在VB.NET 2008中,调用SQL Server存储过程是常见的数据库操作之一,它允许程序员通过预先定义好的数据库函数来执行复杂的数据处理任务。本示例将深入讲解如何在VB.NET中调用存储过程,包括参数传递、接收返回值、处理存储过程中的错误以及控制流程。 我们需要创建一个连接到SQL Server数据库的对象。这可以通过`SqlConnection`类实现,提供数据库的连接字符串。例如: ```csharp Dim connectionString As String = "Server=myServerAddress;Database=myDataBase;User Id=myUsername;Password=myPassword;" Dim connection As New SqlConnection(connectionString) connection.Open() ``` 接下来,我们定义一个`SqlCommand`对象,用于执行存储过程。在这个对象中,我们需要设置命令文本为存储过程的名称,并且指定连接对象: ```csharp Dim command As New SqlCommand("usp_MyProcedure", connection) command.CommandType = CommandType.StoredProcedure ``` 现在,我们可以通过`SqlParameter`类添加参数到`SqlCommand`对象,以传递给存储过程。假设存储过程接受两个输入参数`@param1`和`@param2`,我们可以这样设置: ```csharp Dim param1 As New SqlParameter("@param1", SqlDbType.Int) param1.Value = 123 command.Parameters.Add(param1) Dim param2 As New SqlParameter("@param2", SqlDbType.NVarChar, 50) param2.Value = "Example Value" command.Parameters.Add(param2) ``` 要接收存储过程的返回值,我们需要创建一个`SqlParameter`对象,设置其Direction属性为`ParameterDirection.Output`,并将其添加到命令参数列表中。假设存储过程有一个名为`@returnVal`的输出参数: ```csharp Dim returnParam As New SqlParameter("@returnVal", SqlDbType.Int) returnParam.Direction = ParameterDirection.Output command.Parameters.Add(returnParam) ``` 执行存储过程的方法是调用`ExecuteNonQuery`(如果存储过程不返回任何结果集)或`ExecuteReader`(如果返回结果集)。在这个例子中,我们将使用`ExecuteNonQuery`: ```csharp command.ExecuteNonQuery() ' 获取存储过程的返回值 Dim returnValue As Integer = CInt(returnParam.Value) ``` 对于存储过程中可能出现的错误,VB.NET提供了异常处理机制。我们可以使用`Try...Catch`语句捕获并处理异常: ```csharp Try command.ExecuteNonQuery() ' 获取存储过程的返回值 Dim returnValue As Integer = CInt(returnParam.Value) ' 处理返回值... Catch ex As SqlException Console.WriteLine("An error occurred during the execution of the stored procedure: " & ex.Message) End Try ' 关闭数据库连接 connection.Close() ``` 如果存储过程中遇到错误但希望继续执行其他操作,可以使用`TRY...CATCH...FINALLY`结构,并在`CATCH`块中进行错误处理,然后在`FINALLY`块中关闭连接。如果需要停止执行,可以在`CATCH`块中抛出一个新的异常或设置一个标志来指示程序应停止。 VB.NET 2008调用SQL Server存储过程涉及到创建数据库连接、定义命令对象、设置参数、执行存储过程并处理返回值和异常。这个过程允许开发者高效地与数据库交互,同时处理可能发生的错误情况。





























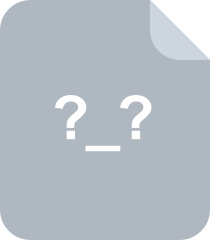
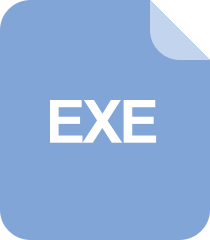
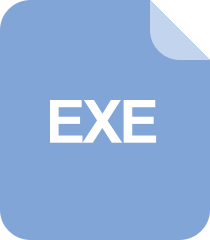
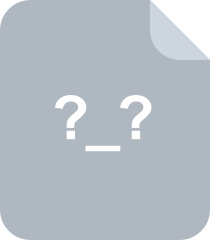
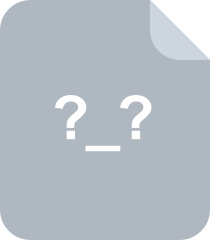

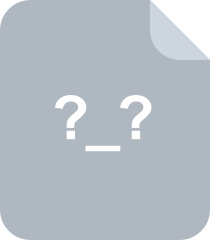
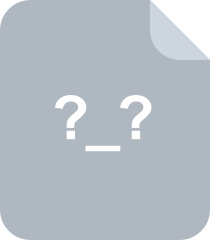


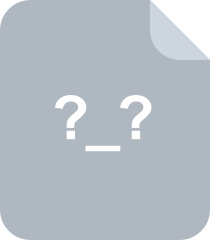
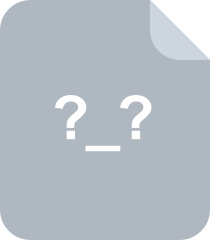
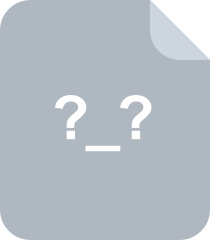
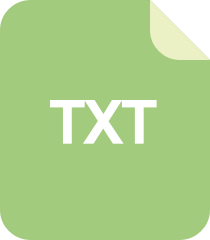
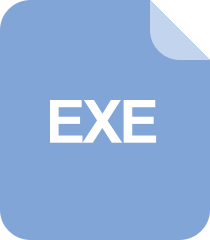
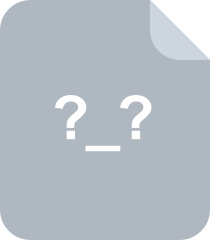

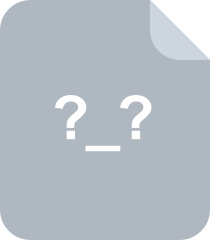
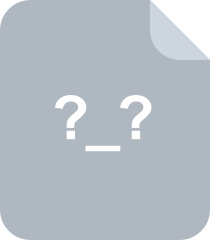

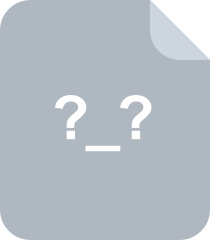

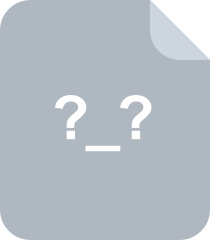
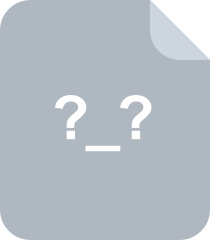
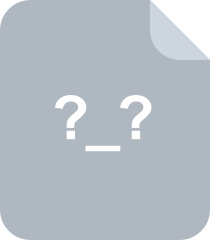
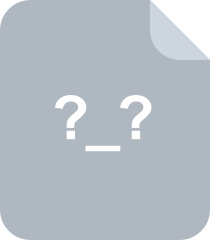
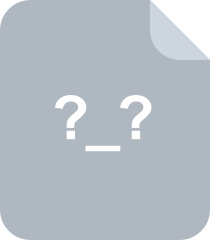
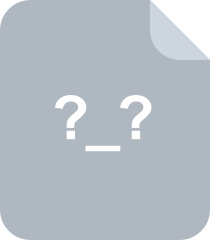
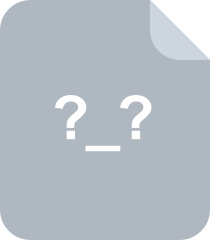
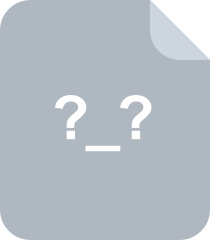
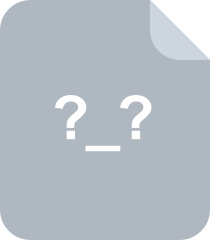
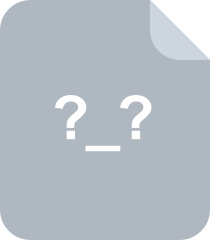
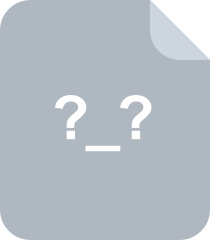
- 1

- 粉丝: 3
- 资源: 6
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

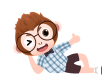
最新资源
- DSPF28335定时器0设置教程
- LabVIEW面向对象编程中简单工厂模式与RS485 Modbus通讯的应用实践
- Midterm project.zip
- PV-SOC-Gou-VSG.slx
- 车辆横向控制中模糊+滑膜轨迹跟踪算法的Matlab实现及应用
- 图神经架构搜索综述及广泛应用
- 辽宁沈阳沈阳-2025-01.xlsx
- 假新闻数据集,假新闻文本数据集,适用于机器学习,自然语言算法
- 2025自考人力资源管理人力资源统计学真题含答案
- uniapp封装目录树(支持多选、单选、全选、父子级联动、目录树过滤)
- nginx 部署二级路径应用
- 卷积神经网络在深度学习领域的研究进展及其在医学影像和心电图分类中的应用
- 51单片机两轴步进电机控制与LCD1602菜单界面操作指南
- 2-基于改进高斯模型的哈尔滨市PM2.5扩散问题实证分析-魏薇.pdf
- 基于YOLOv8深度学习的面部表情检测系统(带GUI界面)(Python源码+Pyqt5界面+25200多张标注好的数据集+安装使用教程+训练好的模型+评估指标曲线+演示图片视频),开箱即用
- 神经网络后门攻击与防御技术综述:原理、方法及未来发展方向

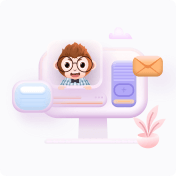

- 1
- 2
前往页