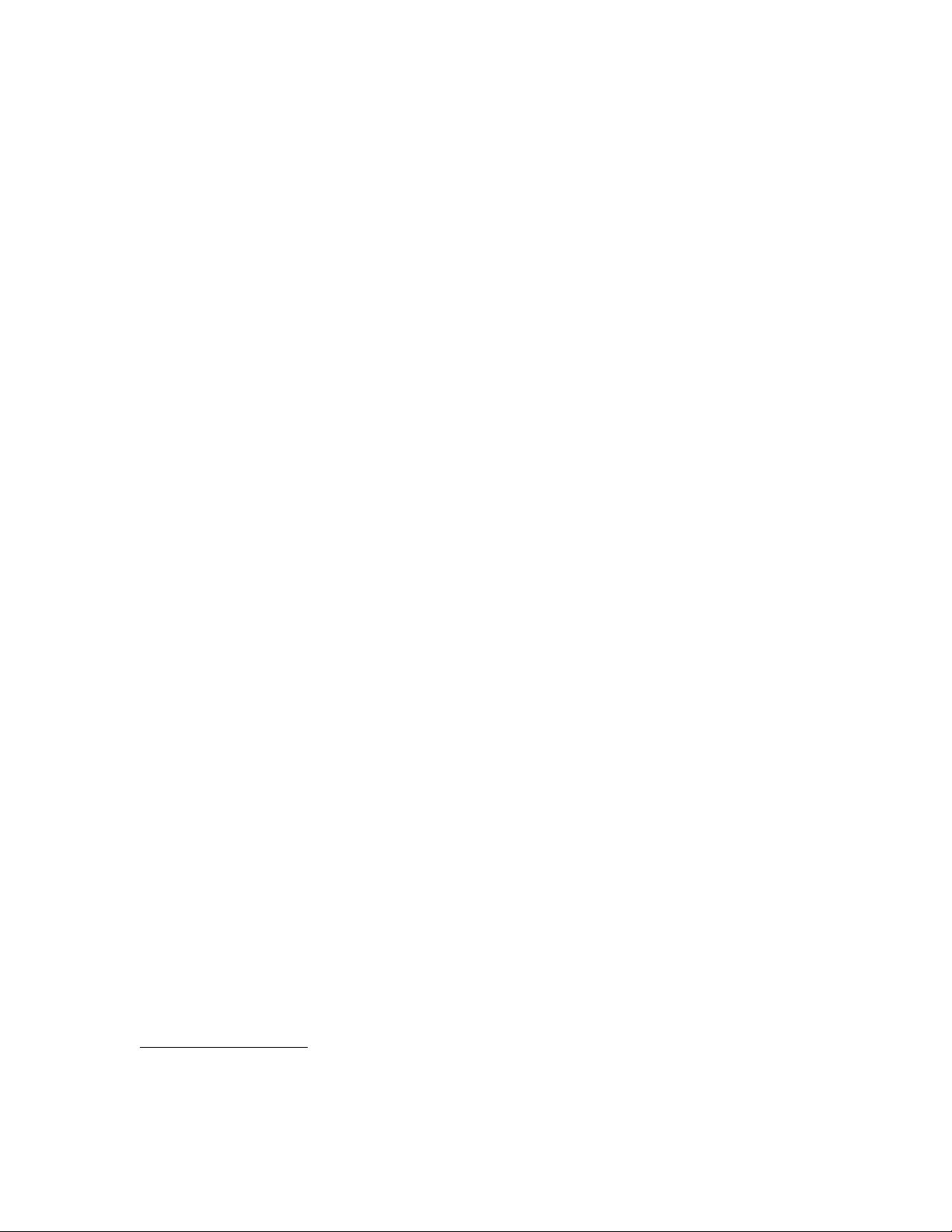
Prepared exclusively for Kwonjin Jeong
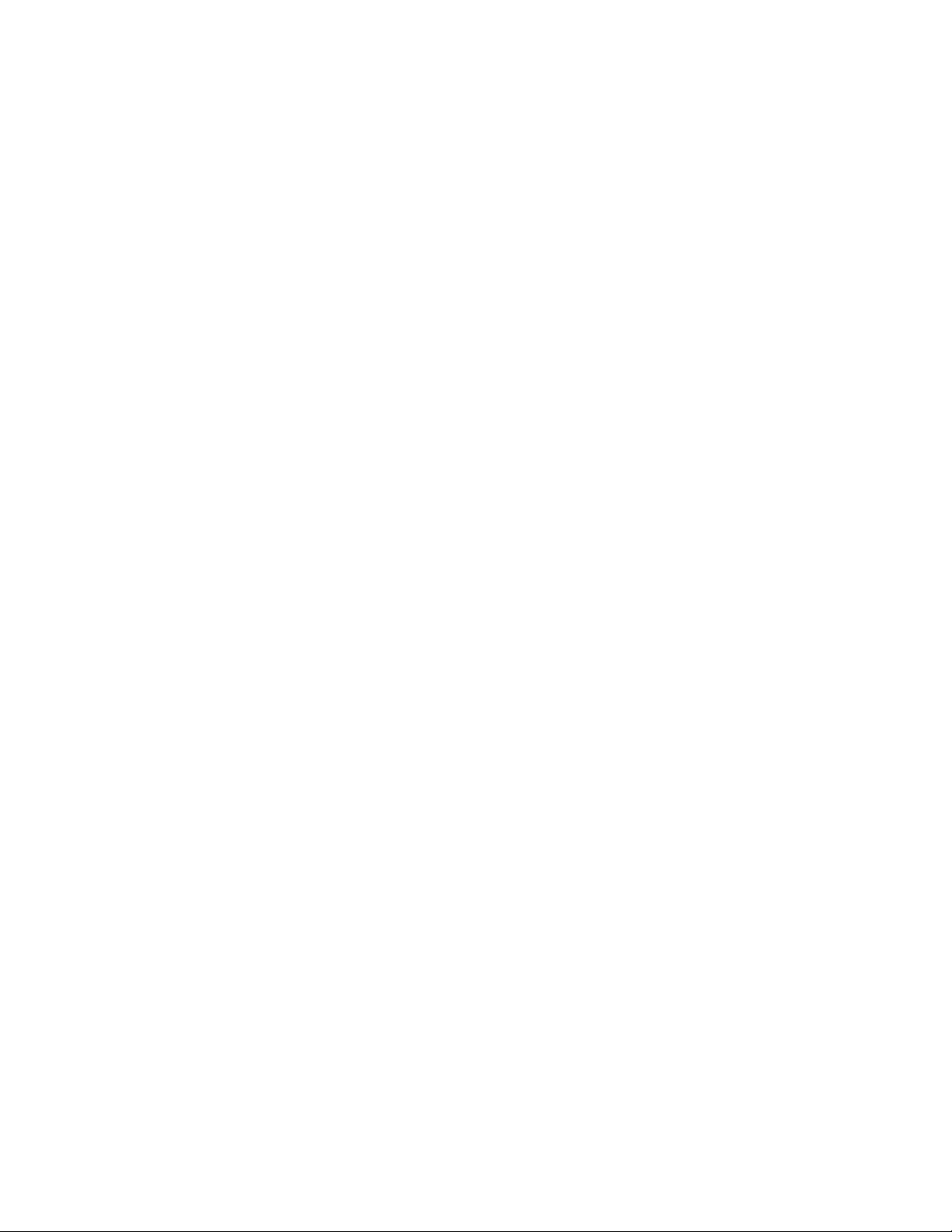
The world is parallel.
If we want to write programs that behave as other objects behave in
the real world, then these programs will have a concurrent str ucture.
Use a language that was designed for writing concurrent applications,
and development becomes a lot easier.
Erlang programs model how we think and interact.
Joe Armstrong
Prepared exclusively for Kwonjin Jeong
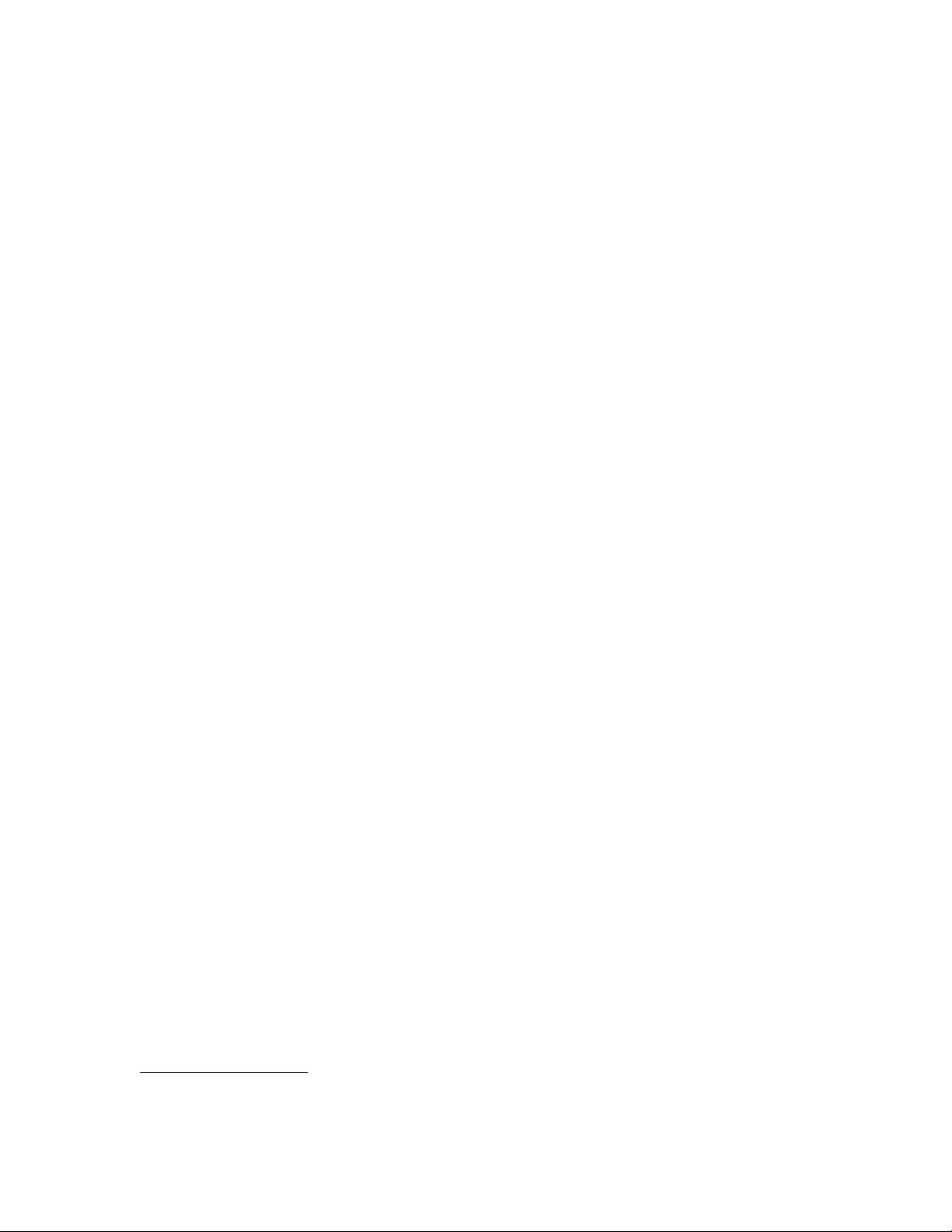
Programming Erlan g
Software for a Concurrent World
Joe Armstrong
The Pragmatic Bookshelf
Raleigh, North Carolina Dallas, Texas
Prepared exclusively for Kwonjin Jeong
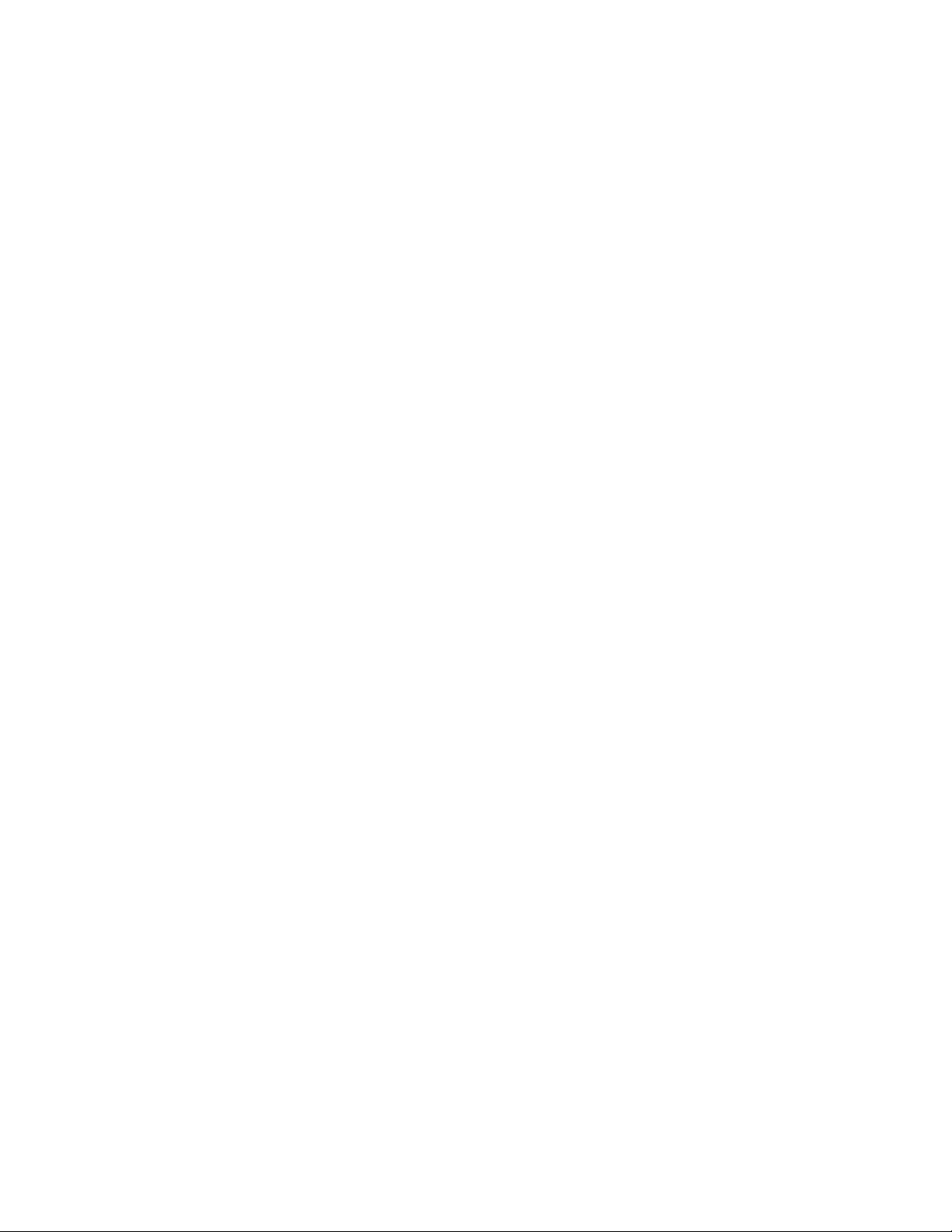
Many of the designations used by manufacturers and sellers to distinguish their prod-
ucts are c l aimed as trademarks. Wh ere those designations appear in this book, and The
Pragmatic Pr ogrammers, LLC was aware of a trademark claim, the designations have
been printed in initial capital letters or i n all capitals. The Pragmatic Starter Kit, The
Pragmatic Programmer, Prag ma tic Programming, Pragmatic Bookshelf and the linking g
device are trademarks of The Pragmatic Programmers, LLC.
Every precaution was taken in the preparation of this book. However, the publisher
assumes no responsibility for errors or omissions, or for damages that may result from
the use of information (including program listings) contained herein.
Our Pragmatic courses, workshops, and othe r products can help you and your te am
create better software and h ave more fun. For more information, as well as the latest
Pragmatic titles, please visit us at
http://www.pragmaticprogrammer.com
Copyright
©
2007 armstrongonsoftware.
All rights reserved.
No part of this publication may be reproduced, stored in a retrieval system, or tran smit-
ted, in any form, or by any means, elec tronic, mechanical, photocopying, recording, or
otherwise, without the prior consent of the publisher.
Printed in the United States of America.
ISBN-10: 1-9343560-0-X
ISBN-13: 978-1-934356-00-5
Printed on acid-free paper with 50% recycled, 15% post-consumer content.
P1.1 printing, July, 2007
Version: 2007-7-17
Prepared exclusively for Kwonjin Jeong
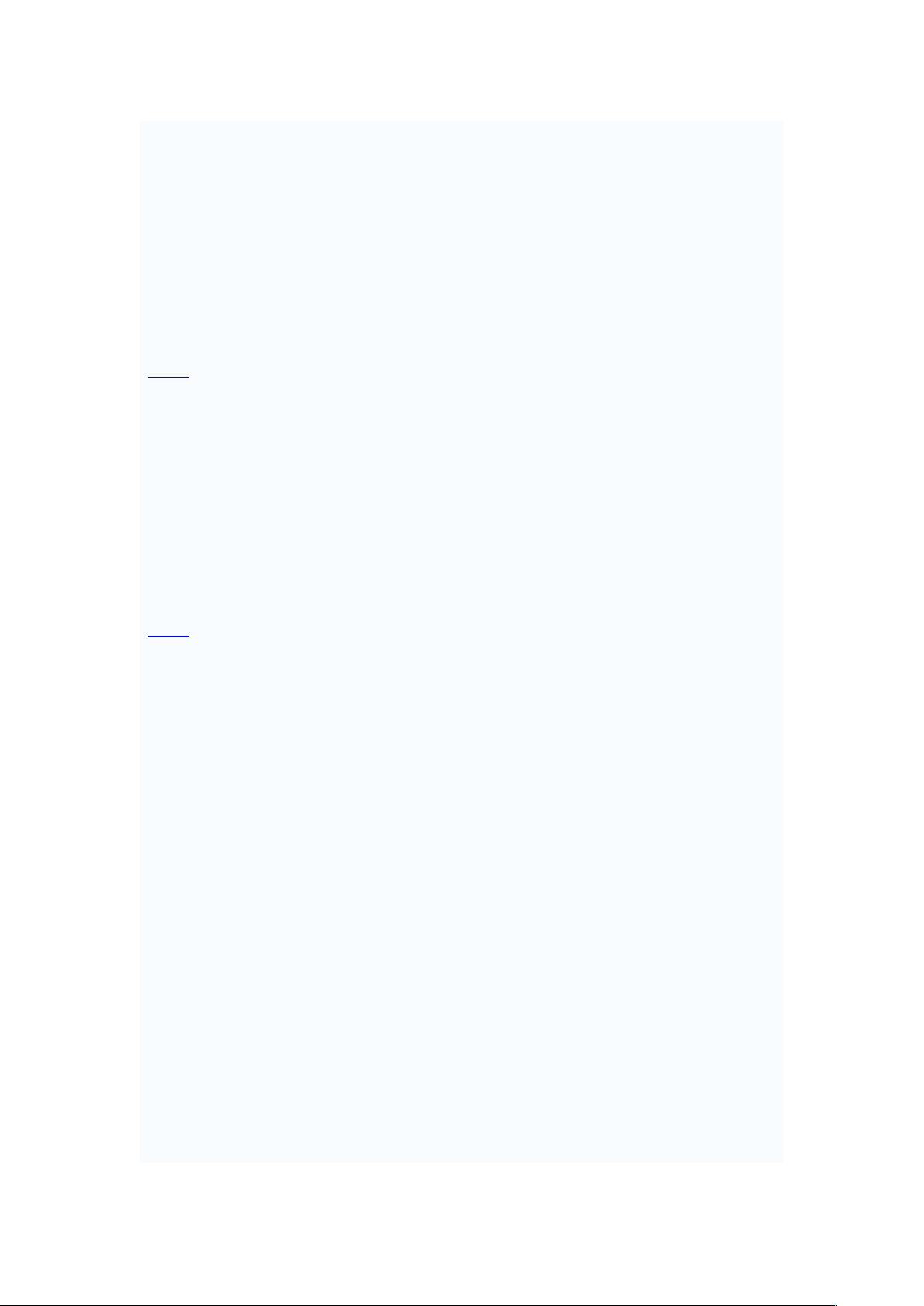
Contents
1 Begin 12
1.1 Road Map . . . . . . . . . . . . . . . . . . . . . . . . . . 13
1.2 Begin Again . . . . . . . . . . . . . . . . . . . . . . . . . 16
1.3 Acknowledgments . . . . . . . . . . . . . . . . . . . . . . 17
2 Getting Started 18
2.1 Overview . . . . . . . . . . . . . . . . . . . . . . . . . . . 18
2.2 Installing Erlang . . . . . . . . . . . . . . . . . . . . . . 21
2.3 The Code in This Book . . . . . . . . . . . . . . . . . . . 23
2.4 Starting the Shell . . . . . . . . . . . . . . . . . . . . . . 24
2.5 Simple Integer Arithmetic . . . . . . . . . . . . . . . . . 25
2.6 Variables . . . . . . . . . . . . . . . . . . . . . . . . . . . 27
2.7 Floating-Point Numbers . . . . . . . . . . . . . . . . . . 32
2.8 Atoms . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 33
2.9 Tuples . . . . . . . . . . . . . . . . . . . . . . . . . . . . 35
2.10 Lists . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 38
2.11 Strings . . . . . . . . . . . . . . . . . . . . . . . . . . . . 40
2.12 Pattern Matching Again . . . . . . . . . . . . . . . . . . 41
3 Sequential Programming 43
3.1 Modules . . . . . . . . . . . . . . . . . . . . . . . . . . . 43
3.2 Back to Shopping . . . . . . . . . . . . . . . . . . . . . . 49
3.3 Functions with the Same Name and Different Arity . . 52
3.4 Funs . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 52
3.5 Simple List Processing . . . . . . . . . . . . . . . . . . . 58
3.6 List Comprehensions . . . . . . . . . . . . . . . . . . . . 61
3.7 Arithmetic Expressions . . . . . . . . . . . . . . . . . . 64
3.8 Guards . . . . . . . . . . . . . . . . . . . . . . . . . . . . 65
3.9 Records . . . . . . . . . . . . . . . . . . . . . . . . . . . . 69
3.10 case and if Expressions . . . . . . . . . . . . . . . . . . 72
3.11 Building Lists in Natural Order . . . . . . . . . . . . . . 73
3.12 Accumulators . . . . . . . . . . . . . . . . . . . . . . . . 74
Prepared exclusively for Kwonjin Jeong