package com.gym.utils;
import javax.crypto.Cipher;
import javax.crypto.KeyGenerator;
import javax.crypto.SecretKey;
import javax.crypto.spec.IvParameterSpec;
import javax.crypto.spec.SecretKeySpec;
import java.security.*;
import java.security.interfaces.RSAPrivateKey;
import java.security.interfaces.RSAPublicKey;
import java.security.spec.PKCS8EncodedKeySpec;
import java.security.spec.X509EncodedKeySpec;
import java.util.Base64;
import java.util.HashMap;
/**
* @author Feri
* 实现常用的加解密 方法,支持 SHA AES RSA Base64
* ━━━━━━神兽出没━━━━━━
* ┏┓ ┏┓
* ┏┛┻━━━┛┻┓
* ┃ ┃
* ┃ ━ ┃
* ┃ ┳┛ ┗┳ ┃
* ┃ ┃
* ┃ ┻ ┃
* ┃ ┃
* ┗━┓ ┏━┛Code is far away from bug with the animal protecting
* ┃ ┃ 神兽保佑,代码无bug
* ┃ ┃
* ┃ ┗━━━┓
* ┃ ┣┓
* ┃ ┏┛
* ┗┓┓┏━┳┓┏┛
* ┃┫┫ ┃┫┫
* ┗┻┛ ┗┻┛
* <p>
* ━━━━━━感觉萌萌哒━━━━━━
*/
public class PassUtils {
public static final String SHA1 = "SHA-1";
public static final String SHA256 = "SHA-256";
public static final String SHA384 = "SHA-384";
public static final String SHA512 = "SHA-512";
public static final String PUBKEY = "public_key";
public static final String PRIKEY = "private_key";
//1、编码格式
//base64 编码
public static String base64enc(String msg) {
return Base64.getEncoder ().encodeToString (msg.getBytes ());
}
private static String base64encByte(byte[] msg) {
return Base64.getEncoder ().encodeToString (msg);
}
private static byte[] base64decByte(String msg) {
return Base64.getDecoder ().decode (msg);
}
//
// base64 解码
public static String base64dec(String msg) {
return new String (Base64.getDecoder ().decode (msg));
}
//MD5 摘要
public static String md5(String msg) {
try {
//创建摘要算法对象
MessageDigest messageDigest = MessageDigest.getInstance ("MD5");
messageDigest.update (msg.getBytes ());
return base64encByte (messageDigest.digest ());
} catch (NoSuchAlgorithmException e) {
e.printStackTrace ();
}
return null;
}
//SHA 摘要 SHA-1 SHA-256 SHA-384 SHA-512
public static String sha(String type, String msg) {
try {
//创建摘要算法对象
MessageDigest messageDigest = MessageDigest.getInstance (type);
messageDigest.update (msg.getBytes ());
return base64encByte (messageDigest.digest ());
} catch (NoSuchAlgorithmException e) {
e.printStackTrace ();
}
return null;
}
//创建 对称加密---密钥
public static String createAESKey() {
try {
//1、创建随机key
KeyGenerator generator = KeyGenerator.getInstance ("AES");
generator.init (128);
SecretKey key = generator.generateKey ();
return base64encByte (key.getEncoded ());
} catch (NoSuchAlgorithmException e) {
e.printStackTrace ();
}
return null;
}
//AES 加密 返回的是base64格式
public static String aesenc(String key, String msg) {
SecretKeySpec secretKeySpec = new SecretKeySpec (base64decByte (key), "AES");
try {
Cipher cipher = Cipher.getInstance ("AES");
cipher.init (Cipher.ENCRYPT_MODE, secretKeySpec);
return base64encByte (cipher.doFinal (msg.getBytes ()));
} catch (Exception e) {
e.printStackTrace ();
}
return null;
}
//AES 解密 返回的是base64格式
public static String aesdec(String key, String msg) {
SecretKeySpec secretKeySpec = new SecretKeySpec (base64decByte (key), "AES");
try {
Cipher cipher = Cipher.getInstance ("AES");
cipher.init (Cipher.DECRYPT_MODE, secretKeySpec);
return new String (cipher.doFinal (base64decByte (msg)));
} catch (Exception e) {
e.printStackTrace ();
}
return null;
}
//创建-RSA 密钥 一对儿 公私钥
public static HashMap<String, String> createRSAKey() {
try {
KeyPairGenerator generator = KeyPairGenerator.getInstance ("RSA");
KeyPair keyPair = generator.generateKeyPair ();
//创建使用私钥
RSAPrivateKey privateKey = (RSAPrivateKey) keyPair.getPrivate ();
//创建使用公钥
RSAPublicKey publicKey = (RSAPublicKey) keyPair.getPublic ();
HashMap<String, String> keys = new HashMap<> ();
keys.put (PUBKEY, base64encByte (publicKey.getEncoded()));
keys.put (PRIKEY, base64encByte (privateKey.getEncoded ()));
return keys;
} catch (NoSuchAlgorithmException e) {
e.printStackTrace ();
}
return null;
}
/**
* rsa 公钥-加密*/
public static String rsaEncPub(String key,String msg){
X509EncodedKeySpec keySpec = new X509EncodedKeySpec(base64decByte(key));
try {
KeyFactory keyFactory = KeyFactory.getInstance ("RSA");
PublicKey publicKey = keyFactory.generatePublic (keySpec);
Cipher cipher = Cipher.getInstance ("RSA");
cipher.init (Cipher.ENCRYPT_MODE, publicKey);
return base64encByte(cipher.doFinal(msg.getBytes()));
} catch (Exception e) {
e.printStackTrace();
}
return null;
}
//RSA 私钥-加密
public static String rsaEnc(String key, String msg) {
try {
//转换私钥
PKCS8EncodedKeySpec keySpec = new PKCS8EncodedKeySpec (base64decByte (key));
KeyFactory keyFactory = KeyFactory.getInstance ("RSA");
PrivateKey privateKey = keyFactory.generatePrivate (keySpec);
Cipher cipher = Cipher.getInstance ("RSA");
cipher.init (Cipher.ENCRYPT_MODE, privateKey);
return base64encByte(cipher.doFinal(msg.getBytes()));
} catch (Exception e) {
e.printStackTrace ();
}
return null;
}
//RSA 公钥-解密
public static String rsaDec(String key, String msg) {
try {
//转换公钥
X509EncodedKeySpec keySpec = new X509EncodedKeySpec (base64decByte (key));
KeyFactory keyFactory = KeyFactory.getInstance ("RSA");
PublicKey publicKey = keyFactory.generatePublic (keySpec);
Cipher cipher = Cipher.getInstance ("RSA");
cipher.init (Cipher.DECRYPT_MODE, publicKey);
return new String (cipher.doFinal (base64decByte (msg)), "UTF-8");
} catch (Exception e) {
e.printStackTrace ();
}
return null;
}
/**
* RSA 私钥-解密
* @param key 私钥
* @param msg 解密的密文
*/
public static String rsaDecPri(String key, String msg) {
try {
//转换私钥
PKCS8EncodedKeySpec keySpec = new PKCS8EncodedKeySpec (base64decByte (key));
KeyFactory keyFactory = KeyFactory.getInstance ("RSA");
PrivateKey privateKey = keyFactory.generatePrivate (keySpec);
Cipher cipher = Cipher.getInstance ("RSA");
cipher.init (Cipher.DECRYPT_MODE, privateKey);
return new String(cipher.doFinal(base64decByte(msg)),"UTF-8");
} catch (Exception e) {
e.printStackTrace ()
没有合适的资源?快使用搜索试试~ 我知道了~
基于甲骨文体育馆灵感的现代化体育馆网页设计源码
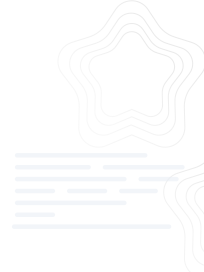
共403个文件
java:161个
html:86个
xml:30个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 166 浏览量
2024-10-04
10:12:27
上传
评论
收藏 9.54MB ZIP 举报
温馨提示
该项目为甲骨文体育馆现代化设计的体育馆网页源码,包含403个文件,涵盖161个Java源文件、86个HTML文件、30个XML文件、23个JavaScript文件、20个PNG图像文件、18个CSS样式文件以及多种其他文件类型,旨在通过融合甲骨文体育馆的设计灵感,打造一个现代化的体育馆网页界面。
资源推荐
资源详情
资源评论
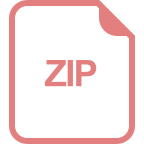
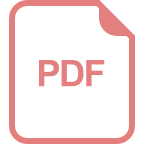
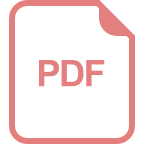
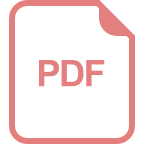
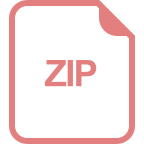
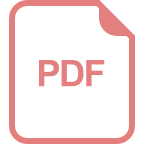
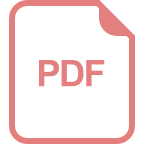
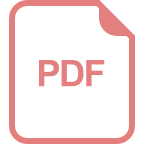
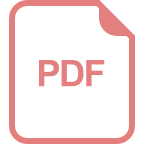
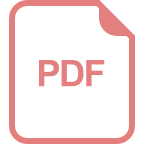
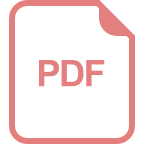
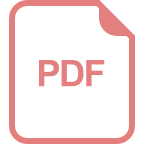
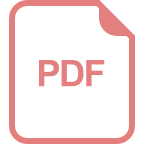
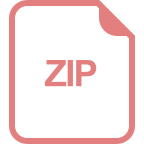
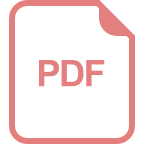
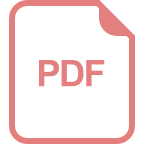
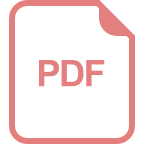
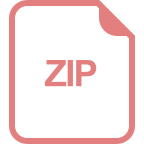
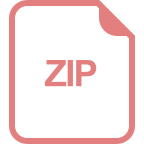
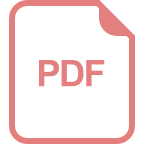
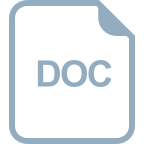
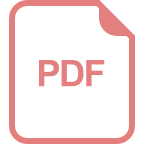
收起资源包目录

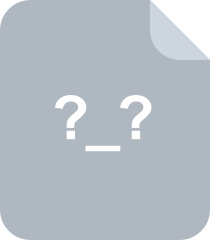
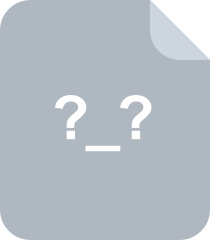
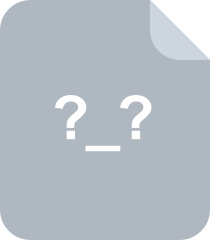
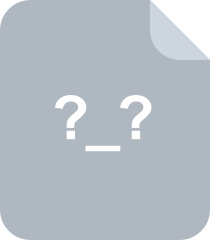
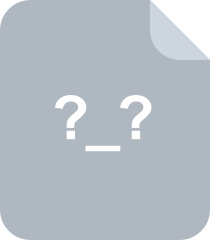
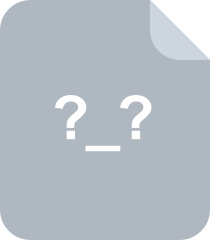
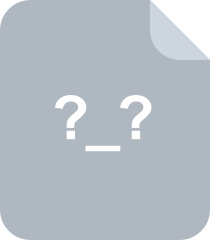
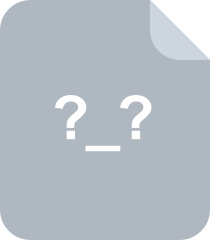
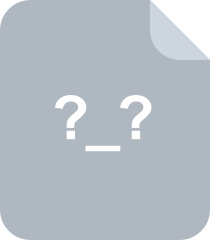
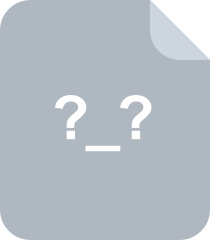
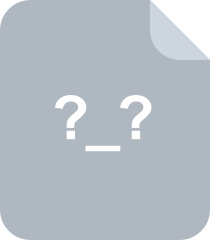
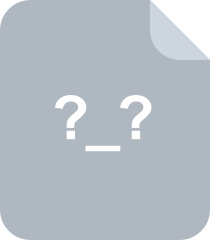
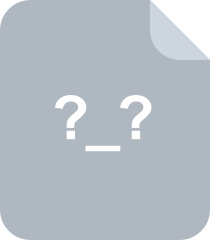
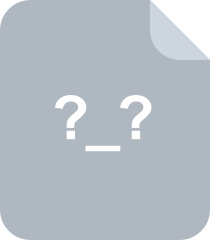
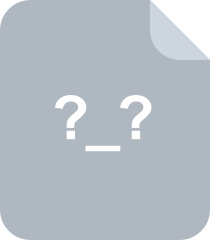
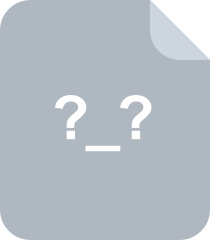
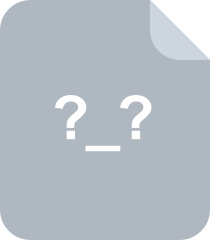
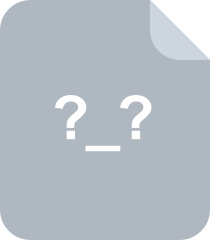
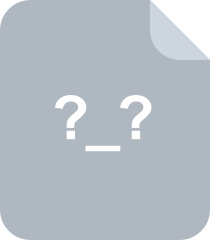
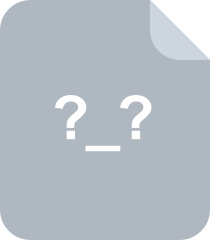
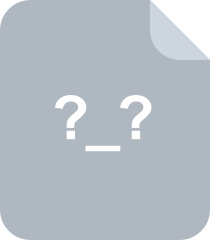
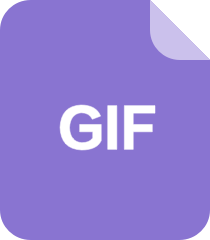
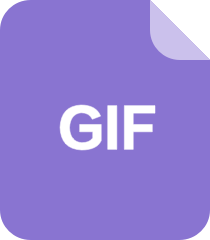
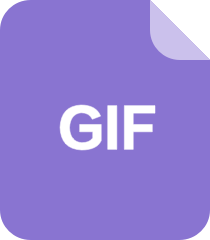
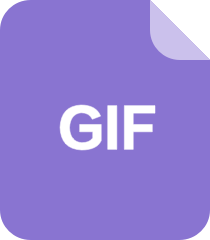
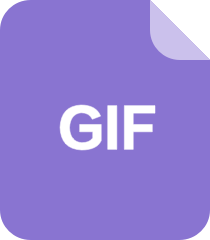
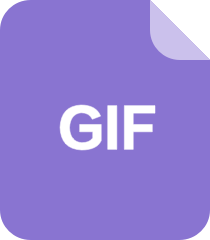
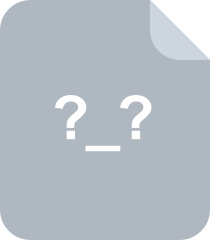
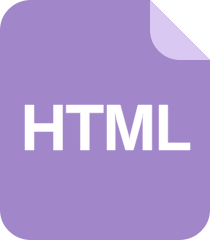
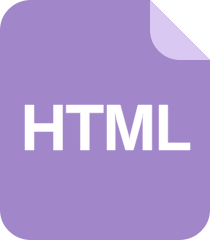
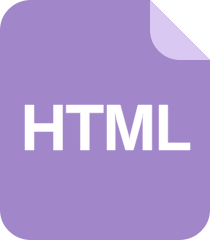
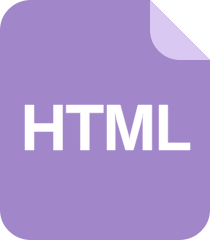
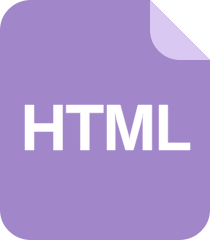
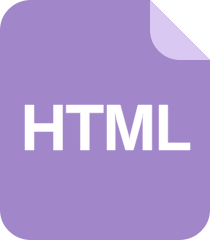
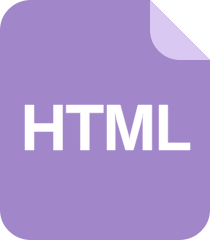
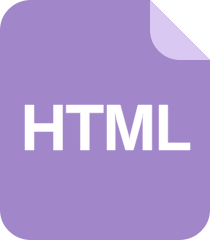
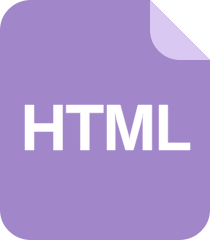
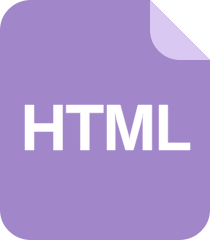
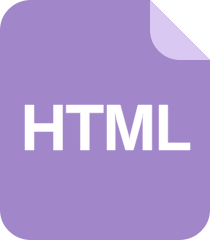
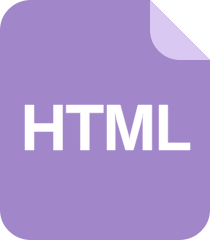
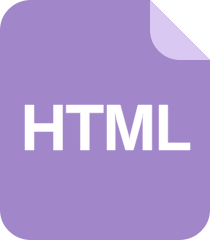
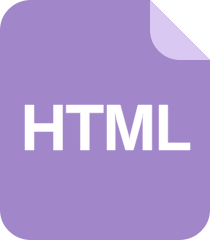
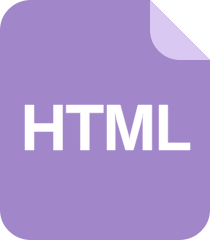
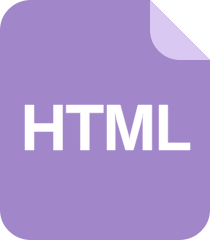
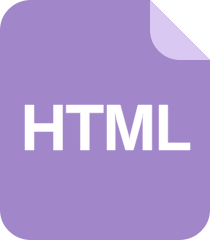
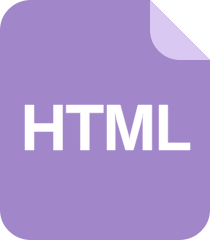
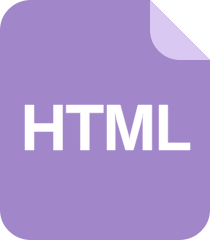
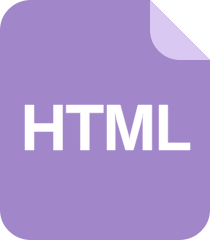
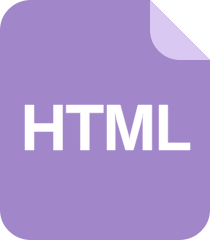
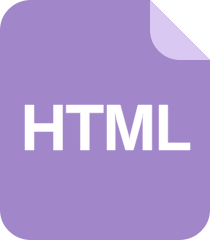
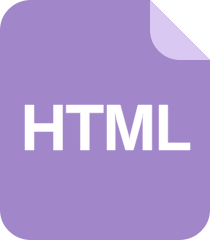
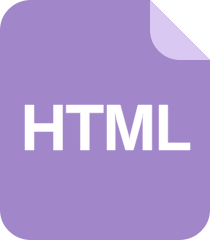
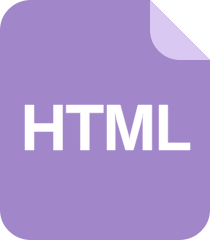
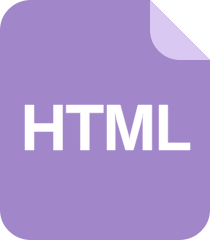
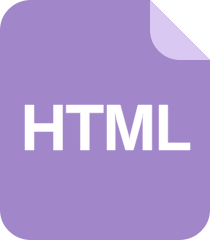
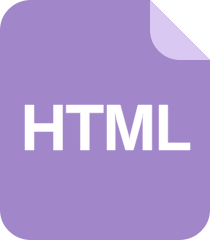
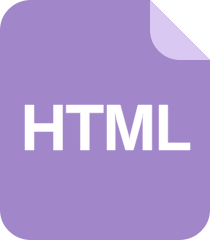
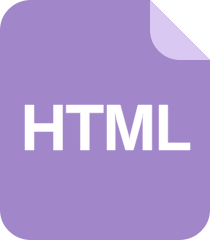
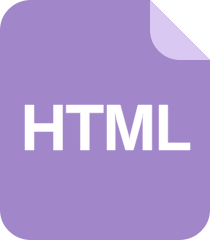
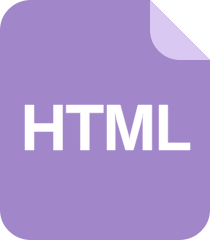
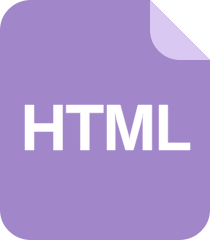
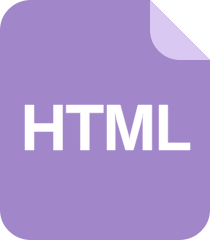
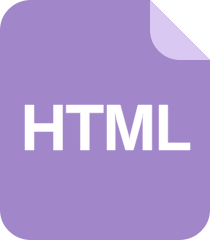
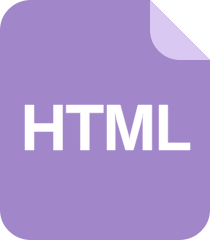
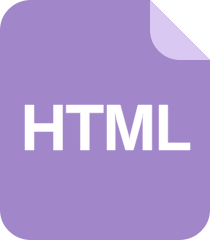
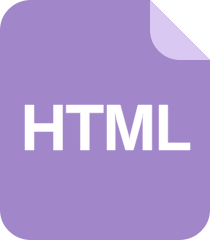
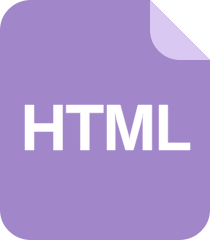
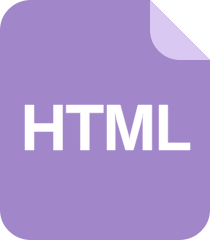
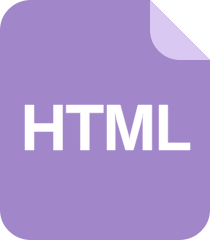
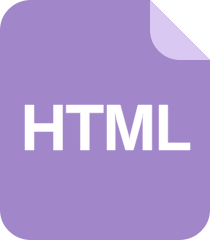
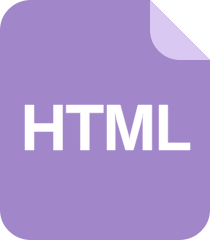
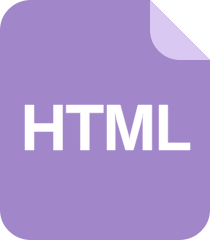
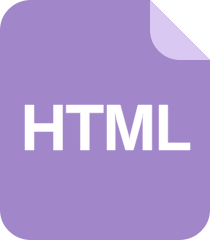
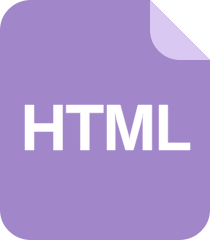
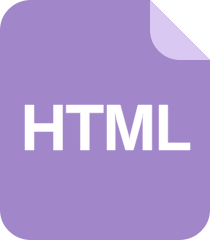
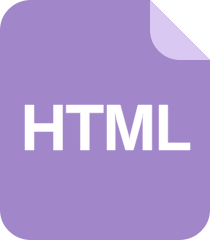
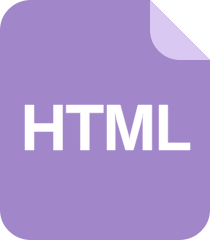
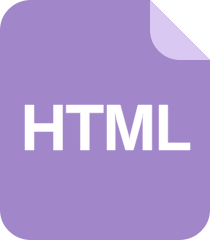
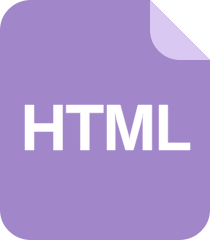
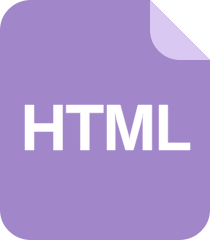
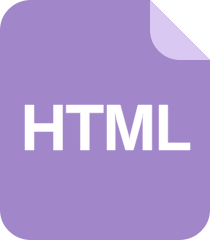
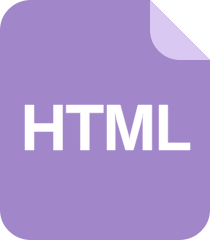
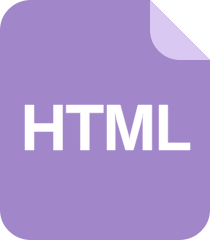
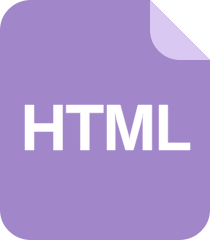
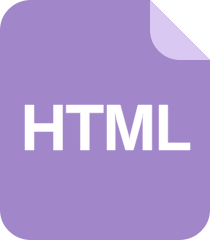
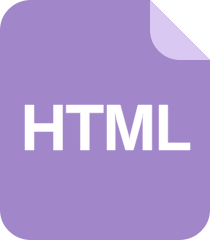
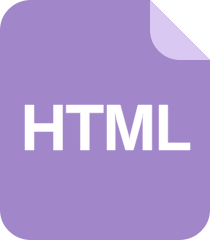
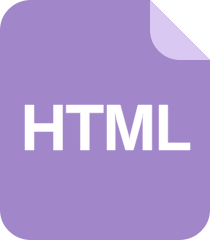
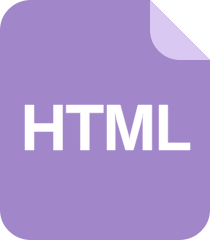
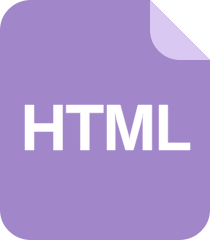
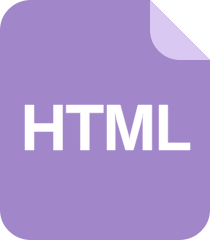
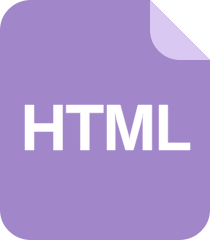
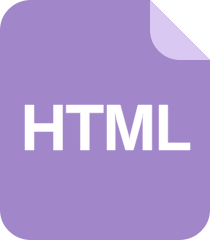
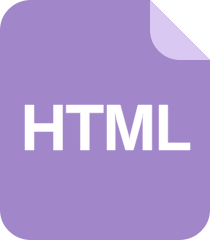
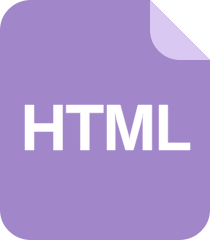
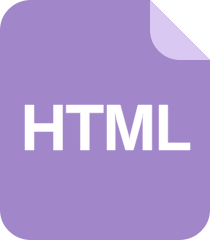
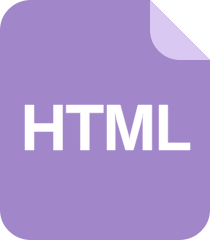
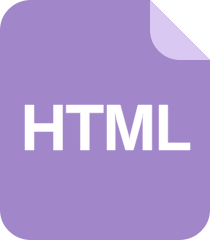
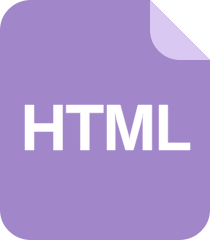
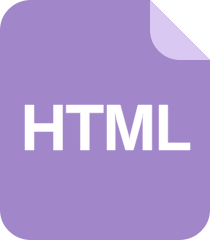
共 403 条
- 1
- 2
- 3
- 4
- 5
资源评论
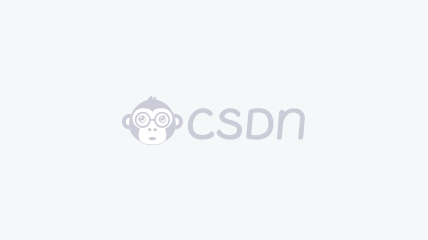

xyq2024
- 粉丝: 2713
- 资源: 5485
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

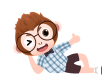
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


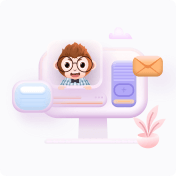
安全验证
文档复制为VIP权益,开通VIP直接复制
