var __decorate = (this && this.__decorate) || function (decorators, target, key, desc) {
var c = arguments.length, r = c < 3 ? target : desc === null ? desc = Object.getOwnPropertyDescriptor(target, key) : desc, d;
if (typeof Reflect === "object" && typeof Reflect.decorate === "function") r = Reflect.decorate(decorators, target, key, desc);
else for (var i = decorators.length - 1; i >= 0; i--) if (d = decorators[i]) r = (c < 3 ? d(r) : c > 3 ? d(target, key, r) : d(target, key)) || r;
return c > 3 && r && Object.defineProperty(target, key, r), r;
};
var _a, _b;
import dayjs from 'dayjs';
import localeData from 'dayjs/plugin/localeData';
import config from '../common/config';
import { SuperComponent, wxComponent } from '../common/src/index';
import props from './props';
import dayjsLocaleMap from './locale/dayjs';
dayjs.extend(localeData);
dayjs.locale('zh-cn');
const defaultLocale = ((_a = dayjsLocaleMap[dayjs.locale()]) === null || _a === void 0 ? void 0 : _a.key) || ((_b = dayjsLocaleMap.default) === null || _b === void 0 ? void 0 : _b.key);
const { prefix } = config;
const name = `${prefix}-date-time-picker`;
var ModeItem;
(function (ModeItem) {
ModeItem["YEAR"] = "year";
ModeItem["MONTH"] = "month";
ModeItem["DATE"] = "date";
ModeItem["HOUR"] = "hour";
ModeItem["MINUTE"] = "minute";
ModeItem["SECOND"] = "second";
})(ModeItem || (ModeItem = {}));
const DATE_MODES = ['year', 'month', 'date'];
const TIME_MODES = ['hour', 'minute', 'second'];
const FULL_MODES = [...DATE_MODES, ...TIME_MODES];
const DEFAULT_MIN_DATE = dayjs('2000-01-01 00:00:00');
const DEFAULT_MAX_DATE = dayjs('2030-12-31 23:59:59');
let DateTimePicker = class DateTimePicker extends SuperComponent {
constructor() {
super(...arguments);
this.properties = props;
this.externalClasses = [`${prefix}-class`, `${prefix}-class-confirm`, `${prefix}-class-cancel`, `${prefix}-class-title`];
this.options = {
multipleSlots: true,
};
this.observers = {
'start, end, value': function () {
this.updateColumns();
},
customLocale(v) {
if (!v || !dayjsLocaleMap[v].key)
return;
this.setData({
locale: dayjsLocaleMap[v].i18n,
dayjsLocale: dayjsLocaleMap[v].key,
});
},
mode(m) {
const fullModes = this.getFullModeArray(m);
this.setData({
fullModes,
});
this.updateColumns();
},
};
this.date = null;
this.data = {
prefix,
classPrefix: name,
columns: [],
columnsValue: [],
fullModes: [],
locale: dayjsLocaleMap[defaultLocale].i18n,
dayjsLocale: dayjsLocaleMap[defaultLocale].key,
};
this.controlledProps = [
{
key: 'value',
event: 'change',
},
];
this.methods = {
updateColumns() {
this.date = this.getParseDate();
const { columns, columnsValue } = this.getValueCols();
this.setData({
columns,
columnsValue,
});
},
getParseDate() {
const { value, defaultValue } = this.properties;
const minDate = this.getMinDate();
const isTimeMode = this.isTimeMode();
let currentValue = value || defaultValue;
if (isTimeMode) {
const dateStr = dayjs(minDate).format('YYYY-MM-DD');
currentValue = dayjs(`${dateStr} ${currentValue}`);
}
const parseDate = dayjs(currentValue || minDate);
const isDateValid = parseDate.isValid();
return isDateValid ? parseDate : minDate;
},
getMinDate() {
const { start } = this.properties;
return start ? dayjs(start) : DEFAULT_MIN_DATE;
},
getMaxDate() {
const { end } = this.properties;
return end ? dayjs(end) : DEFAULT_MAX_DATE;
},
getDateRect(type = 'default') {
const map = {
min: 'getMinDate',
max: 'getMaxDate',
default: 'getDate',
};
const date = this[map[type]]();
const keys = ['year', 'month', 'date', 'hour', 'minute', 'second'];
return keys.map((k) => { var _a; return (_a = date[k]) === null || _a === void 0 ? void 0 : _a.call(date); });
},
getDate() {
return this.clipDate((this === null || this === void 0 ? void 0 : this.date) || DEFAULT_MIN_DATE);
},
clipDate(date) {
const minDate = this.getMinDate();
const maxDate = this.getMaxDate();
return dayjs(Math.min(Math.max(minDate.valueOf(), date.valueOf()), maxDate.valueOf()));
},
setYear(date, year) {
const beforeMonthDays = date.date();
const afterMonthDays = date.year(year).daysInMonth();
const tempDate = date.date(Math.min(beforeMonthDays.valueOf(), afterMonthDays.valueOf()));
return tempDate.year(year);
},
setMonth(date, month) {
const beforeMonthDays = date.date();
const afterMonthDays = date.month(month).daysInMonth();
const tempDate = date.date(Math.min(beforeMonthDays.valueOf(), afterMonthDays.valueOf()));
return tempDate.month(month);
},
getColumnOptions() {
const { fullModes } = this.data;
const columnOptions = [];
fullModes === null || fullModes === void 0 ? void 0 : fullModes.forEach((mode) => {
const columnOption = this.getOptionByType(mode);
columnOptions.push(columnOption);
});
return columnOptions;
},
getOptionByType(type) {
var _a;
const { locale, steps } = this.data;
const options = [];
const minEdge = this.getOptionEdge('min', type);
const maxEdge = this.getOptionEdge('max', type);
const step = (_a = steps === null || steps === void 0 ? void 0 : steps[type]) !== null && _a !== void 0 ? _a : 1;
const dayjsMonthsShort = dayjs().locale(this.data.dayjsLocale).localeData().monthsShort();
for (let i = minEdge; i <= maxEdge; i += step) {
options.push({
value: `${i}`,
label: type === 'month' ? dayjsMonthsShort[i] : `${i + locale[type]}`,
});
}
return options;
},
getYearOptions(dateParams) {
const { locale } = this.data;
const { minDateYear, maxDateYear } = dateParams;
const years = [];
for (let i = minDateYear; i <= maxDateYear; i += 1) {
years.push({
value: `${i}`,
label: `${i + locale.year}`,
});
}
return years;
},
getOptionEdge(minOrMax, type) {
const selDateArray = this.getDateRect();
const compareArray = this.getDateRect(minOrMax);
const edge = {
month: [0, 11],
date: [1, this.getDate().daysInMonth()],
hour: [0, 23],
minute: [0, 59],
没有合适的资源?快使用搜索试试~ 我知道了~
基于微信小程序的医院陪诊预约系统设计源码
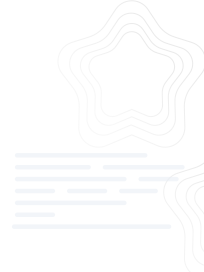
共944个文件
js:293个
ts:267个
wxss:100个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 141 浏览量
2025-01-03
01:15:17
上传
评论
收藏 1.94MB ZIP 举报
温馨提示
本项目是一款基于微信小程序平台的医院陪诊预约系统设计源码,共包含991个文件,涵盖293个JavaScript文件、267个TypeScript文件、114个Markdown文件、95个JSON配置文件、95个WXML模板文件、90个WXSS样式文件、24个WXS组件文件、9个PNG图片文件、2个JPG图片文件、1个Git忽略配置文件。系统采用JavaScript和TypeScript进行开发,旨在为用户提供便捷的医院陪诊预约服务。
资源推荐
资源详情
资源评论
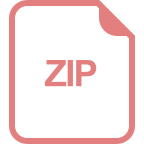
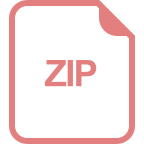
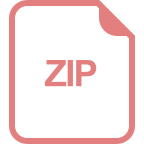
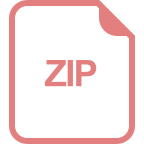
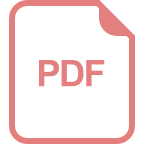
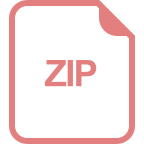
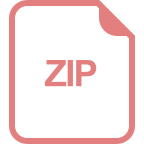
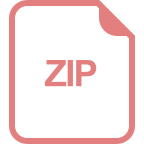
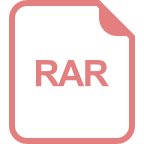
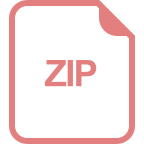
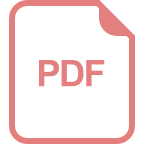
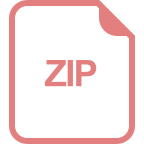
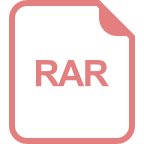
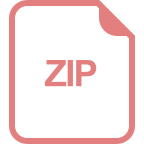
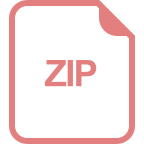
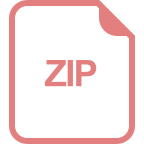
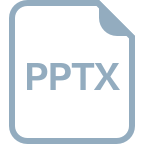
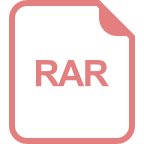
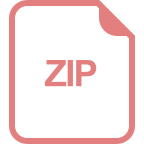
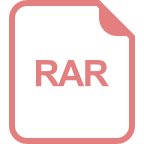
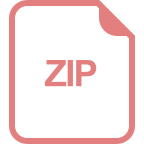
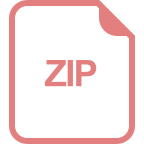
收起资源包目录

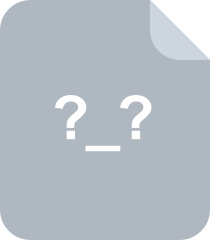
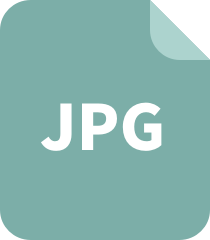
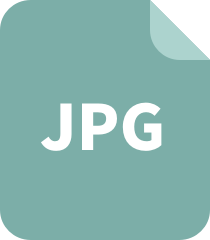
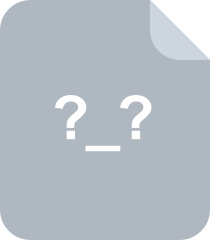
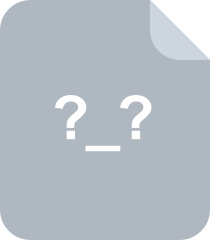
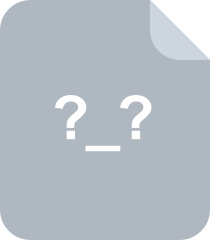
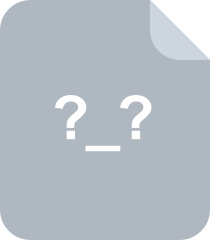
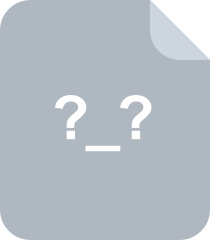
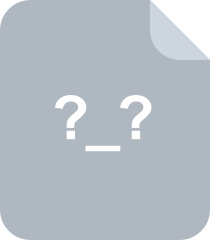
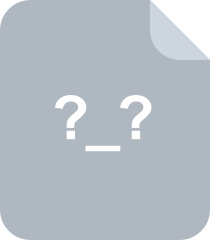
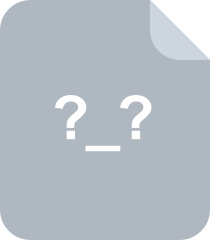
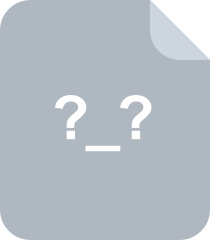
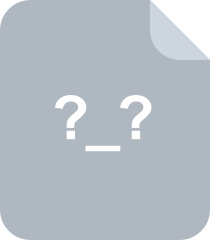
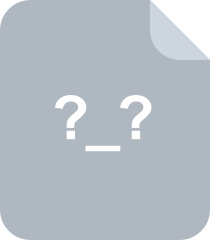
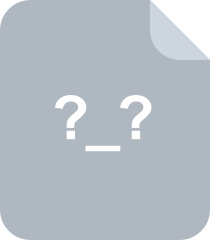
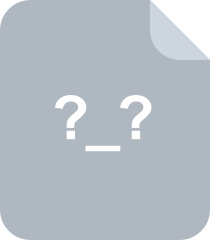
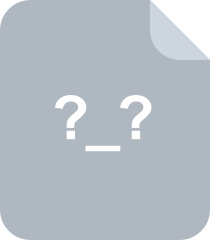
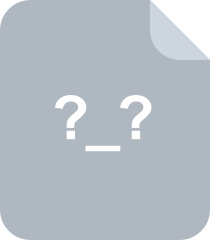
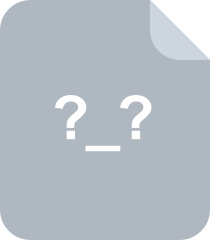
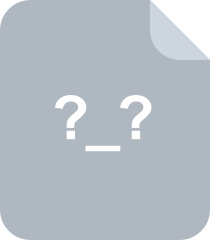
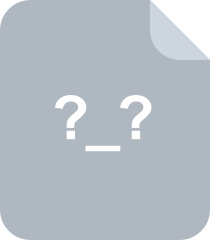
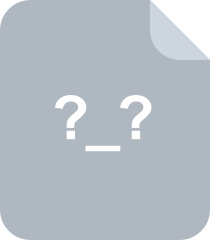
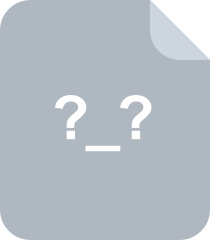
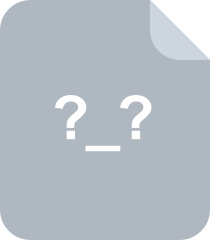
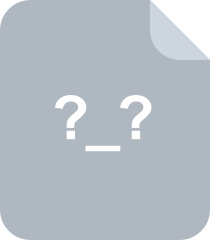
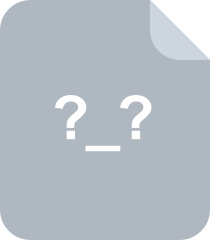
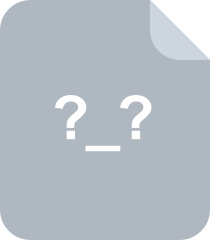
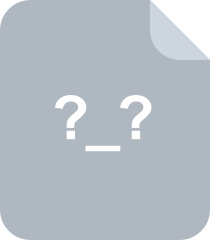
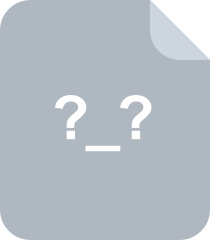
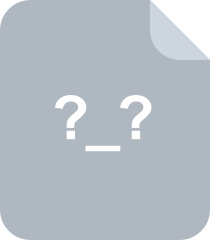
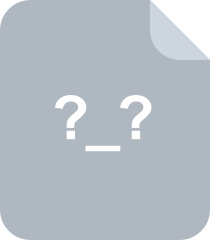
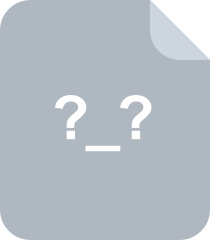
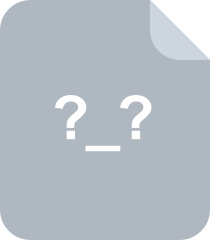
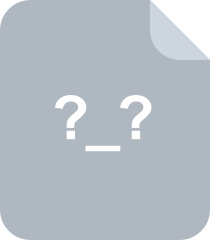
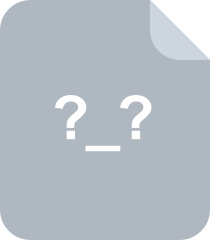
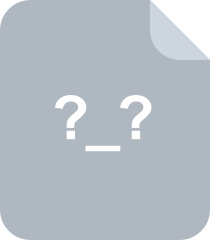
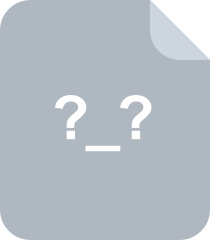
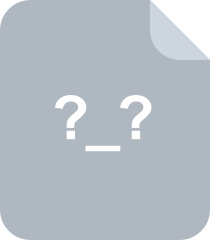
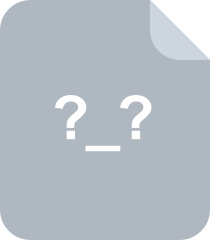
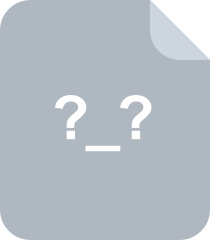
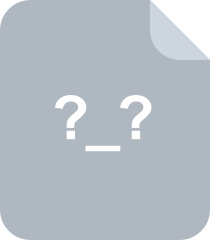
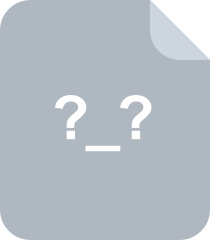
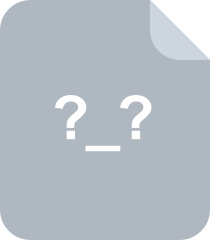
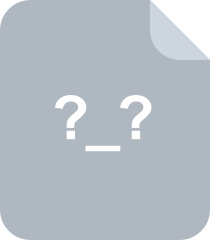
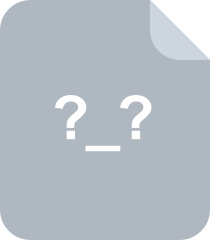
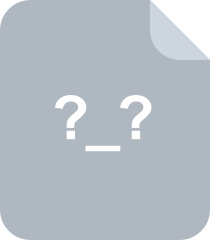
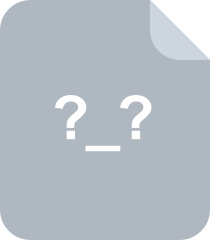
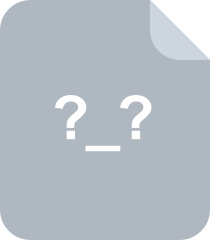
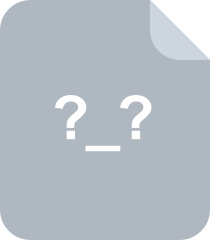
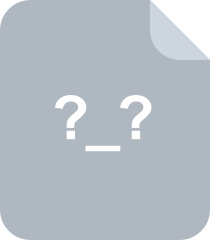
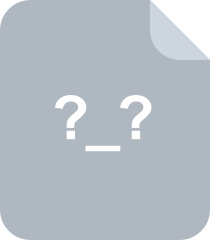
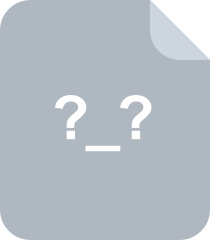
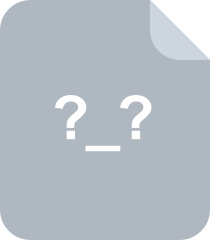
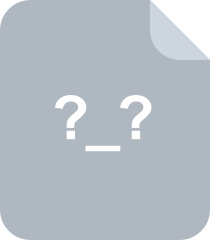
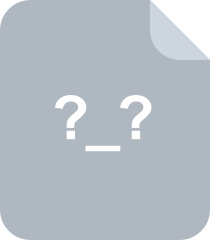
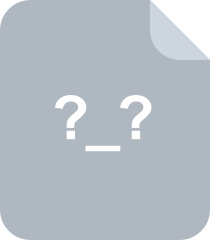
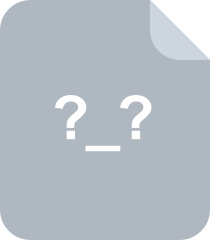
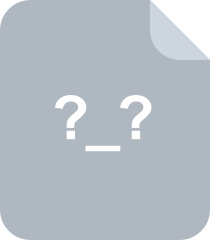
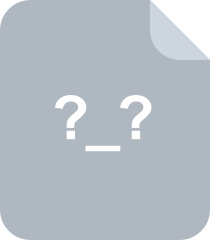
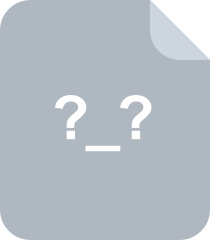
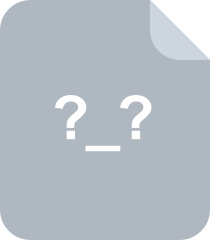
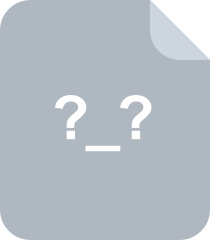
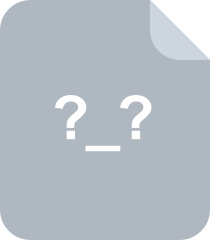
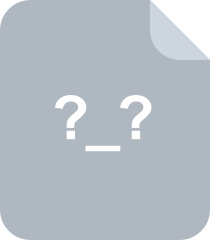
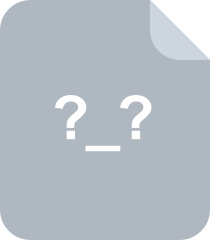
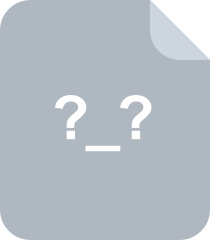
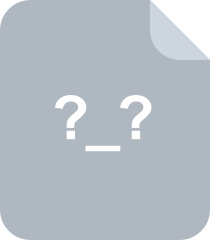
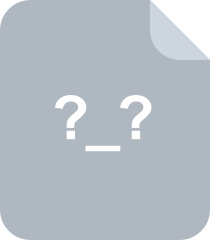
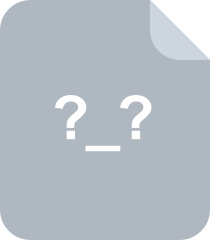
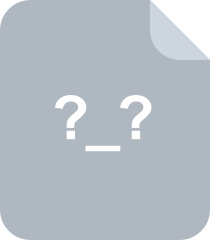
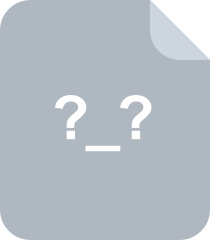
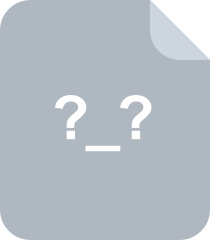
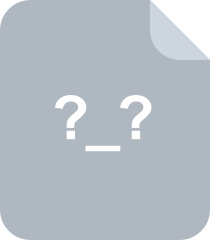
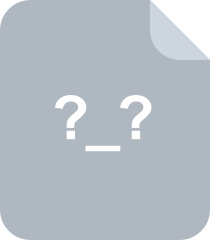
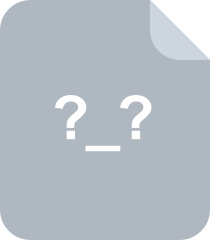
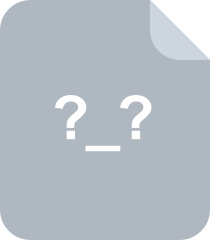
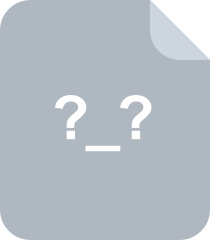
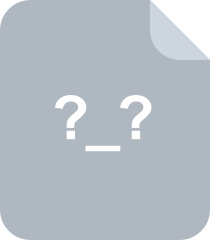
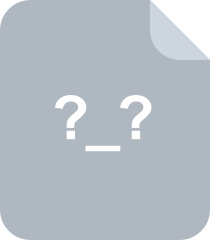
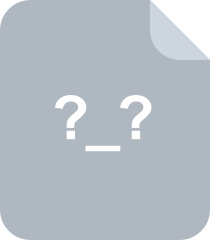
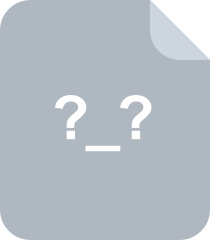
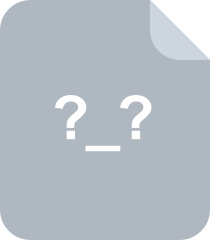
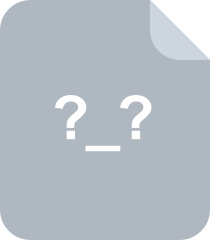
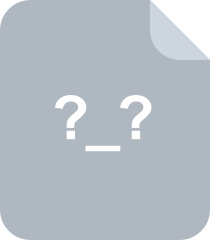
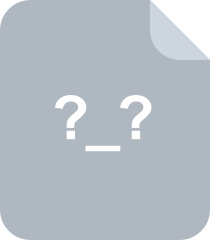
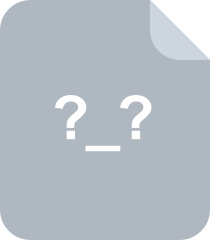
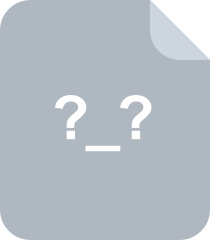
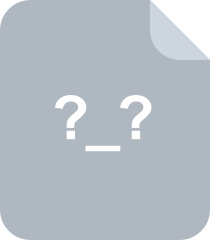
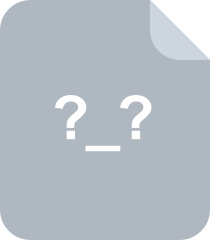
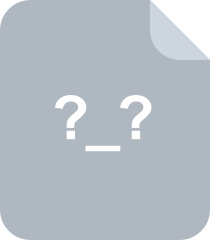
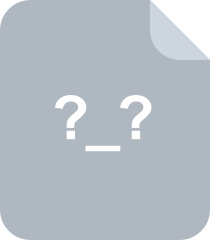
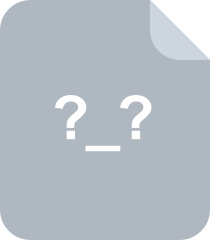
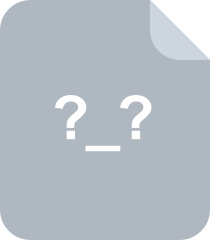
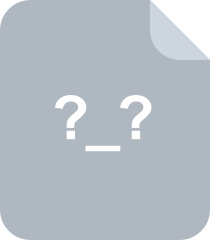
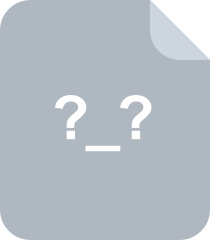
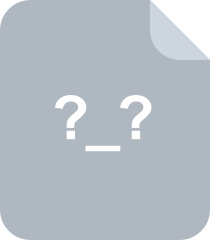
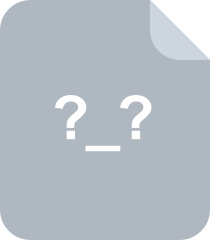
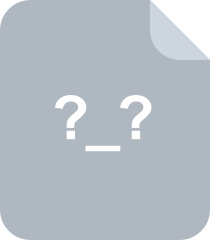
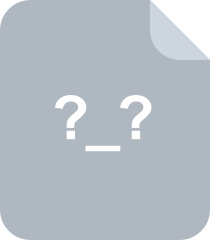
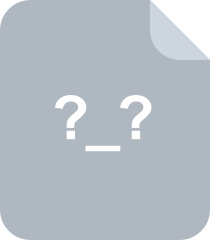
共 944 条
- 1
- 2
- 3
- 4
- 5
- 6
- 10
资源评论
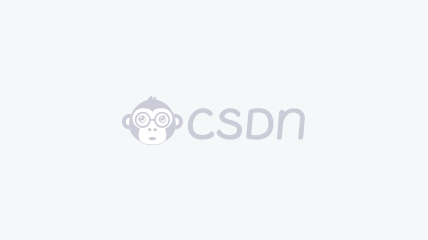

xyq2024
- 粉丝: 2929
- 资源: 5563
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

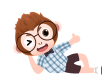
最新资源
- 小霸王游戏机珍藏84合1
- 医疗试管挑样机_x_t全套技术资料100%好用.zip
- Matlab实现TTAO-CNN-BiLSTM-Mutilhead-Attention三角拓扑聚合优化卷积双向长短期记忆神经网络融合多头注意力机制多特征分类预测(含完整的程序,GUI设计和代码详解)
- 移载转板机械手step全套技术资料100%好用.zip
- pll电荷泵锁相环 cppll(已流片)仿真环境搭建好了 电路到版图都已流片验证,另外送PLL书籍电子版和对应工艺库 另加50就可以得到完整版图 三阶二型锁相环 参考频率50-100MHz 分频比可
- Matlab实现ABC-BP人工蜂群算法优化BP神经网络多变量回归预测(含完整的程序,GUI设计和代码详解)
- 大一课设-C语言链表火车票务管理系统开源
- 在线视觉点胶和自动贴合x_t全套技术资料100%好用.zip
- commons-compress-1.21.jar
- 粒子群算法在MPPT中的仿真,还有温度改变的情况,最基础的粒子群在MPPT中的应用,可用于参考学习
- 重型高速电梯安全钳sw19可编辑全套技术资料100%好用.zip
- 1-正整数的频率表.m
- 2-经验累积分布函数图形.m
- 3-绘制正态分布概率图形.m
- 4-样本数据的盒图.m
- 5-增加参考线图形.m
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


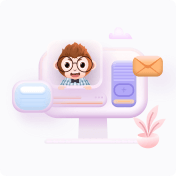
安全验证
文档复制为VIP权益,开通VIP直接复制
