libplctag
=========
This library for Linux and Windows provides a means of accessing PLCs to read and write
simple data.
Stable Version: 2.0
Old Stable Version: 1.5
WARNING - DISCLAIMER
====================
Note: *PLCs control many kinds of equipment and loss of property, production
or even life can happen if mistakes in programming or access are
made. Always use caution when accessing or programming PLCs!*
We make no claims or warrants about the suitability of this code for
any purpose.
Be careful!
Have fun and let us know if this library is useful to you. Please send test
cases if you run into bugs. As PLC hardware is fairly expensive, we may not
be able to test out your test scenarios. If possible, please send patches.
We do not ask that you transfer copyright over to us, but we do ask that you
make any submitted patches under the same LGPL license we use. We will not
take any patches under the GPL license or licenses that are incompatible with the
LGPL.
- the libplctag team.
Goals
=====
The primary goals of this library are to provide:
* a simple, consistent way to access PLCs or similar devices of various types.
* cross-platform support (currently Windows and Linux).
* protocol-agnostic access.
* an easily wrappable library.
* a portable library for big and little-endian, and 32 and 64-bit processors.
We do not hit all those goals yet. A lack of big-endian systems is preventing testing
there. It has been tested in the past on an emulated big-endian MIPS system in QEMU.
Non Goals
=========
This library is not to replace tools such as RSLinx or stacks like OPC. It
only reads and writes tags. Other tools and systems may provide significantly
more functionality. We do not want that. We want to keep it small and simple.
Licensing
=========
See the file LICENSE for our legal disclaimers of responsibility, fitness or
merchantability of this library as well as your rights with regards
to use of this library. This code is licensed under the GNU LGPL.
Current Status
==============
Take a look at the [test page](https://github.com/kyle-github/libplctag/wiki/PLC-Testing-Results "PLC Testing Results") for more on specific PLC models.
The library has been in production use since 2012 and is in use by multiple organizations including some very large ones.
We are on API version 2.0. That includes:
* CMake build system for better cross-platform support.
* support for Rockwell/Allen-Bradley ControlLogix(tm) PLCs via CIP-EtherNet/IP (CIP/EIP or EIP)(tm?). Firmware versions 16, 20 and 31.
* read/write 8, 16, 32, and 64-bit signed and unsigned integers.
* read/write single booleans under some circumstances (BOOL arrays are still tricky).
* read/write 32-bit and 64-bit IEEE format (little endian) floating point.
* raw support for user-defined structures (you need to pull out the data piece by piece)
* read/write arrays of the above.
* multiple-request support per packet.
* packet size negotiation with newer firmward (version 20+).
* support for accessing Logix-class PLCs via PLC/5 protocol.
* support for INT and REAL read and write.
* support for Rockwell/Allen-Bradley MicroLogix 850 PLCs.
* Support as for ControlLogix.
* support for Rockwell/Allen-Bradley MicroLogix 1100 and 1400 series (not CIP-based)
* use as per PLC5/SLC below.
* Bits not directly supported yet.
* Three address form not well supported.
* support for Rockwell/Allen-Bradley PLC5 PLCs (E-series with Ethernet), and SLC 500 with Ethernet via CIP.
* read/write of 16-bit INT.
* read/write of 32-bit floating point.
* read/write of arrays of the above (arrays not tested on SLC 500).
* support for Rockwell/Allen-Bradley PLC5 PLCs accessed over a DH+ bridge (i.e. a LGX chassis with a DHRIO module).
* read/write of 16-bit INT.
* read/write of 32-bit floating point.
* read/write of arrays of the above.
* support for 32 and 64-bit x86 Linux (Ubuntu 12.04-18.10 tested).
* Windows 7 x86 and Windows 10 x64 builds with Visual Studio, not well tested (help very welcome!). Support is basic because:
* we do not use Windows for our deployments.
* only the tag_rw example program has been tested (though that tests most of the API).
* sample code.
* a stable API. The release of 2.0 is the first breaking change in over four years.
* stable C API with wrappers in Python and Java.
* user-contributed/user-supported wrappers for C# and Pascal.
* support for request bundling on supporting PLCs (ControlLogix and CompactLogix). This is automatic within the library.
* we have deployed this in customer environments.
* we have reports of successful use on ARM-based systems including the RaspberryPi boards.
* other groups use this library (if you do, please let us know).
PLC5, SLC 500, MicroLogix, Micro8X0, CompactLogix and ControlLogix are trademarks of Rockwell/Allen Bradley.
Windows and Visual Studio are trademarks of Microsoft. Please let us know if we missed some so
that we can get all the attributions correct!
We need and welcome help with the following:
* bug fixes and reports.
* other protocols like Modbus, SBus etc.
* other platforms like Android, iOS, macOS etc.
* other versions of Windows.
* more language wrappers!
Portability
===========
We have tried to maintain a high level of portability. For the most part the
code conforms to C99. However, we do the following things that may be an
issue for some compilers:
* we make assumptions about the bit layout of IEEE floating point numbers in memory. x86-based processors handle this fine, but nothing else has been tested.
* opaque pointers may be an issue on some compilers, but this is generally not an issue with 2.0 as it no longer presents opaque pointers through the library API.
* we use packed structures and access structure elements off of alignment boundaries.
* threading. We tried to avoid this, but at least Allen-Bradley/Rockwell's protocol is very much asynchronous.
* we still have one spot where we use inline variable declaration. This is about the only remaining C99 requirement.
We have limited access to some types of PLCs and most of our testing
has been on PLC5 and ControlLogix machines.
We are trying to keep things as simple as possible so that this can be easily
deployed in embedded systems. We have limited the API "surface area" as much
as possible. We also have made an effort to limit internal use of things
like malloc and free. If you wrap the library, you will need to make sure that
finalizers take care of calling the destruction functions to deallocate internally
allocated memory.
Threading
=========
Access to the C API is thread-safe. All threads hit a mutex when going through any API call.
By "thread safe" we mean that you should not be able to crash the library by sharing a tag object
between threads. That does not mean that you will get useful results!
Trying to share a tag between threads is possible, but access in only controlled within a
single API call. If you do something like update tag data in one thread and call the read
function in another thread, the order of the operations will be determined by the actual
chronological order in which the threads executed them.
For this reason, we added additional lock/unlock API calls that allow you to synchronize
access to a tag across multiple API calls. If you are using the library wrapped in another
programming language you should use that languages synchronization mechanisms instead.
The Allen-Bradley EIP protocol is very asynchronous and the part of it that we
have implemented does use threading internally. The library uses one thread for all tags
and one thread per target PLC.
The API
=======
The library uses opaque integer handles with accessor functions. There are only a
few functions in the API.
These functions operate on all types of tags. API functions that take a timeout argument
can be used in a synchronous manner or asynchronous manner. Providing a timeout will
cause the
没有合适的资源?快使用搜索试试~ 我知道了~
ethernet-ip协议的C语言库包含源码(可用于与PLC交互)zip.zip 可用于AB的PLC交互,实现各种通信
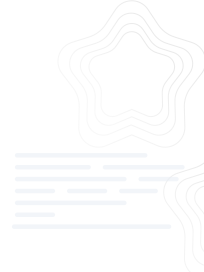
共108个文件
c:45个
h:35个
cpp:5个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉

温馨提示
ethernet-ip协议的C语言库包含源码(可用于与PLC交互)zip.zip 可用于AB的PLC交互,实现各种通信
资源推荐
资源详情
资源评论
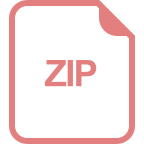
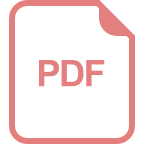
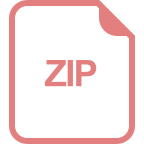
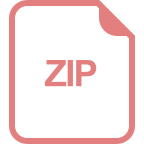
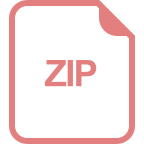
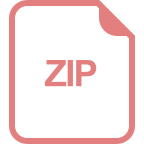
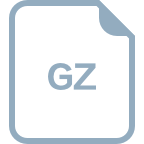
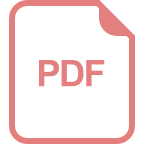
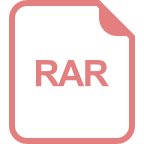
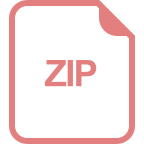
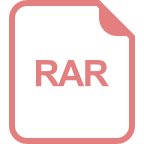
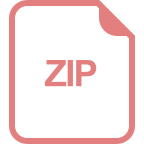
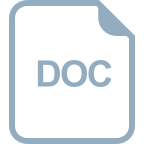
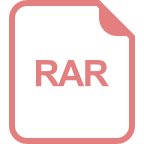
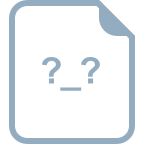
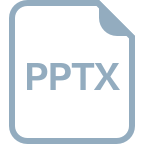
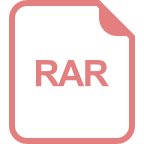
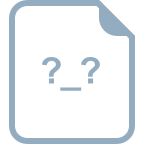
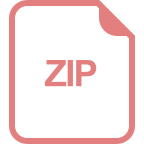
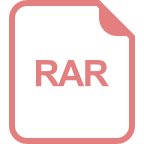
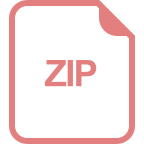
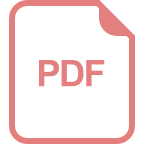
收起资源包目录

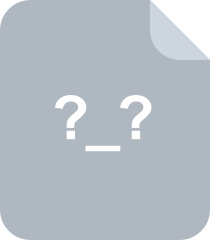
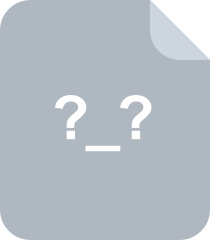
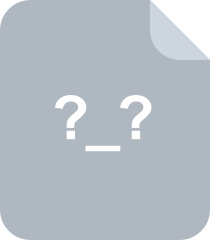
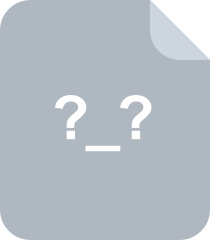
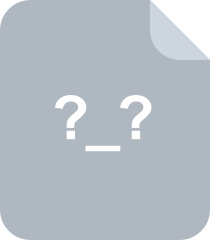
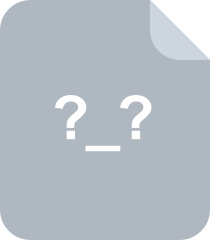
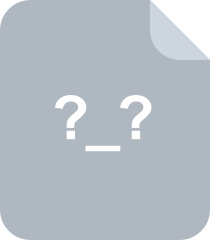
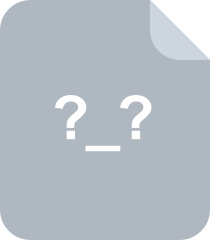
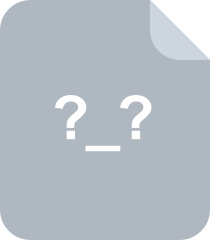
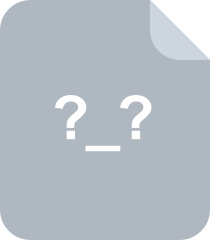
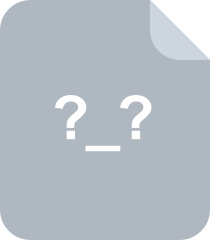
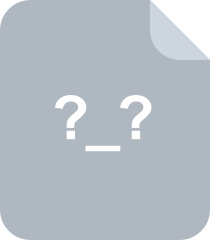
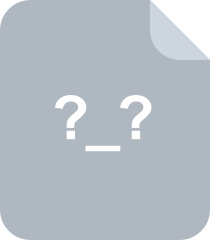
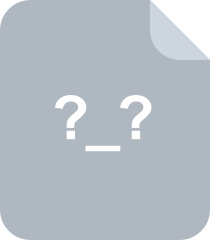
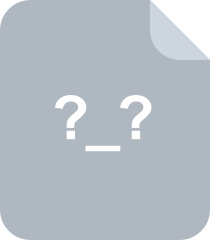
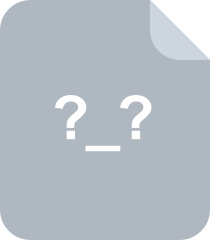
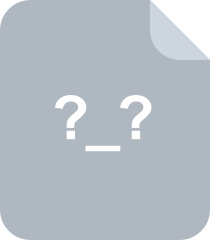
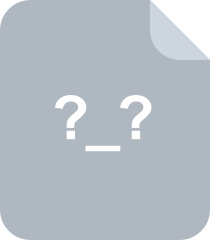
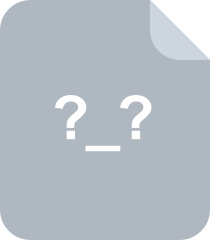
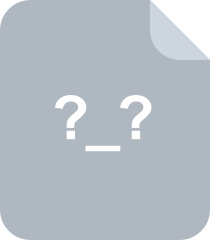
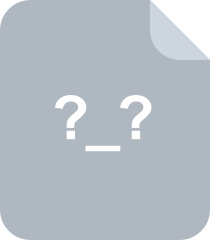
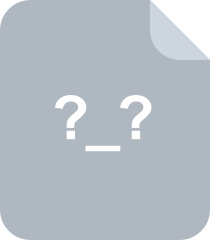
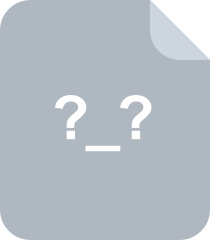
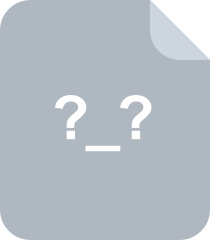
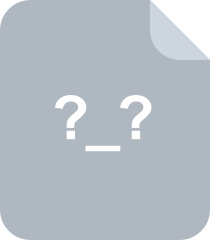
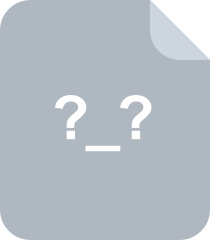
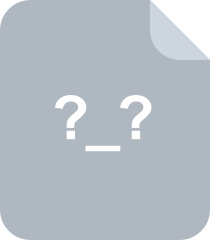
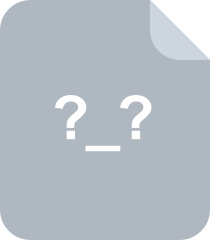
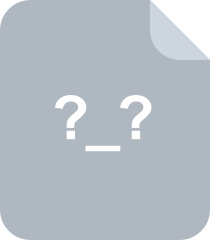
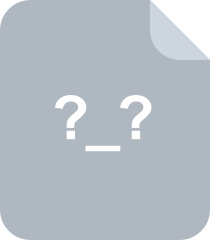
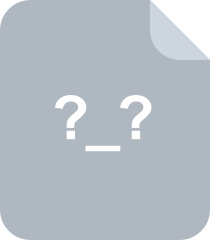
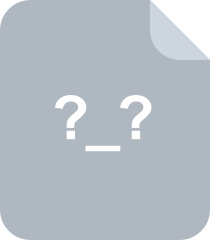
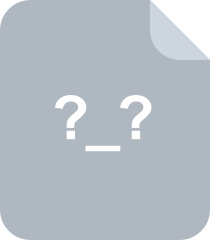
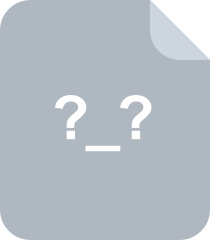
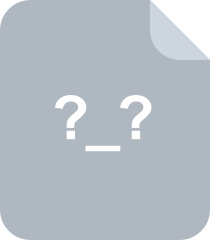
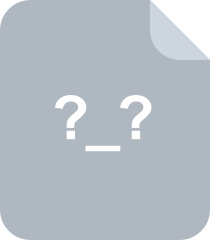
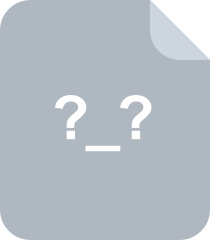
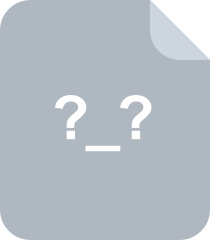
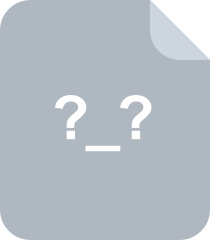
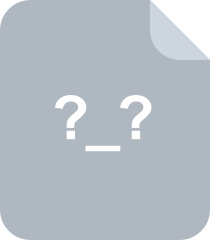
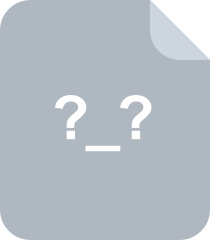
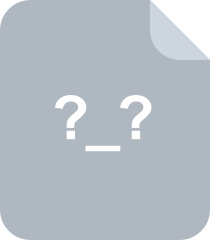
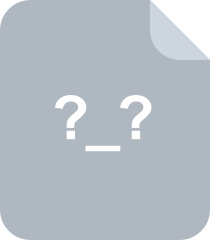
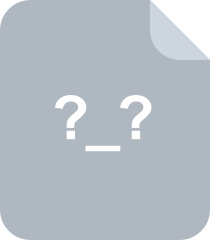
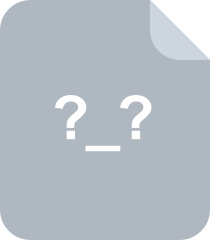
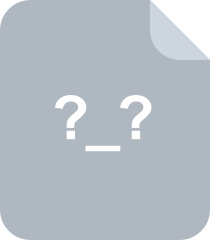
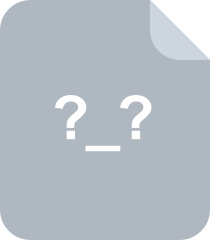
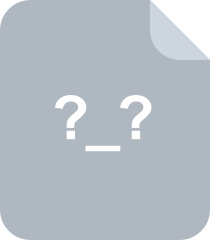
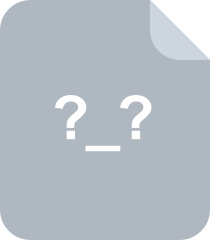
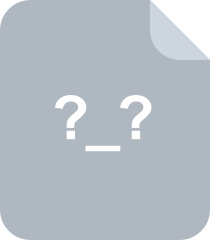
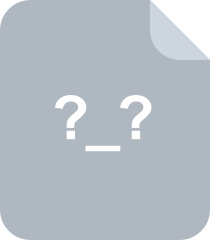
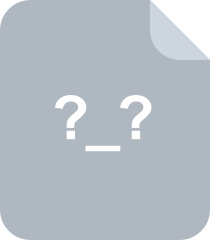
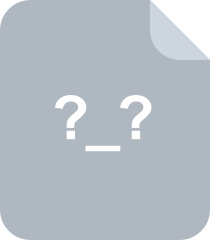
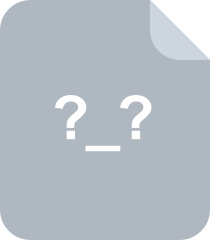
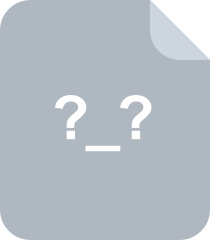
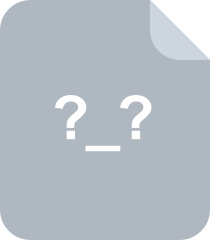
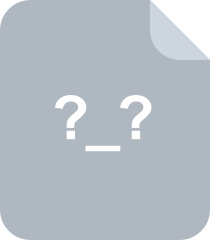
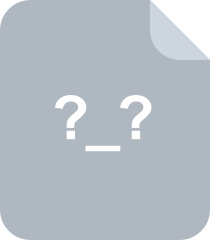
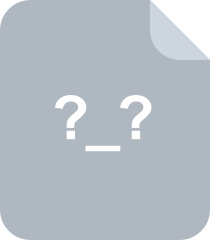
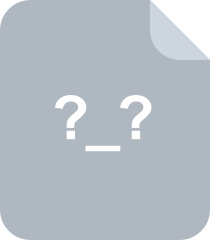
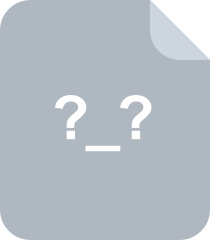
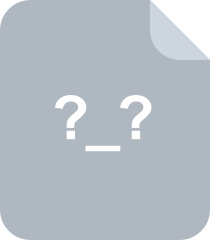
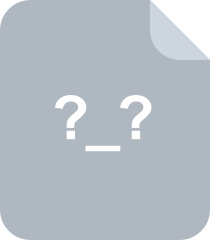
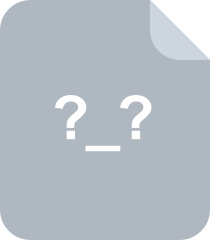
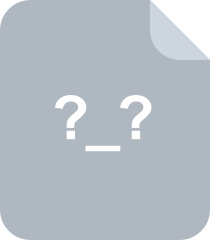
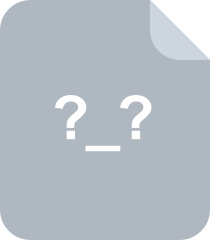
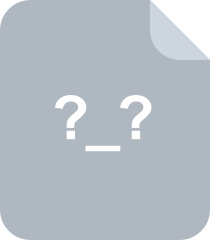
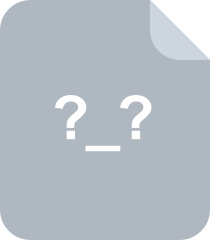
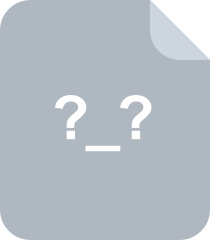
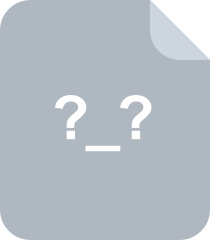
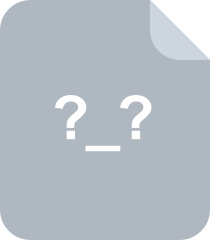
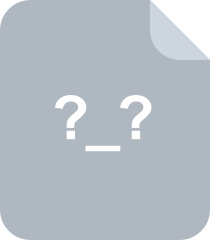
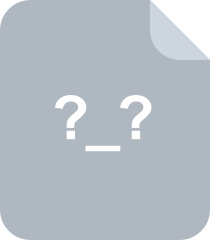
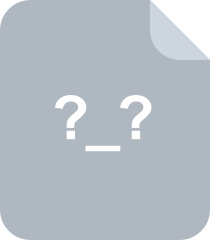
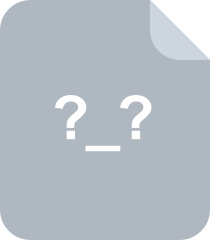
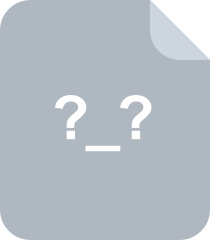
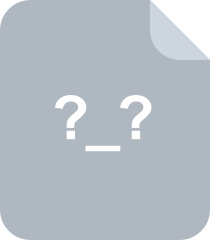
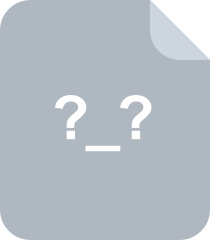
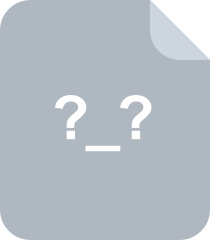
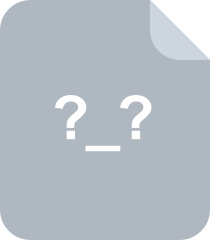
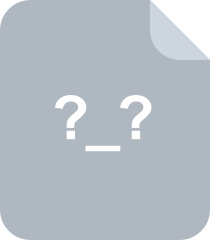
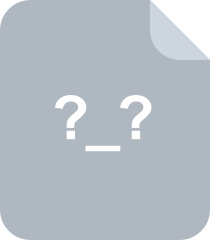
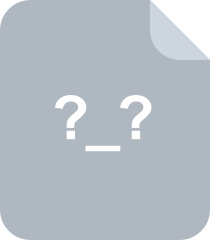
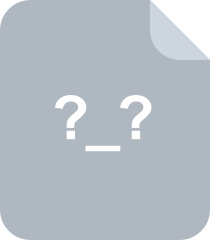
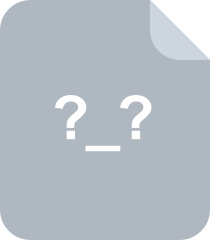
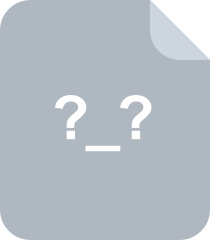
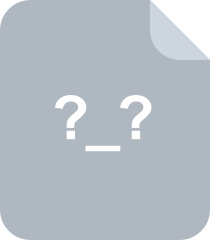
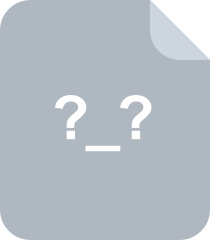
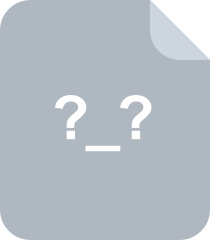
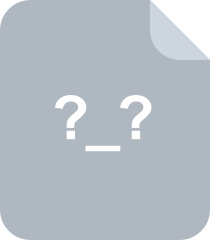
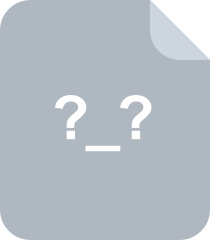
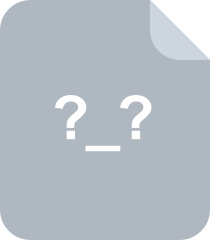
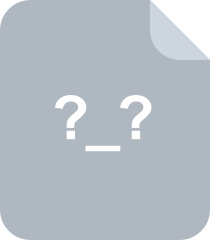
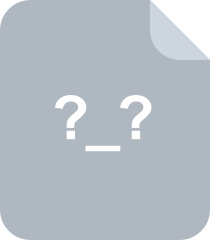
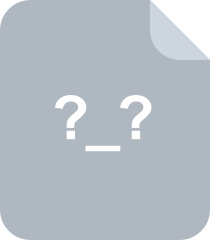
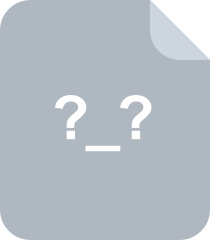
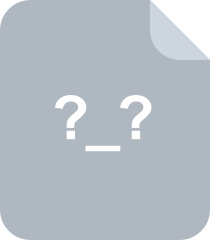
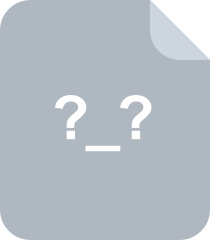
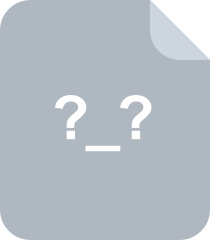
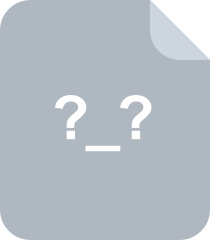
共 108 条
- 1
- 2

卷积神经网络
- 粉丝: 339
- 资源: 8460
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

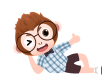
安全验证
文档复制为VIP权益,开通VIP直接复制

- 1
- 2
- 3
前往页