package itext;
import java.awt.Color;
import java.io.FileOutputStream;
import java.io.IOException;
import com.lowagie.text.Cell;
import com.lowagie.text.Document;
import com.lowagie.text.DocumentException;
import com.lowagie.text.Element;
import com.lowagie.text.Font;
import com.lowagie.text.Image;
import com.lowagie.text.PageSize;
import com.lowagie.text.Paragraph;
import com.lowagie.text.Phrase;
import com.lowagie.text.Table;
import com.lowagie.text.pdf.BaseFont;
import com.lowagie.text.rtf.RtfWriter2;
import com.lowagie.text.rtf.style.RtfFont;
public class WordTestDemo {
public void createDocContext(String file) throws DocumentException,
IOException {
String titleStr = "城市管理案件派遣单"; //title
String executor_name = "王大锤"; //管理员
String finish_time = "2015-1-2"; //受理时间
String case_code = "2032215"; //案件编号
String creator_name = "张三"; //投诉人
String creator_tel = "1319219776x"; //投诉人电话
String inspectPic = "http://images.huanqiu.com/sarons/2013/08/8203e7e1343e6d724bba6faad1e7a04f.jpg"; //图片
String veriferPic = "http://images.huanqiu.com/sarons/2013/08/8203e7e1343e6d724bba6faad1e7a04f.jpg"; //图片
String case_road = "广场"; //所在路段
String case_location = "案件地址。。。"; //案件地址
String sourceName = "市民上报"; //案件来源
String class_name = "宣传广告"; // 大类名称
String subclass_name = "非法小广告"; //小类名称
String case_desc = "这是一个案件。。。"; //内容描述
String register_time = "2015-12-13"; //立案时间
String register_name = "管理员"; //值班长
String register_desc = "完全同意"; //立案意见
String dispatch_dept_name = "第一部门"; //责任单位
String endTimeHours = "8小时"; //处理时限
String dispatch_assist_dept_name = "第二部门"; //协助部门
String endStr = "XXXX管理局";
// 设置纸张大小
Document document = new Document(PageSize.A4);
// 建立一个书写器(Writer)与document对象关联,通过书写器(Writer)可以将文档写入到磁盘中
RtfWriter2.getInstance(document, new FileOutputStream(file));
document.open();
// 设置中文字体
BaseFont bfChinese = BaseFont.createFont("STSongStd-Light",
"UniGB-UCS2-H", BaseFont.NOT_EMBEDDED);
// 标题字体风格
RtfFont titleFont = new RtfFont("宋体", 21, Font.BOLD, Color.BLACK);
/* Font titleFont = new Font(bfChinese, 21, Font.BOLD); */
// 正文字体风格
Font contextFont = new Font(bfChinese, 12, Font.NORMAL);
Paragraph title = new Paragraph(titleStr);
// 设置标题格式对齐方式
title.setAlignment(Element.ALIGN_CENTER);
title.setFont(titleFont);
document.add(title);
// 设置 Table 表格
Table aTable = new Table(6);// 设置表格为6列
int width[] = { 11,20 ,17,17,13,22};// 每列的占比例
aTable.setWidths(width);// 设置每列所占比例
aTable.setWidth(100); // 占页面宽度 100%
aTable.setAlignment(Element.ALIGN_CENTER);// 居中显示
aTable.setAlignment(Element.ALIGN_MIDDLE);// 纵向居中显示
aTable.setAutoFillEmptyCells(true); // 自动填满
aTable.setBorderWidth(1); // 边框宽度
aTable.setBorderColor(Color.BLACK); // 边框颜色
aTable.setPadding(18);// 衬距,看效果就知道什么意思了
aTable.setSpacing(0);// 即单元格之间的间距
aTable.setBorder(1);// 边框
Font fontChinese = new Font(bfChinese, 12, Font.NORMAL, Color.black);
Cell cell = new Cell(new Phrase("管理员", fontChinese));
cell.setHorizontalAlignment(Element.ALIGN_CENTER); //水平居中
cell.setVerticalAlignment(Element.ALIGN_MIDDLE); //垂直居中
aTable.addCell(cell);
cell = new Cell(new Phrase(executor_name,fontChinese)); //管理员
cell.setVerticalAlignment(Element.ALIGN_MIDDLE);
cell.setHorizontalAlignment(Element.ALIGN_CENTER);
aTable.addCell(cell);
cell = new Cell(new Phrase("受理时间",fontChinese));
cell.setHorizontalAlignment(Element.ALIGN_CENTER); //水平居中
cell.setVerticalAlignment(Element.ALIGN_MIDDLE); //垂直居中
aTable.addCell(cell);
cell = new Cell(new Phrase(finish_time,fontChinese));
cell.setHorizontalAlignment(Element.ALIGN_CENTER); //水平居中
cell.setVerticalAlignment(Element.ALIGN_MIDDLE); //垂直居中
aTable.addCell(cell);
cell = new Cell(new Phrase("案件编号",fontChinese));
cell.setHorizontalAlignment(Element.ALIGN_CENTER); //水平居中
cell.setVerticalAlignment(Element.ALIGN_MIDDLE); //垂直居中
aTable.addCell(cell);
cell = new Cell(new Phrase(case_code,fontChinese));
cell.setHorizontalAlignment(Element.ALIGN_CENTER); //水平居中
cell.setVerticalAlignment(Element.ALIGN_MIDDLE); //垂直居中
aTable.addCell(cell);
// document.add(aTable);
//
// aTable = new Table(4);
// int width2[] = { 25,25 ,25,25};// 每列的占比例
// aTable.setWidths(width2);// 设置每列所占比例
// aTable.setWidth(100); // 占页面宽度 100%
cell = new Cell(new Phrase("投诉人",fontChinese));
cell.setHorizontalAlignment(Element.ALIGN_CENTER); //水平居中
cell.setVerticalAlignment(Element.ALIGN_MIDDLE); //垂直居中
aTable.addCell(cell);
cell = new Cell(new Phrase(creator_name,fontChinese));
cell.setHorizontalAlignment(Element.ALIGN_CENTER);
aTable.addCell(cell);
cell = new Cell(new Phrase("联系电话",fontChinese));
cell.setHorizontalAlignment(Element.ALIGN_CENTER); //水平居中
cell.setVerticalAlignment(Element.ALIGN_MIDDLE); //垂直居中
aTable.addCell(cell);
cell = new Cell(new Phrase(creator_tel,fontChinese));
cell.setColspan(3);
cell.setHorizontalAlignment(Element.ALIGN_CENTER); //水平居中
cell.setVerticalAlignment(Element.ALIGN_MIDDLE); //垂直居中
aTable.addCell(cell);
//图片
cell = new Cell(new Phrase("图\n片\n证\n据",fontChinese));
cell.setHorizontalAlignment(Element.ALIGN_CENTER); //水平居中
cell.setVerticalAlignment(Element.ALIGN_MIDDLE); //垂直居中
cell.setRowspan(5);
aTable.addCell(cell);
cell = new Cell();
Image img = Image.getInstance(inspectPic); //核实图片
img.setAbsolutePosition(0, 0);
img.scaleAbsolute(180, 150);// 直接设定显示尺寸
//img.scalePercent(50);// 表示显示的大小为原尺寸的50%
//img.scalePercent(25, 50);// 图像高宽的显示比例
//img.setRotation(30);// 图像旋转一定角度
cell.add(img);
cell.setRowspan(5);
cell.setColspan(2);
aTable.addCell(cell);
cell = new Cell(new Phrase("图\n片\n证\n据",fontChinese));
cell.setHorizontalAlignment(Element.ALIGN_CENTER); //水平居中
cell.setVerticalAlignment(Element.ALIGN_MIDDLE); //垂直居中
cell.setRowspan(5);
aTable.addCell(cell);
cell = new Cell();
Image img2 = Image.getInstance(veriferPic); //核查图片
img2.setAbsolutePosition(0, 0);
img2.scaleAbsolute(12, 35);// 直接设定显示尺寸
img2.scalePercent(50);// 表示显示的大小为原尺寸的50%
img2.scalePercent(25, 50);// 图像高宽的显示比例
img2.setRotation(30);// 图像旋转一定角度
cell.add(img2);
cell.setRowspan(5);
cell.setColspan(2);
aTable.addCell(cell);
cell = new Cell(new Phrase("案件\n详细\n信息",fontChinese));
cell.setHorizontalAlignment(Element.ALIGN_CENTER); //水平居中
cell.setVerticalAlignment(Element.ALIGN_MIDDLE); //垂直居中
cell.setRowspan(6);
aTable.addCell(cell);
/* ---------------------------------------------------- */
cell = new Cell(new Phrase("所属路段",fontChinese));
cell.setHorizontalAlignment(Element.ALIGN_CENTER); //水平居中
cell.setVerticalAlignment(Element.ALIGN_MIDDLE); //垂直居中
aTable.addCell(cell);
cell = new Cell

迷失的蜗牛
- 粉丝: 24
- 资源: 5
最新资源
- 【岗位说明】酒店人事部岗位职责.doc
- 【岗位说明】酒店商务中心领班岗位职责.doc
- 【岗位说明】酒店商务中心主管岗位职责.doc
- 【岗位说明】酒店洗手间值班员岗位职责.doc
- 【岗位说明】酒店销售部文员岗位职责.doc
- 【岗位说明】酒店销售部主管岗位职责.doc
- 【岗位说明】酒店迎宾员岗位职责.doc
- 【岗位说明】酒店夜班主管岗位职责.doc
- 【岗位说明】酒店营销部经理岗位职责.doc
- 【岗位说明】酒店预订员岗位职责.doc
- 【岗位说明】酒店迎宾主管岗位职责.doc
- 【岗位说明】酒店运营部岗位职责.doc
- 【岗位说明】酒店值班经理岗位职责.doc
- 【岗位说明】酒水部经理岗位职责.doc
- 【岗位说明】酒水部领班岗位职责.doc
- 【岗位说明】专业酒店岗位职责.doc
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


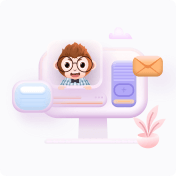