/*
* Copyright (C) 2008 The Android Open Source Project
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.android.launcher3;
import static com.android.launcher3.LauncherAnimUtils.OVERVIEW_TRANSITION_MS;
import static com.android.launcher3.LauncherAnimUtils.SPRING_LOADED_EXIT_DELAY;
import static com.android.launcher3.LauncherAnimUtils.SPRING_LOADED_TRANSITION_MS;
import static com.android.launcher3.LauncherSettings.Favorites.ITEM_TYPE_APPLICATION;
import static com.android.launcher3.LauncherState.ALL_APPS;
import static com.android.launcher3.LauncherState.NORMAL;
import static com.android.launcher3.LauncherState.SPRING_LOADED;
import static com.android.launcher3.config.FeatureFlags.ADAPTIVE_ICON_WINDOW_ANIM;
import static com.android.launcher3.dragndrop.DragLayer.ALPHA_INDEX_OVERLAY;
import android.animation.Animator;
import android.animation.AnimatorListenerAdapter;
import android.animation.LayoutTransition;
import android.animation.ObjectAnimator;
import android.animation.ValueAnimator;
import android.animation.ValueAnimator.AnimatorUpdateListener;
import android.annotation.SuppressLint;
import android.app.WallpaperManager;
import android.appwidget.AppWidgetHostView;
import android.appwidget.AppWidgetProviderInfo;
import android.content.Context;
import android.content.res.Resources;
import android.graphics.Bitmap;
import android.graphics.Canvas;
import android.graphics.Point;
import android.graphics.Rect;
import android.graphics.drawable.Drawable;
import android.os.Handler;
import android.os.IBinder;
import android.os.Message;
import android.os.Parcelable;
import android.os.UserHandle;
import android.text.TextUtils;
import android.util.AttributeSet;
import android.util.Log;
import android.util.SparseArray;
import android.view.LayoutInflater;
import android.view.MotionEvent;
import android.view.View;
import android.view.ViewGroup;
import android.view.ViewTreeObserver;
import android.view.accessibility.AccessibilityNodeInfo;
import android.widget.Toast;
import com.android.launcher3.Launcher.LauncherOverlay;
import com.android.launcher3.LauncherAppWidgetHost.ProviderChangedListener;
import com.android.launcher3.LauncherStateManager.AnimationConfig;
import com.android.launcher3.accessibility.AccessibleDragListenerAdapter;
import com.android.launcher3.accessibility.WorkspaceAccessibilityHelper;
import com.android.launcher3.anim.AnimatorSetBuilder;
import com.android.launcher3.anim.Interpolators;
import com.android.launcher3.dot.FolderDotInfo;
import com.android.launcher3.compat.AppWidgetManagerCompat;
import com.android.launcher3.config.FeatureFlags;
import com.android.launcher3.dragndrop.DragController;
import com.android.launcher3.dragndrop.DragLayer;
import com.android.launcher3.dragndrop.DragOptions;
import com.android.launcher3.dragndrop.DragView;
import com.android.launcher3.dragndrop.SpringLoadedDragController;
import com.android.launcher3.folder.Folder;
import com.android.launcher3.folder.FolderIcon;
import com.android.launcher3.folder.PreviewBackground;
import com.android.launcher3.graphics.DragPreviewProvider;
import com.android.launcher3.graphics.PreloadIconDrawable;
import com.android.launcher3.graphics.RotationMode;
import com.android.launcher3.pageindicators.WorkspacePageIndicator;
import com.android.launcher3.popup.PopupContainerWithArrow;
import com.android.launcher3.shortcuts.ShortcutDragPreviewProvider;
import com.android.launcher3.testing.TestProtocol;
import com.android.launcher3.touch.WorkspaceTouchListener;
import com.android.launcher3.userevent.nano.LauncherLogProto.Action;
import com.android.launcher3.userevent.nano.LauncherLogProto.ContainerType;
import com.android.launcher3.userevent.nano.LauncherLogProto.Target;
import com.android.launcher3.util.IntArray;
import com.android.launcher3.util.IntSet;
import com.android.launcher3.util.IntSparseArrayMap;
import com.android.launcher3.util.ItemInfoMatcher;
import com.android.launcher3.util.PackageUserKey;
import com.android.launcher3.util.Thunk;
import com.android.launcher3.util.WallpaperOffsetInterpolator;
import com.android.launcher3.widget.LauncherAppWidgetHostView;
import com.android.launcher3.widget.PendingAddShortcutInfo;
import com.android.launcher3.widget.PendingAddWidgetInfo;
import com.android.launcher3.widget.PendingAppWidgetHostView;
import java.util.ArrayList;
import java.util.HashSet;
import java.util.function.Predicate;
/**
* The workspace is a wide area with a wallpaper and a finite number of pages.
* Each page contains a number of icons, folders or widgets the user can
* interact with. A workspace is meant to be used with a fixed width only.
*/
public class Workspace extends PagedView<WorkspacePageIndicator>
implements DropTarget, DragSource, View.OnTouchListener,
DragController.DragListener, Insettable, LauncherStateManager.StateHandler,
WorkspaceLayoutManager {
/** The value that {@link #mTransitionProgress} must be greater than for
* {@link #transitionStateShouldAllowDrop()} to return true. */
private static final float ALLOW_DROP_TRANSITION_PROGRESS = 0.25f;
/** The value that {@link #mTransitionProgress} must be greater than for
* {@link #isFinishedSwitchingState()} ()} to return true. */
private static final float FINISHED_SWITCHING_STATE_TRANSITION_PROGRESS = 0.5f;
private static final boolean ENFORCE_DRAG_EVENT_ORDER = false;
private static final int SNAP_OFF_EMPTY_SCREEN_DURATION = 400;
private static final int FADE_EMPTY_SCREEN_DURATION = 150;
private static final int ADJACENT_SCREEN_DROP_DURATION = 300;
private static final int DEFAULT_PAGE = 0;
public static final boolean MAP_NO_RECURSE = false;
public static final boolean MAP_RECURSE = true;
private LayoutTransition mLayoutTransition;
@Thunk final WallpaperManager mWallpaperManager;
private ShortcutAndWidgetContainer mDragSourceInternal;
@Thunk final IntSparseArrayMap<CellLayout> mWorkspaceScreens = new IntSparseArrayMap<>();
@Thunk final IntArray mScreenOrder = new IntArray();
@Thunk Runnable mRemoveEmptyScreenRunnable;
@Thunk boolean mDeferRemoveExtraEmptyScreen = false;
/**
* CellInfo for the cell that is currently being dragged
*/
private CellLayout.CellInfo mDragInfo;
/**
* Target drop area calculated during last acceptDrop call.
*/
@Thunk int[] mTargetCell = new int[2];
private int mDragOverX = -1;
private int mDragOverY = -1;
/**
* The CellLayout that is currently being dragged over
*/
@Thunk CellLayout mDragTargetLayout = null;
/**
* The CellLayout that we will show as highlighted
*/
private CellLayout mDragOverlappingLayout = null;
/**
* The CellLayout which will be dropped to
*/
private CellLayout mDropToLayout = null;
@Thunk final Launcher mLauncher;
@Thunk DragController mDragController;
private final Rect mTempRect = new Rect();
private final int[] mTempXY = new int[2];
private final float[] mTempFXY = new float[2];
@Thunk float[] mDragViewVisualCenter = new float[2];
private final float[] mTempTouchCoordinates = new float[2];
private SpringLoadedDragController mSpringLoadedDragController;
private boolean mIsSwitchingState = false;
boolean mChildrenLayersEnabled = true;
private boolean mStripScreensOnPageStopMoving = false;
private DragPreviewProvider mOutlineProvider = null;
private boolean m

xyq2024
- 粉丝: 2961
- 资源: 5572
最新资源
- 直流有刷电机模型+三闭环控制-SIMULINK
- MMC simulink 模块化多电平变流器 载波移相 双闭环仿真 输出谐波分析,线性自抗扰控制LADRC 有仿真文件
- Java毕设项目:基于spring+mybatis+maven+mysql实现的数学试卷自动生成系统【含源码+数据库】
- 基于simulink的永磁同步电机矢量控制FOC PMSM FOC
- 基于aiXcoder代码大模型的 企业智能化软件开发应用实践PPT(54页).pptx
- 深信服安全GPT:大模型时代下的网络安全建设思路——重新定义安全检测与安全运营PPT(37页).pptx
- 25页-人工智能+教育助力因材施教建设方案.pdf
- 光纤传输,多模光纤模式干涉,结构光场
- Java毕设项目:基于spring+mybatis+maven+mysql实现的大学学术交流论坛系统分前后台【含源码+数据库+答辩PPT+毕业论文】
- Comsol异常折射与折射角精确求解
- 基于双二阶广义积分器的软件锁相环仿真模型
- Java毕设项目:基于spring+mybatis+maven+mysql实现的高校社团管理系统【含源码+数据库+任务书+开题报告+毕业论文】
- 考虑用户舒适度的冷热电多能互补综合能源系统优化调度 关键词:用户舒适度 综合能源 PMV 优化调度 参考文档:《冷热电气多能互补的微能源网鲁棒优化调度》基础模型加舒适度部分模型; 仿真平台:MATL
- 考虑阶梯式碳交易机制与电制氢的综合能源系统热电优化 关键词:碳交易 电制氢 阶梯式碳交易 综合能源系统 热电优化 参考文档:《考虑阶梯式碳交易机制与电制氢的综合能源系统热电优化》基本复现 仿真平台
- 计及电转气协同的含碳捕集与垃圾焚烧电厂优化调度 关键词:碳捕集 电厂 需求响应 优化调度 电转气协同调度 参考文档:《计及电转气协同的含碳捕集与垃圾焚烧电厂优化调度》完全复现 仿真平台:MATL
- Java毕设项目:基于spring+mybatis+maven+mysql实现的国学文化网站【含源码+数据库+毕业论文】
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


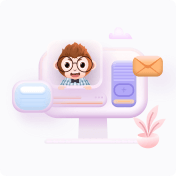