import { isArray, isFunction, set } from 'lodash'
import { request } from '@/utils/request'
import commonApi from '@/api/common'
import tool from '@/utils/tool'
export const allowUseDictComponent = ['radio', 'checkbox', 'select', 'transfer', 'treeSelect', 'tree-select', 'cascader']
export const allowCoverComponent = ['radio', 'checkbox', 'select', 'transfer', 'cascader']
export const requestDict = (url, method, params, data, timeout = 10 * 1000) => request({ url, method, params, data, timeout })
export const handlerDictProps = (item, tmpArr) => {
let data = []
let tran = {}
let colors = {}
let labelName = 'label'
let valueName = 'value'
if (item.dict.name && (!item.dict.url || !item.dict.data)) {
labelName = 'title'
valueName = 'key'
}
if (allowCoverComponent.includes(item.formType)) {
data = tmpArr.map(dicItem => {
const label = dicItem[(item.dict.props && item.dict.props.label) || labelName]
let tmp = dicItem[(item.dict.props && item.dict.props.value) || valueName]
let disabled = (typeof dicItem['disabled'] == 'undefined') ? false : ( dicItem['disabled'] === true ? true : false )
let indeterminate = (typeof dicItem['indeterminate'] == 'undefined') ? false : ( dicItem['indeterminate'] === true ? true : false )
let value
if (item.dict.name || item.dict.data) value = tmp.toString()
else if (tmp === 'true') value = true
else if (tmp === 'false') value = false
else value = tmp
tran[value] = label
colors[value] = item.dict.tagColors && item.dict.tagColors[value] || undefined
return { label, value, disabled, indeterminate }
})
} else {
data = tmpArr
}
data.tran = tran
data.colors = colors
return data
}
export const loadDict = async (dictList, item) => {
if (allowUseDictComponent.includes(item.formType) && item.dict) {
if (item.dict.name) {
const response = await commonApi.getDict(item.dict.name)
if (response.data) {
dictList[item.dataIndex] = handlerDictProps(item, response.data)
}
} else if (item.dict.remote) {
let requestData = {
openPage: item.dict?.openPage ?? false,
remoteOption: item.dict.remoteOption ?? {}
}
requestData = Object.assign(requestData, item.dict.pageOption)
if (requestData.openPage) {
const { data } = await requestDict(item.dict.remote, 'POST', {}, requestData)
dictList[item.dataIndex] = handlerDictProps(item, data.items)
dictList[item.dataIndex].pageInfo = data.pageInfo
} else {
const dictData = tool.local.get('dictData')
if (item.dict.cache && dictData[item.dataIndex]) {
dictList[item.dataIndex] = dictData[item.dataIndex]
} else {
const { data } = await requestDict(item.dict.remote, 'POST', {}, requestData)
dictList[item.dataIndex] = handlerDictProps(item, data)
if (item.dict.cache) {
dictData[item.dataIndex] = dictList[item.dataIndex]
tool.local.set('dictData', dictData)
}
}
}
} else if (item.dict.url) {
let requestData = {
openPage: item.dict?.openPage ?? false,
remoteOption: item.dict.remoteOption ?? {}
}
requestData = Object.assign(requestData, item.dict.pageOption)
if (requestData.openPage) {
if (item.dict?.method === 'GET' || item.dict?.method === 'get') {
item.dict.params = Object.assign(item.dict.params ?? {}, requestData)
} else {
item.dict.body = Object.assign(item.dict.body ?? {}, requestData)
}
const { data } = await requestDict(item.dict.url, item.dict.method || 'GET', item.dict.params || {}, item.dict.body || {})
dictList[item.dataIndex] = handlerDictProps(item, data.items)
dictList[item.dataIndex].pageInfo = data.pageInfo
} else {
const dictData = tool.local.get('dictData')
if (item.dict.cache && dictData[item.dataIndex]) {
dictList[item.dataIndex] = dictData[item.dataIndex]
} else {
const { data } = await requestDict(item.dict.url, item.dict.method || 'GET', item.dict.params || {}, item.dict.body || {})
dictList[item.dataIndex] = handlerDictProps(item, data)
if (item.dict.cache) {
dictData[item.dataIndex] = dictList[item.dataIndex]
tool.local.set('dictData', dictData)
}
}
}
} else if (item.dict.data) {
if (isArray(item.dict.data)) {
dictList[item.dataIndex] = handlerDictProps(item, item.dict.data)
} else if (isFunction(item.dict.data)) {
const response = await item.dict.data()
dictList[item.dataIndex] = handlerDictProps(item, response)
}
}
}
}
const requestCascaderData = async (val, dict, dictList, name) => {
if (dict && (dict.remote || dict.url)) {
let requestData = { openPage: dict?.openPage ?? false, remoteOption: dict.remoteOption ?? {} }
let response
const pageOption = Object.assign(requestData, dict.pageOption)
const url = dict.remote ?? dict.url
if (dict && url.indexOf('{{key}}') > 0) {
response = await requestDict(
url.replace('{{key}}', val), dict.method ?? 'GET',
Object.assign(dict.params || {}, requestData.openPage ? pageOption : {}),
Object.assign(dict.data || {}, requestData.openPage ? pageOption : {})
)
} else {
let temp = Object.assign({ key: val }, requestData.openPage ? pageOption : {})
const params = Object.assign(dict.params || {}, temp)
const data = Object.assign(dict.data || {}, temp)
response = await requestDict(url, dict.method ?? 'GET', params || {}, data || {})
}
if (response.data && response.code === 200) {
let dataIems = requestData.openPage ? response.data.items : response.data
dictList[name] = dataIems.map(dicItem => {
return {
'label': dicItem[ (dict.props && dict.props.label) || 'label' ],
'value': dicItem[ (dict.props && dict.props.value) || 'value' ],
'disabled': (typeof dicItem['disabled'] == 'undefined') ? false : ( dicItem['disabled'] === true ? true : false ),
'indeterminate': (typeof dicItem['indeterminate'] == 'undefined') ? false : ( dicItem['indeterminate'] === true ? true : false )
}
})
dictList[name].pageInfo = response.data.pageInfo ?? undefined
} else {
console.error(response)
}
}
}
export const handlerCascader = async (val, column, columns, dictList, formModel, clearData = true) => {
if (column.cascaderItem && isArray(column.cascaderItem)) {
column.cascaderItem.map(async name => {
const dict = columns.find(col => col.dataIndex === name && col.dict).dict
clearData && set(formModel, name, undefined)
requestCascaderData(val, dict, dictList, name)
})
}
}
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
该设计源码是一款基于Vue3和uniapp技术的全面民宿租赁管理系统,包含1721个文件,其中包括1154张PNG图片、253个JS脚本、251个CSS样式表、37个SVG图形、10个HTML页面、9个GIF动画、2个Markdown文档、2张JPG图片、1个Gitignore配置文件以及1个LICENSE文件。系统支持多端操作,包括租客、房东、管家、总后台和门店,适用于物业托管、民宿长租、短租等多种商业模式。后端管理前端采用Vue3、Vite3、Pinia和Arco技术构建,而租客端、房东端、管家端则通过uniapp实现,支持一次开发,多端运行,可打包为微信、抖音、支付宝小程序,也可作为H5独立运行或打包为Android和iOS应用。
资源推荐
资源详情
资源评论
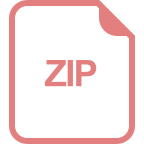
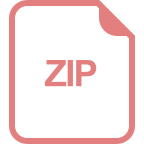
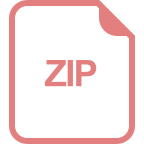
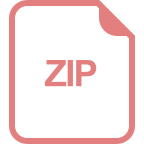
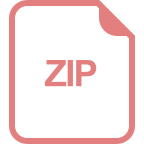
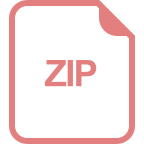
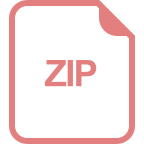
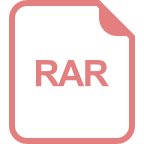
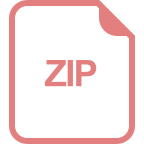
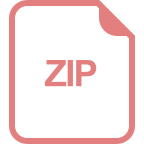
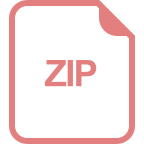
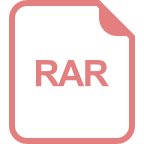
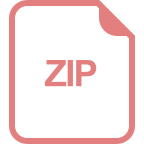
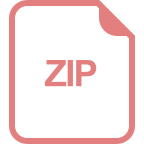
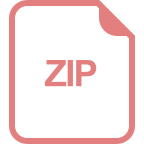
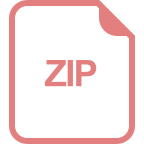
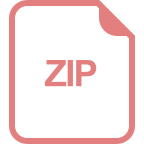
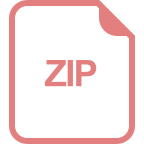
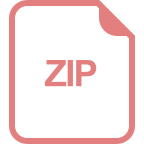
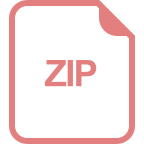
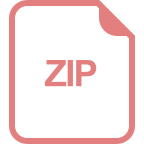
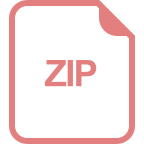
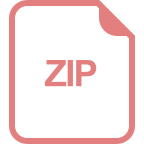
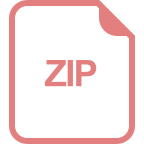
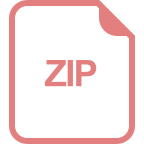
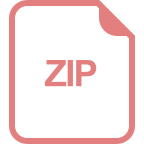
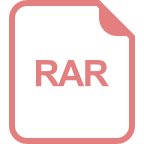
收起资源包目录

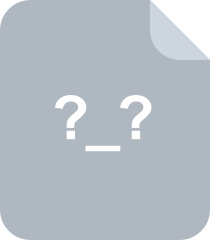
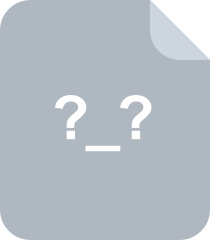
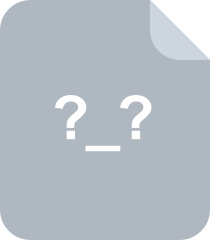
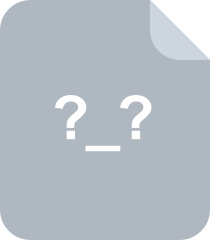
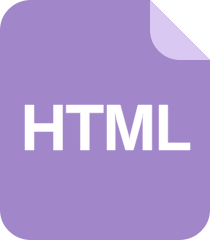
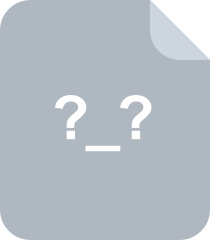
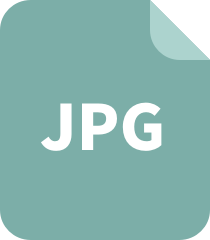
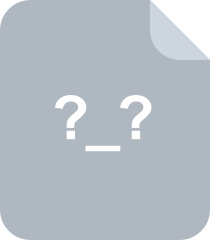
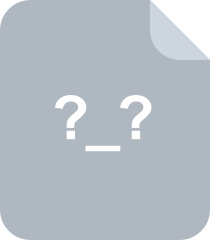
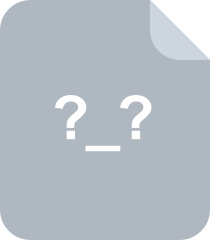
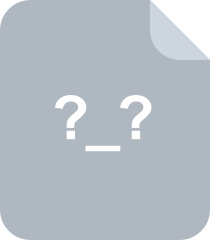
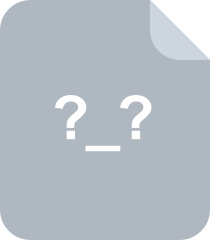
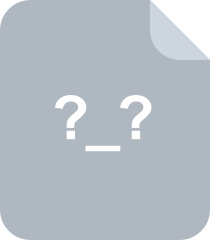
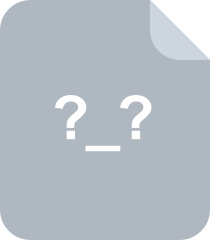
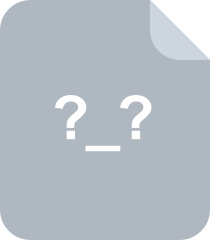
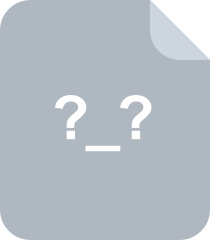
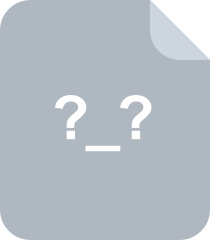
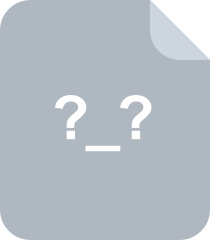
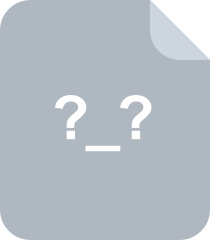
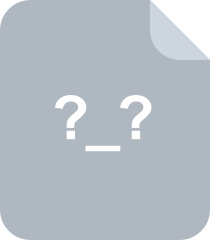
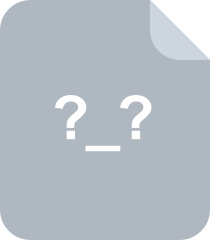
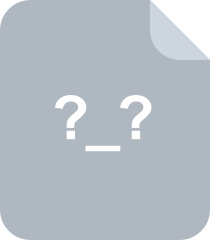
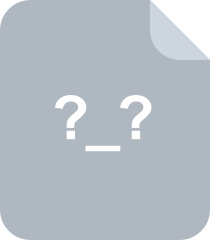
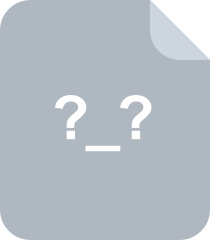
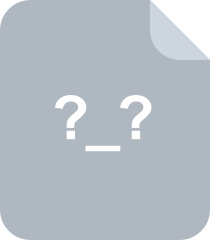
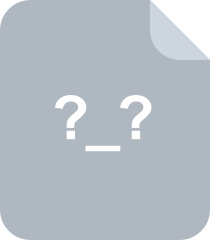
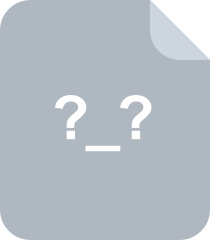
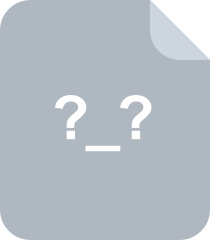
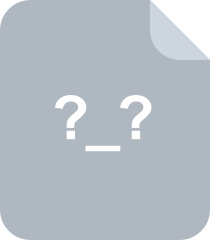
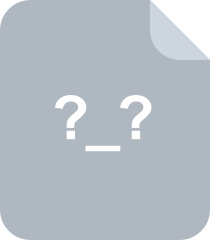
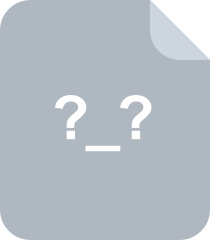
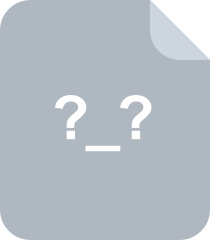
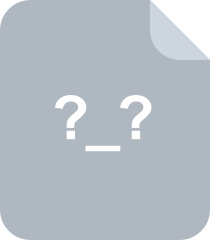
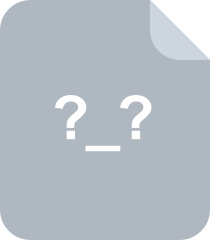
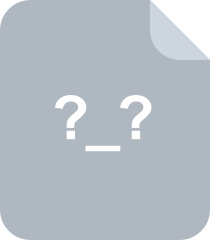
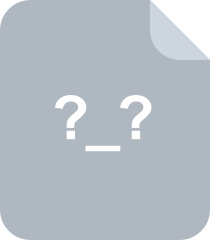
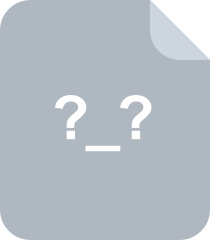
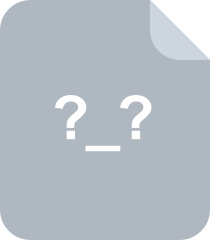
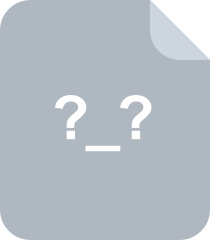
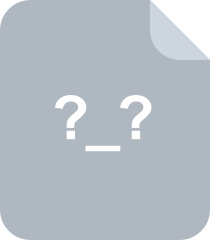
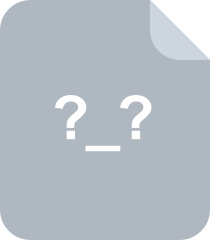
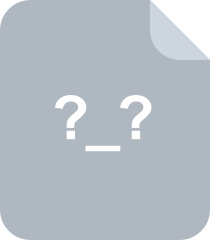
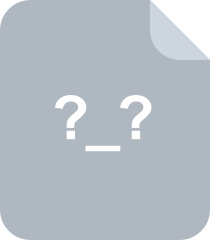
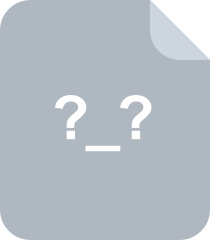
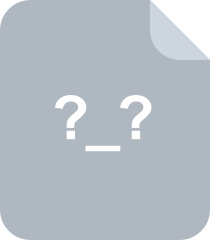
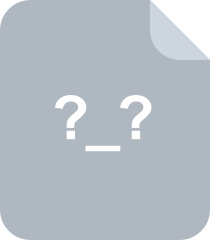
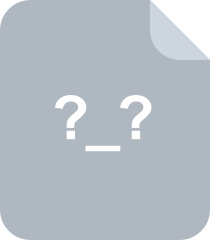
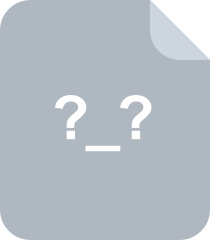
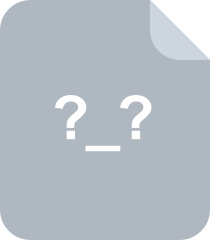
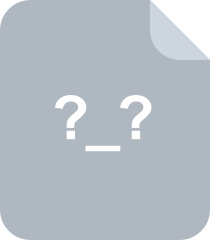
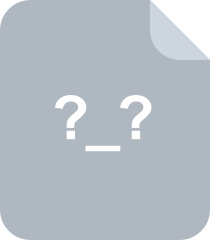
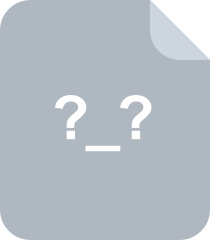
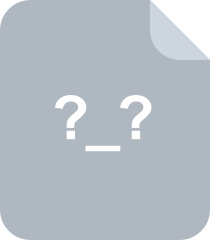
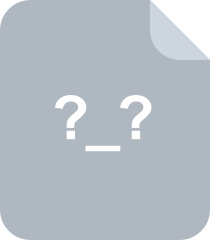
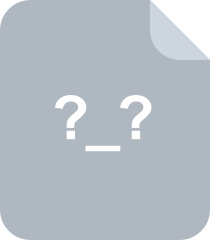
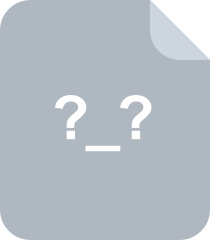
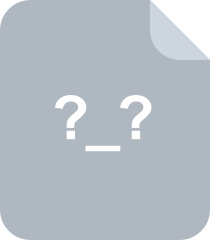
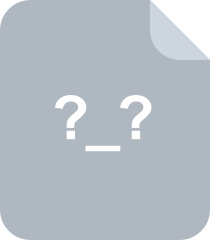
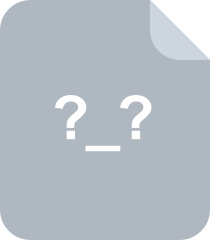
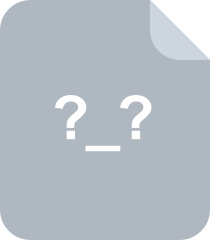
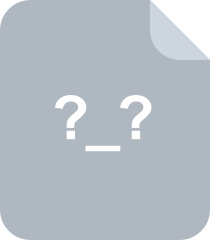
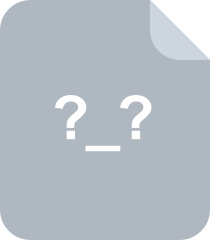
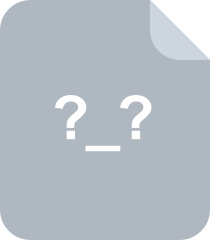
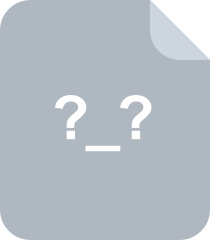
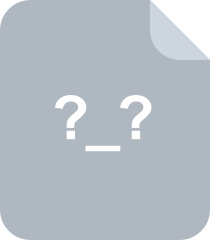
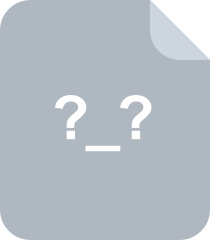
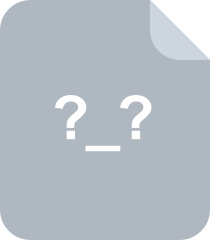
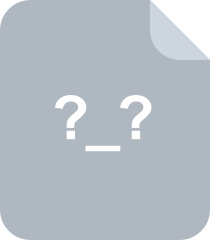
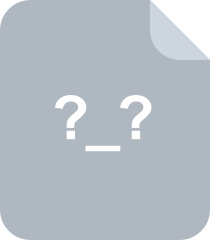
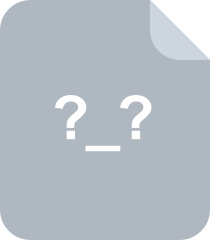
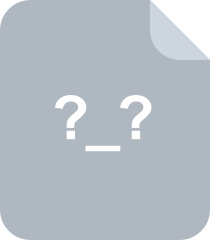
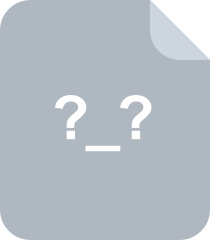
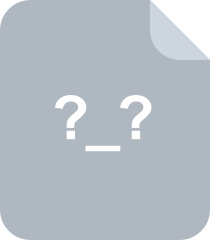
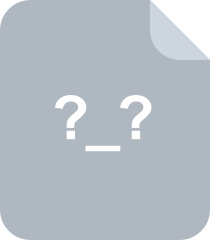
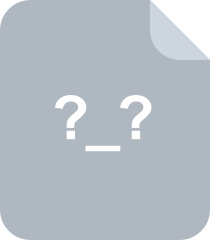
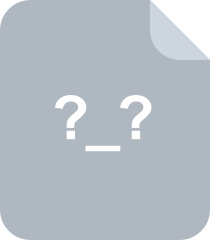
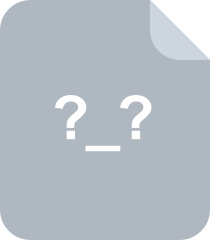
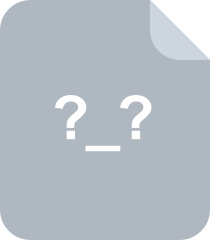
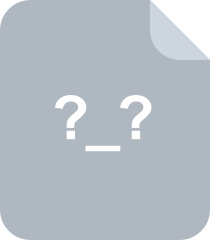
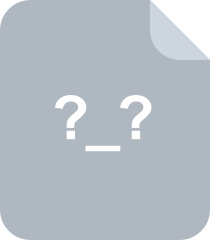
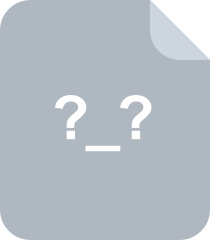
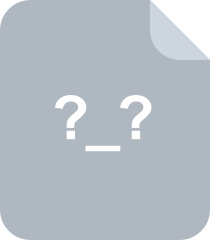
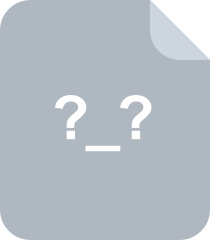
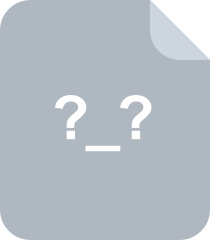
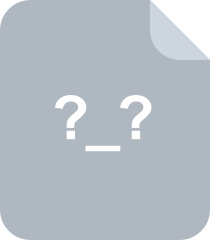
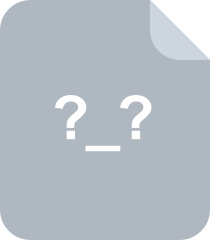
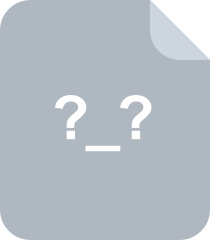
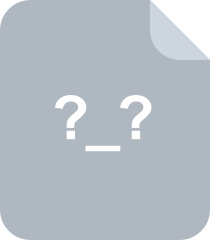
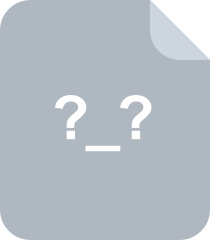
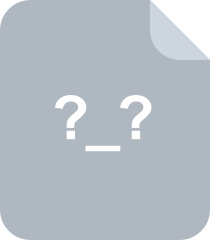
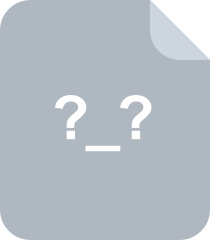
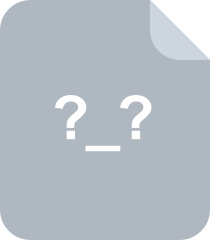
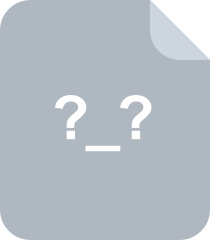
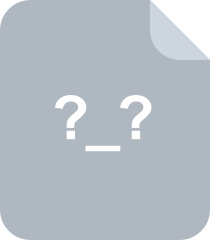
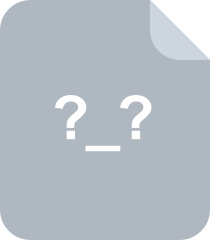
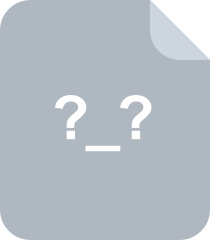
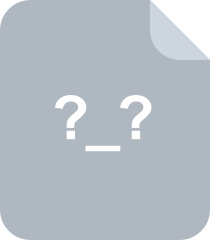
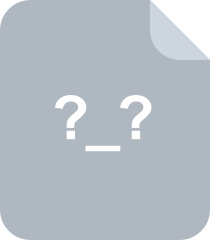
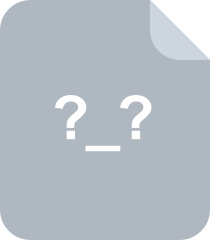
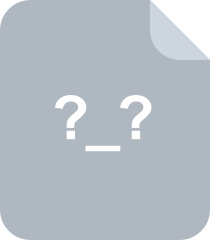
共 389 条
- 1
- 2
- 3
- 4
资源评论
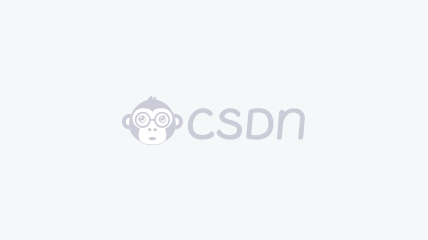

xyq2024
- 粉丝: 2332
- 资源: 5434
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

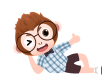
最新资源
- 中国光伏电站安装时间的多边形地理空间数据集(2010-2022年)-最新出炉.zip
- 几种常见简单滤波器用于二维图像降噪,包括均值、中值、高斯、低通、双边滤波器,语言是python
- 二手车管理系统,pc端,小程序端,java后端
- 2011-2022年中国光伏电站遥感识别面矢量数据-最新出炉.zip
- 基于深度学习的边缘计算网络的卸载优化及资源优化python源码+文档说明(高分项目)
- 基于yolov5+超声图像的钢轨缺陷检测python源码+数据集(高分毕设)
- 基于大语言模型的智能审计问答系统python源码+文档说明(高分项目)
- C++程序设计编程题库
- javase停车场管理系统答辩PPT(高级版)
- 軟考 系統架構設計師考試 總結資料
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


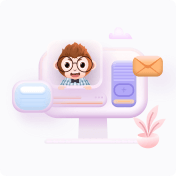
安全验证
文档复制为VIP权益,开通VIP直接复制
