'use strict';
// can we use __proto__?
function getHasProto() {
var hasProto = false;
if ('__proto__' in {}) {
var fn = function () {};
var arr = [];
arr.__proto__ = { push: fn };
hasProto = fn === arr.push;
}
return hasProto;
}
var hasProto = getHasProto();
var _Set; // $flow-disable-line
/* istanbul ignore if */ if (typeof Set !== 'undefined' && isNative(Set)) {
// use native Set when available.
_Set = Set;
} else {
// a non-standard Set polyfill that only works with primitive keys.
_Set = /*@__PURE__*/(function () {
function Set() {
this.set = Object.create(null);
}
Set.prototype.has = function has (key) {
return this.set[key] === true;
};
Set.prototype.add = function add (key) {
this.set[key] = true;
};
Set.prototype.clear = function clear () {
this.set = Object.create(null);
};
return Set;
}());
}
/* istanbul ignore next */
function isNative(Ctor) {
return typeof Ctor === 'function' && /native code/.test(Ctor.toString());
}
/**
* String type check
*/
var isStr = function (v) { return typeof v === 'string'; };
/**
* Number type check
*/
var isNum = function (v) { return typeof v === 'number'; };
/**
* Array type check
*/
var isArr = Array.isArray;
/**
* undefined type check
*/
var isUndef = function (v) { return v === undefined; };
/**
* Function type check
*/
var isFunc = function (v) { return typeof v === 'function'; };
/**
* Quick object check - this is primarily used to tell
* Objects from primitive values when we know the value
* is a JSON-compliant type.
*/
function isObject(obj) {
return obj !== null && typeof obj === 'object';
}
var isObj = isObject;
/**
* Strict object type check. Only returns true
* for plain JavaScript objects.
*/
var _toString = Object.prototype.toString;
function isPlainObject(obj) {
return _toString.call(obj) === '[object Object]';
}
/**
* Check whether the object has the property.
*/
var hasOwnProperty = Object.prototype.hasOwnProperty;
function hasOwn(obj, key) {
return hasOwnProperty.call(obj, key);
}
/**
* Perform no operation.
* Stubbing args to make Flow happy without leaving useless transpiled code
* with ...rest (https://flow.org/blog/2017/05/07/Strict-Function-Call-Arity/)
*/
// eslint-disable-next-line
function noop(a, b, c) {}
/**
* Check if val is a valid array index.
*/
function isValidArrayIndex(val) {
var n = parseFloat(String(val));
return n >= 0 && Math.floor(n) === n && isFinite(val);
}
/**
* Convert an Array-lik object to a real Array
*/
function toArray(list, start) {
if ( start === void 0 ) start = 0;
var i = list.length - start;
var rst = new Array(i);
while (i--) {
rst[i] = list[i + start];
}
return rst;
}
/**
* Cached simply key function return
*/
var cached = function (fn) {
var cache = {};
return function (str) { return cache[str] || (cache[str] = fn(str)); };
};
var camelizeRE = /-(\w)/g;
/**
* camelize words
* e.g. my-key => myKey
*/
var camelize = cached(function (str) { return str.replace(camelizeRE, function (_, c) { return (c ? c.toUpperCase() : ''); }); });
/*
* extend objects
* e.g.
* extend({}, {a: 1}) : extend {a: 1} to {}
* extend(true, [], [1,2,3]) : deep extend [1,2,3] to an empty array
* extend(true, {}, {a: 1}, {b: 2}) : deep extend two objects to {}
*/
function extend() {
var arguments$1 = arguments;
var options,
name,
src,
copy,
copyIsArray,
clone,
target = arguments[0] || {},
i = 1,
length = arguments.length,
deep = false;
// Handle a deep copy situation
if (typeof target === 'boolean') {
deep = target;
// Skip the boolean and the target
target = arguments[i] || {};
i++;
}
// Handle case when target is a string or something (possible in deep copy)
if (typeof target !== 'object' && !(typeof target === 'function')) {
target = {};
}
// Extend jQuery itself if only one argument is passed
if (i === length) {
target = this;
i--;
}
for (; i < length; i++) {
// Only deal with non-null/undefined values
if ((options = arguments$1[i])) {
// Extend the base object
for (name in options) {
src = target[name];
copy = options[name];
// Prevent never-ending loop
if (target === copy) {
continue;
}
// Recurse if we're merging plain objects or arrays
if (deep && copy && (isPlainObject(copy) || (copyIsArray = Array.isArray(copy)))) {
if (copyIsArray) {
copyIsArray = false;
clone = src && Array.isArray(src) ? src : [];
} else {
clone = src && isPlainObject(src) ? src : {};
}
// Never move original objects, clone them
target[name] = extend(deep, clone, copy);
// Don't bring in undefined values => bring undefined values
} else {
target[name] = copy;
}
}
}
}
// Return the modified object
return target;
}
/*
* clone objects, return a cloned object default to use deep clone
* e.g.
* clone({a: 1})
* clone({a: b: {c : 1}}, false);
*/
function clone(sth, deep) {
if ( deep === void 0 ) deep = true;
if (isArr(sth)) {
return extend(deep, [], sth);
} else if ('' + sth === 'null') {
return sth;
} else if (isPlainObject(sth)) {
return extend(deep, {}, sth);
} else {
return sth;
}
}
var WEAPP_APP_LIFECYCLE = ['onLaunch', 'onShow', 'onHide', 'onError', 'onPageNotFound'];
var WEAPP_PAGE_LIFECYCLE = [
'onLoad',
'onShow',
'onReady',
'onHide',
'onUnload',
'onPullDownRefresh',
'onReachBottom',
'onShareAppMessage',
'onPageScroll',
'onTabItemTap',
'onResize'
];
var WEAPP_COMPONENT_LIFECYCLE = ['beforeCreate', 'created', 'attached', 'ready', 'moved', 'detached'];
var WEAPP_LIFECYCLE = []
.concat(WEAPP_APP_LIFECYCLE)
.concat(WEAPP_PAGE_LIFECYCLE)
.concat(WEAPP_COMPONENT_LIFECYCLE);
var config = {};
var warn = noop;
var generateComponentTrace = function(vm) {
return ("Found in component: \"" + (vm.$is) + "\"");
};
{
var hasConsole = typeof console !== 'undefined';
// TODO
warn = function (msg, vm) {
if (hasConsole && !config.silent) {
// eslint-disable-next-line
console.error("[WePY warn]: " + msg + (vm ? generateComponentTrace(vm) : ''));
}
};
}
function handleError(err, vm, info) {
if (vm) {
var cur = vm;
while ((cur = cur.$parent)) {
var hooks = cur.$options.errorCaptured;
if (hooks) {
for (var i = 0; i < hooks.length; i++) {
try {
var capture = hooks[i].call(cur, err, vm, info) === false;
if (capture) { return; }
} catch (e) {
globalHandleError(e, cur, 'errorCaptured hook');
}
}
}
}
}
globalHandleError(err, vm, info);
}
function globalHandleError(err, vm, info) {
if (config.errorHandler) {
try {
return config.errorHandler.call(null, err, vm, info);
} catch (e) {
logError(e, null, 'config.errorHandler');
}
}
logError(err, vm, info);
}
function logError(err, vm, info) {
{
warn(("Error in " + info + ": \"" + (err.toString()) + "\""), vm);
}
/* istanbul ignore else */
if (typeof console !== 'undefined') {
// eslint-disable-next-line
console.error(err);
} else {
throw err;
}
}
var callbacks = [];
var pending = false;
function flushCallbacks() {
pending = false;
var copies = callbacks.slice(0);
callbacks.length = 0;
for (var i = 0; i < copies.length; i++) {
copies[i]();
}
}
// Here we have async deferring wrappers using both micro and macro tasks.
// In < 2.4 we used micro tasks everywhere, but there are some scenarios where
// micro tasks have too high a priority and fires in between supposedly
// sequential events (e.g. #4521, #6690) or even between bubbling of the same
// event (#6566). However, using macro tasks everywhere also has s
没有合适的资源?快使用搜索试试~ 我知道了~
基于WePY框架的微信小程序组件化开发设计源码
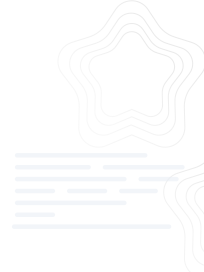
共479个文件
js:277个
json:33个
md:33个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 37 浏览量
2024-09-27
05:34:49
上传
评论
收藏 1.71MB ZIP 举报
温馨提示
该项目为基于WePY框架的微信小程序组件化开发设计源码,包含490个文件,涵盖273个JavaScript文件、50个Markdown文件、33个JSON文件、28个CSS文件、15个TypeScript文件、14个LESS文件、13个STYL文件、11个WXML文件、11个HTML文件、10个npmignore文件。代码支持JavaScript、CSS、TypeScript、HTML、微信小程序和Shell等多种语言,旨在通过框架的预编译功能和细节优化,如Promise和Async Functions的引入,简化小程序开发过程,提高开发效率。
资源推荐
资源详情
资源评论
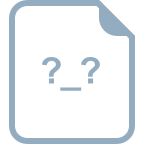
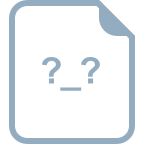
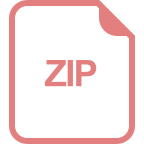
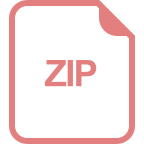
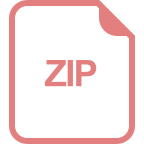
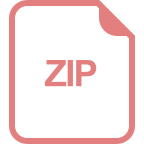
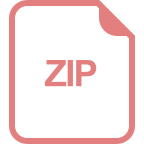
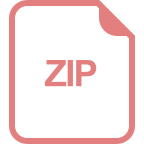
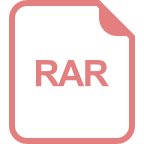
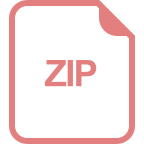
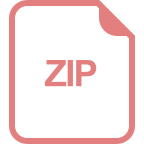
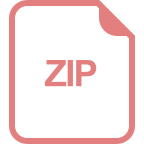
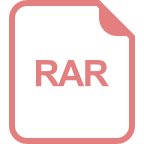
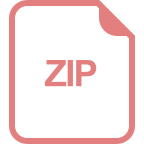
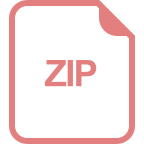
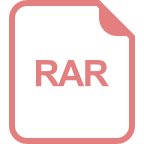
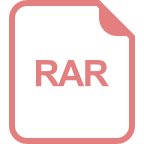
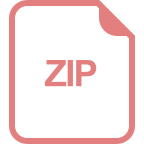
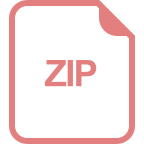
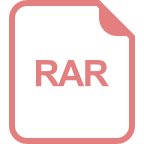
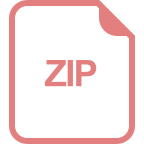
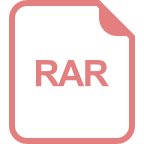
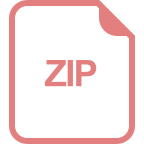
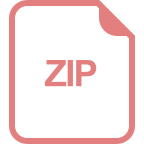
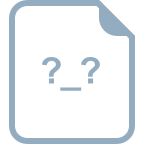
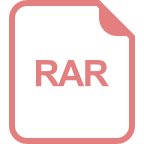
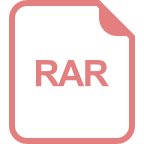
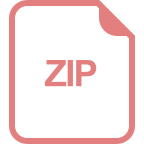
收起资源包目录

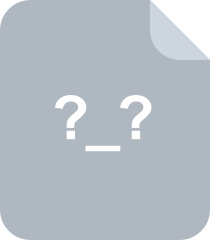
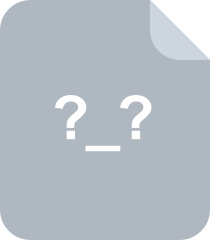
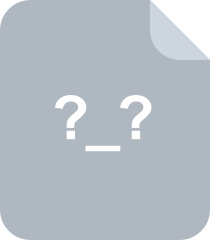
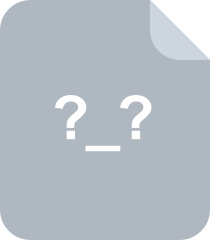
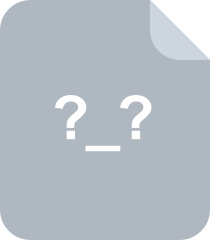
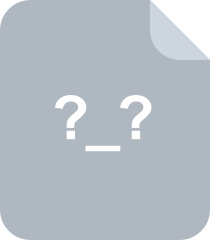
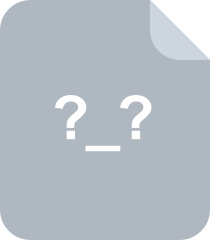
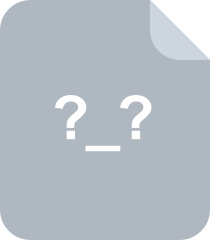
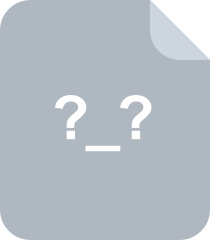
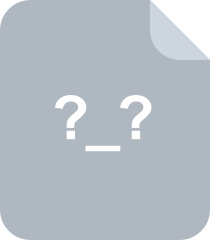
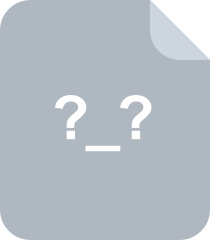
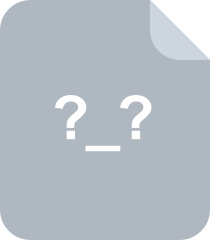
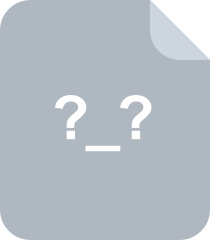
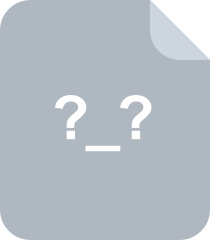
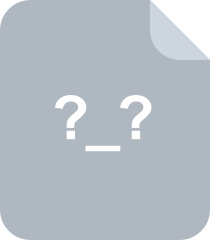
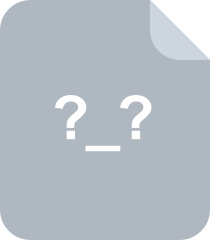
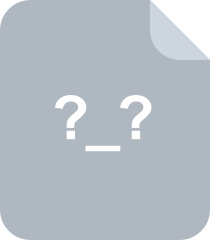
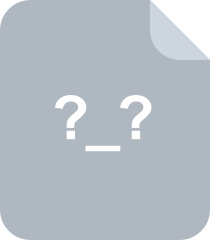
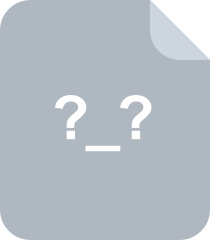
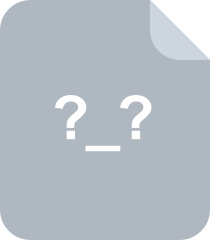
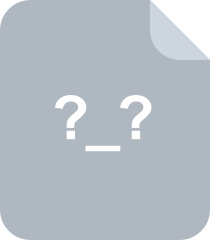
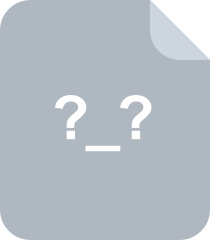
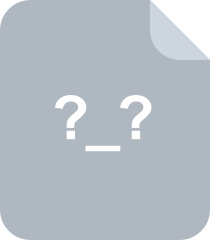
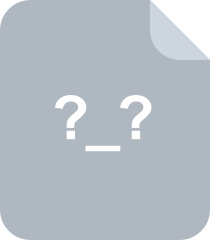
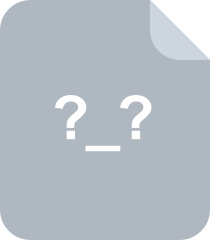
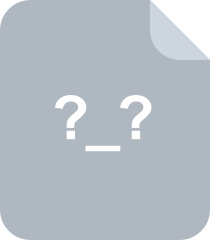
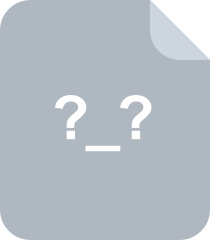
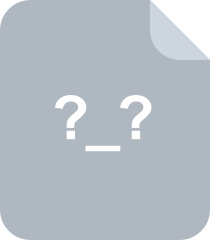
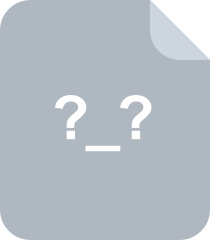
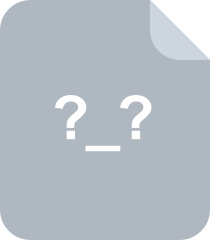
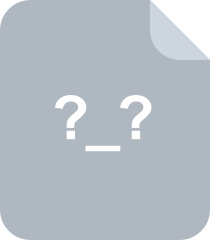
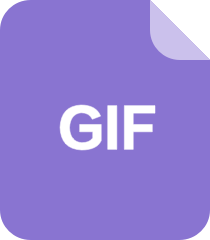
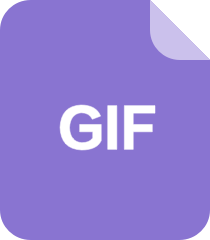
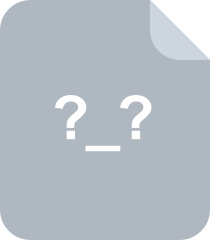
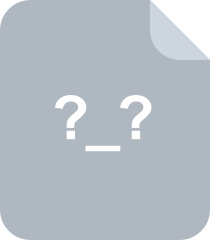
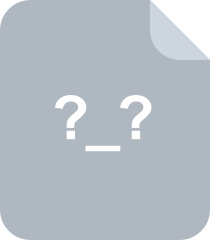
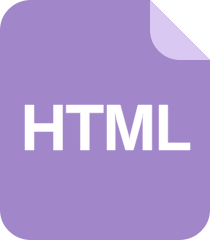
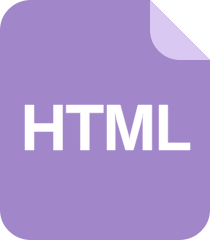
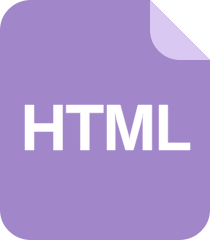
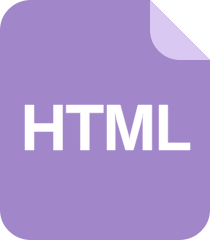
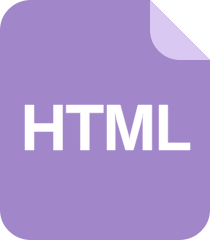
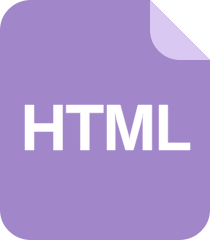
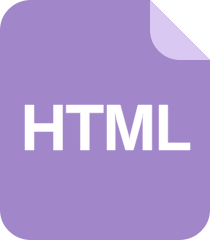
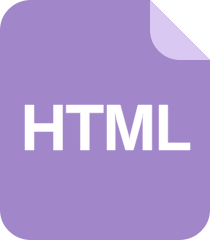
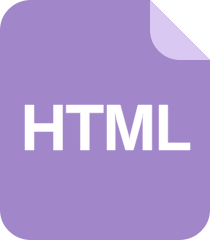
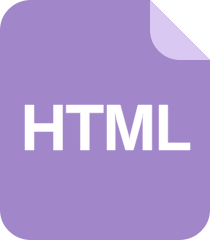
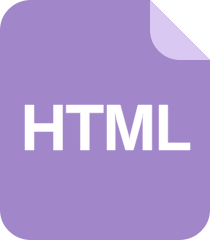
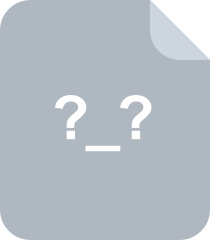
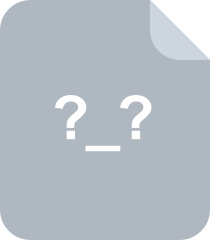
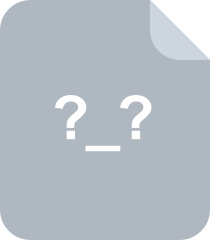
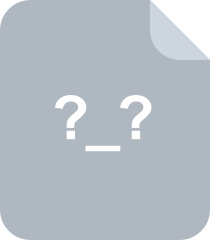
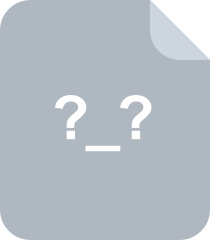
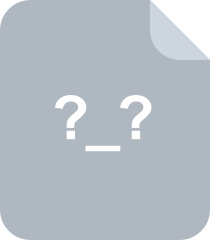
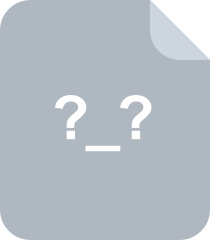
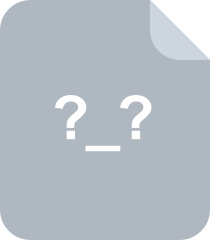
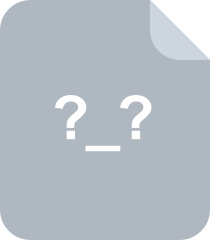
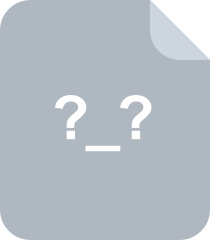
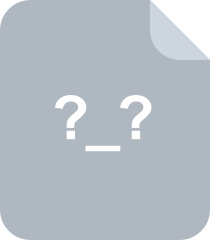
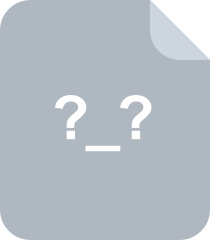
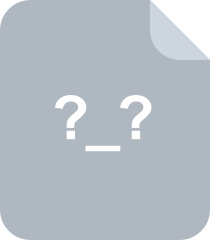
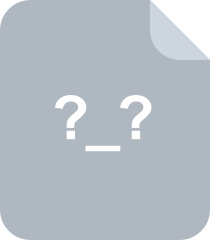
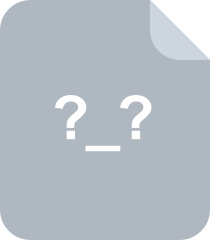
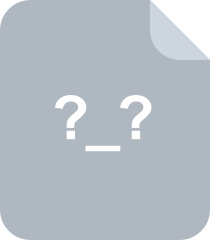
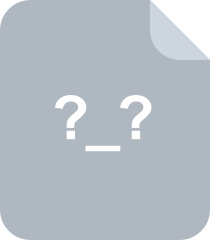
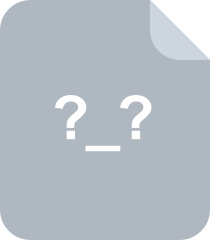
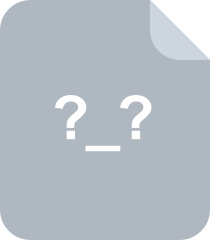
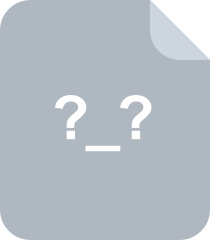
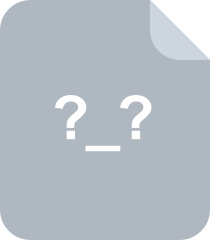
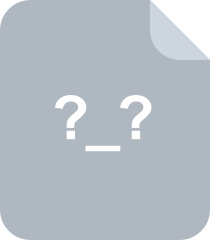
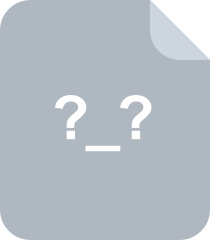
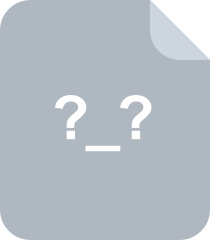
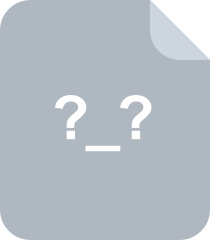
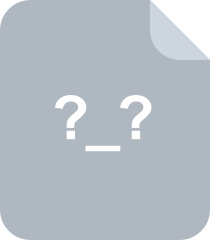
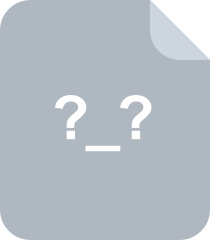
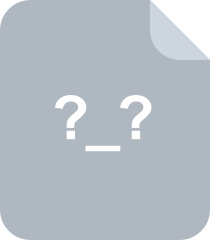
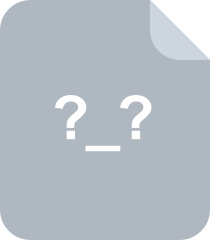
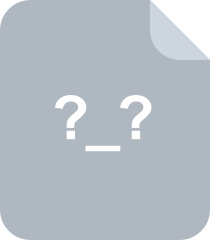
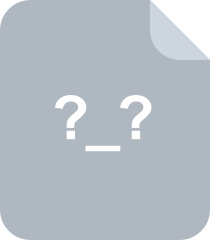
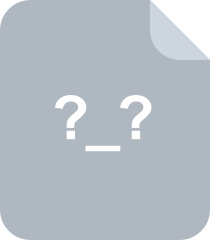
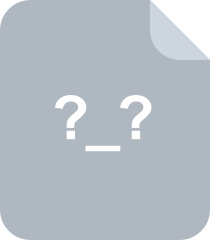
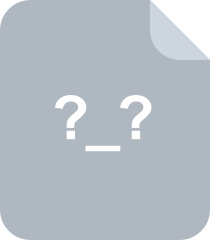
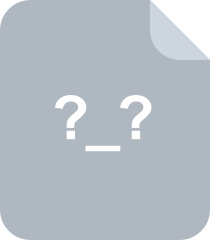
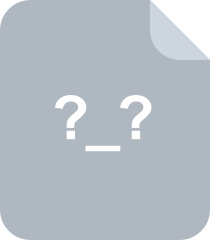
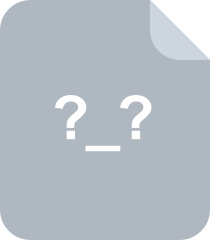
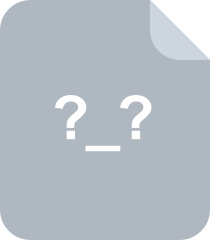
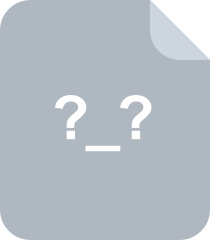
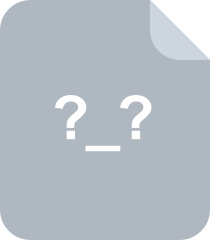
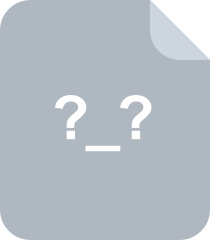
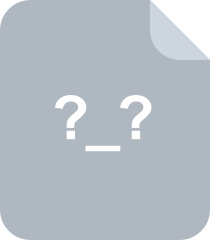
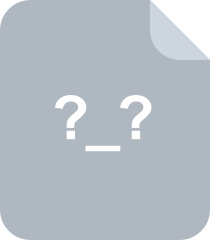
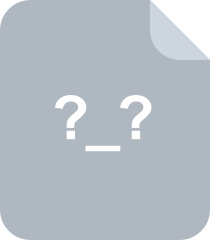
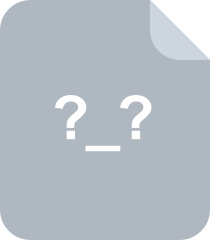
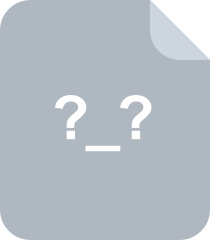
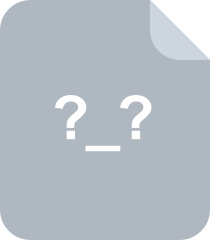
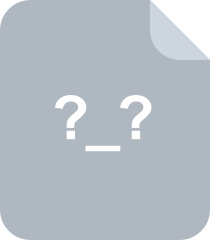
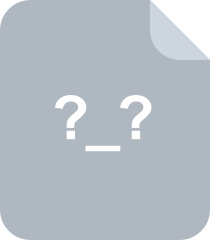
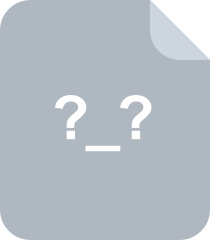
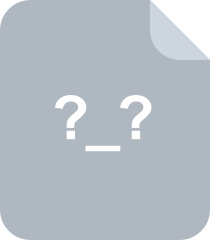
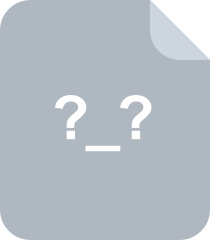
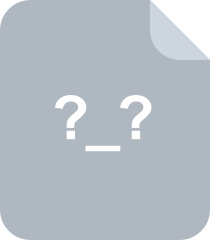
共 479 条
- 1
- 2
- 3
- 4
- 5
资源评论
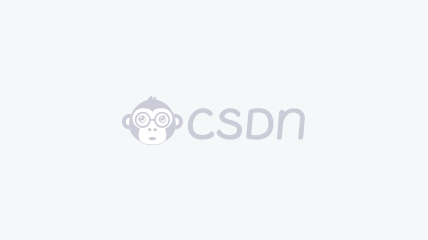

xyq2024
- 粉丝: 2375
- 资源: 5443
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

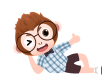
最新资源
- C183579-123578-c1235789.jpg
- Qt5.14 绘画板 Qt Creator C++项目
- python实现Excel表格合并
- Java实现读取Excel批量发送邮件.zip
- 【java毕业设计】商城后台管理系统源码(springboot+vue+mysql+说明文档).zip
- 【java毕业设计】开发停车位管理系统(调用百度地图API)源码(springboot+vue+mysql+说明文档).zip
- 星耀软件库(升级版).apk.1
- 基于Django后端和Vue前端的多语言购物车项目设计源码
- 基于Python与Vue的浮光在线教育平台源码设计
- 31129647070291Eclipson MXS R.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


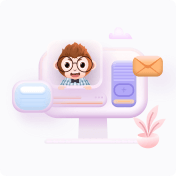
安全验证
文档复制为VIP权益,开通VIP直接复制
