/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.oszero.deliver.admin.service.impl;
import com.baomidou.mybatisplus.core.conditions.query.LambdaQueryWrapper;
import com.oszero.deliver.admin.enums.DateSelectEnum;
import com.oszero.deliver.admin.exception.BusinessException;
import com.oszero.deliver.admin.model.dto.request.DashboardDateSelectRequestDto;
import com.oszero.deliver.admin.model.dto.response.DashboardHeadDataResponseDto;
import com.oszero.deliver.admin.model.dto.response.DashboardInfoResponseDto;
import com.oszero.deliver.admin.model.dto.response.MessageInfoResponseReactDto;
import com.oszero.deliver.admin.model.dto.response.MessageInfoResponseVueDto;
import com.oszero.deliver.admin.model.entity.MessageRecord;
import com.oszero.deliver.admin.service.*;
import lombok.RequiredArgsConstructor;
import org.springframework.stereotype.Service;
import java.time.*;
import java.time.temporal.TemporalAdjusters;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
import java.util.stream.Collectors;
/**
* 仪表盘 serviceImpl
*
* @author oszero
* @version 1.0.0
*/
@Service
@RequiredArgsConstructor
public class DashboardServiceImpl implements DashboardService {
private final MessageRecordService messageRecordService;
private final TemplateService templateService;
private final AppService appService;
private final PlatformFileService platformFileService;
/**
* 获取数据面板头数据
*
* @return dto
*/
@Override
public DashboardHeadDataResponseDto getDashboardHeadData() {
// 获取当日的本地日期
LocalDate today = LocalDate.now();
// 获取当日的开始时间(凌晨)
LocalDateTime startOfDay = today.atStartOfDay();
// 获取当日的结束时间(最后一刻)
LocalDateTime endOfDay = today.atTime(23, 59, 59, 999999999);
// 将本地时间转换为ZonedDateTime以便处理时区信息
ZoneId zoneId = ZoneId.systemDefault();
ZonedDateTime startOfDayZoned = startOfDay.atZone(zoneId);
ZonedDateTime endOfDayZoned = endOfDay.atZone(zoneId);
LambdaQueryWrapper<MessageRecord> wrapper = new LambdaQueryWrapper<>();
wrapper.ge(MessageRecord::getCreateTime, startOfDayZoned).lt(MessageRecord::getCreateTime, endOfDayZoned);
long messageCount = messageRecordService.count(wrapper);
long platformFiles = platformFileService.count();
long templateCount = templateService.count();
long appCount = appService.count();
return DashboardHeadDataResponseDto.builder()
.numberOfMessagesToday(String.valueOf(messageCount))
.numberOfPlatformFiles(String.valueOf(platformFiles))
.accumulatedTemplateOwnership(String.valueOf(templateCount))
.numberOfApps(String.valueOf(appCount)).build();
}
/**
* 获取消息柱状图
*
* @param dto 日期选择
* @return 柱状图信息
*/
@Override
public MessageInfoResponseVueDto getMessageInfoVue(DashboardDateSelectRequestDto dto) {
Integer dateSelect = dto.getDateSelect();
DateSelectEnum instanceByCode = DateSelectEnum.getInstanceByCode(dateSelect);
MessageInfoResponseVueDto messageInfoResponseVueDto = new MessageInfoResponseVueDto();
List<List<Object>> messageInfoList = new ArrayList<>();
if (Objects.isNull(instanceByCode)) {
throw new BusinessException("传递的日期选择错误,在 1-4!!!");
}
// 进行具体处理
switch (instanceByCode) {
case DAY: {
// 初始化一个基础时间,当日凌晨
LocalDateTime baseTime = LocalDateTime.now().with(LocalTime.MIDNIGHT);
for (int i = 0; i < 6; i++) {
LocalDateTime startTime = baseTime.plusHours(i * 4); // 起始时间
LocalDateTime endTime = baseTime.plusHours((i + 1) * 4); // 结束时间
// 获取消息数量
Long successCount = getMessageCountByTime(startTime, endTime, 1);
Long failCount = getMessageCountByTime(startTime, endTime, 0);
// 组装返回值
List<Object> ans = new ArrayList<>();
ans.add(i * 4 + "-" + (1 + i) * 4 + "h");
ans.add(successCount);
ans.add(failCount);
messageInfoList.add(ans);
}
break;
}
case WEEK: {
// 得到这周开始日
LocalDateTime weekStart = LocalDateTime.now().with(TemporalAdjusters.previousOrSame(DayOfWeek.MONDAY)).toLocalDate().atTime(0, 0, 0, 0);
for (int i = 0; i < 7; i++) {
// 获取开始结束时间
LocalDateTime startTime = weekStart.plusDays(i);
LocalDateTime endTime = startTime.plusDays(1);
// 获取消息数量
Long successCount = getMessageCountByTime(startTime, endTime, 1);
Long failCount = getMessageCountByTime(startTime, endTime, 0);
// 组装返回值
List<Object> ans = new ArrayList<>();
ans.add("Week " + (i + 1));
ans.add(successCount);
ans.add(failCount);
messageInfoList.add(ans);
}
break;
}
case MONTH: {
// 初始化一个基础时间,当月的第一天与最后一天
LocalDate baseDate = LocalDate.now().withDayOfMonth(1);
LocalDate lastDate = LocalDate.now().with(TemporalAdjusters.lastDayOfMonth());
for (int i = 0; i < 4; i++) {
LocalDate periodStart = baseDate.plusDays(i * 7);
LocalDate periodEnd = baseDate.plusDays((i + 1) * 7).isAfter(lastDate) ? lastDate : baseDate.plusDays((i + 1) * 7);
// 获取开始结束时间
LocalDateTime startTime = periodStart.atStartOfDay();
LocalDateTime endTime = periodEnd.atStartOfDay();
// 获取消息数量
Long successCount = getMessageCountByTime(startTime, endTime, 1);
Long failCount = getMessageCountByTime(startTime, endTime, 0);
// 组装返回值
List<Object> ans = new ArrayList<>();
ans.add((i * 7 + 1) + "-" + (i + 1) * 7 + "日");
ans.add(successCount);
ans.add(failCount);
messageInfoList.add(ans);
}
// 处理不足 7 天的剩余部分
if (lastDate.getDayOfMonth() - baseDate.plusDays(28).getDayOfMonth() > 0) {
LocalDate remainingStart = baseDate.plusDays(28);
LocalDateTime startTime = remainingStart.atStartOfDay();
LocalDateTime endTime = lastDate.atStartOfDay();
Long successCoun
没有合适的资源?快使用搜索试试~ 我知道了~
基于SpringBoot3的轻量级企业级消息推送平台Deliver设计源码
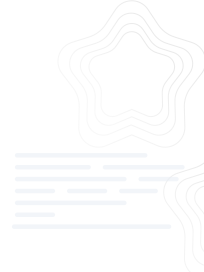
共441个文件
java:311个
ts:32个
vue:24个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 57 浏览量
2024-10-01
19:37:54
上传
评论
收藏 3.23MB ZIP 举报
温馨提示
该项目是一款基于SpringBoot3构建的轻量级企业级消息推送平台Deliver的设计源码。该项目包含441个文件,涵盖311个Java源文件、32个TypeScript脚本文件、24个Vue组件文件、19个PNG图片文件、18个XML配置文件、9个YAML配置文件、5个JSON数据文件、3个Shell脚本文件、2个Git忽略文件、2个CommonJS模块文件。Deliver平台旨在为企业提供高效、便捷的消息传递和通知服务,支持多种通信渠道,是内部沟通和协作的理想解决方案。
资源推荐
资源详情
资源评论
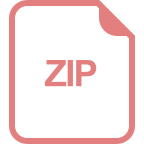
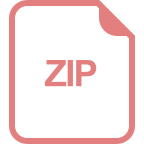
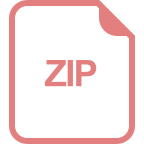
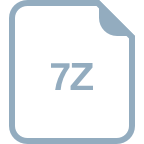
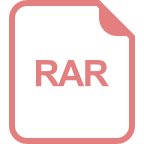
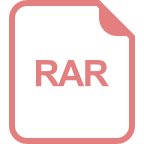
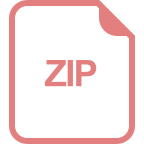
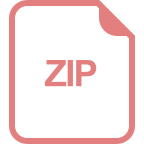
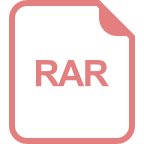
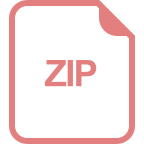
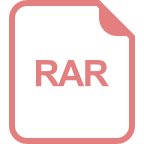
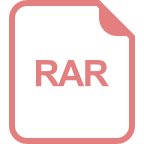
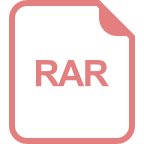
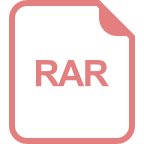
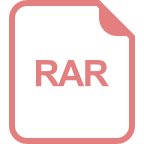
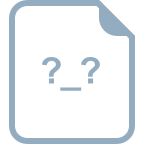
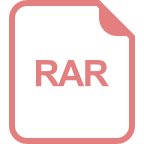
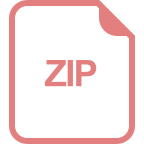
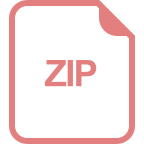
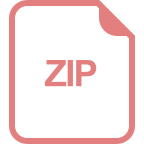
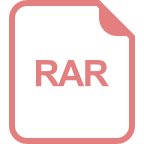
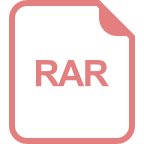
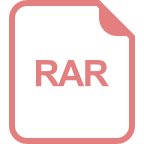
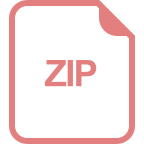
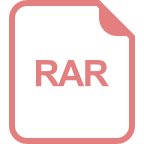
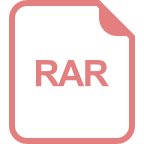
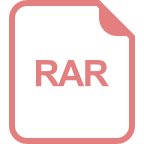
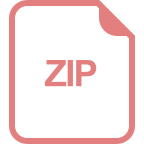
收起资源包目录

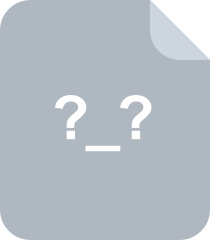
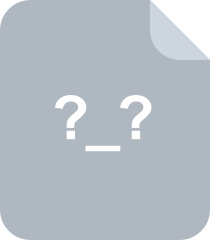
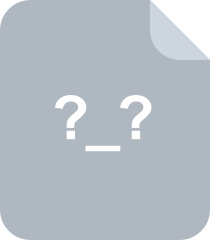
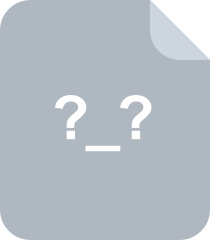
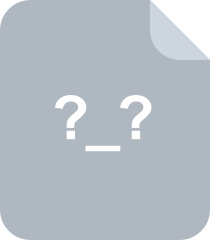
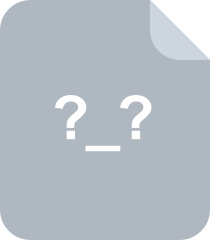
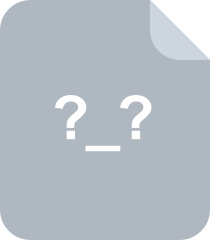
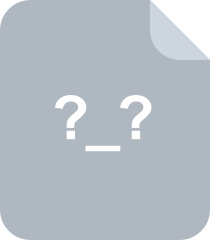
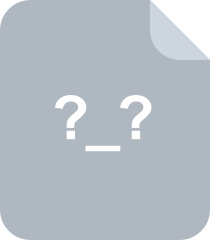
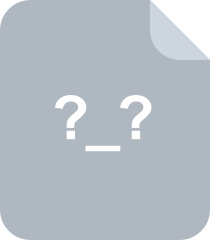
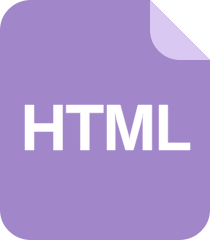
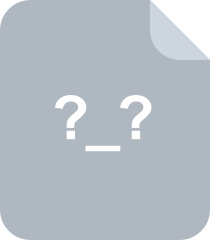
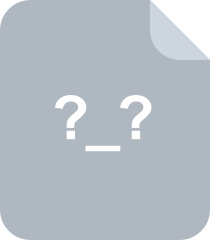
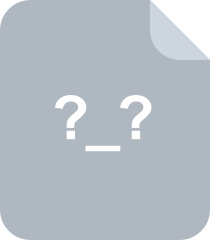
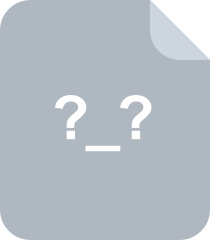
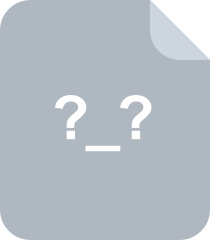
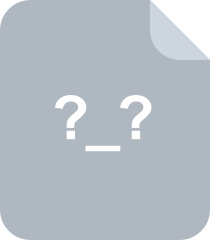
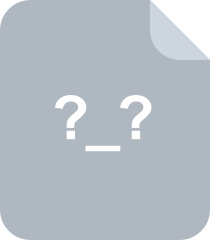
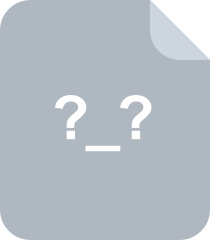
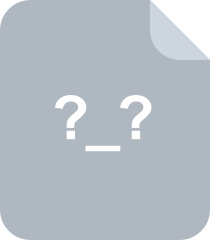
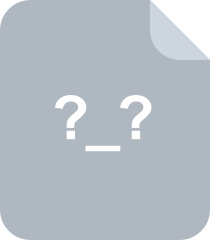
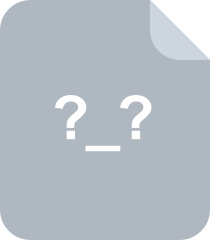
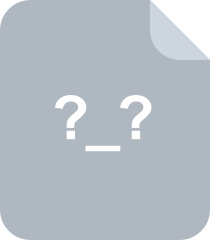
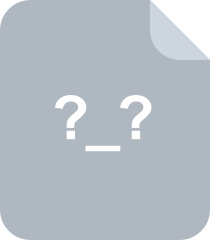
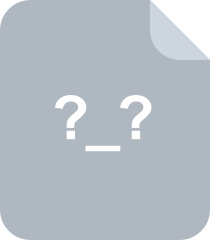
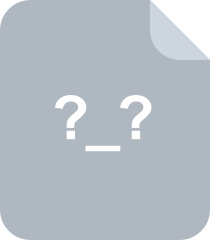
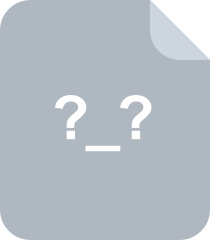
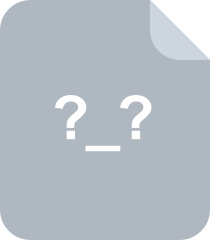
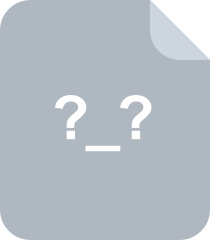
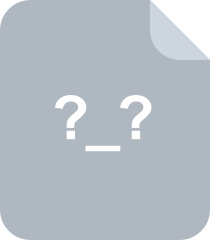
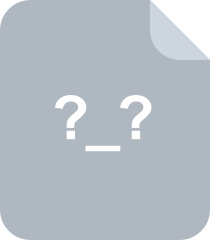
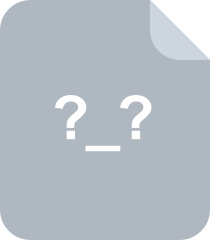
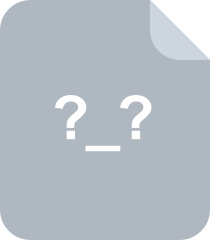
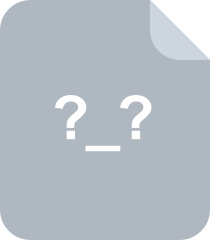
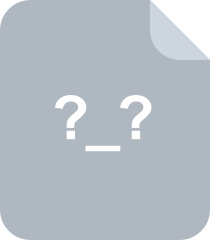
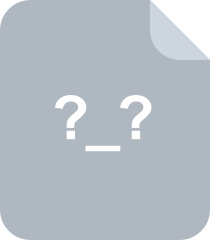
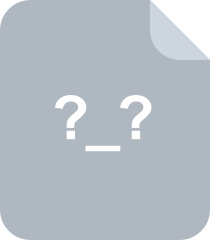
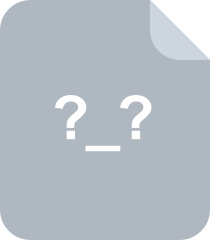
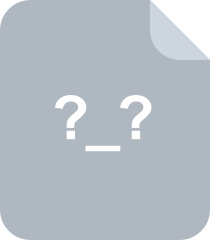
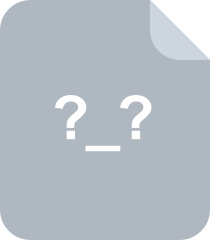
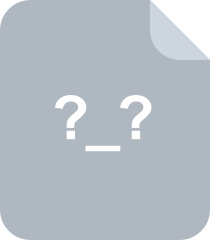
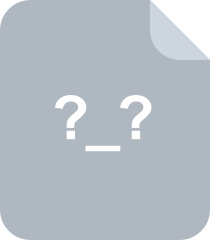
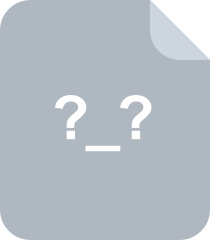
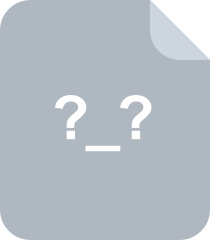
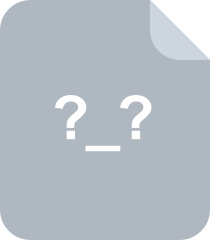
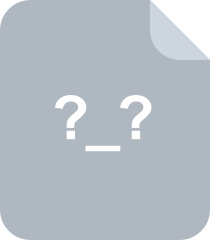
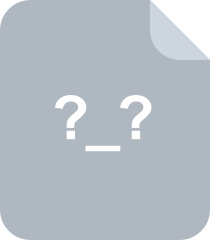
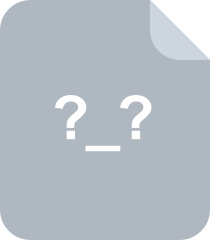
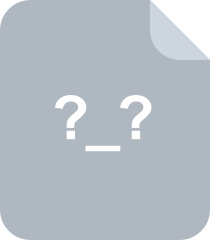
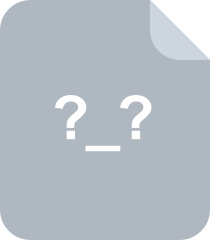
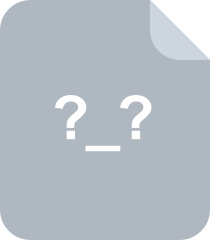
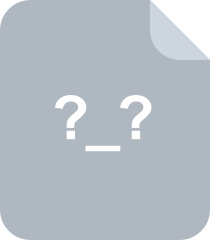
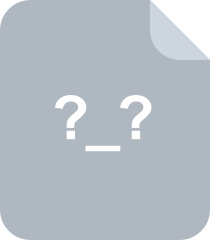
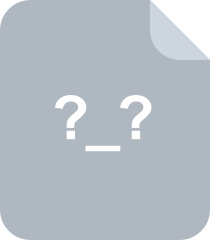
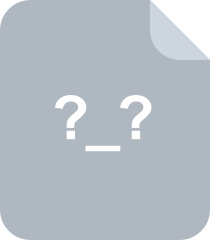
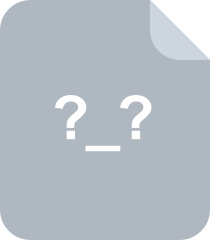
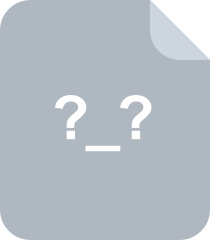
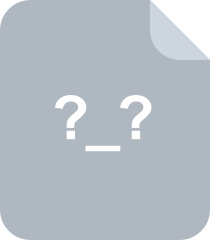
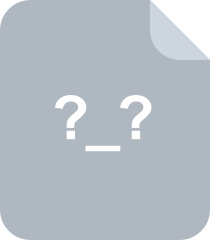
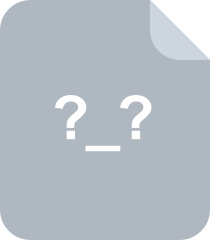
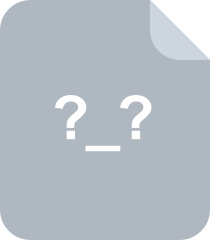
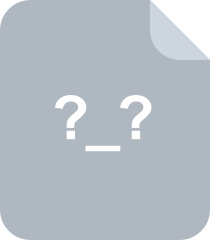
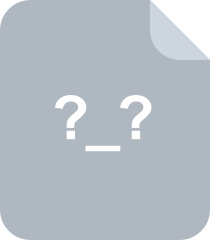
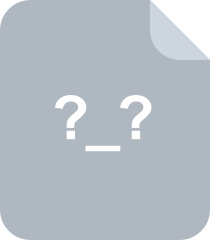
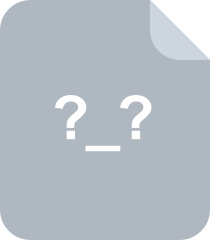
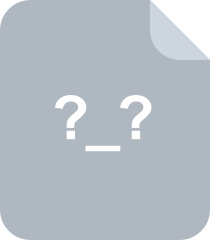
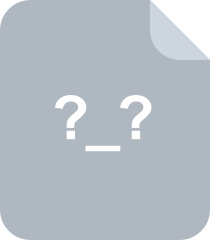
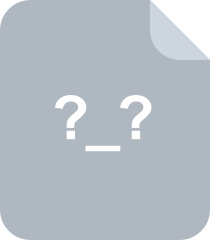
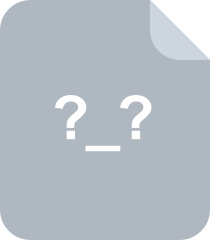
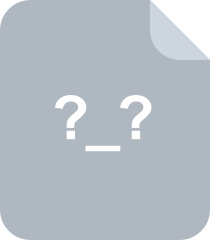
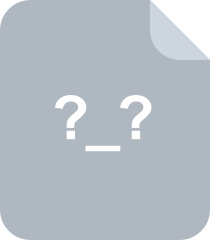
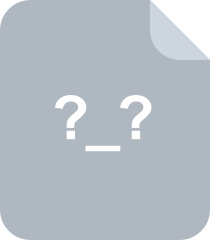
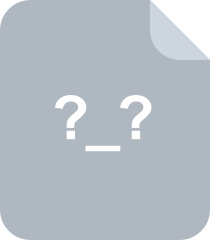
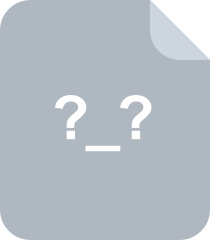
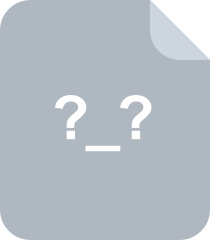
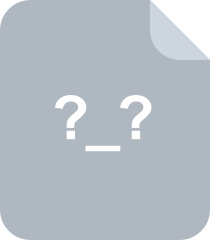
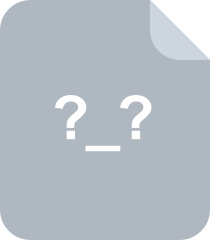
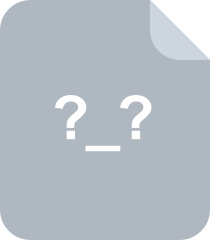
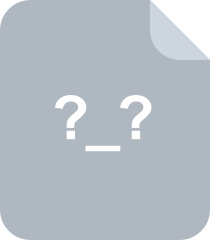
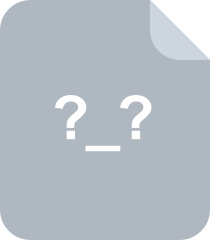
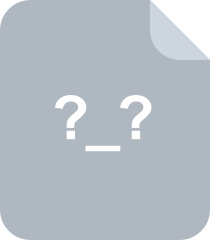
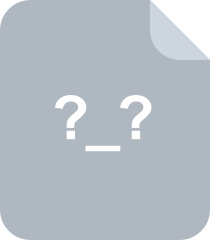
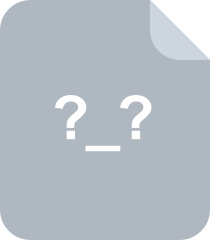
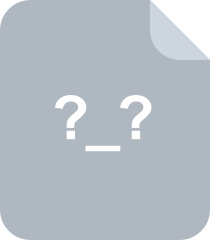
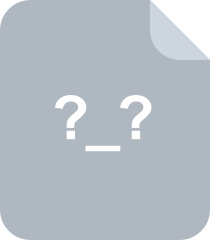
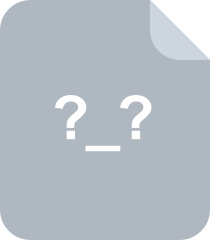
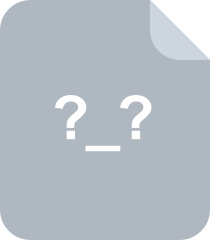
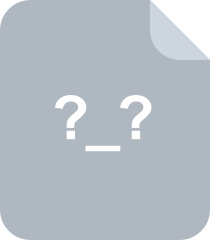
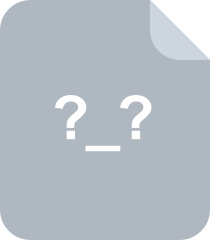
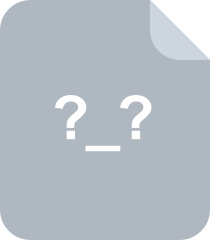
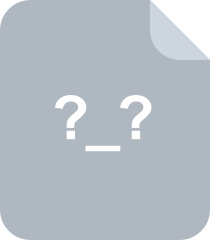
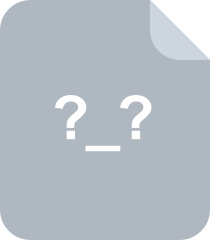
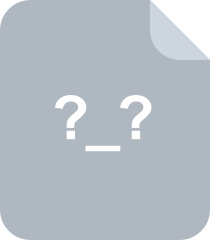
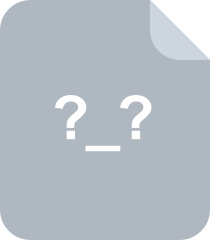
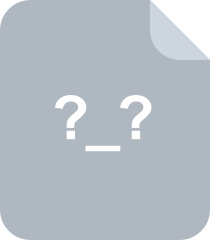
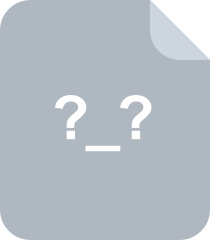
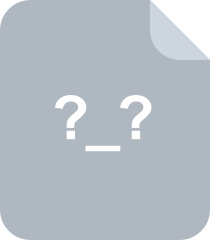
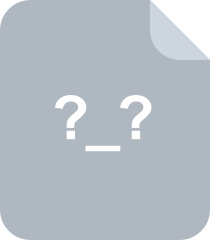
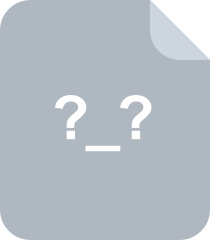
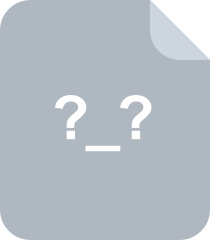
共 441 条
- 1
- 2
- 3
- 4
- 5
资源评论
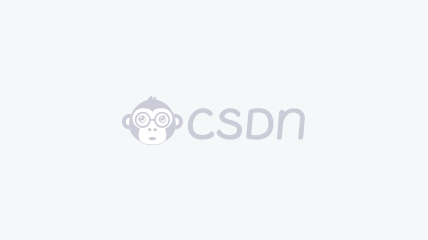

xyq2024
- 粉丝: 2713
- 资源: 5491
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

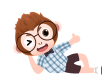
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


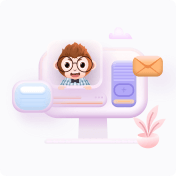
安全验证
文档复制为VIP权益,开通VIP直接复制
