JavaScript面向对象编程指南.pdf

### JavaScript面向对象编程指南 #### 一、面向对象编程(OOP)简介 面向对象编程(Object-Oriented Programming,简称OOP)是一种编程范式,它通过“对象”这一概念来组织代码结构,使得程序更加模块化、易于理解和维护。在JavaScript中实现面向对象编程,可以通过构造函数、原型链继承、类(class)等方式来实现。 #### 二、JavaScript中的对象与面向对象 在JavaScript中,几乎所有的数据类型都可以被视为对象,这为在JavaScript中进行面向对象编程提供了基础。对象是键值对的集合,其中键为字符串,而值则可以是任何类型的数据,包括函数、数组和其他对象等。 ##### 2.1 对象的创建方式 - **字面量方式**:直接使用 `{}` 来创建一个对象,并可以在大括号内定义属性。 ```javascript let person = { name: "Tom", age: 30 }; ``` - **构造函数方式**:通过定义构造函数并使用 `new` 关键字来实例化对象。 ```javascript function Person(name, age) { this.name = name; this.age = age; } let tom = new Person("Tom", 30); ``` - **类的方式**:ES6引入了类的概念,使得面向对象编程更加直观。 ```javascript class Person { constructor(name, age) { this.name = name; this.age = age; } } let tom = new Person("Tom", 30); ``` ##### 2.2 对象的属性与方法 对象不仅包含数据属性,还可以包含行为方法。这些方法通常用于描述对象的行为或与其他对象进行交互。 ```javascript class Person { constructor(name, age) { this.name = name; this.age = age; } introduce() { console.log(`Hello, my name is ${this.name} and I am ${this.age} years old.`); } } let tom = new Person("Tom", 30); tom.introduce(); // 输出: Hello, my name is Tom and I am 30 years old. ``` #### 三、继承与封装 面向对象编程中的另一个重要特性是继承,它允许创建一个新类来继承现有类的属性和方法。在JavaScript中,可以通过原型链或者类的继承机制来实现继承。 ##### 3.1 原型链继承 在JavaScript中,每个对象都有一个原型对象(`__proto__`),这个原型对象也可以有自己的原型,形成了一个原型链。当尝试访问一个对象的属性时,如果该对象本身没有这个属性,则会沿着原型链向上查找,直到找到该属性为止。 ```javascript function Person(name, age) { this.name = name; this.age = age; } Person.prototype.sayHello = function() { console.log(`Hello, my name is ${this.name}.`); }; function Student(name, age, grade) { Person.call(this, name, age); // 使用call调用父类构造函数 this.grade = grade; } // 设置Student的原型为Person的实例 Student.prototype = Object.create(Person.prototype); // 需要手动修正constructor指向 Student.prototype.constructor = Student; Student.prototype.study = function(subject) { console.log(`${this.name} is studying ${subject}.`); }; let student = new Student("Alice", 25, "Computer Science"); student.sayHello(); // 继承自Person student.study("Math"); // 学生特有的方法 ``` ##### 3.2 类的继承 ES6的类提供了一种更现代且直观的方式来实现继承。 ```javascript class Person { constructor(name, age) { this.name = name; this.age = age; } sayHello() { console.log(`Hello, my name is ${this.name}.`); } } class Student extends Person { constructor(name, age, grade) { super(name, age); this.grade = grade; } study(subject) { console.log(`${this.name} is studying ${subject}.`); } } let student = new Student("Alice", 25, "Computer Science"); student.sayHello(); // 继承自Person student.study("Math"); // 学生特有的方法 ``` #### 四、多态性 多态性是指允许不同子类型的对象对同一消息做出响应;即允许不同类的对象使用相同的接口。在JavaScript中,可以通过方法重写来实现多态性。 ```javascript class Shape { draw() { console.log("Drawing shape..."); } } class Circle extends Shape { draw() { console.log("Drawing circle..."); } } class Square extends Shape { draw() { console.log("Drawing square..."); } } let shapes = [new Circle(), new Square()]; shapes.forEach(shape => shape.draw()); ``` #### 五、封装 封装是指将数据和操作数据的方法绑定在一起,隐藏对象内部的具体实现细节。在JavaScript中,可以使用私有变量和私有方法来实现封装。 ```javascript class BankAccount { #balance; // 私有变量 constructor(initialBalance) { this.#balance = initialBalance; } deposit(amount) { this.#balance += amount; } withdraw(amount) { if (amount <= this.#balance) { this.#balance -= amount; } else { console.log("Insufficient funds."); } } getBalance() { return this.#balance; } } let account = new BankAccount(1000); account.deposit(500); console.log(account.getBalance()); // 输出: 1500 ``` 通过以上内容的学习,我们可以看出JavaScript作为一种动态语言,在实现面向对象编程方面具有非常强大的灵活性和扩展性。无论是通过传统的构造函数和原型链,还是使用ES6引入的类,都能方便地实现面向对象的设计模式,从而编写出更易于维护和扩展的应用程序。
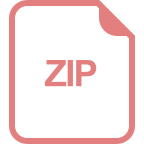
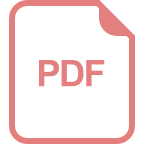
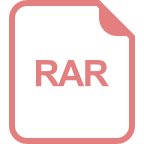
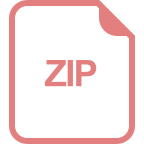
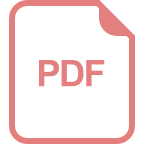
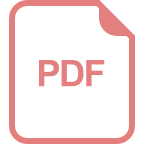
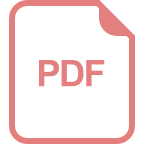
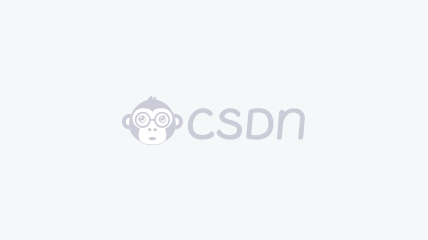
- 都疯了2018-09-15研究中 谢谢分享

- 粉丝: 2369
- 资源: 45
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

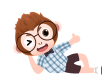
最新资源
- 运用python生成的跳跃的爱心
- 基于 Java 实现的 Socket.IO 服务器 实时 Java 框架.zip
- 基于 Ant 的 Java 项目示例.zip
- 各种字符串相似度和距离算法的实现Levenshtein、Jaro-winkler、n-Gram、Q-Gram、Jaccard index、最长公共子序列编辑距离、余弦相似度…….zip
- 运用python生成的跳跃的爱心
- 包括用 Java 编写的程序 欢迎您在此做出贡献!.zip
- (源码)基于QT框架的学生管理系统.zip
- 功能齐全的 Java Socket.IO 客户端库,兼容 Socket.IO v1.0 及更高版本 .zip
- 功能性 javascript 研讨会 无需任何库(即无需下划线),只需 ES5 .zip
- 分享Java相关的东西 - Java安全漫谈笔记相关内容.zip

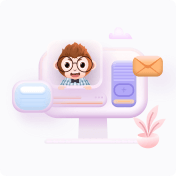
