package view.util;
import java.util.ArrayList;
import java.util.List;
import javax.faces.model.SelectItem;
import oracle.adf.model.BindingContext;
import oracle.adf.model.binding.DCBindingContainer;
import oracle.adf.model.binding.DCIteratorBinding;
import oracle.adf.model.binding.DCParameter;
import oracle.adf.share.logging.ADFLogger;
import oracle.binding.AttributeBinding;
import oracle.binding.BindingContainer;
import oracle.binding.ControlBinding;
import oracle.binding.OperationBinding;
import oracle.jbo.ApplicationModule;
import oracle.jbo.Key;
import oracle.jbo.Row;
import oracle.jbo.uicli.binding.JUCtrlValueBinding;
/**
* A series of convenience functions for dealing with ADF Bindings.
* Note: Updated for JDeveloper 11
*
* @author Duncan Mills
* @author Steve Muench
*
* $Id: ADFUtils.java 2513 2007-09-20 20:39:13Z ralsmith $.
*/
public class ADFUtils {
public static final ADFLogger LOGGER = ADFLogger.createADFLogger(ADFUtils.class);
/**
* Get application module for an application module data control by name.
* @param name application module data control name
* @return ApplicationModule
*/
public static ApplicationModule getApplicationModuleForDataControl(String name) {
return (ApplicationModule)JSFUtils.resolveExpression("#{data." + name +
".dataProvider}");
}
/**
* A convenience method for getting the value of a bound attribute in the
* current page context programatically.
* @param attributeName of the bound value in the pageDef
* @return value of the attribute
*/
public static Object getBoundAttributeValue(String attributeName) {
return findControlBinding(attributeName).getInputValue();
}
/**
* A convenience method for setting the value of a bound attribute in the
* context of the current page.
* @param attributeName of the bound value in the pageDef
* @param value to set
*/
public static void setBoundAttributeValue(String attributeName,
Object value) {
findControlBinding(attributeName).setInputValue(value);
}
/**
* Returns the evaluated value of a pageDef parameter.
* @param pageDefName reference to the page definition file of the page with the parameter
* @param parameterName name of the pagedef parameter
* @return evaluated value of the parameter as a String
*/
public static Object getPageDefParameterValue(String pageDefName,
String parameterName) {
BindingContainer bindings = findBindingContainer(pageDefName);
DCParameter param =
((DCBindingContainer)bindings).findParameter(parameterName);
return param.getValue();
}
/**
* Convenience method to find a DCControlBinding as an AttributeBinding
* to get able to then call getInputValue() or setInputValue() on it.
* @param bindingContainer binding container
* @param attributeName name of the attribute binding.
* @return the control value binding with the name passed in.
*
*/
public static AttributeBinding findControlBinding(BindingContainer bindingContainer,
String attributeName) {
if (attributeName != null) {
if (bindingContainer != null) {
ControlBinding ctrlBinding =
bindingContainer.getControlBinding(attributeName);
if (ctrlBinding instanceof AttributeBinding) {
return (AttributeBinding)ctrlBinding;
}
}
}
return null;
}
/**
* Convenience method to find a DCControlBinding as a JUCtrlValueBinding
* to get able to then call getInputValue() or setInputValue() on it.
* @param attributeName name of the attribute binding.
* @return the control value binding with the name passed in.
*
*/
public static AttributeBinding findControlBinding(String attributeName) {
return findControlBinding(getBindingContainer(), attributeName);
}
/**
* Return the current page's binding container.
* @return the current page's binding container
*/
public static BindingContainer getBindingContainer() {
return (BindingContainer)JSFUtils.resolveExpression("#{bindings}");
}
/**
* Return the Binding Container as a DCBindingContainer.
* @return current binding container as a DCBindingContainer
*/
public static DCBindingContainer getDCBindingContainer() {
return (DCBindingContainer)getBindingContainer();
}
/**
* Get List of ADF Faces SelectItem for an iterator binding.
*
* Uses the value of the 'valueAttrName' attribute as the key for
* the SelectItem key.
*
* @param iteratorName ADF iterator binding name
* @param valueAttrName name of the value attribute to use
* @param displayAttrName name of the attribute from iterator rows to display
* @return ADF Faces SelectItem for an iterator binding
*/
public static List<SelectItem> selectItemsForIterator(String iteratorName,
String valueAttrName,
String displayAttrName) {
return selectItemsForIterator(findIterator(iteratorName),
valueAttrName, displayAttrName);
}
/**
* Get List of ADF Faces SelectItem for an iterator binding with description.
*
* Uses the value of the 'valueAttrName' attribute as the key for
* the SelectItem key.
*
* @param iteratorName ADF iterator binding name
* @param valueAttrName name of the value attribute to use
* @param displayAttrName name of the attribute from iterator rows to display
* @param descriptionAttrName name of the attribute to use for description
* @return ADF Faces SelectItem for an iterator binding with description
*/
public static List<SelectItem> selectItemsForIterator(String iteratorName,
String valueAttrName,
String displayAttrName,
String descriptionAttrName) {
return selectItemsForIterator(findIterator(iteratorName),
valueAttrName, displayAttrName,
descriptionAttrName);
}
/**
* Get List of attribute values for an iterator.
* @param iteratorName ADF iterator binding name
* @param valueAttrName value attribute to use
* @return List of attribute values for an iterator
*/
public static List attributeListForIterator(String iteratorName,
String valueAttrName) {
return attributeListForIterator(findIterator(iteratorName),
valueAttrName);
}
/**
* Get List of Key objects for rows in an iterator.
* @param iteratorName iterabot binding name
* @return List of Key objects for rows
*/
public static List<Key> keyListForIterator(String iteratorName) {
return keyListForIterator(findIterator(iteratorName));
}
/**
* Get List of Key objects for rows in an iterator.
* @param iter iterator binding
* @return List of Key objects for rows
*/
public static List<Key> keyListForIterator(DCIteratorBinding iter) {
List<Key> attributeList = new ArrayList<Key>();
没有合适的资源?快使用搜索试试~ 我知道了~
ADF动态添加Form
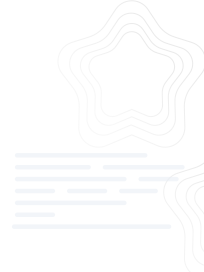
共69个文件
xml:36个
class:7个
java:3个


温馨提示
在ADF的Table中,我们能实现在表格中添加,编辑,删除行的功能,以及一行数据中的级联。然而在多数时候,当一行的列太多,表格中的数据量不多的时候,这种在table中直接添加的方式则显得很不友好,因为要来回的拉动横向的滚动条。那么有什么办法能以表单的方式来添加一行,添加一条数据时添加一个表单,直到有多个表单出现在页面上,当然也包括删除.
资源推荐
资源详情
资源评论
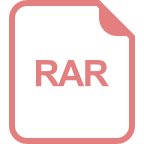
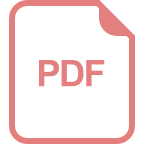
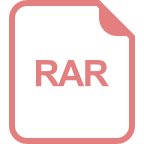
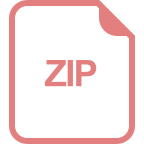
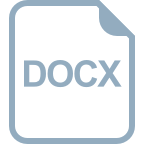
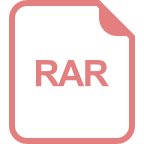
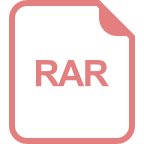
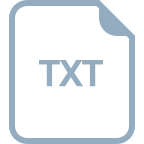
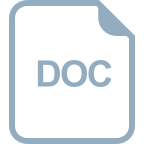
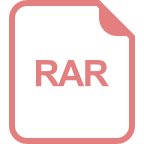
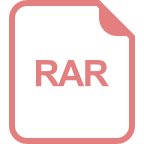
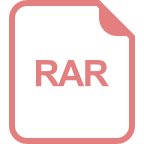
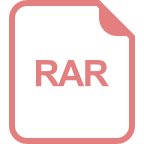
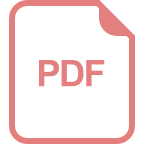
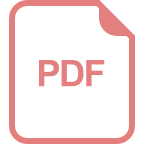
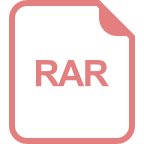
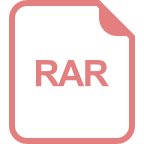
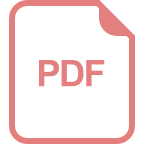
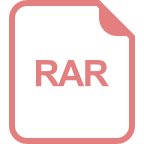
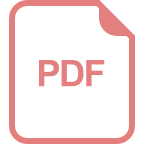
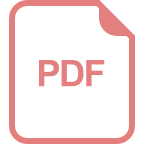
收起资源包目录


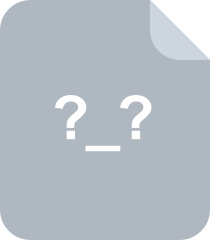


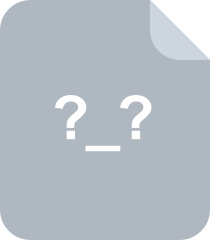
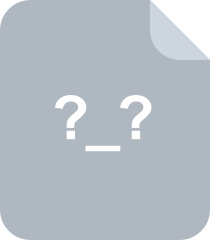
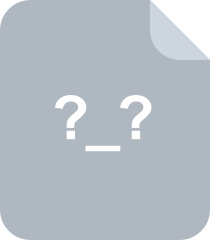



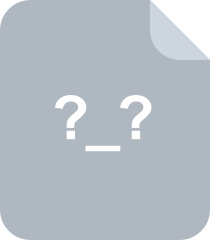


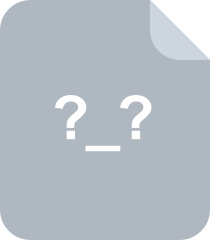
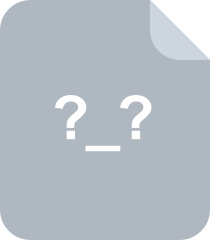
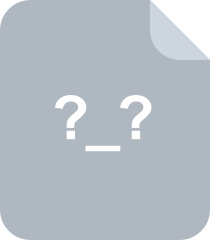
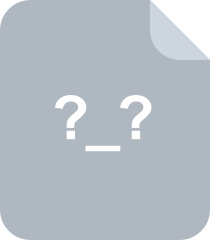

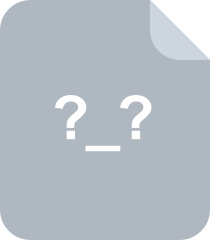

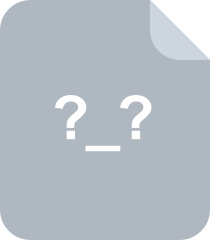


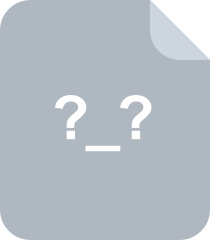
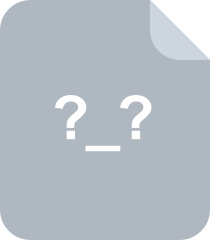

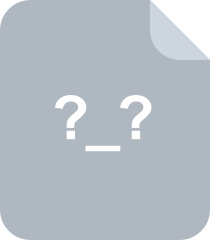
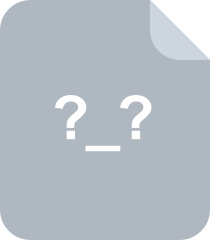
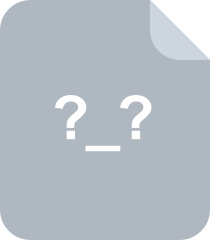
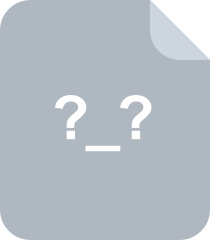


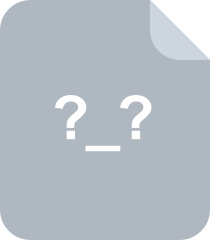
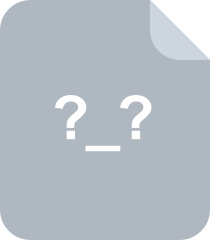



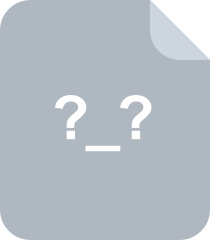
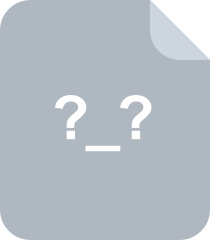
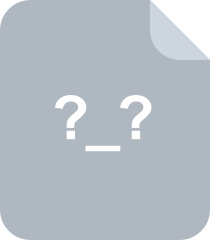
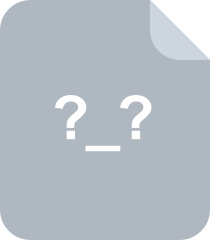

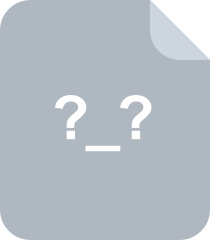

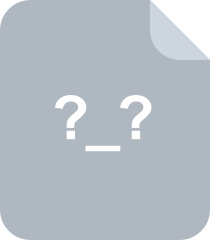


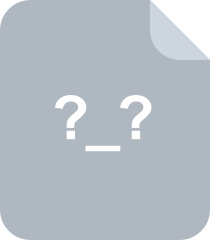
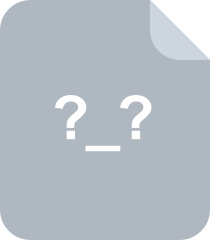

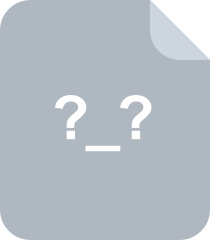
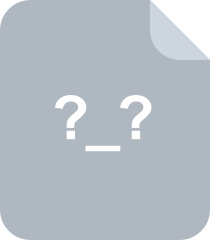
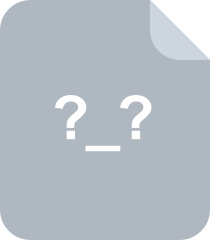



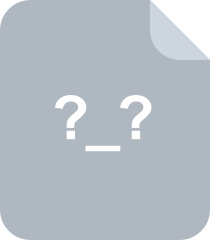
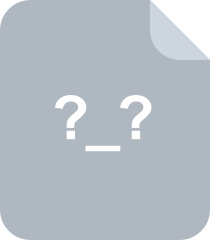
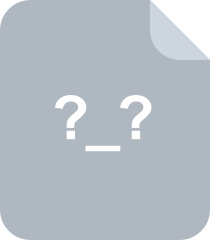

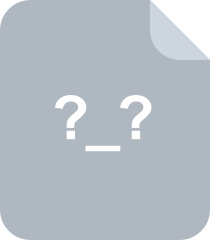




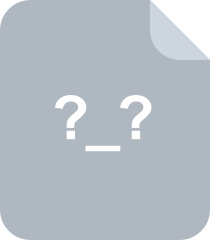
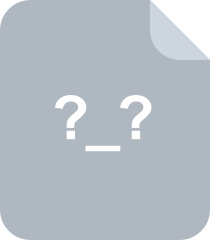
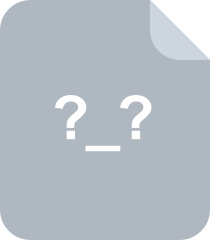
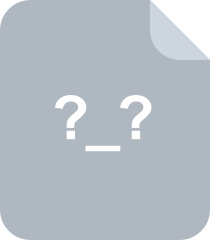
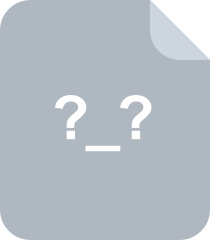
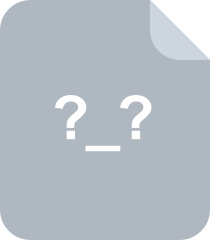



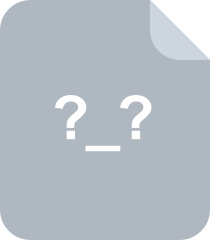

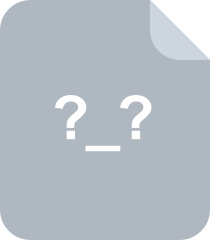
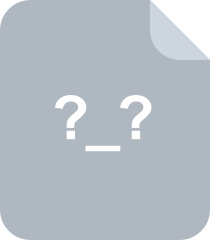

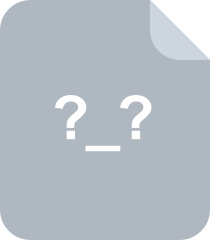
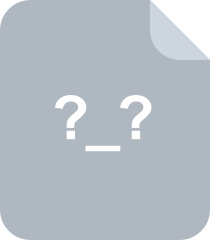
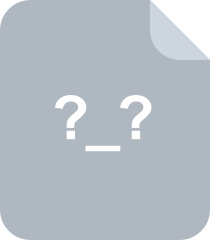

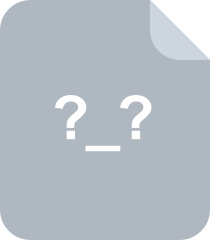
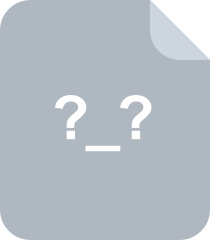

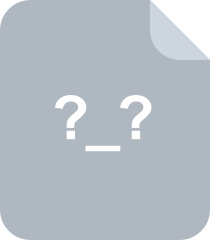
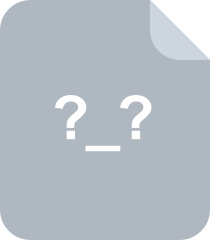


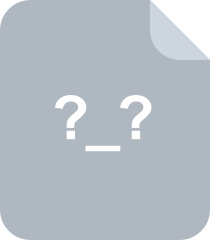
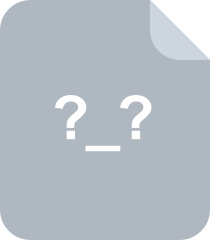

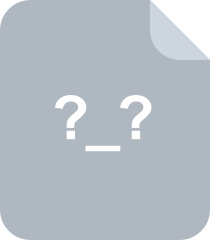
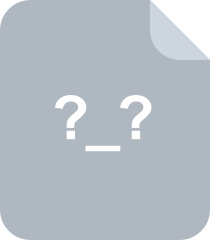

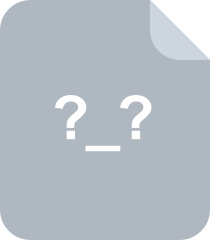

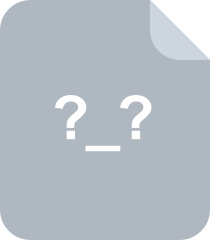
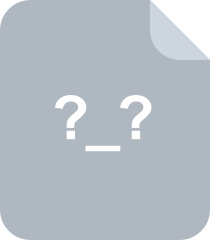
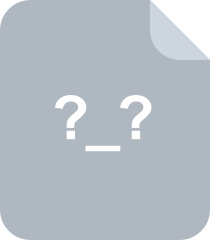


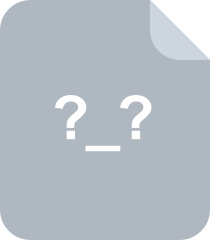



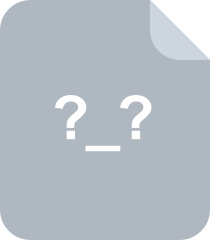

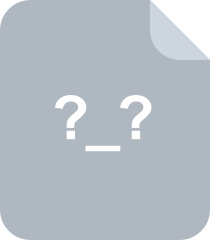
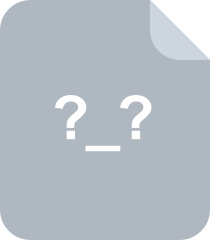



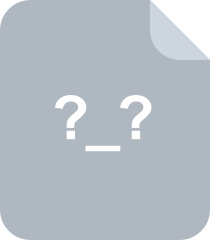
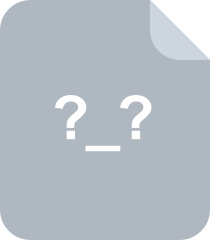

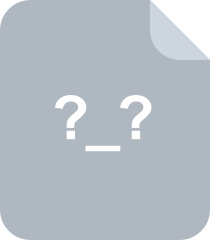

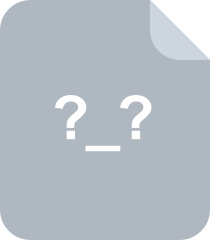
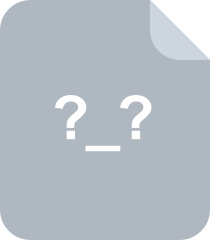


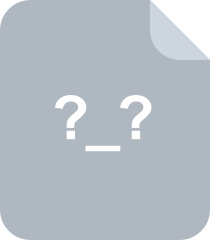
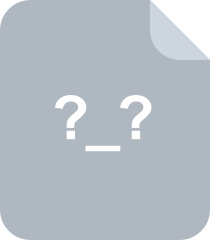
共 69 条
- 1
资源评论
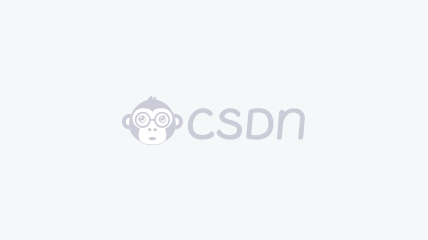
- 爱玩的老周2014-11-07ADF这个内容里面比较有针对性的一项。还可以哦。

小尹
- 粉丝: 96
- 资源: 19
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

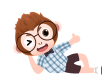
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


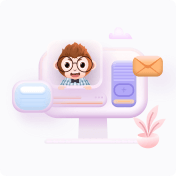
安全验证
文档复制为VIP权益,开通VIP直接复制
