/*
* XExtends Core v1.0.1
*
* Free open source
* Author: xu_liangzhan@163.com
*/
(function(){
'use strict';
var
str_num = 'number',
str_undefined = 'undefined',
symbol_iterator = 'iterator',
global = typeof window === str_undefined ? this : window,
__xe = global.XExtends = {version : "1.0.0"},
__symbolMap = {},
__symbolForList = [],
__incrementId = Math.round(Math.random()*100),
__decrementId = Math.round(Math.random()*10000);
function getSymbolIndex(key, type){
for(var index = 0, len = __symbolForList.length; index < len; index++){
if(key === __symbolForList[index][type]){
return index;
}
}
}
/*
* ES6 不可变的数据类型,用来产生唯一的标识,但是却无法直接访问这个标识
*/
function XESymbol(key){
var value;
if(this && this.constructor === XESymbol){
throw new TypeError('Symbol is not a constructor');
}
if(__symbolMap.hasOwnProperty(key)){
throw new TypeError('Cannot convert a Symbol value to a string');
}
return __symbolMap[value = 'XESymbol(' + key + ') ^' + (__decrementId--) + Date.now() + '' + (__incrementId++) + '' + Math.random()] = key, value;
}
XESymbol.iterator = XESymbol(symbol_iterator);
XESymbol['for'] = function(key){
var value, index = getSymbolIndex(key, 0);
if(index === undefined){
__symbolForList.push([key, value = XESymbol(key)]);
}else{
value = __symbolForList[index][1];
}
return value;
}
XESymbol.keyFor = function(key){
var index = getSymbolIndex(key, 1);
if(typeof index === str_num){
return __symbolForList[index][0];
}
if(!__symbolMap.hasOwnProperty(key)){
throw new TypeError(key + ' is not a symbol');
}
}
XESymbol.isSymbol = function(value){
return typeof getSymbolIndex(value, 1) === str_num || __symbolMap.hasOwnProperty(value);
}
if(!global.Symbol){
global.Symbol = XESymbol;
}
__xe.Symbol = XESymbol;
if(!global.Symbol.isSymbol){
global.Symbol.isSymbol = function(value){
return typeof value === "symbol";
}
}
})();
(function(){
'use strict';
var
str_obj = 'object',
str_fn = 'function',
str_str = 'string',
str_arr = 'array',
str_num = 'number',
str_bool = 'boolean',
str_undefined = 'undefined',
str_error_nullobject = 'Cannot convert undefined or null to object',
proto_string = String.prototype,
proto_array = Array.prototype,
iterator_index = Symbol('Iterator Index'),
iterator_list = Symbol('Iterator Value'),
global = typeof window === str_undefined ? this : window,
__xe = global.XExtends;
function findItem(list, callback, context, has){
for(var index = 0, len = list.length; index < len; index++){
if(callback.call(context || global, list[index], index, list)){
return {index : index, value : has ? true : list[index]};
};
}
return {index : -1, value : has ? false : has};
}
function getArrayIteratorResult(iterator, value, done){
return done = iterator[iterator_index]++ >= iterator[iterator_list].length, {done : done, value : done ? undefined : value};
}
function ArrayEntriesIterator(list){
this[iterator_index] = 0;
this[iterator_list] = list;
this.next = function(){
return getArrayIteratorResult(this, [this[iterator_index], this[iterator_list][this[iterator_index]]]);
}
}
function ArrayIndexIterator(list){
this[iterator_index] = 0;
this[iterator_list] = list;
this.next = function(){
return getArrayIteratorResult(this, this[iterator_index]);
}
}
function ArrayValueIterator(list){
this[iterator_index] = 0;
this[iterator_list] = list;
this.next = function(){
return getArrayIteratorResult(this, this[iterator_list][this[iterator_index]]);
}
}
function each(obj, callback, context){
for(var key in obj){
if(obj.hasOwnProperty(key)){
callback.call(context, obj[key], key, obj)
}
}
}
function getObjectIterators(obj, getIndex){
if(__xe.isObject(obj)){
var result = [];
for(var key in obj){
if(obj.hasOwnProperty(key)){
result.push([key, obj[key], [key, obj[key]]][getIndex]);
}
}
return result;
}
throw new TypeError(str_error_nullobject);
}
function toAssignValue(obj){
return __xe.isObject(obj) || __xe.isFunction(obj) ? obj : Array.from(obj);
}
each({Object : str_obj, Undefined : str_undefined, Boolean : str_bool, String : str_str, Number : str_num, Function : str_fn}, function(type, method){
__xe['is' + method] = function(obj){
return typeof obj === type;
};
});
each({isRegExp : RegExp, isPlainObject : Object, isArray : Array, isDate : Date, isError : Error, isTypeError : TypeError}, function(object, method){
__xe[method] = function(obj){
return obj && obj.constructor === object || false;
}
});
/*
* Object method
*/
each({
/*
* ES5 浅拷贝一个或者多个对象到目标对象中
*
* @param Object target 对象
* @param Object ...
* @return Object
*/
assign : function(target){
if(target === null || target === undefined){
throw new TypeError(str_error_nullobject);
}
target = toAssignValue(target);
for(var source, index = 1, len = arguments.length; index < len; index++){
source = toAssignValue(arguments[index]);
for(var key in source){
if(source.hasOwnProperty(key)){
target[key] = source[key];
}
}
}
return target;
},
/*
* ES6判断两个值是否是相同的值
*
* @param Object v1 值
* @param Object v2 值
* @return Boolean
*/
is : function(v1, v2){
if(v1 === v2){
return v1 !== 0 || 1/v1 === 1/v2;
}
return v1 !== v1 && v2 !== v2;
},
/*
* ES5 遍历对象的属性
*
* @param Object obj 对象
* @return Array
*/
keys : function(obj){
return getObjectIterators(obj, 0);
},
/*
* ES8遍历对象的属性值
*
* @param Object obj 对象
* @return Array
*/
values : function(obj){
return getObjectIterators(obj, 1);
},
/*
* ES8 遍历对象的属性名和属性值
*
* @param Object obj 对象
* @return Array
*/
entries : function(obj){
return getObjectIterators(obj, 2);
},
/*
* ES5 在一个对象上定义新的属性或修改现有属性
*
* @param Object obj 对象
* @param Object props 属性
* @return Object
*/
defineProperties : function(obj, props){
if(obj && (__xe.isObject(obj) || __xe.isFunction(obj))){
if(props === null || props === undefined){
throw new TypeError(str_error_nullobject);
}
for(var key in props){
if(props.hasOwnProperty(key)){
Object.defineProperty(obj, key, props[key]);
}
}
return obj;
}
throw new TypeError('Object.defineProperties called on non-object');
}
}, function(fn, name){
if(!Object[name]){
Object[name] = fn;
}
});
/*
* Function method
*/
each({
/*
* ES5 创建一个新的函数, 当被调用时,将其this关键字设置为提供的值
*
* @param Object context 上下文
* @return Function
*/
bind : function(context){
var fn = this;
return function(){
return fn.apply(context, arguments);
}
}
}, function(fn, name){
if(!Function.prototype[name]){
Function.prototype[name] = fn;
}
});
/*
* Date method
*/
each({
/*
* ES5 返回至今毫秒数
*
* @return Number
*/
now : function(){
return new Date().getTime();
}
}, function(fn, name){
if(!Date[name]){
Date[name] = fn;
}
});
/*
* Number method
*/
each({
/*
* ES6 检查一个数值是否非无穷
*
* @param Number val 数值
* @return Boolean
*/
isFinite : function(val){
r
没有合适的资源?快使用搜索试试~ 我知道了~
core.1.0.1.js
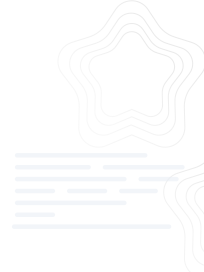
共2个文件
js:2个

需积分: 13 16 下载量 88 浏览量
2017-12-17
14:53:47
上传
评论
收藏 11KB RAR 举报
温馨提示
xExtends core.js prototype.* 兼容函数 ES5 ES6 ES7 Symbol 一个不可变的数据类型,用来产生唯一的标识,但是却无法直接访问这个标识 Promise 异步编程函数 Set 有序列表集合,它不会包含重复项 WeakSet 列表集合,和Set一样,只不过它的值只能是非空对象 Map 有序键值对集合,键值对的 key和 value都可以是任何类型的元素 WeakMap 键值对集合,和Map一样,只不过 它的 key只能是非空对象
资源推荐
资源详情
资源评论
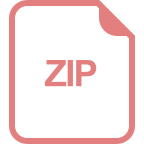
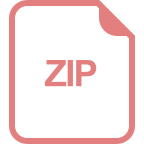
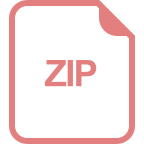
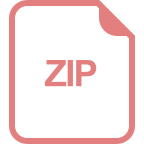
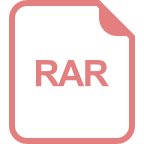
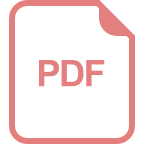
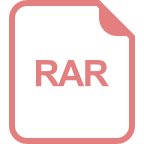
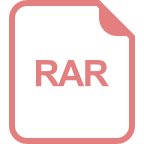
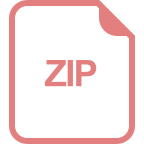
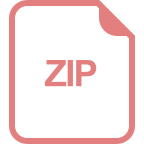
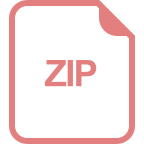
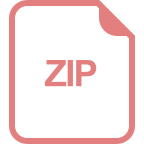
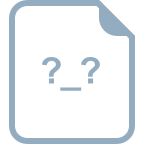
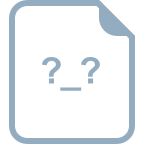
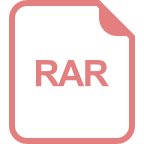
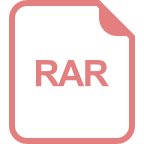
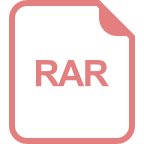
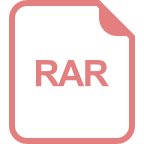
收起资源包目录



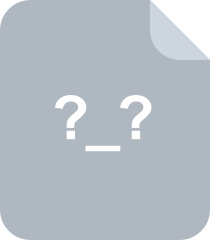
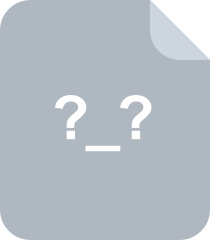
共 2 条
- 1
资源评论
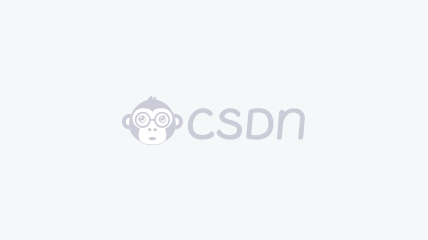

大猩猩X
- 粉丝: 289
- 资源: 8
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

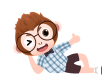
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


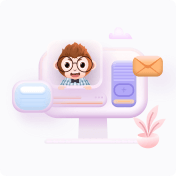
安全验证
文档复制为VIP权益,开通VIP直接复制
