在.NET开发中,获取不同地区的天气预报和外网IP地址是一项常见的需求,这通常涉及到网络通信和API调用。本文将详细讲解如何利用.NET技术来实现这一功能,主要围绕两个核心概念:天气预报的获取与外网IP地址的查询。 让我们关注天气预报的获取。在.NET中,我们可以借助各种开放的天气预报API,如OpenWeatherMap、AccuWeather等,这些服务提供者通常会提供RESTful API供开发者使用。以OpenWeatherMap为例,你需要注册并获取API密钥,然后使用HttpClient类发起HTTP请求到API接口,请求中包含目标城市的地理坐标或城市名。以下是一个简单的示例: ```csharp using System; using System.Net.Http; using System.Threading.Tasks; public async Task<WeatherData> GetWeatherAsync(string apiKey, string city) { using var httpClient = new HttpClient(); using var response = await httpClient.GetAsync($"http://api.openweathermap.org/data/2.5/weather?q={city}&appid={apiKey}&units=metric"); if (response.IsSuccessStatusCode) { var content = await response.Content.ReadAsStringAsync(); // 解析JSON数据,转换为WeatherData对象 return JsonConvert.DeserializeObject<WeatherData>(content); } throw new Exception("Failed to get weather data."); } ``` 在这个示例中,我们使用了`HttpClient`类发送GET请求,并处理返回的JSON数据。`WeatherData`是自定义的数据模型,用于存储从API获取的天气信息。 接下来,我们讨论如何获取外网IP地址。在.NET中,最简单的方法是向提供IP查询服务的网站发送HTTP请求,例如"ifconfig.me"或者"ipinfo.io"。以下是一个获取外网IP的例子: ```csharp using System; using System.Net.Http; using System.Threading.Tasks; public async Task<string> GetExternalIpAddressAsync() { using var httpClient = new HttpClient(); using var response = await httpClient.GetAsync("https://api.ipify.org"); if (response.IsSuccessStatusCode) { return await response.Content.ReadAsStringAsync(); } throw new Exception("Failed to get external IP address."); } ``` 这个示例中,我们向`api.ipify.org`发送请求,它会返回请求者的外网IP地址作为响应内容。 在实际项目中,你可能需要将这些功能封装成一个服务,例如WCF(Windows Communication Foundation)服务,以便其他应用程序或客户端能够调用。WCF是一种在.NET框架中创建分布式应用程序的服务模型,它可以跨平台、跨语言工作。创建WCF服务,你需要定义服务接口、实现服务操作、配置服务宿主,然后发布到IIS或其他服务器上。 以下是一个简单的WCF服务接口定义,用于提供天气预报和外网IP的服务: ```csharp using System.ServiceModel; [ServiceContract] public interface IWeatherAndIpService { [OperationContract] Task<WeatherData> GetWeatherAsync(string apiKey, string city); [OperationContract] Task<string> GetExternalIpAddressAsync(); } ``` 在实现WCF服务后,客户端可以使用WCF客户端代理类(通常由 svcutil 工具生成)来调用这些服务操作,获取所需信息。 总结,通过.NET,我们可以轻松地获取不同地区的天气预报和外网IP地址。这涉及到HTTP请求、JSON解析、WCF服务的创建和使用。了解并掌握这些技能,对于.NET开发者来说是非常有价值的。
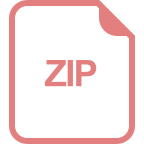
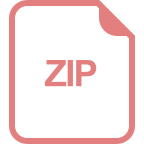
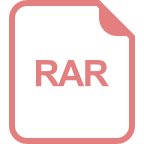
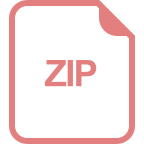
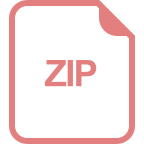
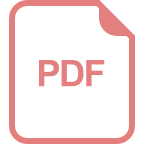
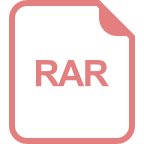
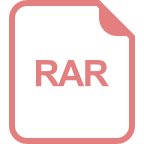
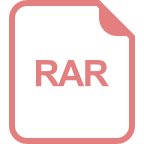
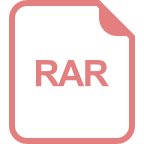
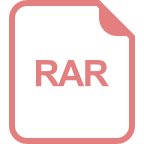
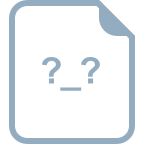
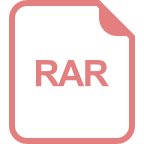
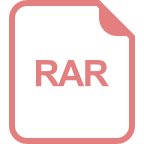
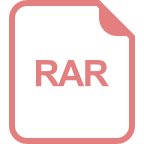
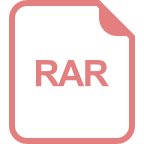
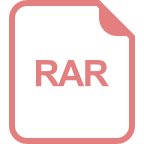
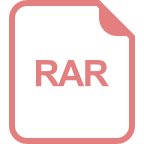
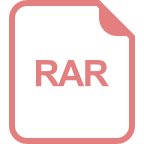



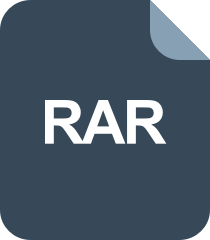
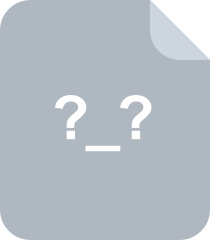
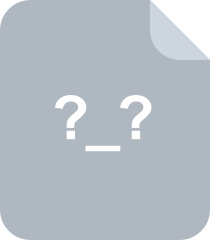
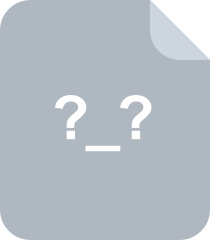
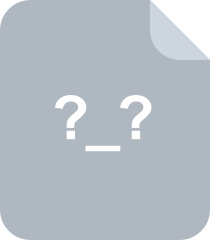



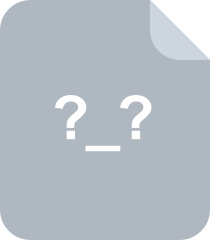

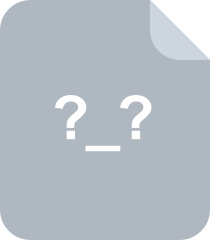

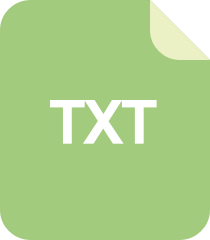

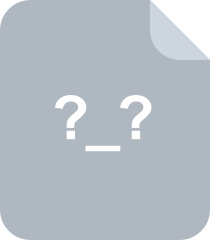
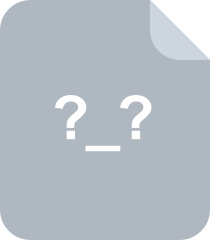
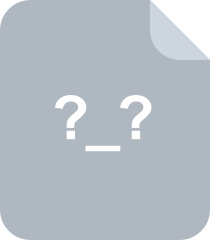
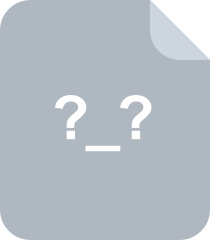
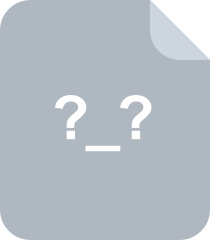
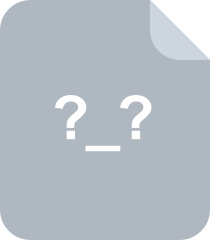
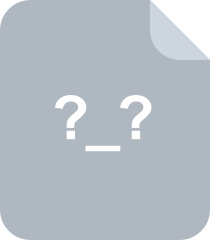
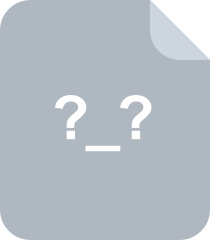
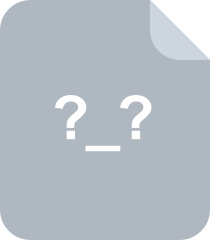
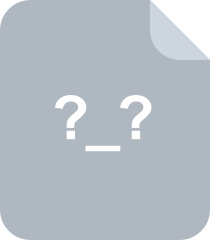
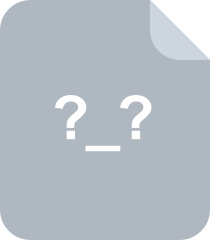
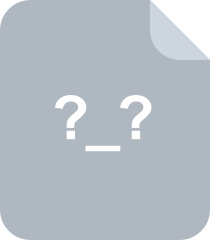
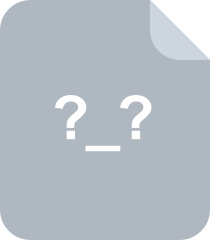
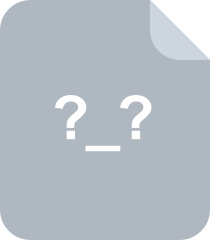
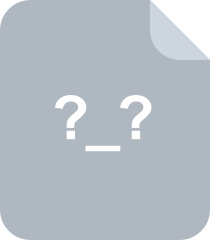
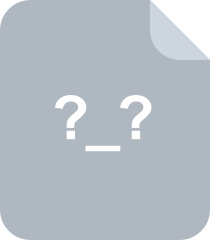
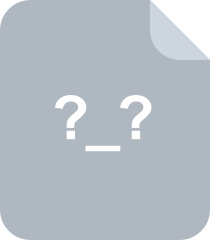
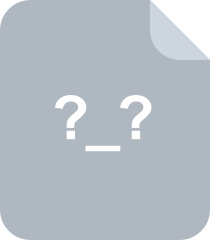
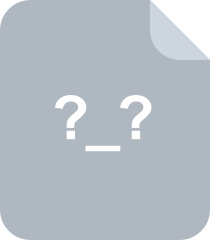
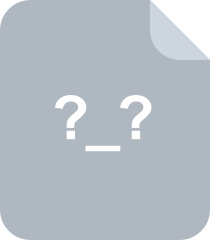
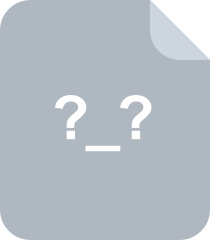
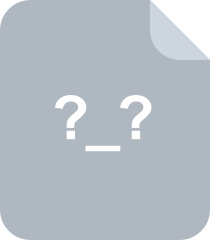
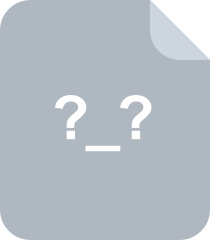

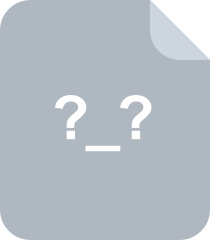
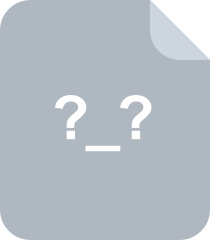
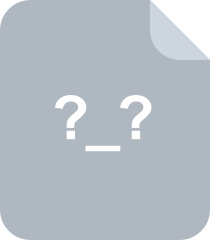
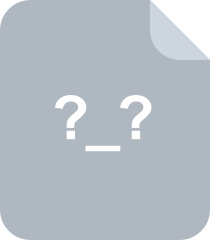
- 1
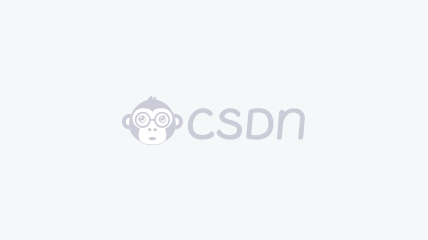
- woaixiaomo_20082013-03-03不错的例子,我想简单点调用网络资源,这个给我很大 的启发,谢谢
- piaoyu5812012-08-29是调用其他网站的ip地址分析,不是我需要的。

- 粉丝: 10
- 资源: 4
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

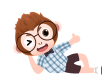
最新资源

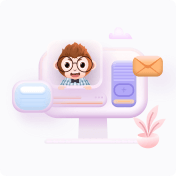
