/* Copyright 1999-2004 The Apache Software Foundation
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
/*
* httpd.c: simple http daemon for answering WWW file requests
*
*
* 03-21-93 Rob McCool wrote original code (up to NCSA HTTPd 1.3)
*
* 03-06-95 blong
* changed server number for child-alone processes to 0 and changed name
* of processes
*
* 03-10-95 blong
* Added numerous speed hacks proposed by Robert S. Thau (rst@ai.mit.edu)
* including set group before fork, and call gettime before to fork
* to set up libraries.
*
* 04-14-95 rst / rh
* Brandon's code snarfed from NCSA 1.4, but tinkered to work with the
* Apache server, and also to have child processes do accept() directly.
*
* April-July '95 rst
* Extensive rework for Apache.
*/
#ifndef SHARED_CORE_BOOTSTRAP
#ifndef SHARED_CORE_TIESTATIC
#ifdef SHARED_CORE
#define REALMAIN ap_main
int ap_main(int argc, char *argv[]);
#else
#define REALMAIN main
#endif
#define CORE_PRIVATE
#include "httpd.h"
#include "http_main.h"
#include "http_log.h"
#include "http_config.h" /* for read_config */
#include "http_protocol.h" /* for read_request */
#include "http_request.h" /* for process_request */
#include "http_conf_globals.h"
#include "http_core.h" /* for get_remote_host */
#include "http_vhost.h"
#include "util_script.h" /* to force util_script.c linking */
#include "util_uri.h"
#include "scoreboard.h"
#include "multithread.h"
#include <sys/stat.h>
#ifdef USE_SHMGET_SCOREBOARD
#include <sys/types.h>
#include <sys/ipc.h>
#include <sys/shm.h>
#endif
#ifdef SecureWare
#include <sys/security.h>
#include <sys/audit.h>
#include <prot.h>
#endif
#ifdef WIN32
#include "../os/win32/getopt.h"
#elif !defined(BEOS) && !defined(TPF) && !defined(NETWARE) && !defined(OS390) && !defined(CYGWIN)
#include <netinet/tcp.h>
#endif
#ifdef HAVE_BSTRING_H
#include <bstring.h> /* for IRIX, FD_SET calls bzero() */
#endif
#ifdef HAVE_SET_DUMPABLE /* certain levels of Linux */
#include <sys/prctl.h>
#endif
#ifdef MULTITHREAD
/* special debug stuff -- PCS */
/* Set this non-zero if you are prepared to put up with more than one log entry per second */
#define SEVERELY_VERBOSE 0
/* APD1() to APD5() are macros to help us debug. They can either
* log to the screen or the error_log file. In release builds, these
* macros do nothing. In debug builds, they send messages at priority
* "debug" to the error log file, or if DEBUG_TO_CONSOLE is defined,
* to the console.
*/
# ifdef _DEBUG
# ifndef DEBUG_TO_CONSOLE
# define APD1(a) ap_log_error(APLOG_MARK,APLOG_DEBUG|APLOG_NOERRNO,server_conf,a)
# define APD2(a,b) ap_log_error(APLOG_MARK,APLOG_DEBUG|APLOG_NOERRNO,server_conf,a,b)
# define APD3(a,b,c) ap_log_error(APLOG_MARK,APLOG_DEBUG|APLOG_NOERRNO,server_conf,a,b,c)
# define APD4(a,b,c,d) ap_log_error(APLOG_MARK,APLOG_DEBUG|APLOG_NOERRNO,server_conf,a,b,c,d)
# define APD5(a,b,c,d,e) ap_log_error(APLOG_MARK,APLOG_DEBUG|APLOG_NOERRNO,server_conf,a,b,c,d,e)
# else
# define APD1(a) printf("%s\n",a)
# define APD2(a,b) do { printf(a,b);putchar('\n'); } while(0);
# define APD3(a,b,c) do { printf(a,b,c);putchar('\n'); } while(0);
# define APD4(a,b,c,d) do { printf(a,b,c,d);putchar('\n'); } while(0);
# define APD5(a,b,c,d,e) do { printf(a,b,c,d,e);putchar('\n'); } while(0);
# endif
# else /* !_DEBUG */
# define APD1(a)
# define APD2(a,b)
# define APD3(a,b,c)
# define APD4(a,b,c,d)
# define APD5(a,b,c,d,e)
# endif /* _DEBUG */
#endif /* MULTITHREAD */
/* This next function is never used. It is here to ensure that if we
* make all the modules into shared libraries that core httpd still
* includes the full Apache API. Without this function the objects in
* main/util_script.c would not be linked into a minimal httpd.
* And the extra prototype is to make gcc -Wmissing-prototypes quiet.
*/
API_EXPORT(void) ap_force_library_loading(void);
API_EXPORT(void) ap_force_library_loading(void) {
ap_add_cgi_vars(NULL);
}
#include "explain.h"
#if !defined(max)
#define max(a,b) (a > b ? a : b)
#endif
#ifdef WIN32
#include "../os/win32/service.h"
#include "../os/win32/registry.h"
#define DEFAULTSERVICENAME "Apache"
#define PATHSEPARATOR '\\'
#else
#define PATHSEPARATOR '/'
#endif
#ifdef MINT
long _stksize = 32768;
#endif
#ifdef USE_OS2_SCOREBOARD
/* Add MMAP style functionality to OS/2 */
#define INCL_DOSMEMMGR
#define INCL_DOSEXCEPTIONS
#define INCL_DOSSEMAPHORES
#include <os2.h>
#include <umalloc.h>
#include <stdio.h>
caddr_t create_shared_heap(const char *, size_t);
caddr_t get_shared_heap(const char *);
#endif
DEF_Explain
/* Defining GPROF when compiling uses the moncontrol() function to
* disable gprof profiling in the parent, and enable it only for
* request processing in children (or in one_process mode). It's
* absolutely required to get useful gprof results under linux
* because the profile itimers and such are disabled across a
* fork(). It's probably useful elsewhere as well.
*/
#ifdef GPROF
extern void moncontrol(int);
#define MONCONTROL(x) moncontrol(x)
#else
#define MONCONTROL(x)
#endif
#ifndef MULTITHREAD
/* this just need to be anything non-NULL */
void *ap_dummy_mutex = &ap_dummy_mutex;
#endif
/*
* Actual definitions of config globals... here because this is
* for the most part the only code that acts on 'em. (Hmmm... mod_main.c?)
*/
#ifdef NETWARE
BOOL ap_main_finished = FALSE;
unsigned int ap_thread_stack_size = 65536;
#endif
int ap_thread_count = 0;
API_VAR_EXPORT int ap_standalone=0;
API_VAR_EXPORT int ap_configtestonly=0;
int ap_docrootcheck=1;
API_VAR_EXPORT uid_t ap_user_id=0;
API_VAR_EXPORT char *ap_user_name=NULL;
API_VAR_EXPORT gid_t ap_group_id=0;
#ifdef MULTIPLE_GROUPS
gid_t group_id_list[NGROUPS_MAX];
#endif
API_VAR_EXPORT int ap_max_requests_per_child=0;
API_VAR_EXPORT int ap_threads_per_child=0;
API_VAR_EXPORT int ap_excess_requests_per_child=0;
API_VAR_EXPORT char *ap_pid_fname=NULL;
API_VAR_EXPORT char *ap_scoreboard_fname=NULL;
API_VAR_EXPORT char *ap_lock_fname=NULL;
API_VAR_EXPORT char *ap_server_argv0=NULL;
API_VAR_EXPORT struct in_addr ap_bind_address={0};
API_VAR_EXPORT int ap_daemons_to_start=0;
API_VAR_EXPORT int ap_daemons_min_free=0;
API_VAR_EXPORT int ap_daemons_max_free=0;
API_VAR_EXPORT int ap_daemons_limit=0;
API_VAR_EXPORT time_t ap_restart_time=0;
API_VAR_EXPORT int ap_suexec_enabled = 0;
API_VAR_EXPORT int ap_listenbacklog=0;
#ifdef AP_ENABLE_EXCEPTION_HOOK
int ap_exception_hook_enabled=0;
#endif
struct accept_mutex_methods_s {
void (*child_init)(pool *p);
void (*init)(pool *p);
void (*on)(void);
void (*off)(void);
char *name;
};
typedef struct accept_mutex_methods_s accept_mutex_methods_s;
accept_mutex_methods_s *amutex;
#ifdef SO_ACCEPTFILTER
int ap_acceptfilter =
#ifdef AP_ACCEPTFILTER_OFF
0;
#else
1;
#endif
#endif
int ap_dump_settings = 0;
API_VAR_EXPORT int ap_extended_status = 0;
/*
* The max child slot ever assigned, preserved across restarts. Necessary
* to deal with MaxClients changes across SIGUSR1 restarts. We use this
* value to optimize routines that have to scan the entire scoreboard.
*/
static int max_daemons_limit = -1;
/*
* During config time, listeners is treated as a NULL-terminated list.
* child_main previously would start at the beginning of the list each time
* through the loop, so a socket early on in the list could easily starve out
* sockets later on in the list. The solution is to start at th
没有合适的资源?快使用搜索试试~ 我知道了~
linux的apache服务器

温馨提示
linux apache服务器apache服务器apache服务器apache服务器apache服务器apache服务器apache服务器apache服务器apache服务器apache服务器apache服务器
资源推荐
资源详情
资源评论
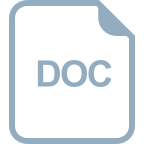
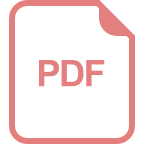
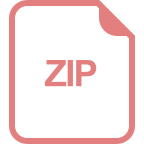
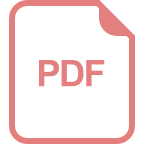
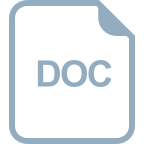
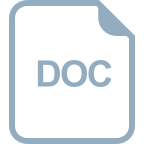
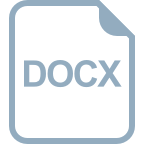
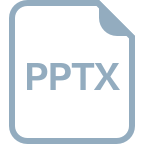
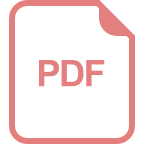
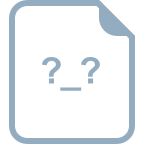
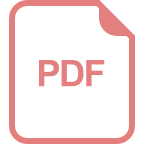
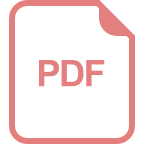
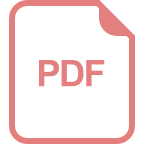
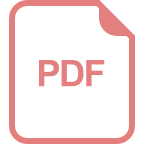
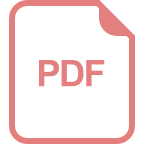
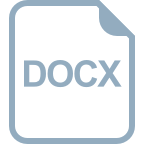
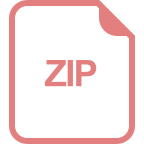
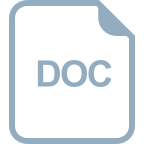
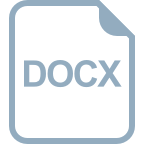
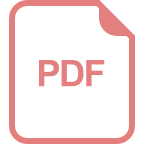
收起资源包目录

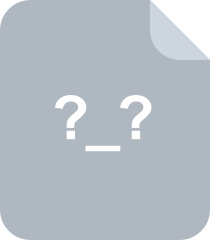
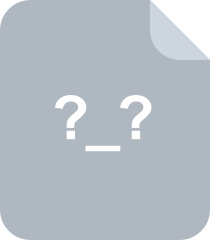
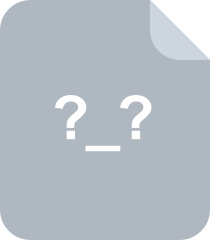
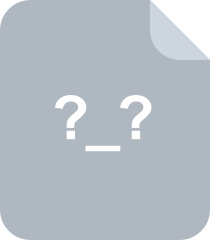
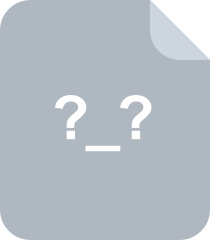
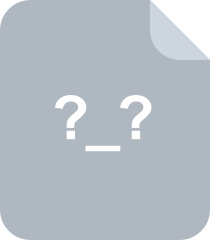
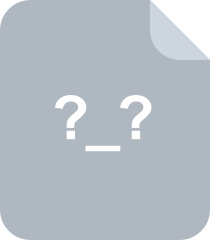
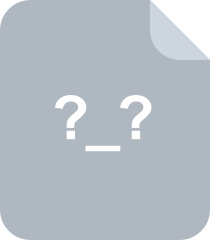
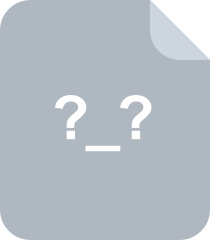
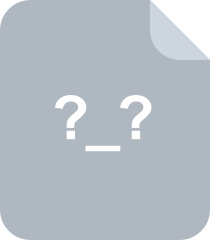
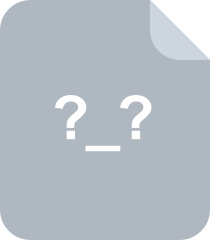
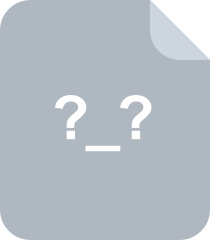
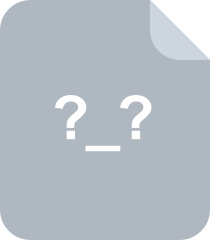
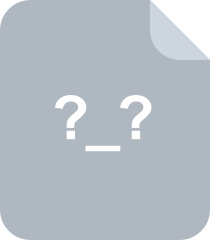
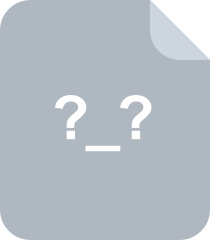
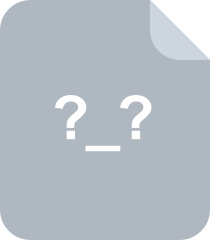
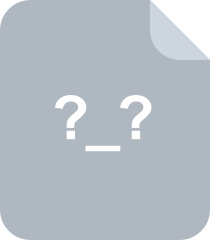
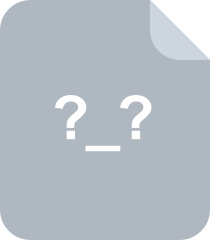
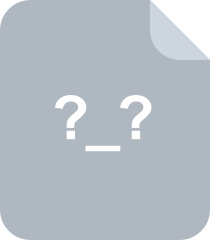
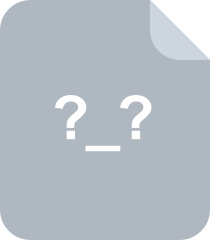
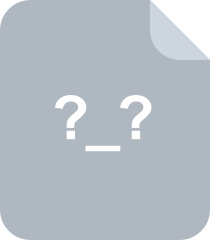
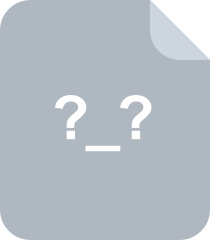
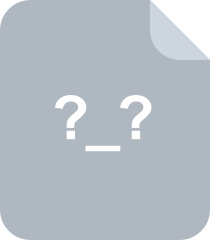
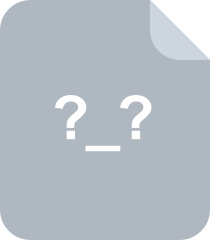
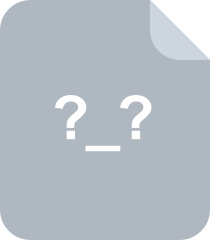
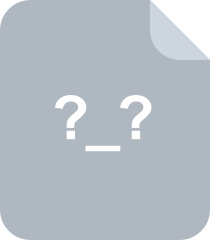
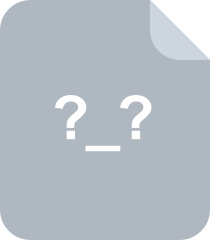
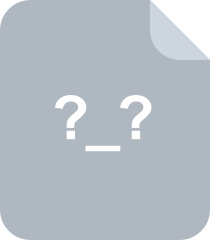
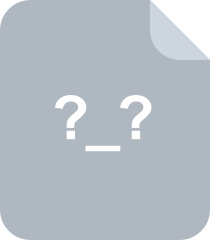
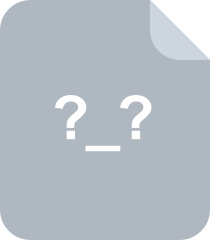
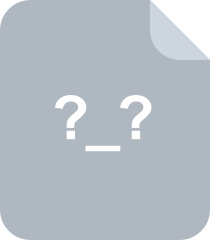
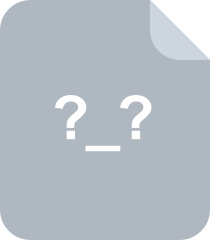
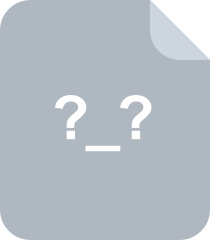
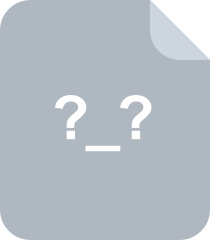
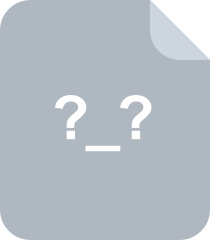
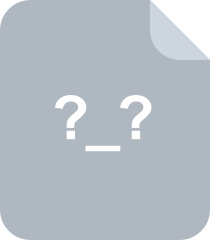
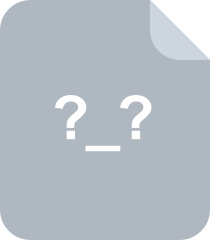
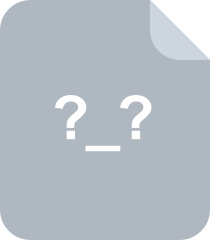
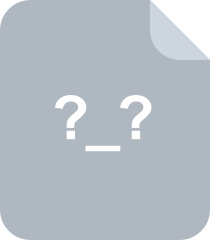
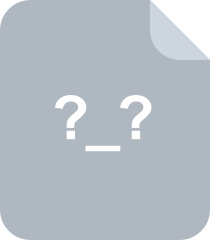
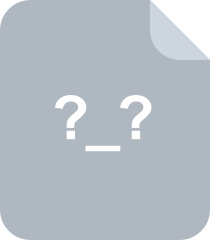
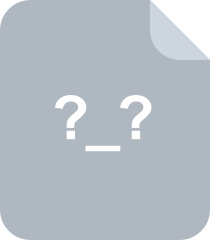
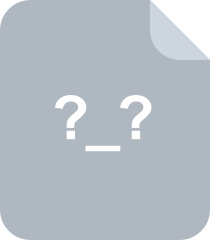
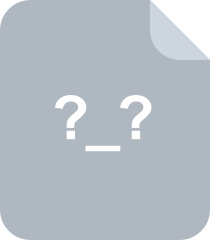
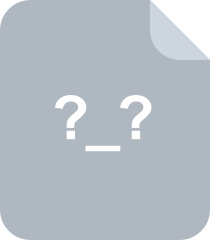
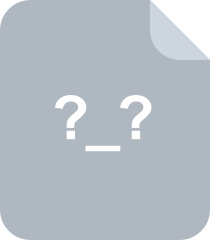
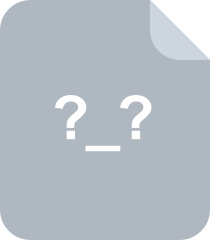
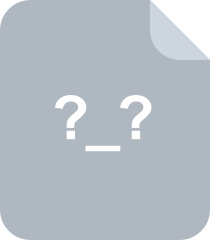
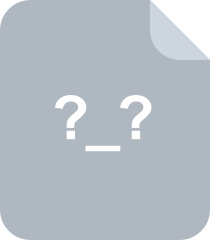
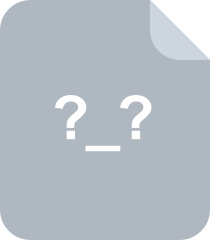
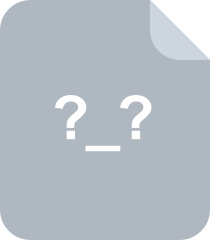
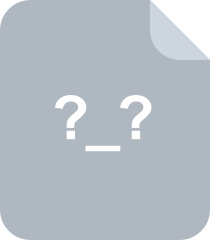
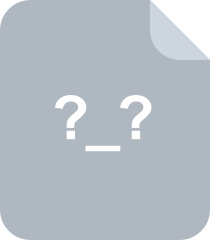
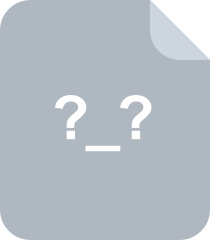
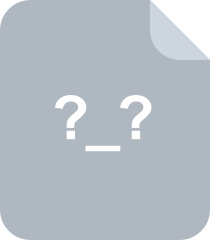
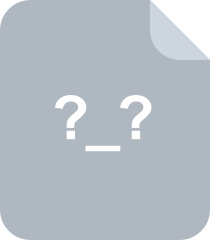
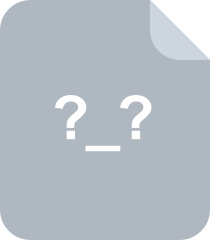
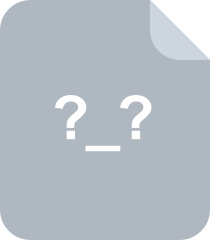
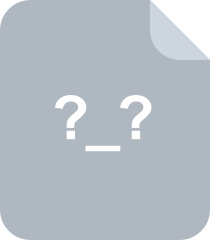
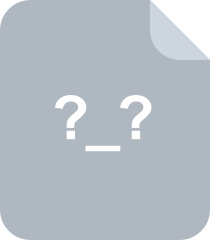
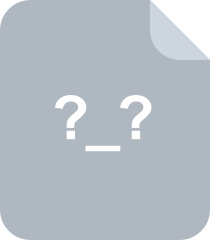
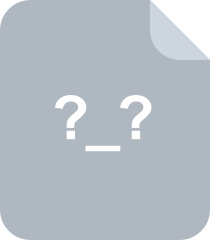
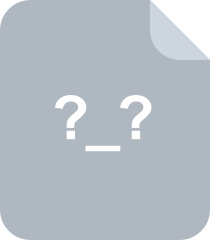
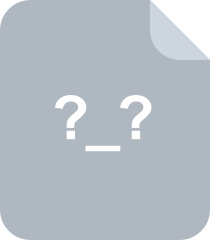
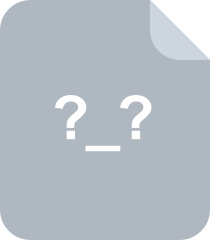
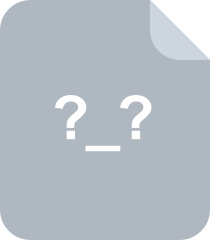
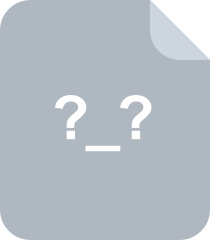
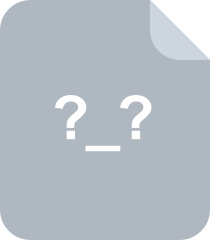
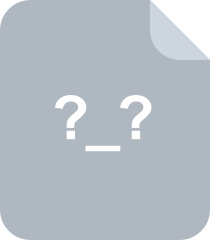
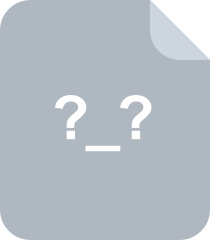
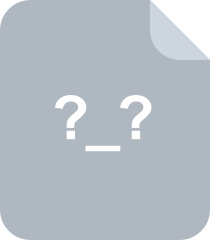
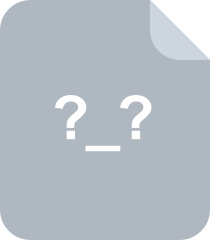
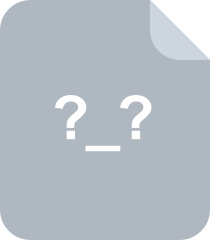
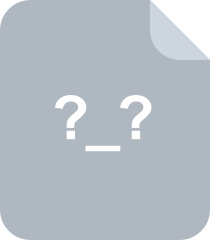
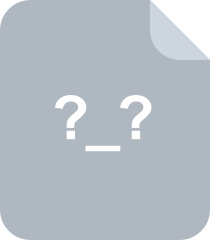
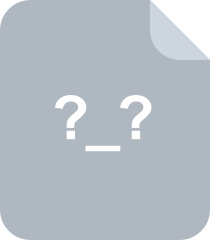
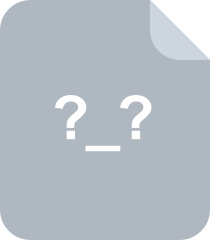
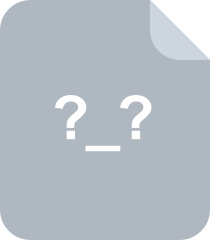
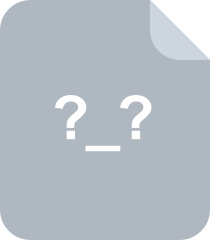
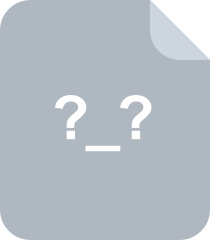
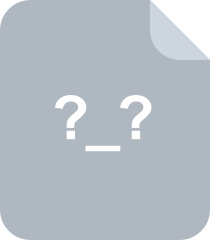
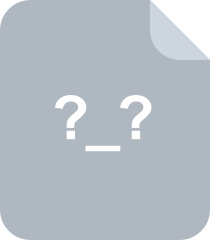
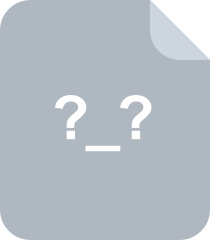
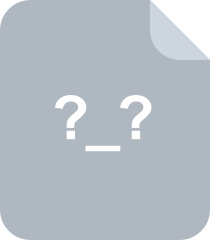
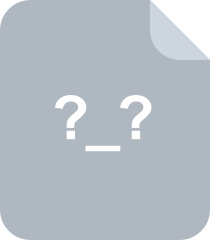
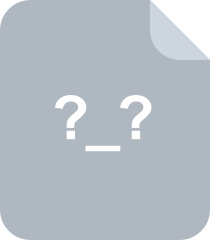
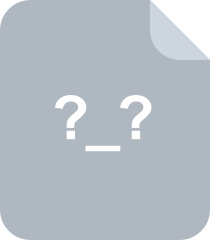
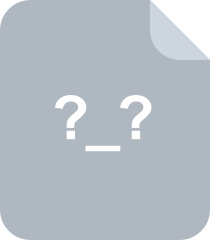
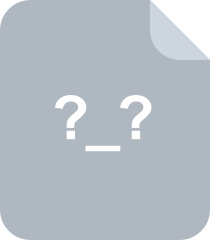
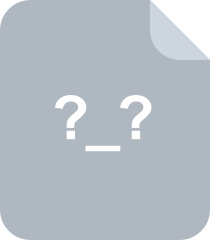
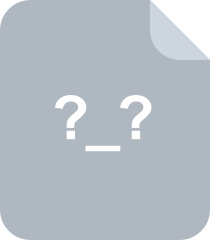
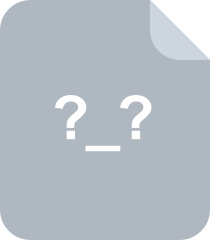
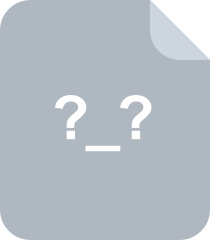
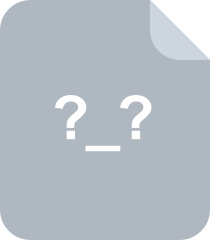
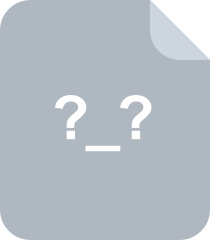
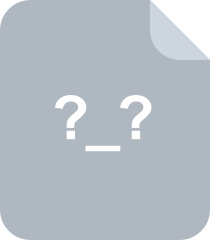
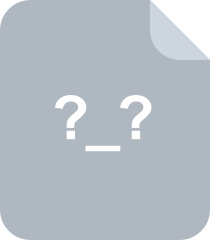
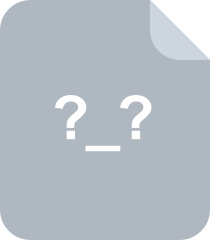
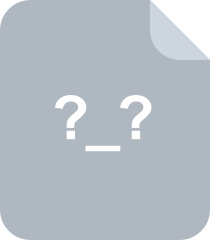
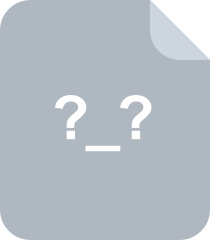
共 1075 条
- 1
- 2
- 3
- 4
- 5
- 6
- 11
资源评论
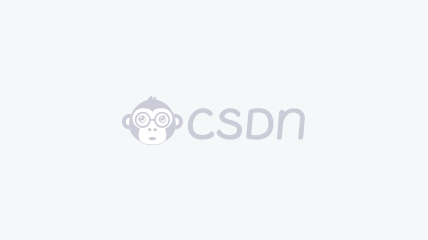
- SuperQDI2012-06-23只是把基本功能罗列了一下 没有具体项目实现
- 云醉红尘2013-12-29只是把基本功能罗列了一下
- lengyuecanqiu2011-10-18文如其名。。。内容很单调,不过很深入。。

xiaql110
- 粉丝: 0
- 资源: 1
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

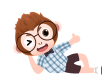
最新资源
- 离线OCR(此软件解压后双击即可运行, 免费)
- 公开整理-上市公司员工学历及工资数据(1999-2023年).xlsx
- 公开整理-上市公司员工学历及工资数据集(1999-2023年).dta
- GDAL-3.4.3-cp38-cp38-win-amd64.whl(GDAL轮子-免编译pip直接装,下载即用)
- 基于Java实现WIFI探针的商业大数据分析技术
- 抖音5.6版本、抖音短视频5.6版、抖音iOS5.6版、抖音ipa包5.6
- 图像处理领域、QT技术、架构,可直接借鉴
- 【源码+数据库】基于Spring Boot+Mybatis+Thymeleaf实现的宠物医院管理系统
- H5漂流瓶交友源码 社交漂流瓶H5源码+对接Z支付+视频教程
- 华为ICT大赛云赛道真题资源库.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


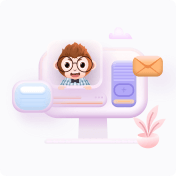
安全验证
文档复制为VIP权益,开通VIP直接复制
