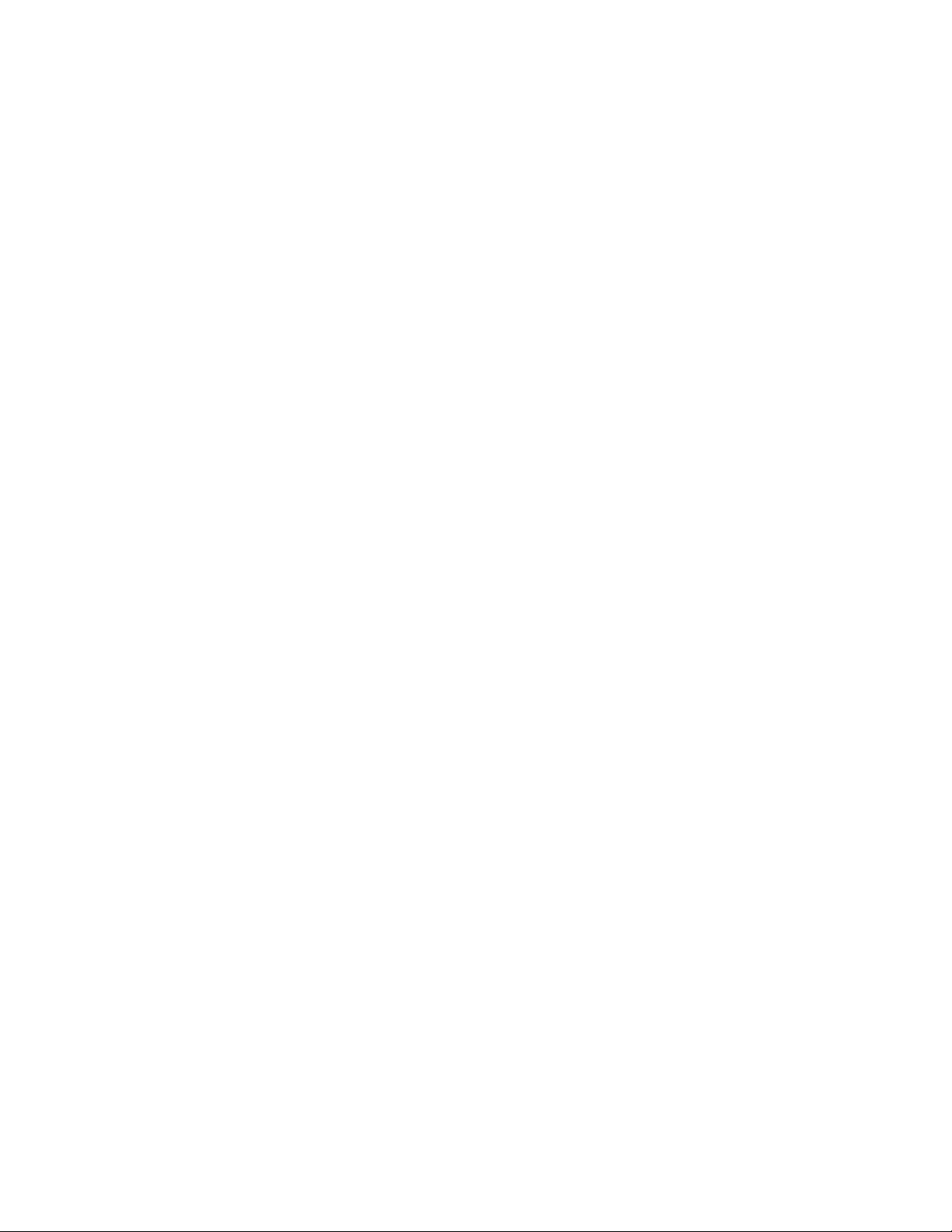
Contents
2
Contents
About the Java driver......................................................................................................4
Architecture...................................................................................................................... 5
The driver and its dependencies........................................................................................................ 5
Writing your first client...................................................................................................7
Connecting to a Cassandra cluster.....................................................................................................7
Using a session to execute CQL statements..................................................................................... 9
Using bound statements....................................................................................................................13
Java driver reference.....................................................................................................16
Four simple rules for coding with the driver..................................................................................... 16
Asynchronous I/O.............................................................................................................................. 17
Automatic failover.............................................................................................................................. 18
BATCH statements............................................................................................................................19
Cluster configuration..........................................................................................................................21
Tuning policies........................................................................................................................21
Connection options................................................................................................................. 22
Connection requirements...................................................................................................................24
CQL data types to Java types.......................................................................................................... 24
CQL statements.................................................................................................................................25
Building dynamic queries programmatically with the QueryBuilder API............................ 25
Debugging..........................................................................................................................................28
Exceptions...............................................................................................................................29
Monitoring............................................................................................................................... 29
Enabling tracing...................................................................................................................... 31
Enabling debug-level logging................................................................................................. 36
Node discovery..................................................................................................................................38
Object-mapping API.......................................................................................................................... 38
Basic CRUD operations..........................................................................................................38
Mapping UDTs........................................................................................................................41
Accessor-annotated interfaces............................................................................................... 43
Setting up your Java development environment...............................................................................44
Tuple types........................................................................................................................................44
User-defined types............................................................................................................................ 45
UDT API..................................................................................................................................45
Direct field manipulation......................................................................................................... 46
FAQ..................................................................................................................................48
Can I check if a conditional statement (lightweight transaction) was successful?............................ 48
What is a parameterized statement and how can I use it?.............................................................. 48
Does a parameterized statement escape parameters?....................................................................48
What's the difference between a parameterized statement and a Prepared statement?..................48
Can I combine Prepared statements and normal statements in a batch?........................................49
Can I get the raw bytes of a text column?....................................................................................... 49
How to increment counters with QueryBuilder?................................................................................49