% Developed in MATLAB R2013b
% Source codes demo version 1.0
% _____________________________________________________
% Main paper:
% Harris hawks optimization: Algorithm and applications
% Ali Asghar Heidari, Seyedali Mirjalili, Hossam Faris, Ibrahim Aljarah, Majdi Mafarja, Huiling Chen
% Future Generation Computer Systems,
% DOI: https://doi.org/10.1016/j.future.2019.02.028
% https://www.sciencedirect.com/science/article/pii/S0167739X18313530
% _____________________________________________________
% You can run the HHO code online at codeocean.com https://doi.org/10.24433/CO.1455672.v1
% You can find the HHO code at https://github.com/aliasghar68/Harris-hawks-optimization-Algorithm-and-applications-.git
% _____________________________________________________
% Author, inventor and programmer: Ali Asghar Heidari,
% PhD research intern, Department of Computer Science, School of Computing, National University of Singapore, Singapore
% Exceptionally Talented Ph. DC funded by Iran's National Elites Foundation (INEF), University of Tehran
% 03-03-2019
% Researchgate: https://www.researchgate.net/profile/Ali_Asghar_Heidari
% e-Mail: as_heidari@ut.ac.ir, aliasghar68@gmail.com,
% e-Mail (Singapore): aliasgha@comp.nus.edu.sg, t0917038@u.nus.edu
% _____________________________________________________
% Co-author and Advisor: Seyedali Mirjalili
%
% e-Mail: ali.mirjalili@gmail.com
% seyedali.mirjalili@griffithuni.edu.au
%
% Homepage: http://www.alimirjalili.com
% _____________________________________________________
% Co-authors: Hossam Faris, Ibrahim Aljarah, Majdi Mafarja, and Hui-Ling Chen
% Homepage: http://www.evo-ml.com/2019/03/02/hho/
% _____________________________________________________
%% %%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%
% Harris's hawk optimizer: In this algorithm, Harris' hawks try to catch the rabbit.
% T: maximum iterations, N: populatoin size, CNVG: Convergence curve
% To run HHO: [Rabbit_Energy,Rabbit_Location,CNVG]=HHO(N,T,lb,ub,dim,fobj)
function [Rabbit_Energy,Rabbit_Location,CNVG]=TTHHO(N,T,lb,ub,dim,fobj)
disp('TTHHO is now tackling your problem')
tic
% initialize the location and Energy of the rabbit
Rabbit_Location=zeros(1,dim);
Rabbit_Energy=inf;
%Initialize the locations of Harris' hawks
X=initialization(N,dim,ub,lb);
CNVG=zeros(1,T);
t=0; % Loop counter
while t<T
for i=1:size(X,1)
% Check boundries
FU=X(i,:)>ub;FL=X(i,:)<lb;X(i,:)=(X(i,:).*(~(FU+FL)))+ub.*FU+lb.*FL;
% fitness of locations
fitness=fobj(X(i,:));
% Update the location of Rabbit
if fitness<Rabbit_Energy
Rabbit_Energy=fitness;
Rabbit_Location=X(i,:);
best_voltage=Rabbit_Location;
end
end
K=1; % K is a real can be 0, 1, 2,....
E1=2*(1-(t/T)); % factor to show the decreaing energy of rabbit
% Update the location of Harris' hawks
delta=rand()*(sin((pi/2)*(t/T))+cos((pi/2)*(t/T))-1);
for i=1:size(X,1)
r1=rand();r2=rand();
r3 = rand();
L=2*E1*r1-E1;
C1=K*r2*E1+1;
E0=2*rand()-1; %-1<E0<1
Escaping_Energy=E1*(E0); % escaping energy of rabbit
r9=(2*pi)*rand();
r10=2*rand;
r11=rand();
if abs(Escaping_Energy)>=1
%% Exploration:
% Harris' hawks perch randomly based on 2 strategy:
q=rand();
rand_Hawk_index = floor(N*rand()+1);
X_rand = X(rand_Hawk_index, :);
if q<0.5
% perch based on other family members
if r3<0.5
if r11<0.5
X(i,:)=X_rand-rand()*abs(X_rand-2*rand()*(best_voltage+exp(-L)*(X(i,:)+(E1*sin(r9)*abs(r10*best_voltage-X(i,:)))-C1*best_voltage)));
elseif r11>=0.5
X(i,:)=X_rand-rand()*abs(X_rand-2*rand()*(best_voltage+exp(-L)*(X(i,:)+(E1*cos(r9)*abs(r10*best_voltage-X(i,:)))-C1*best_voltage)));
end
elseif r3>=0.5
if r11<0.5
X(i,:)=X_rand-rand()*abs(X_rand-2*rand()*(best_voltage+exp(-L)*(cos(L*2*pi)+tan(L*2*pi))*abs(X(i,:)+(E1*sin(r9)*abs(r10*best_voltage-X(i,:)))-C1*best_voltage)));
elseif r11 >= 0.5
X(i,:)=X_rand-rand()*abs(X_rand-2*rand()*(best_voltage+exp(-L)*(tan(L*2*pi)+sin(L*2*pi))*abs(X(i,:)+(E1*cos(r9)*abs(r10*best_voltage-X(i,:)))-C1*best_voltage)));
end
end
elseif q>=0.5
% perch on a random tall tree (random site inside group's home range)
X(i,:)=(Rabbit_Location(1,:)-mean(X))-rand()*((ub-lb)*rand+lb);
end
elseif abs(Escaping_Energy)<1
%% Exploitation:
% Attacking the rabbit using 4 strategies regarding the behavior of the rabbit
%% phase 1: surprise pounce (seven kills)
% surprise pounce (seven kills): multiple, short rapid dives by different hawks
r=rand(); % probablity of each event
if r>=0.5 && abs(Escaping_Energy)<0.5 % Hard besiege
if r3<0.5
if r11<0.5
X(i,:)=(Rabbit_Location)-Escaping_Energy*abs(Rabbit_Location-(best_voltage+exp(-L)*(X(i,:)+(E1*sin(r9)*abs(r10*best_voltage-X(i,:)))-C1*best_voltage)));
elseif r11>=0.5
X(i,:)=(Rabbit_Location)-Escaping_Energy*abs(Rabbit_Location-(best_voltage+exp(-L)*(X(i,:)+(E1*cos(r9)*abs(r10*best_voltage-X(i,:)))-C1*best_voltage)));
end
elseif r3>=0.5
if r11<0.5
X(i,:)=(Rabbit_Location)-Escaping_Energy*abs(Rabbit_Location-(best_voltage+exp(-L)*(cos(L*2*pi)+tan(L*2*pi))*abs(X(i,:)+(E1*sin(r9)*abs(r10*best_voltage-X(i,:)))-C1*best_voltage)));
elseif r11 >= 0.5
X(i,:)=(Rabbit_Location)-Escaping_Energy*abs(Rabbit_Location-(best_voltage+exp(-L)*(tan(L*2*pi)+sin(L*2*pi))*abs(X(i,:)+(E1*cos(r9)*abs(r10*best_voltage-X(i,:)))-C1*best_voltage)));
end
end
end
if r>=0.5 && abs(Escaping_Energy)>=0.5 % Soft besiege
Jump_strength=2*(1-rand()); % random jump strength of the rabbit
if r3<0.5
if r11<0.5
X(i,:)=(Rabbit_Location-(best_voltage+exp(-L)*(X(i,:)+(E1*sin(r9)*abs(r10*best_voltage-X(i,:)))-C1*best_voltage)))-Escaping_Energy*abs(Jump_strength*Rabbit_Location-(best_voltage+exp(-L)*(X(i,:)+(E1*sin(r9)*abs(r10*best_voltage-X(i,:)))-C1*best_voltage)));
elseif r11>=0.5
X(i,:)=(Rabbit_Location-(best_voltage+exp(-L)*(X(i,:)+(E1*cos(r9)*abs(r10*best_voltage-X(i,:)))-C1*best_voltage)))-Escaping_Energy*abs(Jump_strength*Rabbit_Location-(best_voltage+exp(-L)*(X(i,:)+(E1*cos(r9)*abs(r10*best_voltage-X(i,:)))-C1*best_voltage)));
end
elseif r3>=0.5
if r11<0.5
X(i,:)=(Rabbit_Location-(best_voltage+exp(-L)*(cos(L*2*pi)+tan(L*2*pi))*abs(X(i,:)+(E1*sin(r9)*abs(r10*best_voltage-X(i,:)))-C1*best_voltage)))-Escaping_Energy*abs(Jump_strength*Rabbit_Location-(best_voltage+exp(-L)*(cos(L*2*pi)+tan(L*2*pi))*abs(X(i,:)+(E1*sin(r9)*abs(r10*best_voltage-X(i,:)))-C1*best_voltage)));
elseif r11 >= 0.5
X(i,:)=(Rabbit_Location-(best_voltage+exp(-L)*(tan(L*2*pi)+sin(L*2*pi))*abs(X(i,:)+(E1*cos(r9)*abs(r10*best_voltage-X(i,:)))-C1*best_voltage)))-Escaping_Energy*abs(Jump_streng
没有合适的资源?快使用搜索试试~ 我知道了~
混合优化算法用于解决优化问题-是TSO、SCA和HHO算法的混合体-matlab
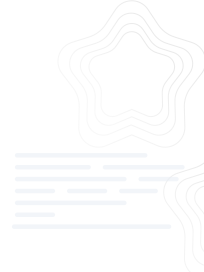
共4个文件
m:4个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 184 浏览量
2024-07-31
17:47:39
上传
评论
收藏 7KB ZIP 举报
温馨提示
瞬态三角哈里斯-霍克斯优化器 提出了一种新的混合优化算法, 一种新的混合优化算法,即瞬态三角哈里斯-霍克斯优化器(TTHHO),专门用于解决优化问题。它是TSO、SCA和HHO算法的混合体。
资源推荐
资源详情
资源评论
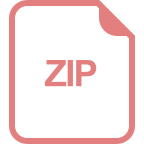
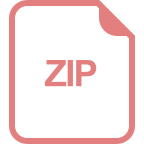
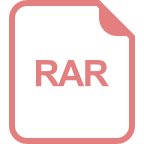
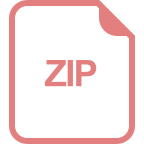
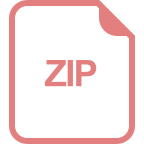
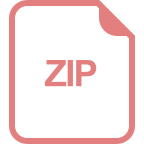
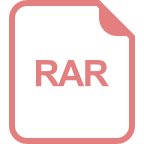
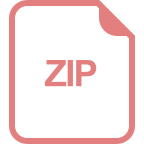
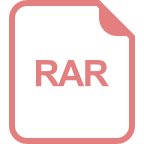
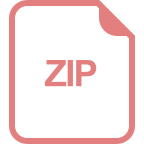
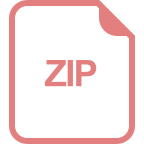
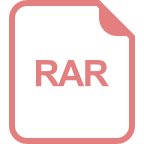
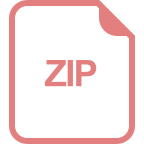
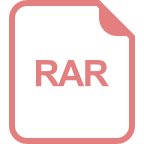
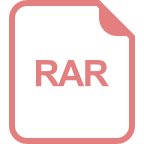
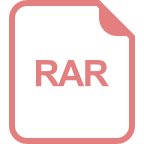
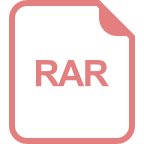
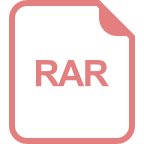
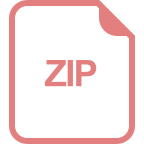
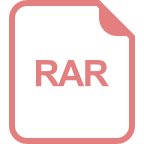
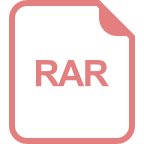
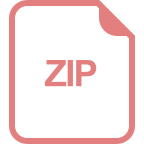
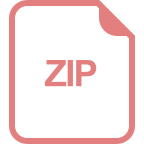
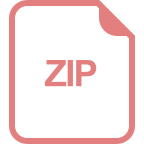
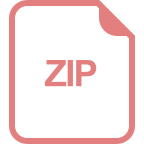
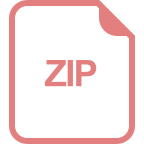
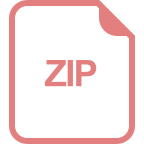
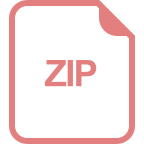
收起资源包目录

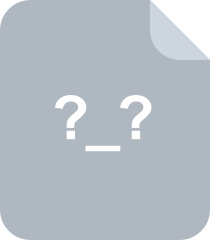
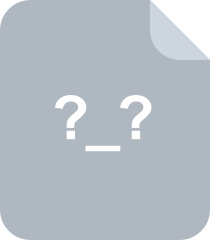
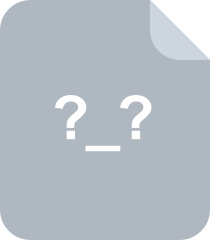
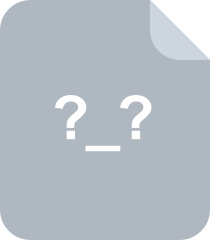
共 4 条
- 1
资源评论
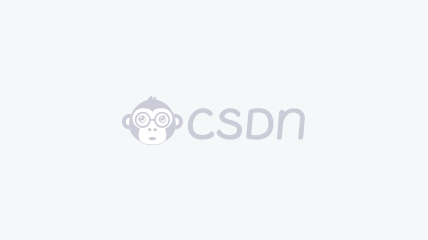

自不量力的A同学
- 粉丝: 763
- 资源: 2785
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

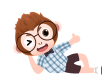
最新资源
- 全国基础地理信息数据含水系、高程、气象站shp、乡镇行政shp、DEM等最新整理.zip
- SLAM-混合稀疏视觉测距-优质项目实战.zip
- WPF入门-03路由事件(附c#代码)
- SLAM-分段针孔单目鱼眼视觉SLAM系统设计-优质项目实战.zip
- IMG_2185.jpg
- 基于SpringBoot+Vue的鲜果产销交互平台(前端代码)
- test01(1).py
- 基于SpringBoot+Vue的鲜果产销交互平台(后端代码)
- SLAM-动态语义SLAM-目标检测+VSLAM+光流+多视角几何动态物体检测+octomap地图+目标数据库-优质项目.zip
- PTA平台上C语言编程示例:超速判断程序解析
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


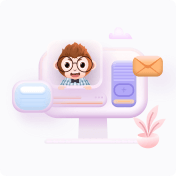
安全验证
文档复制为VIP权益,开通VIP直接复制
