/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.oceansoft.mobile.econsole.common.util.time;
import com.oceansoft.mobile.econsole.common.util.StringUtils;
import java.text.ParseException;
import java.text.ParsePosition;
import java.text.SimpleDateFormat;
import java.util.*;
/**
* 常用日期工具
* <p>A suite of utilities surrounding the use of the
* {@link java.util.Calendar} and {@link java.util.Date} object.</p>
* <p/>
* <p>DateUtils contains a lot of common methods considering manipulations
* of Dates or Calendars. Some methods require some extra explanation.
* The truncate, ceiling and round methods could be considered the Math.floor(),
* Math.ceil() or Math.round versions for dates
* This way date-fields will be ignored in bottom-up order.
* As a complement to these methods we've introduced some fragment-methods.
* With these methods the Date-fields will be ignored in top-down order.
* Since a date without a year is not a valid date, you have to decide in what
* kind of date-field you want your result, for instance milliseconds or days.
* </p>
*
* @author Apache Software Foundation
* @author <a href="mailto:sergek@lokitech.com">Serge Knystautas</a>
* @author Janek Bogucki
* @author <a href="mailto:ggregory@seagullsw.com">Gary Gregory</a>
* @author Phil Steitz
* @author Robert Scholte
* @version $Id: DateUtils.java 911986 2010-02-19 21:19:05Z niallp $
* @since 2.0
*/
public class DateUtils {
/**
* The UTC time zone (often referred to as GMT).
*/
public static final TimeZone UTC_TIME_ZONE = TimeZone.getTimeZone("GMT");
/**
* Number of milliseconds in a standard second.
*
* @since 2.1
*/
public static final long MILLIS_PER_SECOND = 1000;
/**
* Number of milliseconds in a standard minute.
*
* @since 2.1
*/
public static final long MILLIS_PER_MINUTE = 60 * MILLIS_PER_SECOND;
/**
* Number of milliseconds in a standard hour.
*
* @since 2.1
*/
public static final long MILLIS_PER_HOUR = 60 * MILLIS_PER_MINUTE;
/**
* Number of milliseconds in a standard day.
*
* @since 2.1
*/
public static final long MILLIS_PER_DAY = 24 * MILLIS_PER_HOUR;
/**
* This is half a month, so this represents whether a date is in the top
* or bottom half of the month.
*/
public final static int SEMI_MONTH = 1001;
private static final int[][] fields = {{Calendar.MILLISECOND}, {Calendar.SECOND}, {Calendar.MINUTE}, {Calendar.HOUR_OF_DAY, Calendar.HOUR}, {Calendar.DATE, Calendar.DAY_OF_MONTH, Calendar.AM_PM
/* Calendar.DAY_OF_YEAR, Calendar.DAY_OF_WEEK, Calendar.DAY_OF_WEEK_IN_MONTH */}, {Calendar.MONTH, DateUtils.SEMI_MONTH}, {Calendar.YEAR}, {Calendar.ERA}};
/**
* A week range, starting on Sunday.
*/
public final static int RANGE_WEEK_SUNDAY = 1;
/**
* A week range, starting on Monday.
*/
public final static int RANGE_WEEK_MONDAY = 2;
/**
* A week range, starting on the day focused.
*/
public final static int RANGE_WEEK_RELATIVE = 3;
/**
* A week range, centered around the day focused.
*/
public final static int RANGE_WEEK_CENTER = 4;
/**
* A month range, the week starting on Sunday.
*/
public final static int RANGE_MONTH_SUNDAY = 5;
/**
* A month range, the week starting on Monday.
*/
public final static int RANGE_MONTH_MONDAY = 6;
/**
* Constant marker for truncating
*/
private final static int MODIFY_TRUNCATE = 0;
/**
* Constant marker for rounding
*/
private final static int MODIFY_ROUND = 1;
/**
* Constant marker for ceiling
*/
private final static int MODIFY_CEILING = 2;
/**
* <p><code>DateUtils</code> instances should NOT be constructed in
* standard programming. Instead, the class should be used as
* <code>DateUtils.parse(str);</code>.</p>
* <p/>
* <p>This constructor is public to permit tools that require a JavaBean
* instance to operate.</p>
*/
public DateUtils() {
super();
}
//-----------------------------------------------------------------------
/**
* <p>Checks if two date objects are on the same day ignoring time.</p>
* <p/>
* <p>28 Mar 2002 13:45 and 28 Mar 2002 06:01 would return true.
* 28 Mar 2002 13:45 and 12 Mar 2002 13:45 would return false.
* </p>
*
* @param date1 the first date, not altered, not null
* @param date2 the second date, not altered, not null
* @return true if they represent the same day
* @throws IllegalArgumentException if either date is <code>null</code>
* @since 2.1
*/
public static boolean isSameDay(Date date1, Date date2) {
if (date1 == null || date2 == null) {
throw new IllegalArgumentException("The date must not be null");
}
Calendar cal1 = Calendar.getInstance();
cal1.setTime(date1);
Calendar cal2 = Calendar.getInstance();
cal2.setTime(date2);
return isSameDay(cal1, cal2);
}
/**
* <p>Checks if two calendar objects are on the same day ignoring time.</p>
* <p/>
* <p>28 Mar 2002 13:45 and 28 Mar 2002 06:01 would return true.
* 28 Mar 2002 13:45 and 12 Mar 2002 13:45 would return false.
* </p>
*
* @param cal1 the first calendar, not altered, not null
* @param cal2 the second calendar, not altered, not null
* @return true if they represent the same day
* @throws IllegalArgumentException if either calendar is <code>null</code>
* @since 2.1
*/
public static boolean isSameDay(Calendar cal1, Calendar cal2) {
if (cal1 == null || cal2 == null) {
throw new IllegalArgumentException("The date must not be null");
}
return (cal1.get(Calendar.ERA) == cal2.get(Calendar.ERA) &&
cal1.get(Calendar.YEAR) == cal2.get(Calendar.YEAR) &&
cal1.get(Calendar.DAY_OF_YEAR) == cal2.get(Calendar.DAY_OF_YEAR));
}
//-----------------------------------------------------------------------
/**
* <p>Checks if two date objects represent the same instant in time.</p>
* <p/>
* <p>This method compares the long millisecond time of the two objects.</p>
*
* @param date1 the first date, not altered, not null
* @param date2 the second date, not altered, not null
* @return true if they represent the same millisecond instant
* @throws IllegalArgumentException if either date is <code>null</code>
* @since 2.1
*/
public static boolean isSameInstant(Date date1, Date date2) {
if (date1 == null || date2 == null) {
throw new IllegalArgumentException("The date must not be null");
}
return date1.getTime() == date2.getTime();
}
/**
* <p>Checks if two calendar objects represent the same instant in time.</p>
* <p/>
* <p>This method compares the long millisecond time of the two objects.</p>
*
* @param cal1 the first calendar, not altered, not null
* @param cal2 the second calendar, not altered, not null
没有合适的资源?快使用搜索试试~ 我知道了~
基于Java、CSS、JavaScript和HTML的云南微信Vue报警功能设计源码
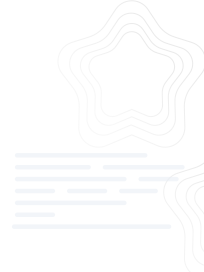
共2000个文件
java:833个
css:339个
js:337个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 4 浏览量
2024-10-05
12:46:20
上传
评论
收藏 71.65MB ZIP 举报
温馨提示
本项目为云南微信Vue平台的报警功能设计源码,整合了Java、CSS、JavaScript和HTML等多种技术。源码包含3917个文件,其中Java源文件833个,CSS样式文件339个,JavaScript脚本文件337个,HTML文件81个。此外,还包含1254个PNG图片、345个GIF动画、224个JPG图片、102个SVG图形文件等。该源码旨在为云南微信平台提供高效的报警功能实现。
资源推荐
资源详情
资源评论
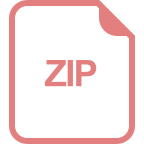
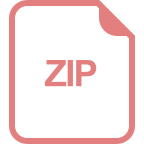
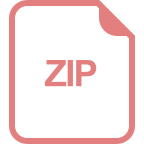
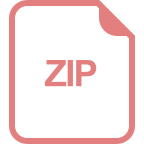
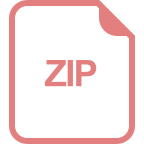
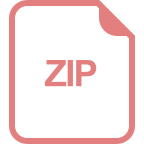
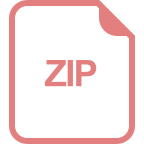
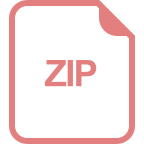
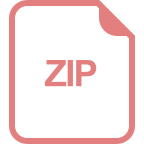
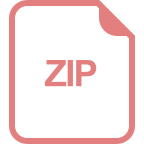
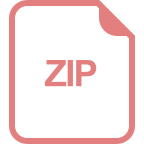
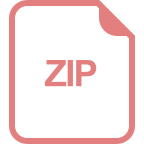
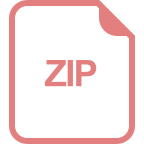
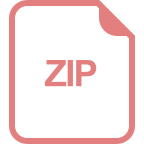
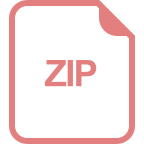
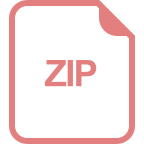
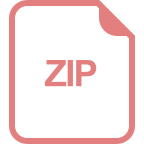
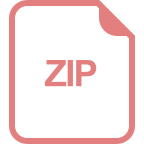
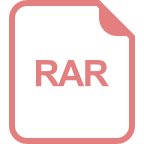
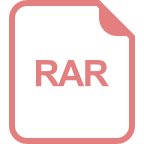
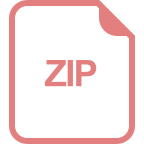
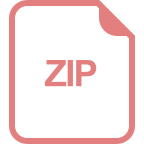
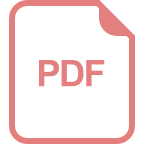
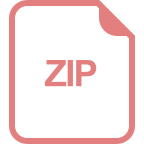
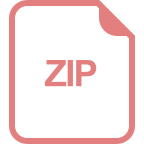
收起资源包目录

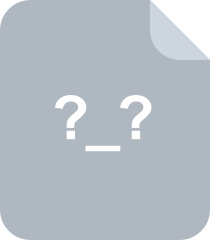
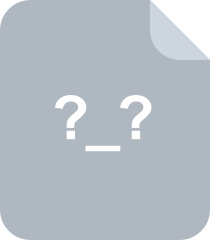
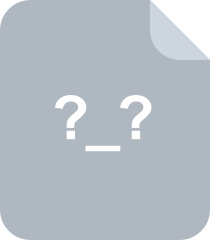
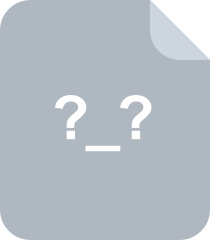
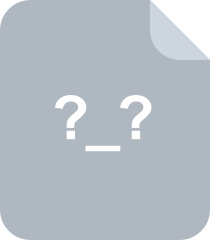
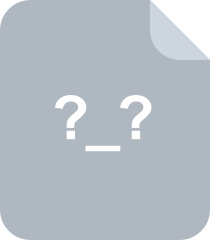
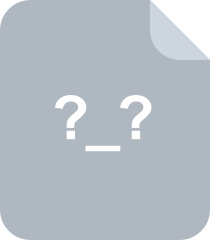
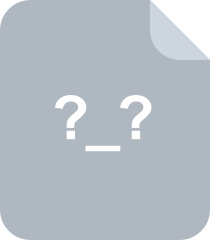
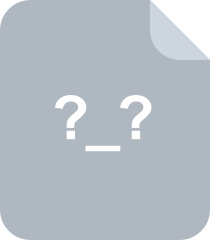
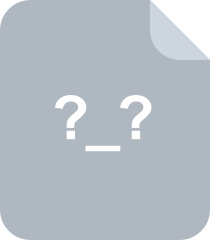
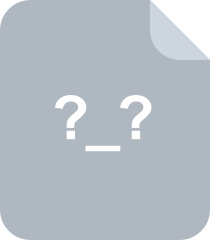
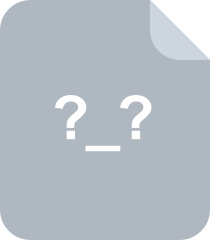
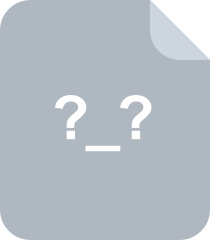
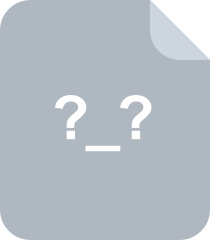
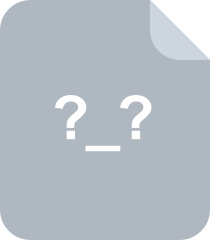
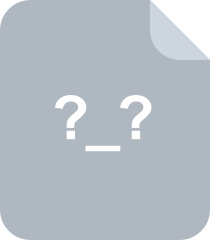
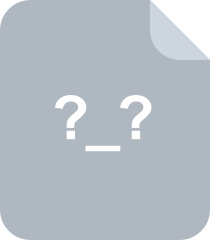
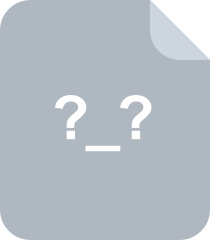
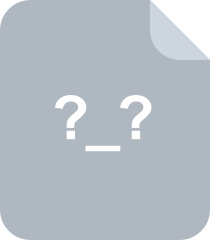
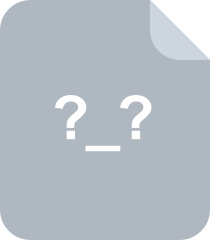
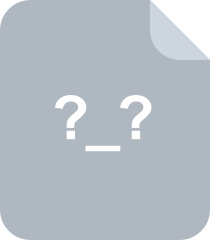
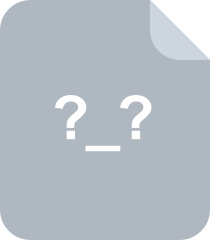
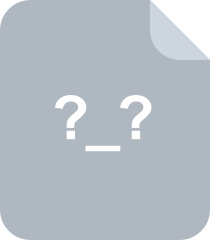
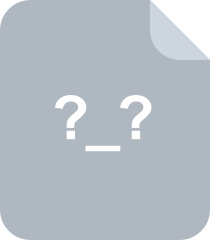
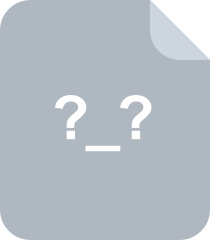
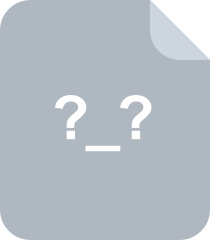
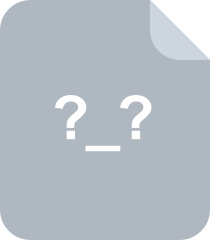
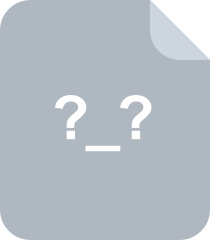
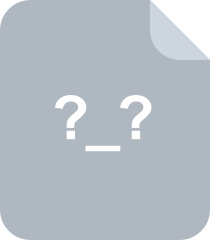
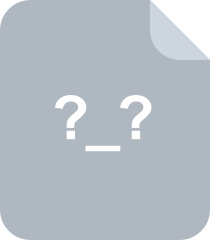
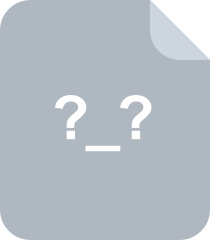
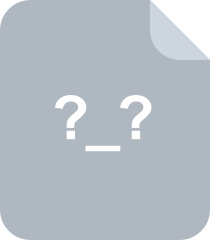
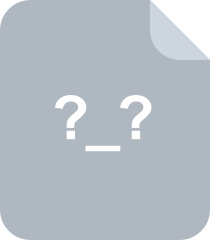
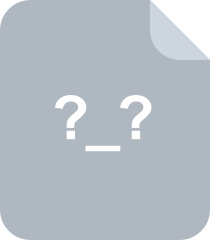
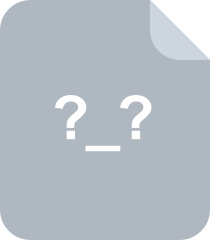
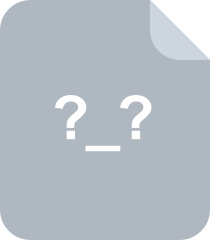
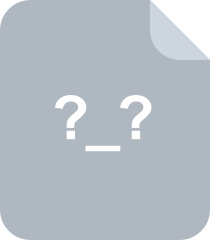
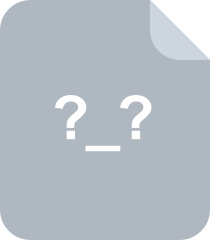
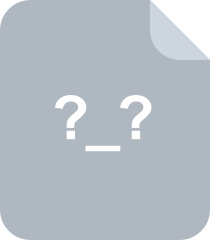
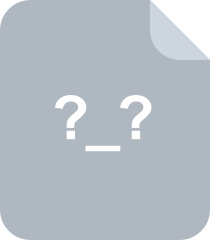
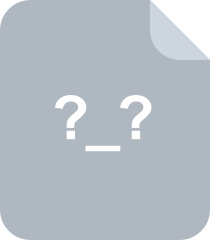
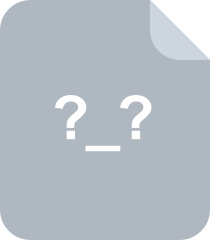
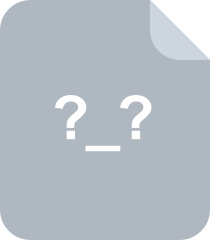
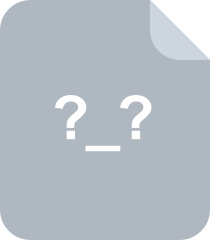
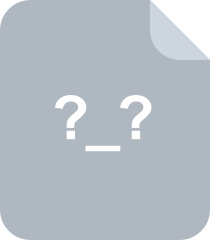
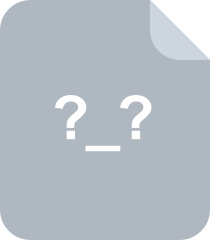
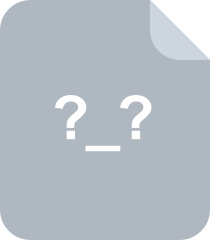
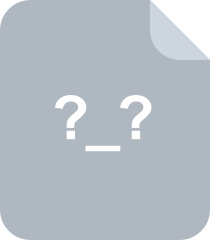
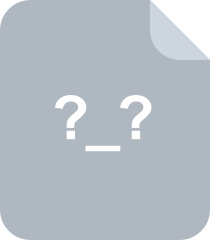
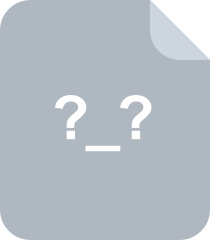
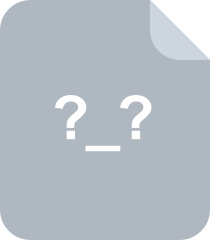
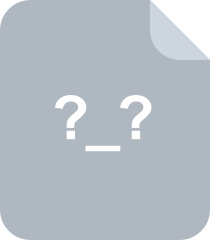
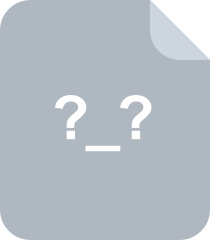
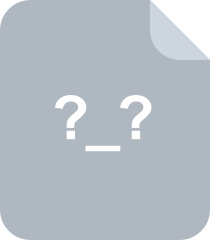
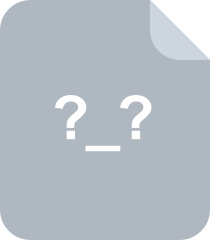
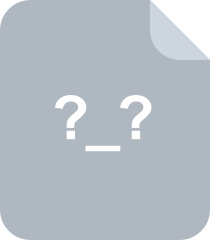
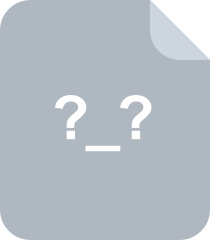
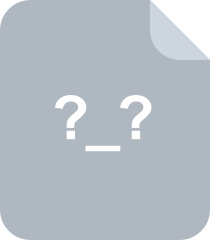
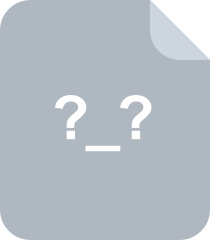
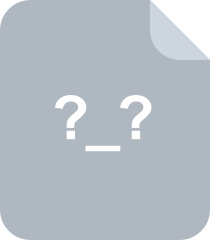
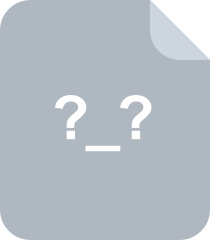
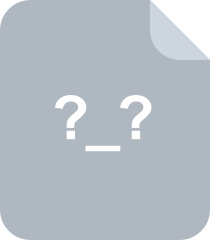
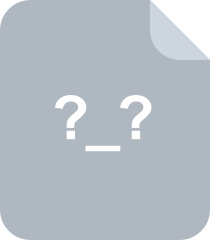
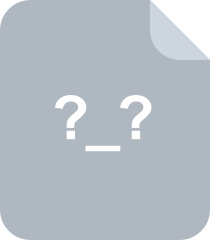
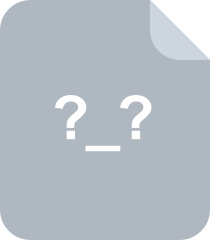
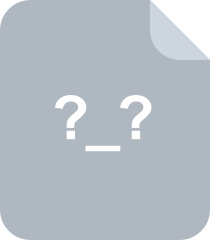
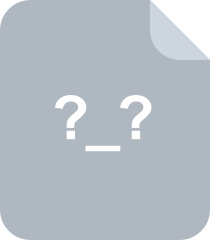
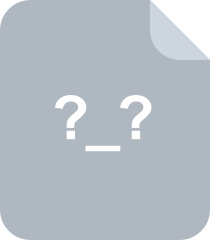
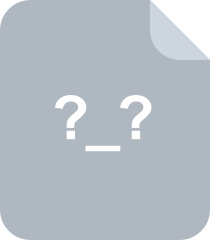
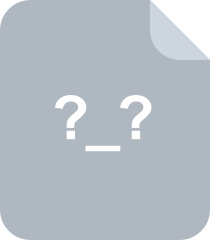
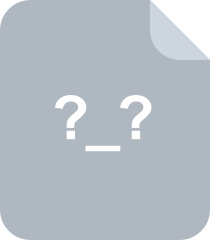
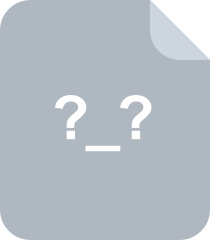
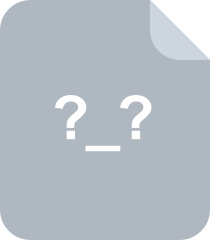
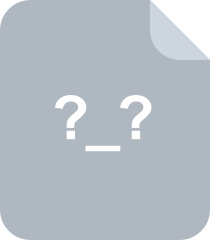
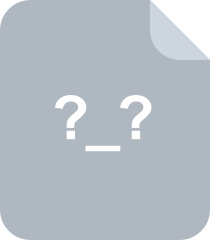
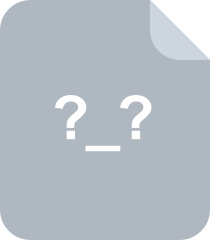
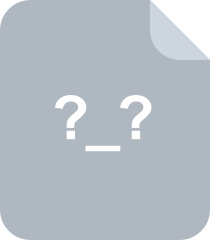
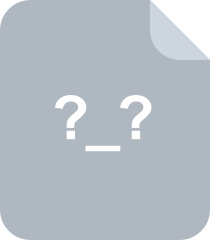
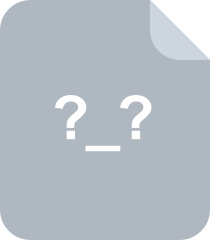
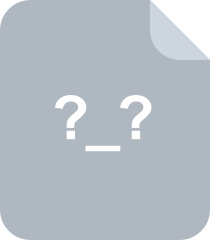
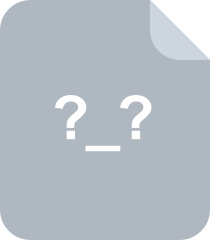
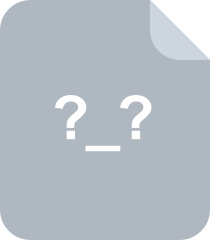
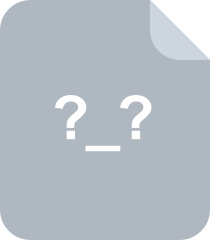
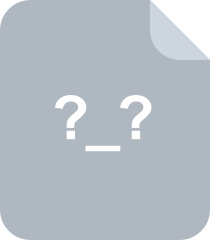
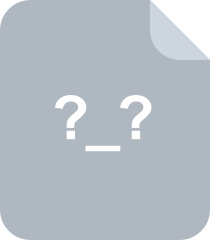
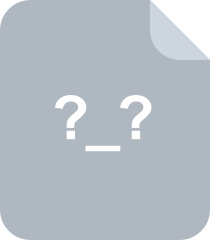
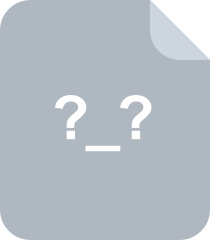
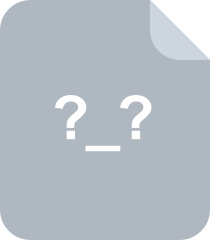
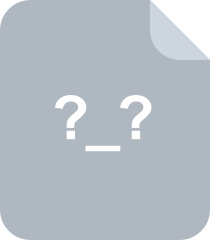
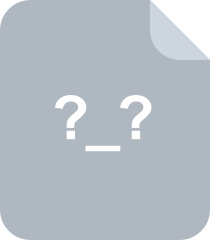
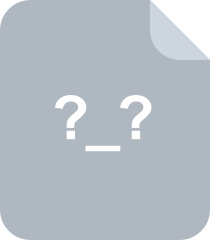
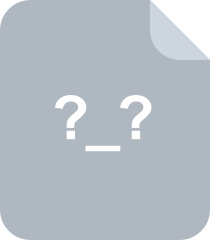
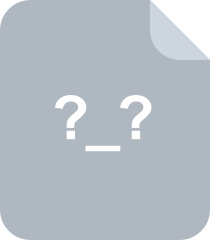
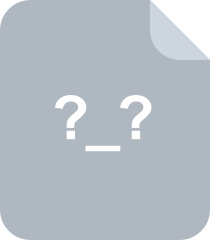
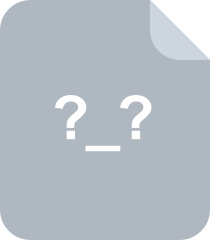
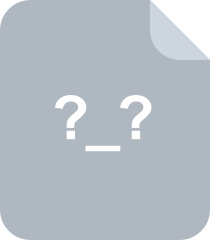
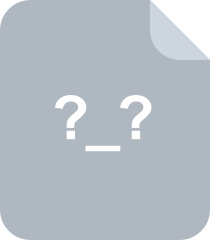
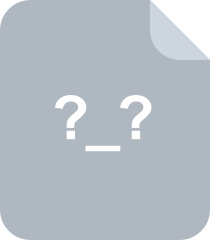
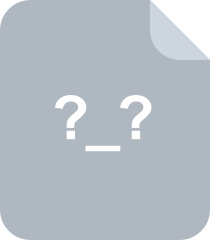
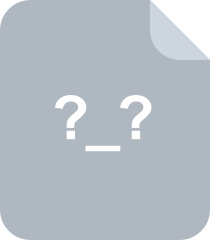
共 2000 条
- 1
- 2
- 3
- 4
- 5
- 6
- 20
资源评论
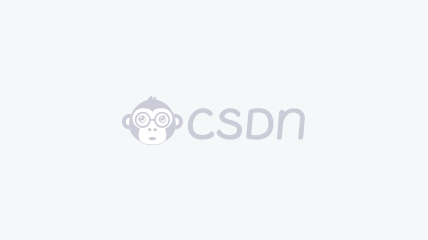

xyq2024
- 粉丝: 2929
- 资源: 5563
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

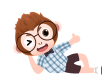
最新资源
- 【岗位说明】广告公司各职员职务说明书(精美版).doc
- 【岗位说明】广告公司各职员职务说明书.doc
- 【岗位说明】广告公司各岗位职责.docx
- 【岗位说明】XX培训机构岗位职责行政职责.doc
- 【岗位说明】风华教育培训中心岗位职责说明书.doc
- 【岗位说明】高校行政人员岗位职责.doc
- 【岗位说明】教师各岗位岗位职责.doc
- 【岗位说明】教学秘书岗位职责.doc
- 【岗位说明】培训机构助教老师岗位职责.doc
- 【岗位说明】培训机构老师岗位职责.doc
- 【岗位说明】培训学校人员岗位职责及任职要求.doc
- 【岗位说明】学校及培训机构岗位职责大全.doc
- 【岗位说明】幼儿园岗位责任制度.doc
- 【岗位说明】幼儿园岗位职责.doc
- 【岗位说明】幼儿园各类人员岗位职责.doc
- 【岗位说明】辅导机构各岗位职责.docx
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


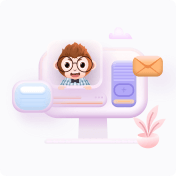
安全验证
文档复制为VIP权益,开通VIP直接复制
