package y2javaee.sg.util;
import java.util.*;
import java.sql.*;
import javax.servlet.jsp.jstl.sql.*;
/*
* 通用的JDBC数据库访问类
* Y2JavaEE的ch06的工具类
*/
public class SQLCommandBean {
private Connection conn;
private String sqlValue;
private List values;
/**
* 设定连接类
*/
public void setConnection(Connection conn) {
this.conn = conn;
}
/**
* 设定SQL语句.
*/
public void setSqlValue(String sqlValue) {
this.sqlValue = sqlValue;
}
/**
* 设定SQL语句的参数
*/
public void setValues(List values) {
this.values = values;
}
/**
* 执行查询
*
* @return a javax.servlet.jsp.jstl.sql.Result object
* @exception SQLException
*/
public Result executeQuery() throws SQLException {
Result result = null;
ResultSet rs = null;
PreparedStatement pstmt = null;
Statement stmt = null;
try {
if (values != null && values.size() > 0) {
// Use a PreparedStatement and set all values
pstmt = conn.prepareStatement(sqlValue);
setValues(pstmt, values);
rs = pstmt.executeQuery();
}
else {
// Use a regular Statement
stmt = conn.createStatement();
rs = stmt.executeQuery(sqlValue);
}
result = ResultSupport.toResult(rs);
}finally {
if (rs != null) {
try {rs.close();} catch (SQLException e) {}
}
if (stmt != null) {
try {stmt.close();} catch (SQLException e) {}
}
if (pstmt != null) {
try {pstmt.close();} catch (SQLException e) {}
}
}
return result;
}
/**
* 执行Update语句
*
* @return the number of rows affected
* @exception SQLException
*/
public int executeUpdate() throws SQLException {
int noOfRows = 0;
ResultSet rs = null;
PreparedStatement pstmt = null;
Statement stmt = null;
try {
if (values != null && values.size() > 0) {
// Use a PreparedStatement and set all values
pstmt = conn.prepareStatement(sqlValue);
setValues(pstmt, values);
noOfRows = pstmt.executeUpdate();
}
else {
// Use a regular Statement
stmt = conn.createStatement();
noOfRows = stmt.executeUpdate(sqlValue);
}
}
finally {
if (rs != null) {
try {rs.close();} catch (SQLException e) {}
}
if (stmt != null) {
try {stmt.close();} catch (SQLException e) {}
}
if (pstmt != null) {
try {pstmt.close();} catch (SQLException e) {}
}
}
return noOfRows;
}
/**
* 设定语句的参数.
*
* @param pstmt the PreparedStatement
* @param values a List with objects
* @exception SQLException
*/
private void setValues(PreparedStatement pstmt, List values)
throws SQLException {
for (int i = 0; i < values.size(); i++) {
Object v = values.get(i);
// Set the value using the method corresponding to the type.
// Note! Set methods are indexed from 1, so we add 1 to i
pstmt.setObject(i + 1, v);
}
}
}
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
accp5.0\教学电子课件\Y2\开发基于JSP Servlet JavaBean的网上交易系统(JSP Servlet JavaBean Web Service)\ACCP5.0 开发基于JSP Servlet JavaBean的网上交易系统理论课贯穿案例.rar
资源推荐
资源详情
资源评论
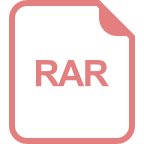
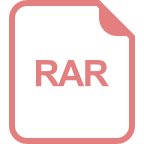
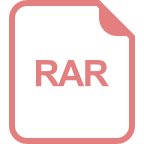
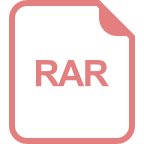
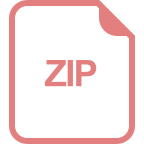
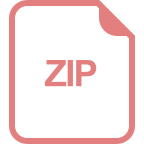
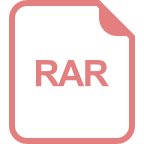
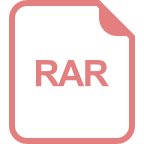
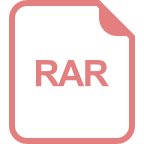
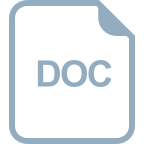
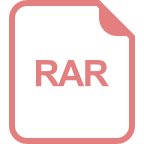
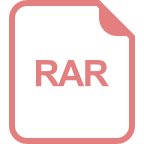
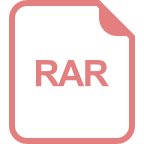
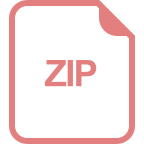
收起资源包目录

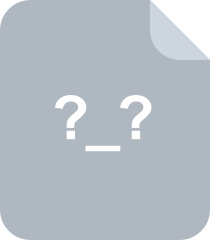
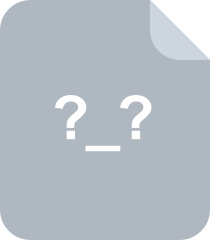
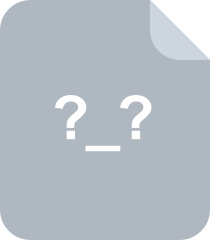
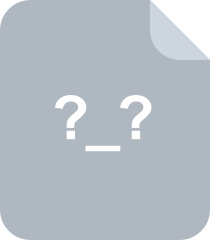
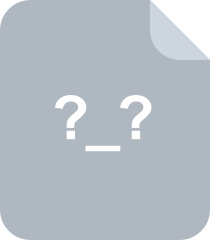
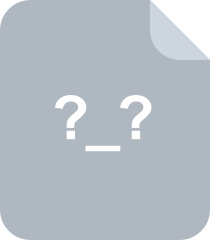
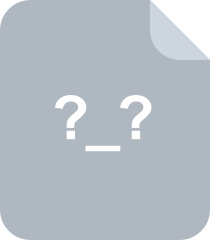
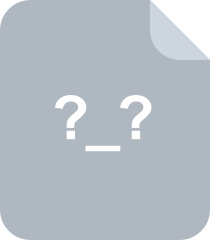
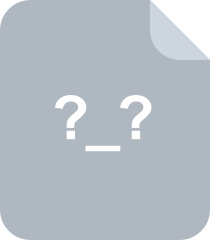
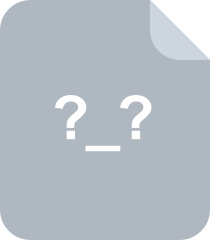
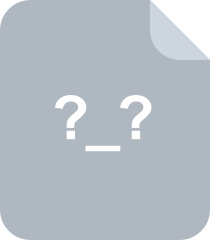
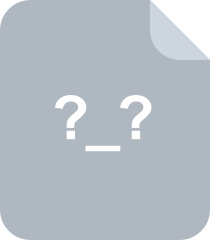
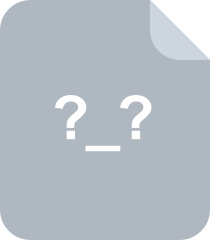
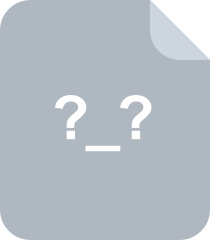
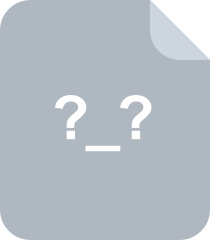
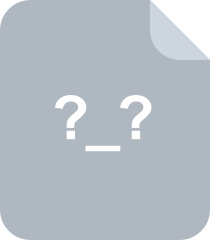
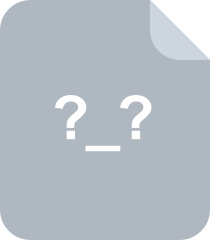
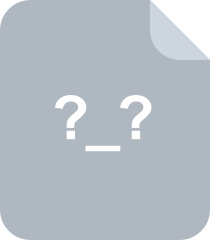
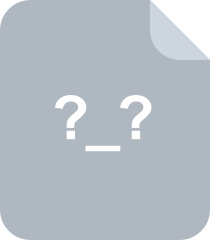
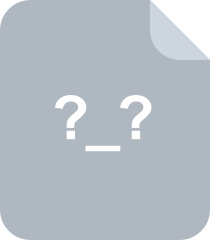
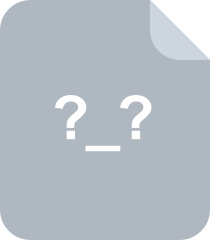
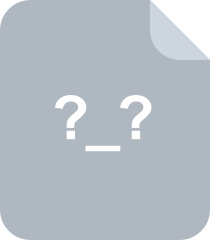
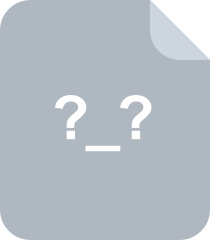
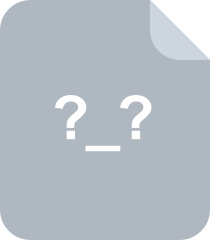
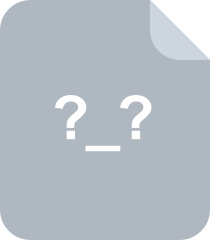
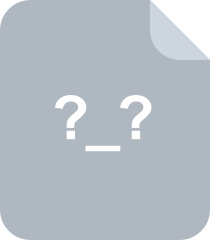
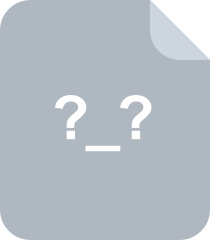
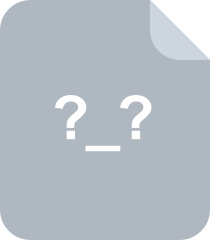
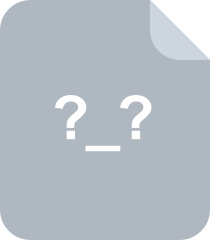
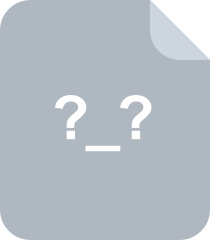
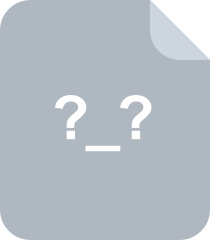
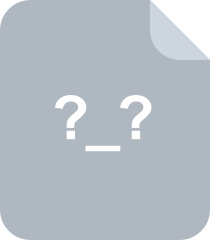
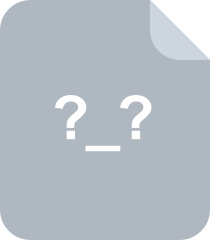
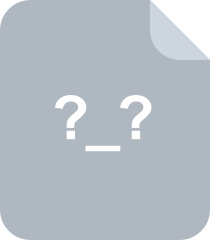
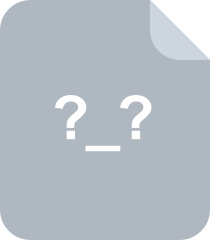
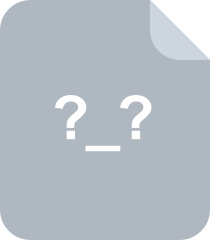
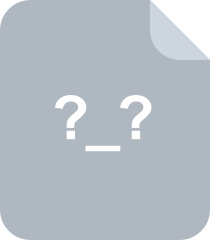
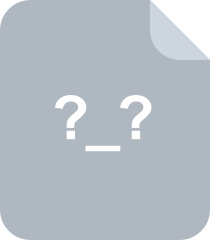
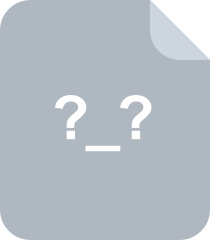
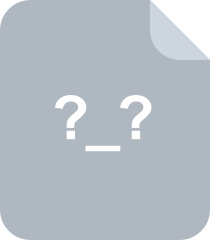
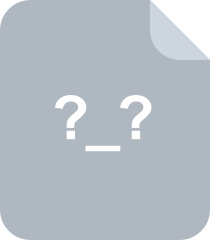
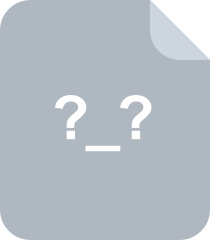
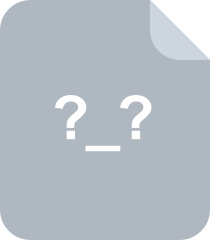
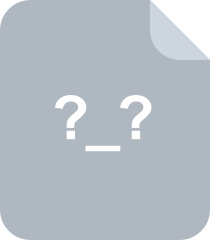
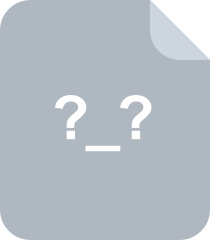
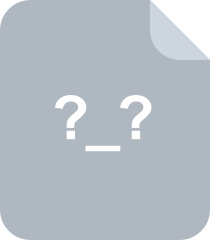
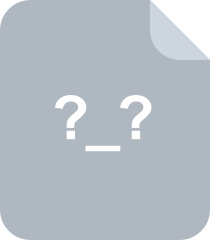
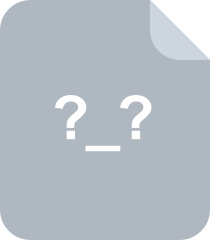
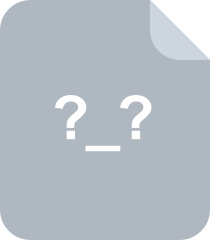
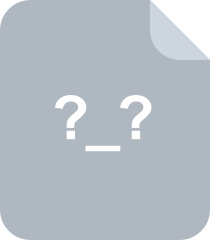
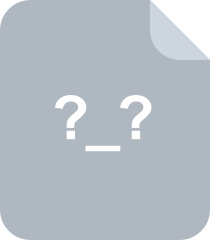
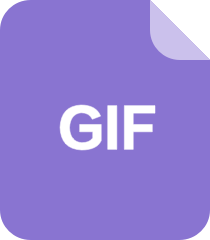
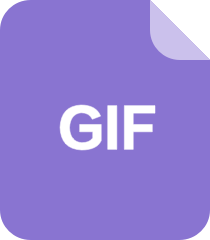
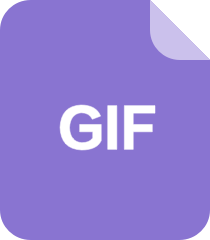
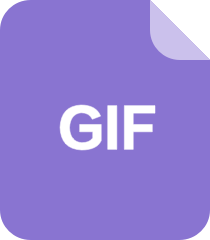
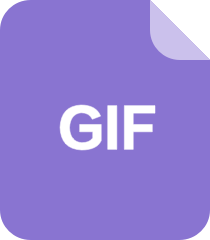
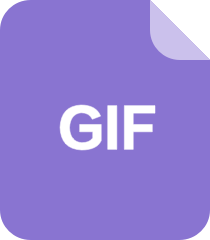
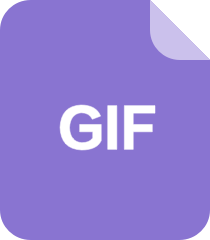
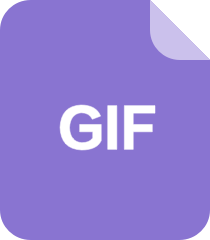
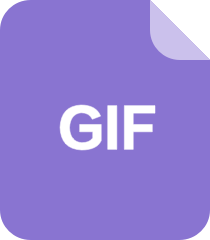
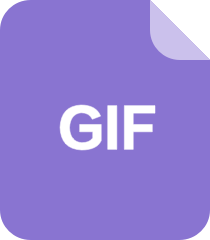
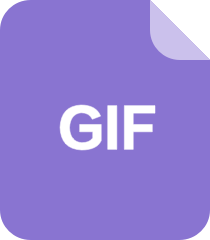
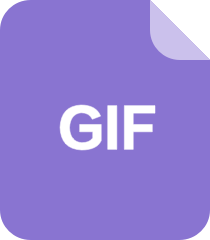
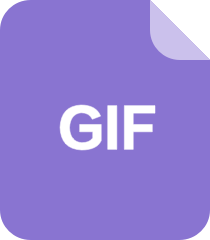
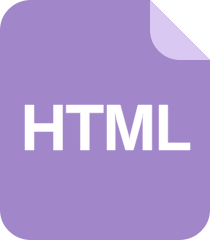
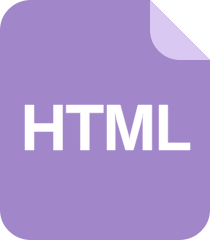
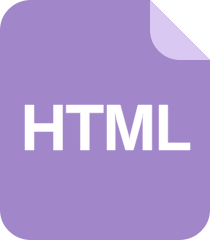
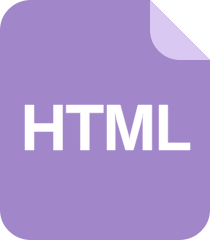
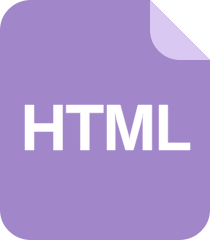
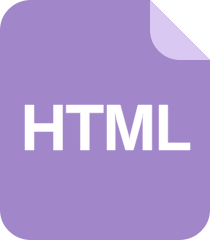
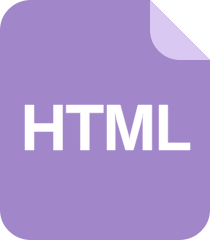
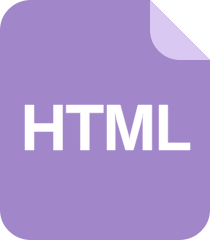
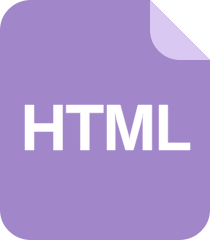
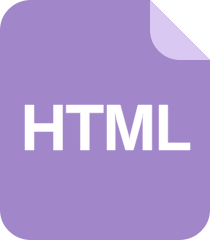
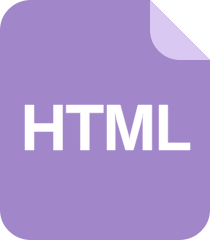
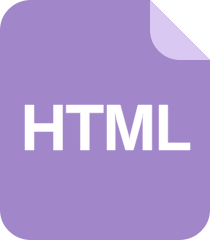
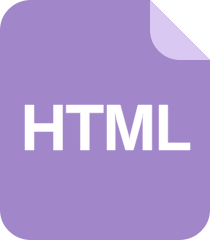
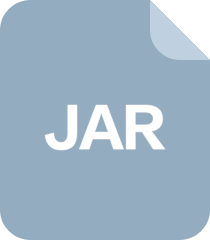
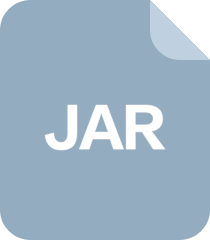
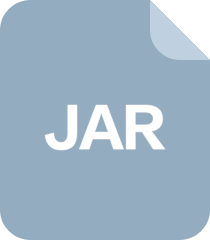
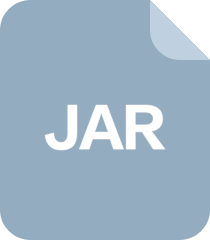
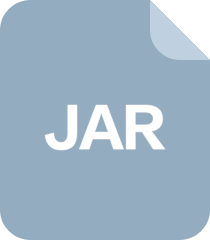
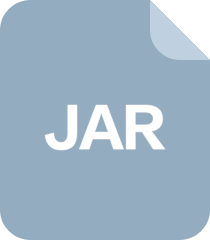
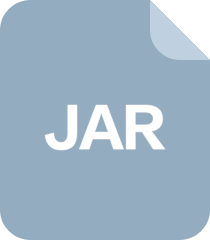
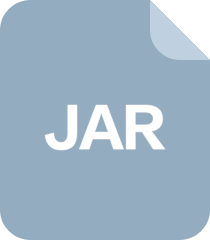
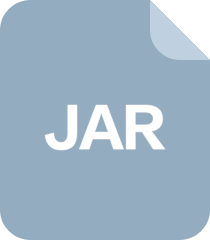
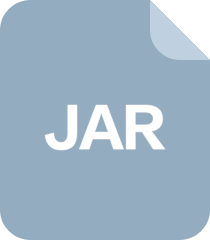
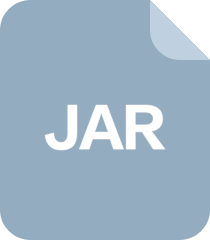
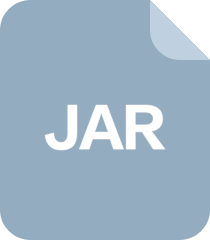
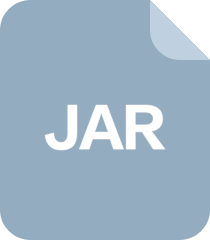
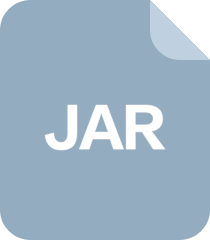
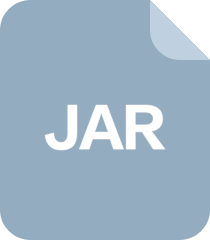
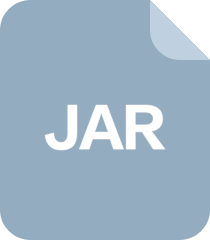
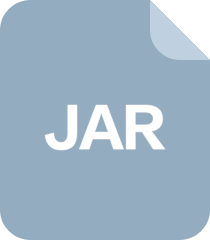
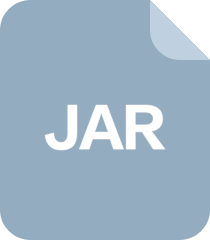
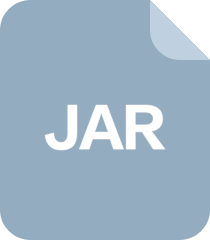
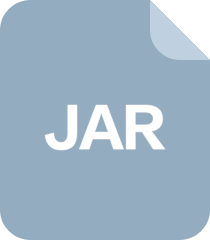
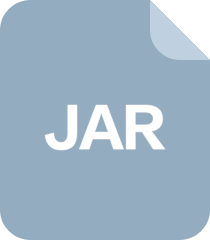
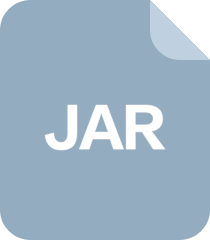
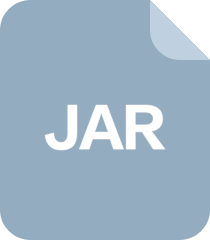
共 250 条
- 1
- 2
- 3
资源评论
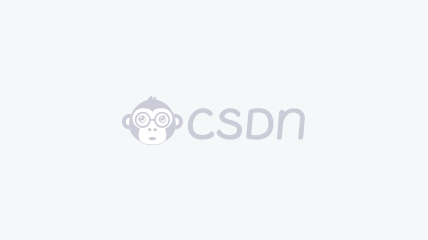
- ivan6454066892012-11-22项目贯穿案例不错,清晰地显示代码的详解。可以作为学习的辅助文件,熟练掌握Servlet的使用

xuyuan87
- 粉丝: 4
- 资源: 29
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

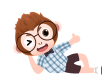
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


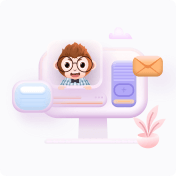
安全验证
文档复制为VIP权益,开通VIP直接复制
