package com.player.smallBear.utils;
import android.os.Handler;
import android.os.Looper;
import android.util.Log;
import androidx.annotation.CallSuper;
import androidx.annotation.IntRange;
import androidx.annotation.NonNull;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Timer;
import java.util.TimerTask;
import java.util.concurrent.ConcurrentHashMap;
import java.util.concurrent.CountDownLatch;
import java.util.concurrent.Executor;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.LinkedBlockingQueue;
import java.util.concurrent.RejectedExecutionException;
import java.util.concurrent.ThreadFactory;
import java.util.concurrent.ThreadPoolExecutor;
import java.util.concurrent.TimeUnit;
import java.util.concurrent.atomic.AtomicBoolean;
import java.util.concurrent.atomic.AtomicInteger;
import java.util.concurrent.atomic.AtomicLong;
/**
* <pre>
* author: Blankj
* blog : http://blankj.com
* time : 2018/05/08
* desc : utils about thread
* </pre>
*/
public final class ThreadUtils {
private static final Handler HANDLER = new Handler(Looper.getMainLooper());
private static final Map<Integer, Map<Integer, ExecutorService>> TYPE_PRIORITY_POOLS = new HashMap<>();
private static final Map<Task, ExecutorService> TASK_POOL_MAP = new ConcurrentHashMap<>();
private static final int CPU_COUNT = Runtime.getRuntime().availableProcessors();
private static final Timer TIMER = new Timer();
private static final byte TYPE_SINGLE = -1;
private static final byte TYPE_CACHED = -2;
private static final byte TYPE_IO = -4;
private static final byte TYPE_CPU = -8;
private static Executor sDeliver;
/**
* Return whether the thread is the main thread.
*
* @return {@code true}: yes<br>{@code false}: no
*/
public static boolean isMainThread() {
return Looper.myLooper() == Looper.getMainLooper();
}
public static Handler getMainHandler() {
return HANDLER;
}
public static void runOnUiThread(final Runnable runnable) {
if (Looper.myLooper() == Looper.getMainLooper()) {
runnable.run();
} else {
HANDLER.post(runnable);
}
}
public static void runOnUiThreadDelayed(final Runnable runnable, long delayMillis) {
HANDLER.postDelayed(runnable, delayMillis);
}
/**
* Return a thread pool that reuses a fixed number of threads
* operating off a shared unbounded queue, using the provided
* ThreadFactory to create new threads when needed.
*
* @param size The size of thread in the pool.
* @return a fixed thread pool
*/
public static ExecutorService getFixedPool(@IntRange(from = 1) final int size) {
return getPoolByTypeAndPriority(size);
}
/**
* Return a thread pool that reuses a fixed number of threads
* operating off a shared unbounded queue, using the provided
* ThreadFactory to create new threads when needed.
*
* @param size The size of thread in the pool.
* @param priority The priority of thread in the poll.
* @return a fixed thread pool
*/
public static ExecutorService getFixedPool(@IntRange(from = 1) final int size,
@IntRange(from = 1, to = 10) final int priority) {
return getPoolByTypeAndPriority(size, priority);
}
/**
* Return a thread pool that uses a single worker thread operating
* off an unbounded queue, and uses the provided ThreadFactory to
* create a new thread when needed.
*
* @return a single thread pool
*/
public static ExecutorService getSinglePool() {
return getPoolByTypeAndPriority(TYPE_SINGLE);
}
/**
* Return a thread pool that uses a single worker thread operating
* off an unbounded queue, and uses the provided ThreadFactory to
* create a new thread when needed.
*
* @param priority The priority of thread in the poll.
* @return a single thread pool
*/
public static ExecutorService getSinglePool(@IntRange(from = 1, to = 10) final int priority) {
return getPoolByTypeAndPriority(TYPE_SINGLE, priority);
}
/**
* Return a thread pool that creates new threads as needed, but
* will reuse previously constructed threads when they are
* available.
*
* @return a cached thread pool
*/
public static ExecutorService getCachedPool() {
return getPoolByTypeAndPriority(TYPE_CACHED);
}
/**
* Return a thread pool that creates new threads as needed, but
* will reuse previously constructed threads when they are
* available.
*
* @param priority The priority of thread in the poll.
* @return a cached thread pool
*/
public static ExecutorService getCachedPool(@IntRange(from = 1, to = 10) final int priority) {
return getPoolByTypeAndPriority(TYPE_CACHED, priority);
}
/**
* Return a thread pool that creates (2 * CPU_COUNT + 1) threads
* operating off a queue which size is 128.
*
* @return a IO thread pool
*/
public static ExecutorService getIoPool() {
return getPoolByTypeAndPriority(TYPE_IO);
}
/**
* Return a thread pool that creates (2 * CPU_COUNT + 1) threads
* operating off a queue which size is 128.
*
* @param priority The priority of thread in the poll.
* @return a IO thread pool
*/
public static ExecutorService getIoPool(@IntRange(from = 1, to = 10) final int priority) {
return getPoolByTypeAndPriority(TYPE_IO, priority);
}
/**
* Return a thread pool that creates (CPU_COUNT + 1) threads
* operating off a queue which size is 128 and the maximum
* number of threads equals (2 * CPU_COUNT + 1).
*
* @return a cpu thread pool for
*/
public static ExecutorService getCpuPool() {
return getPoolByTypeAndPriority(TYPE_CPU);
}
/**
* Return a thread pool that creates (CPU_COUNT + 1) threads
* operating off a queue which size is 128 and the maximum
* number of threads equals (2 * CPU_COUNT + 1).
*
* @param priority The priority of thread in the poll.
* @return a cpu thread pool for
*/
public static ExecutorService getCpuPool(@IntRange(from = 1, to = 10) final int priority) {
return getPoolByTypeAndPriority(TYPE_CPU, priority);
}
/**
* Executes the given task in a fixed thread pool.
*
* @param size The size of thread in the fixed thread pool.
* @param task The task to execute.
* @param <T> The type of the task's result.
*/
public static <T> void executeByFixed(@IntRange(from = 1) final int size, final Task<T> task) {
execute(getPoolByTypeAndPriority(size), task);
}
/**
* Executes the given task in a fixed thread pool.
*
* @param size The size of thread in the fixed thread pool.
* @param task The task to execute.
* @param priority The priority of thread in the poll.
* @param <T> The type of the task's result.
*/
public static <T> void executeByFixed(@IntRange(from = 1) final int size,
final Task<T> task,
@IntRange(from = 1, to = 10) final int priority) {
execute(getPoolByTypeAndPriority(size, priority), task);
}
/**
* Executes the given task in a fixed thread pool after the given delay.
*
* @param size The size of thread in the fixed thread pool.
* @param task The task to execute.
* @param delay The time from now to delay execution.
* @param unit The time unit of the delay parameter.
* @param <T> The type of the task's result.
*/
public static <T> void executeByFixedWithDelay(@IntRange(from = 1) final int size,
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
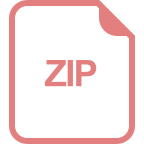
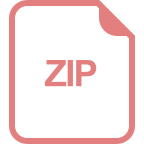
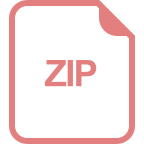
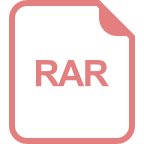
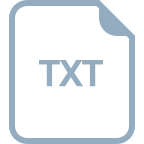
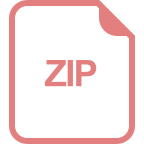
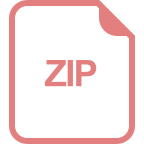
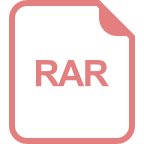
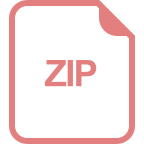
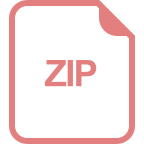
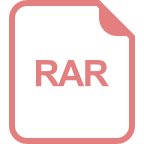
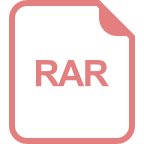
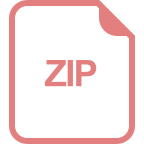
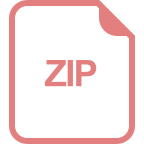
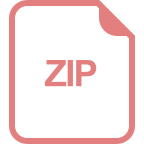
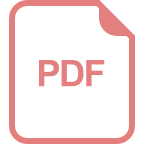
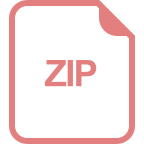
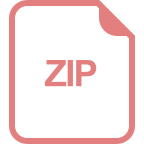
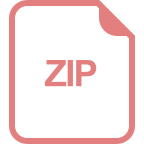
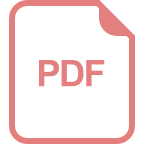
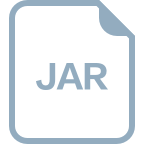
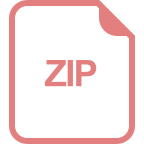
收起资源包目录

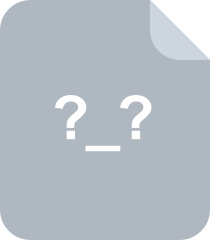
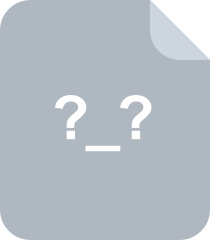
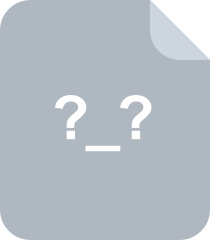
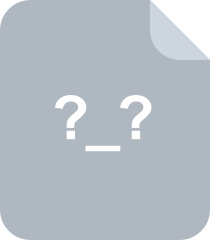
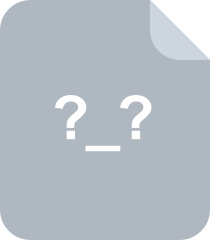
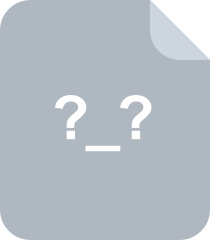
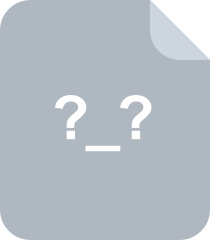
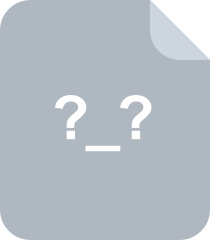
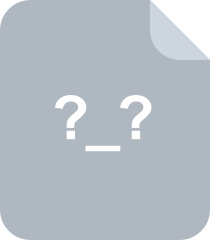
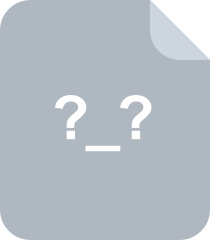
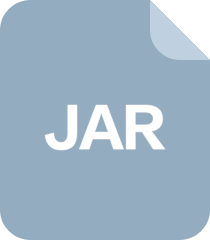
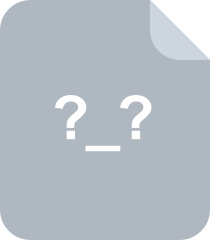
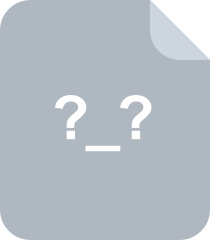
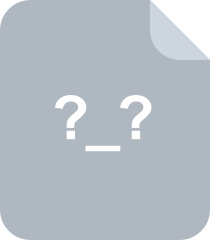
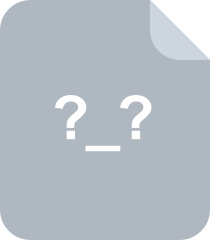
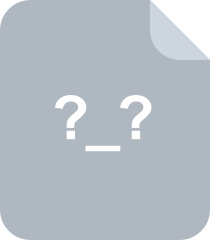
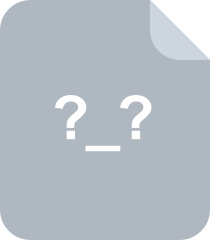
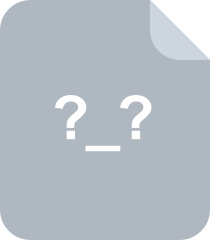
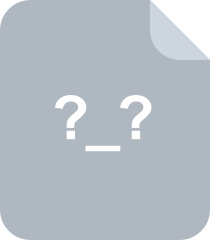
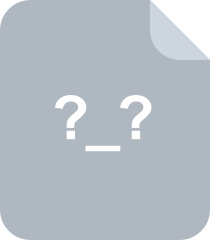
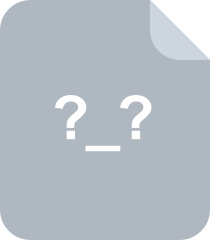
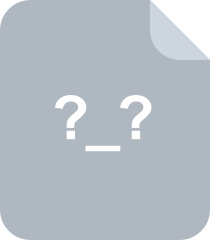
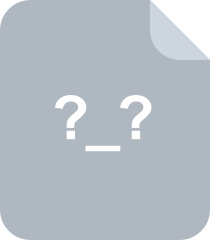
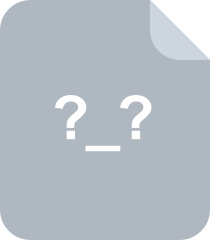
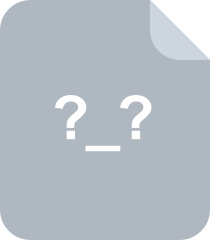
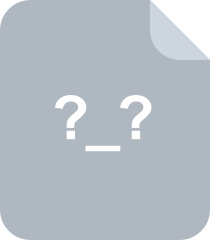
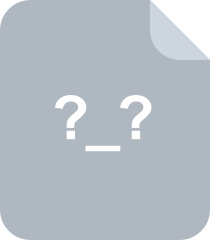
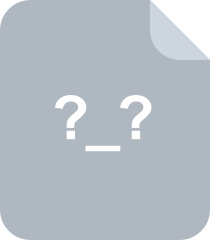
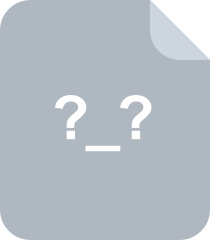
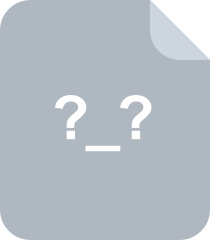
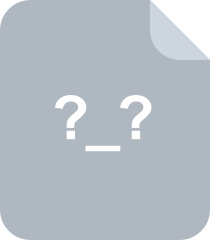
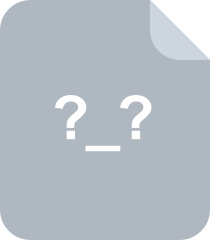
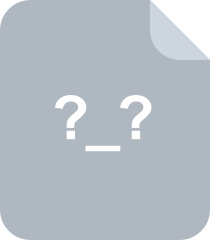
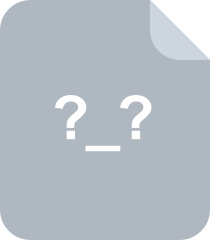
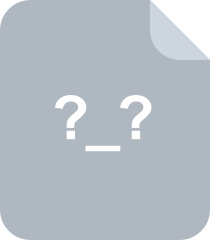
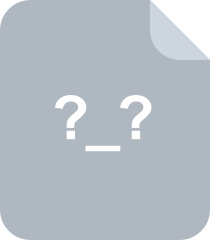
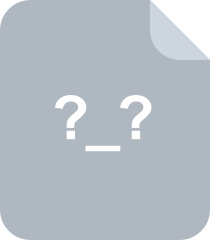
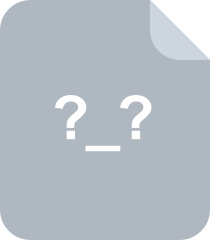
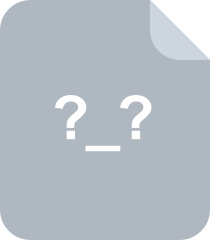
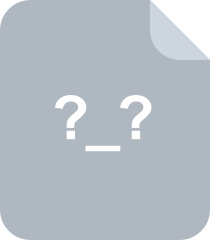
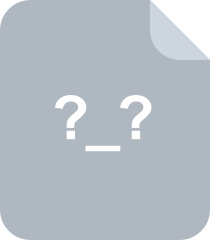
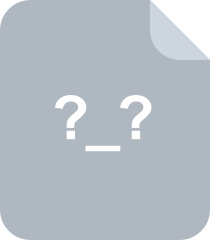
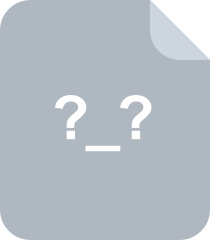
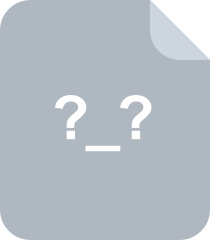
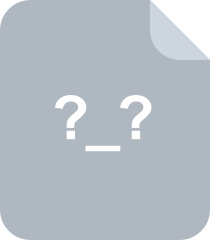
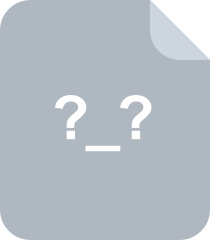
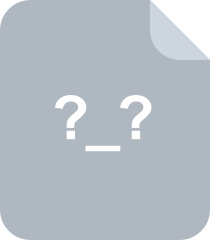
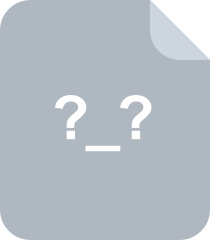
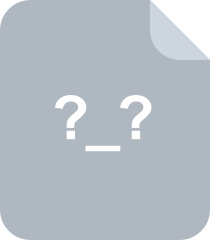
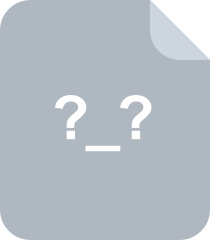
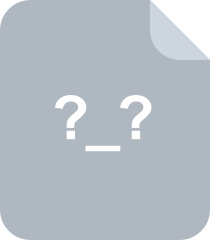
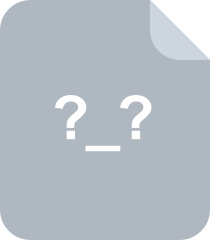
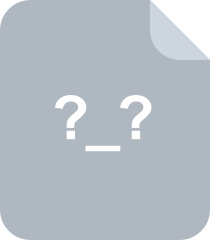
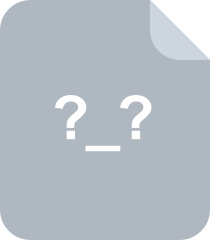
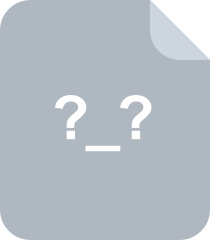
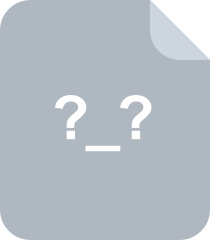
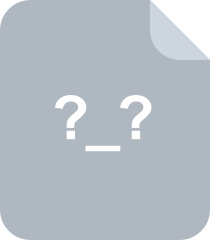
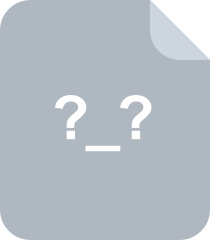
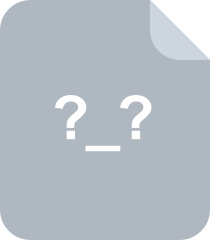
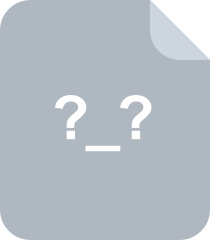
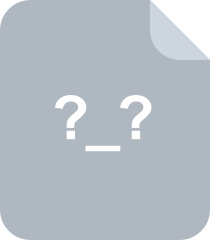
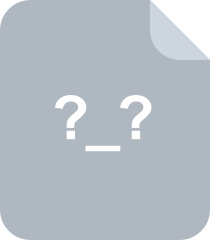
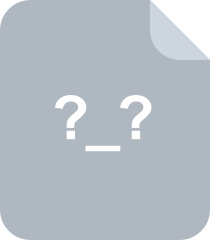
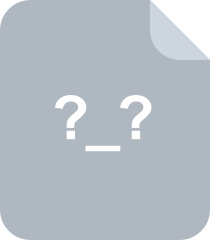
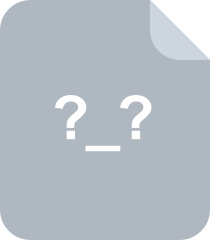
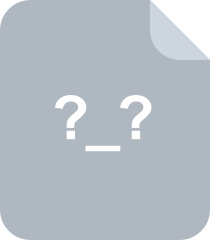
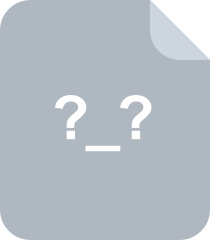
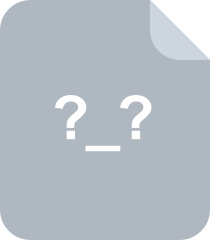
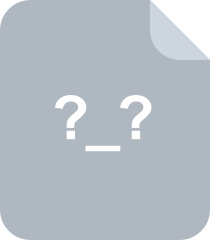
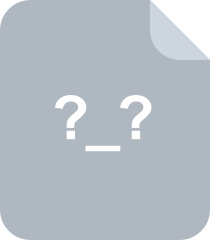
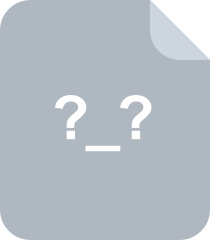
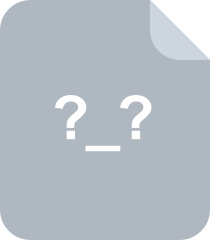
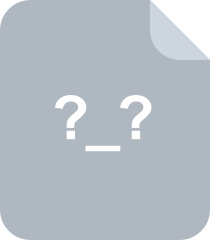
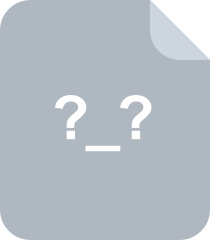
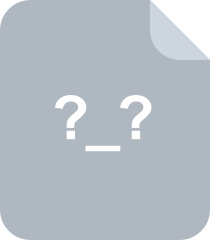
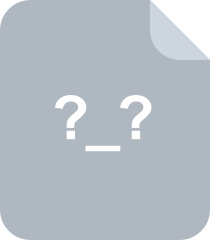
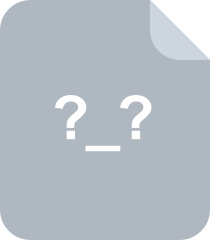
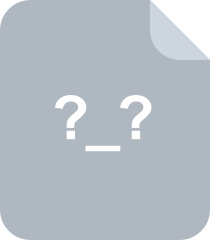
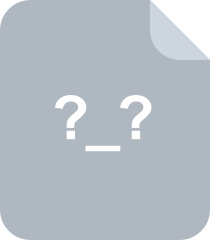
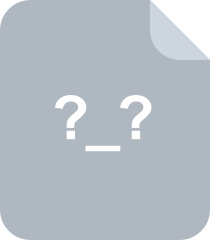
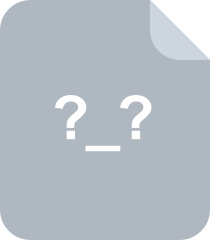
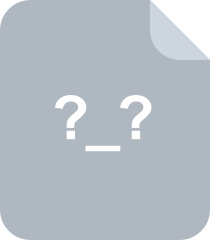
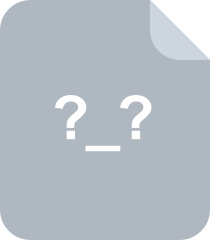
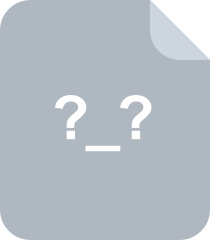
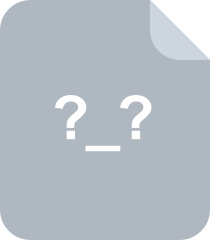
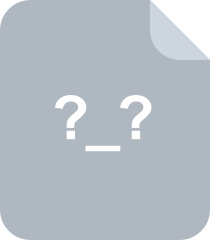
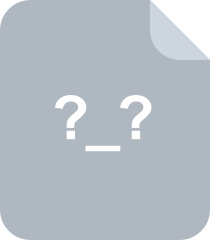
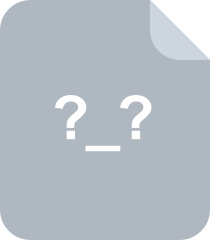
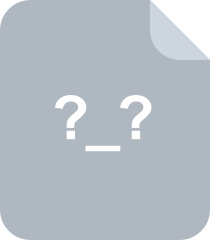
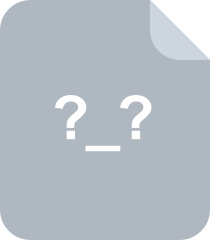
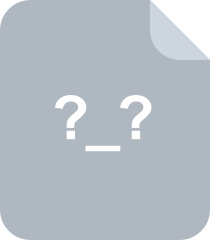
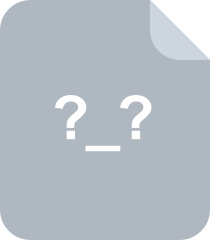
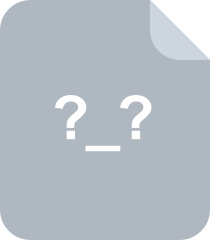
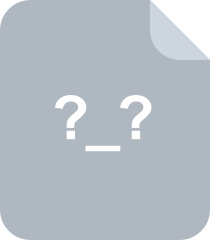
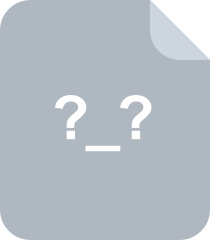
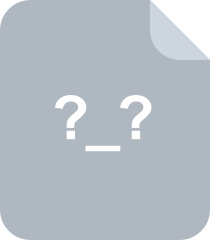
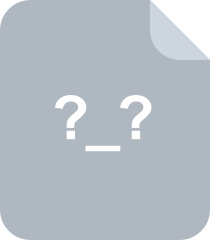
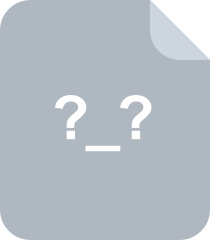
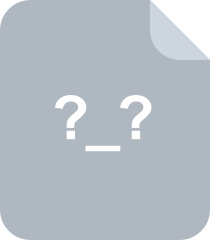
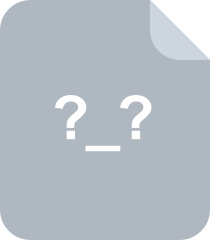
共 310 条
- 1
- 2
- 3
- 4
资源评论
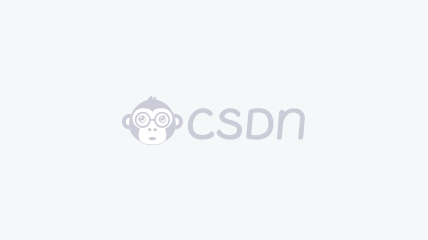

xyq2024
- 粉丝: 1897
- 资源: 4620
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

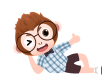
最新资源
- 基于JavaScript的PHP和Python多语言支持的其他box设计源码
- UCOSII文档UCOSII中文注释版及其资料
- 基于Java语言的自定义IndicatorSeekBar设计源码
- 基于Python编程的宝宝诗词题库设计源码
- UCOSII文档UCOSII在STM32上面的移植详解
- 基于Python的实时交通系统光电杯混分设计源码
- 基于SpringBoot3和Vue3的漫画管理平台设计源码
- 基于Springboot框架的垃圾分类后台管理系统设计源码
- 基于Java的阿里巴巴easyExcel设计源码解析与应用实践
- 基于永磁同步电机模型参考自适应MRAS学习参考模型 复现华科lunwen中的模型,有公式推导和原理解释
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


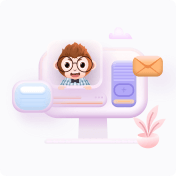
安全验证
文档复制为VIP权益,开通VIP直接复制
