package com.spy.mall.shop.product.service.impl;
import com.baomidou.mybatisplus.core.conditions.query.QueryWrapper;
import com.spy.mall.common.constant.Constants;
import com.spy.mall.common.domain.ExecuteResult;
import com.spy.mall.common.domain.PageBean;
import com.spy.mall.shop.api.domain.entity.ShopProduct;
import com.spy.mall.shop.api.domain.entity.ShopProductPicture;
import com.spy.mall.shop.api.domain.entity.ShopSellType;
import com.spy.mall.shop.api.domain.vo.ShopDetailProductVo;
import com.spy.mall.shop.api.domain.vo.ShopIndexProductVo;
import com.spy.mall.shop.api.domain.vo.ShopProductPictureVo;
import com.spy.mall.shop.api.domain.vo.ShopProductVo;
import com.spy.mall.shop.product.mapper.ShopProductMapper;
import com.spy.mall.shop.product.mapper.ShopProductPictureMapper;
import com.spy.mall.shop.product.mapper.ShopSellTypeMapper;
import com.spy.mall.shop.product.repository.IndexProductElasticRepository;
import com.spy.mall.shop.product.service.ShopProductService;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.beans.BeanUtils;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import org.springframework.transaction.annotation.Transactional;
import java.util.Arrays;
import java.util.List;
import java.util.stream.Collectors;
/**
* @Author: spy
* @Date: 2019/2/7 17:22
* @Version 1.0
*/
@Transactional
@Service
public class ShopProductServiceImpl implements ShopProductService {
@Autowired
private ShopProductMapper productMapper;
@Autowired
private ShopProductPictureMapper productPictureMapper;
@Autowired
private ShopSellTypeMapper sellTypeMapper;
@Autowired
private IndexProductElasticRepository indexProductElasticRepository;
private static final Logger LOGGER = LoggerFactory.getLogger(ShopProductServiceImpl.class);
@Override
public ExecuteResult<ShopProductVo> insert(ShopProduct entity) {
ExecuteResult<ShopProductVo> result = new ExecuteResult<>();
try {
int insert = productMapper.insert(entity);
if (insert > 0) {
// 成功
successed(result, "添加成功!");
// 封装传输对象
ShopProductVo productVo = new ShopProductVo();
BeanUtils.copyProperties(entity, productVo);
result.setEntityVo(productVo);
} else {
executed(result, "添加失败!");
}
} catch (Exception e) {
LOGGER.error("系统运行时错误!", e);
throw e;
}
return result;
}
@Override
public ExecuteResult<ShopProductVo> updateById(ShopProduct entity) {
ExecuteResult<ShopProductVo> result = new ExecuteResult<>();
try {
int insert = productMapper.updateById(entity);
if (insert > 0) {
// 更新es中的数据
List<ShopIndexProductVo> productVos = productMapper.selectIndexProductsByProductId(entity.getProductId());
indexProductElasticRepository.save(productVos);
// 封装传输对象
ShopProductVo productVo = new ShopProductVo();
BeanUtils.copyProperties(entity, productVo);
result.setEntityVo(productVo);
successed(result, "更新成功!");
} else {
executed(result, "更新失败!");
}
} catch (Exception e) {
LOGGER.error("系统运行时错误!", e);
throw e;
}
return result;
}
@Override
public ExecuteResult<ShopProductVo> selectById(Long id) {
ExecuteResult<ShopProductVo> result = new ExecuteResult<>();
try {
ShopProductVo productVo = productMapper.selectDetailById(id);
if (productVo != null) {
// 成功
successed(result, "查询成功!");
result.setEntityVo(productVo);
} else {
// 失败
executed(result, "查询失败!");
}
} catch (Exception e) {
LOGGER.error("系统运行时错误!", e);
throw e;
}
return result;
}
@Override
public ExecuteResult<ShopProductVo> deleteById(Long id) {
ExecuteResult<ShopProductVo> result = new ExecuteResult<>();
try {
int delete = productMapper.deleteById(id);
if (delete > 0) {
List<ShopIndexProductVo> productVos = productMapper.selectIndexProductsByProductId(id);
indexProductElasticRepository.delete(productVos);
// 删除成功
successed(result, "删除成功!");
} else {
// 删除失败
executed(result, "删除失败!");
}
} catch (Exception e) {
LOGGER.error("有相关数据存在,不能删除!!", e);
throw e;
}
return result;
}
@Override
public ExecuteResult<ShopProductVo> deleteBatchIds(String ids) {
ExecuteResult<ShopProductVo> result = new ExecuteResult<>();
try {
String[] idStrs = ids.split(Constants.ID_SPLIT_SIGN);
int deleteBatchIds = productMapper.deleteBatchIds(Arrays.asList(idStrs));
if (deleteBatchIds > 0) {
successed(result, "删除成功!");
for (String idStr : idStrs) {
List<ShopIndexProductVo> productVos = productMapper.selectIndexProductsByProductId(Long.valueOf(idStr));
indexProductElasticRepository.delete(productVos);
}
} else {
executed(result, "删除失败!");
}
} catch (Exception e) {
LOGGER.error("有相关数据存在,不能删除!", e);
throw e;
}
return result;
}
@Override
public ExecuteResult<PageBean<ShopProductVo>> selectByPage(PageBean<ShopProductVo> pageBean) {
ExecuteResult<PageBean<ShopProductVo>> result = new ExecuteResult<>();
try {
List<ShopProductVo> productVos = productMapper.selectByPage(pageBean);
long total = productMapper.selectTotal(pageBean);
pageBean.setRecords(productVos);
pageBean.setTotal(total);
result.setEntityVo(pageBean);
successed(result, "查询成功!");
} catch (Exception e) {
LOGGER.error("系统运行时错误!", e);
throw e;
}
return result;
}
@Override
public ExecuteResult<ShopProductVo> selectAllIdAndName() {
ExecuteResult<ShopProductVo> result = new ExecuteResult<>();
try {
List<ShopProductVo> productVos = productMapper.selectAllIdAndName();
result.setEntityVos(productVos);
successed(result, "查询成功!");
} catch (Exception e) {
LOGGER.error("系统运行时错误!", e);
throw e;
}
return result;
}
@Override
public ExecuteResult<ShopProductVo> selectOneIdAndName(Long id) {
ExecuteResult<ShopProductVo> result = new ExecuteResult<>();
try {
ShopProductVo productVo = productMapper.selectOneIdAndName(id);
result.setEntityVo(productVo);
successed(result, "查询成功!");
} catch (Exception e) {
LOGGER.error("系统运行时错误!", e);
throw e;
}
return result;
}
@Override
public ExecuteResult<ShopProductPictureVo> saveProductPicture(ShopProductPicture productPicture) {
ExecuteResult<ShopProductPictureVo> result = new ExecuteResult<>();
try {
int insert = productPictureMapper.insert(productPicture);
if (insert > 0) {
successed(result, "商�

xyq2024
- 粉丝: 2960
- 资源: 5562
最新资源
- Delphi 12 控件之sqlite-dll-win-x64-3470200.zip
- s41392-024-02093-8.pdf
- 基于OpenCV和Pyzbar的二维码条形码识别
- 使用C语言做的新年烟花特效程序,程序中注释完整
- TweakPNG用于检查和修改PNG图像文件
- 单相H桥级联五电平逆变器仿真(SPWM)2021b 可降版本 闭环仿真 逆变器采用H桥级联的形式连接,单相负载构成 采用SPWM调制,具体关键性波形请看图片
- node-v18.20.5-win-x64.zip
- 23电平MMC逆变器并网仿真(PI控制) 基于Matlab Simulink仿真平台 采用基于PI控制器的双闭环控制 模型中包含环流抑制控制器 模型中添加基于排序算法的子模块均压方法 采用基于最近电平
- 使用MATLAB完成的renyi熵的计算,原创
- Delphi 12 控件之a7d60-main.zip
- 简约大气的毕业答辩PPT模板,十分完整,只需要更改文字即可
- 光伏MPPT仿真-直接电压法(恒定电压法)加PID控制,
- 回文串特性解析及其在多领域的应用场景与研究进展
- 绝对原创MATLAB Simulink 2021b 直流微电网 风、光、储、负载、逆变器 风力发电和光伏发电采用MPPT控制 储能单元采用双环控制直流测电压为750V 逆变器采用PQ控制 风机功率慢慢
- 江南大学轴承数据集,现阶段做轴承故障诊断效果较好的数据集
- 详解:Ubuntu 20.04 LTS的完整安装与初始配置教程
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


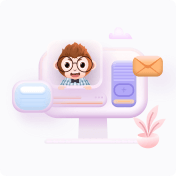