根据提供的文件信息,我们可以深入探讨ASP.NET的基本概念及其在网页开发中的应用。下面将详细介绍ASP.NET的基本原理、页面生命周期以及代码示例中的关键组件。 ### ASP.NET简介 ASP.NET是微软开发的一种服务器端脚本环境,它允许开发者使用C#或VB.NET等编程语言创建动态Web应用程序。ASP.NET构建于.NET Framework之上,提供了丰富的类库支持,使得开发者可以轻松地进行Web开发工作。 ### ASP.NET页面生命周期 理解ASP.NET页面生命周期对于编写高效、可靠的Web应用程序至关重要。ASP.NET页面生命周期主要包含以下几个阶段: 1. **初始化**:在此阶段,页面控件被创建并初始化。 2. **加载视图状态**:如果页面存在视图状态,则加载视图状态。 3. **加载回发状态**:如果页面包含回发事件,则加载这些事件的状态。 4. **处理回发事件**:处理由用户触发的任何回发事件。 5. **渲染**:将页面输出到客户端浏览器。 6. **卸载**:页面资源被释放。 ### 文件分析 在提供的代码片段中,我们看到了一个简单的ASP.NET页面示例,该页面使用了VBScript作为脚本语言。下面将详细解释这段代码的关键部分: #### HTML头部定义 ```html <%@Language=VBScript%> <html> <head> <title>2000081402</title> </head> ``` 这里定义了页面使用的脚本语言为VBScript,并设置了HTML文档的基本结构。 #### 表单提交处理 ```vb <% If Request.Form.Count <> 0 Then Response.Write "Your name is " Response.Write Request.Form("txtName") Response.Write ", and your favorite color is " Response.Write Request.Form("selColor") Response.Write "<br>" End If %> ``` 这段代码用于处理表单提交后的数据。当用户提交表单后,服务器会检查`Request.Form`集合是否为空(即是否有数据提交)。如果不为空,则使用`Response.Write`方法显示用户的姓名和最喜欢的颜色。 #### 表单元素 ```html <form id="sample1" method="post" action="sample1.asp"> <table cellSpacing=0 cellPadding=4 border=0> <tr> <td><p align="right">What is your name:</p></td> <td><input type="text" name="txtName" value="<%=Request.Form("txtName")%>"></td> </tr> <tr> <td><p align="right">What is your favorite color:</p></td> <td> <select name="selColor"> <option<% if Request.Form("selColor") = "Black" Then Response.Write "selected" %> >Black</option> <option<% if Request.Form("selColor") = "Blue" Then Response.Write "selected" %> >Blue</option> <option<% if Request.Form("selColor") = "Green" Then Response.Write "selected" %> >Green</option> <option<% if Request.Form("selColor") = "Pink" Then Response.Write "selected" %> >Pink</option> <option<% if Request.Form("selColor") = "Red" Then Response.Write "selected" %> >Red</option> </select> </td> </tr> <tr> <td></td> <td><input type="submit" id="submit" value="Submit"></td> </tr> </table> </form> ``` 此部分包含了两个表单字段:文本框和下拉列表。其中: - **文本框**用于输入姓名,其值通过`<%=Request.Form("txtName")%>`进行预填充。 - **下拉列表**让用户选择自己喜欢的颜色。如果`Request.Form("selColor")`有值,则相应选项会被选中。 ### 总结 通过上述分析,我们可以看到ASP.NET提供了一种强大的方式来构建交互式Web应用程序。通过结合HTML、CSS和服务器端脚本(如VBScript),开发者可以创建功能丰富且用户友好的界面。此外,对ASP.NET页面生命周期的理解有助于更好地管理页面的状态,从而提高应用程序的整体性能和用户体验。
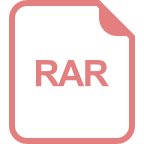
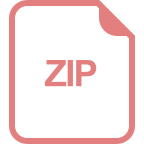
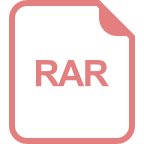
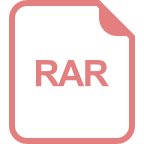
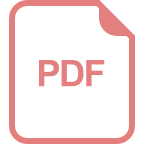
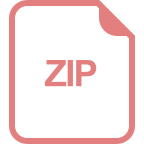
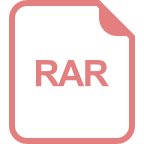
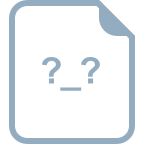
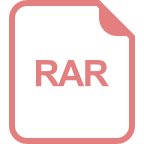
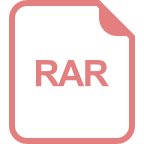
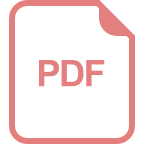
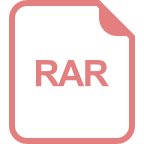
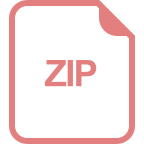
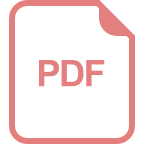
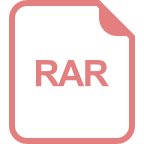
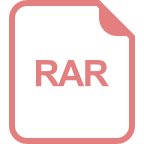
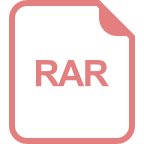
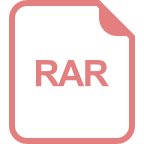
<html>
<head>
<title>2000081402</title>
</head>
<body>
<form id="sample1" method="post"action="sample1.asp">
<%
If Request.Form.Count <> 0 Then
Response.Write "Your name is "
Response.Write Request.Form("txtName")
Response.Write ", and your favoritecolor is "
Response.Write Request.Form("selColor")
Response.Write "<br>"
End If
%>
<table cellSpacing=0 cellPadding=4 border=0>
<tr>
<td><p align=right>What is yourname:</p></td>
<td><input type="text"name= txtNamevalue="<%=Request.Form("txtName")%>"></td></tr>
<tr>
<td><p align=right>What is yourfavorite color:</p></td>
<td>
<select name=selColor>
<option <%if Request.Form("selColor")= "Black" Then Response.Write "selected"%>>Black</option>
<option <%if Request.Form("selColor")= "Blue" Then Response.Write "selected"%>>Blue</option>
<option <%if Request.Form("selColor")= "Green" Then Response.Write "selected"%>>Green</option>
<option <%if Request.Form("selColor")= "Pink" Then Response.Write "selected"%>>Pink</option>
<option <%if Request.Form("selColor")= "Red" Then Response.Write "selected"%>>Red</option>
</select>
<tr>
<td> </td>
<td><input type="submit"id=submit value="Submit"></td></tr>
</table>
</form>
</body>
</html>
我们看到,在这里我们不得不将ASP代码和HTML混在一起,使得代码非常的难以看懂,想象一下如果一个非常复杂的页面……
我们的页面首先判断是否是回传,还是第一次访问。我们通过检查Request.Form集合。如果是0,表示是第一次访问,否则表示提交的按钮被按下了,我们将通过Response.Write输出一条信息给用户。
<%
If Request.Form.Count <> 0 Then
Response.Write "Your name is "
Response.Write Request.Form("txtName")
Response.Write ", and your favoritecolor is "
Response.Write Request.Form("selColor")
Response.Write "<br>"
End If
%>
对于我们表单中的每个元素,我们必须通过代码来使它们保持状态。这些代码简单而相似。
<td><input type="text"name= txtNamevalue="<%=Request.Form("txtName")%>"></td></tr>
...
<select name=selColor>
<option <%if Request.Form("selColor")= "Black" Then Response.Write "selected"%>>Black</option>
<option <%if Request.Form("selColor")= "Blue" Then Response.Write "selected"%>>Blue</option>
<option <%if Request.Form("selColor")= "Green" Then Response.Write "selected"%>>Green</option>
<option <%if Request.Form("selColor")= "Pink" Then Response.Write "selected"%>>Pink</option>
<option <%if Request.Form("selColor")= "Red" Then Response.Write "selected"%>>Red</option>
剩余5页未读,继续阅读
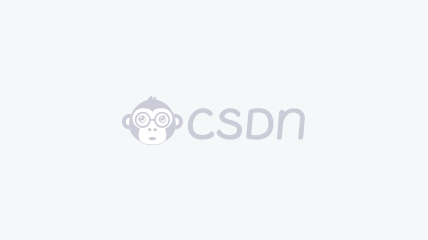

- 粉丝: 1
- 资源: 11
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

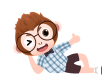
最新资源

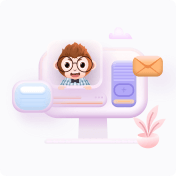
