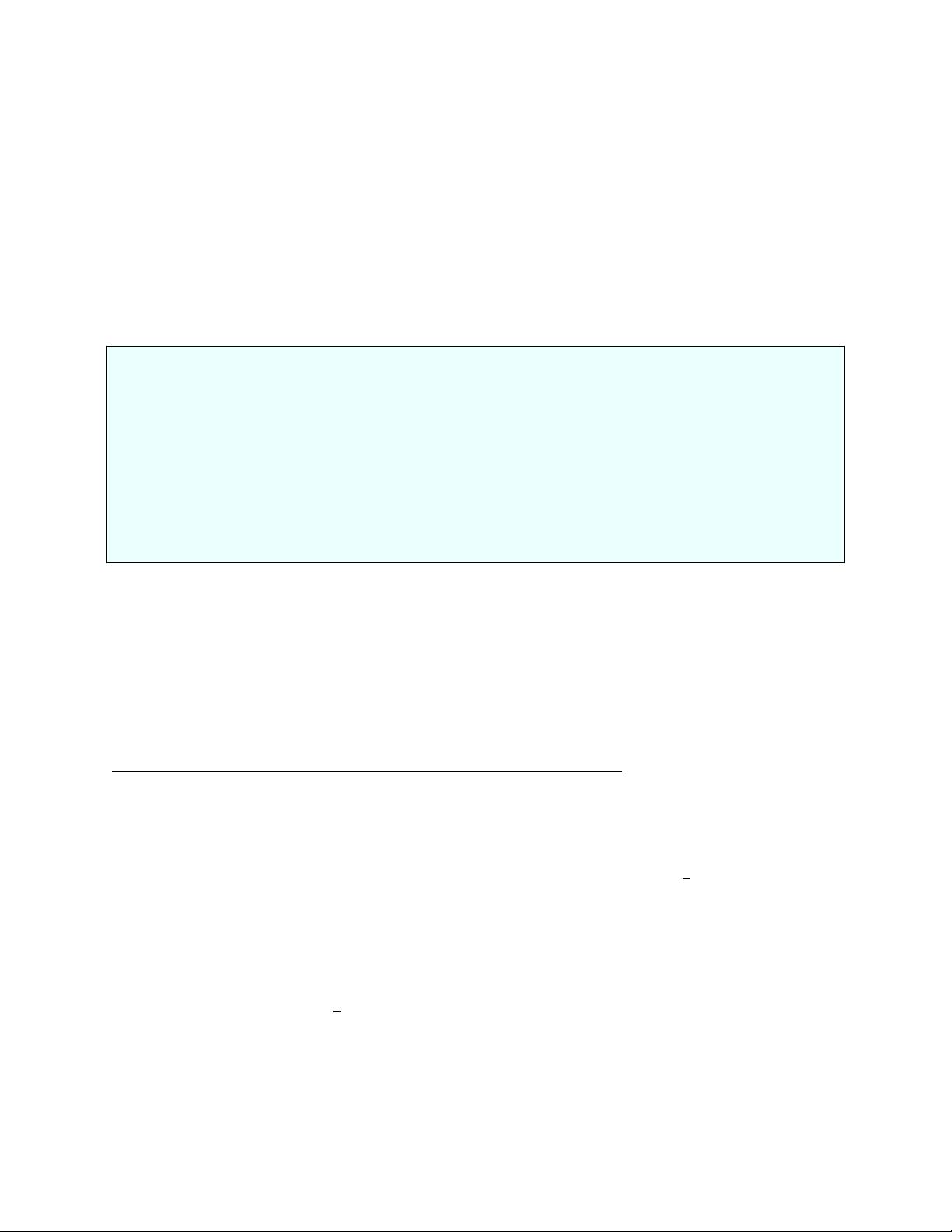
To determine the square root of x
Initialize:
Start with r = x
Iterate as often as desired:
Replace r by the average of r and x/r.
Let’s try this method to compute the square root of 2. The instruction to iterate “as often as desired” will
be interpreted to mean that we will stop as soon as |2 − r
2
| < 1.0e − 6.
Our code might look like this:
n = 0
while ( True ) :
i f ( n == 0 ) :
r = x
e ls e :
r = ( r + x / r ) / 2 . 0
e = abs ( x − r ∗ r )
i f ( e < t o l ) :
break
n = n + 1
Notice that for this iteration, we don’t specify in advance how many steps we will take. We do try to keep
track of this number by updating the value of n, but our while() loop uses the condition True which means,
just keep looping forever. Since we definitely don’t want to loop forever, we have to escape by specifying
some condition that we are waiting for. In our case, we want |x − r
2
| < tol, and when we see that has
happened, we break from the loop.
The other thing to notice is that, instead of initializing r outside the loop, we use an if() statement, which
combines the initialization and update formulas into a single block.
The table below shows how our iteration proceeds when searching for the square root of 2:
n r x/r x − r
2
0 2.0 1.0 2.0
1 1.5 1.3333333333333333 0.25
2 1.4166666666666665 1.411764705882353 0.006944444444444198
3 1.4142156862745097 1.41421143847487 6.007304882427178e-06
4 1.4142135623746899 1.4142135623715002 4.510614104447086e-12
This result was surprisingly quick. Looking up the actual value to 16 digits, we get
√
2 ≈ 1.414213562373095
so we’ve done pretty well.
Some questions naturally arise:
• Is there some explanation for why this method works?
• Would this same procedure work for x = 10, or for x = 1, 000, 000?
• Why does r seem to decrease on every step, while x/r rises?
• Can we get 100 digits of
√
2 this way?
• Can we devise a similar method for, say, cube roots?
For some help with the first question, consider the following: On each iteration, we are averaging two
numbers, r and x/r, with x/r <= r, and whose product is x. Our next estimate for the square root is the
average of these two values, so it must be between x/r and r. That is, the next value of r must be smaller
2