package com.controller;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.text.SimpleDateFormat;
import java.util.*;
import javax.servlet.http.HttpServletRequest;
import com.alibaba.fastjson.JSON;
import com.utils.StringUtil;
import org.apache.commons.lang3.StringUtils;
import org.json.JSONObject;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.util.ResourceUtils;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
import com.annotation.IgnoreAuth;
import com.baidu.aip.face.AipFace;
import com.baidu.aip.face.MatchRequest;
import com.baidu.aip.util.Base64Util;
import com.baomidou.mybatisplus.mapper.EntityWrapper;
import com.baomidou.mybatisplus.mapper.Wrapper;
import com.entity.ConfigEntity;
import com.service.CommonService;
import com.service.ConfigService;
import com.utils.BaiduUtil;
import com.utils.FileUtil;
import com.utils.R;
/**
* 通用接口
*/
@RestController
public class CommonController{
private static final Logger logger = LoggerFactory.getLogger(CommonController.class);
@Autowired
private CommonService commonService;
@Autowired
private ConfigService configService;
private static AipFace client = null;
private static String BAIDU_DITU_AK = null;
@RequestMapping("/location")
public R location(String lng,String lat) {
if(BAIDU_DITU_AK==null) {
BAIDU_DITU_AK = configService.selectOne(new EntityWrapper<ConfigEntity>().eq("name", "baidu_ditu_ak")).getValue();
if(BAIDU_DITU_AK==null) {
return R.error("请在配置管理中正确配置baidu_ditu_ak");
}
}
Map<String, String> map = BaiduUtil.getCityByLonLat(BAIDU_DITU_AK, lng, lat);
return R.ok().put("data", map);
}
/**
* 人脸比对
*
* @param face1 人脸1
* @param face2 人脸2
* @return
*/
@RequestMapping("/matchFace")
public R matchFace(String face1, String face2, HttpServletRequest request) {
if(client==null) {
/*String AppID = configService.selectOne(new EntityWrapper<ConfigEntity>().eq("name", "AppID")).getValue();*/
String APIKey = configService.selectOne(new EntityWrapper<ConfigEntity>().eq("name", "APIKey")).getValue();
String SecretKey = configService.selectOne(new EntityWrapper<ConfigEntity>().eq("name", "SecretKey")).getValue();
String token = BaiduUtil.getAuth(APIKey, SecretKey);
if(token==null) {
return R.error("请在配置管理中正确配置APIKey和SecretKey");
}
client = new AipFace(null, APIKey, SecretKey);
client.setConnectionTimeoutInMillis(2000);
client.setSocketTimeoutInMillis(60000);
}
JSONObject res = null;
try {
File file1 = new File(request.getSession().getServletContext().getRealPath("/upload")+"/"+face1);
File file2 = new File(request.getSession().getServletContext().getRealPath("/upload")+"/"+face2);
String img1 = Base64Util.encode(FileUtil.FileToByte(file1));
String img2 = Base64Util.encode(FileUtil.FileToByte(file2));
MatchRequest req1 = new MatchRequest(img1, "BASE64");
MatchRequest req2 = new MatchRequest(img2, "BASE64");
ArrayList<MatchRequest> requests = new ArrayList<MatchRequest>();
requests.add(req1);
requests.add(req2);
res = client.match(requests);
System.out.println(res.get("result"));
} catch (FileNotFoundException e) {
e.printStackTrace();
return R.error("文件不存在");
} catch (IOException e) {
e.printStackTrace();
}
return R.ok().put("data", com.alibaba.fastjson.JSONObject.parse(res.get("result").toString()));
}
/**
* 获取table表中的column列表(联动接口)
* @return
*/
@RequestMapping("/option/{tableName}/{columnName}")
@IgnoreAuth
public R getOption(@PathVariable("tableName") String tableName, @PathVariable("columnName") String columnName,String level,String parent) {
Map<String, Object> params = new HashMap<String, Object>();
params.put("table", tableName);
params.put("column", columnName);
if(StringUtils.isNotBlank(level)) {
params.put("level", level);
}
if(StringUtils.isNotBlank(parent)) {
params.put("parent", parent);
}
List<String> data = commonService.getOption(params);
return R.ok().put("data", data);
}
/**
* 根据table中的column获取单条记录
* @return
*/
@RequestMapping("/follow/{tableName}/{columnName}")
@IgnoreAuth
public R getFollowByOption(@PathVariable("tableName") String tableName, @PathVariable("columnName") String columnName, @RequestParam String columnValue) {
Map<String, Object> params = new HashMap<String, Object>();
params.put("table", tableName);
params.put("column", columnName);
params.put("columnValue", columnValue);
Map<String, Object> result = commonService.getFollowByOption(params);
return R.ok().put("data", result);
}
/**
* 修改table表的sfsh状态
* @param map
* @return
*/
@RequestMapping("/sh/{tableName}")
public R sh(@PathVariable("tableName") String tableName, @RequestBody Map<String, Object> map) {
map.put("table", tableName);
commonService.sh(map);
return R.ok();
}
/**
* 获取需要提醒的记录数
* @param tableName
* @param columnName
* @param type 1:数字 2:日期
* @param map
* @return
*/
@RequestMapping("/remind/{tableName}/{columnName}/{type}")
@IgnoreAuth
public R remindCount(@PathVariable("tableName") String tableName, @PathVariable("columnName") String columnName,
@PathVariable("type") String type,@RequestParam Map<String, Object> map) {
map.put("table", tableName);
map.put("column", columnName);
map.put("type", type);
if(type.equals("2")) {
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd");
Calendar c = Calendar.getInstance();
Date remindStartDate = null;
Date remindEndDate = null;
if(map.get("remindstart")!=null) {
Integer remindStart = Integer.parseInt(map.get("remindstart").toString());
c.setTime(new Date());
c.add(Calendar.DAY_OF_MONTH,remindStart);
remindStartDate = c.getTime();
map.put("remindstart", sdf.format(remindStartDate));
}
if(map.get("remindend")!=null) {
Integer remindEnd = Integer.parseInt(map.get("remindend").toString());
c.setTime(new Date());
c.add(Calendar.DAY_OF_MONTH,remindEnd);
remindEndDate = c.getTime();
map.put("remindend", sdf.format(remindEndDate));
}
}
int count = commonService.remindCount(map);
return R.ok().put("count", count);
}
/**
* 圖表统计
*/
@IgnoreAuth
@RequestMapping("/group/{tableName}")
public R group1(@PathVariable("tableName") String tableName, @RequestParam Map<String,Object> params) {
params.put("table1", tableName);
List<Map<String, Object>> result = commonService.chartBoth(params);
return R.ok().put("data", result);
}
/**
* 单列求和
*/
@RequestMapping("/cal/{tableName}/{columnName}")
@IgnoreAuth
public R cal(@PathVariable("tableName") String tableName, @PathVariable("columnName") String columnName) {
Map<String, Object> params = new HashMap<String, Object>();
params.put("table", tableName);
params.put("column", columnName);
Map<String, Object> result = commonService.selectCal(params);
return R.ok().put("data", result);
}
/**
* 分组统计
*/
@RequestMapping("/group/{tableName}/{columnName}")
@IgnoreAuth
public R group(@PathVariable("tableName") String tableName, @PathVariable("columnName") String columnName) {
Map<String, Object> params = new HashMap<String, Object>();
params.put("table", tableN
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
传统办法管理信息首先需要花费的时间比较多,其次数据出错率比较高,而且对错误的数据进行更改也比较困难,最后,检索数据费事费力。因此,在计算机上安装养老院管理系统软件来发挥其高效地信息处理的作用,可以规范信息管理流程,让管理工作可以系统化和程序化,同时,养老院管理系统的有效运用可以帮助管理人员准确快速地处理信息。 养老院管理系统在对开发工具的选择上也很慎重,为了便于开发实现,选择的开发工具为Eclipse,选择的数据库工具为Mysql。以此搭建开发环境实现养老院管理系统的功能。其中管理员管理用户,新闻公告。 养老院管理系统是一款运用软件开发技术设计实现的应用系统,在信息处理上可以达到快速的目的,不管是针对数据添加,数据维护和统计,以及数据查询等处理要求,养老院管理系统都可以轻松应对。 关键词:养老院管理系统;SpringBoot框架,系统分析,数据库设计
资源推荐
资源详情
资源评论
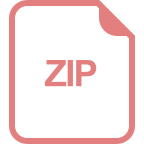
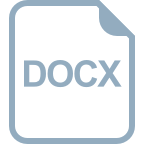
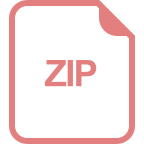
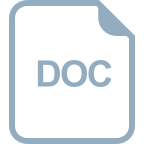
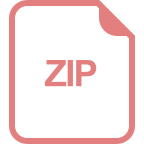
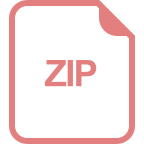
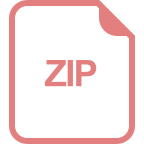
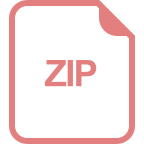
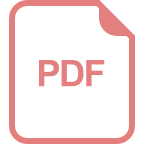
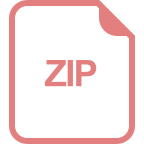
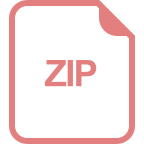
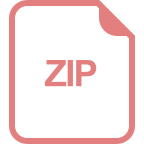
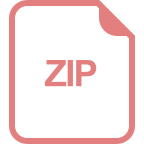
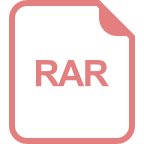
收起资源包目录

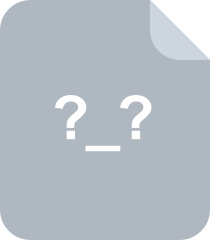
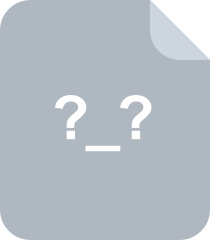
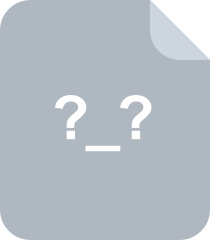
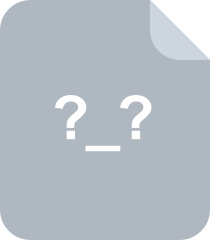
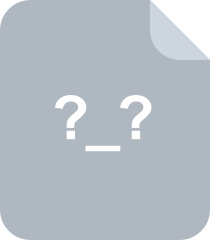
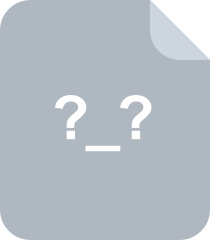
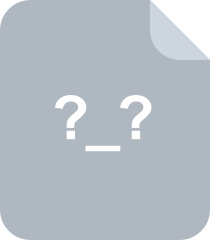
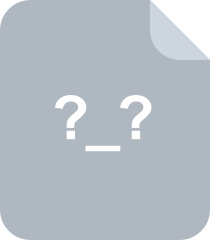
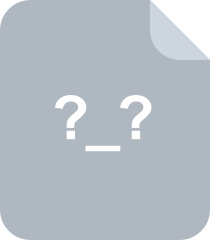
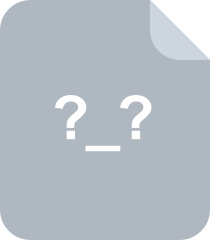
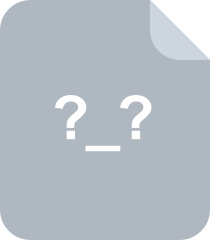
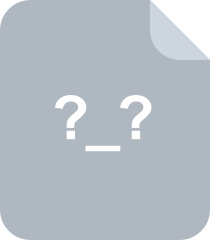
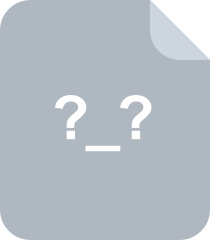
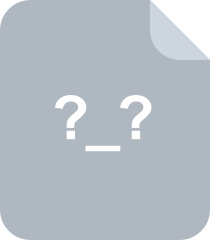
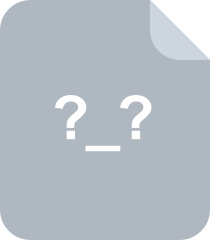
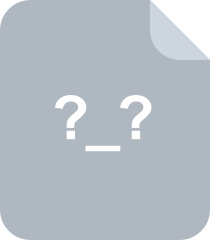
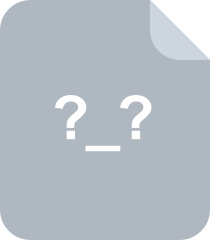
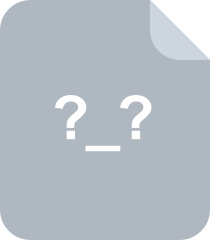
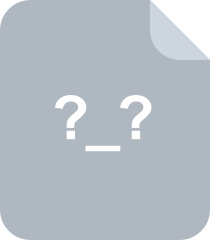
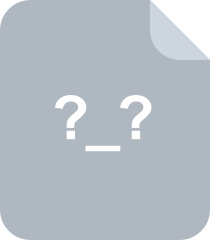
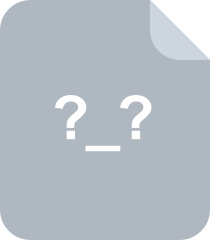
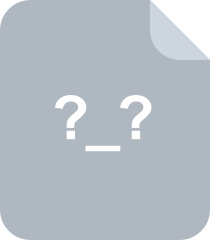
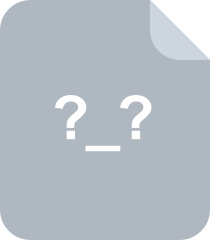
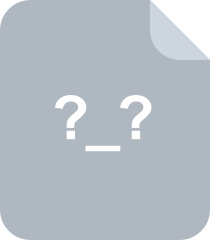
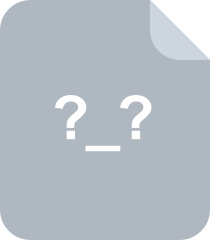
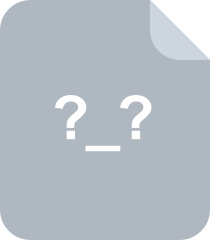
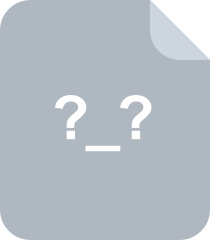
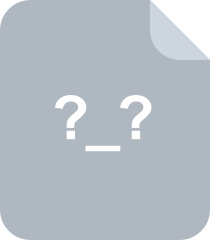
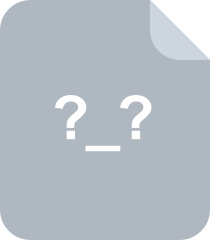
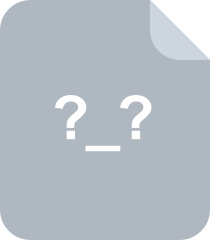
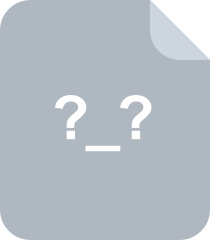
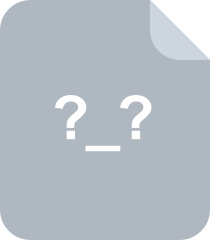
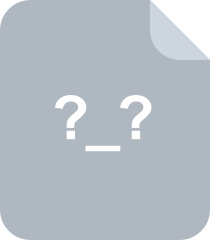
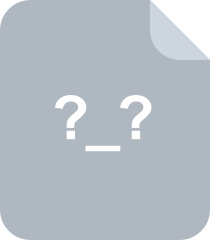
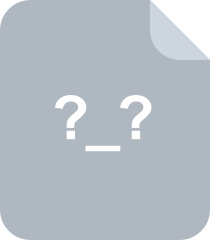
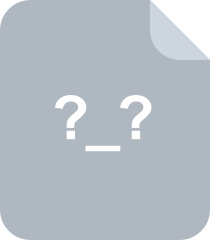
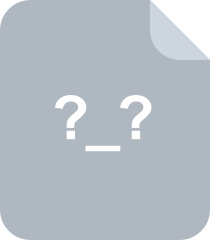
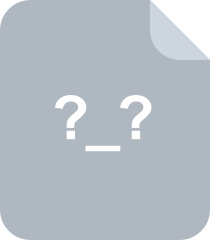
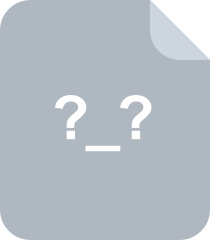
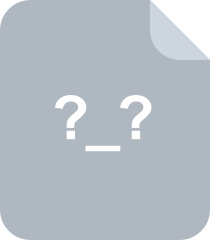
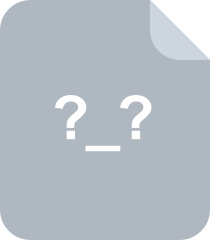
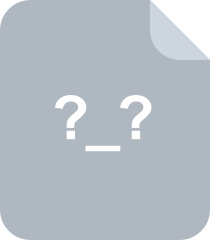
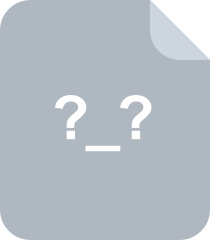
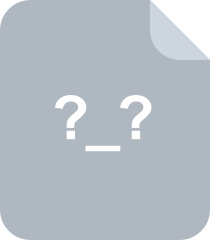
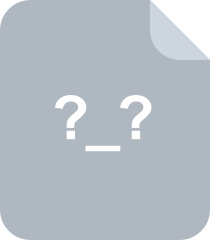
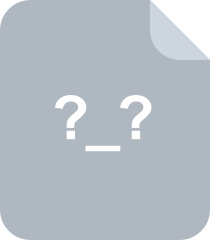
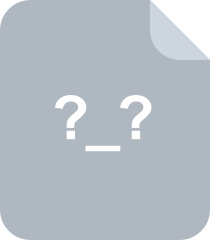
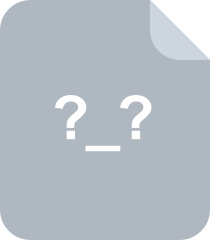
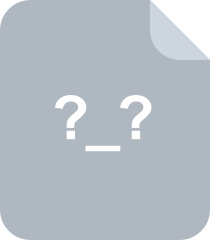
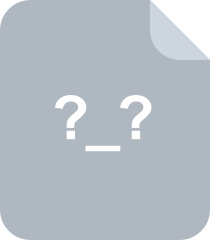
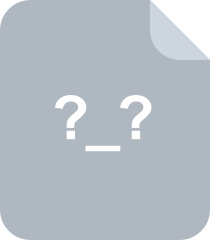
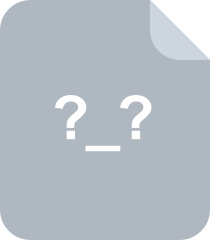
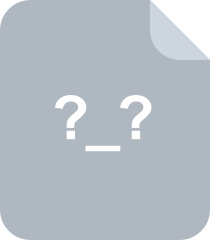
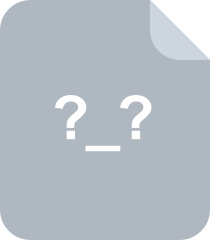
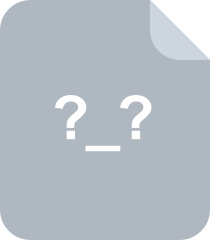
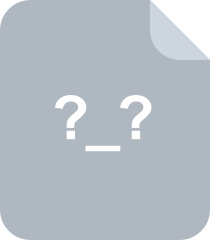
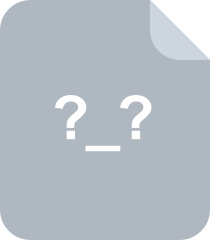
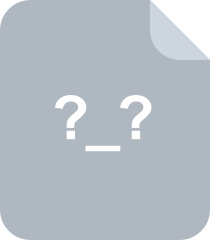
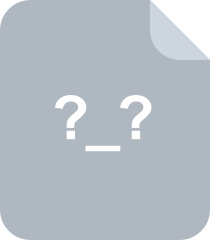
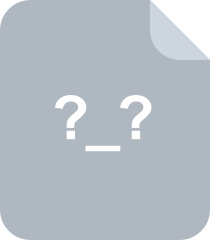
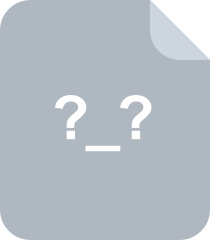
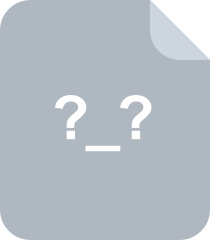
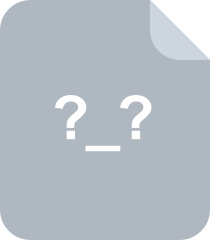
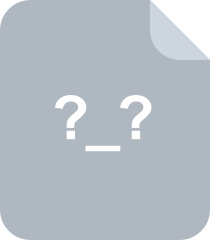
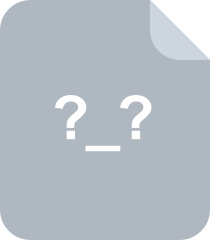
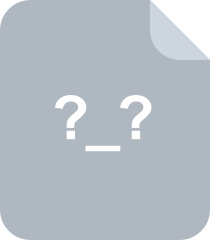
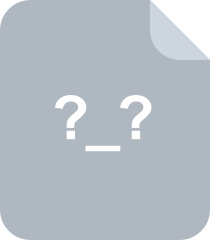
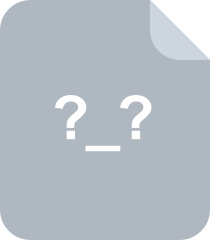
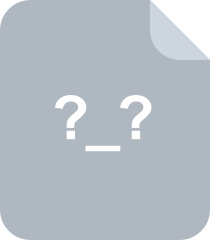
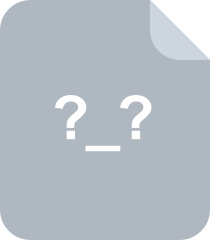
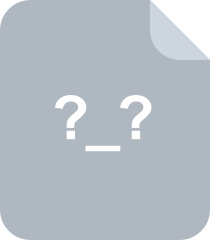
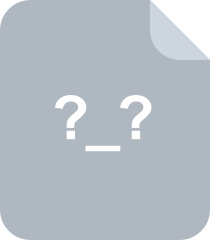
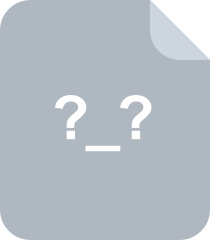
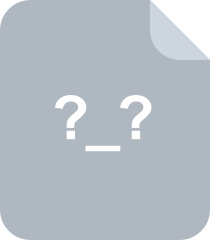
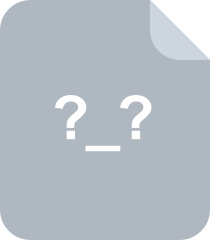
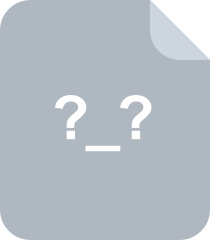
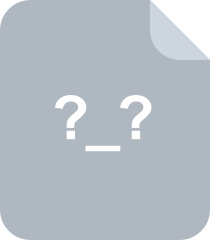
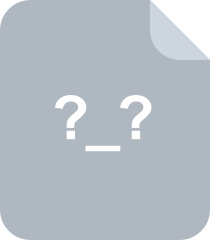
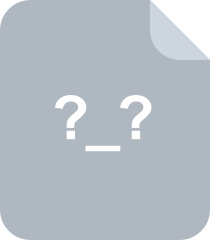
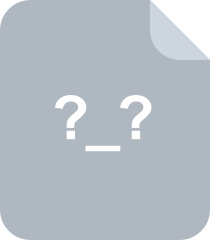
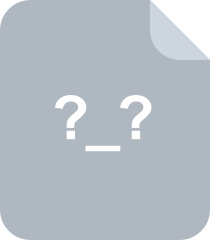
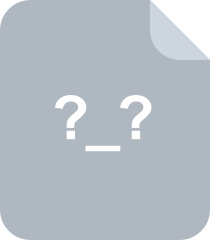
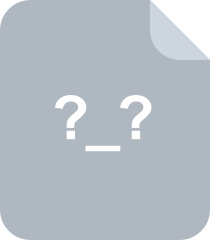
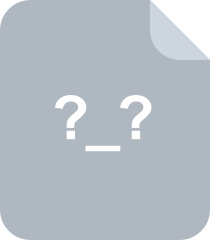
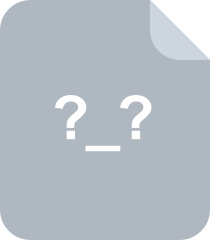
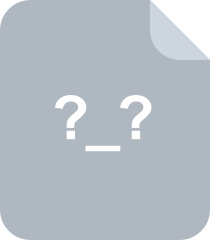
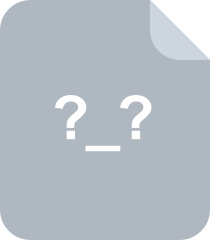
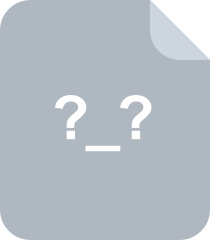
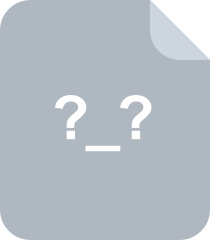
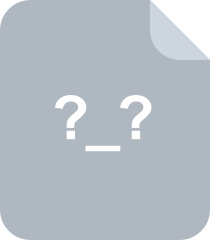
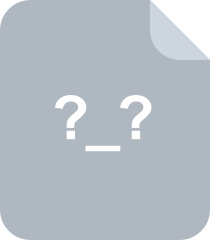
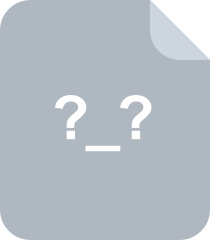
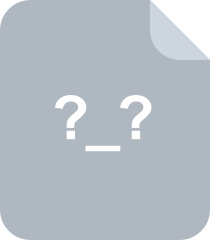
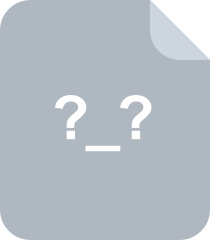
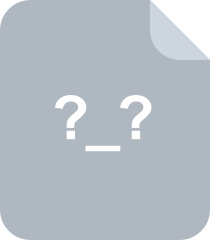
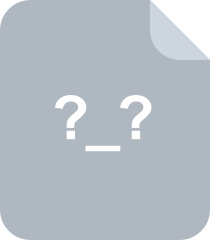
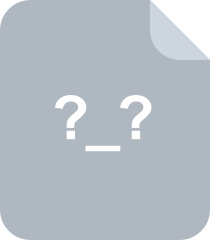
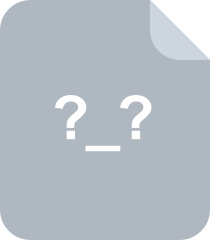
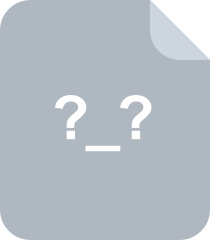
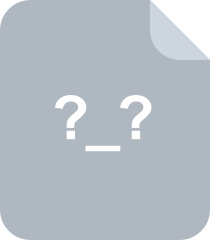
共 1183 条
- 1
- 2
- 3
- 4
- 5
- 6
- 12
资源评论
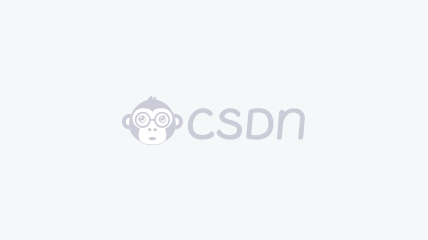

.让你三行代码.
- 粉丝: 154
- 资源: 4
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

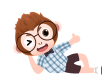
最新资源
- FAQ-76677495-280824-2032-854538.pdf
- Ubuntu系统硬盘扩容及挂载新硬盘,附件为操作说明和分区工具
- statically compensated voltage model
- STM32智能小车控制系统.docx
- STM32智能小车控制系统.docx
- 安装VMwaretools及解决灰色问题
- MobaXterm、WindTerm、xshell、finalshell、soureCRT快捷指令工具cxtool-4.0.0
- 点云分割-基于Ransac随机采样算法实现的地面点云分割-附项目源码+流程教程-优质项目实战.zip
- 多台电脑共用键鼠,多台电脑共用键盘和鼠标
- 点云分割-基于Pytorch实现的Point-Transformer点云分割算法-附项目源码-优质项目实战.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


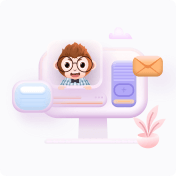
安全验证
文档复制为VIP权益,开通VIP直接复制
