自定义属性控件
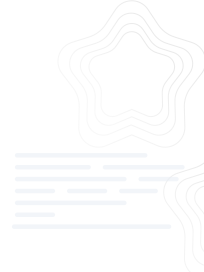


在Android开发中,自定义属性控件是一种常见的需求,它允许开发者根据项目需求创建具有独特功能和样式的UI组件。本篇文章将深入探讨如何创建一个自定义属性控件,以`CustomLinearLayout`为例,该控件能实现自动换行的功能。 我们需要创建一个新的Java类,继承自Android内置的布局控件,如LinearLayout。在这个例子中,我们将继承LinearLayout,并命名为`CustomLinearLayout`。在`CustomLinearLayout.java`文件中,我们首先进行必要的初始化操作: ```java public class CustomLinearLayout extends LinearLayout { public CustomLinearLayout(Context context) { super(context); init(context, null, 0); } public CustomLinearLayout(Context context, AttributeSet attrs) { super(context, attrs); init(context, attrs, 0); } public CustomLinearLayout(Context context, AttributeSet attrs, int defStyleAttr) { super(context, attrs, defStyleAttr); init(context, attrs, defStyleAttr); } private void init(Context context, AttributeSet attrs, int defStyleAttr) { // 在这里可以进行一些初始化操作,比如设置默认样式等 } } ``` 接下来,我们要为自定义控件添加自定义属性。这些属性可以在`res/values/attrs.xml`文件中定义。例如,我们可以定义一个属性`autoWrap`来控制是否开启自动换行功能: ```xml <resources> <declare-styleable name="CustomLinearLayout"> <attr name="autoWrap" format="boolean" /> </declare-styleable> </resources> ``` 现在,我们需要在`CustomLinearLayout`的构造函数中解析这些自定义属性。通过`obtainStyledAttributes()`方法获取到`AttributeSet`,然后调用`getAttributeBooleanValue()`读取`autoWrap`属性的值: ```java private void init(Context context, AttributeSet attrs, int defStyleAttr) { TypedArray a = context.obtainStyledAttributes(attrs, R.styleable.CustomLinearLayout, defStyleAttr, 0); boolean autoWrap = a.getBoolean(R.styleable.CustomLinearLayout_autoWrap, false); // 默认值为false a.recycle(); // 根据autoWrap的值来决定是否开启自动换行功能 // ... } ``` 有了这个属性,我们就可以在XML布局文件中使用`CustomLinearLayout`并设置`autoWrap`属性: ```xml <com.example.myapp.CustomButtonLinearLayout android:layout_width="match_parent" android:layout_height="wrap_content" app:autoWrap="true"> <!-- 子视图在这里 --> </com.example.myapp.CustomButtonLinearLayout> ``` 为了实现自动换行的功能,我们需要重写`onMeasure()`方法。在`onMeasure()`中,我们可以遍历子视图,根据它们的宽度和当前行的总宽度来判断是否需要换行。这需要对测量过程有深入的理解,因为Android的布局系统是基于测量和布局两个步骤的。 ```java @Override protected void onMeasure(int widthMeasureSpec, int heightMeasureSpec) { super.onMeasure(widthMeasureSpec, heightMeasureSpec); int lineWidth = 0; int lineCount = 0; int childCount = getChildCount(); int widthSize = MeasureSpec.getSize(widthMeasureSpec); for (int i = 0; i < childCount; i++) { View child = getChildAt(i); measureChild(child, widthMeasureSpec, heightMeasureSpec); // 获取子视图的测量宽度 int childWidth = child.getMeasuredWidth(); // 如果加上当前子视图后,宽度超过了父视图宽度或已达到自动换行条件 if (lineWidth + childWidth > widthSize || autoWrap && lineWidth > 0) { lineWidth = childWidth; // 重新计算行宽 lineCount++; // 行数加一 } else { lineWidth += childWidth; // 继续累加子视图宽度 } } // 计算最终高度 int heightSize = getPaddingTop() + getPaddingBottom() + (lineCount * (getChildrenRectHeight() + getVerticalSpacing())) - getVerticalSpacing(); setMeasuredDimension(widthSize, resolveSize(heightSize, heightMeasureSpec)); } private int getChildrenRectHeight() { // 返回子视图的矩形高度,可以根据实际情况调整 return getMeasuredHeight(); } private int getVerticalSpacing() { // 返回子视图之间的垂直间距,可以根据实际情况调整 return dpToPx(getContext(), 5); // 假设我们有一个dpToPx方法用于dp转换成px } ``` 别忘了处理边界情况,例如当没有子视图或者所有子视图都在同一行内时。此外,可能还需要考虑水平和垂直居中对齐等其他布局选项。 以上就是创建一个自定义属性控件`CustomLinearLayout`的全过程,它具有自动换行功能。通过这种方式,开发者可以灵活地扩展Android的内置控件,满足项目的个性化需求。在实际开发中,还可以根据需要添加更多的自定义属性,以实现更复杂的逻辑和布局效果。
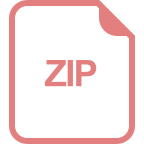
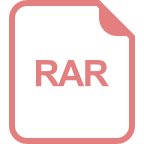
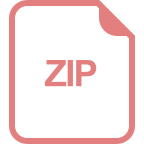
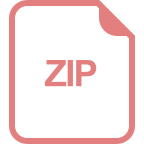
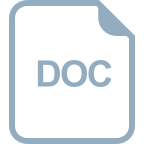
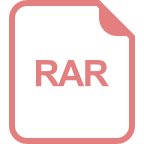
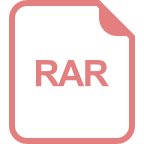
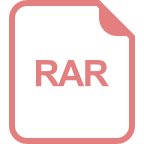
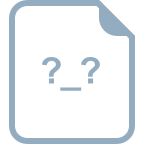
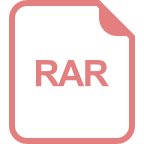
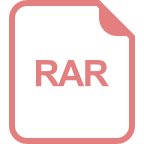
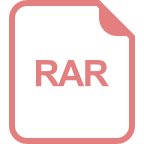
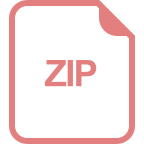
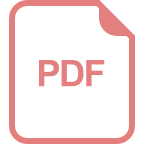
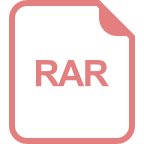
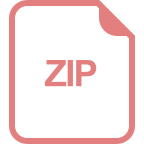
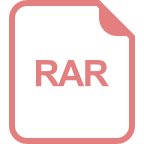
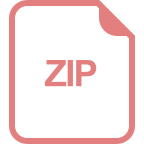
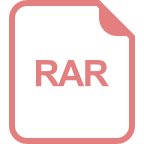
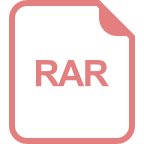
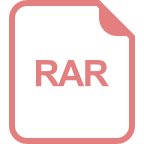
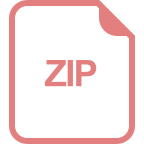
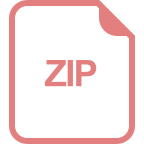
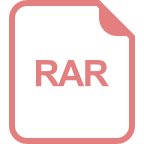
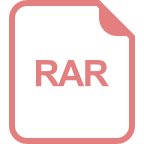
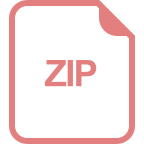
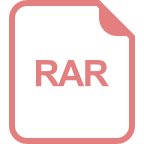


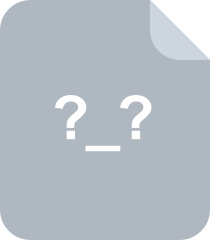

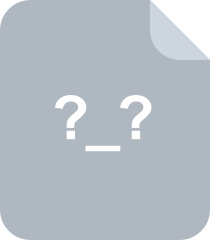
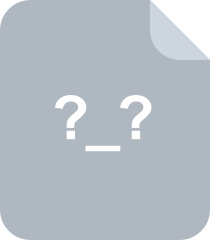
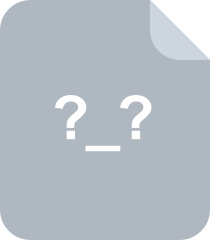

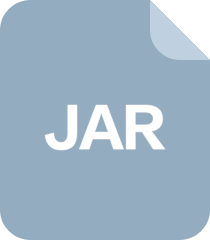



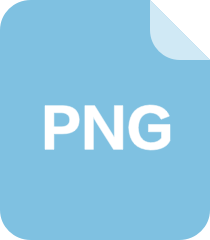

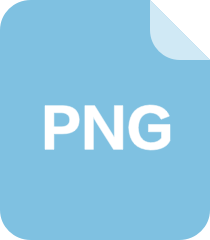

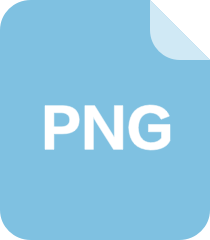

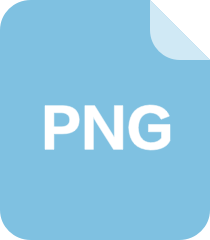
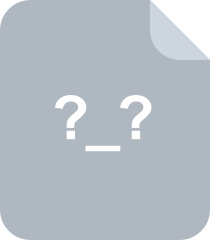




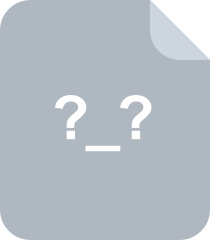
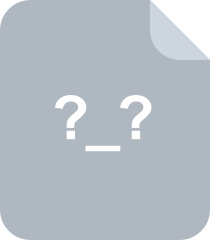
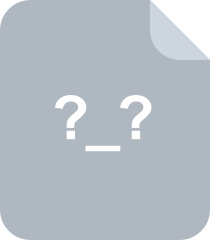
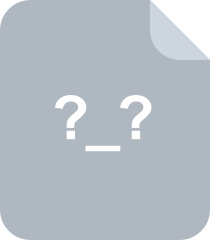
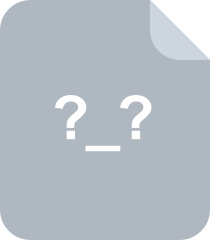
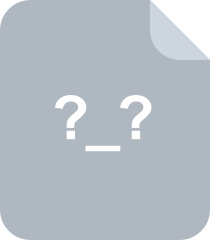
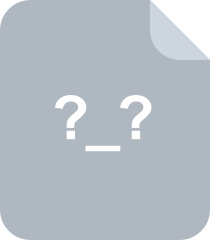
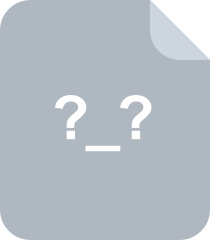
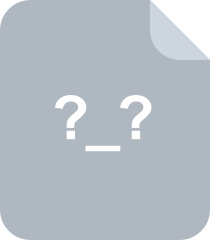
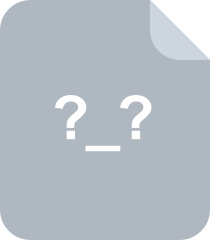
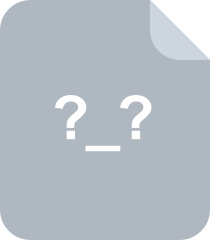
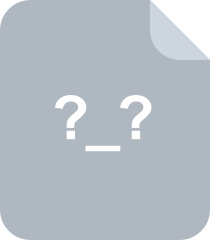
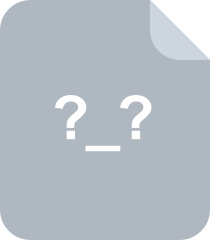
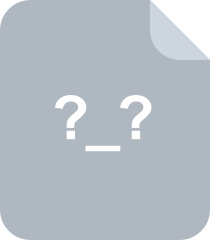
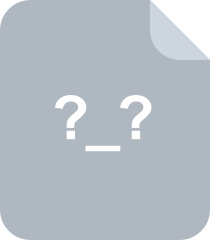
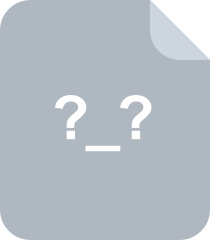
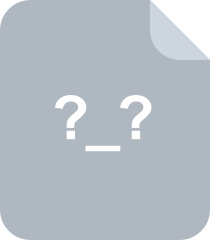
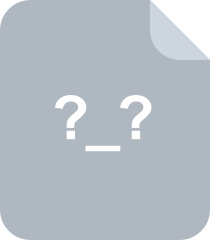
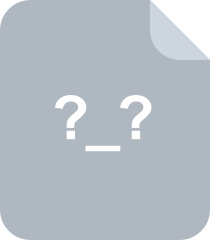
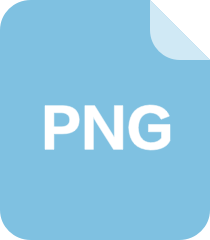
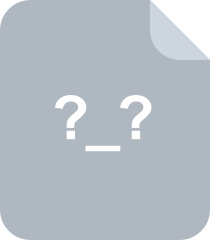
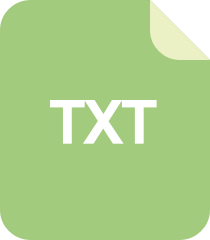

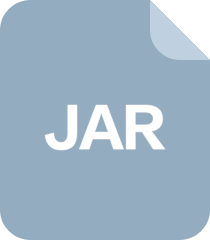




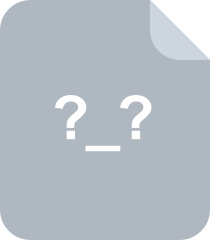
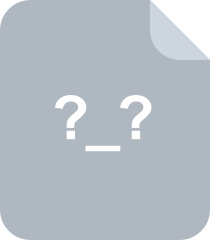
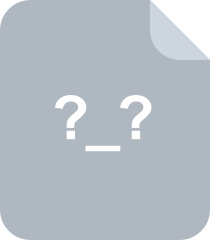
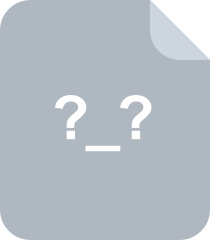
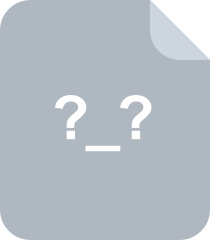


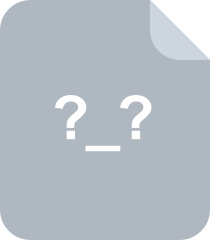
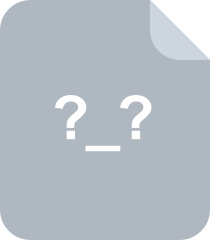
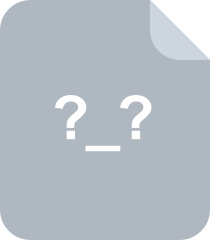
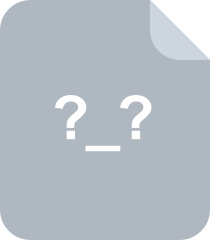

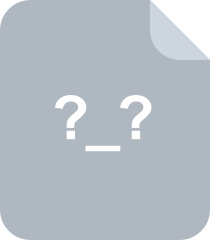

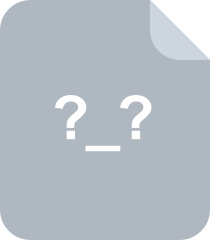
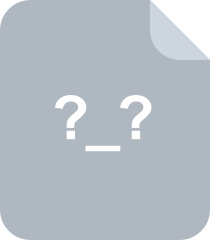


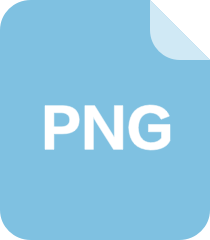

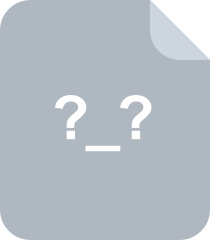

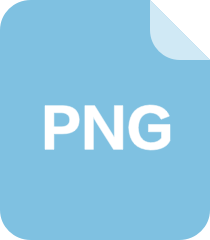

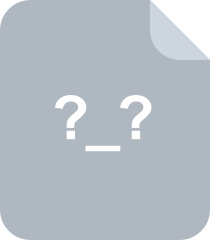

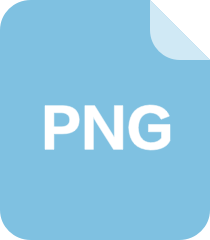

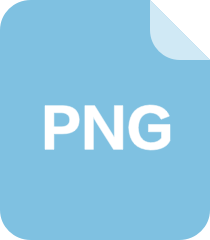

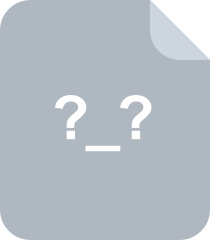

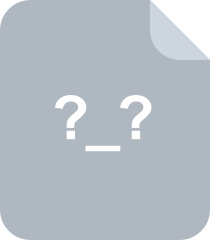
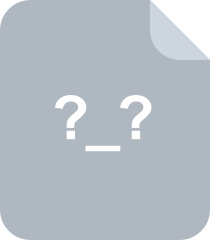




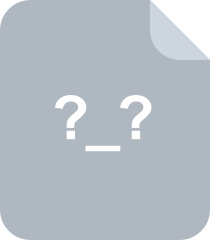
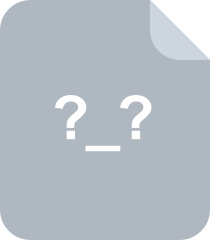

- 1

- 粉丝: 98
- 资源: 12
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

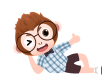
最新资源

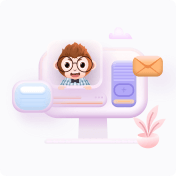

- 1
- 2
- 3
前往页