/*
* This program is free software; you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation; either version 2 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program; if not, write to the Free Software
* Foundation, Inc., 675 Mass Ave, Cambridge, MA 02139, USA.
*/
/*
* WLSVM.java
* Copyright (C) 2005 Yasser EL-Manzalawy
*
*/
/*
* An implementation of a custom Weka classifier that provides an access to LibSVM.
* Available at: http://www.cs.iastate.edu/~yasser/wlsvm
*/
package wlsvm;
import weka.classifiers.Classifier;
import weka.core.*;
import weka.filters.*;
import weka.filters.unsupervised.attribute.*;
import weka.classifiers.*;
import libsvm.*;
import java.util.*;
public class WLSVM extends Classifier implements WeightedInstancesHandler {
protected static final long serialVersionUID = 14172;
protected svm_parameter param; // LibSVM oprions
protected int normalize; // normalize input data
protected svm_problem prob; // LibSVM Problem
protected svm_model model; // LibSVM Model
protected String error_msg;
protected Filter filter = null;
public WLSVM() {
String[] dummy = {};
try{
setOptions(dummy);
} catch (Exception e) {
e.printStackTrace();
}
}
/** The filter used to make attributes numeric. */
//protected NominalToBinary m_NominalToBinary = null;
/**
* Returns a string describing classifier
*
* @return a description suitable for displaying in the
* explorer/experimenter gui
*/
public String globalInfo() {
return "An implementation of a custom Weka classifier that provides an access to LibSVM."+
"Available at: http://www.cs.iastate.edu/~yasser/wlsvm";
}
/**
* Returns an enumeration describing the available options.
*
* @return an enumeration of all the available options.
*/
public Enumeration listOptions() {
Vector newVector = new Vector(13);
newVector.addElement(new Option("\t set type of SVM (default 0)\n"
+ "\t\t 0 = C-SVC\n" + "\t\t 1 = nu-SVC\n"
+ "\t\t 2 = one-class SVM\n" + "\t\t 3 = epsilon-SVR\n"
+ "\t\t 4 = nu-SVR", "S", 1, "-S <int>"));
newVector
.addElement(new Option(
"\t set type of kernel function (default 2)\n"
+ "\t\t 0 = linear: u'*v\n"
+ "\t\t 1 = polynomial: (gamma*u'*v + coef0)^degree\n"
+ "\t\t 2 = radial basis function: exp(-gamma*|u-v|^2)\n"
+ "\t\t 3 = sigmoid: tanh(gamma*u'*v + coef0)",
"K", 1, "-K <int>"));
newVector.addElement(new Option(
"\t set degree in kernel function (default 3)", "D", 1,
"-D <int>"));
newVector.addElement(new Option(
"\t set gamma in kernel function (default 1/k)", "G", 1,
"-G <double>"));
newVector.addElement(new Option(
"\t set coef0 in kernel function (default 0)", "R", 1,
"-R <double>"));
newVector
.addElement(new Option(
"\t set the parameter C of C-SVC, epsilon-SVR, and nu-SVR (default 1)",
"C", 1, "-C <double>"));
newVector
.addElement(new Option(
"\t set the parameter nu of nu-SVC, one-class SVM, and nu-SVR (default 0.5)",
"N", 1, "-N <double>"));
newVector.addElement(new Option(
"\t whether to normalize input data, 0 or 1 (default 0)", "Z",
1, "-Z"));
newVector
.addElement(new Option(
"\t set the epsilon in loss function of epsilon-SVR (default 0.1)",
"P", 1, "-P <double>"));
newVector.addElement(new Option(
"\t set cache memory size in MB (default 40)", "M", 1,
"-M <double>"));
newVector.addElement(new Option(
"\t set tolerance of termination criterion (default 0.001)",
"E", 1, "-E <double>"));
newVector.addElement(new Option(
"\t whether to use the shrinking heuristics, 0 or 1 (default 1)",
"H", 1, "-H <int>"));
newVector.addElement(new Option(
"\t whether to train a SVC or SVR model for probability estimates, 0 or 1 (default 0)",
"B", 1, "-B <int>"));
newVector.addElement(new Option(
"\t set the parameters C of class i to weight[i]*C, for C-SVC (default 1)",
"W", 1, "-W <double>"));
return newVector.elements();
}
/**
* Sets type of SVM (default 0)
*
* @param svm_type
*/
public void setSVMType(int svm_type) {
param.svm_type = svm_type;
}
/**
* Gets type of SVM
*
* @return
*/
public int getSVMType() {
return param.svm_type;
}
/**
* Sets type of kernel function (default 2)
*
* @param kernel_type
*/
public void setKernelType(int kernel_type) {
param.kernel_type = kernel_type;
}
/**
* Gets type of kernel function
*
* @return
*/
public int getKernelType() {
return param.kernel_type;
}
/**
* Sets the degree of the kernel
*
* @param degree
*/
public void setDegree(double degree) {
param.degree = degree;
}
/**
* Gets the degree of the kernel
*
* @return
*/
public double getDegree() {
return param.degree;
}
/**
* Sets gamma (default = 1/no of attributes)
*
* @param gamma
*/
public void setGamma(double gamma) {
param.gamma = gamma;
}
/**
* Gets gamma
*
* @return
*/
public double getGamma() {
return param.gamma;
}
/**
* Sets coef (default 0)
*
* @param coef0
*/
public void setCoef0(double coef0) {
param.coef0 = coef0;
}
/**
* Gets coef
*
* @return
*/
public double getCoef0() {
return param.coef0;
}
/**
* Sets nu of nu-SVC, one-class SVM, and nu-SVR (default 0.5)
*
* @param nu
*/
public void setNu(double nu) {
param.nu = nu;
}
/**
* Gets nu of nu-SVC, one-class SVM, and nu-SVR (default 0.5)
*
* @return
*/
public double getNu() {
return param.nu;
}
/**
* Sets cache memory size in MB (default 40)
*
* @param cache_size
*/
public void setCache(double cache_size) {
param.cache_size = cache_size;
}
/**
* Gets cache memory size in MB
*
* @return
*/
public double getCache() {
return param.cache_size;
}
/**
* Sets the parameter C of C-SVC, epsilon-SVR, and nu-SVR (default 1)
*
* @param cost
*/
public void setCost(double cost) {
param.C = cost;
}
/**
* Sets the parameter C of C-SVC, epsilon-SVR, and nu-SVR
*
* @return
*/
public double getCost() {
return param.C;
}
/**
* Sets tolerance of termination criterion (default 0.001)
*
* @param eps
*/
public void setEps(double eps) {
param.eps = eps;
}
/**
* Gets tolerance of termination criterion
*
* @return
*/
public double getEps() {
return param.eps;
}
/**
* Sets the epsilon in loss function of epsilon-SVR (default 0.1)
*
* @param loss
*/
public void setLoss(double loss) {
param.p = loss;
}
/**
* Gets the epsilon in loss function of epsilon-SVR
*
* @return
*/
public double getLoss() {
return param.p;
}
/**
* whether to use the shrinking heuristics, 0 or 1 (default 1)
*
* @param shrink
*/
public void setShrinking(int shrink) {
param.shrinking = shrink;
}
/**
* whether to use the shrinking heuristics, 0 or 1 (default 1)
*
* @return
*/
public double getShrinking() {
return param.shrinking;
}
/**
* whether to train a SVC or SVR model for probability estimates, 0 or

子龙朱雀
- 粉丝: 7
- 资源: 2
最新资源
- apache-maven-3.6.1-bin.zip
- c593f5fc-d4a7-4b43-8ab2-51afc90f3f62
- IIR滤波器参数计算函数
- WPF树菜单拖拽功能,下级目录拖到上级目录,上级目录拖到下级目录.zip
- CDH6.3.2版本hive2.1.1修复HIVE-14706后的jar包
- 鸿蒙项目实战-天气项目(当前城市天气、温度、湿度,24h天气,未来七天天气预报,生活指数,城市选择等)
- Linux环境下oracle数据库服务器配置中文最新版本
- Linux操作系统中Oracle11g数据库安装步骤详细图解中文最新版本
- SMA中心接触件插合力量(插入力及分离力)仿真
- 变色龙记事本,有NPP功能,JSONview功能
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


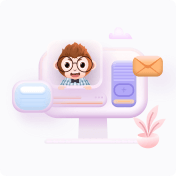
- 1
- 2
- 3
- 4
- 5
- 6
前往页