//#include "stdafx.h"
# include "cv.h"
# include "highgui.h"
# include <iostream>
# include "math.h"
using namespace std;
void main()
{
IplImage *mode = cvLoadImage("8.bmp");
CvMemStorage* storage=cvCreateMemStorage();
CvMemStorage* storage0=cvCreateMemStorage();
CvMemStorage* storage2=cvCreateMemStorage();
CvMemStorage* storage3=cvCreateMemStorage();
CvMemStorage* storage1=NULL;
CvSeq* firstContour=NULL;
CvArr * img_contours=NULL;
CvSeq* lines =NULL;
CvArr* points;
float rotation_angle;
CvPoint pt0;
//CvMat * map_matrix=NULL;
float PI = 3.141592653589793 ;
IplImage *dst_gray = cvCreateImage(cvGetSize(mode),8,1);//灰度图
cvCvtColor(mode ,dst_gray ,CV_BGR2GRAY);
//IplImage *dst0 =cvCreateImage(cvGetSize(dst_gray),8,1);//灰度图
IplImage* dst_image=cvCreateImage(cvGetSize(dst_gray),8,1);//灰度图
IplImage *copy_dst=cvCreateImage(cvGetSize(dst_gray),8,1);
IplImage *dst0=cvCreateImage(cvGetSize(dst_gray),8,1);
IplImage *dst=cvCreateImage(cvGetSize(dst_gray),8,1);
IplImage *dst1=cvCreateImage(cvGetSize(dst_gray),8,1);
IplImage *dst2=cvCreateImage(cvGetSize(dst_gray),8,1);
IplImage *dst3=cvCreateImage(cvGetSize(dst_gray),8,1);
IplImage *dst20=cvCreateImage(cvGetSize(dst_gray),8,1);
cvSmooth(dst_gray ,dst1,CV_GAUSSIAN,3,0,0);
cvThreshold(dst1,dst3,80,255, CV_THRESH_BINARY);
//图像进行形态学操作 闭运算
//cvCanny(dst0,dst1,20,10,3);
//cvErode(dst1,dst2,NULL,2);
//cvDilate(dst1,dst2,NULL,1);
//cvMorphologyEx(dst2,dst20,0,CV_SHAPE_RECT,CV_MOP_CLOSE,1);
//cvCanny(dst1,dst3,10,20,3);
//cvCanny(dst2,dst3,10,15,5);
int max_length=0;
cvFindContours(dst3, storage, &firstContour,sizeof(CvContour),CV_RETR_EXTERNAL,CV_CHAIN_APPROX_NONE);
CvSeq * Contour=NULL;
//cvCloneSeq(firstContour,storage3);
for (CvSeq *c=firstContour;c!=NULL;c=c->h_next )
{
int arc_length=cvArcLength(c,CV_WHOLE_SEQ,-1);
if (arc_length>max_length)
{ // cvClearSeq(Contour);
max_length=arc_length;
//Contour=cvCloneSeq(c,storage2);
Contour=c;
}
}
cvDrawContours( dst3,Contour,CV_RGB(255,255,255), CV_RGB(255,255,255),0,1,8,cvPoint(0,0));
// cvDrawContours(img_contours,firstContour,CV_RGB(255,255,255), CV_RGB(0,255,0),0,1,8,cvPoint(0,0));
/*
int count = firstContour->total;
/*CvPoint *p = NULL;
for (int i=0;i<count;++i)
{
p=(CvPoint *)cvGetSeqElem((CvSeq *)storage,i);
cout << p->x << ",";
cout << p->y << "\n";
//printf("(%d,%d)\n",p->x,p->y);
}
CvSeqReader reader = {0};
cvStartReadSeq (firstContour,&reader,0);
CvPoint *r=NULL;
for(int j=0;j<count;j++)
{
r = CV_GET_SEQ_ELEM( CvPoint, firstContour, j );
printf("(%d,%d),",r->x,r->y);
}
*/
/*CvPoint * PointArray = (CvPoint *)malloc(count * sizeof(CvPoint));
cvCvtSeqToArray(firstContour, PointArray, CV_WHOLE_SEQ);
//for(int i=0;i<count;i++)
// printf("(%d,%d),",PointArray[i]);
CvSeq* ptseq = cvCreateSeq( CV_SEQ_KIND_GENERIC|CV_32SC2, sizeof(CvContour),
sizeof(CvPoint), storage );
CvSeqReader reader = {0};
cvStartReadSeq (firstContour,&reader,0);
for(int i = 0; i < count; i++ )
{ CV_READ_SEQ_ELEM(PointArray[i],reader);
pt0.x = PointArray[i].x;
pt0.y = PointArray[i].y;
cvSeqPush( ptseq, &pt0 );
}
*/
CvPoint temp_point;
CvPoint pt1,pt2,pt3,pt4;
// 初始化轮廓点的坐标,左上角为pt1,左下角为pt2
pt2.x=0;
pt2.y=0;
pt1.x=0;
pt1.y=0;
//求得最下外接矩形
CvBox2D box=cvMinAreaRect2( Contour,0);
double angle = box.angle*CV_PI/180;
float a = (float)cos(angle)*0.5f;
float b = (float)sin(angle)*0.5f;
//三角几何变换得出
pt1.x = box.center.x - a*box.size.height - b*box.size.width;
pt1.y = box.center.y + b*box.size.height - a*box.size.width;
pt2.x = box.center.x + a*box.size.height - b*box.size.width;
pt2.y = box.center.y - b*box.size.height - a*box.size.width;
pt3.x = 2*box.center.x - pt1.x;
pt3.y = 2*box.center.y - pt1.y;
pt4.x = 2*box.center.x - pt2.x;
pt4.y = 2*box.center.y - pt2.y;
cvLine( dst_image, pt1, pt2,CV_RGB(255,255,255), 2, 8, 0 );
cvLine( dst_image, pt2, pt3,CV_RGB(255,255,255), 2, 8, 0 );
cvLine( dst_image, pt3, pt4,CV_RGB(255,255,255), 2, 8, 0 );
cvLine( dst_image, pt4, pt1,CV_RGB(255,255,255), 2, 8, 0 );
cvShowImage("box",dst_image);
// 求得最左边直线与X轴夹角,反正切求出弧度
float theta=atan2(float(pt1.y-pt2.y),float(pt1.x-pt2.x));
float angle0=theta*180/PI;
if(pt1.y<box.center.y&&pt2.y<box.center.y)
rotation_angle=180.0-angle0;
if (pt1.y>box.center.y||pt2.y>box.center.y)
rotation_angle=90.0-angle0;
float m[6];
CvMat mat=cvMat(2,3,CV_32FC1,m);
m[0] = (float)(1*cos(rotation_angle*CV_PI/180.));
m[1] = (float)(1*sin(rotation_angle*CV_PI/180.));
m[2] = box.center.x;
m[3] = -m[1];
m[4] = m[0];
m[5] = box.center.y;
// cv2DRotationMatrix(box.center,rotation_angle,1,&mat);
cvGetQuadrangleSubPix( dst_gray, copy_dst,&mat);
cvShowImage("name",dst_gray);
cvShowImage("name00",dst3);
cvShowImage("name000",copy_dst);
cvShowImage("name0",mode);
// cvDestroyWindow("123.bmp");
// cvReleaseImage(&dst);
// cvReleaseImage(&dst);
// cvReleaseImage(&mode);
}
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
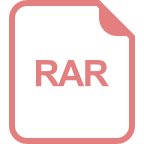
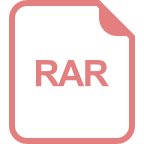
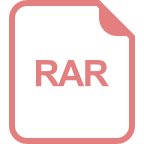
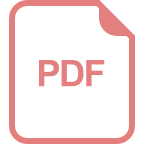
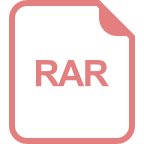
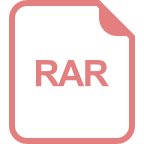
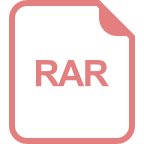
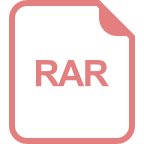
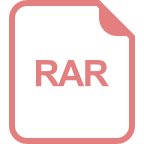
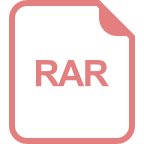
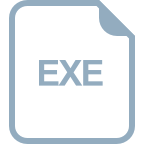
收起资源包目录


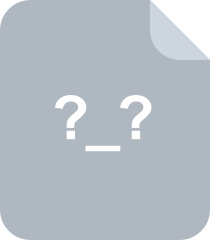
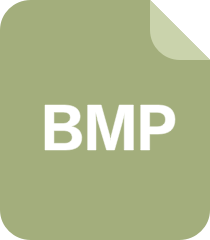
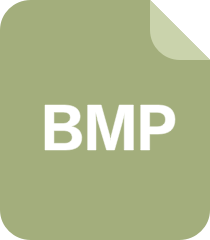
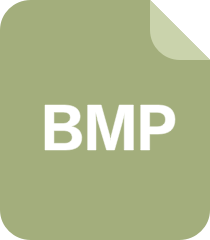
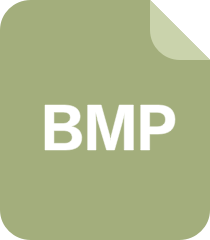

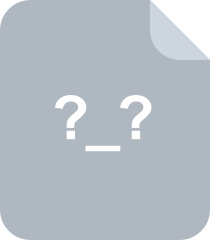
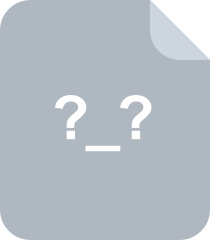
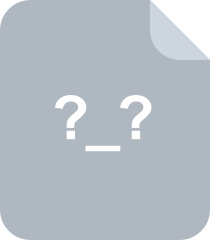
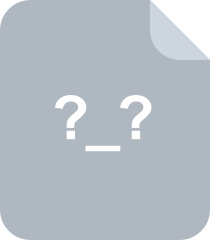
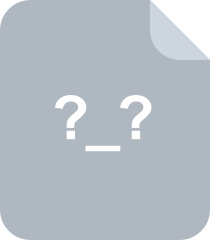
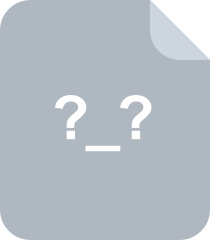
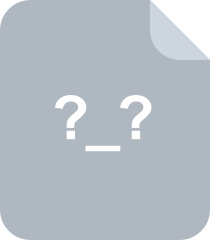
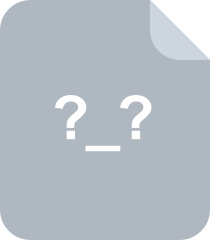
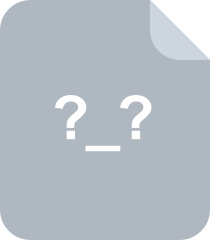
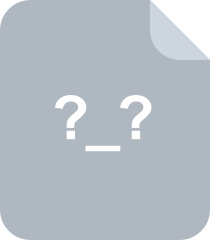
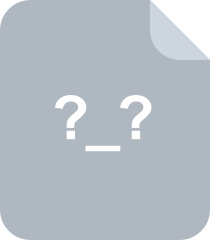
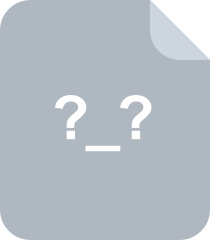
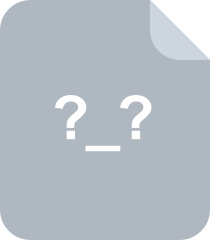
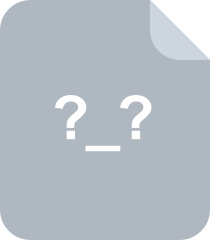
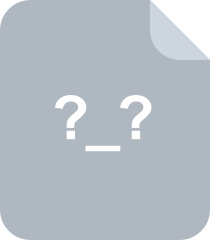
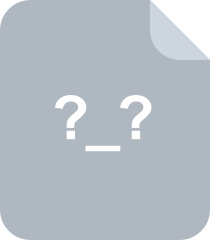
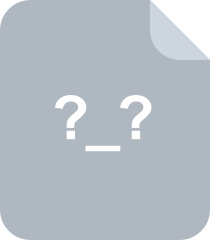
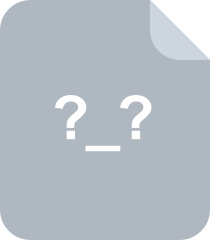
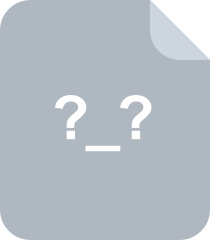
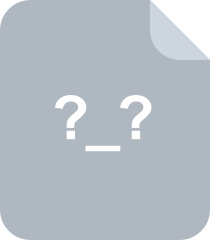
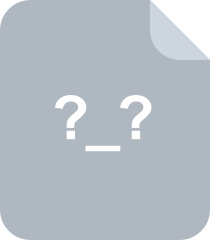
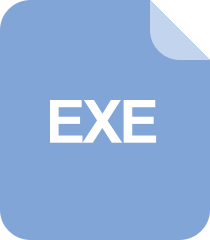
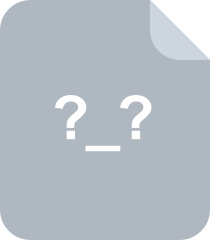
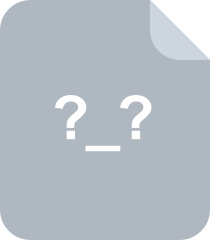
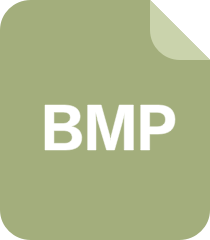
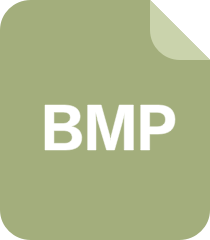
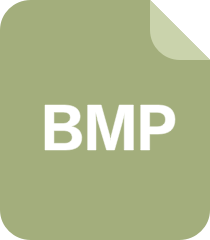
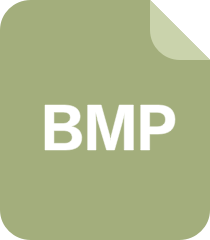
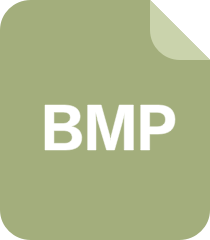
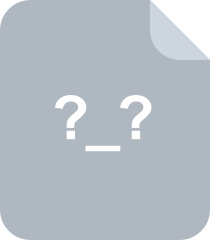
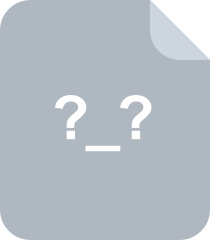
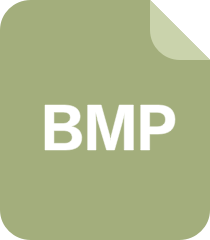
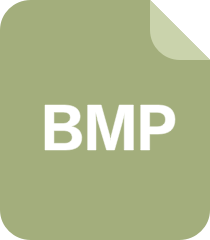
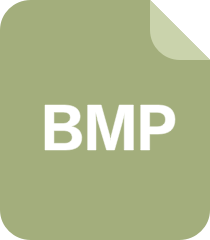
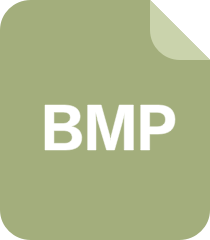
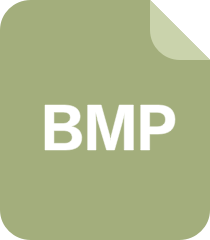
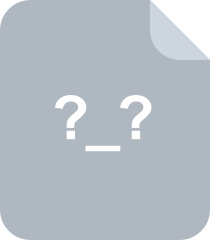
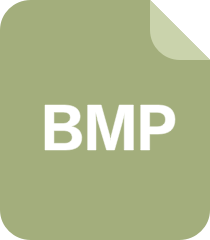
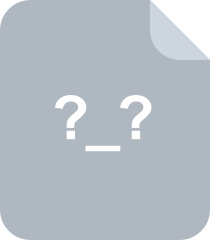
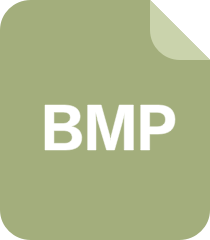
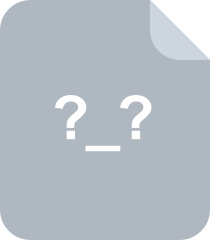
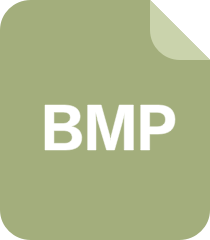
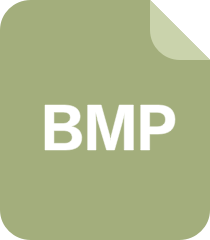

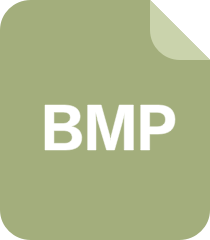
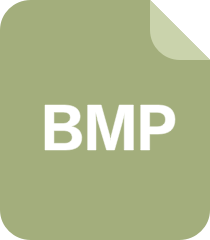
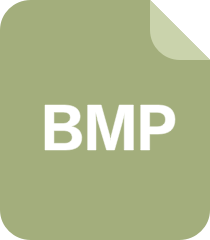
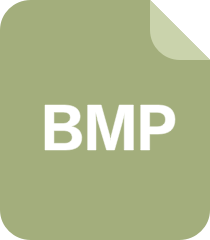
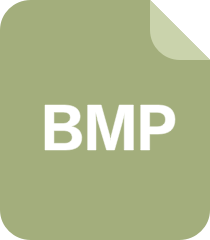
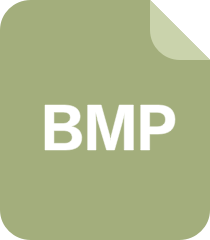
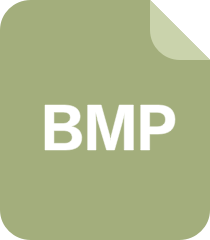
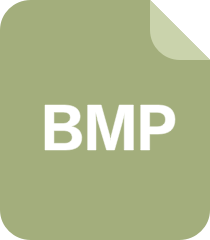
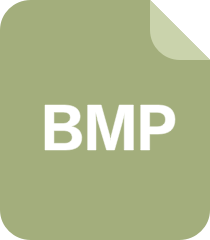
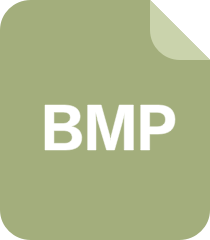
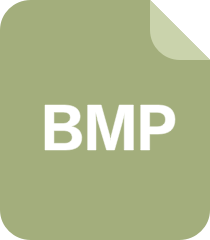
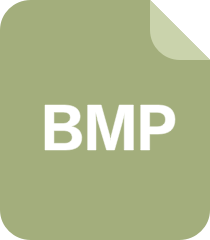
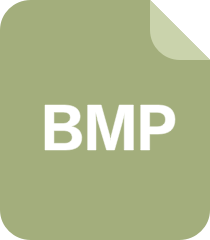
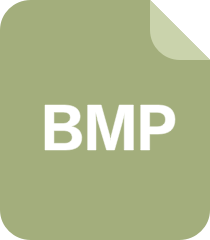
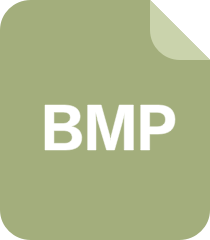
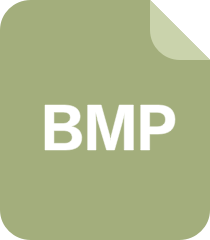
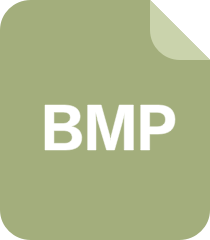
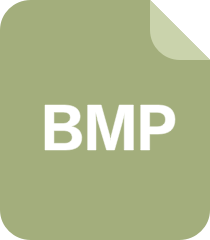
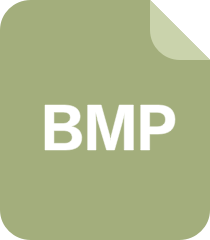
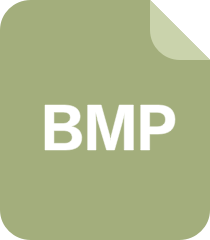
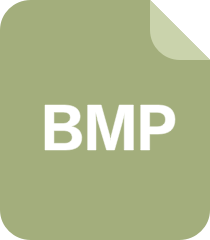
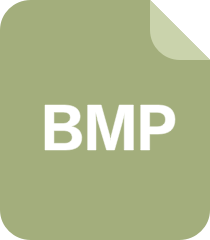
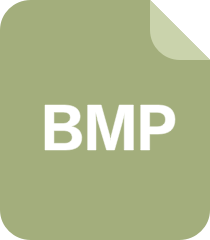
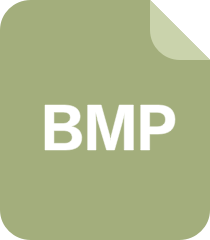
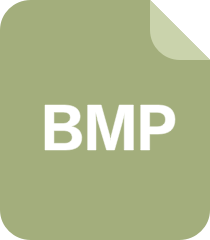
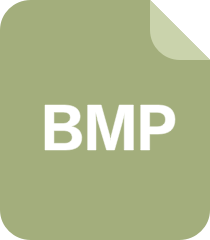
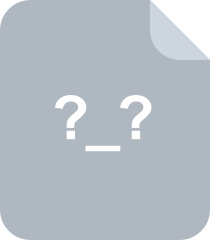
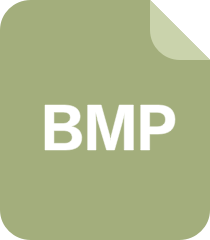
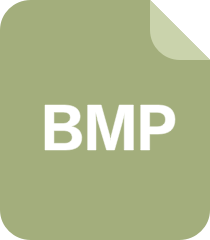
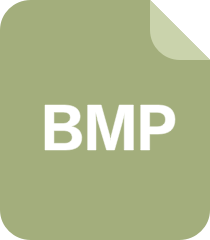
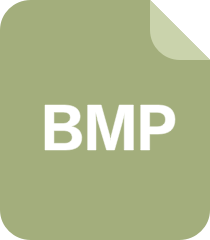
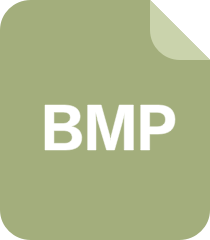
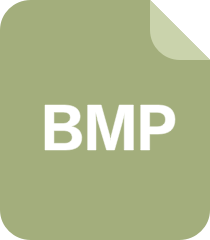
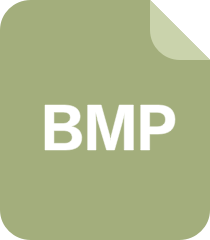
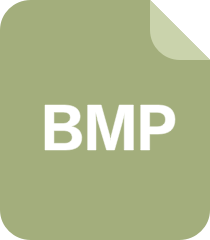
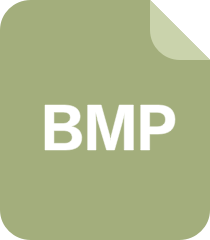
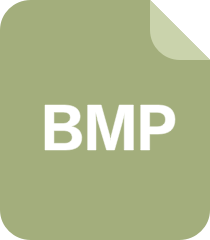
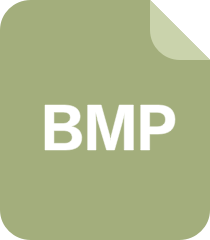
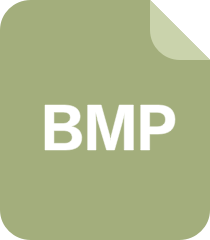
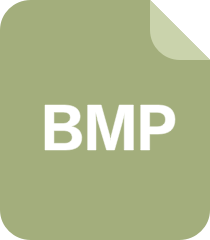
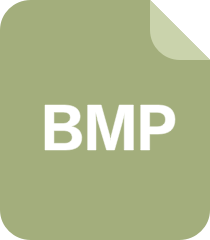
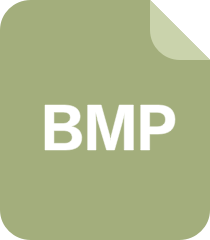
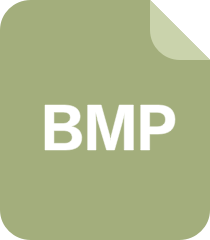
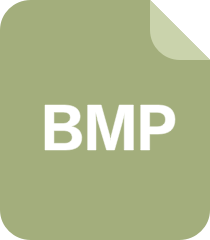
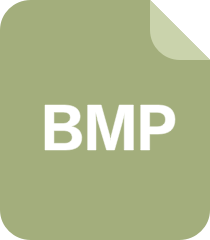
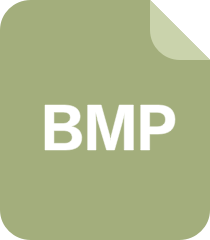
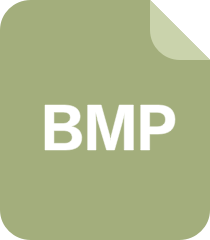
共 95 条
- 1

xiaohuoyuai
- 粉丝: 0
- 资源: 12
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

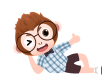
安全验证
文档复制为VIP权益,开通VIP直接复制

- 1
- 2
- 3
- 4
- 5
- 6
前往页