/* ====================================================================
* Copyright (c) 1999 The OpenSSL Project. All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions
* are met:
*
* 1. Redistributions of source code must retain the above copyright
* notice, this list of conditions and the following disclaimer.
*
* 2. Redistributions in binary form must reproduce the above copyright
* notice, this list of conditions and the following disclaimer in
* the documentation and/or other materials provided with the
* distribution.
*
* 3. All advertising materials mentioning features or use of this
* software must display the following acknowledgment:
* "This product includes software developed by the OpenSSL Project
* for use in the OpenSSL Toolkit. (http://www.openssl.org/)"
*
* 4. The names "OpenSSL Toolkit" and "OpenSSL Project" must not be used to
* endorse or promote products derived from this software without
* prior written permission. For written permission, please contact
* openssl-core@openssl.org.
*
* 5. Products derived from this software may not be called "OpenSSL"
* nor may "OpenSSL" appear in their names without prior written
* permission of the OpenSSL Project.
*
* 6. Redistributions of any form whatsoever must retain the following
* acknowledgment:
* "This product includes software developed by the OpenSSL Project
* for use in the OpenSSL Toolkit (http://www.openssl.org/)"
*
* THIS SOFTWARE IS PROVIDED BY THE OpenSSL PROJECT ``AS IS'' AND ANY
* EXPRESSED OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR
* PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE OpenSSL PROJECT OR
* ITS CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL,
* SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT
* NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES;
* LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION)
* HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT,
* STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
* ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED
* OF THE POSSIBILITY OF SUCH DAMAGE.
* ====================================================================
*
* This product includes cryptographic software written by Eric Young
* (eay@cryptsoft.com). This product includes software written by Tim
* Hudson (tjh@cryptsoft.com).
*
*/
#ifndef HEADER_SAFESTACK_H
# define HEADER_SAFESTACK_H
# include <openssl/stack.h>
#ifdef __cplusplus
extern "C" {
#endif
# ifndef CHECKED_PTR_OF
# define CHECKED_PTR_OF(type, p) \
((void*) (1 ? p : (type*)0))
# endif
/*
* In C++ we get problems because an explicit cast is needed from (void *) we
* use CHECKED_STACK_OF to ensure the correct type is passed in the macros
* below.
*/
# define CHECKED_STACK_OF(type, p) \
((_STACK*) (1 ? p : (STACK_OF(type)*)0))
# define CHECKED_SK_COPY_FUNC(type, p) \
((void *(*)(void *)) ((1 ? p : (type *(*)(const type *))0)))
# define CHECKED_SK_FREE_FUNC(type, p) \
((void (*)(void *)) ((1 ? p : (void (*)(type *))0)))
# define CHECKED_SK_CMP_FUNC(type, p) \
((int (*)(const void *, const void *)) \
((1 ? p : (int (*)(const type * const *, const type * const *))0)))
# define STACK_OF(type) struct stack_st_##type
# define PREDECLARE_STACK_OF(type) STACK_OF(type);
# define DECLARE_STACK_OF(type) \
STACK_OF(type) \
{ \
_STACK stack; \
};
# define DECLARE_SPECIAL_STACK_OF(type, type2) \
STACK_OF(type) \
{ \
_STACK stack; \
};
/* nada (obsolete in new safestack approach)*/
# define IMPLEMENT_STACK_OF(type)
/*-
* Strings are special: normally an lhash entry will point to a single
* (somewhat) mutable object. In the case of strings:
*
* a) Instead of a single char, there is an array of chars, NUL-terminated.
* b) The string may have be immutable.
*
* So, they need their own declarations. Especially important for
* type-checking tools, such as Deputy.
*
* In practice, however, it appears to be hard to have a const
* string. For now, I'm settling for dealing with the fact it is a
* string at all.
*/
typedef char *OPENSSL_STRING;
typedef const char *OPENSSL_CSTRING;
/*
* Confusingly, LHASH_OF(STRING) deals with char ** throughout, but
* STACK_OF(STRING) is really more like STACK_OF(char), only, as mentioned
* above, instead of a single char each entry is a NUL-terminated array of
* chars. So, we have to implement STRING specially for STACK_OF. This is
* dealt with in the autogenerated macros below.
*/
DECLARE_SPECIAL_STACK_OF(OPENSSL_STRING, char)
/*
* Similarly, we sometimes use a block of characters, NOT nul-terminated.
* These should also be distinguished from "normal" stacks.
*/
typedef void *OPENSSL_BLOCK;
DECLARE_SPECIAL_STACK_OF(OPENSSL_BLOCK, void)
/*
* SKM_sk_... stack macros are internal to safestack.h: never use them
* directly, use sk_<type>_... instead
*/
# define SKM_sk_new(type, cmp) \
((STACK_OF(type) *)sk_new(CHECKED_SK_CMP_FUNC(type, cmp)))
# define SKM_sk_new_null(type) \
((STACK_OF(type) *)sk_new_null())
# define SKM_sk_free(type, st) \
sk_free(CHECKED_STACK_OF(type, st))
# define SKM_sk_num(type, st) \
sk_num(CHECKED_STACK_OF(type, st))
# define SKM_sk_value(type, st,i) \
((type *)sk_value(CHECKED_STACK_OF(type, st), i))
# define SKM_sk_set(type, st,i,val) \
sk_set(CHECKED_STACK_OF(type, st), i, CHECKED_PTR_OF(type, val))
# define SKM_sk_zero(type, st) \
sk_zero(CHECKED_STACK_OF(type, st))
# define SKM_sk_push(type, st, val) \
sk_push(CHECKED_STACK_OF(type, st), CHECKED_PTR_OF(type, val))
# define SKM_sk_unshift(type, st, val) \
sk_unshift(CHECKED_STACK_OF(type, st), CHECKED_PTR_OF(type, val))
# define SKM_sk_find(type, st, val) \
sk_find(CHECKED_STACK_OF(type, st), CHECKED_PTR_OF(type, val))
# define SKM_sk_find_ex(type, st, val) \
sk_find_ex(CHECKED_STACK_OF(type, st), \
CHECKED_PTR_OF(type, val))
# define SKM_sk_delete(type, st, i) \
(type *)sk_delete(CHECKED_STACK_OF(type, st), i)
# define SKM_sk_delete_ptr(type, st, ptr) \
(type *)sk_delete_ptr(CHECKED_STACK_OF(type, st), CHECKED_PTR_OF(type, ptr))
# define SKM_sk_insert(type, st,val, i) \
sk_insert(CHECKED_STACK_OF(type, st), CHECKED_PTR_OF(type, val), i)
# define SKM_sk_set_cmp_func(type, st, cmp) \
((int (*)(const type * const *,const type * const *)) \
sk_set_cmp_func(CHECKED_STACK_OF(type, st), CHECKED_SK_CMP_FUNC(type, cmp)))
# define SKM_sk_dup(type, st) \
(STACK_OF(type) *)sk_dup(CHECKED_STACK_OF(type, st))
# define SKM_sk_pop_free(type, st, free_func) \
sk_pop_free(CHECKED_STACK_OF(type, st), CHECKED_SK_FREE_FUNC(type, free_func))
# define SKM_sk_deep_copy(type, st, copy_func, free_func) \
(STACK_OF(type) *)sk_deep_copy(CHECKED_STACK_OF(type, st), CHECKED_SK_COPY_FUNC(type, copy_func), CHECKED_SK_FREE_FUNC(type, free_func))
# define SKM_sk_shift(type, st) \
(type *)sk_shift(CHECKED_STACK_OF(type, st))
# define SKM_sk_pop(type, st) \
(type *)sk_pop(CHECKED_STACK_OF(type, st))
# define SKM_sk_sort(type, st) \
sk_sort(CHECKED_STACK_OF(type, st))
# define SKM_sk_is_sorted(type, st) \
sk_is_sorted(CHECKED_STACK_OF(type, st))
# define SKM_ASN1_SET_OF_d2i(type, st, pp, length, d2i_func, free_func, ex_tag, ex_class) \
(STACK_OF(type) *)d2i_ASN1_SET( \
(STACK_OF(OPENSSL_BLOCK) **)CHECKED_PTR_OF(STACK_OF(type)*, st), \
pp, length, \
CHECKED_D2I_OF(type, d2i_func), \
CHECKED_SK_FREE_FUNC(type, free_func), \
ex_tag, ex_class
没有合适的资源?快使用搜索试试~ 我知道了~
openssl-1.0.2r_build-vc17-win32-lib.rar
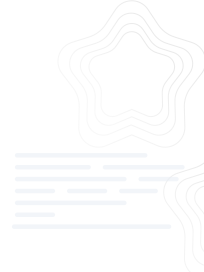
共79个文件
h:75个
lib:2个
cnf:1个

需积分: 10 32 下载量 174 浏览量
2019-05-22
09:04:48
上传
评论
收藏 4.14MB RAR 举报
温馨提示
通过vs2017编译openssl-1.0.2 release版本,有需要的可以通过官网下载编译(下载地址及编译步骤可参考https://blog.csdn.net/y601500359/article/details/89518497)
资源推荐
资源详情
资源评论
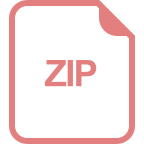
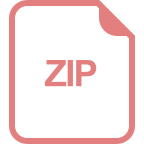
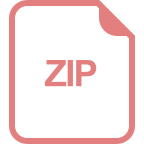
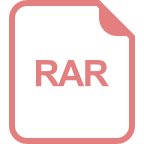
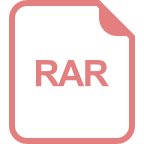
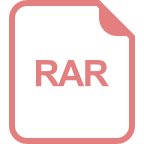
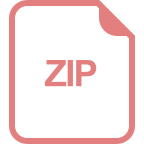
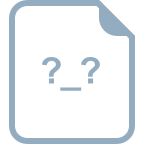
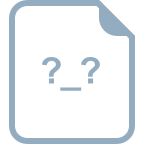
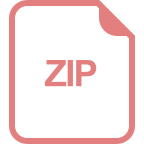
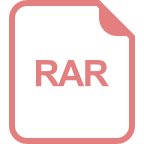
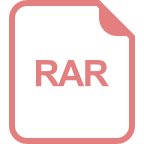
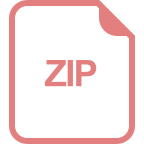
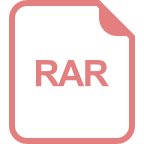
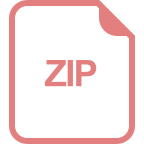
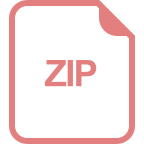
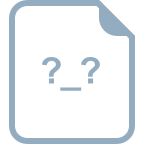
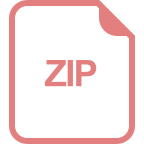
收起资源包目录



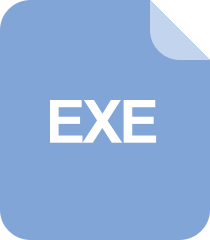

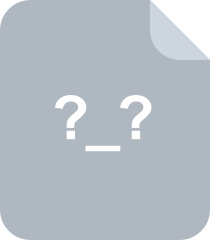


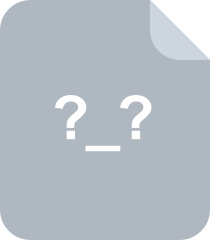
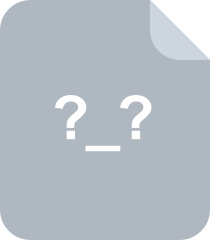
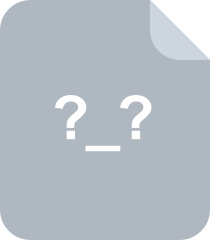
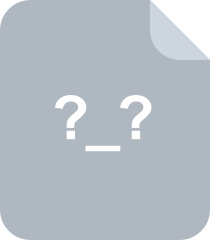
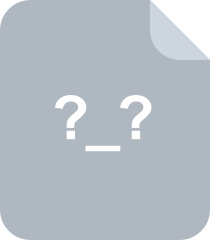
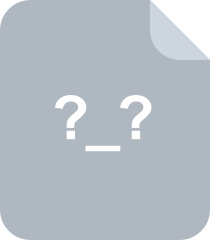
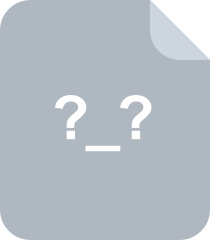
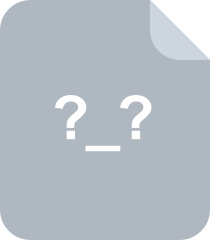
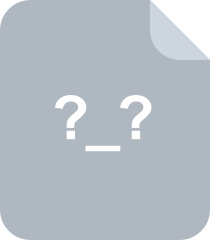
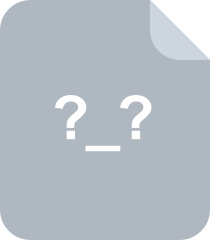
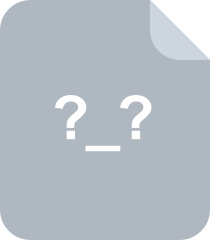
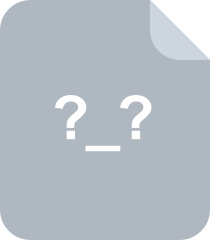
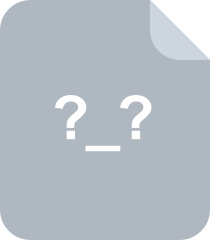
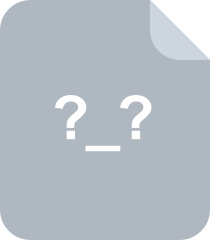
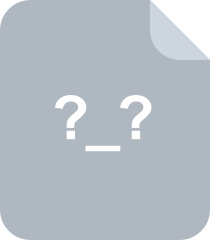
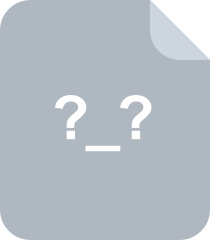
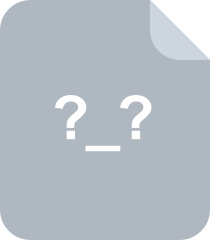
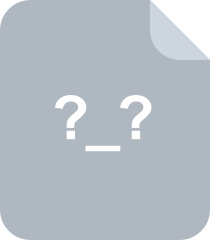
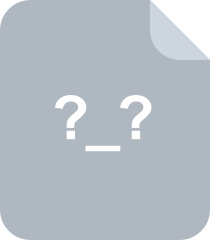
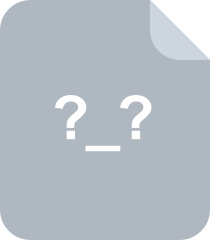
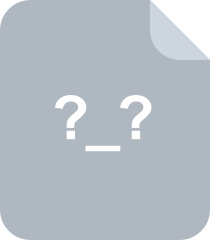
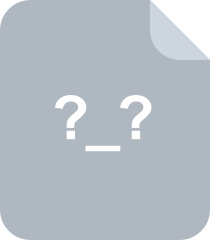
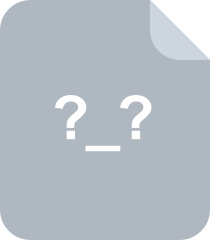
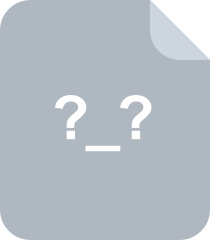
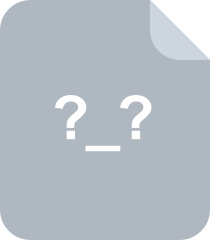
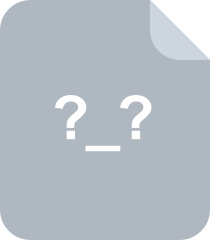
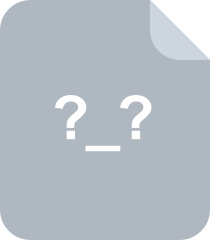
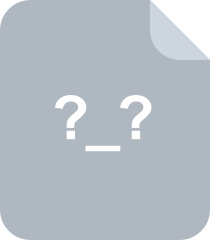
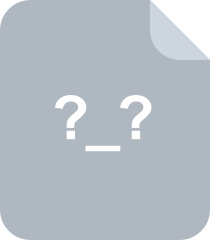
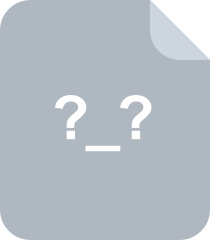
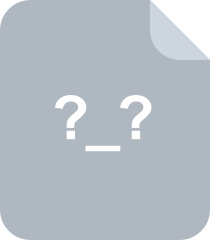
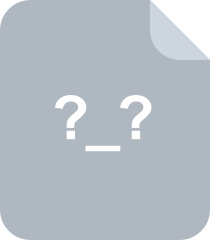
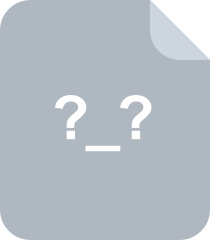
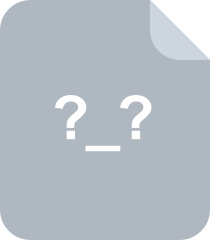
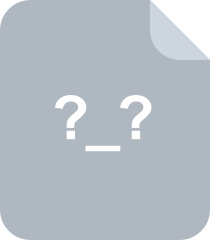
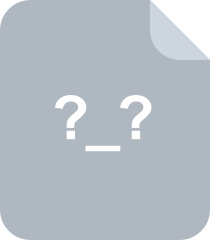
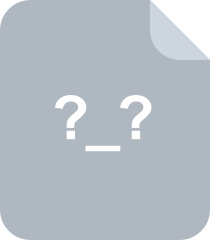
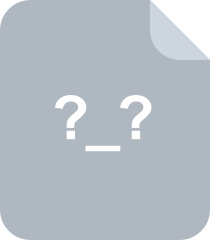
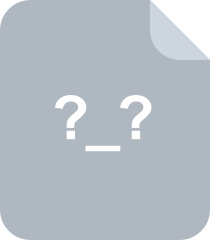
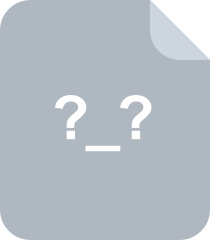
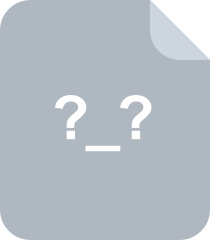
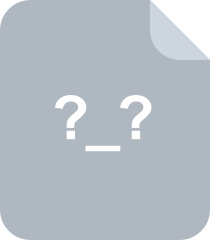
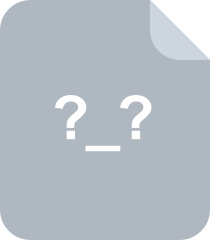
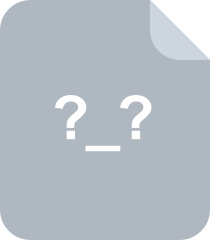
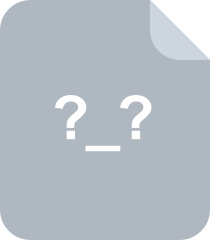
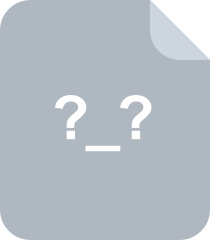
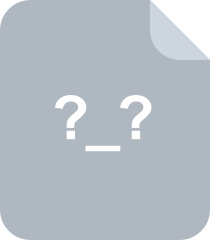
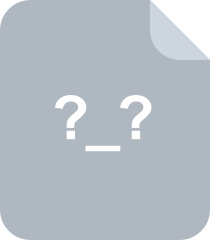
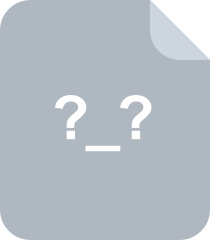
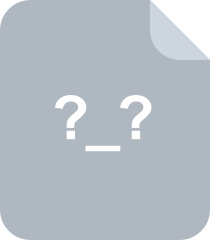
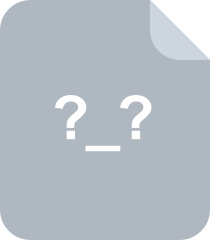
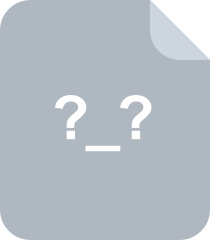
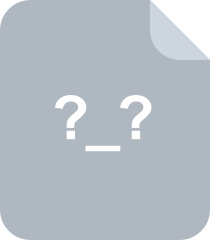
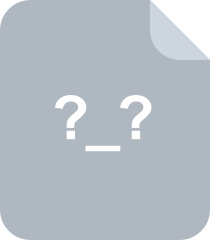
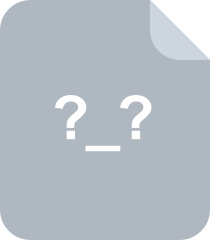
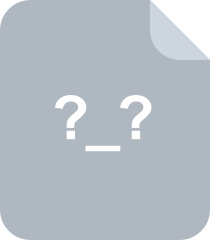
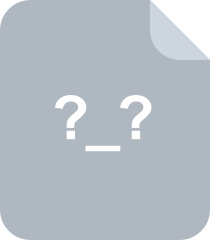
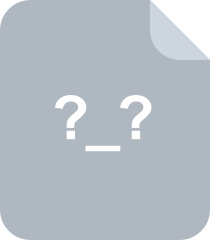
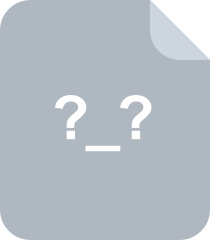
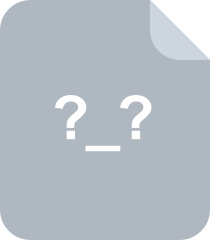
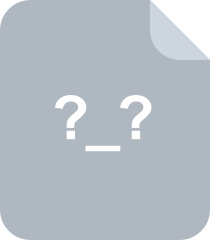
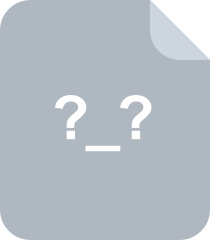
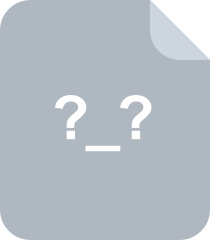
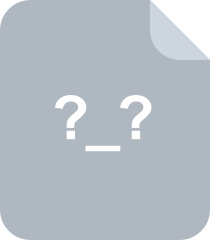
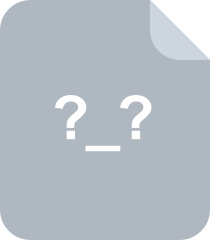
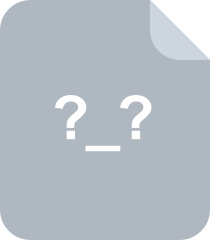
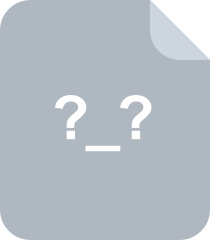
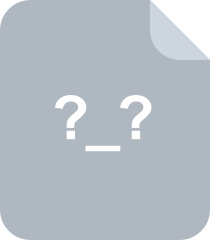
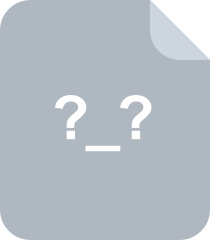
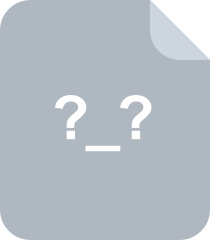
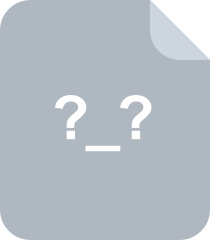
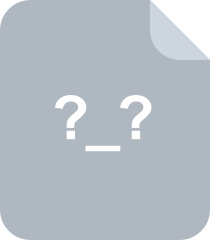
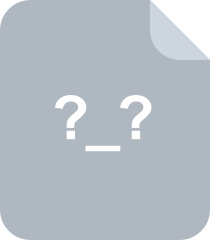
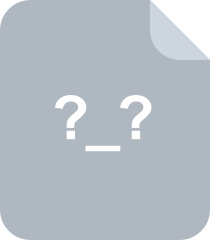
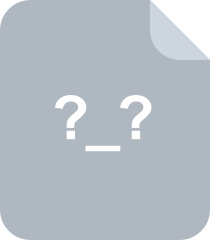

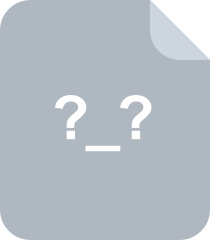
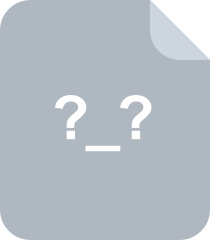
共 79 条
- 1
资源评论
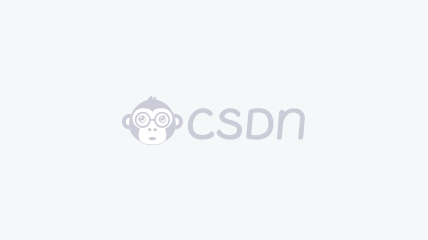

SunkingYang
- 粉丝: 2w+
- 资源: 56
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

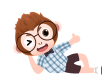
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


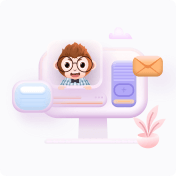
安全验证
文档复制为VIP权益,开通VIP直接复制
