# Copyright (c) 2021-2023 Alibaba Cloud Computing Ltd.
# SPDX-License-Identifier: MulanPSL-2.0
import os
import sys
import unittest
from time import sleep
from selenium import webdriver
from selenium.webdriver.common.keys import Keys
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
from selenium.webdriver import ActionChains
sys.path.append(os.path.abspath(os.path.join(os.getcwd(), "..")))
from common import keentuneInit
class TestKeenTuneUiSmartNormal(unittest.TestCase):
@classmethod
def setUpClass(self, no_ui=False) -> None:
if 'linux' in sys.platform:
option = webdriver.ChromeOptions()
option.add_argument('headless')
option.add_argument('no-sandbox')
option.add_argument('--start-maximized')
option.add_argument('--disable-gpu')
option.add_argument('lang=zh_CN.UTF-8')
option.add_argument('--window-size=1920,1080')
self.driver = webdriver.Chrome(options=option)
self.driver.implicitly_wait(10)
self.wait = WebDriverWait(self.driver, 90, 0.5)
self.AC = ActionChains(self.driver)
else:
if no_ui:
option = webdriver.ChromeOptions()
option.add_argument('headless')
option.add_argument('--start-maximized')
self.driver = webdriver.Chrome(chrome_options=option)
self.wait = WebDriverWait(self.driver, 90, 0.5)
self.AC = ActionChains(self.driver)
else:
self.driver = webdriver.Chrome()
self.driver.maximize_window()
self.wait = WebDriverWait(self.driver, 90, 0.5)
self.AC = ActionChains(self.driver)
keentuneInit(self)
self.driver.get("http://{}:8082/list/tuning-task/".format(self.web_ip))
value = self.driver.find_element(By.XPATH, '//div[@class="ant-pro-table-list-toolbar-title"]').text
if "智能参数调优任务记录" not in value:
self.driver.find_element(By.XPATH,
'//div[@class="ant-space ant-space-horizontal ant-space-align-center right___3L8KG"]/div/div/img').click()
@classmethod
def tearDownClass(self) -> None:
self.driver.get("http://{}:8082/list/tuning-task".format(self.web_ip))
del_list = ["auto_test_HORD", "auto_test_Random", "auto_test2_HORD","auto_test2_Random"]
for i in range(9):
first_text = self.wait.until(
EC.visibility_of_element_located((By.XPATH, '//tbody[@class="ant-table-tbody"]/tr[1]/td[2]'))).text
if first_text in del_list:
self.wait.until(EC.element_to_be_clickable(
(By.XPATH, '//tbody[@class="ant-table-tbody"]/tr[1]/td[12]/div'))).click()
self.wait.until(EC.element_to_be_clickable((By.XPATH,
'//ul[@class="ant-dropdown-menu ant-dropdown-menu-root ant-dropdown-menu-vertical ant-dropdown-menu-light"]/li[6]/span[1]'))).click()
self.wait.until(EC.element_to_be_clickable((By.XPATH,
'//div[@class="ant-modal-confirm-body-wrapper"]//button[@class="ant-btn ant-btn-primary"]'))).click()
sleep(5)
self.driver.quit()
def tearDown(self) -> None:
self.driver.refresh()
sleep(5)
def test_createjob(self):
Algorithm_list = ["HORD", "Random"]
# 遍历创建不同算法任务
for i in Algorithm_list:
self.wait.until(
EC.element_to_be_clickable((By.XPATH, '//button[@class="ant-btn ant-btn-default"]'))).click()
self.wait.until(EC.visibility_of_element_located((By.ID, "name"))).send_keys(Keys.CONTROL, "a")
self.wait.until(EC.visibility_of_element_located((By.ID, "name"))).send_keys(Keys.BACKSPACE)
self.wait.until(EC.visibility_of_element_located((By.ID, "name"))).send_keys("auto_test_" + i)
self.wait.until(EC.visibility_of_element_located((By.ID, "algorithm"))).send_keys(Keys.CONTROL, "a")
self.wait.until(EC.visibility_of_element_located((By.ID, "algorithm"))).send_keys(Keys.BACKSPACE)
self.wait.until(EC.visibility_of_element_located((By.ID, "algorithm"))).send_keys(i)
self.wait.until(EC.visibility_of_element_located((By.ID, "algorithm"))).send_keys(Keys.ENTER)
self.wait.until(EC.visibility_of_element_located((By.ID, "iteration"))).send_keys(Keys.CONTROL, "a")
self.wait.until(EC.visibility_of_element_located((By.ID, "iteration"))).send_keys(Keys.BACKSPACE)
self.wait.until(EC.visibility_of_element_located((By.ID, "iteration"))).send_keys(10)
self.wait.until(EC.element_to_be_clickable(
(By.XPATH, '//div[@class="ant-modal-mask"]/../div[2]/div[1]/div[2]/div[3]/div[1]/div[2]'))).click()
# 等待任务执行完成,任务完成重新创建下一个,超时则结束
for j in range(1, 9):
sleep(20)
self.driver.refresh()
Total_Time = self.wait.until(
EC.visibility_of_element_located((By.XPATH, '//tbody[@class="ant-table-tbody"]/tr[1]/td[11]'))).text
if Total_Time != "-":
break
elif j == 8:
self.assertNotIn("-", Total_Time)
status = self.wait.until(
EC.visibility_of_element_located((By.XPATH, '//tbody[@class="ant-table-tbody"]/tr[1]/td[4]'))).text
name = self.wait.until(
EC.visibility_of_element_located((By.XPATH, '//tbody[@class="ant-table-tbody"]/tr[1]/td[2]'))).text
if status != 'finish':
print(f'{name} execution failure')
self.assertIn("auto_test_" + Algorithm_list[-1], name)
def test_createjob02(self):
Algorithm_list = ["HORD", "Random"]
# 分别创建不同参数任务
for i in Algorithm_list:
self.wait.until(
EC.element_to_be_clickable((By.XPATH, '//button[@class="ant-btn ant-btn-default"]'))).click()
self.wait.until(EC.visibility_of_element_located((By.ID, "name"))).send_keys(Keys.CONTROL, "a")
self.wait.until(EC.visibility_of_element_located((By.ID, "name"))).send_keys(Keys.BACKSPACE)
self.wait.until(EC.visibility_of_element_located((By.ID, "name"))).send_keys("auto_test2_" + i)
self.wait.until(EC.visibility_of_element_located((By.ID, "algorithm"))).send_keys(Keys.CONTROL, "a")
self.wait.until(EC.visibility_of_element_located((By.ID, "algorithm"))).send_keys(Keys.BACKSPACE)
self.wait.until(EC.visibility_of_element_located((By.ID, "algorithm"))).send_keys(i)
self.wait.until(EC.visibility_of_element_located((By.ID, "algorithm"))).send_keys(Keys.ENTER)
self.wait.until(EC.visibility_of_element_located((By.ID, "iteration"))).send_keys(Keys.CONTROL, "a")
self.wait.until(EC.visibility_of_element_located((By.ID, "iteration"))).send_keys(Keys.BACKSPACE)
self.wait.until(EC.visibility_of_element_located((By.ID, "iteration"))).send_keys(10)
if i == "TPE":
self.wait.until(EC.visibility_of_element_located((By.ID, "baseline_bench_round"))).send_keys(
Keys.CONTROL, "a")
self.wait.until(EC.visibility_of_element_located((By.ID, "baseline_bench_round"))).send_keys(
Keys.BACKSPACE)
self.wait.until(EC.visibility_of_element_located((By.ID, "baseline_bench_round"))).send_keys(2)
self.wait.until(EC.visibility_of_element_located((By.ID, "tuning_bench_round"))).send_keys(Keys.CONTROL,
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
该项目是一款基于AI算法与专家知识库的操作系统全栈式智能优化产品KeenTune的源码,包含326个文件,涵盖130个Go语言文件、89个Python脚本、38个配置文件、20个JSON文件、13个Markdown文件、6个Shell脚本、5个PNG图片文件,以及少量其他文件类型。该产品旨在为各类操作系统提供轻量级、跨平台的性能调优方案,通过智能定制环境助力应用实现最优性能表现。
资源推荐
资源详情
资源评论
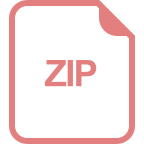
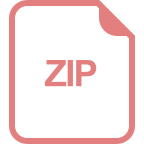
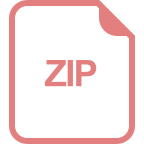
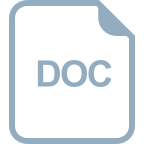
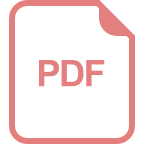
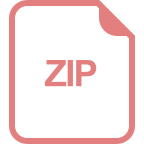
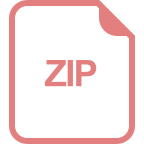
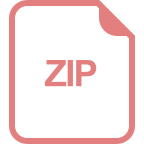
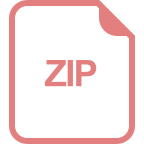
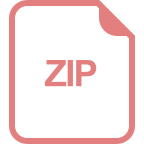
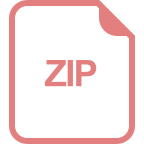
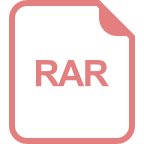
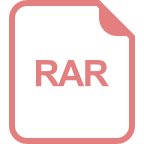
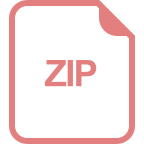
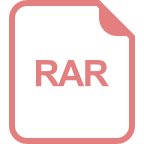
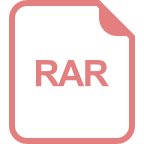
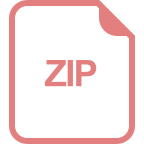
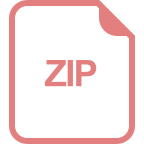
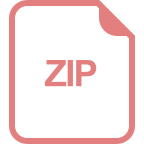
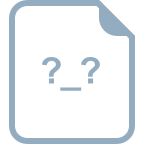
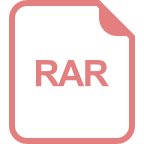
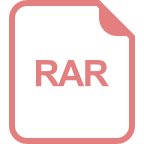
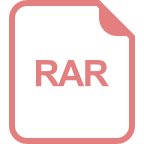
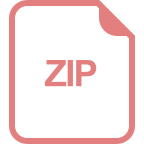
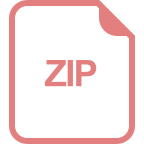
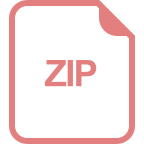
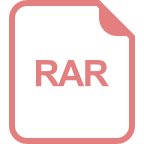
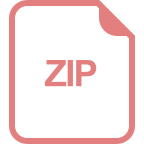
收起资源包目录

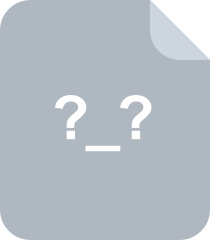
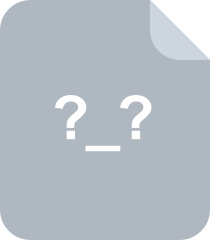
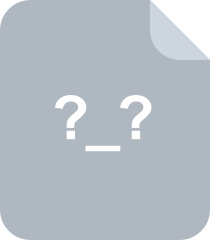
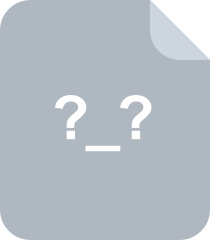
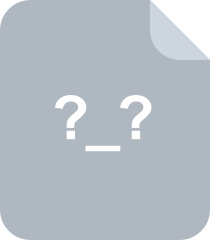
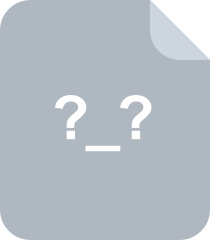
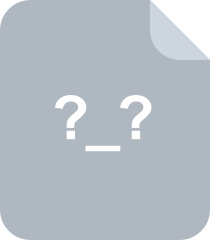
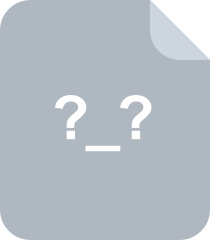
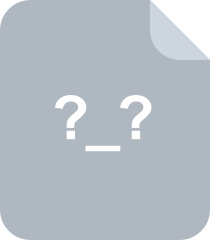
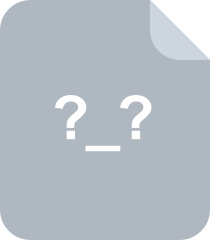
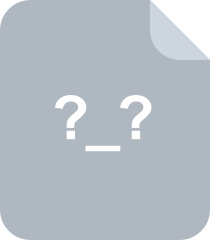
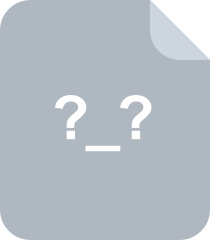
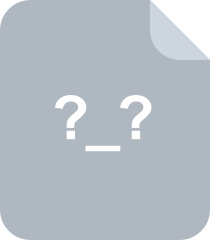
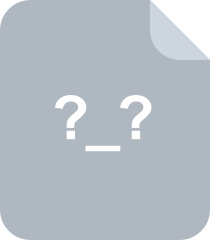
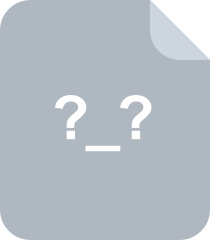
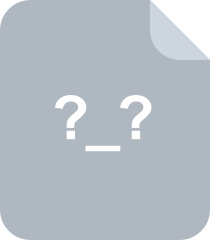
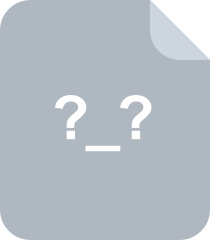
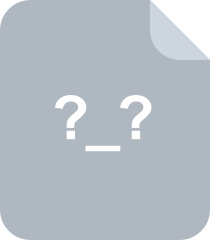
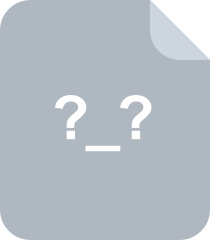
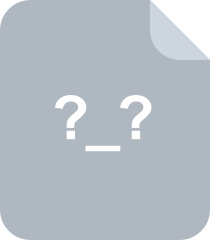
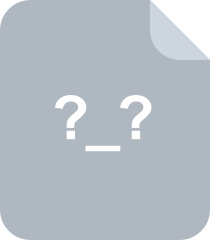
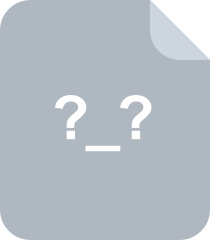
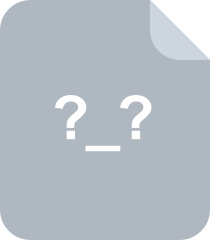
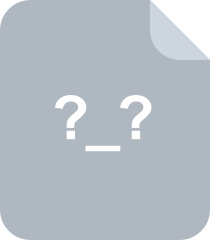
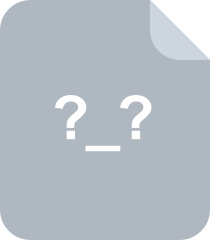
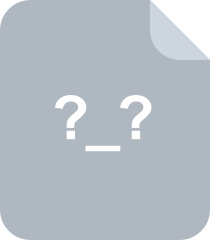
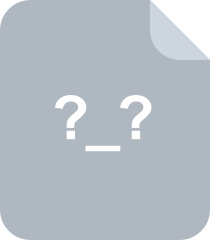
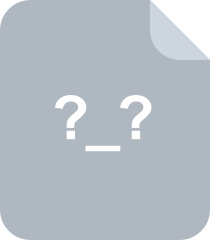
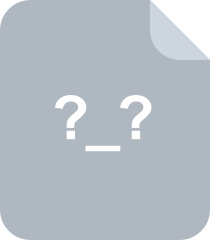
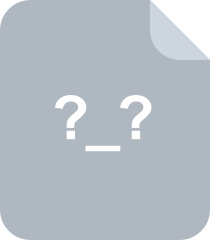
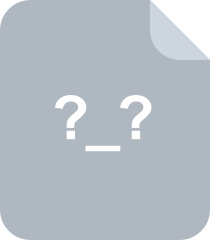
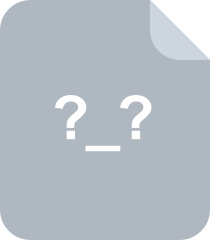
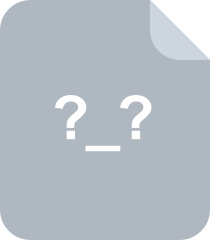
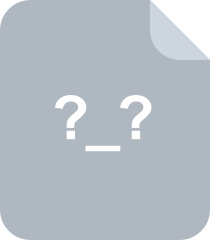
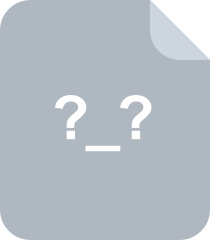
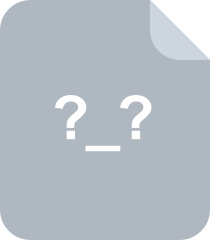
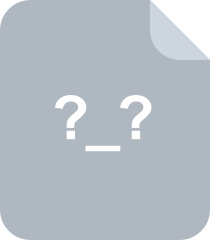
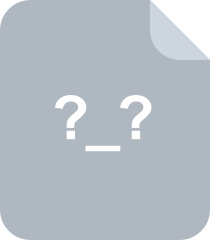
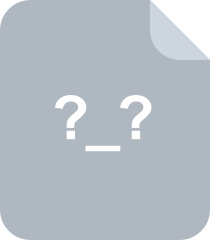
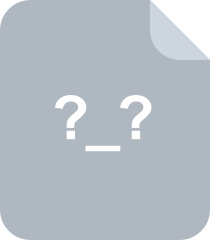
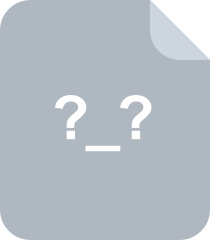
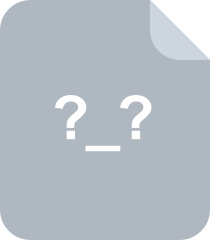
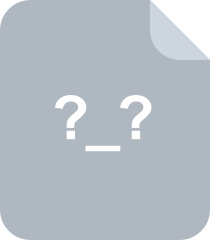
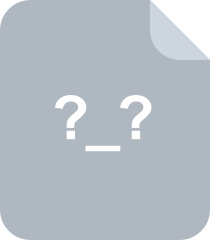
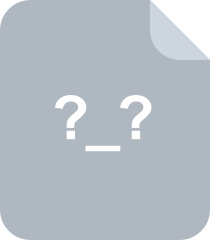
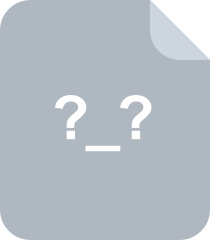
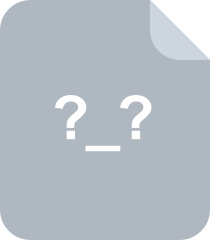
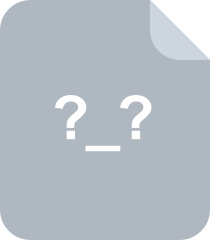
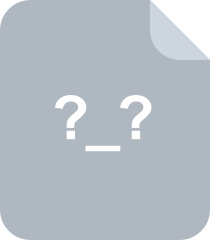
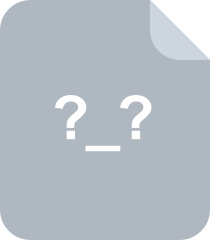
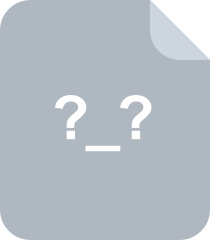
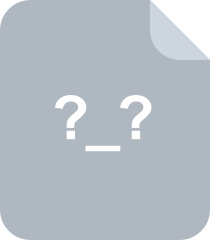
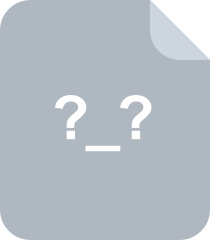
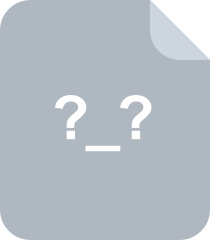
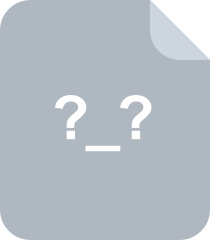
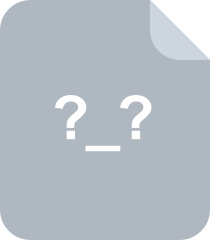
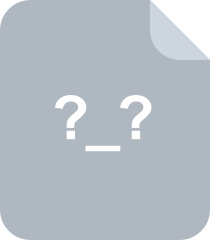
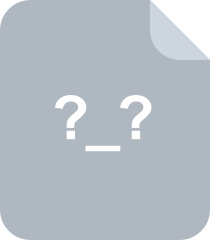
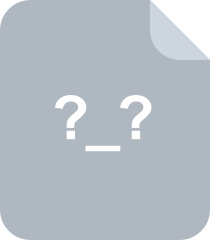
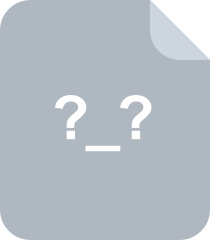
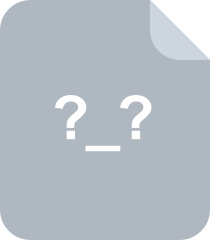
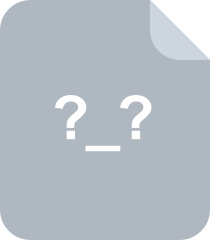
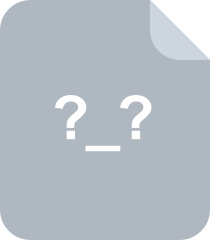
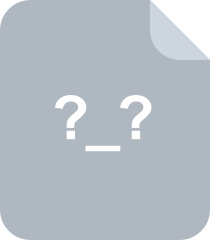
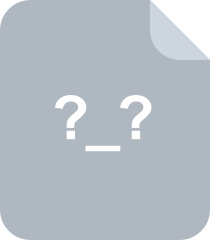
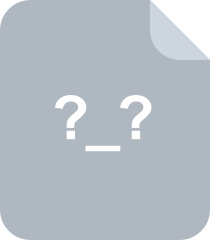
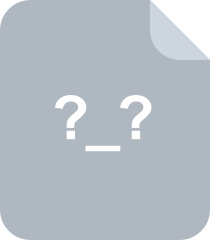
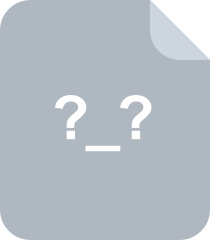
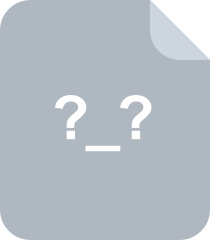
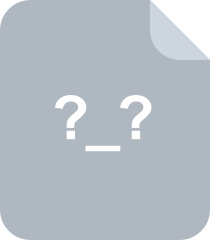
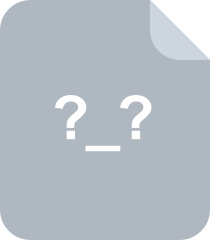
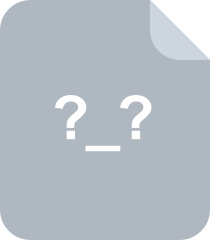
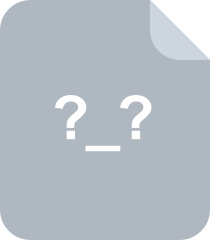
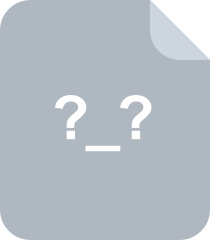
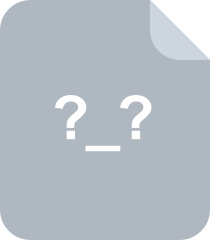
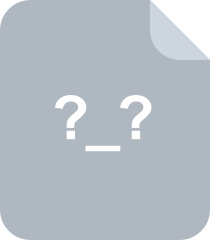
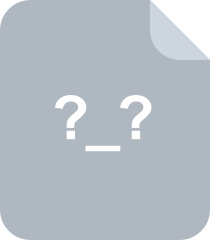
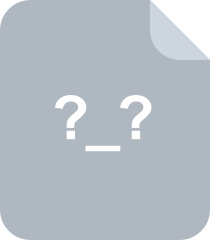
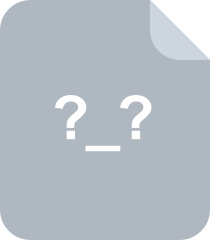
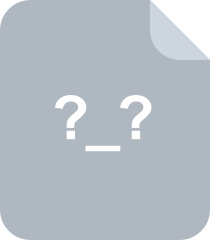
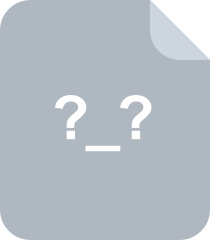
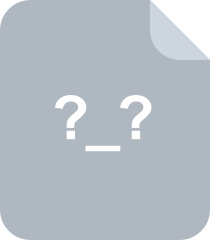
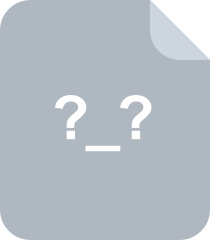
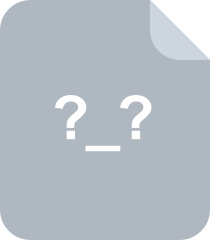
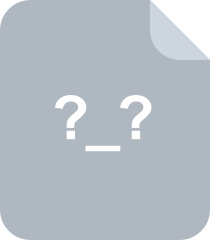
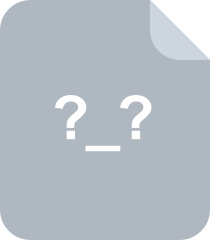
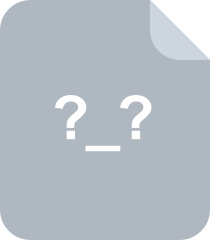
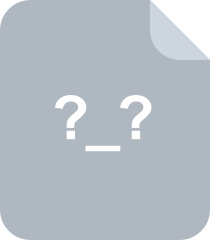
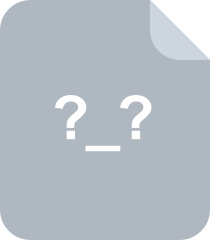
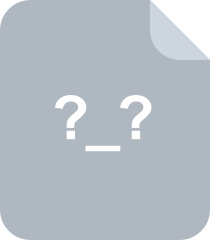
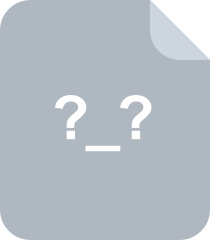
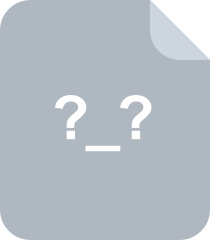
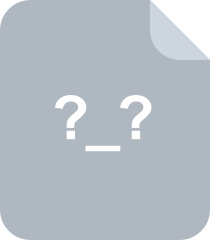
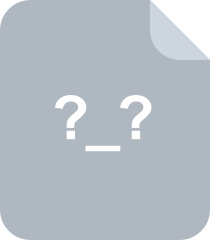
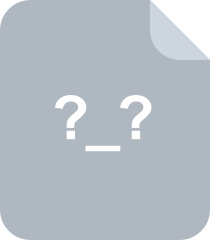
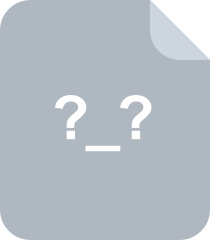
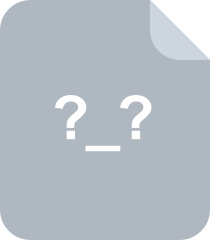
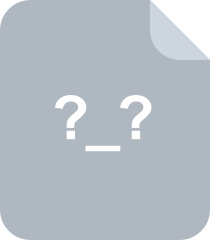
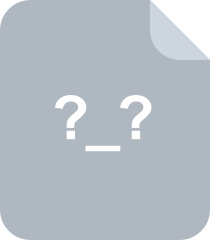
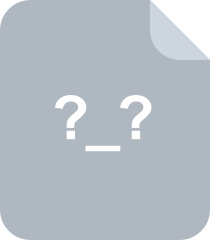
共 331 条
- 1
- 2
- 3
- 4
资源评论
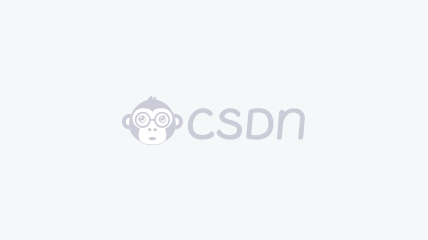

xyq2024
- 粉丝: 2929
- 资源: 5563
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

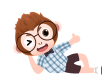
最新资源
- 【岗位说明】广告公司各职员职务说明书(精美版).doc
- 【岗位说明】广告公司各职员职务说明书.doc
- 【岗位说明】广告公司各岗位职责.docx
- 【岗位说明】XX培训机构岗位职责行政职责.doc
- 【岗位说明】风华教育培训中心岗位职责说明书.doc
- 【岗位说明】高校行政人员岗位职责.doc
- 【岗位说明】教师各岗位岗位职责.doc
- 【岗位说明】教学秘书岗位职责.doc
- 【岗位说明】培训机构助教老师岗位职责.doc
- 【岗位说明】培训机构老师岗位职责.doc
- 【岗位说明】培训学校人员岗位职责及任职要求.doc
- 【岗位说明】学校及培训机构岗位职责大全.doc
- 【岗位说明】幼儿园岗位责任制度.doc
- 【岗位说明】幼儿园岗位职责.doc
- 【岗位说明】幼儿园各类人员岗位职责.doc
- 【岗位说明】辅导机构各岗位职责.docx
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


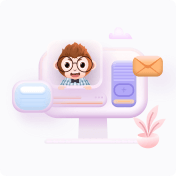
安全验证
文档复制为VIP权益,开通VIP直接复制
