#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#include <string.h>
#include <string>
#include <algorithm>
#include "utils.h"
////////////////////////////////////////////////////////////////////////////////
/// string utils
////////////////////////////////////////////////////////////////////////////////
inline int char_is_delims(char c, char d)
{
if( c == d )
return 1;
else
return 0;
}
StringArray split_text(const std::string &intext, const std::string &delims)
{
StringArray r;
int st;
int n, nd, i, j, k, dd;
char *buf;
const char *pb, *pd;
n = intext.size();
nd = delims.size();
pb = intext.c_str();
pd = delims.c_str();
buf = new char[n+10];
st = 0;
i = 0;
k = 0;
buf[0] = 0;
while( i<n ) {
for(dd = 0, j=0; j<nd; j++) dd += char_is_delims(pb[i], pd[j]);
if( dd > 0 ) {
buf[k] = 0;
r.push_back(buf);
k = 0;
st = 1;
} else {
buf[k++] = pb[i];
st = 0;
}
i++;
}
// process last character
if( st == 0 ) {
buf[k] = 0;
r.push_back(buf);
} else {
buf[0] = 0;
r.push_back(buf);
}
delete [] buf;
return r;
}
std::string join_text(const StringArray& sa, const std::string& delims)
{
std::string s;
for(int i=0; i<sa.size(); i++) {
if( i == 0 ) s = sa[i];
else s = s + delims + sa[i];
}
return s;
}
std::string& str_toupper(std::string &s)
{
for(size_t i=0; i < s.size(); i++) {
s[i] = toupper(s[i]);
}
return s;
}
std::string& str_tolower(std::string &s)
{
for(size_t i=0; i < s.size(); i++) {
s[i] = tolower(s[i]);
}
return s;
}
std::string trim(const std::string &s)
{
std::string delims = " \t\n\r",
r;
std::string::size_type i, j;
i = s.find_first_not_of(delims);
j = s.find_last_not_of(delims);
if( i == std::string::npos ) {
r = "";
return r;
}
if( j == std::string::npos ) {
r = "";
return r;
}
r = s.substr(i, j-i+1);
return r;
}
std::string& trim2(std::string& s)
{
if (s.empty()) {
return s;
}
std::string& r = s;
std::string::iterator c;
// Erase whitespace before the string
for (c = r.begin(); c != r.end() && isspace(*c++););
r.erase(r.begin(), --c);
// Erase whitespace after the string
for (c = r.end(); c != r.begin() && isspace(*--c););
r.erase(++c, r.end());
return r;
}
// string trim functions
std::string ltrim(const std::string &s)
{
std::string delims = " \t\n\r",
r;
std::string::size_type i;
i = s.find_first_not_of(delims);
if( i == std::string::npos )
r = "";
else
r = s.substr(i, s.size() - i);
return r;
}
std::string rtrim(const std::string &s)
{
std::string delims = " \t\n\r",
r;
std::string::size_type i;
i = s.find_last_not_of(delims);
if( i == std::string::npos )
r = "";
else
r = s.substr(0, i+1);
return r;
}
int str_to_int(const std::string &s)
{
return atoi(s.c_str());
}
float str_to_float(const std::string &s)
{
return atof(s.c_str());
}
double str_to_double(const std::string &s)
{
return atof(s.c_str());
}
////////////////////////////////////////////////////////////////////////////////
/// time utils
////////////////////////////////////////////////////////////////////////////////
int64_t time_utc(struct tm *tm);
#ifdef _WIN32 // WIN
#include <windows.h>
#include <io.h>
#include <time.h>
#include <ctype.h>
#include <sys/types.h>
#include <sys/stat.h>
uint64_t tm_get_millis(void)
{
return GetTickCount();
}
uint64_t tm_get_ms(void)
{
return GetTickCount();
}
uint64_t tm_get_us(void)
{
FILETIME t;
uint64_t t_ret;
// get UTC time
GetSystemTimeAsFileTime(&t);
t_ret = 0;
t_ret |= t.dwHighDateTime;
t_ret <<= 32;
t_ret |= t.dwLowDateTime;
// convert 100 ns to [ms]
return t_ret/10;
}
double tm_getTimeStamp(void)
{
FILETIME t;
uint64_t t_ret;
double ts;
// get UTC time
GetSystemTimeAsFileTime(&t);
t_ret = 0;
t_ret |= t.dwHighDateTime;
t_ret <<= 32;
t_ret |= t.dwLowDateTime;
// convert 100 ns to second
ts = 1.0 * t_ret / 1e7;
return ts;
}
uint32_t tm_getTimeStampUnix(void)
{
FILETIME t;
uint64_t t_ret;
uint32_t ts;
// get UTC time
GetSystemTimeAsFileTime(&t);
t_ret = 0;
t_ret |= t.dwHighDateTime;
t_ret <<= 32;
t_ret |= t.dwLowDateTime;
// convert 100 ns to second
ts = t_ret / 10000000;
return ts;
}
void tm_sleep(uint32_t t)
{
Sleep(t);
}
void tm_sleep_us(uint64_t t)
{
HANDLE timer;
LARGE_INTEGER ft;
// Convert to 100 nanosecond interval, negative value indicates relative time
ft.QuadPart = -(10*t);
timer = CreateWaitableTimer(NULL, TRUE, NULL);
SetWaitableTimer(timer, &ft, 0, NULL, NULL, 0);
WaitForSingleObject(timer, INFINITE);
CloseHandle(timer);
}
const char * strp_weekdays[] =
{ "sunday", "monday", "tuesday", "wednesday", "thursday", "friday", "saturday"};
const char * strp_monthnames[] =
{ "january", "february", "march", "april", "may", "june", "july", "august", "september", "october", "november", "december"};
bool strp_atoi(const char * & s, int & result, int low, int high, int offset)
{
bool worked = false;
char * end;
unsigned long num = strtoul(s, & end, 10);
if (num >= (unsigned long)low && num <= (unsigned long)high)
{
result = (int)(num + offset);
s = end;
worked = true;
}
return worked;
}
char * strptime(const char *s, const char *format, struct tm *tm)
{
bool working = true;
while (working && *format && *s)
{
switch (*format)
{
case '%':
{
++format;
switch (*format)
{
case 'a':
case 'A': // weekday name
tm->tm_wday = -1;
working = false;
for (size_t i = 0; i < 7; ++ i)
{
size_t len = strlen(strp_weekdays[i]);
if (!strnicmp(strp_weekdays[i], s, len))
{
tm->tm_wday = i;
s += len;
working = true;
break;
}
else if (!strnicmp(strp_weekdays[i], s, 3))
{
tm->tm_wday = i;
s += 3;
working = true;
break;
}
}
break;
case 'b':
case 'B':
case 'h': // month name
tm->tm_mon = -1;
working = false;
for (size_t i = 0; i < 12; ++ i)
{
size_t len = strlen(strp_monthnames[i]);
if (!strnicmp(strp_monthnames[i], s, len))
{
tm->tm_mon = i;
s += len;
working = true;
break;
}
else if (!strnicmp(strp_monthnames[i], s, 3))
{
tm->tm_mon = i;
s += 3;
working = true;
break;
}
}
break;
case 'd':
case 'e': // day of month number
working = strp_atoi(s, tm->tm_mday, 1,
没有合适的资源?快使用搜索试试~ 我知道了~
基于C语言的简易地面控制站SimpGCS设计源码
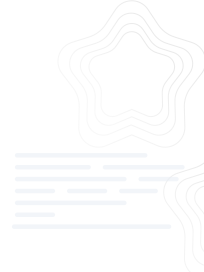
共886个文件
h:745个
cpp:62个
png:20个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 90 浏览量
2025-01-03
00:08:49
上传
评论
收藏 23.08MB ZIP 举报
温馨提示
该项目是一款名为SimpGCS的简易地面控制站设计源码,主要使用C语言编写,并涉及C++和C++11的扩展。整个项目包含886个文件,其中包括745个头文件(h),62个C++源文件(cpp),20个PNG图像文件(png),18个XML配置文件(xml),10个CMake构建脚本文件(cmake),5个SVG矢量图形文件(svg),2个Markdown文件(md),2个项目构建文件(pro),2个库文件(lib)和2个JPEG图像文件(jpg)。SimpGCS是一个基于C语言的地面控制站解决方案,适用于无人机和其他航空器的地面控制需求。
资源推荐
资源详情
资源评论
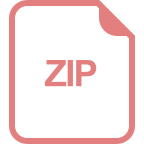
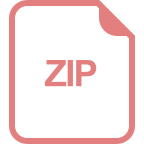
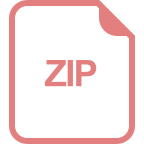
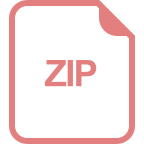
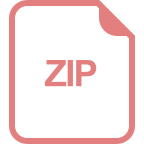
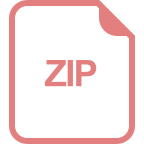
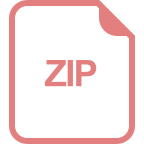
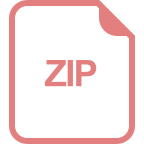
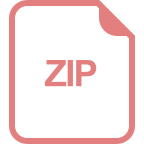
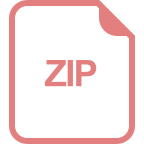
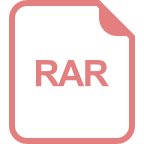
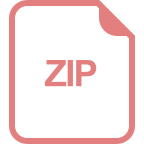
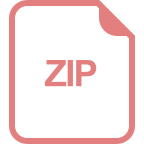
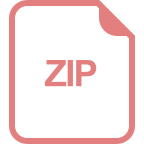
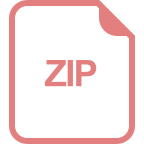
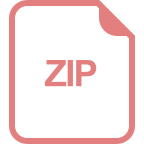
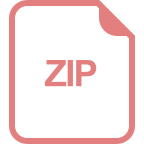
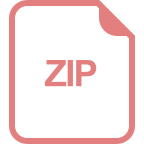
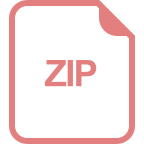
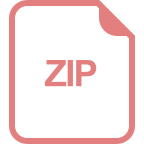
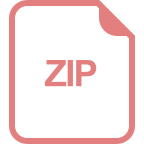
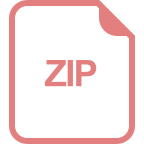
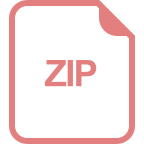
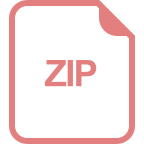
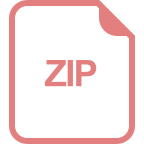
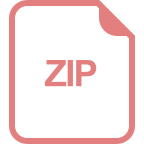
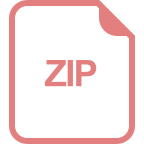
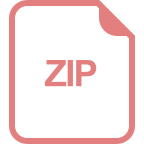
收起资源包目录

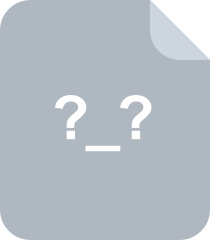
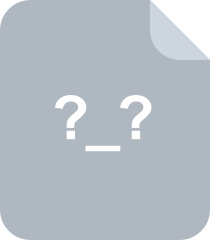
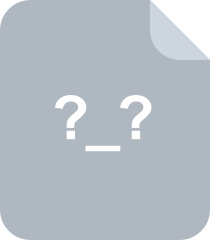
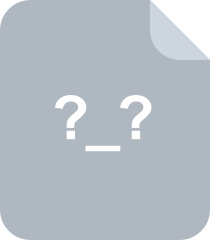
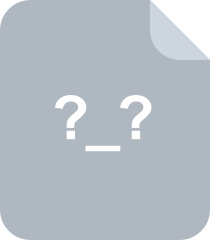
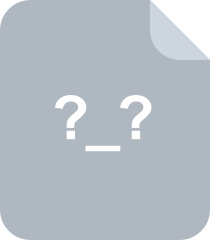
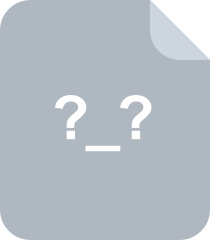
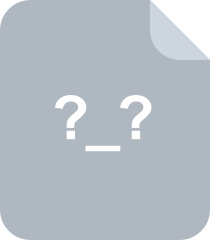
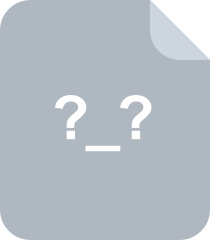
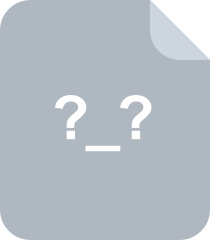
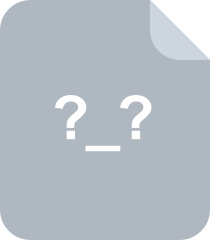
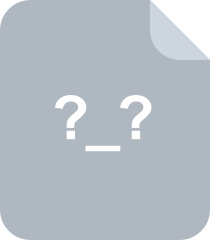
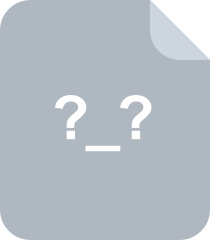
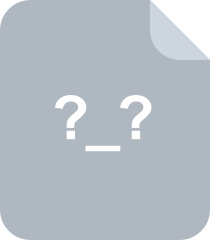
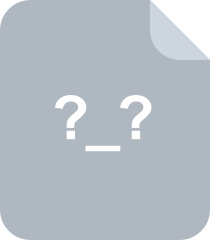
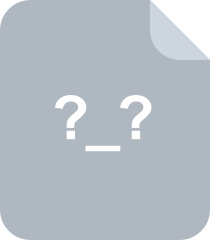
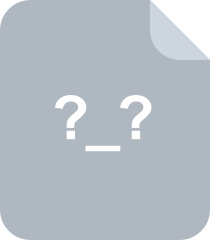
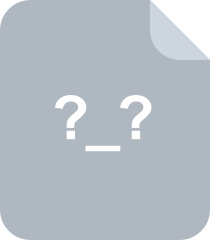
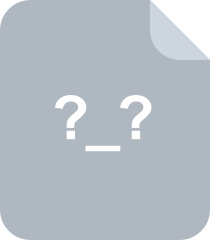
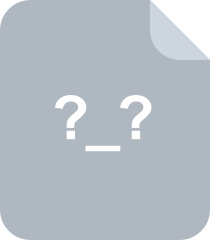
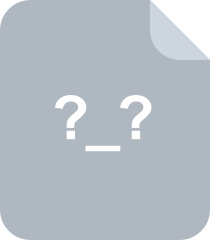
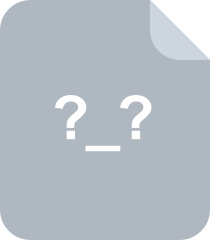
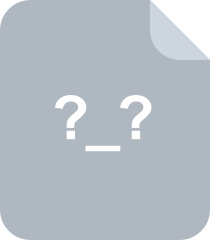
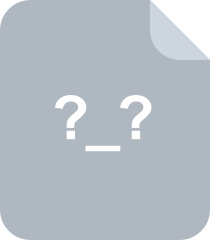
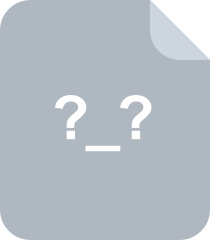
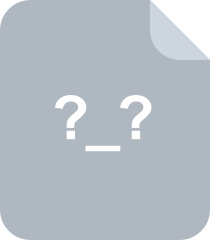
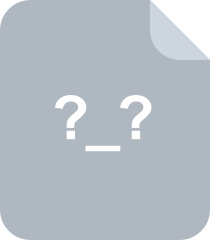
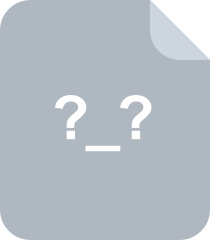
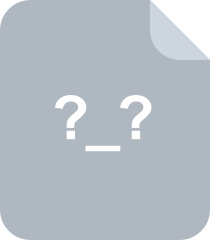
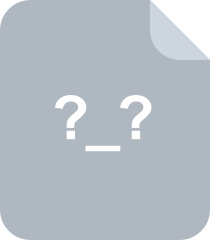
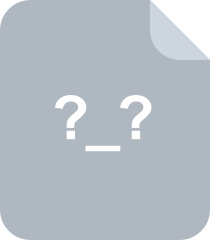
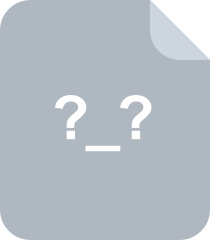
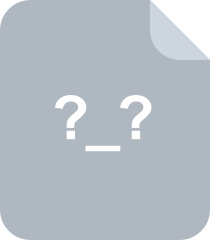
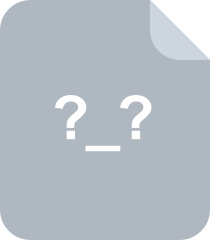
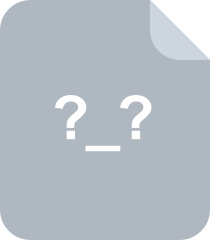
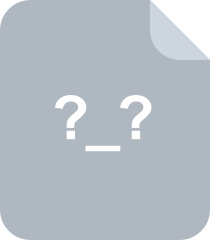
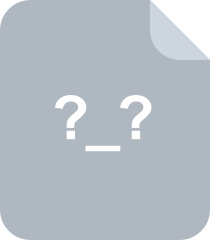
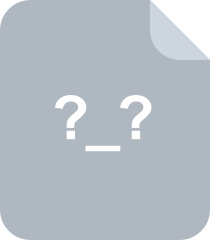
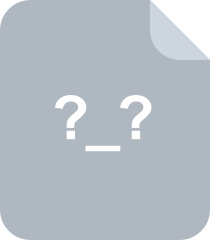
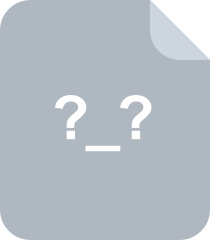
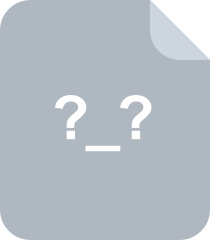
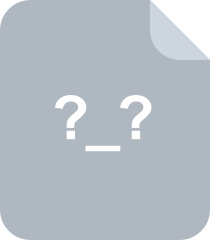
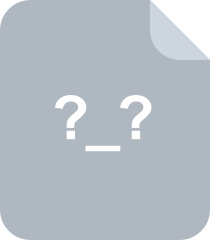
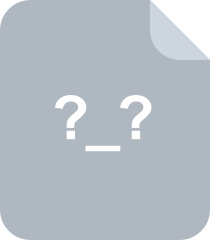
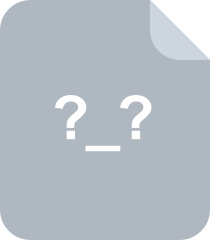
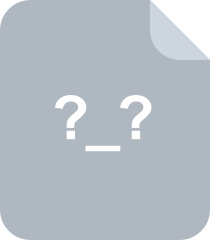
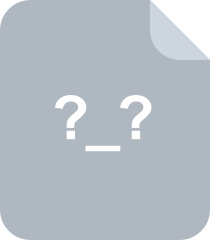
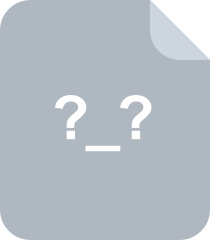
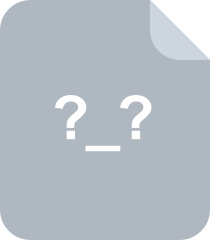
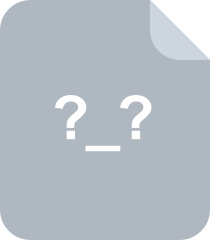
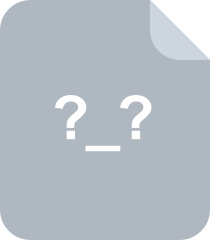
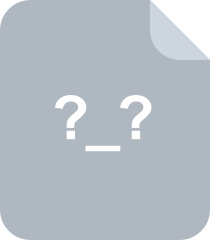
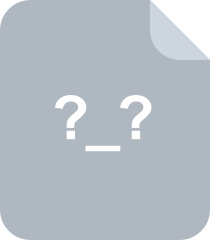
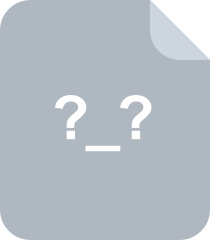
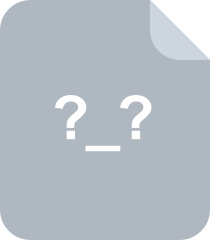
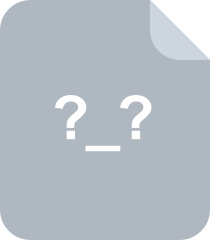
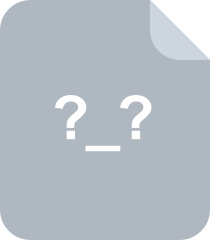
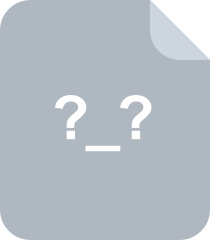
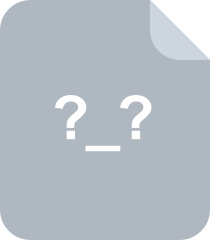
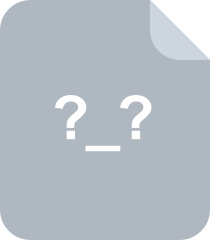
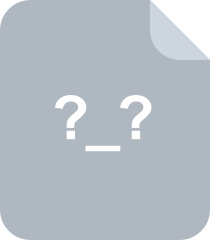
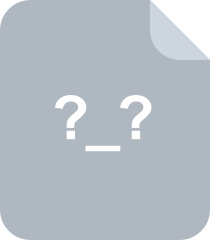
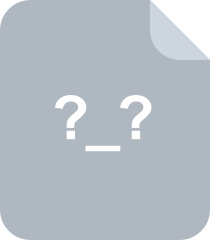
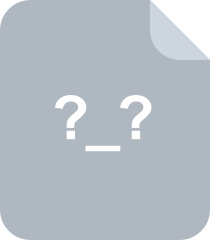
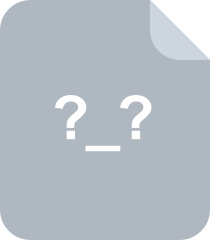
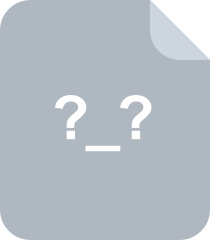
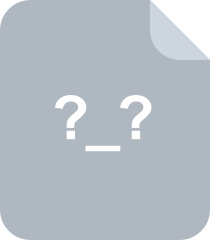
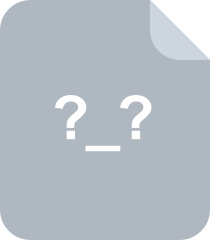
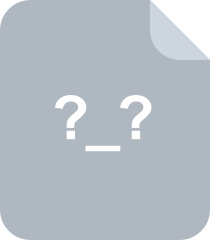
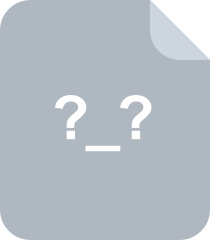
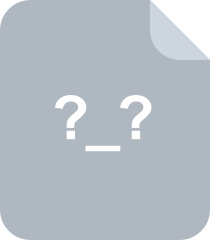
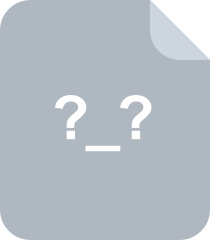
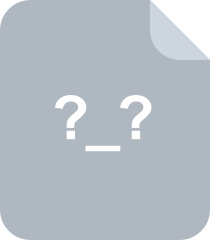
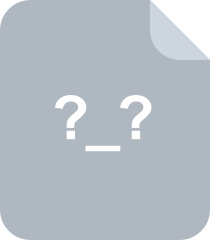
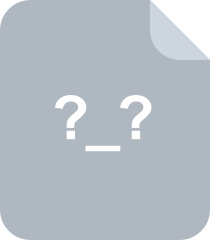
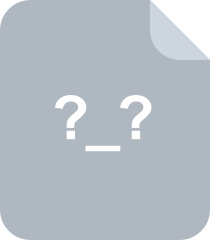
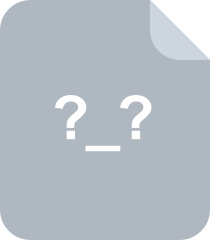
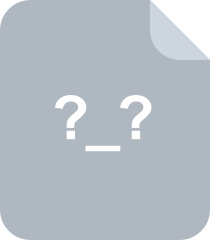
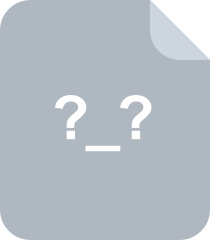
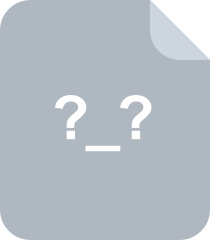
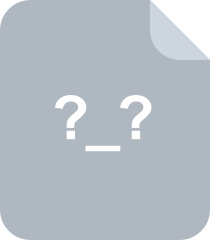
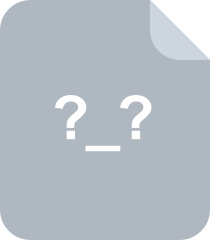
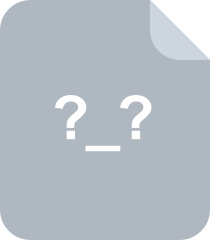
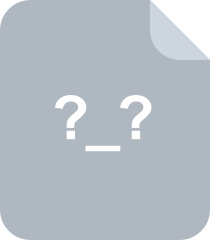
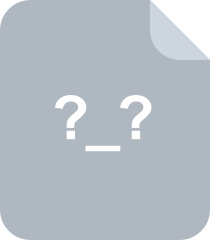
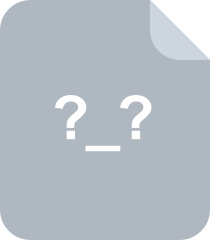
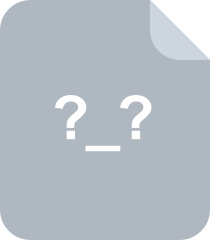
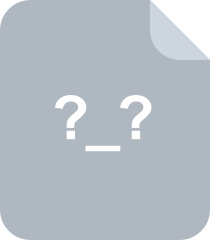
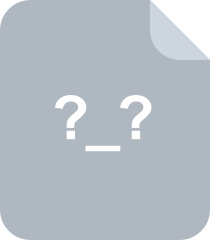
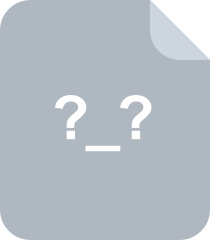
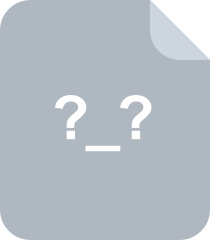
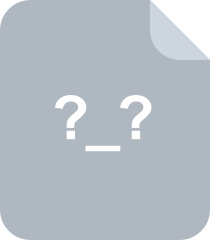
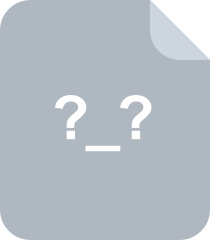
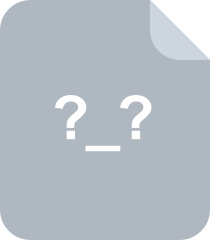
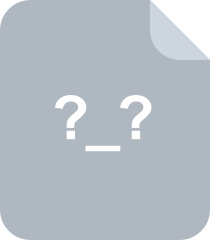
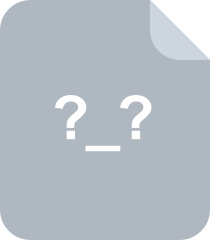
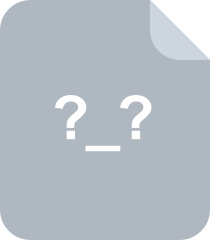
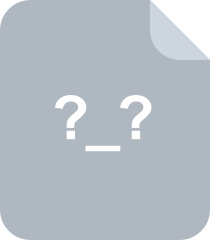
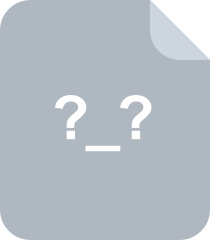
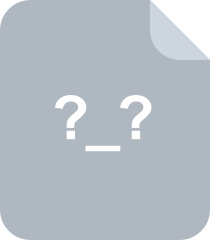
共 886 条
- 1
- 2
- 3
- 4
- 5
- 6
- 9
资源评论
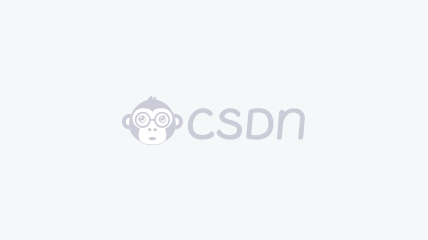

xyq2024
- 粉丝: 2929
- 资源: 5563
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

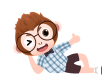
最新资源
- 马歇尔击实仪sw20可编辑全套技术资料100%好用.zip
- 轮辋压力机step全套技术资料100%好用.zip
- 门板边挡板分离喂料机sw19全套技术资料100%好用.zip
- 关于一个线性表示代码,y=wx+b,w是一个n行四列的矩阵,x是一个4行1列的向量 这段代码实现了一个简单的线性回归模型
- 一个简单的Python爬虫示例,使用了requests库来发送HTTP请求,以及BeautifulSoup库来解析HTML页面 这个示例将从一个简单的网页中获取标题并打印出来
- arcgis矢量shp格式遵义县地图
- arcgis矢量shp格式淄博市地图
- 门式夹持器起重机sw21全套技术资料100%好用.zip
- arcgis矢量shp格式涿州地图
- 很多事卡级号大卡司机会大手机卡等哈手机卡很大刷卡机出
- arcgis矢量shp格式重庆地图
- 高频注入仿真pmsm 无感控制 解决0速转矩输出问题 插入式永磁同步电机,凸极,高频注入 MATLAB simulink仿真,供研究学习
- 门板加强筋封头自动放料工作站sw19可编辑全套技术资料100%好用.zip
- arcgis矢量shp格式中山全市地图
- Cisco-300-710.pdf
- Windows自动更新禁用/恢复工具(Win10/Win11/WinServer2016/WinServer2022/WinServer2025)
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


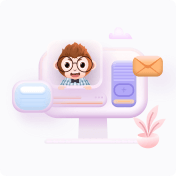
安全验证
文档复制为VIP权益,开通VIP直接复制
