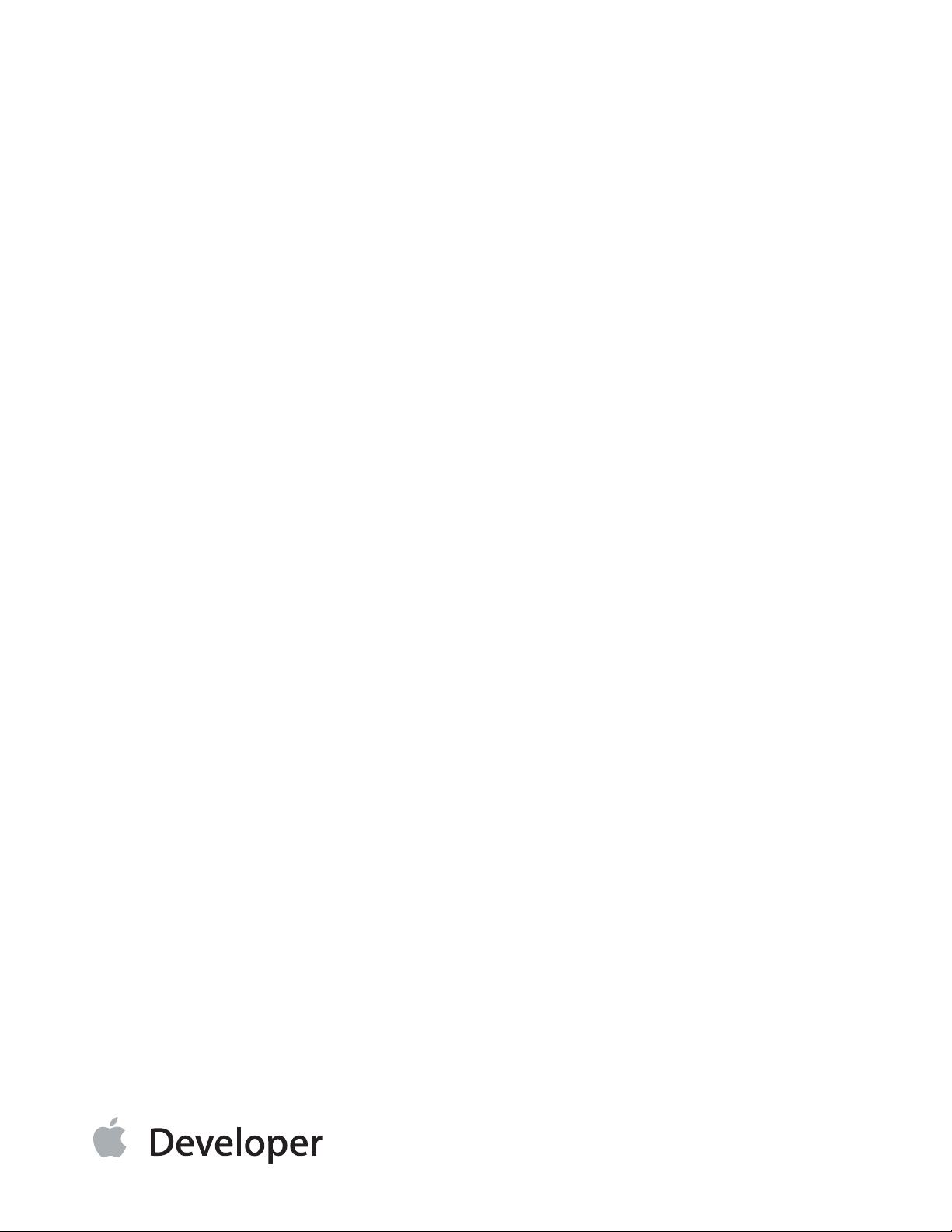
iOS App Programming
Guide
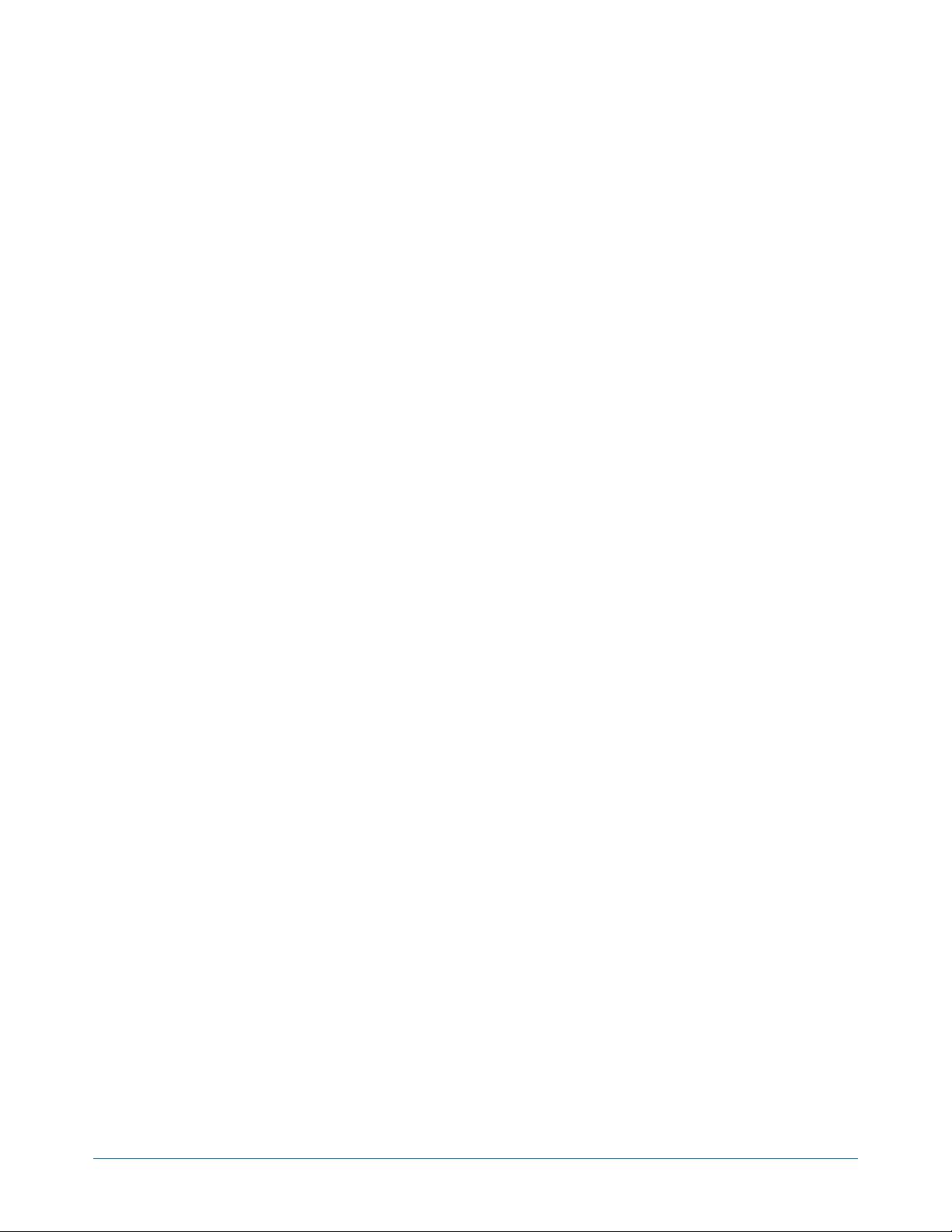
Contents
About iOS App Programming 8
At a Glance 9
Translate Your Initial Idea into an Implementation Plan 9
UIKit Provides the Core of Your App 9
Apps Must Behave Differently in the Foreground and Background 9
iCloud Affects the Design of Your Data Model and UI Layers 10
Apps Require Some Specific Resources 10
Apps Should Restore Their Previous UI State at Launch Time 10
Many App Behaviors Can Be Customized 10
Apps Must Be Tuned for Performance 11
The iOS Environment Affects Many App Behaviors 11
How to Use This Document 11
Prerequisites 11
See Also 12
App Design Basics 13
Doing Your Initial Design 13
Learning the Fundamental iOS Design Patterns and Techniques 14
Translating Your Initial Design into an Action Plan 14
Starting the App Creation Process 15
Core App Objects 18
The Core Objects of Your App 18
The Data Model 21
Defining a Custom Data Model 22
Defining a Structured Data Model Using Core Data 25
Defining a Document-Based Data Model 25
Integrating iCloud Support Into Your App 27
The User Interface 27
Building an Interface Using UIKit Views 28
Building an Interface Using Views and OpenGL ES 30
The App Bundle 31
App States and Multitasking 34
2013-01-28 | © 2013 Apple Inc. All Rights Reserved.
2
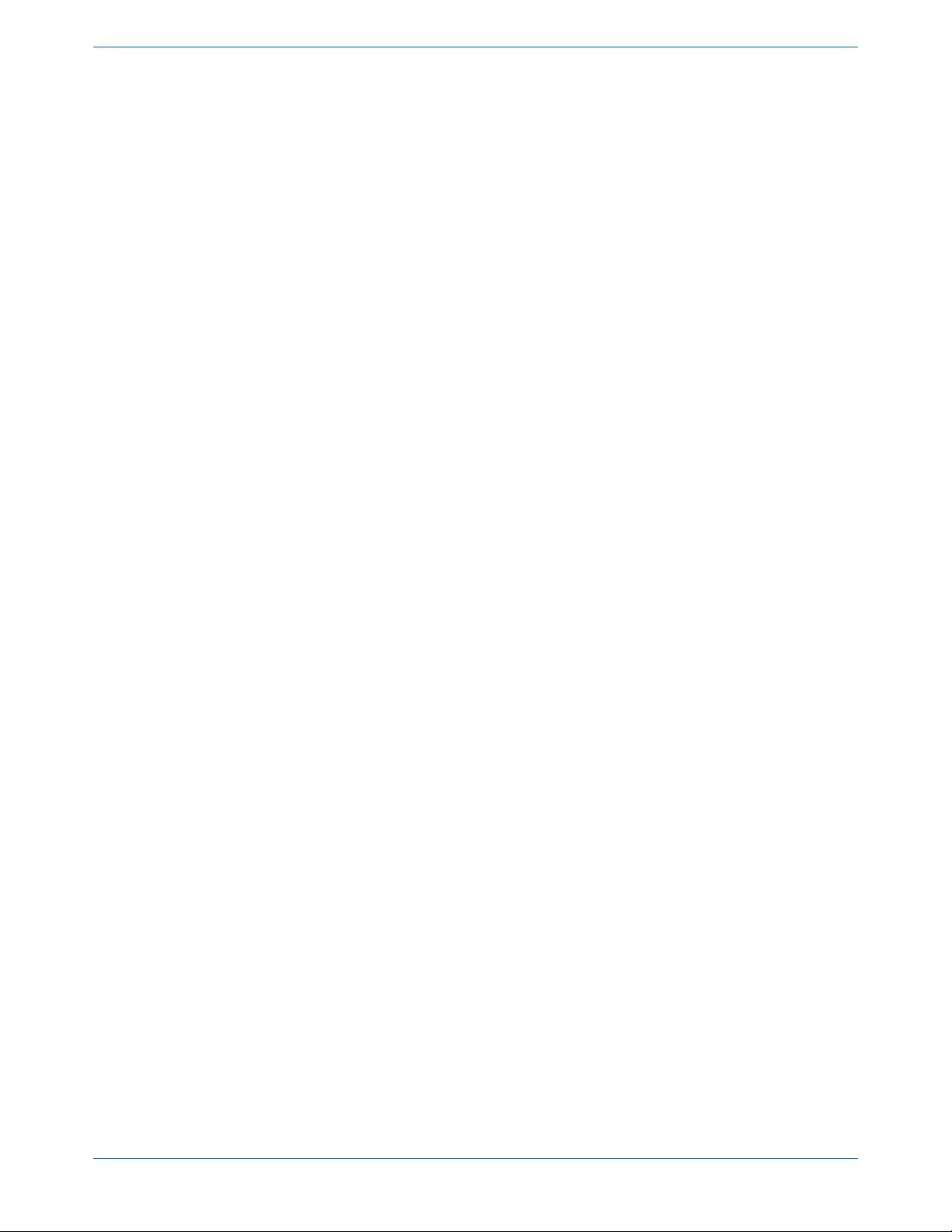
Managing App State Changes 35
The App Launch Cycle 37
Responding to Interruptions 44
Moving to the Background 46
Returning to the Foreground 50
App Termination 53
The Main Run Loop 54
Background Execution and Multitasking 56
Determining Whether Multitasking Is Available 56
Executing a Finite-Length Task in the Background 57
Scheduling the Delivery of Local Notifications 58
Implementing Long-Running Background Tasks 60
Being a Responsible Background App 65
Opting out of Background Execution 67
Concurrency and Secondary Threads 68
State Preservation and Restoration 69
The Preservation and Restoration Process 69
Flow of the Preservation Process 76
Flow of the Restoration Process 77
What Happens When You Exclude Groups of View Controllers? 80
Checklist for Implementing State Preservation and Restoration 83
Enabling State Preservation and Restoration in Your App 84
Preserving the State of Your View Controllers 84
Marking Your View Controllers for Preservation 85
Restoring Your View Controllers at Launch Time 85
Encoding and Decoding Your View Controller’s State 87
Preserving the State of Your Views 88
UIKit Views with Preservable State 89
Preserving the State of a Custom View 89
Implementing Preservation-Friendly Data Sources 90
Preserving Your App’s High-Level State 91
Mixing UIKit’s State Preservation with Your Own Custom Mechanisms 92
Tips for Saving and Restoring State Information 93
App-Related Resources 95
App Store Required Resources 95
The Information Property List File 95
Declaring the Required Device Capabilities 96
Declaring Your App’s Supported Document Types 99
2013-01-28 | © 2013 Apple Inc. All Rights Reserved.
3
Contents
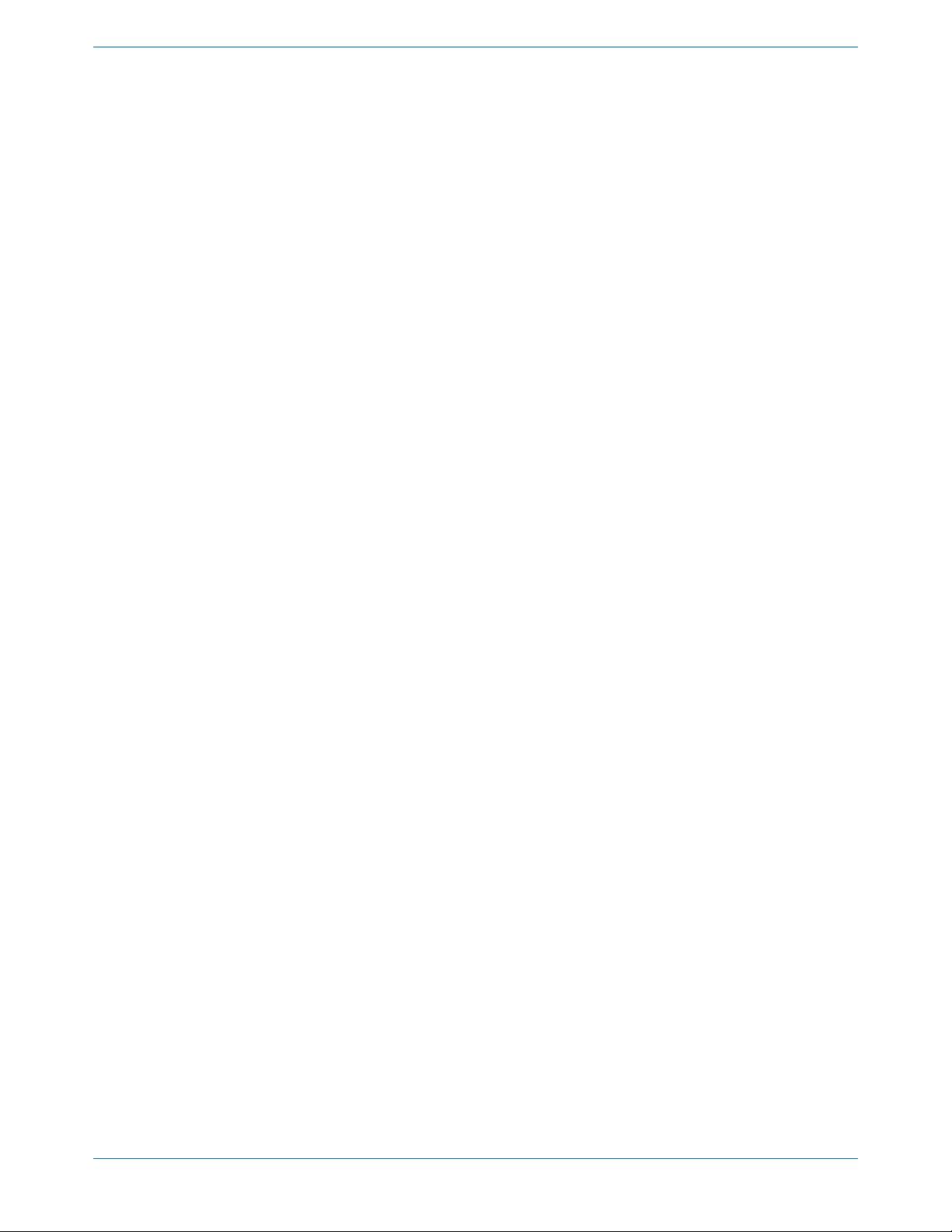
App Icons 100
App Launch (Default) Images 102
Providing Launch Images for Different Orientations 103
Providing Device-Specific Launch Images 104
Providing Launch Images for Custom URL Schemes 105
The Settings Bundle 106
Localized Resource Files 107
Loading Resources Into Your App 108
Advanced App Tricks 110
Configuring Your App to Support iPhone 5 110
Creating a Universal App 110
Updating Your Info.plist Settings 111
Implementing Your View Controllers and Views 112
Updating Your Resource Files 113
Using Runtime Checks to Create Conditional Code Paths 113
Supporting Multiple Versions of iOS 114
Launching in Landscape Mode 115
Installing App-Specific Data Files at First Launch 116
Protecting Data Using On-Disk Encryption 116
Tips for Developing a VoIP App 118
Configuring Sockets for VoIP Usage 118
Installing a Keep-Alive Handler 120
Configuring Your App’s Audio Session 120
Using the Reachability Interfaces to Improve the User Experience 120
Communicating with Other Apps 121
Implementing Custom URL Schemes 122
Registering Custom URL Schemes 122
Handling URL Requests 123
Showing and Hiding the Keyboard 127
Turning Off Screen Locking 128
Performance Tuning 129
Make App Backups More Efficient 129
App Backup Best Practices 129
Files Saved During App Updates 130
Use Memory Efficiently 131
Observe Low-Memory Warnings 131
Reduce Your App’s Memory Footprint 132
Allocate Memory Wisely 133
2013-01-28 | © 2013 Apple Inc. All Rights Reserved.
4
Contents
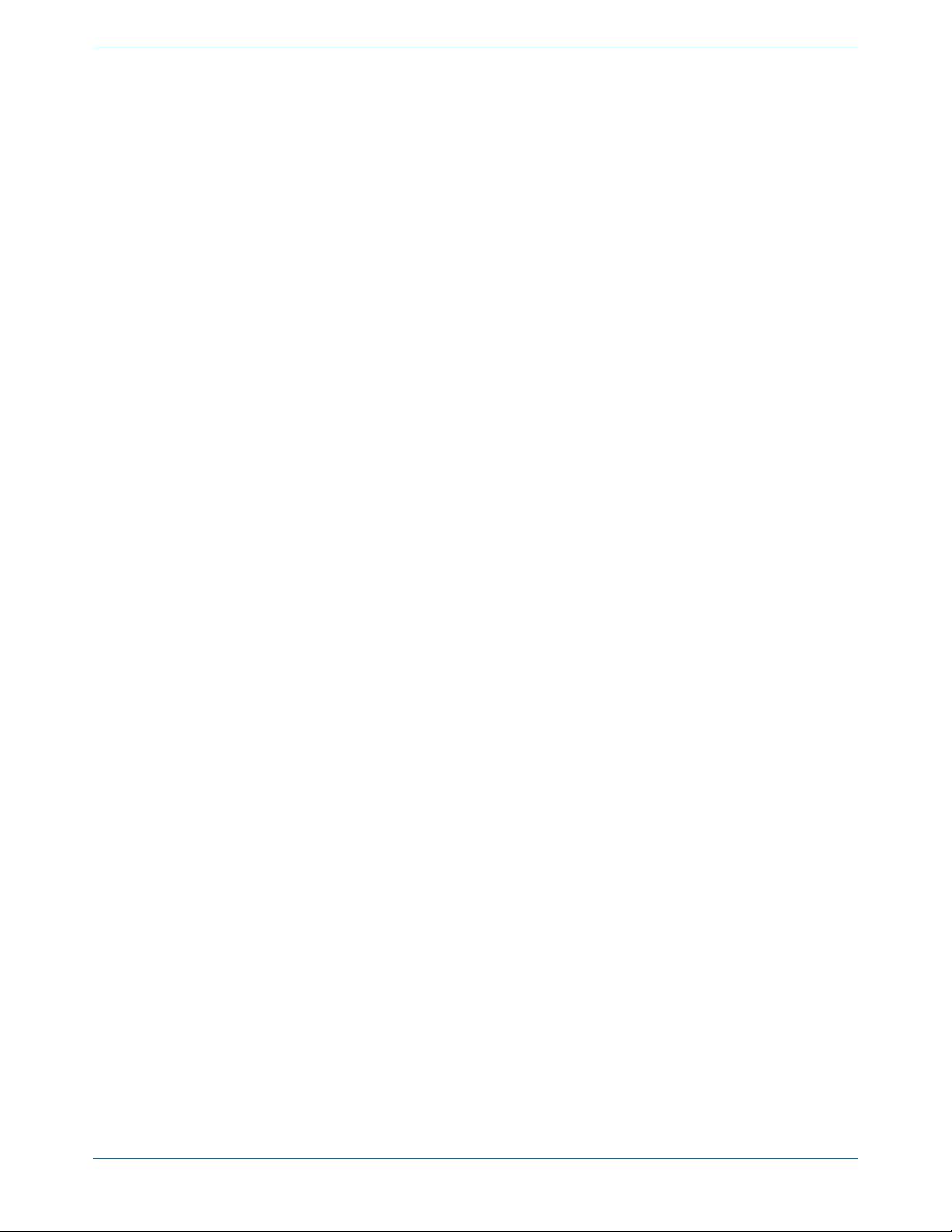
Move Work off the Main Thread 133
Floating-Point Math Considerations 134
Reduce Power Consumption 134
Tune Your Code 136
Improve File Access Times 136
Tune Your Networking Code 137
Tips for Efficient Networking 137
Using Wi-Fi 138
The Airplane Mode Alert 138
The iOS Environment 139
Specialized System Behaviors 139
The Virtual Memory System 139
The Automatic Sleep Timer 139
Multitasking Support 140
Security 140
The App Sandbox 140
Keychain Data 142
Document Revision History 143
2013-01-28 | © 2013 Apple Inc. All Rights Reserved.
5
Contents
- 1
- 2
- 3
前往页