#include <Arduino.h>
#include <xfont.h>
#ifdef ARDUINO_GFX
#include "Arduino_GFX_Library.h"
#elif defined(TFT_ESPI)
#include <TFT_eSPI.h>
#endif
XFont::XFont()
{
// Serial.println("Init.");
// InitTFT();
XFont(false);
}
#ifdef ARDUINO_GFX
XFont::XFont(Arduino_GFX *gfx_tft)
{
unsigned long beginTime = millis();
InitTFT(gfx_tft);
//初始化字库,获得字库中的所有字符集
Serial.printf(" TFT初始化耗时:%2f 秒.\r\n",(millis() - beginTime)/1000.0);
beginTime = millis();
initZhiku(fontFilePath);
Serial.printf(" 装载字符集耗时:%2f 秒.\r\n",(millis() - beginTime)/1000.0);
}
#endif
XFont::XFont(bool isTFT)
{
unsigned long beginTime = millis();
if(isTFT==true)
{
InitTFT();
//初始化字库,获得字库中的所有字符集
Serial.printf(" TFT初始化耗时:%2f 秒.\r\n",(millis() - beginTime)/1000.0);
}
beginTime = millis();
initZhiku(fontFilePath);
Serial.printf(" 装载字符集耗时:%2f 秒.\r\n",(millis() - beginTime)/1000.0);
}
XFont:: ~XFont(void){
clear();
}
void XFont:: clear(void){
if(fontFile)fontFile.close();
strAllUnicodes=String("");
}
void XFont::InitTFT()
{
#ifdef ARDUINO_GFX
if (!tft->begin())
{
Serial.println("gfx->begin() failed!");
}
#ifdef GFX_BL
pinMode(GFX_BL, OUTPUT);
digitalWrite(GFX_BL, HIGH);
#endif
tft->setRotation(1);
tft->setCursor(10, 10);
tft->fillScreen(BLACK);
tft->setTextColor(GREEN);
// delay(200); // 5 seconds
#elif defined(TFT_ESPI)
tft.begin();
tft.setRotation(1);
tft.fillScreen(TFT_BLACK);
tft.setTextColor(TFT_GREEN);
// tft.drawString("pls waiting!",10, 10);
// tft.drawPixel(1,1,TFT_GREEN);
// tft.drawPixel(100,100,TFT_GREEN);
// tft.println("pls waiting.");
// tft.drawString("pls wait.",10,10);
#endif
isTftInited=true;
}
#ifdef ARDUINO_GFX
void XFont:: InitTFT(Arduino_GFX *gfx_tft){
tft=gfx_tft;
if (!tft->begin())
{
Serial.println("gfx->begin() failed!");
}
#ifdef GFX_BL
pinMode(GFX_BL, OUTPUT);
digitalWrite(GFX_BL, HIGH);
#endif
tft->setRotation(1);
tft->setCursor(10, 10);
tft->fillScreen(BLACK);
tft->setTextColor(GREEN);
isTftInited=true;
}
#endif
// 转化字符数组为字符串
String XFont::getStringFromChars(uint8_t *bs, int l)
{
String ret;
// ret=(char *)bs;
// int l=(int)(strlen(*bs));
// int l=sizeof(bs) ;
// Serial.println(l);
// ret.reserve(l);
// for (int i = 0; i < l; i++)
// {
// ret += (char)bs[i];
// // Serial.println(ret.length());
// }
// return ret;
char* input2 = (char *) bs;
ret = input2;
// Serial.println(ret);
return ret.substring(0,l);
}
// 把utf8编码字符转unicode编码
String XFont::getUnicodeFromUTF8(String s)
{
// Serial.println(s.length());
// 32-127
char character[s.length()];
String string_to_hex = "";
int n = 0;
for (uint16_t i = 0; i < s.length(); i++)
{
// if (s[i] > 0 and s[i] < 32)
// { // 特判ascii小于 32
// if (s[i] == 10 or s[i] == 13)
// { // 特判回车换行
// string_to_hex += "000a";
// continue;
// }
// if (s[i] == 9)
// { // 特判回车换行
// string_to_hex += "0009";
// continue;
// }
// s[i] = 32;
// }
if (s[i] >= 1 and s[i] <= 127)
{
// Serial.print(s[i]+" :");
// Serial.println(s[i],DEC);
String ss1 = String(s[i], HEX);
ss1 = ss1.length() == 1 ? "0" + ss1 : ss1;
string_to_hex += "00" + ss1;
}
else
{
character[n + 1] = ((s[i + 1] & 0x3) << 6) + (s[i + 2] & 0x3F);
character[n] = ((s[i] & 0xF) << 4) + ((s[i + 1] >> 2) & 0xF);
String ss1 = String(character[n], HEX);
String ss2 = String(character[n + 1], HEX);
// 下面的代码用于处理出现x xx和xx x这种情况,补足前面的0为0x xx或者xx 0x
string_to_hex += ss1.length() == 1 ? "0" + ss1 : ss1;
string_to_hex += ss2.length() == 1 ? "0" + ss2 : ss2;
n = n + 2;
i = i + 2;
}
}
// Serial.println(string_to_hex);
return string_to_hex;
}
// 依照字号和编码方式计算每个字符存储展位
int XFont::getFontPage(int font_size, int bin_type)
{
int total = font_size * font_size;
int hexCount = 8;
if (bin_type == 32)
hexCount = 10;
if (bin_type == 64)
hexCount = 12;
int hexAmount = int(total / hexCount);
if (total % hexCount > 0)
{
hexAmount += 1;
// total+=hexCount-total%hexCount;
}
// print(hexAmount*2,total)
return hexAmount * 2;
}
// 从字符的像素64进制字符重新转成二进制字符串
String XFont::getPixDataFromHex(String s)
{
// Serial.println(s);
int l = s.length();
// char ch[font_size * font_size];
// char * ch2=(char*)malloc(font_size * font_size * sizeof(char));;
int cnt=0;
String ret="";
// 注意,这里是两个字符进行的处理
int cc = 5;
for (int ii = 0; ii < l; ii++)
{
int d=strchr( s64,s[ii])-s64;
// Serial.println(d);
// 下面用了bitread来获取数字对应的二进制,bitread(value,k)是读取数字value中的二进制的第k位的值。
// 使用bitread就没有使用getBin这种方式了,但是保留了两种getbin函数
for (int k = cc; k >= 0; k--)
{
// ch[cnt]=48+(int)bitRead(d, k);
ret+=(String)bitRead(d, k);
// ch2[cnt]=48+(int)bitRead(d, k);
// ch2[cnt]=ch[cnt];
cnt++;
}
}
// ret=(String)ch2;
// ch2=" ";
// free(ch2) ;
// Serial.println(ch2);
// return ret.substring(0,font_size * font_size);
return ret;
}
void XFont::reInitZhiku(String fontPath){
isInit=false;
initZhiku( fontPath);
}
void XFont::initZhiku(String fontPath)
{
if (isInit == true)
return;
if(LittleFS.begin()==false){
Serial.println("littleFS 文件系统初始化失败,请检查相关配置。");
return;
}
if (LittleFS.exists(fontPath))
{
fontFile = LittleFS.open(fontPath, "r");
static uint8_t buf_total_str[6];
static uint8_t buf_font_size[2];
static uint8_t buf_bin_type[2];
// Serial.println(file.position());
fontFile.read(buf_total_str, 6);
// Serial.println(file.position());
fontFile.read(buf_font_size, 2);
fontFile.read(buf_bin_type, 2);
// 下面代码获取总字数和字号
String s1 = getStringFromChars(buf_total_str, 6);
String s2 = getStringFromChars(buf_font_size, 2);
String s3 = getStringFromChars(buf_bin_type, 2);
total_font_cnt = strtoll(s1.c_str(), NULL, 16);
font_size = s2.toInt();
bin_type = s3.toInt();
font_page = getFontPage(font_size, bin_type);
// free(buf_total_str);free(buf_font_size);free(buf_bin_type);
// Serial.println(s1);
// Serial.println(s2);
// Serial.println(s3);
// Serial.println(font_page);
// 待读取的总编码长度,每个字都对应着一个uxxxx,所以乘5
font_unicode_cnt = total_font_cnt * 5;
// String font_unicode = "";
// 如果是esp32系统,因为ram比较大,一次性读取出所有字符
// #if defined(ESP32)
// uint8_t *buf_total_str_unicode;
// buf_total_str_unicode = (uint8_t *)malloc(font_unicode_cnt);
// Serial.println(font_u


应用市场
- 粉丝: 943
- 资源: 4246
最新资源
- 某名企年度培训计划.doc
- 年度培训计划表.doc
- 年度培训预算制订的几个困惑.doc
- 年度培训计划制定五步曲.doc
- 培训制度.doc
- 企业集团员工培训计划(2016年度)(DOC 5页).doc
- 企业如何做培训规划.doc
- 企业年度培训计划制定实务.doc
- 新人入职15天强化培训计划(DOC 7页).doc
- 傻瓜式开展年度培训规划工作.doc
- 宇辉2015培训方案(管理人员)(DOC 8页).doc
- 逸阳服饰2015年培训规划.doc
- 2024年中国经济复苏与出口新动能研究报告
- 通过python实现一个堆排序示例代码.zip
- 02助代-集团消费品经营理念(ppt 15)).PPT
- 03助代-营业人员专业准则.PPT
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


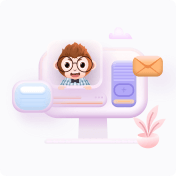