/*******************************************************************************
* 文件名称:basic_rf.c
* 功 能:Basic RF 库
* 作 者:POWER
* 公 司:湘潭斯凯电子科技有限公司
* www.sikai-tech.com
* 日 期:2009-09-09
******************************************************************************/
/* 包含头文件 */
/********************************************************************/
#include "hal_int.h"
#include "hal_mcu.h" // 为了使用 halMcuWaitUs()
#include "hal_rf.h"
#ifdef SECURITY_CCM
#include "hal_rf_security.h"
#endif
#include "basic_rf.h"
#ifdef SECURITY_CCM
#include "basic_rf_security.h"
#endif
#include "util.h"
#include "string.h"
/********************************************************************/
/* 宏定义 */
/********************************************************************/
// 数据包长度相关定义
#define PKT_LEN_MIC 8
#define PKT_LEN_SEC PKT_LEN_UNSEC + PKT_LEN_MIC
#define PKT_LEN_AUTH 8
#define PKT_LEN_ENCR 24
// 数据包格式:长度字段(1字节) 帧控制字段(2字节) 序号字段(1字节) 个域网ID字段(2字节)
// 目的地址字段(2字节) 源地址字段(2字节) 帧载荷字段(长度可变) 帧校验序列(2字节)
// 注意:长度字段不包含在数据包开销中
#define BASIC_RF_PACKET_OVERHEAD_SIZE ((2 + 1 + 2 + 2 + 2) + (2))
#define BASIC_RF_MAX_PAYLOAD_SIZE (127 - BASIC_RF_PACKET_OVERHEAD_SIZE - \
BASIC_RF_AUX_HDR_LENGTH - BASIC_RF_LEN_MIC)
#define BASIC_RF_ACK_PACKET_SIZE 5 // 确认帧长度为5字节
#define BASIC_RF_FOOTER_SIZE 2
#define BASIC_RF_HDR_SIZE 10
/* 确认期限:在发送完数据包后等待对该数据包确认的时间 */
#define BASIC_RF_ACK_DURATION (0.5 * 32 * 2 * ((4 + 1) + (1) + (2 + 1) + (2)))
#define BASIC_RF_SYMBOL_DURATION (32 * 0.5) // 符号期限
#define BASIC_RF_PLD_LEN_MASK 0x7F // 长度字段掩码
/* 帧控制字段 */
#define BASIC_RF_FCF_NOACK 0x8841
#define BASIC_RF_FCF_ACK 0x8861
#define BASIC_RF_FCF_ACK_BM 0x0020
#define BASIC_RF_FCF_BM (~BASIC_RF_FCF_ACK_BM)
#define BASIC_RF_SEC_ENABLED_FCF_BM 0x0008
// 帧控制字段LSB
#define BASIC_RF_FCF_NOACK_L LO_UINT16(BASIC_RF_FCF_NOACK)
#define BASIC_RF_FCF_ACK_L LO_UINT16(BASIC_RF_FCF_ACK)
#define BASIC_RF_FCF_ACK_BM_L LO_UINT16(BASIC_RF_FCF_ACK_BM)
#define BASIC_RF_FCF_BM_L LO_UINT16(BASIC_RF_FCF_BM)
#define BASIC_RF_SEC_ENABLED_FCF_BM_L LO_UINT16(BASIC_RF_SEC_ENABLED_FCF_BM)
/* 辅助安全首部 */
#define BASIC_RF_AUX_HDR_LENGTH 5
#define BASIC_RF_LEN_AUTH BASIC_RF_PACKET_OVERHEAD_SIZE + \
BASIC_RF_AUX_HDR_LENGTH - BASIC_RF_FOOTER_SIZE
#define BASIC_RF_SECURITY_M 2
#define BASIC_RF_LEN_MIC 8
#ifdef SECURITY_CCM
#undef BASIC_RF_HDR_SIZE
#define BASIC_RF_HDR_SIZE 15
#endif
#define BASIC_RF_CRC_OK_BM 0x80 // CRC校验掩码
/********************************************************************/
/* 类型定义 */
/********************************************************************/
// 接收信息结构体
/*typedef struct
{
uint8 seqNumber;
uint16 srcAddr;
uint16 srcPanId;
int8 length;
uint8* pPayload;
uint8 ackRequest;
int8 rssi;
volatile uint8 isReady;
uint8 status;
} basicRfRxInfo_t;*/
// 发送状态结构体
typedef struct
{
uint8 txSeqNumber;
volatile uint8 ackReceived;
uint8 receiveOn;
uint32 frameCounter;
} basicRfTxState_t;
// Basic RF 数据包首部(IEEE 802.15.4)
typedef struct
{
uint8 packetLength;
uint8 fcf0; // Frame control field LSB
uint8 fcf1; // Frame control field MSB
uint8 seqNumber;
uint16 panId;
uint16 destAddr;
uint16 srcAddr;
#ifdef SECURITY_CCM
uint8 securityControl;
uint8 frameCounter[4];
#endif
} basicRfPktHdr_t;
/********************************************************************/
/* 本地变量 */
/********************************************************************/
static basicRfRxInfo_t rxi= { 0xFF }; // Make sure sequence numbers are
static basicRfTxState_t txState= { 0x00 }; // initialised and distinct.
static basicRfCfg_t* pConfig;
static uint8 txMpdu[BASIC_RF_MAX_PAYLOAD_SIZE+BASIC_RF_PACKET_OVERHEAD_SIZE+1];
static uint8 rxMpdu[128];
/********************************************************************/
/* 本地函数 */
/*********************************************************************
* 函数名称:basicRfBuildHeader
* 功 能:根据IEEE 802.15.4帧格式构造数据包首部
* 入口参数:buffer 指向写首部的缓冲区的指针
* destAddr 目的短地址
* payloadLength 载荷长度
* 出口参数:无
* 返 回 值:首部长度
********************************************************************/
static uint8 basicRfBuildHeader(uint8* buffer, uint16 destAddr, uint8 payloadLength)
{
basicRfPktHdr_t *pHdr;
uint16 fcf;
pHdr= (basicRfPktHdr_t*)buffer;
/* 填充数据包首部 */
pHdr->packetLength = payloadLength + BASIC_RF_PACKET_OVERHEAD_SIZE;
//pHdr->frameControlField = pConfig->ackRequest ? BASIC_RF_FCF_ACK : BASIC_RF_FCF_NOACK;
fcf= pConfig->ackRequest ? BASIC_RF_FCF_ACK : BASIC_RF_FCF_NOACK;
pHdr->fcf0 = LO_UINT16(fcf);
pHdr->fcf1 = HI_UINT16(fcf);
pHdr->seqNumber= txState.txSeqNumber;
pHdr->panId= pConfig->panId;
pHdr->destAddr= destAddr;
pHdr->srcAddr= pConfig->myAddr;
/* 使用安全机制时,对首部还要进行如下构造 */
#ifdef SECURITY_CCM
// Add security to FCF, length and security header
pHdr->fcf0 |= BASIC_RF_SEC_ENABLED_FCF_BM_L;
pHdr->packetLength += PKT_LEN_MIC;
pHdr->packetLength += BASIC_RF_AUX_HDR_LENGTH;
pHdr->securityControl= SECURITY_CONTROL;
pHdr->frameCounter[0]= LO_UINT16(LO_UINT32(txState.frameCounter));
pHdr->frameCounter[1]= HI_UINT16(LO_UINT32(txState.frameCounter));
pHdr->frameCounter[2]= LO_UINT16(HI_UINT32(txState.frameCounter));
pHdr->frameCounter[3]= HI_UINT16(HI_UINT32(txState.frameCounter));
#endif
/* 确保各字段是网络字节顺序 */
UINT16_HTON(pHdr->panId);
UINT16_HTON(pHdr->destAddr);
UINT16_HTON(pHdr->srcAddr);
return BASIC_RF_HDR_SIZE;
}
/*********************************************************************
* 函数名称:basicRfBuildMpdu
* 功 能:根据IEEE 802.15.4帧格式构造MAC层协议数据单元MPDU(MAC首部+载荷)
* 入口参数:destAddr 目的短地址
* pPayload 指向存储负载的缓冲区的指针
* payloadLength 载荷长度
* 出口参数:无
* 返 回 值:MPDU长度
********************************************************************/
static uint8 basicRfBuildMpdu(uint16 destAddr, uint8* pPayload, uint8 payloadLength)
{
uint8 hdrLength, n;
hdrLength = basicRfBuildHeader(txMpdu, destAddr, payloadLength);
for(n=0;n<payloadLength;n++)
{
txMpdu[hdrLength+n] = pPayload[n];
}
return hdrLength + payloadLength; // 全部mpdu长度
}
/*********************************************************************
* 函数名称:basicRfRxFrmDoneIsr
* 功 能:对从射频接收到的数据帧(数据或确认)的中断服务函数
* 入口参数:无
* 出口参数:rxi 从最后输入的帧中提取的信息
* txState 发送状态信息
* 返 回 值:无
********************************************************************/
static void basicRfRxFrmDoneIsr(void)
{
basicRfPktHdr_t *pHdr;
uint8 *pStatusWord;
#ifdef SECURITY_CCM
uint8 authStatus=0;
#endif
pHdr= (basicRfPktHdr_t*)rxMpdu; // 映射首部到数据包缓冲区
halRfDisableRxInterrupt(); // 清除中断标志并禁止接收中断
halIntOn(); // 使能其他所有的中断源(使能中断嵌套)
halRfReadRxBuf(&pHdr->packetLength,1); // 读取载荷
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
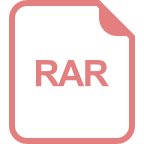
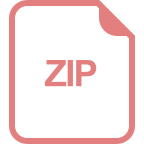
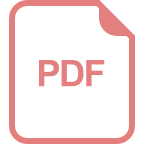
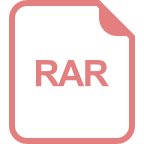
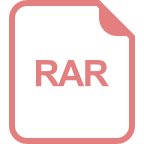
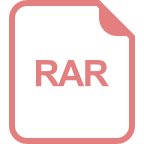
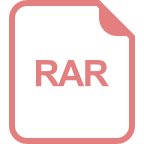
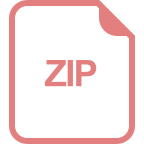
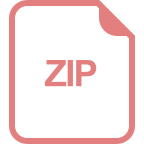
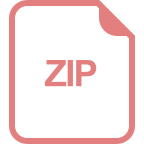
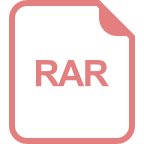
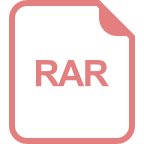
收起资源包目录

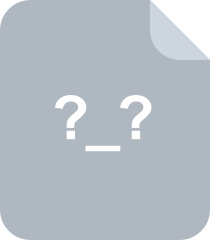
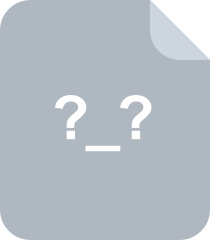
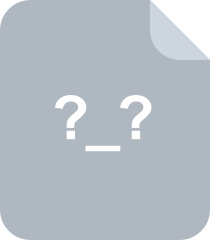
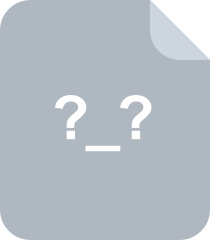
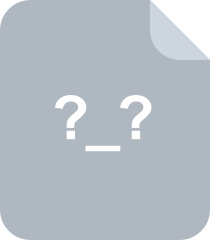
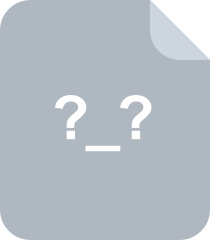
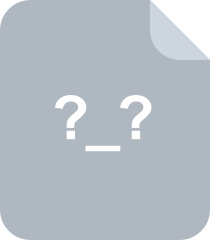
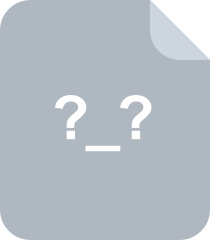
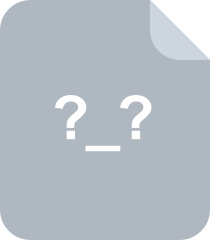
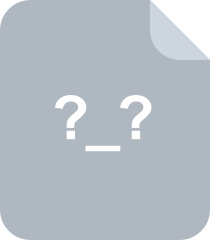
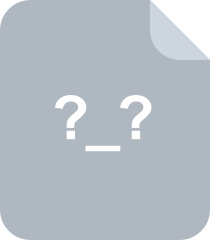
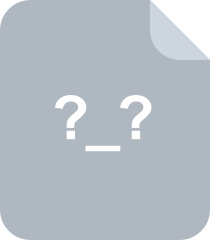
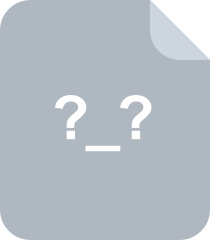
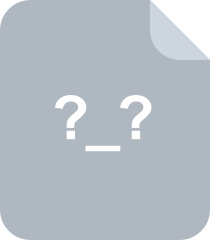
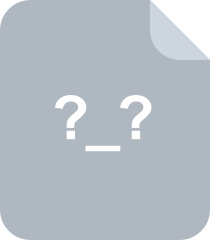
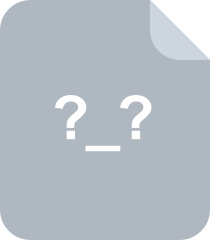
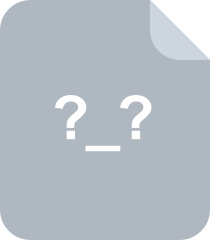
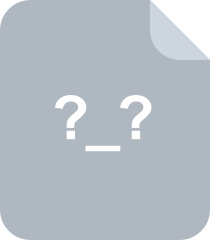
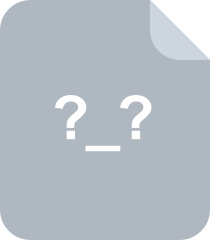
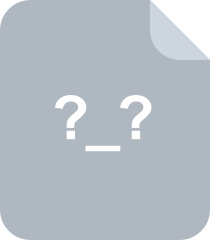
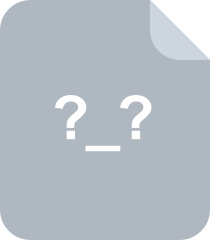
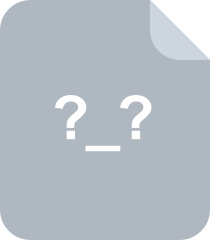
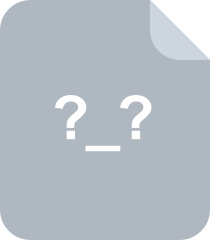
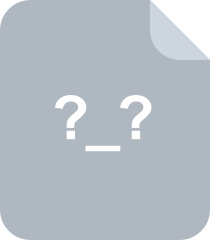
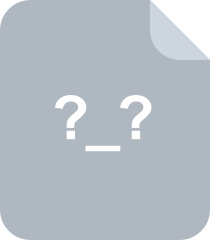
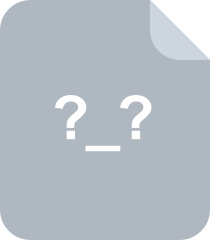
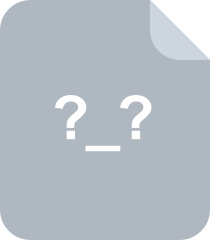
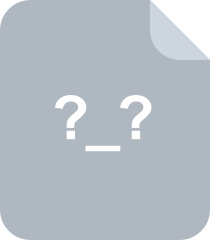
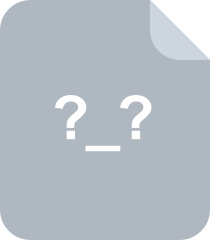
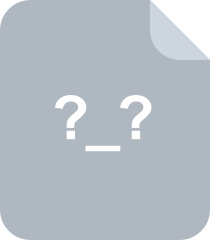
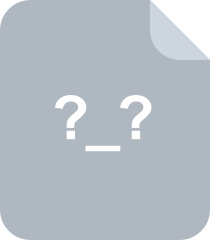
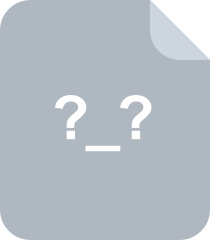
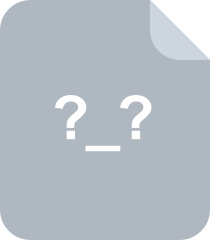
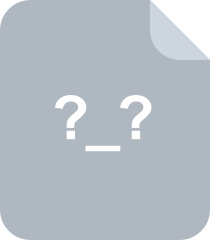
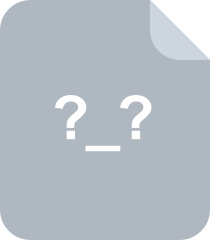
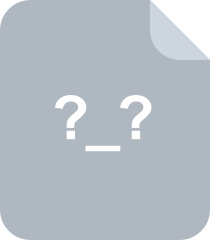
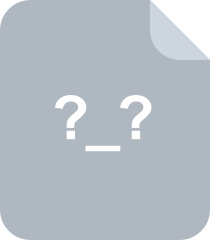
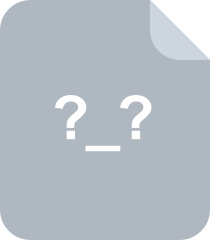
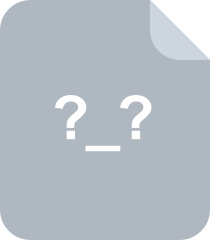
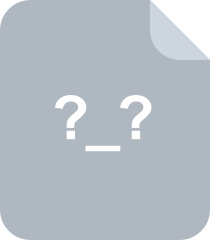
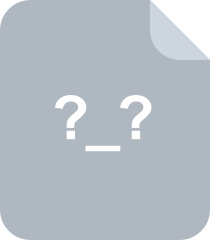
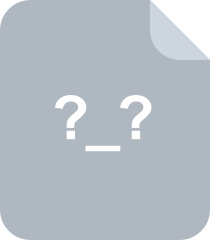
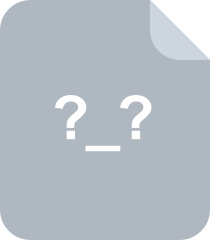
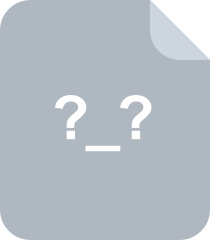
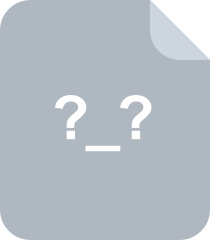
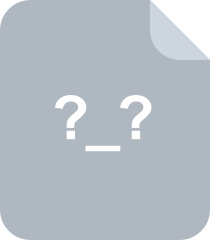
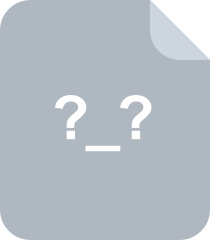
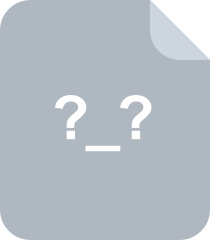
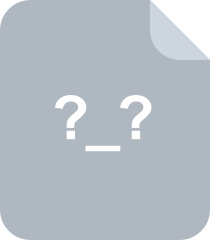
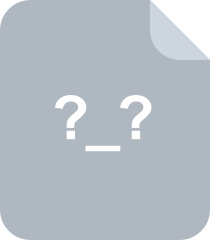
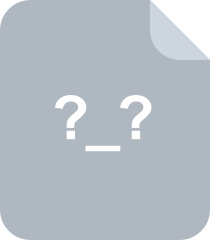
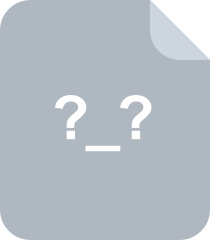
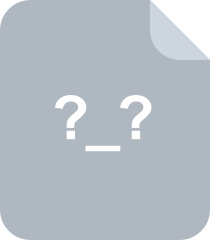
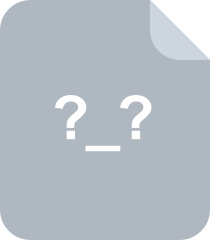
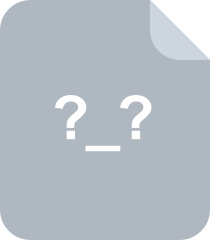
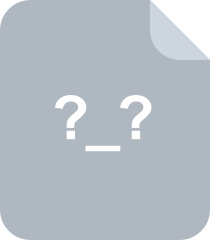
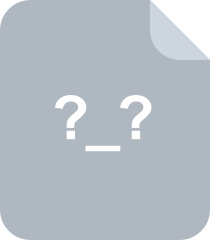
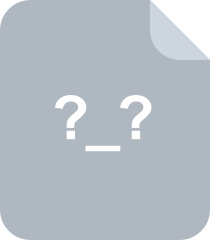
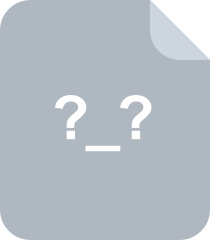
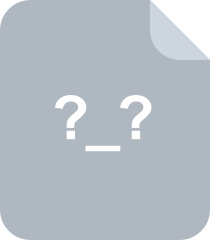
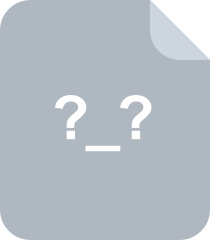
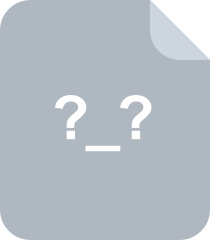
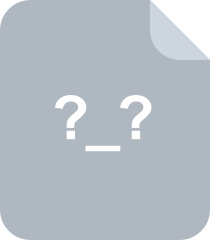
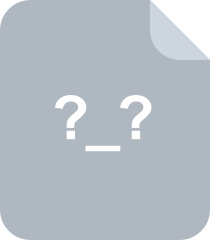
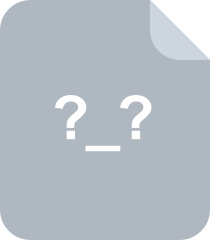
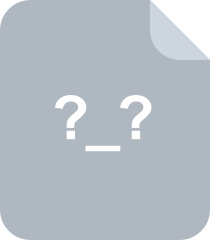
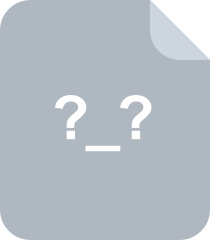
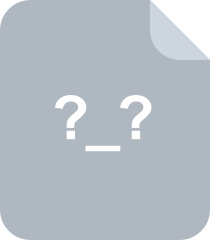
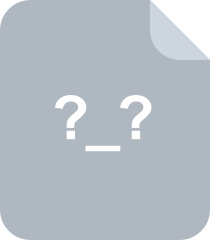
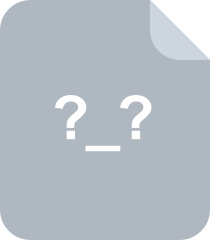
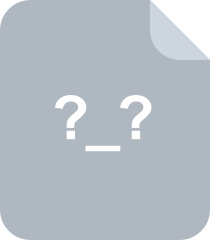
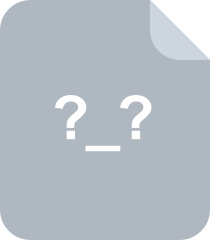
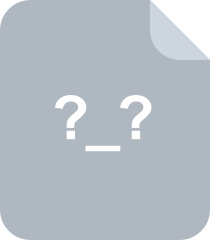
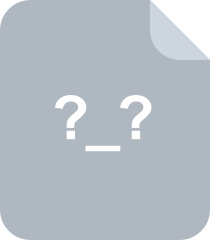
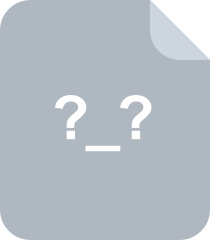
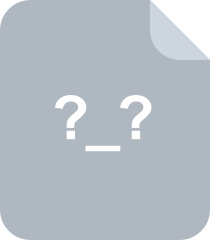
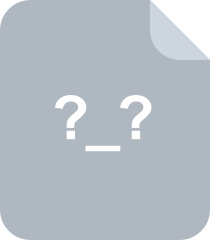
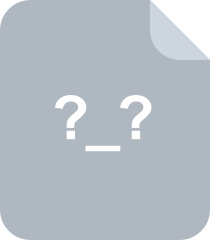
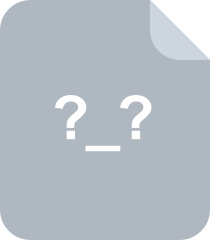
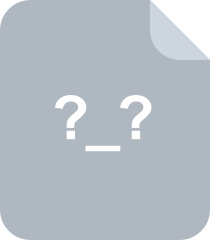
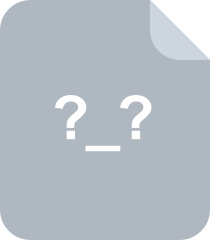
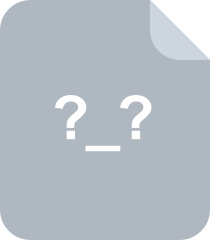
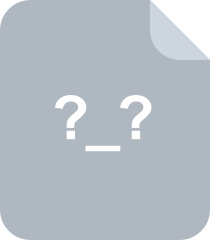
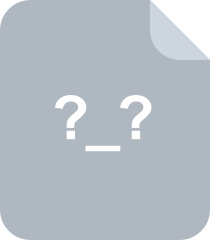
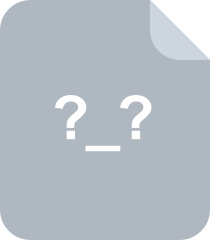
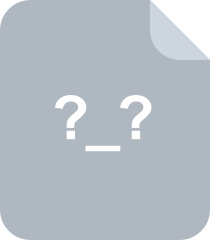
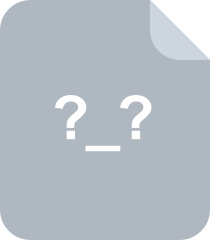
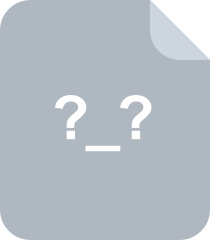
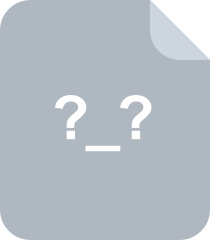
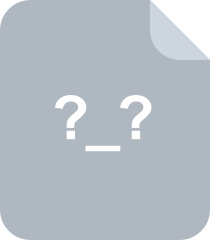
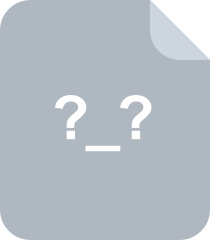
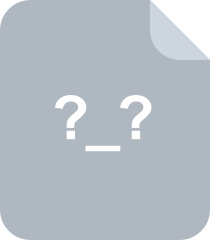
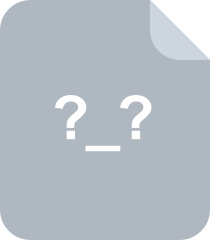
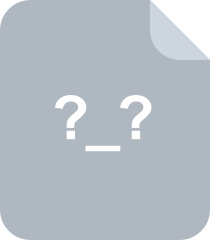
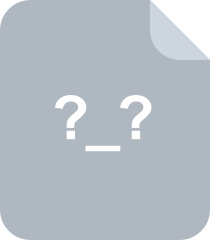
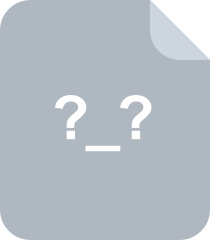
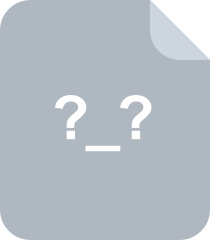
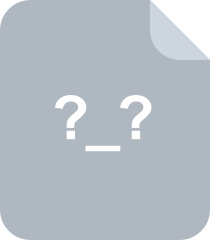
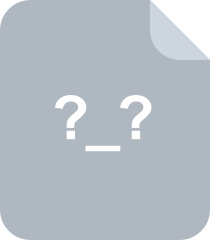
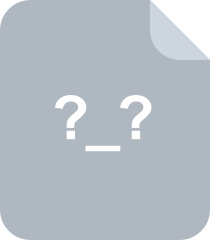
共 169 条
- 1
- 2
资源评论
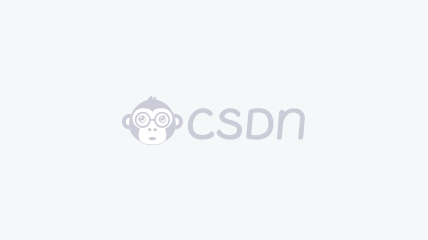

十水雨木
- 粉丝: 1
- 资源: 7
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

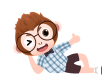
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


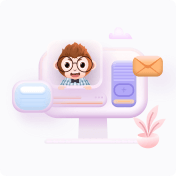
安全验证
文档复制为VIP权益,开通VIP直接复制
