
xujing_abc123
- 粉丝: 0
- 资源: 3
最新资源
- 基于java的图书管理系统+jsp源码(java毕业设计完整源码+LW).zip
- 扫描全能王 2024-12-31 00.01.pdf
- 基于java的物流管理系统设计与实现+jsp源码(java毕业设计完整源码+LW).zip
- 工业焊接机器sw18可编辑全套技术资料100%好用.zip
- 基于java的汽车养护管理系统+jsp源码(java毕业设计完整源码+LW).zip
- 12基于java的汽车租赁系统设计新版源码+数据库+说明
- 工件定位小型流水线sw18全套技术资料100%好用.zip
- 基于Java的菜匣子优选系统设计与实现+jsp源码(java毕业设计完整源码+LW).zip
- 滚刀式裁切机sw18可编辑+cad全套技术资料100%好用.zip
- 管状输送带sw18可编辑全套技术资料100%好用.zip
- 基于java的安徽新华学院实验中心管理系统的设计与实现+jsp源码(java毕业设计完整源码+LW).zip
- 海绵条贴附装置(sw18可编辑+工程图)全套技术资料100%好用.zip
- 基于java的在线云音乐系统的设计与实现+jsp源码(java毕业设计完整源码+LW).zip
- 红外线流水线烤箱方案(sw18可编辑+cad)全套技术资料100%好用.zip
- 基于java的龙腾公司员工信息管理系统的设计与实现+jsp源码(java毕业设计完整源码+LW).zip
- 回形传送线sw20可编辑全套技术资料100%好用.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


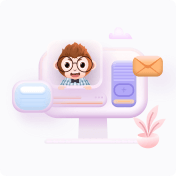