在编程领域,C++是一种强大的面向对象的编程语言,它允许开发者自定义数据类型来满足特定的需求。在本实例中,我们将深入探讨如何在Visual C++环境中实现一个复数类,该类支持基本的数学运算如加、减、乘、除。复数是由实部和虚部组成的数,通常表示为`a + bi`的形式,其中`a`是实部,`b`是虚部,`i`是虚数单位,满足`i^2 = -1`。 我们需要定义一个名为`Complex`的类,它包含两个私有成员变量,分别存储实部和虚部: ```cpp class Complex { private: double real; double imaginary; public: // 构造函数 Complex(double r = 0, double i = 0) : real(r), imaginary(i) {} // 获取实部和虚部的方法 double getReal() const { return real; } double getImaginary() const { return imaginary; } // 运算符重载:+ Complex operator+(const Complex& other) const { return Complex(real + other.real, imaginary + other.imaginary); } // 运算符重载:- Complex operator-(const Complex& other) const { return Complex(real - other.real, imaginary - other.imaginary); } // 运算符重载:* Complex operator*(const Complex& other) const { return Complex(real * other.real - imaginary * other.imaginary, real * other.imaginary + imaginary * other.real); } // 运算符重载:/ Complex operator/(const Complex& other) const { double denominator = other.real * other.real + other.imaginary * other.imaginary; return Complex((real * other.real + imaginary * other.imaginary) / denominator, (imaginary * other.real - real * other.imaginary) / denominator); } // 输出复数的方法 friend std::ostream& operator<<(std::ostream& os, const Complex& c) { os << c.real << " + " << c.imaginary << "i"; return os; } }; ``` 在上述代码中,我们定义了`Complex`类,并为其提供了构造函数,用于初始化复数的实部和虚部。同时,我们重载了加、减、乘、除四个运算符,每个运算符都返回一个新的`Complex`对象,表示运算的结果。在`operator<<`中,我们定义了流输出操作,使得可以方便地将复数对象输出到控制台或其他流中。 接下来,在Visual C++环境中,创建一个新的C++项目,将以上代码放入源文件(如`Complex.cpp`),并确保包含对应的头文件。然后,你可以在主函数`main()`中测试这个复数类的功能: ```cpp int main() { Complex c1(3, 4); Complex c2(1, -2); Complex sum = c1 + c2; Complex diff = c1 - c2; Complex product = c1 * c2; Complex quotient = c1 / c2; std::cout << "c1 = " << c1 << "\nc2 = " << c2 << "\n" << "Sum: " << sum << "\nDifference: " << diff << "\nProduct: " << product << "\nQuotient: " << quotient << std::endl; return 0; } ``` 这段`main()`函数创建了两个复数对象`c1`和`c2`,然后进行加、减、乘、除运算,并输出结果。运行程序,你会看到符合预期的复数运算结果。 通过这样的实现,我们可以方便地处理复数,并利用C++的面向对象特性实现复数运算的封装。这个`Complex`类的设计和实现展示了C++中类、构造函数、运算符重载和友元函数等核心概念。这不仅有助于理解和掌握C++语言,也为解决更复杂的数学问题提供了基础。
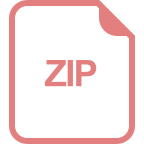
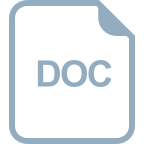
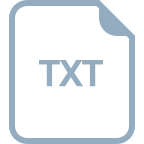
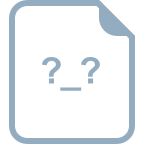
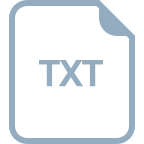
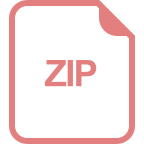
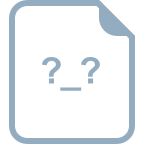
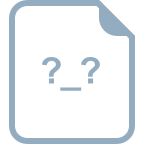
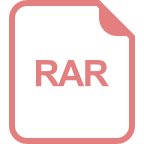
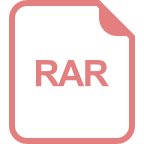
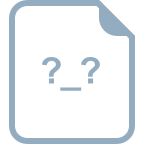
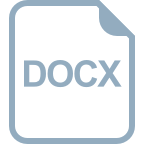
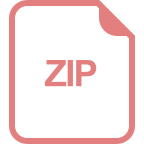
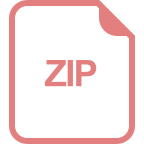
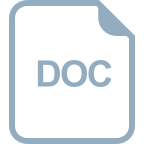
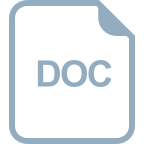
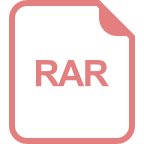
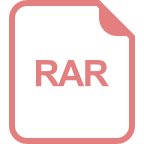


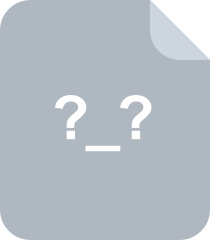
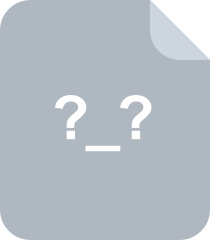
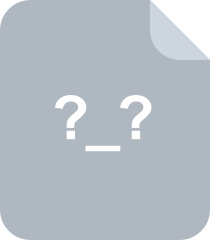
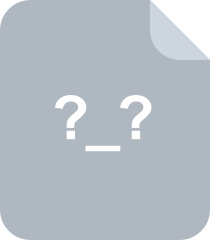
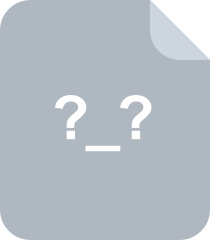
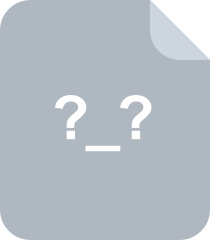
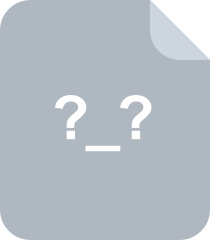
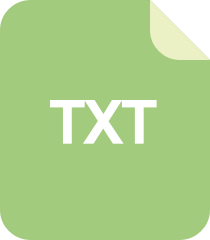
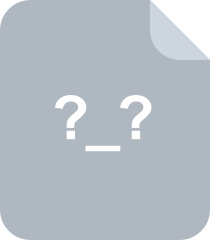
- 1
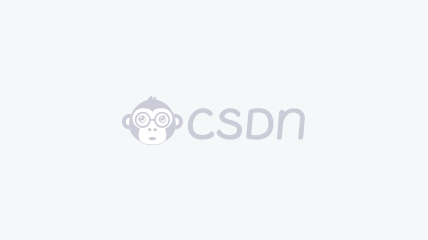
- a0110561232012-03-23一个比较初级的源代码,刚开始接触类的时候看看还是挺好的。

- 粉丝: 55
- 资源: 34
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

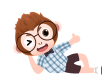
最新资源
- 5G模组升级刷模块救砖以及5G模组资料路由器固件
- C183579-123578-c1235789.jpg
- Qt5.14 绘画板 Qt Creator C++项目
- python实现Excel表格合并
- Java实现读取Excel批量发送邮件.zip
- 【java毕业设计】商城后台管理系统源码(springboot+vue+mysql+说明文档).zip
- 【java毕业设计】开发停车位管理系统(调用百度地图API)源码(springboot+vue+mysql+说明文档).zip
- 星耀软件库(升级版).apk.1
- 基于Django后端和Vue前端的多语言购物车项目设计源码
- 基于Python与Vue的浮光在线教育平台源码设计

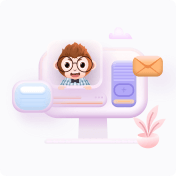
