package com.example.secondhandsysapi.Controller;
import com.example.secondhandsysapi.Entity.GoodsInfo;
import com.example.secondhandsysapi.Service.GoodsInfoService;
import com.example.secondhandsysapi.Vo.CategoryGoodsInfo;
import com.example.secondhandsysapi.Vo.GoodsPostInfo;
import com.example.secondhandsysapi.Vo.MultGoods;
import com.example.secondhandsysapi.util.FileUtil;
import com.github.pagehelper.PageInfo;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.format.annotation.DateTimeFormat;
import org.springframework.web.bind.annotation.*;
import org.springframework.web.multipart.MultipartFile;
import javax.persistence.criteria.CriteriaBuilder;
import java.sql.Date;
import java.time.LocalDate;
import java.util.List;
@CrossOrigin
@RestController
@RequestMapping("goods")
public class GoodsInfoController {
@Autowired
private GoodsInfoService goodsInfoService;
//首页的潮玩推荐,随机取八个商品
@GetMapping("/index")
public List<GoodsInfo> getRandomGoodsInfo() {
return goodsInfoService.getRandomGoodsInfo();
}
//我已发布的商品
@GetMapping("/published")
public List<GoodsPostInfo> getPublishedGoodsInfo(@RequestParam Integer user_id) {
return goodsInfoService.getPublicProduct(user_id);
}
@GetMapping("/query")
public List<GoodsInfo> queryGoodsInfoByName(@RequestParam String name){
return goodsInfoService.getAllGoodsInfo(name);
}
@GetMapping("/productdetail")
public List<GoodsInfo> getProductDetail(@RequestParam Integer id) {
return goodsInfoService.getProductDetailById(id);
}
//更新已发布的商品信息
@PostMapping("/updateproduct")
public Integer updateProductInfo(@RequestBody GoodsInfo goodsInfo) {
return goodsInfoService.updateProductInfo(goodsInfo);
}
//删除已发布的商品信息
@GetMapping("/delproduct/{goodsid}")
public Integer delProduct(@PathVariable Integer goodsid) {
return goodsInfoService.deleteById(goodsid);
}
@GetMapping("/queryDetails")
public List<GoodsInfo> queryGoodsDetailsByGoodsId(@RequestParam(required = false) Integer goods_id) {
return goodsInfoService.getAllGoodsDetailsInfoByGoodsId(goods_id);
}
@GetMapping("/backendallgoods")
public List<GoodsInfo> getBackendAllGoods() {
return goodsInfoService.getAllBackendGoodsInfo();
}
@GetMapping("querygoods")
public List<GoodsInfo> queryGoodsInfoByPrice(@RequestParam(required = false) String name,@RequestParam(required = false) Integer price){
List<GoodsInfo> goodsPri = null;
if(name != null && price != null){
// 公告类型和主题名称都不为空时,根据类型和主题名查公告信息
goodsPri = goodsInfoService.getAllGoodsInfoByPriceAndName(name,price);
} else if(price != null) {
// 当类型不为空时,根据类型查公告信息
goodsPri = goodsInfoService.getAllGoodsInfoByPrice(price);
} else if(name != null) {
// 当主题名不为空时,根据主题名查公告信息
goodsPri = goodsInfoService.getAllGoodsInfo(name);
} else {
// 当主题名和类型都为空时,返回所有公告信息
goodsPri = goodsInfoService.getAllBackendGoodsInfo();
}
return goodsPri;
}
@GetMapping("del/{goodsid}")
private Integer delGoodsInfo(@PathVariable Integer goodsid) {
return goodsInfoService.deleteById(goodsid);
}
// http://localhost:8080/goods/checkgoods
@GetMapping("checkgoods")
public List<GoodsInfo> checkGoodsByStatus(@RequestParam(required = false) Integer user_id, @RequestParam(required = false)String goods_name){
List<GoodsInfo> result;
if ((user_id == null || user_id==0) && (goods_name == null || goods_name.isEmpty())) { // 全为空
result = goodsInfoService.getAllGoodsInfo1();
} else if (user_id == null || user_id==0) { // user_name不为空,user_id为空
result = goodsInfoService.getAllGoodsInfoByName(goods_name);
} else if (goods_name == null || goods_name.isEmpty()) { // user_name为空,user_id不为空
result = goodsInfoService.getAllGoodsInfoByID(user_id);
} else { // 均不为空
result = goodsInfoService.getAllUpdateGoodsInfo(user_id, goods_name);
}
return result;
}
// http://localhost:8080/goods/page
@GetMapping("page")
public PageInfo<GoodsInfo> getGoodsInfo(@RequestParam Integer page, @RequestParam Integer size){
return goodsInfoService.getGoodsInfo(page,size);
}
// http://localhost:8080/goods/updateStatus/
@PostMapping("updateStatus/{goods_id}")
public Integer updateStatus(@PathVariable Integer goods_id){
Integer i= goodsInfoService.updateStatus(goods_id);
return 1;
}
// http://localhost:8080/goods/updateMutlStatus
@PostMapping("updateMutlStatus")
public Integer updateMutlStatus(@RequestBody MultGoods multGoods){
Integer num = 0;
for (Integer id : multGoods.getGoods_id()) {
goodsInfoService.updateStatus(id);
num = num + 1;
}
return num;
}
//http:localhost:8080/goods/updateMultCheck_info
//多选的时候,按照给来的list做遍历,一个一个的做拆分出来执行updateCheck_Info方法
@PostMapping("updateMultCheck_info")
public Integer updateMultCheck_info(@RequestBody MultGoods multGoods){
Integer num = 0;
for (String info: multGoods.getChecks_info()) {
for (Integer id : multGoods.getGoods_id()) {
goodsInfoService.updateCheck_Info(info, id);
num = num + 1;
}
}
return num;
}
//单次的修改拒绝备注
// http://localhost:8080/goods/updateCheck_info
@PostMapping("updateCheck_info")
public Integer updateCheckInfo( String check_info,Integer goods_id){
Integer i =goodsInfoService.updateCheck_Info(check_info,goods_id);
return i;
}
//http://localhost:8080/goods/post
@PostMapping("/post")
public Integer insertGoodsInfo(@RequestBody GoodsPostInfo goodsPostInfo){
Integer i =goodsInfoService.insertGoodsInfo(goodsPostInfo);
return i;
}
//http://localhost:8080/goods/upload
@PostMapping("/upload")
public String getImageFileName(MultipartFile file) {
String oldFileName = file.getOriginalFilename();
System.out.println(oldFileName);
String typeName = oldFileName.substring(oldFileName.lastIndexOf("."));
String filePath = FileUtil.getUpLoadFilePath();
System.out.println(typeName);
String newFileName = System.currentTimeMillis() + typeName;
try {
FileUtil.uploadFile(file.getBytes(),filePath,newFileName);
} catch (Exception e) {
throw new RuntimeException(e);
}
return newFileName;
}
@GetMapping("/queryName")
List<GoodsInfo> queryNamePrice(@RequestParam(required = false) String name) {
return goodsInfoService.getAllGoodsInfoName(name);
}
@GetMapping("/queryPostCategory")
public List<GoodsInfo> queryPostCategory(@RequestParam(required = false)Date goods_post, @RequestParam(required = false) Integer category_id) {
if (goods_post != null&&category_id != null) {
return goodsInfoService.getAllGoodsInfoPostCategory(goods_post,category_id);
} else if (goods_post!=null&&category_id==null) {
return goodsInfoService.getAllGoodsInfoPost(goods_post);
} else if (goods_post == null&&category_id != null) {
return goodsInfoService.getAllGoodsInfoCategory(category_id);
}else{
return goodsInfoService.getAllGoodsInfo3();
}
}
@GetMapping("/queryId")
public List<CategoryGoodsIn
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
该项目为基于HTML和Java的潮玩二手交易平台设计源码,包含464个文件,涵盖98个Java源文件、49个HTML文件、42个JavaScript文件、41个Vue文件、30个CSS文件以及相应数量的图片和字体资源。系统采用现代化的Web技术栈,支持用户进行潮玩二手交易,界面友好,功能丰富。
资源推荐
资源详情
资源评论
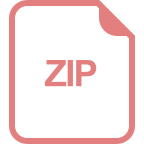
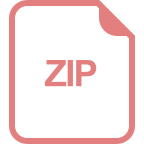
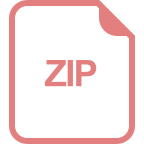
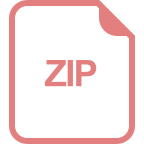
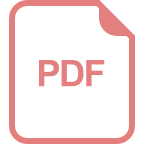
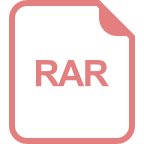
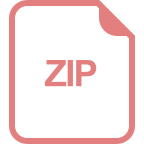
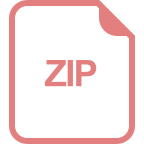
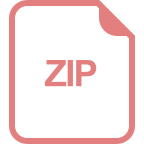
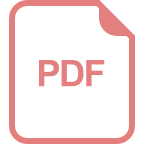
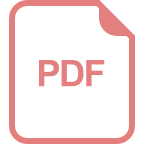
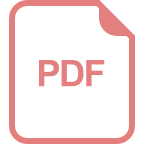
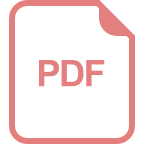
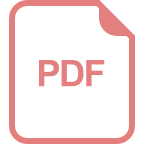
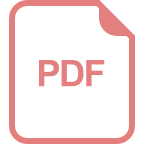
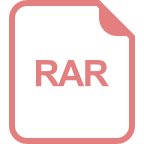
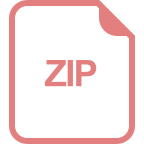
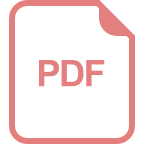
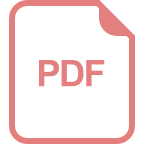
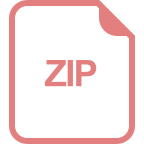
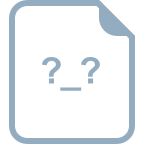
收起资源包目录

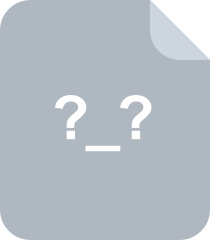
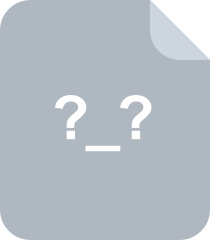
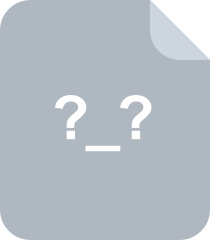
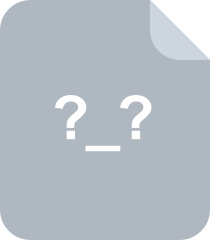
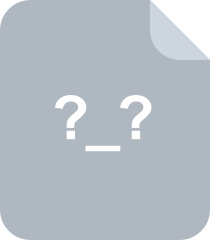
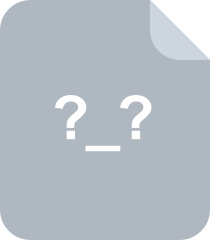
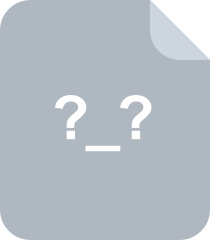
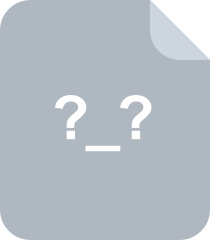
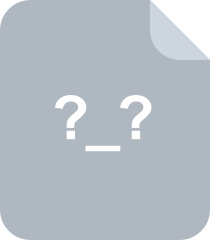
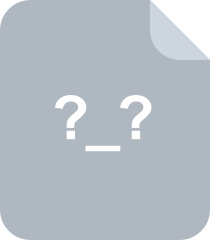
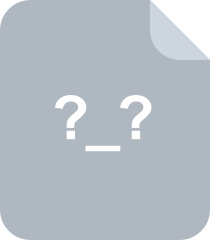
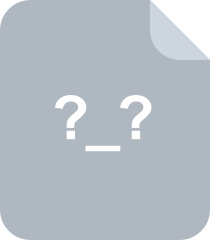
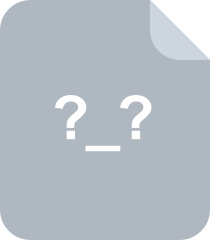
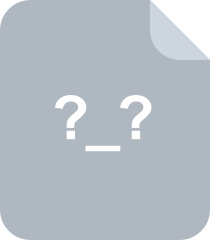
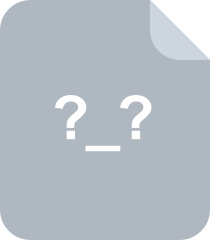
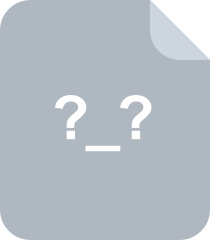
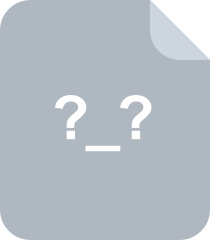
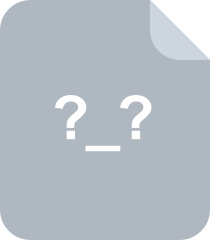
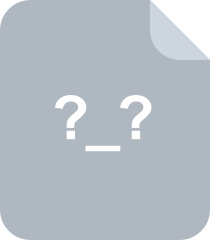
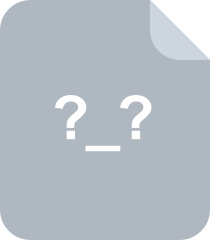
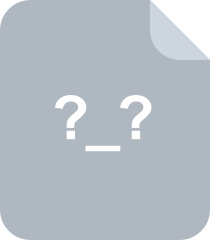
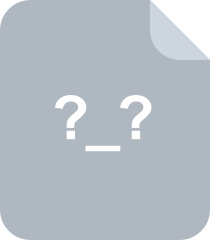
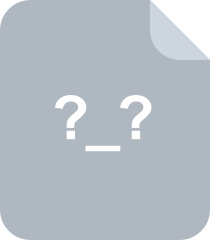
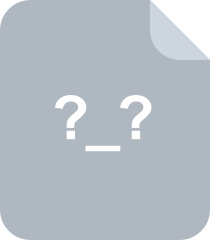
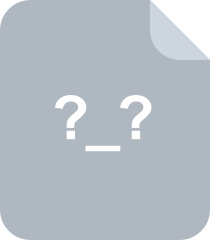
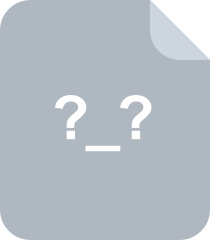
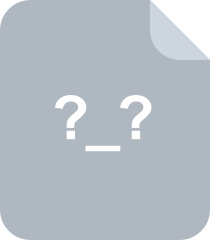
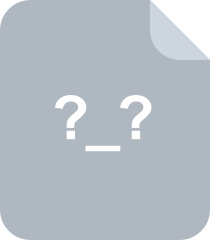
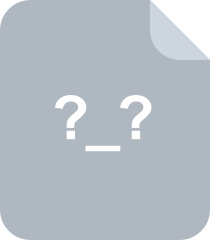
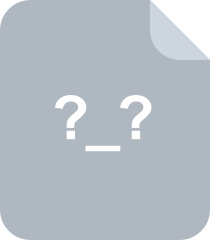
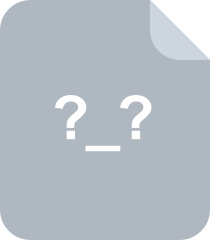
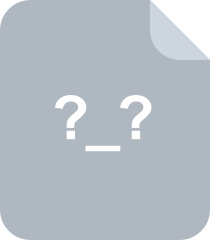
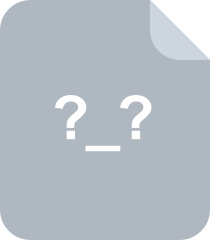
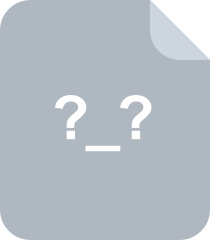
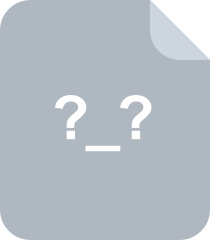
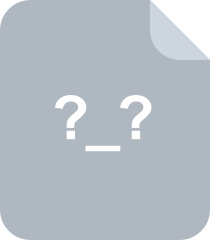
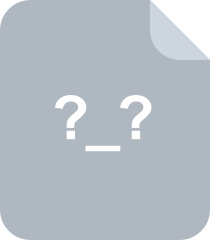
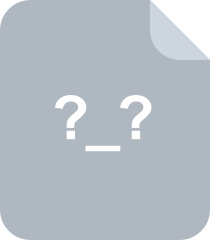
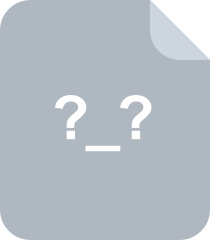
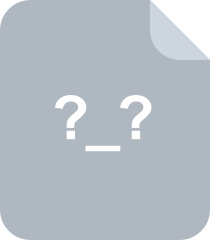
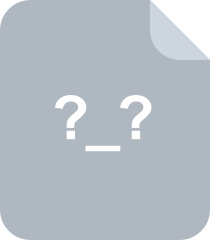
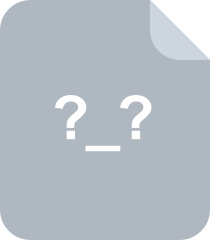
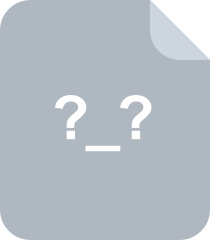
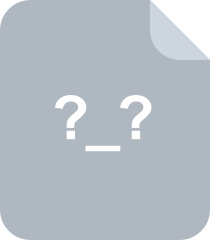
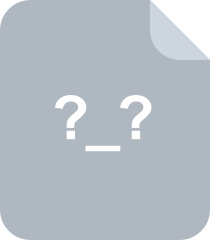
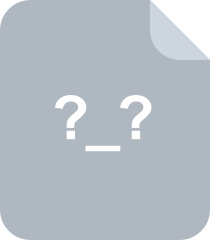
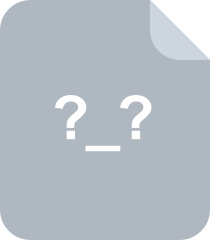
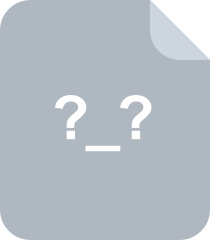
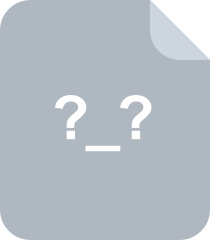
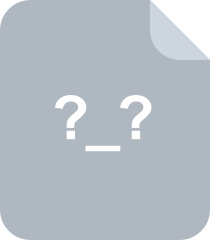
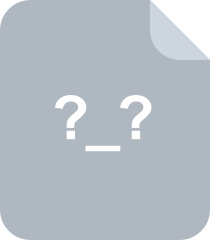
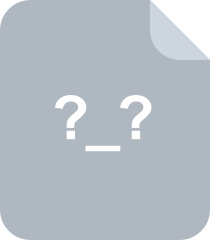
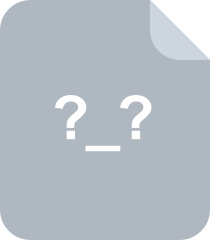
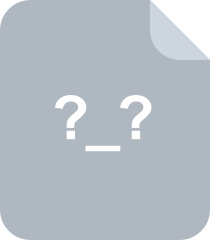
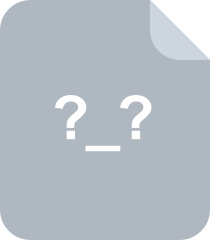
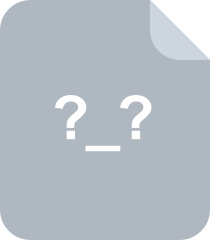
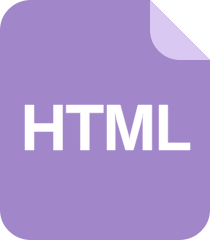
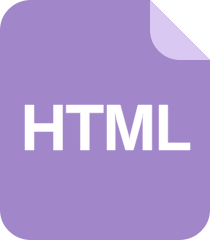
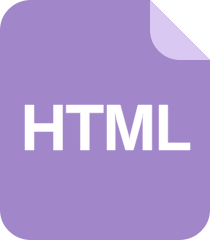
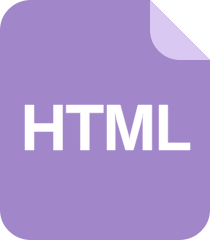
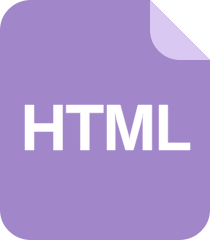
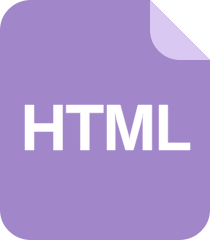
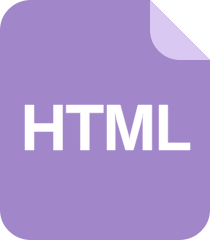
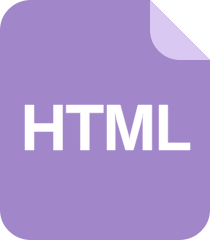
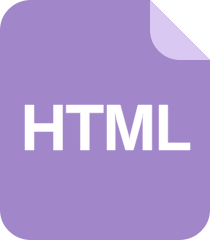
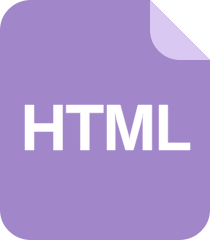
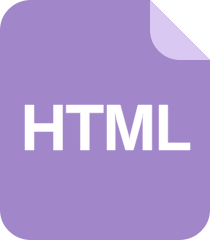
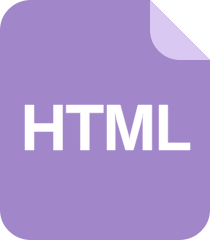
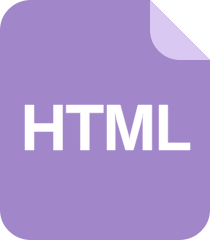
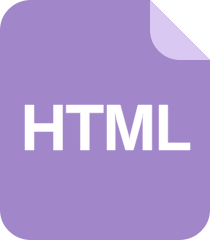
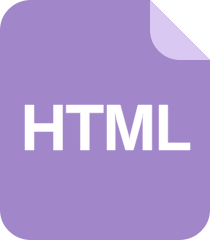
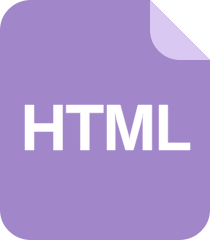
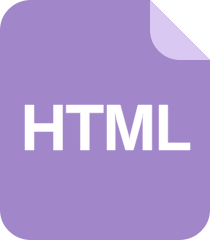
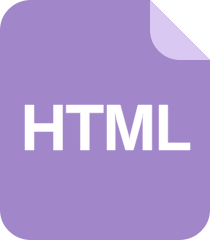
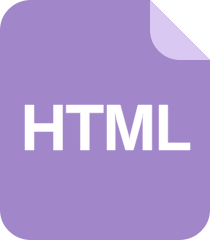
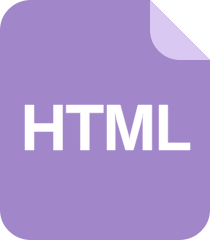
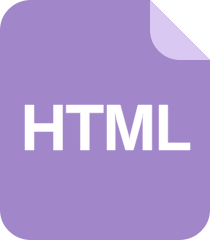
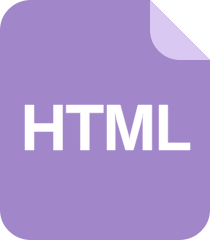
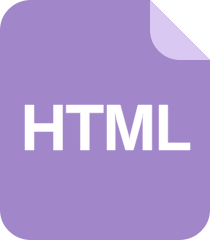
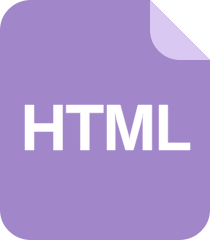
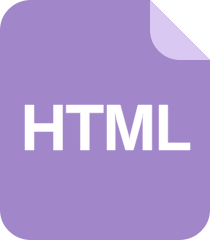
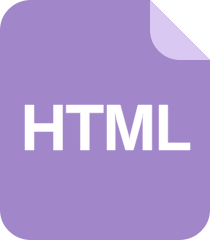
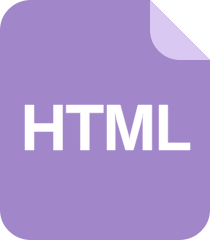
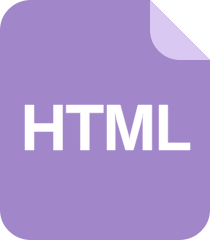
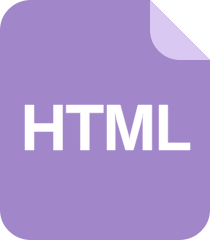
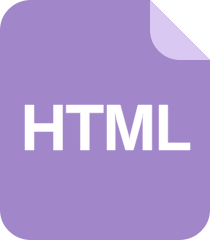
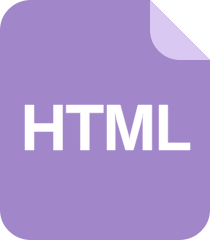
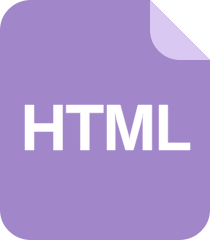
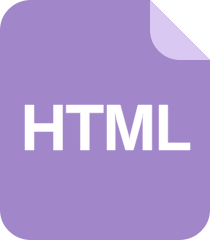
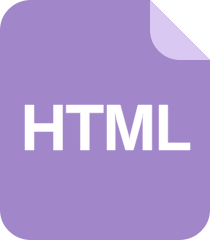
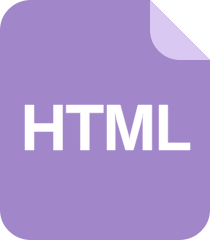
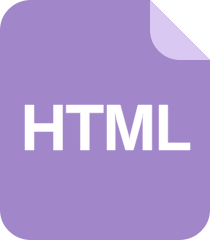
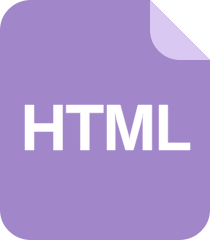
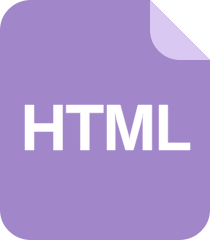
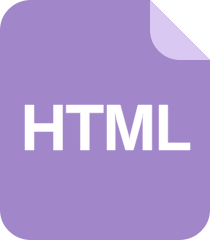
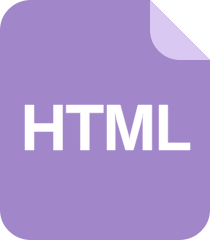
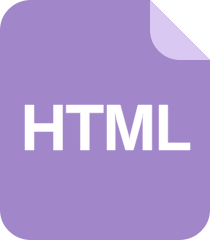
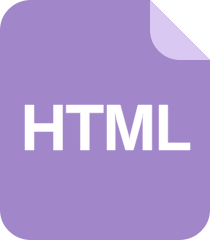
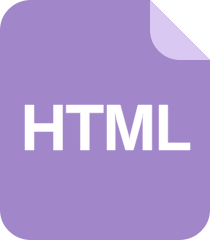
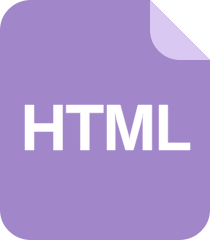
共 463 条
- 1
- 2
- 3
- 4
- 5
资源评论
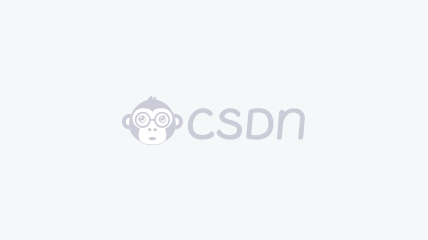

xyq2024
- 粉丝: 2318
- 资源: 5418
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

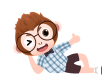
安全验证
文档复制为VIP权益,开通VIP直接复制
