# Prophecy
[](https://packagist.org/packages/phpspec/prophecy)
[](https://travis-ci.org/phpspec/prophecy)
Prophecy is a highly opinionated yet very powerful and flexible PHP object mocking
framework. Though initially it was created to fulfil phpspec2 needs, it is flexible
enough to be used inside any testing framework out there with minimal effort.
## A simple example
```php
<?php
class UserTest extends PHPUnit_Framework_TestCase
{
private $prophet;
public function testPasswordHashing()
{
$hasher = $this->prophet->prophesize('App\Security\Hasher');
$user = new App\Entity\User($hasher->reveal());
$hasher->generateHash($user, 'qwerty')->willReturn('hashed_pass');
$user->setPassword('qwerty');
$this->assertEquals('hashed_pass', $user->getPassword());
}
protected function setup()
{
$this->prophet = new \Prophecy\Prophet;
}
protected function tearDown()
{
$this->prophet->checkPredictions();
}
}
```
## Installation
### Prerequisites
Prophecy requires PHP 5.3.3 or greater.
### Setup through composer
First, add Prophecy to the list of dependencies inside your `composer.json`:
```json
{
"require-dev": {
"phpspec/prophecy": "~1.0"
}
}
```
Then simply install it with composer:
```bash
$> composer install --prefer-dist
```
You can read more about Composer on its [official webpage](http://getcomposer.org).
## How to use it
First of all, in Prophecy every word has a logical meaning, even the name of the library
itself (Prophecy). When you start feeling that, you'll become very fluid with this
tool.
For example, Prophecy has been named that way because it concentrates on describing the future
behavior of objects with very limited knowledge about them. But as with any other prophecy,
those object prophecies can't create themselves - there should be a Prophet:
```php
$prophet = new Prophecy\Prophet;
```
The Prophet creates prophecies by *prophesizing* them:
```php
$prophecy = $prophet->prophesize();
```
The result of the `prophesize()` method call is a new object of class `ObjectProphecy`. Yes,
that's your specific object prophecy, which describes how your object would behave
in the near future. But first, you need to specify which object you're talking about,
right?
```php
$prophecy->willExtend('stdClass');
$prophecy->willImplement('SessionHandlerInterface');
```
There are 2 interesting calls - `willExtend` and `willImplement`. The first one tells
object prophecy that our object should extend specific class, the second one says that
it should implement some interface. Obviously, objects in PHP can implement multiple
interfaces, but extend only one parent class.
### Dummies
Ok, now we have our object prophecy. What can we do with it? First of all, we can get
our object *dummy* by revealing its prophecy:
```php
$dummy = $prophecy->reveal();
```
The `$dummy` variable now holds a special dummy object. Dummy objects are objects that extend
and/or implement preset classes/interfaces by overriding all their public methods. The key
point about dummies is that they do not hold any logic - they just do nothing. Any method
of the dummy will always return `null` and the dummy will never throw any exceptions.
Dummy is your friend if you don't care about the actual behavior of this double and just need
a token object to satisfy a method typehint.
You need to understand one thing - a dummy is not a prophecy. Your object prophecy is still
assigned to `$prophecy` variable and in order to manipulate with your expectations, you
should work with it. `$dummy` is a dummy - a simple php object that tries to fulfil your
prophecy.
### Stubs
Ok, now we know how to create basic prophecies and reveal dummies from them. That's
awesome if we don't care about our _doubles_ (objects that reflect originals)
interactions. If we do, we need to use *stubs* or *mocks*.
A stub is an object double, which doesn't have any expectations about the object behavior,
but when put in specific environment, behaves in specific way. Ok, I know, it's cryptic,
but bear with me for a minute. Simply put, a stub is a dummy, which depending on the called
method signature does different things (has logic). To create stubs in Prophecy:
```php
$prophecy->read('123')->willReturn('value');
```
Oh wow. We've just made an arbitrary call on the object prophecy? Yes, we did. And this
call returned us a new object instance of class `MethodProphecy`. Yep, that's a specific
method with arguments prophecy. Method prophecies give you the ability to create method
promises or predictions. We'll talk about method predictions later in the _Mocks_ section.
#### Promises
Promises are logical blocks, that represent your fictional methods in prophecy terms
and they are handled by the `MethodProphecy::will(PromiseInterface $promise)` method.
As a matter of fact, the call that we made earlier (`willReturn('value')`) is a simple
shortcut to:
```php
$prophecy->read('123')->will(new Prophecy\Promise\ReturnPromise(array('value')));
```
This promise will cause any call to our double's `read()` method with exactly one
argument - `'123'` to always return `'value'`. But that's only for this
promise, there's plenty others you can use:
- `ReturnPromise` or `->willReturn(1)` - returns a value from a method call
- `ReturnArgumentPromise` or `->willReturnArgument($index)` - returns the nth method argument from call
- `ThrowPromise` or `->willThrow` - causes the method to throw specific exception
- `CallbackPromise` or `->will($callback)` - gives you a quick way to define your own custom logic
Keep in mind, that you can always add even more promises by implementing
`Prophecy\Promise\PromiseInterface`.
#### Method prophecies idempotency
Prophecy enforces same method prophecies and, as a consequence, same promises and
predictions for the same method calls with the same arguments. This means:
```php
$methodProphecy1 = $prophecy->read('123');
$methodProphecy2 = $prophecy->read('123');
$methodProphecy3 = $prophecy->read('321');
$methodProphecy1 === $methodProphecy2;
$methodProphecy1 !== $methodProphecy3;
```
That's interesting, right? Now you might ask me how would you define more complex
behaviors where some method call changes behavior of others. In PHPUnit or Mockery
you do that by predicting how many times your method will be called. In Prophecy,
you'll use promises for that:
```php
$user->getName()->willReturn(null);
// For PHP 5.4
$user->setName('everzet')->will(function () {
$this->getName()->willReturn('everzet');
});
// For PHP 5.3
$user->setName('everzet')->will(function ($args, $user) {
$user->getName()->willReturn('everzet');
});
// Or
$user->setName('everzet')->will(function ($args) use ($user) {
$user->getName()->willReturn('everzet');
});
```
And now it doesn't matter how many times or in which order your methods are called.
What matters is their behaviors and how well you faked it.
#### Arguments wildcarding
The previous example is awesome (at least I hope it is for you), but that's not
optimal enough. We hardcoded `'everzet'` in our expectation. Isn't there a better
way? In fact there is, but it involves understanding what this `'everzet'`
actually is.
You see, even if method arguments used during method prophecy creation look
like simple method arguments, in reality they are not. They are argument token
wildcards. As a matter of fact, `->setName('everzet')` looks like a simple call just
because Prophecy automatically transforms it under the hood into:
```php
$user->setName(new Prophecy\Argument\Token\ExactValueToken('everzet'));
```
Those argument tokens are simple PHP classes, that implement
`Prophecy\Argument\Token\TokenInterface` and tell Prophecy how to compare real arguments
with your expectations. And yes, those cl
没有合适的资源?快使用搜索试试~ 我知道了~
php7实践指南配书源代码.
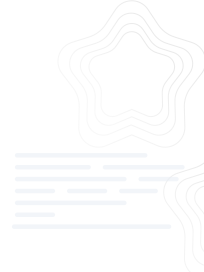
共1735个文件
php:1197个
phpt:136个
md:60个


温馨提示
在Web开发领域,PHP因免费开源、语法简单属于类C风格语言,具有良好的跨平台性而受到广大业内人士的支持。经过多个预发布版本, PHP 5.0在2004年7月13日发布。该版本使用Zend引擎Ⅱ,并且加入了新功能,完全支持面向对象。2015年12月3日,PHP 7.0.0 GA发布,性能较PHP 5.6提升了两倍,新增了一些操作符和函数的返回类型声明,也增加了对匿名类的支持等。关于PHP 7的讨论在网上也逐渐展开。不过到目前为止,国内有关专门介绍PHP 7应用开发的书籍还很少,本书的目的就是对现有的PHP 7技术进行一个汇总,书中内容是笔者在PHP 7学习和实际工作项目中的心得体会和系统总结,希望能够帮助PHP 7学习者更好地了解其新特性,并应用于实际开发中。
资源推荐
资源详情
资源评论
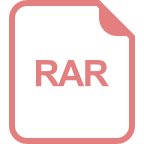
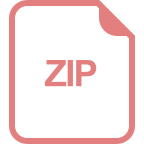
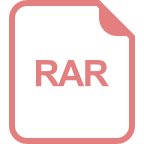
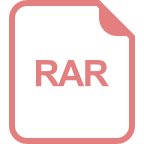
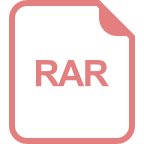
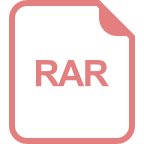
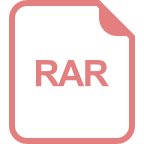
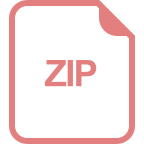
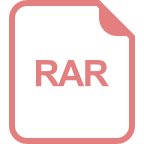
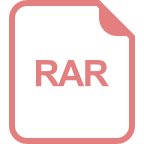
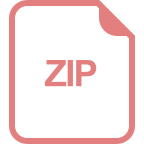
收起资源包目录

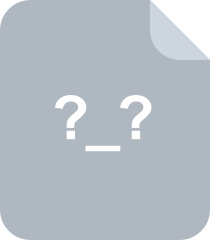
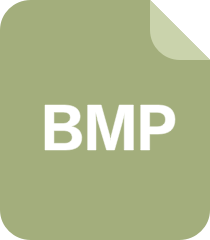
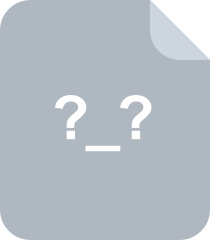
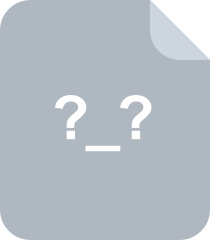
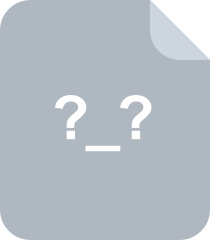
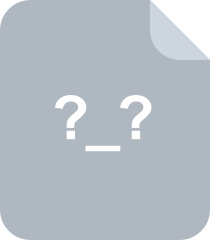
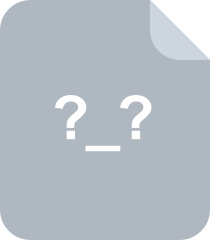
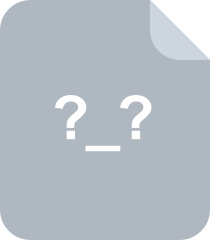
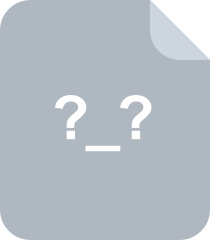
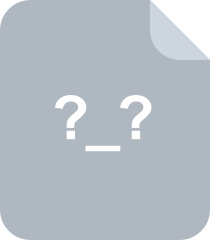
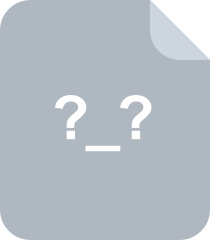
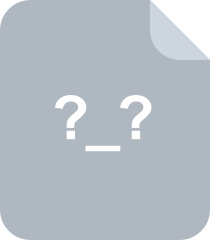
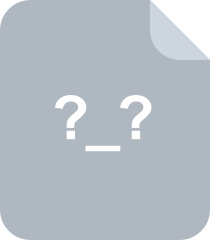
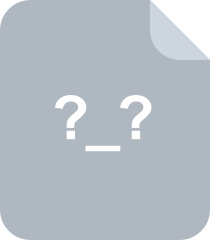
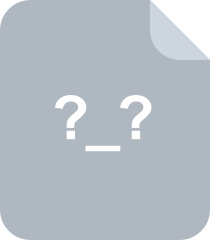
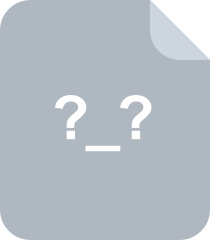
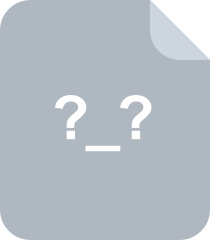
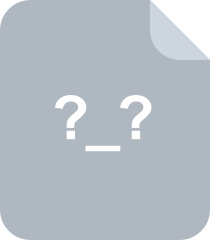
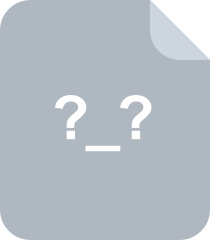
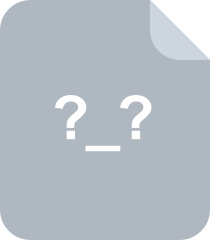
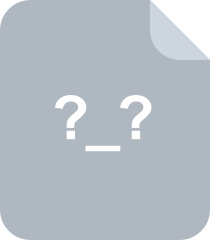
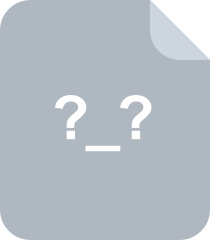
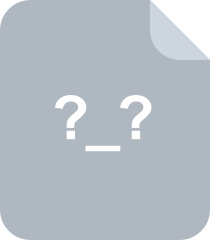
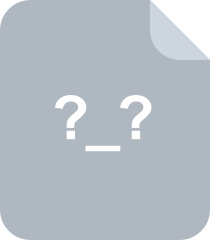
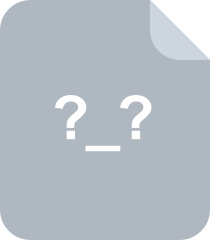
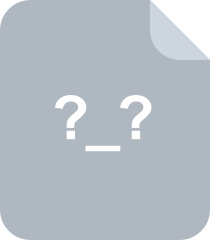
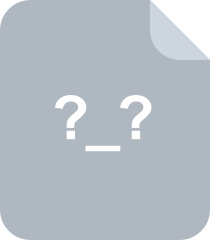
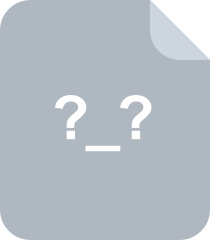
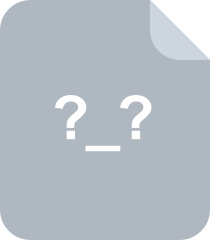
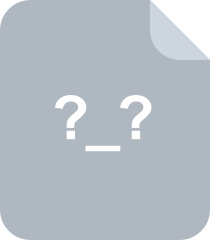
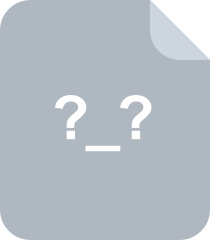
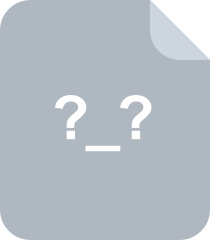
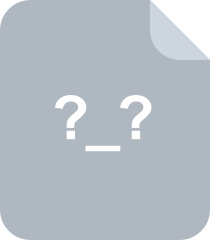
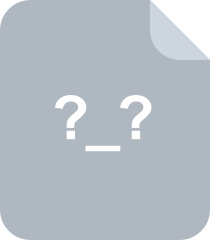
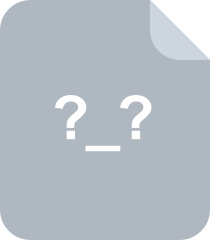
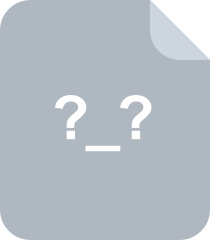
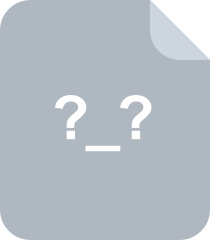
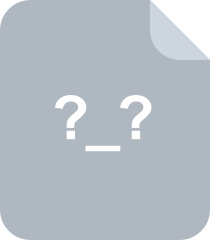
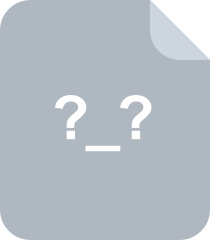
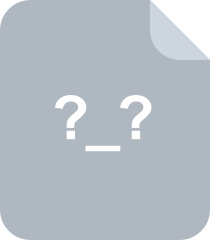
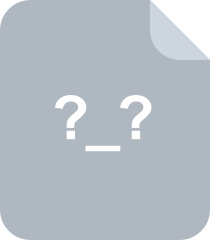
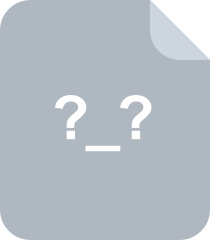
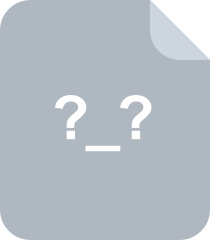
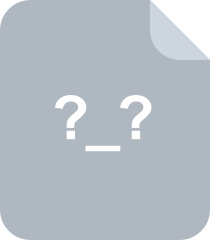
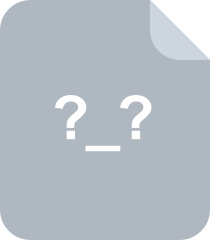
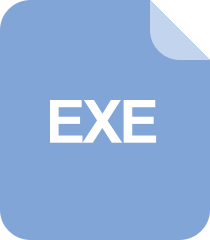
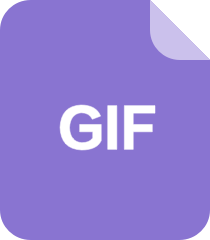
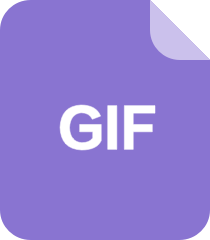
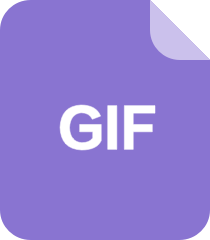
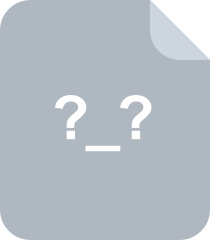
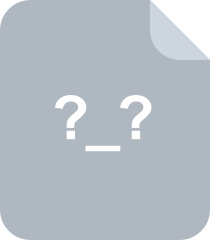
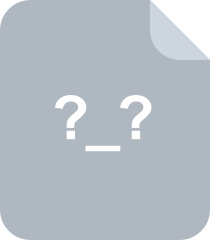
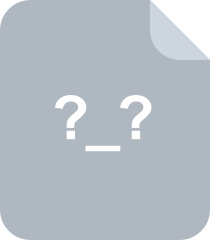
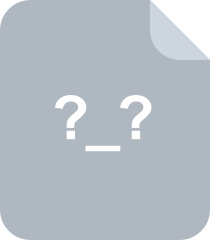
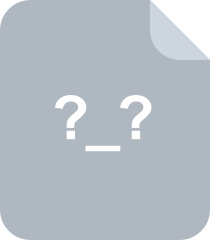
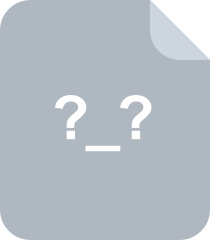
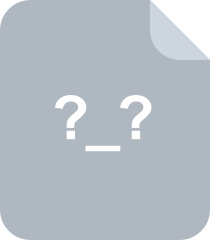
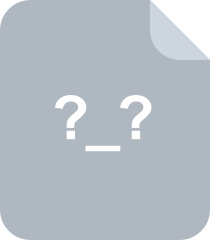
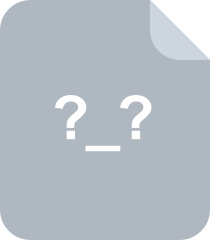
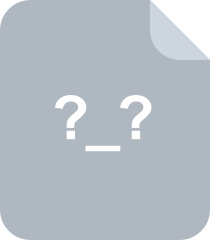
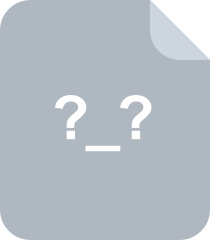
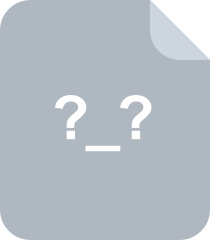
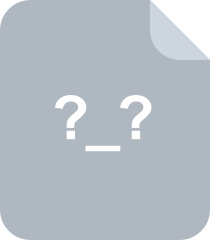
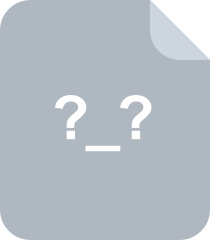
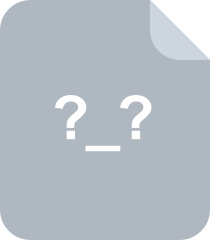
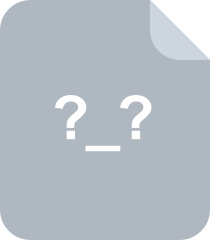
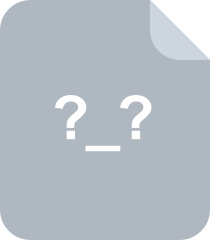
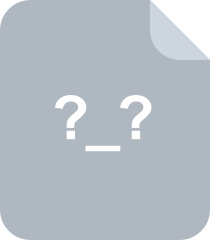
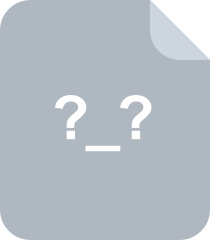
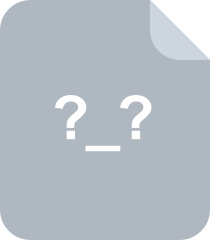
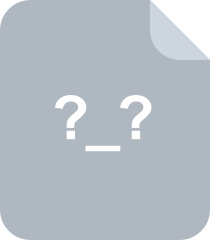
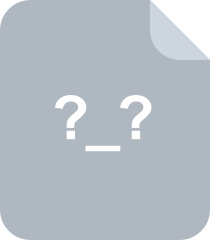
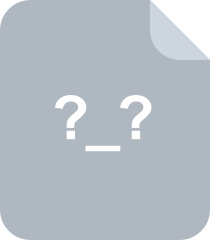
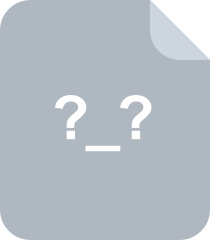
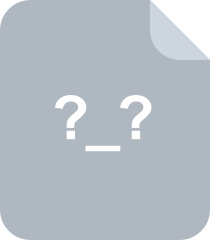
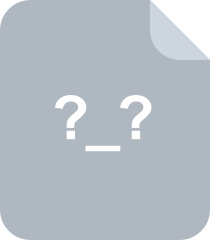
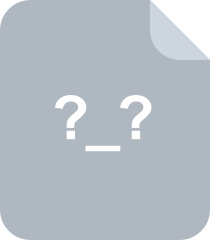
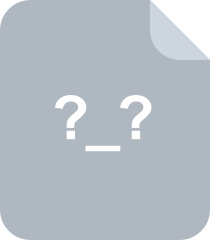
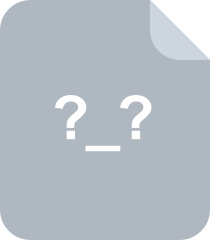
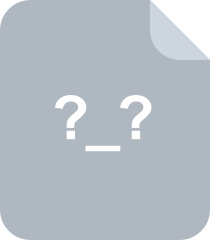
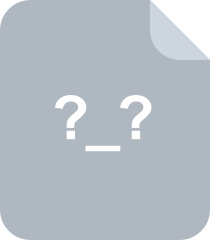
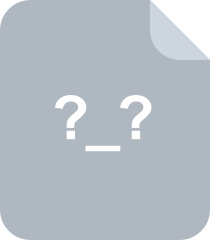
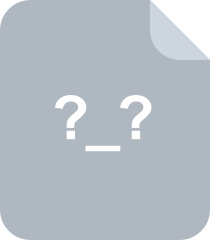
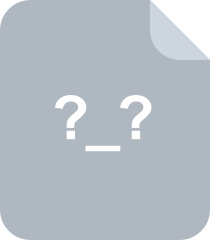
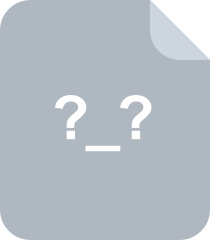
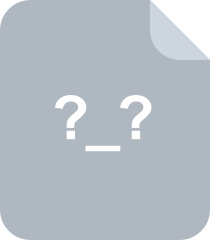
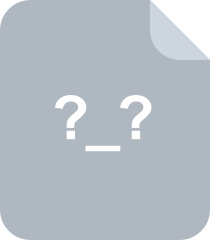
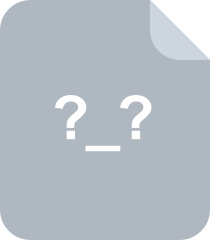
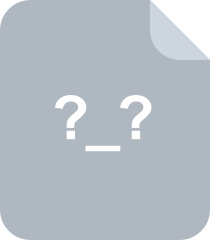
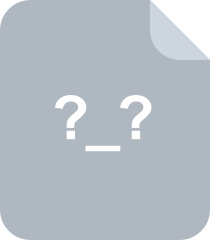
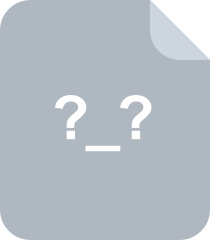
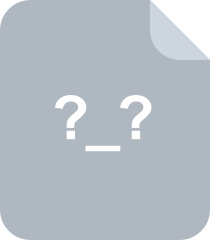
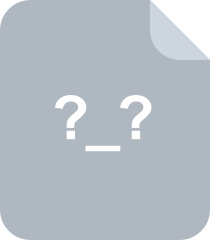
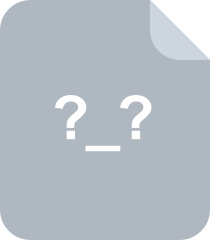
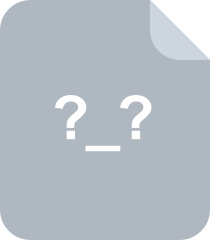
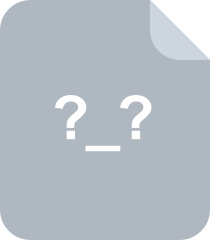
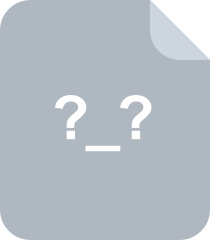
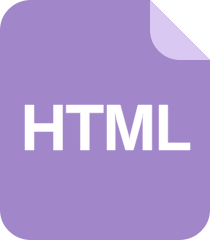
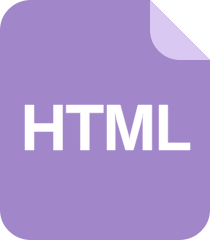
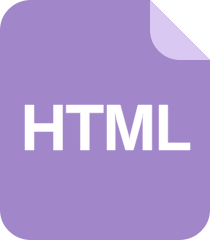
共 1735 条
- 1
- 2
- 3
- 4
- 5
- 6
- 18
资源评论
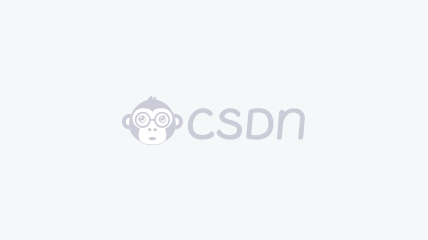
- 好名字占了2020-08-24不知道是什么书的代码, 乱七八糟的,根本看不成。不建议下!

yanganmy
- 粉丝: 1
- 资源: 5
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

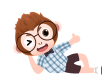
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


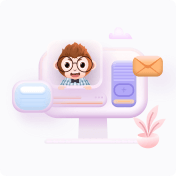
安全验证
文档复制为VIP权益,开通VIP直接复制
