js input 密码只能输入数字或字母
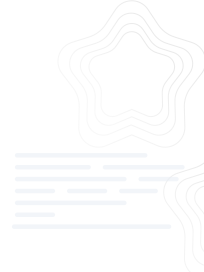

在JavaScript(JS)中,为实现输入框(input)只允许用户输入数字或字母的功能,我们需要对用户的输入进行实时验证。这种需求在很多Web应用中都常见,比如注册、登录页面的密码输入。以下是一个详细的方法来实现这个功能: 1. HTML部分: 创建一个input元素,设置type为"text"或"password",根据需求决定是否显示明文密码。添加一个id以便于在JavaScript中引用,并可以添加placeholder属性提供提示。 ```html <input type="password" id="customInput" placeholder="请输入数字或字母"> ``` 2. JavaScript部分: 接下来,我们需要监听键盘事件,通常选择`onkeydown`、`onkeyup`或`oninput`事件。这里我们使用`oninput`事件,因为它可以捕获所有类型的输入更改,包括粘贴或剪切的文本。 ```javascript document.getElementById('customInput').addEventListener('input', function(e) { validateInput(e); }); ``` 3. 验证函数validateInput: 定义一个函数,用于检查输入值是否只包含字母和数字。这里我们可以使用正则表达式来实现: ```javascript function validateInput(event) { const regex = /^[a-zA-Z0-9]*$/; // 正则表达式,匹配字母和数字 const value = event.target.value; if (!regex.test(value)) { // 如果输入不符合正则,则清除不符合的部分 event.target.value = value.replace(/[^a-zA-Z0-9]/g, ''); } } ``` 在这个函数中,我们首先定义了一个正则表达式`/^[a-zA-Z0-9]*$/`,它会匹配任何由字母或数字组成的字符串。然后,我们获取当前input元素的值,并使用正则表达式的`test`方法来检查该值是否符合规范。如果不符合,我们就使用`replace`方法去掉所有非字母和数字的字符。 4. 优化用户体验: 为了提高用户体验,可以在输入不合法时给予用户反馈,例如通过CSS改变输入框的边框颜色。这可以通过添加额外的CSS类来实现,如下所示: ```javascript function validateInput(event) { // ... if (!regex.test(value)) { event.target.classList.add('invalid-input'); } else { event.target.classList.remove('invalid-input'); } } ``` 并添加相应的CSS样式: ```css .invalid-input { border-color: red; outline: none; /* 防止聚焦时默认红色边框 */ } ``` 5. 源码: 考虑到你提到的"源码"标签,完整示例代码如下: ```html <!DOCTYPE html> <html lang="zh"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <style> .invalid-input { border-color: red; outline: none; } </style> </head> <body> <input type="password" id="customInput" placeholder="请输入数字或字母"> <script> document.getElementById('customInput').addEventListener('input', function(e) { validateInput(e); }); function validateInput(event) { const regex = /^[a-zA-Z0-9]*$/; const value = event.target.value; if (!regex.test(value)) { event.target.value = value.replace(/[^a-zA-Z0-9]/g, ''); event.target.classList.add('invalid-input'); } else { event.target.classList.remove('invalid-input'); } } </script> </body> </html> ``` 以上就是实现“js input 密码只能输入数字或字母”的完整方法,确保了用户在输入时只会看到符合要求的字符。这个功能对于确保数据的正确性和安全性非常重要,特别是在处理敏感信息如密码时。
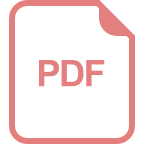
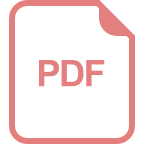
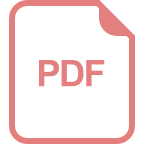
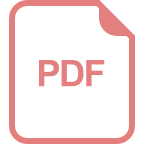
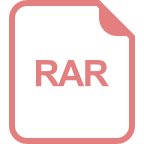
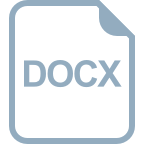
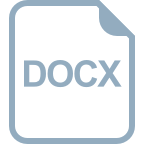
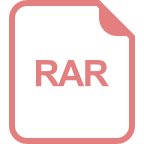
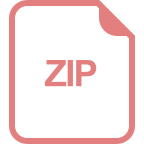
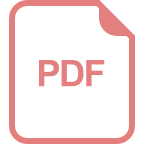
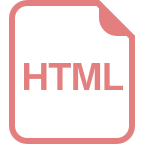

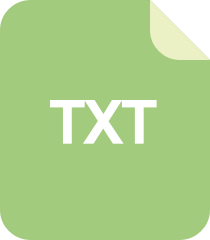
- 1
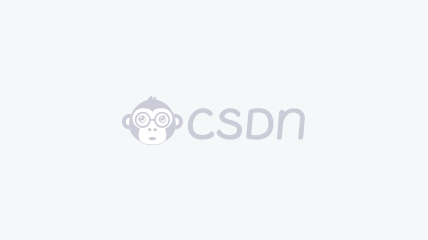

- 粉丝: 1
- 资源: 15
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

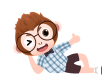
最新资源
- (源码)基于Qt和AVR的FestosMechatronics系统终端.zip
- (源码)基于Java的DVD管理系统.zip
- (源码)基于Java RMI的共享白板系统.zip
- (源码)基于Spring Boot和WebSocket的毕业设计选题系统.zip
- (源码)基于C++的机器人与船舶管理系统.zip
- (源码)基于WPF和Entity Framework Core的智能货架管理系统.zip
- SAP Note 532932 FAQ Valuation logic with active material ledger
- (源码)基于Spring Boot和Redis的秒杀系统.zip
- (源码)基于C#的计算器系统.zip
- (源码)基于ESP32和ThingSpeak的牛舍环境监测系统.zip

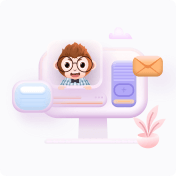
