//===--- CSSimplify.cpp - Constraint Simplification -----------------------===//
//
// This source file is part of the Swift.org open source project
//
// Copyright (c) 2014 - 2018 Apple Inc. and the Swift project authors
// Licensed under Apache License v2.0 with Runtime Library Exception
//
// See https://swift.org/LICENSE.txt for license information
// See https://swift.org/CONTRIBUTORS.txt for the list of Swift project authors
//
//===----------------------------------------------------------------------===//
//
// This file implements simplifications of constraints within the constraint
// system.
//
//===----------------------------------------------------------------------===//
#include "CSDiagnostics.h"
#include "TypeCheckConcurrency.h"
#include "TypeCheckEffects.h"
#include "swift/AST/ASTPrinter.h"
#include "swift/AST/Decl.h"
#include "swift/AST/ExistentialLayout.h"
#include "swift/AST/GenericEnvironment.h"
#include "swift/AST/GenericSignature.h"
#include "swift/AST/Initializer.h"
#include "swift/AST/NameLookupRequests.h"
#include "swift/AST/PackExpansionMatcher.h"
#include "swift/AST/ParameterList.h"
#include "swift/AST/PropertyWrappers.h"
#include "swift/AST/ProtocolConformance.h"
#include "swift/AST/Requirement.h"
#include "swift/AST/SourceFile.h"
#include "swift/AST/Types.h"
#include "swift/Basic/Assertions.h"
#include "swift/Basic/StringExtras.h"
#include "swift/ClangImporter/ClangModule.h"
#include "swift/Sema/CSFix.h"
#include "swift/Sema/ConstraintSystem.h"
#include "swift/Sema/IDETypeChecking.h"
#include "llvm/ADT/SetVector.h"
#include "llvm/Support/Compiler.h"
using namespace swift;
using namespace constraints;
MatchCallArgumentListener::~MatchCallArgumentListener() { }
bool MatchCallArgumentListener::extraArgument(unsigned argIdx) { return true; }
std::optional<unsigned>
MatchCallArgumentListener::missingArgument(unsigned paramIdx,
unsigned argInsertIdx) {
return std::nullopt;
}
bool MatchCallArgumentListener::missingLabel(unsigned paramIdx) { return true; }
bool MatchCallArgumentListener::extraneousLabel(unsigned paramIdx) {
return true;
}
bool MatchCallArgumentListener::incorrectLabel(unsigned paramIdx) {
return true;
}
bool MatchCallArgumentListener::outOfOrderArgument(
unsigned argIdx, unsigned prevArgIdx, ArrayRef<ParamBinding> bindings) {
return true;
}
bool MatchCallArgumentListener::relabelArguments(ArrayRef<Identifier> newNames){
return true;
}
bool MatchCallArgumentListener::shouldClaimArgDuringRecovery(unsigned argIdx) {
return true;
}
bool MatchCallArgumentListener::canClaimArgIgnoringNameMismatch(
const AnyFunctionType::Param &arg) {
return false;
}
/// Produce a score (smaller is better) comparing a parameter name and
/// potentially-typo'd argument name.
///
/// \param paramName The name of the parameter.
/// \param argName The name of the argument.
/// \param maxScore The maximum score permitted by this comparison, or
/// 0 if there is no limit.
///
/// \returns the score, if it is good enough to even consider this a match.
/// Otherwise, an empty optional.
///
static std::optional<unsigned> scoreParamAndArgNameTypo(StringRef paramName,
StringRef argName,
unsigned maxScore) {
using namespace camel_case;
// Compute the edit distance.
unsigned dist = argName.edit_distance(paramName, /*AllowReplacements=*/true,
/*MaxEditDistance=*/maxScore);
// If the edit distance would be too long, we're done.
if (maxScore != 0 && dist > maxScore)
return std::nullopt;
// The distance can be zero due to the "with" transformation above.
if (dist == 0)
return 1;
// If this is just a single character label on both sides,
// simply return distance.
if (paramName.size() == 1 && argName.size() == 1)
return dist;
// Only allow about one typo for every two properly-typed characters, which
// prevents completely-wacky suggestions in many cases.
if (dist > (argName.size() + 1) / 3)
return std::nullopt;
return dist;
}
bool constraints::isPackExpansionType(Type type) {
if (type->is<PackExpansionType>())
return true;
if (auto *typeVar = type->getAs<TypeVariableType>())
return typeVar->getImpl().isPackExpansion();
return false;
}
bool constraints::isSingleUnlabeledPackExpansionTuple(Type type) {
auto *tuple = type->getRValueType()->getAs<TupleType>();
return tuple && (tuple->getNumElements() == 1) &&
isPackExpansionType(tuple->getElementType(0)) &&
!tuple->getElement(0).hasName();
}
Type constraints::getPatternTypeOfSingleUnlabeledPackExpansionTuple(Type type) {
if (isSingleUnlabeledPackExpansionTuple(type)) {
auto tuple = type->getRValueType()->castTo<TupleType>();
const auto &tupleElement = tuple->getElementType(0);
if (auto *expansion = tupleElement->getAs<PackExpansionType>()) {
return expansion->getPatternType();
}
if (auto *typeVar = tupleElement->getAs<TypeVariableType>()) {
auto *locator = typeVar->getImpl().getLocator();
if (auto expansionElement =
locator->getLastElementAs<LocatorPathElt::PackExpansionType>()) {
return expansionElement->getOpenedType()->getPatternType();
}
}
}
return {};
}
bool constraints::containsPackExpansionType(ArrayRef<AnyFunctionType::Param> params) {
return llvm::any_of(params, [&](const auto ¶m) {
return isPackExpansionType(param.getPlainType());
});
}
bool constraints::containsPackExpansionType(TupleType *tuple) {
return llvm::any_of(tuple->getElements(), [&](const auto &elt) {
return isPackExpansionType(elt.getType());
});
}
bool constraints::doesMemberRefApplyCurriedSelf(Type baseTy,
const ValueDecl *decl) {
assert(decl->getDeclContext()->isTypeContext() &&
"Expected a member reference");
// For a reference to an instance method on a metatype, we want to keep the
// curried self.
if (decl->isInstanceMember()) {
assert(baseTy);
if (isa<AbstractFunctionDecl>(decl) &&
baseTy->getRValueType()->is<AnyMetatypeType>())
return false;
}
// Otherwise the reference applies self.
return true;
}
static bool areConservativelyCompatibleArgumentLabels(
ConstraintSystem &cs, OverloadChoice choice,
SmallVectorImpl<FunctionType::Param> &args,
MatchCallArgumentListener &listener,
std::optional<unsigned> unlabeledTrailingClosureArgIndex) {
ValueDecl *decl = nullptr;
switch (choice.getKind()) {
case OverloadChoiceKind::Decl:
case OverloadChoiceKind::DeclViaBridge:
case OverloadChoiceKind::DeclViaDynamic:
case OverloadChoiceKind::DeclViaUnwrappedOptional:
decl = choice.getDecl();
break;
// KeyPath application is not filtered in `performMemberLookup`.
case OverloadChoiceKind::KeyPathApplication:
case OverloadChoiceKind::DynamicMemberLookup:
case OverloadChoiceKind::KeyPathDynamicMemberLookup:
case OverloadChoiceKind::TupleIndex:
case OverloadChoiceKind::MaterializePack:
case OverloadChoiceKind::ExtractFunctionIsolation:
return true;
}
// If this is a member lookup, the call arguments (if we have any) will
// generally be applied to the second level of parameters, with the member
// lookup applying the curried self at the first level. But there are cases
// where we can get an unapplied declaration reference back.
auto hasAppliedSelf =
decl->hasCurriedSelf() &&
doesMemberRefApplyCurriedSelf(choice.getBaseType(), decl);
AnyFunctionType *fnType = nullptr;
if (decl->hasParameterList()) {
fnType = decl->getInterfaceType()->castTo<AnyFunctionType>();
if (hasAppliedSelf) {
fnType = fnType->getResult()->getAs<AnyFunctionType>();
assert(fnType && "Parameter list curry level does not match type");
}
} else if (auto *VD = dyn_cas
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
该项目是一套基于Swift语言的iOS和OS X应用开发设计源码,涵盖了19280个文件,包括13652个Swift源文件、1572个头文件(.h)、916个C++源文件(.cpp)、756个Swift接口定义文件(.sil)、313个预期文件(.expected)、232个文本文件(.txt)、225个Python脚本(.py)、201个GYB模板文件(.gyb)、144个JSON配置文件、141个模块映射文件(.modulemap),以及少量其他类型的文件。Swift是苹果公司为OS X和iOS应用开发而推出的现代编程语言,以其简洁、快速、安全和高效的特性超越了Objective-C,同时保持与Objective-C的兼容性。
资源推荐
资源详情
资源评论



























收起资源包目录

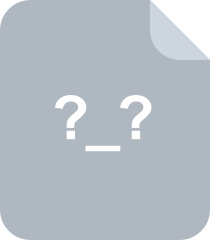
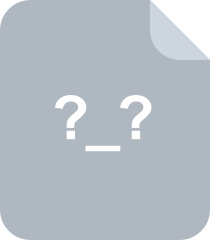
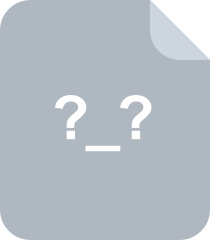
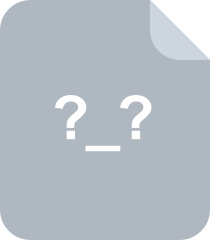
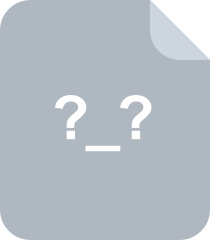
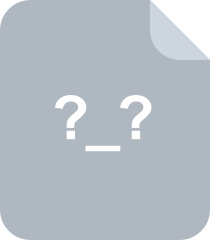
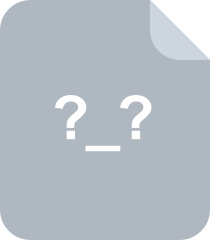
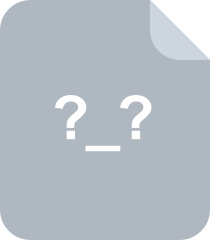
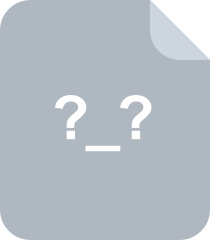
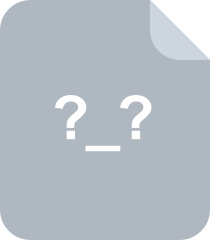
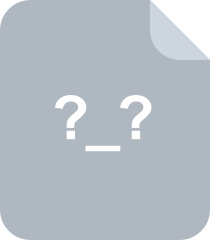
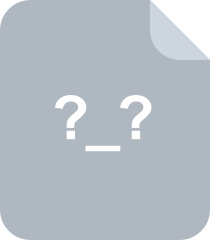
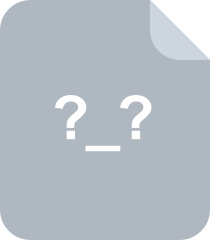
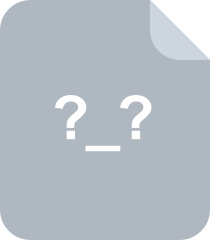
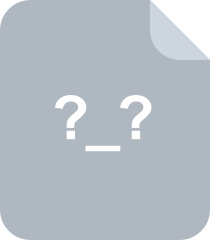
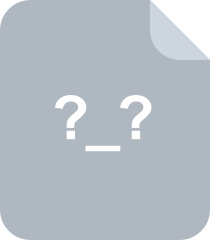
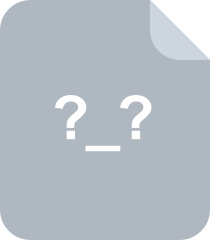
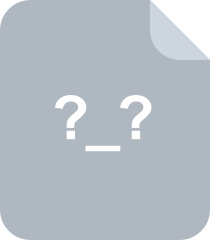
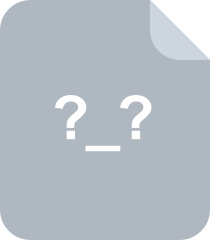
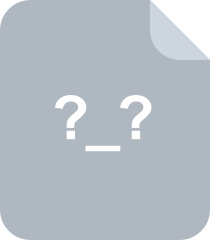
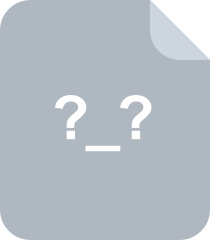
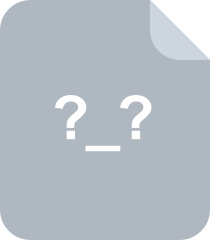
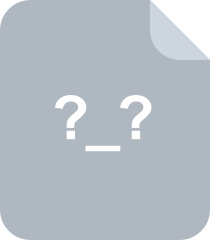
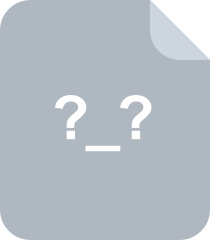
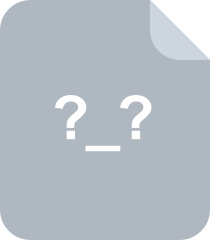
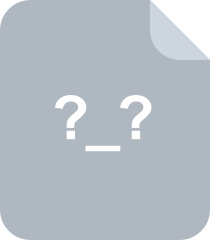
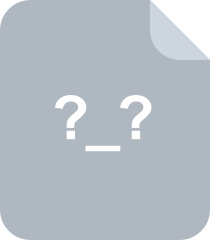
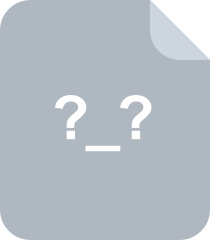
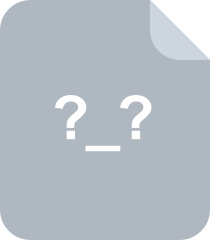
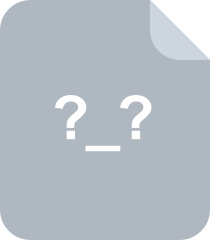
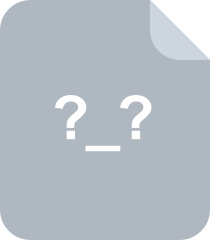
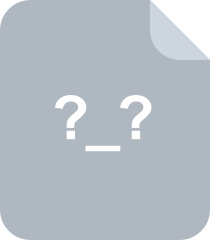
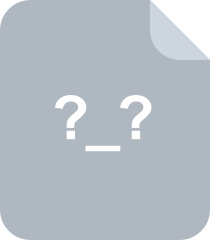
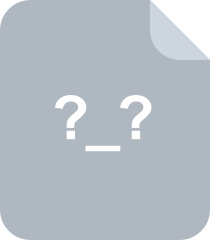
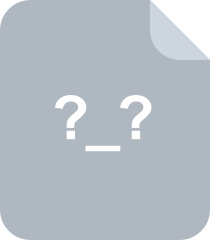
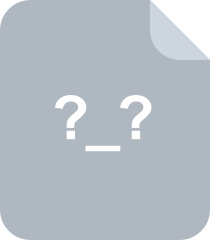
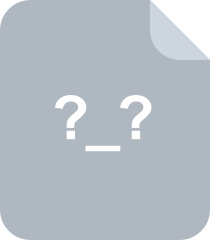
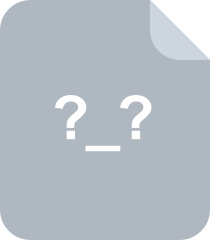
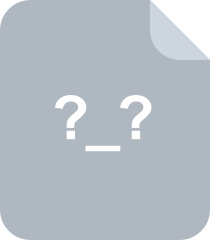
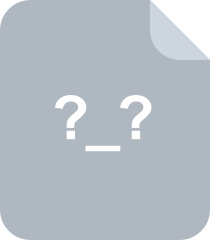
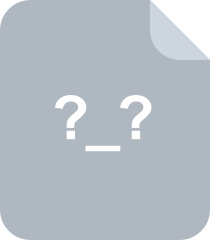
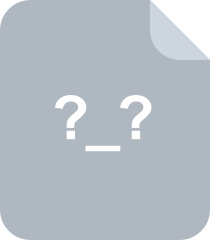
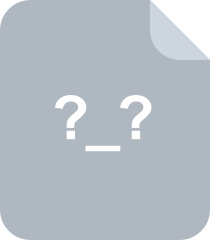
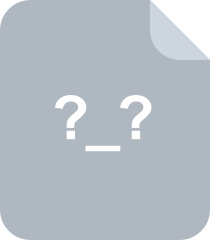
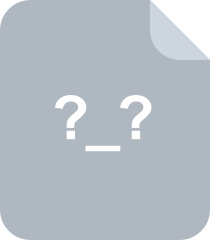
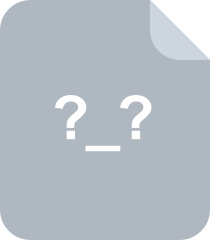
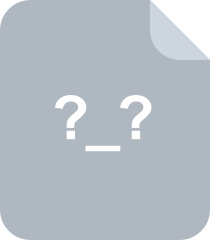
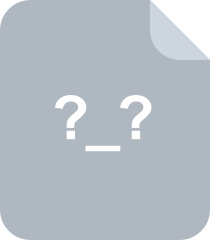
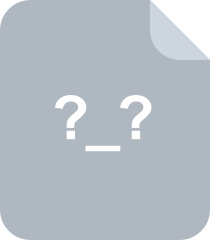
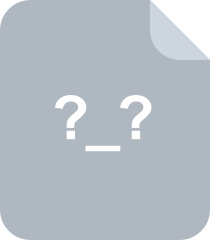
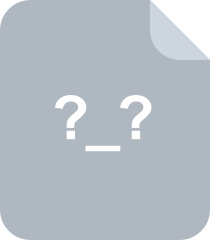
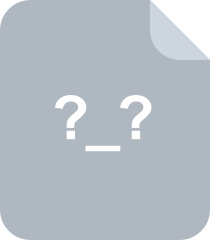
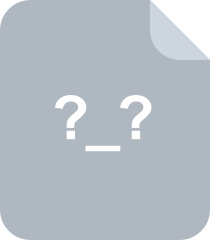
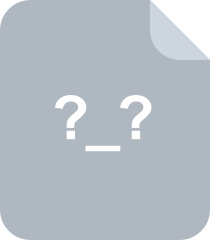
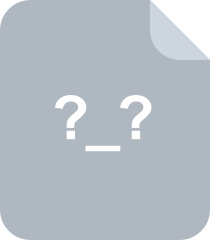
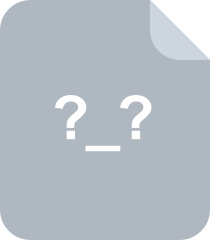
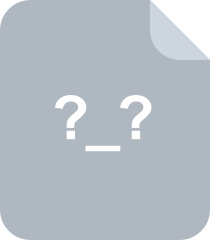
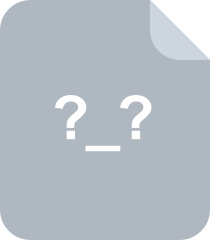
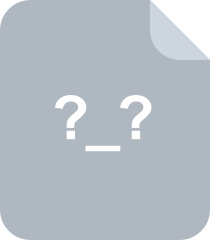
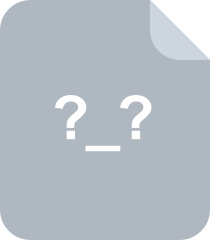
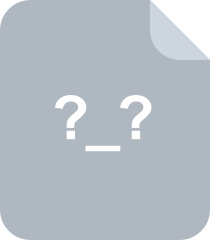
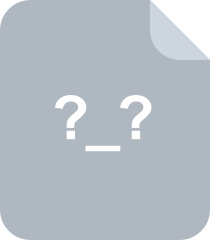
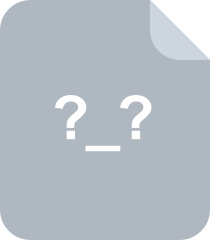
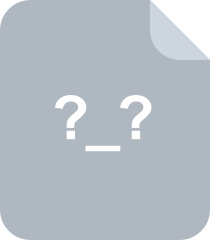
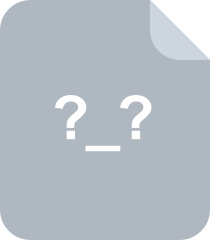
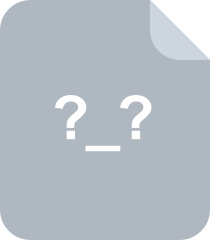
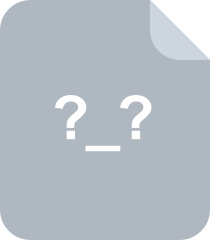
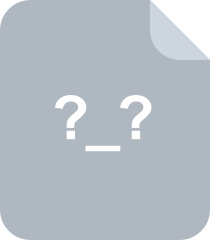
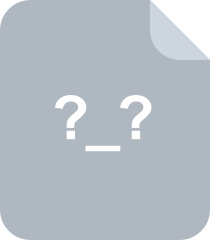
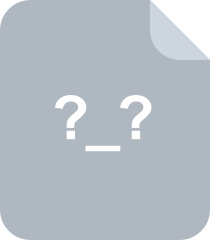
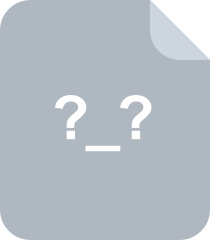
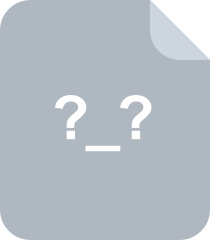
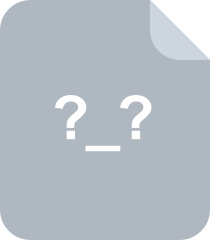
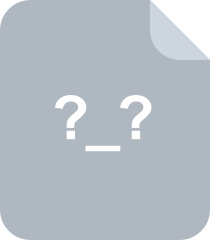
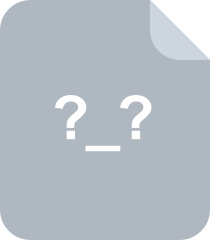
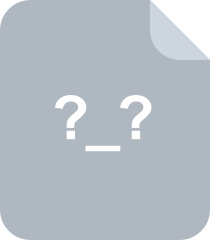
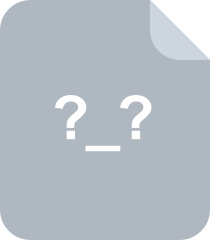
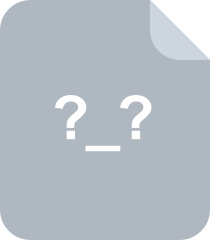
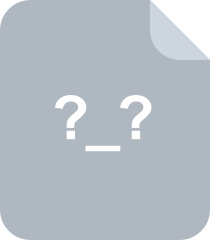
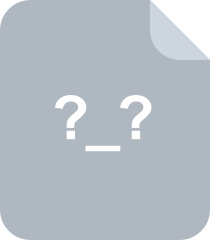
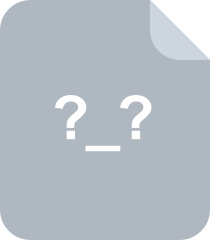
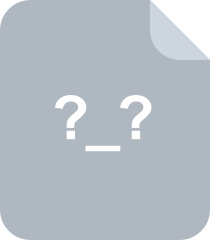
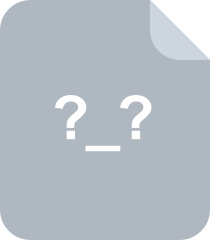
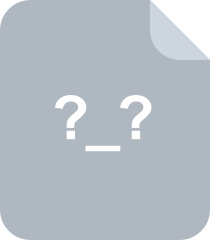
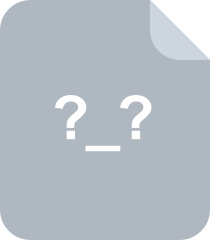
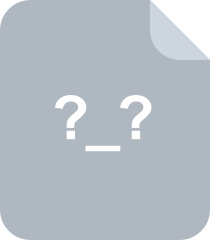
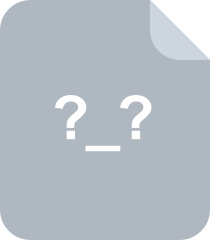
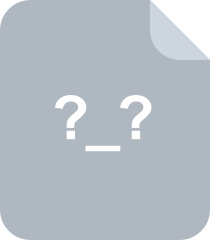
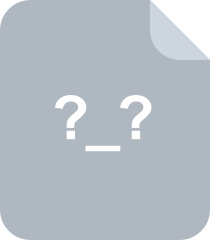
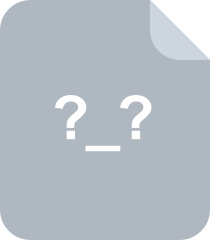
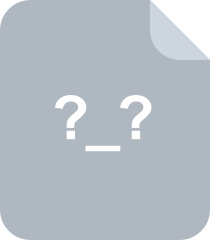
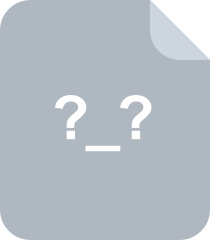
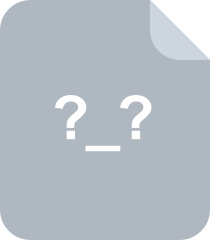
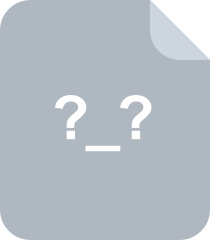
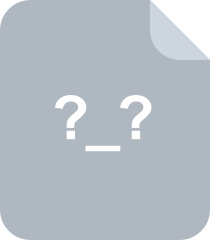
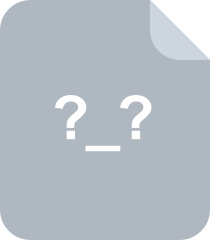
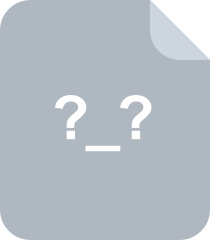
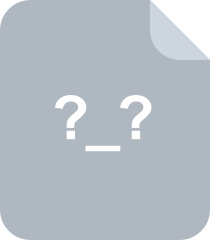
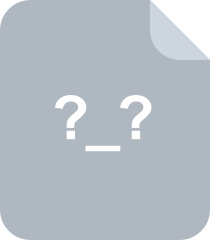
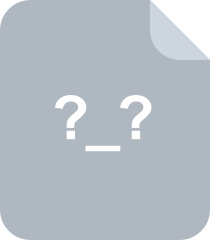
共 2000 条
- 1
- 2
- 3
- 4
- 5
- 6
- 20
资源评论
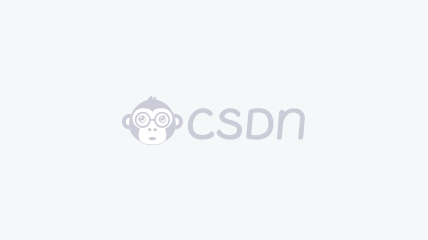

xyq2024
- 粉丝: 3479
- 资源: 6662
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

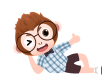
最新资源
- [AB PLC例程源码]示例程序2(船用).zip
- [AB PLC例程源码]上海飞奥做的天燃器末站PLC系统程序.zip
- [AB PLC例程源码]水厂程序.zip
- [AB PLC例程源码]水厂程序(1).zip
- [AB PLC例程源码]小型压合机.zip
- [AB PLC例程源码]天津锦湖轮胎的设备程序.zip
- [AB PLC例程源码]污水厂的处理系统.zip
- [AB PLC例程源码]一个500伺服程序.zip
- [AB PLC例程源码]一个DNET的SLC500实例.zip
- [AB PLC例程源码]一个AB PID控制的例子.zip
- [AB PLC例程源码]一个RSEmulator5000的测试小程序.zip
- [AB PLC例程源码]一个PLC5实例.zip
- [AB PLC例程源码]一个RSLOGIX500编的程序.zip
- [AB PLC例程源码]一条自动输送生产线程序(SLC500).zip
- [AB PLC例程源码]一个初学者可以看懂的SLC500 程序.zip
- [AB PLC例程源码]一个自己做的AB的pid模拟程序,供新人学习.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


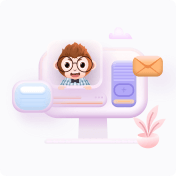
安全验证
文档复制为VIP权益,开通VIP直接复制
