<?php
namespace app\admin\command;
use fast\Form;
use think\Config;
use think\console\Command;
use think\console\Input;
use think\console\input\Option;
use think\console\Output;
use think\Db;
use think\Exception;
use think\exception\ErrorException;
use think\Lang;
use think\Loader;
class Crud extends Command
{
protected $stubList = [];
protected $internalKeywords = [
'abstract', 'and', 'array', 'as', 'break', 'callable', 'case', 'catch', 'class', 'clone', 'const', 'continue', 'declare', 'default', 'die', 'do', 'echo', 'else', 'elseif', 'empty', 'enddeclare', 'endfor', 'endforeach', 'endif', 'endswitch', 'endwhile', 'eval', 'exit', 'extends', 'final', 'for', 'foreach', 'function', 'global', 'goto', 'if', 'implements', 'include', 'include_once', 'instanceof', 'insteadof', 'interface', 'isset', 'list', 'namespace', 'new', 'or', 'print', 'private', 'protected', 'public', 'require', 'require_once', 'return', 'static', 'switch', 'throw', 'trait', 'try', 'unset', 'use', 'var', 'while', 'xor'
];
/**
* Selectpage搜索字段关联
*/
protected $fieldSelectpageMap = [
'nickname' => ['user_id', 'user_ids', 'admin_id', 'admin_ids']
];
/**
* Enum类型识别为单选框的结尾字符,默认会识别为单选下拉列表
*/
protected $enumRadioSuffix = ['data', 'state', 'status'];
/**
* Set类型识别为复选框的结尾字符,默认会识别为多选下拉列表
*/
protected $setCheckboxSuffix = ['data', 'state', 'status'];
/**
* Int类型识别为日期时间的结尾字符,默认会识别为日期文本框
*/
protected $intDateSuffix = ['time'];
/**
* 开关后缀
*/
protected $switchSuffix = ['switch'];
/**
* 富文本后缀
*/
protected $editorSuffix = ['content'];
/**
* 城市后缀
*/
protected $citySuffix = ['city'];
/**
* JSON后缀
*/
protected $jsonSuffix = ['json'];
/**
* Selectpage对应的后缀
*/
protected $selectpageSuffix = ['_id', '_ids'];
/**
* Selectpage多选对应的后缀
*/
protected $selectpagesSuffix = ['_ids'];
/**
* 以指定字符结尾的字段格式化函数
*/
protected $fieldFormatterSuffix = [
'status' => ['type' => ['varchar', 'enum'], 'name' => 'status'],
'icon' => 'icon',
'flag' => 'flag',
'url' => 'url',
'image' => 'image',
'images' => 'images',
'avatar' => 'image',
'switch' => 'toggle',
'time' => ['type' => ['int', 'timestamp'], 'name' => 'datetime']
];
/**
* 识别为图片字段
*/
protected $imageField = ['image', 'images', 'avatar', 'avatars'];
/**
* 识别为文件字段
*/
protected $fileField = ['file', 'files'];
/**
* 保留字段
*/
protected $reservedField = ['admin_id'];
/**
* 排除字段
*/
protected $ignoreFields = [];
/**
* 排序字段
*/
protected $sortField = 'weigh';
/**
* 筛选字段
* @var string
*/
protected $headingFilterField = 'status';
/**
* 添加时间字段
* @var string
*/
protected $createTimeField = 'createtime';
/**
* 更新时间字段
* @var string
*/
protected $updateTimeField = 'updatetime';
/**
* 软删除时间字段
* @var string
*/
protected $deleteTimeField = 'deletetime';
/**
* 编辑器的Class
*/
protected $editorClass = 'editor';
/**
* langList的key最长字节数
*/
protected $fieldMaxLen = 0;
protected function configure()
{
$this
->setName('crud')
->addOption('table', 't', Option::VALUE_REQUIRED, 'table name without prefix', null)
->addOption('controller', 'c', Option::VALUE_OPTIONAL, 'controller name', null)
->addOption('model', 'm', Option::VALUE_OPTIONAL, 'model name', null)
->addOption('fields', 'i', Option::VALUE_OPTIONAL, 'model visible fields', null)
->addOption('force', 'f', Option::VALUE_OPTIONAL, 'force override or force delete,without tips', null)
->addOption('local', 'l', Option::VALUE_OPTIONAL, 'local model', 1)
->addOption('relation', 'r', Option::VALUE_OPTIONAL | Option::VALUE_IS_ARRAY, 'relation table name without prefix', null)
->addOption('relationmodel', 'e', Option::VALUE_OPTIONAL | Option::VALUE_IS_ARRAY, 'relation model name', null)
->addOption('relationforeignkey', 'k', Option::VALUE_OPTIONAL | Option::VALUE_IS_ARRAY, 'relation foreign key', null)
->addOption('relationprimarykey', 'p', Option::VALUE_OPTIONAL | Option::VALUE_IS_ARRAY, 'relation primary key', null)
->addOption('relationfields', 's', Option::VALUE_OPTIONAL | Option::VALUE_IS_ARRAY, 'relation table fields', null)
->addOption('relationmode', 'o', Option::VALUE_OPTIONAL | Option::VALUE_IS_ARRAY, 'relation table mode,hasone or belongsto', null)
->addOption('delete', 'd', Option::VALUE_OPTIONAL, 'delete all files generated by CRUD', null)
->addOption('menu', 'u', Option::VALUE_OPTIONAL, 'create menu when CRUD completed', null)
->addOption('setcheckboxsuffix', null, Option::VALUE_OPTIONAL | Option::VALUE_IS_ARRAY, 'automatically generate checkbox component with suffix', null)
->addOption('enumradiosuffix', null, Option::VALUE_OPTIONAL | Option::VALUE_IS_ARRAY, 'automatically generate radio component with suffix', null)
->addOption('imagefield', null, Option::VALUE_OPTIONAL | Option::VALUE_IS_ARRAY, 'automatically generate image component with suffix', null)
->addOption('filefield', null, Option::VALUE_OPTIONAL | Option::VALUE_IS_ARRAY, 'automatically generate file component with suffix', null)
->addOption('intdatesuffix', null, Option::VALUE_OPTIONAL | Option::VALUE_IS_ARRAY, 'automatically generate date component with suffix', null)
->addOption('switchsuffix', null, Option::VALUE_OPTIONAL | Option::VALUE_IS_ARRAY, 'automatically generate switch component with suffix', null)
->addOption('citysuffix', null, Option::VALUE_OPTIONAL | Option::VALUE_IS_ARRAY, 'automatically generate citypicker component with suffix', null)
->addOption('jsonsuffix', null, Option::VALUE_OPTIONAL | Option::VALUE_IS_ARRAY, 'automatically generate fieldlist component with suffix', null)
->addOption('selectpagesuffix', null, Option::VALUE_OPTIONAL | Option::VALUE_IS_ARRAY, 'automatically generate selectpage component with suffix', null)
->addOption('selectpagessuffix', null, Option::VALUE_OPTIONAL | Option::VALUE_IS_ARRAY, 'automatically generate multiple selectpage component with suffix', null)
->addOption('ignorefields', null, Option::VALUE_OPTIONAL | Option::VALUE_IS_ARRAY, 'ignore fields', null)
->addOption('sortfield', null, Option::VALUE_OPTIONAL, 'sort field', null)
->addOption('headingfilterfield', null, Option::VALUE_OPTIONAL, 'heading filter field', null)
->addOption('editorclass', null, Option::VALUE_OPTIONAL, 'automatically generate editor class', null)
->addOption('db', null, Option::VALUE_OPTIONAL, 'database config name', 'database')
->setDescription('Build CRUD controller and model from table');
}
protected function execute(Input $input, Output $output)
{
$adminPath = dirname(__DIR__) . DS;
//数据库
$db = $input->getOption('db');
//表名
$table = $input->getOption('table') ?: '';
//自定义控制器
$controller = $input->getOption('controller');
//自定义模型
$model = $input->getOption('model');
$model = $model ? $model : $controller;
//验证器类
$validat
没有合适的资源?快使用搜索试试~ 我知道了~
基于PHP的农家直采后台管理系统源码
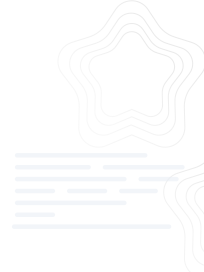
共980个文件
php:306个
less:158个
html:153个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 23 浏览量
2024-10-04
19:22:10
上传
评论
收藏 25.03MB ZIP 举报
温馨提示
本项目是一款基于PHP开发的农家直采后台管理系统源码,包含978个文件,涵盖306个PHP脚本、158个LESS样式表、151个HTML页面、102个JavaScript文件、71个PNG图片、39个CSS样式文件、33个stub文件、27个GIF图片、14个SVG矢量图、14个字体文件(.ttf)以及少量其他文件。系统采用HTML、JavaScript和CSS进行前端展示和交互,适用于管理农家直采业务,提升供应链效率。
资源推荐
资源详情
资源评论
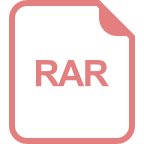
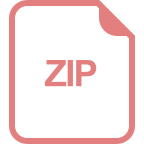
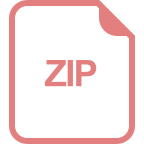
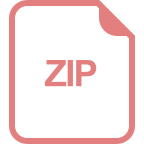
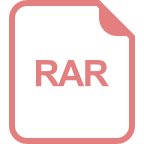
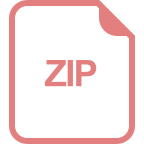
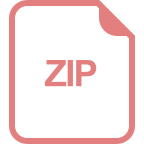
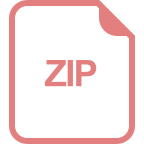
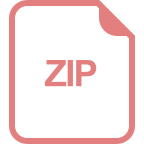
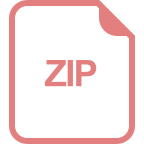
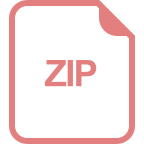
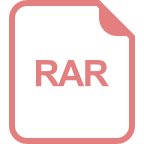
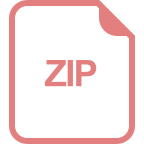
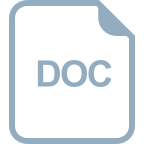
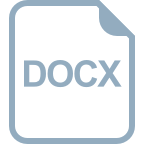
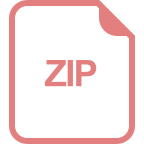
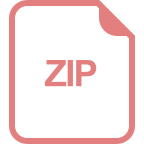
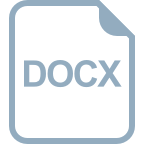
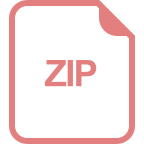
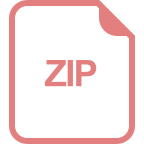
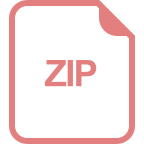
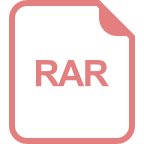
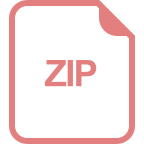
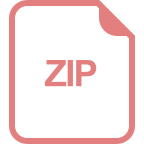
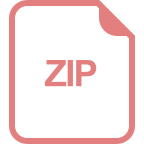
收起资源包目录

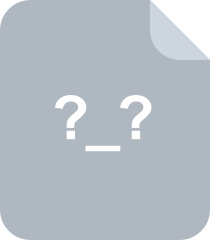
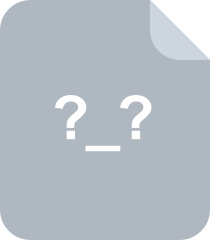
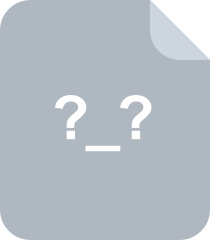
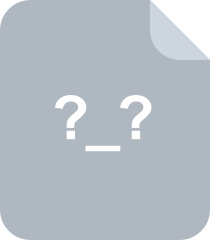
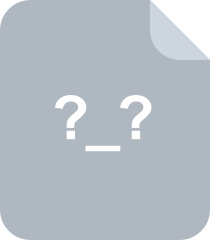
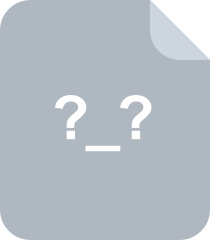
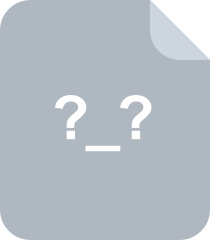
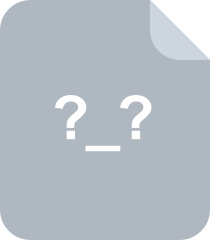
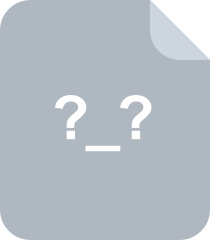
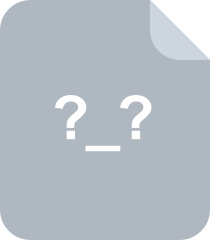
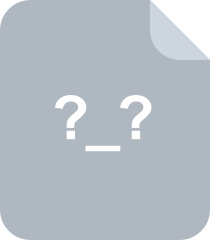
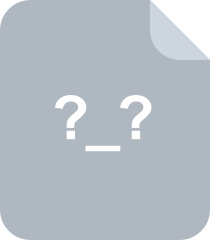
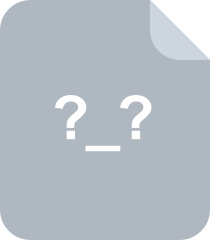
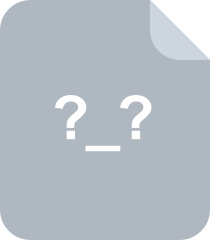
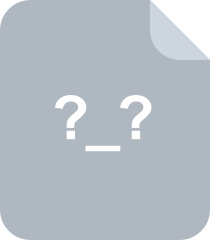
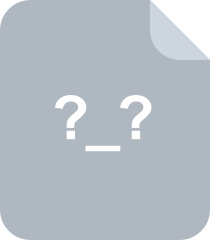
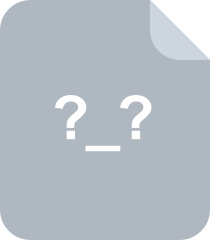
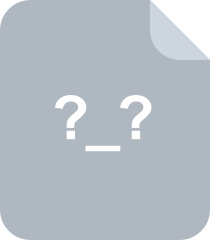
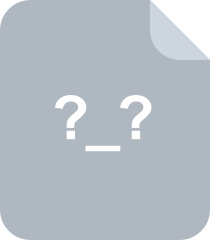
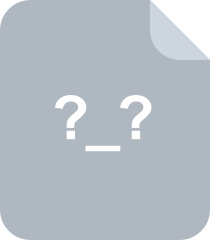
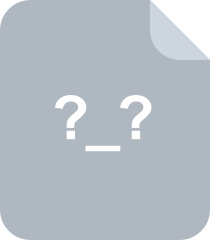
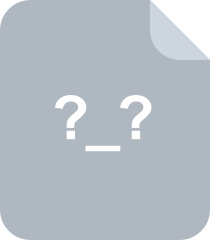
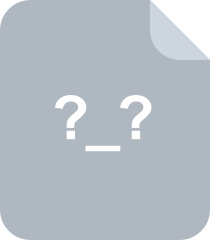
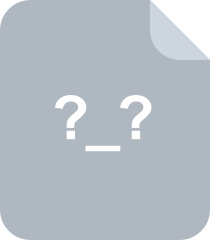
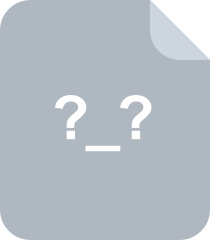
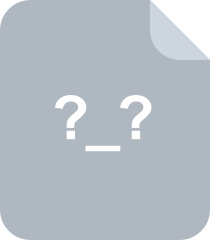
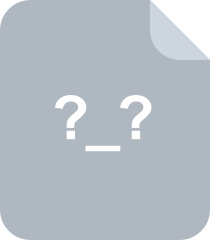
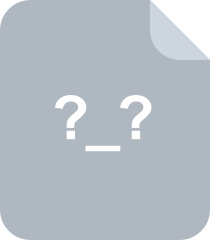
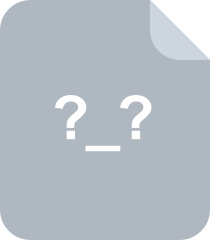
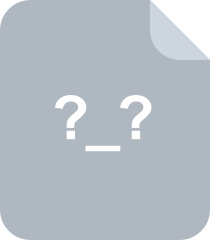
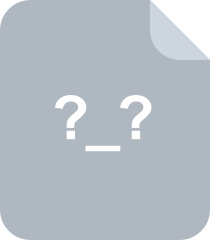
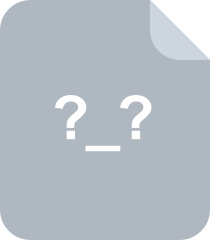
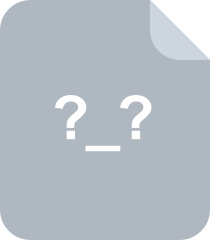
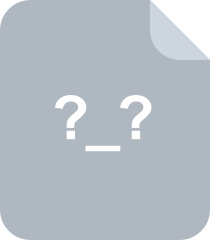
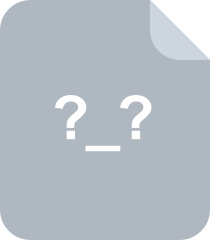
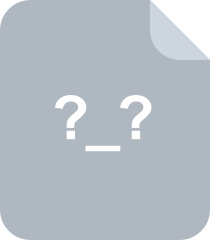
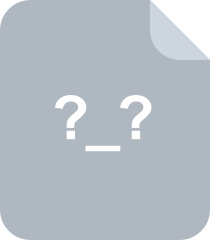
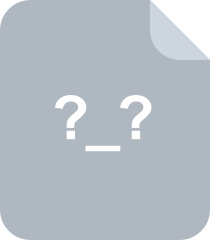
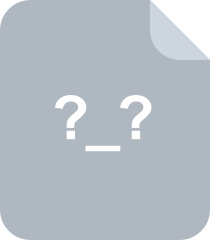
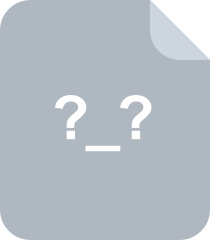
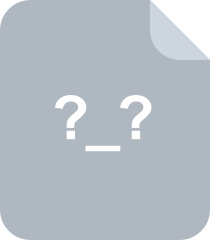
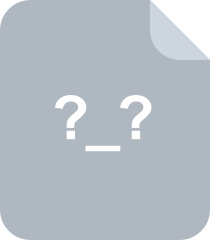
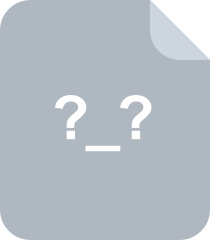
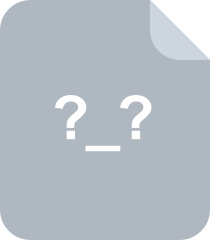
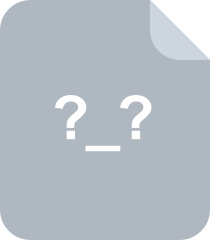
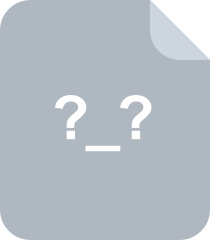
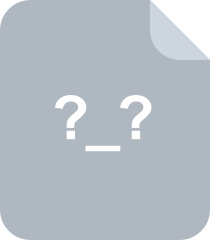
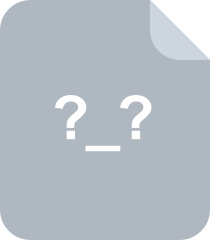
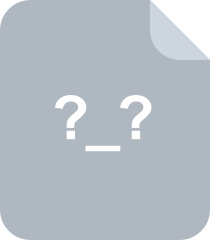
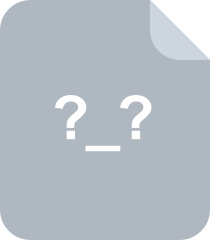
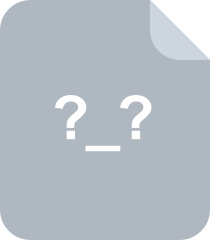
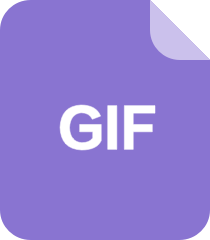
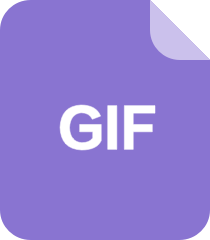
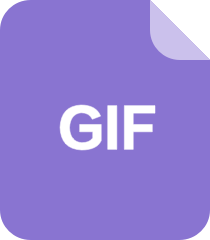
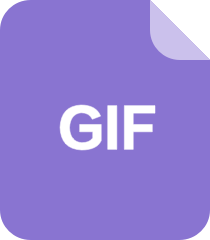
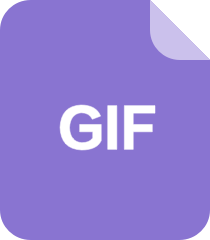
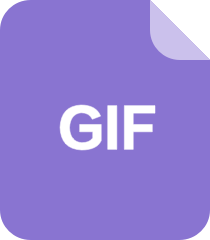
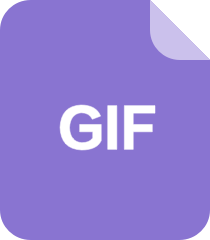
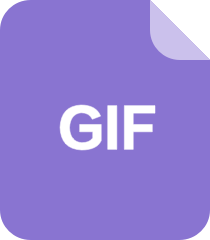
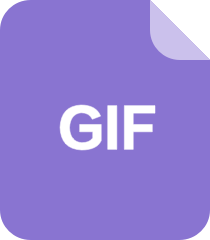
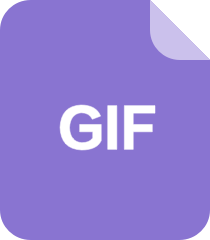
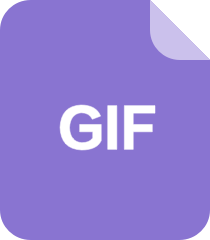
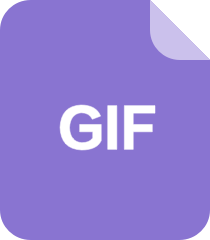
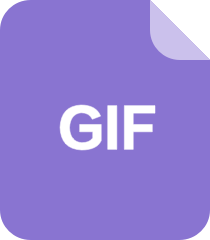
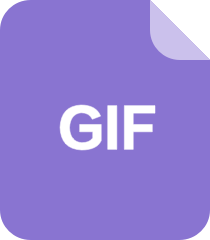
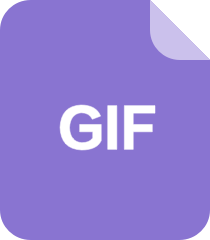
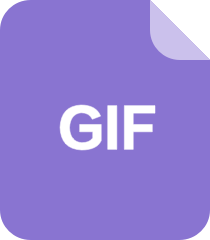
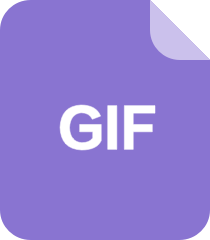
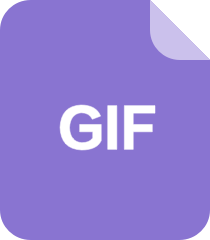
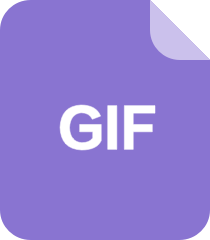
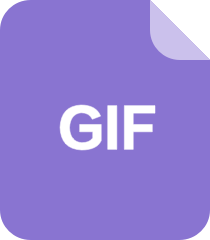
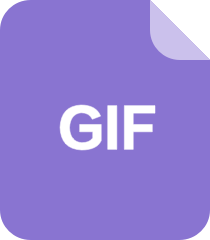
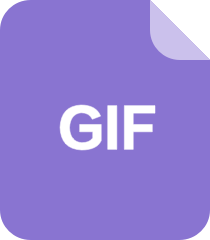
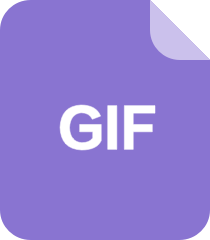
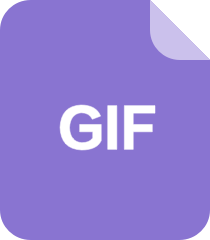
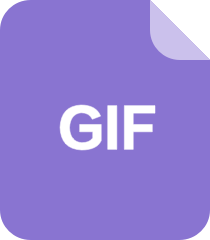
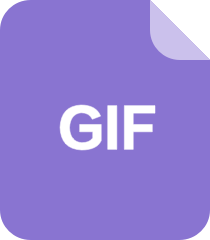
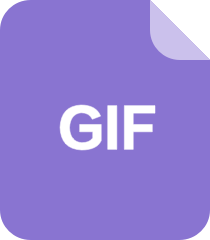
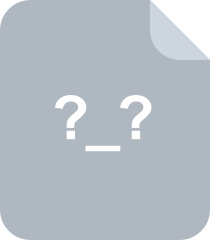
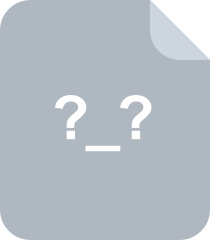
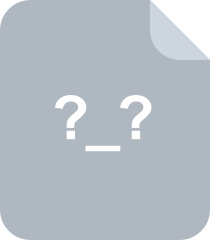
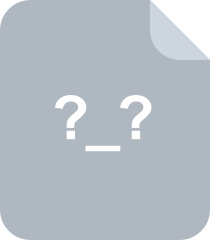
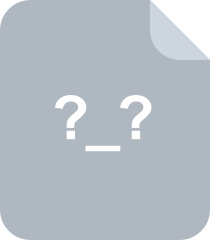
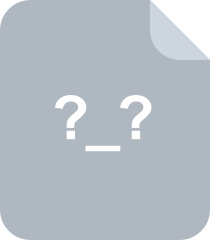
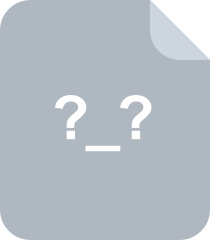
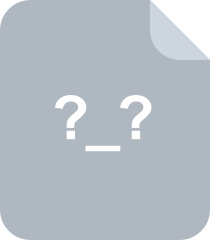
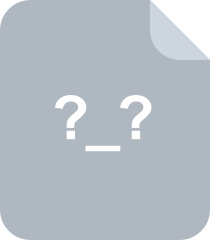
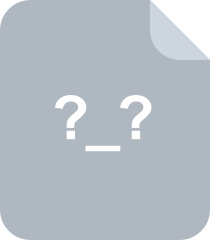
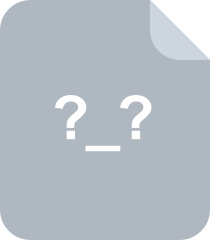
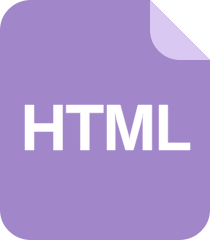
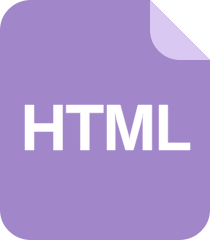
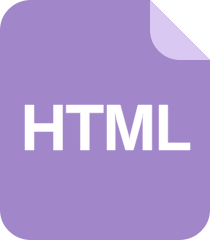
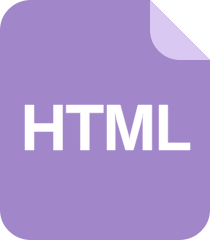
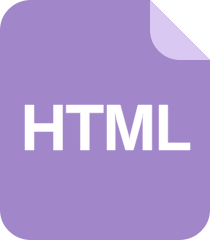
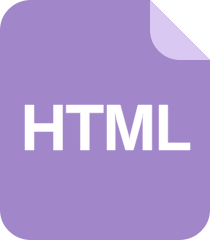
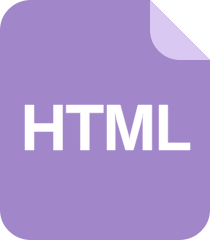
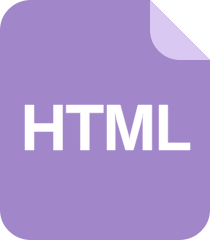
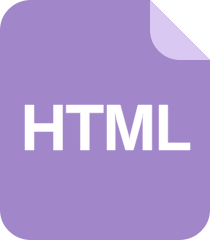
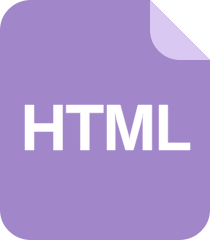
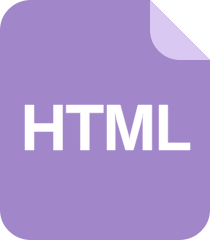
共 980 条
- 1
- 2
- 3
- 4
- 5
- 6
- 10
资源评论
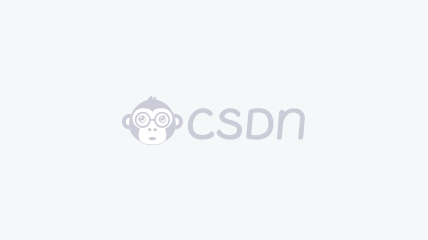

xyq2024
- 粉丝: 2343
- 资源: 5434
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

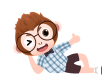
最新资源
- 百度EasyDL经典版数据集管理API NodeSDK-easydlclassic.zip
- 没有大师傅大师傅士大夫撒旦
- 2024年10月29日全A逐笔tick数据
- 用QT实现的桌面端聊天室软件,含服务端和客户端,使用经过SSL加密的TCP通
- 一款基于 MATLAB 的 EEG 神经反馈训练系统 在神经反馈实验过程中可实时观察并记录 EEG 信号和神经反馈实验标记
- Java SSM 商户管理系统 客户管理 库存管理 销售报表 项目源码 本商品卖的是源码,合适的地方直接拿来使用,不合适的根据
- 基于Spring boot 的Starter机制提供一个开箱即用的多数据源抽取工具包,计划对RDMS(关系型
- 水泵系统水力计算公式-标准版
- Wesley是一套为经销商量身定制的全业务流程渠道 分销管理系统(手机APP称为经销商管家)
- Adaptive Autosar EM 标准规范
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


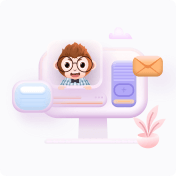
安全验证
文档复制为VIP权益,开通VIP直接复制
