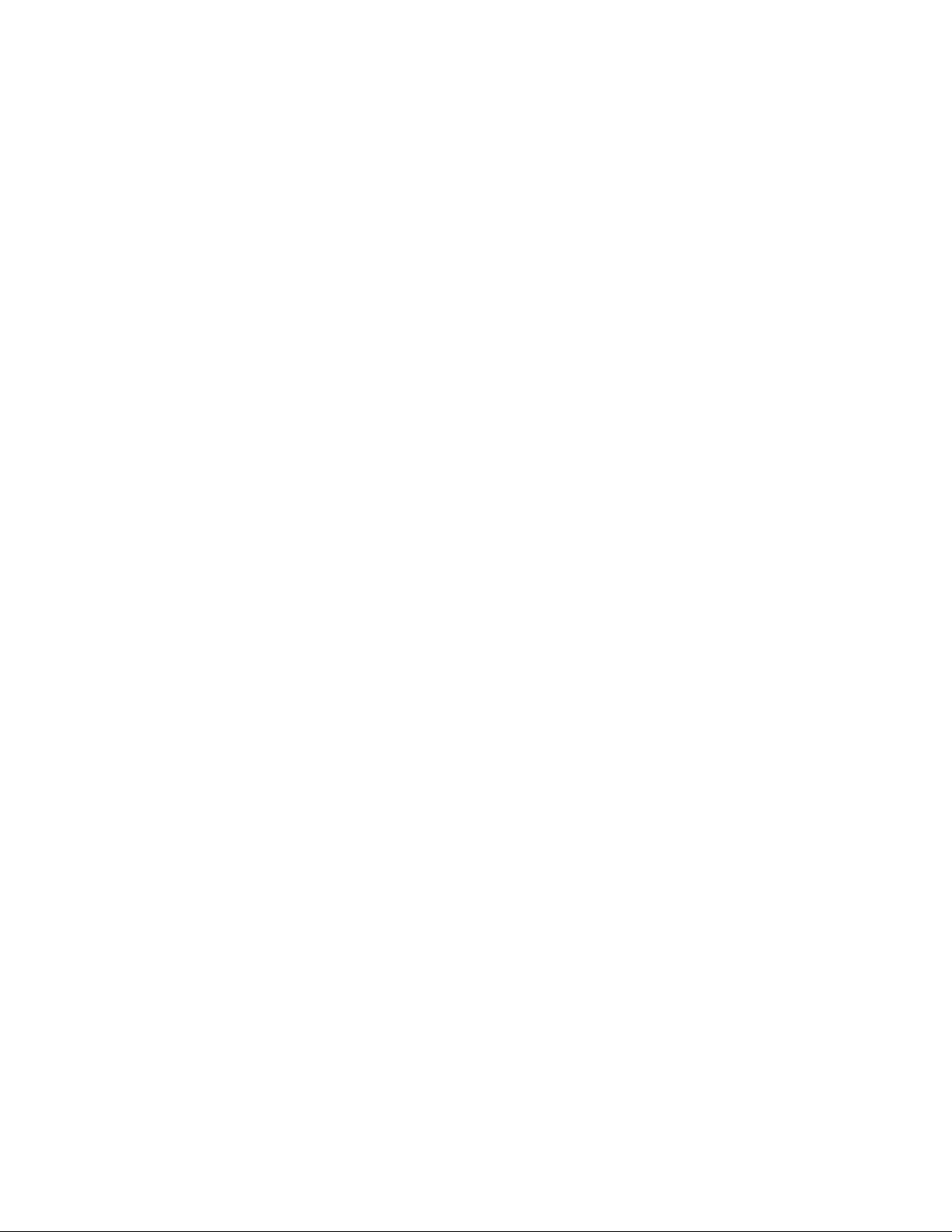
Table of Contents
1. Driver Basics....................................................................................................................15
Driver Entry and Exit points ....................................................................................15
module_init .......................................................................................................15
module_exit.......................................................................................................15
Atomic and pointer manipulation...........................................................................16
atomic_read .......................................................................................................16
atomic_set ..........................................................................................................16
atomic_add ........................................................................................................17
atomic_sub.........................................................................................................18
atomic_sub_and_test........................................................................................18
atomic_inc..........................................................................................................19
atomic_dec.........................................................................................................20
atomic_dec_and_test ........................................................................................20
atomic_inc_and_test.........................................................................................21
atomic_add_negative .......................................................................................21
get_unaligned....................................................................................................22
put_unaligned...................................................................................................23
Delaying, scheduling, and timer routines ..............................................................23
schedule_timeout..............................................................................................24
2. Data Types........................................................................................................................25
Doubly Linked Lists...................................................................................................25
list_add...............................................................................................................25
list_add_tail .......................................................................................................25
list_del ................................................................................................................26
list_del_init ........................................................................................................26
list_empty ..........................................................................................................27
list_splice............................................................................................................27
list_entry ............................................................................................................28
list_for_each.......................................................................................................29
3. Basic C Library Functions .............................................................................................31
String Conversions.....................................................................................................31
simple_strtol......................................................................................................31
simple_strtoll.....................................................................................................31
simple_strtoul....................................................................................................32
simple_strtoull ..................................................................................................33
vsnprintf.............................................................................................................33
snprintf...............................................................................................................34
vsprintf ...............................................................................................................35
sprintf .................................................................................................................35
String Manipulation...................................................................................................36
strcpy ..................................................................................................................36
strncpy................................................................................................................37
strcat....................................................................................................................37
strncat .................................................................................................................38
strcmp.................................................................................................................39
strncmp ..............................................................................................................39
strchr...................................................................................................................40
strrchr .................................................................................................................40
strlen ...................................................................................................................41
strnlen.................................................................................................................41
5