/**
* Copyright (c) 2022-2023, Mybatis-Flex (fuhai999@gmail.com).
* <p>
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
* <p>
* http://www.apache.org/licenses/LICENSE-2.0
* <p>
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.mybatisflex.admin.controller;
import com.mybatisflex.admin.manager.AccountService;
import com.mybatisflex.admin.mapper.AccountMapper;
import com.mybatisflex.admin.model.Account;
import com.mybatisflex.admin.utils.Util;
import com.mybatisflex.core.paginate.Page;
import com.mybatisflex.core.query.QueryWrapper;
import com.mybatisflex.core.row.Db;
import com.mybatisflex.core.row.Row;
import jakarta.annotation.Resource;
import org.springframework.transaction.annotation.Transactional;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import java.util.Date;
import java.util.List;
import static com.mybatisflex.admin.model.table.AccountTableDef.ACCOUNT;
@RestController
@RequestMapping("/slave")
public class AccountSlaveController {
@Resource
private AccountMapper accountMapper;
@Resource
private AccountService accountService;
@GetMapping("/")
List<Account> index() {
return Util.switchDS("slave1", ()-> accountMapper.selectAll());
}
@GetMapping("/all")
List<Account> all() {
return Util.switchDS("slave1", ()-> accountMapper.getAll());
}
@GetMapping("/wrappers")
List<Account> selectList() {
return Util.switchDS("slave1", ()-> {
//构造 QueryWrapper,也支持使用 QueryWrapper.create() 构造,效果相同
QueryWrapper query = new QueryWrapper();
query.where(ACCOUNT.ID.ge(1));
//通过 query 查询数据列表返回
return accountMapper.selectListByQuery(query);
});
}
/**
* db+row 分页查询:每页 10 条数据,查询第 1 页的年龄大于或等于 18 的用户
* @return
*/
@GetMapping("/pages")
Page<Row> getPage(){
return Util.switchDS("slave1", ()-> {
QueryWrapper query=QueryWrapper.create()
.where(ACCOUNT.AGE.ge(18));
Page<Row> rowPage= Db.paginate("tb_account",1,10,query);
return rowPage;
});
}
@GetMapping("/save")
@Transactional(rollbackFor = Exception.class)
public void save() {
Util.switchDS("slave1", ()-> {
Account account2 = new Account();
account2.setUserName("用户slave");
account2.setAge(11);
account2.setBirthday(new Date());
return accountMapper.insert(account2);
});
}
/**
* IService 示例
*/
@GetMapping("/services")
@Transactional(rollbackFor = Exception.class)
public void saveByService() {
Util.switchDS("slave1", ()-> {
Account account2 = new Account();
account2.setUserName("用户");
account2.setAge(1);
account2.setBirthday(new Date());
accountService.saveOne(account2);
return 0;
});
}
/**
* 事务
*/
@GetMapping("/tx")
@Transactional(rollbackFor = Exception.class)
public void saveTx() {
Account account = new Account();
account.setUserName("用户master-tx");
account.setAge(1);
account.setBirthday(new Date());
accountMapper.insert(account);
Util.switchDS("slave1", ()-> {
Account account2 = new Account();
account2.setUserName("用户slave-tx");
account2.setAge(11);
account2.setBirthday(new Date());
accountMapper.insert(account2);
throw new RuntimeException("模拟事务中抛出异常!");
});
}
}
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
### mybatisFlex增强框架应用java源代码 这是一个springboot3+mybatisFlex的案例,内含数据库脚本文件(app.sql) 软件架构说明: springboot3 + jdk17 + mybatis3.0.1 + mybatis-flex1.5.6 关于mybatis-flex: MyBatis-Flex是一个优雅的MyBatis增强框架,它非常轻量、同时拥有极高的性能与灵活性。我们可以使用MyBatis-Flex链接任何数据库,其内置的QueryWrapper帮助我们极大的减少了SQL编写的工作的同时,减少出错的可能性。 源代码说明: 1. 包括表tb_account的数据访问,分别在mapper包下,可在单元测试AccountControllerTest以及AccountControllerTest2测试使用 2. 基于MVC架构实现,mapper数据持久层为数据库访问操作,对应的自定义sql文件mapper.xml,按常规在resources\mapper\下 3. test模块的单元测试,可以用来调试程序。基于jUnit5+mockMvc框架
资源推荐
资源详情
资源评论
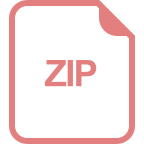
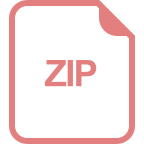
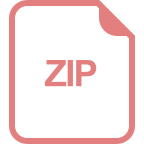
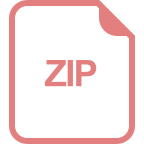
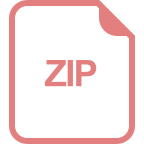
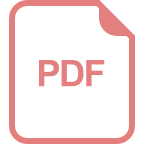
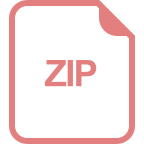
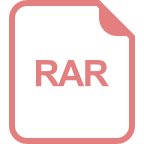
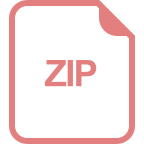
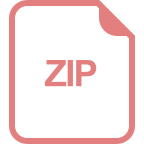
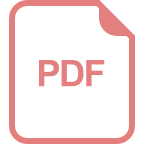
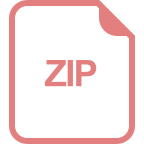
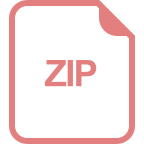
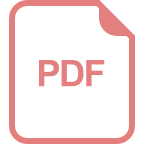
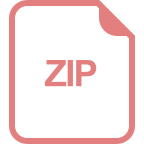
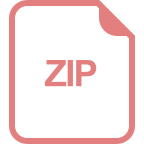
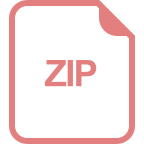
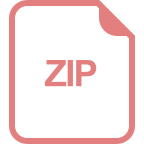
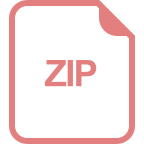
收起资源包目录


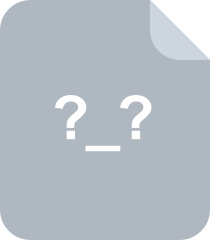







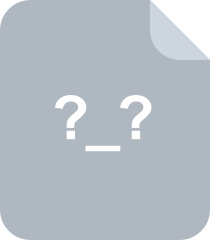
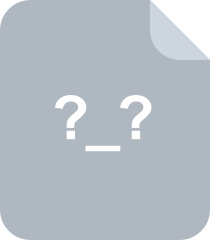



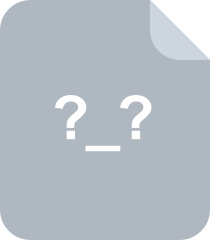
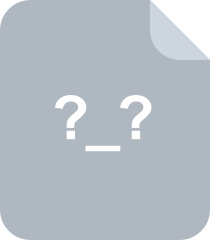
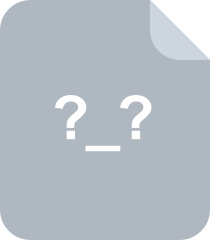


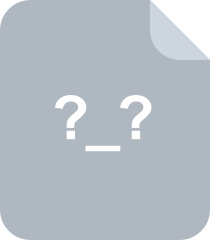




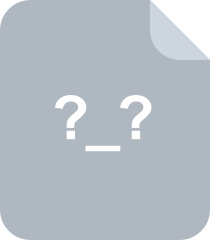

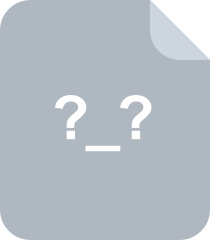

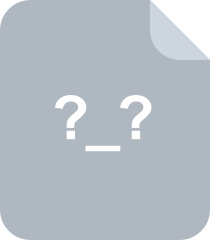
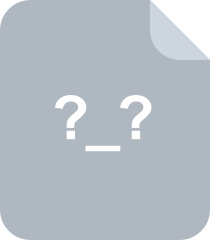

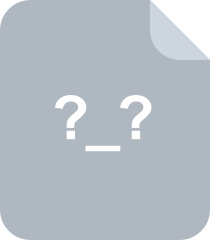


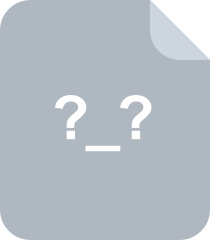
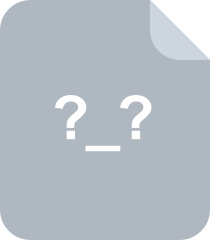

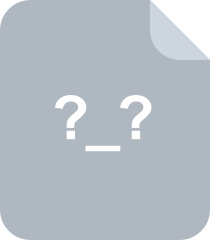

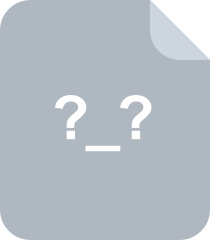

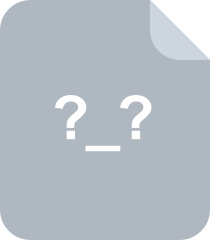
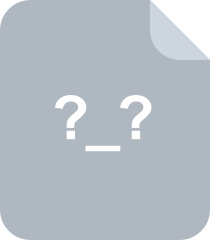
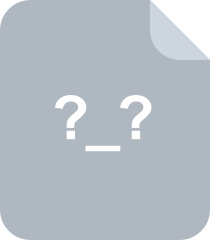
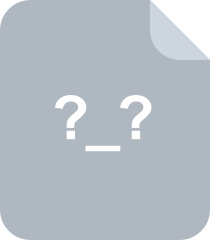
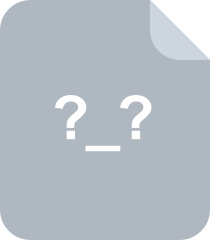
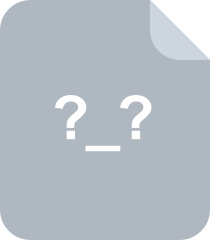
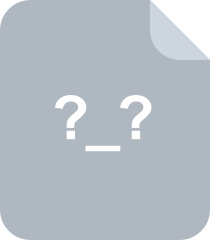
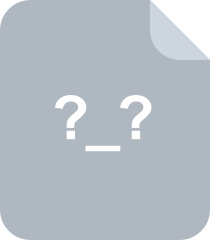



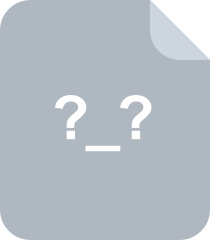
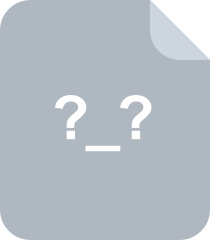






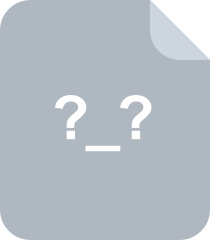
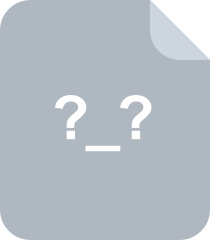


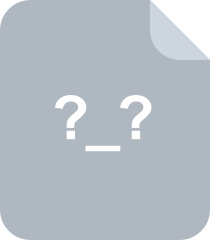




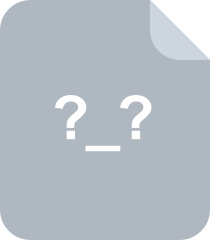
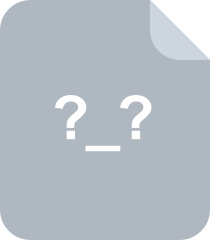

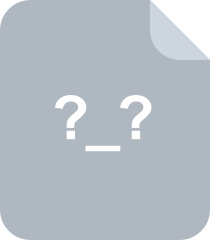
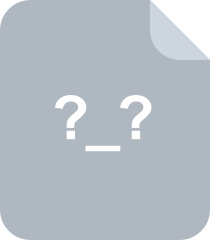

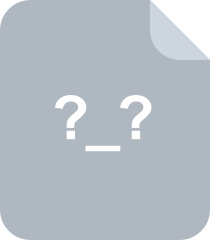

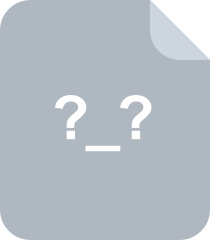

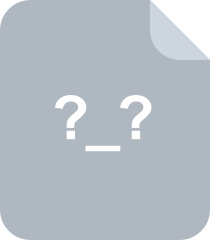

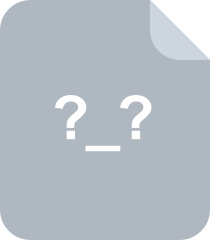

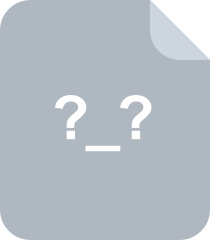

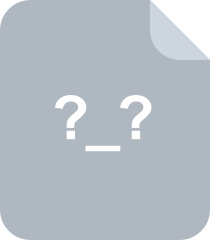





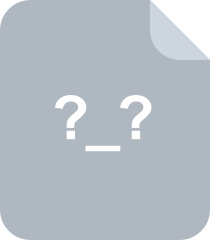
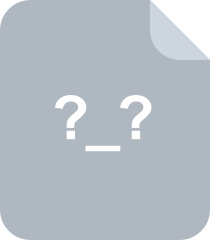




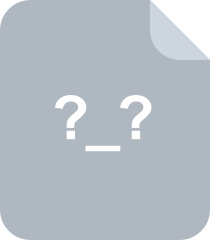
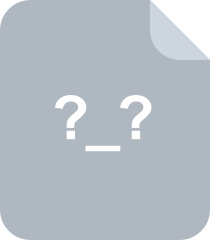


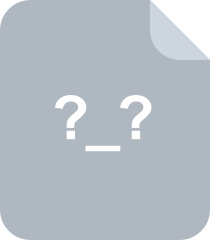
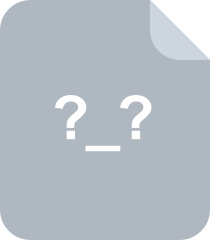

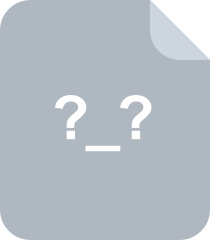


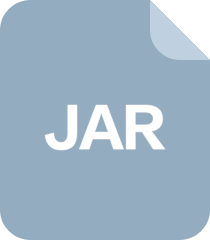







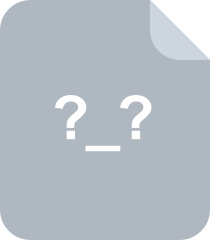
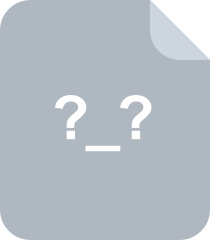
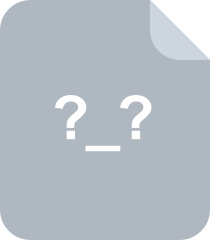
共 50 条
- 1
资源评论
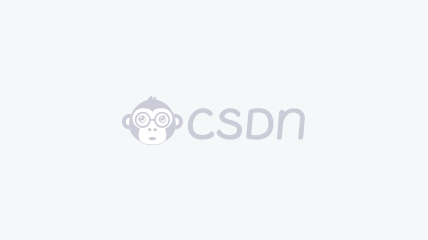

yellow1019
- 粉丝: 49
- 资源: 102
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

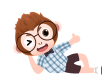
最新资源
- 数据结构上机实验大作业-线性表选题.zip
- 字幕网页文字检测20-YOLO(v5至v11)、COCO、CreateML、Paligemma、TFRecord、VOC数据集合集.rar
- 雪毅云划算试客系统v2.9.7标准版 含购物返利+免费试用+9.9包邮+品牌折扣+推广中心等
- 冒泡排序算法详解及Java与Python实现
- 实时 零代码、全功能、强安全 ORM 库 后端接口和文档零代码,前端(客户端) 定制返回 JSON 的数据和结构
- 混合有源滤波器(HAPF) MATLAB-Simulink仿真 仿真模拟的HAPF补偿前后,系统所含的谐波对比如下图所示
- csi-driver-nfs
- 认识小动物-教案反思.docx
- pdfjs2.5.207和4.9.155
- 2023-04-06-项目笔记 - 第三百五十五阶段 - 4.4.2.353全局变量的作用域-353 -2025.12.22
- OPCClient-UA源码OPC客户端源码(c#开发) 另外有opcserver,opcclient的da,ua版本的见其他链接 本项目为VS2019开发,可用VS其他版本的编辑器打开项目 已应
- 2023-04-06-项目笔记 - 第三百五十五阶段 - 4.4.2.353全局变量的作用域-353 -2025.12.22
- PHP快速排序算法实现与优化
- deploy.yaml
- 家庭用具检测15-YOLO(v8至v11)数据集合集.rar
- RuoYi-Cloud-Plus 微服务通用权限管理系统
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


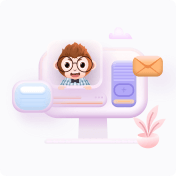
安全验证
文档复制为VIP权益,开通VIP直接复制
