/*
* Copyright (c) 2013-2022 Andreas Unterweger
*
* This file is part of FFmpeg.
*
* FFmpeg is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* FFmpeg is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with FFmpeg; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA
*/
/**
* @file audio transcoding to MPEG/AAC API usage example
* @example transcode_aac.c
*
* Convert an input audio file to AAC in an MP4 container. Formats other than
* MP4 are supported based on the output file extension.
* @author Andreas Unterweger (dustsigns@gmail.com)
*/
#include <stdio.h>
#include "libavformat/avformat.h"
#include "libavformat/avio.h"
#include "libavcodec/avcodec.h"
#include "libavutil/audio_fifo.h"
#include "libavutil/avassert.h"
#include "libavutil/avstring.h"
#include "libavutil/channel_layout.h"
#include "libavutil/frame.h"
#include "libavutil/opt.h"
#include "libswresample/swresample.h"
/* The output bit rate in bit/s */
#define OUTPUT_BIT_RATE 96000
/* The number of output channels */
#define OUTPUT_CHANNELS 2
/**
* Open an input file and the required decoder.
* @param filename File to be opened
* @param[out] input_format_context Format context of opened file
* @param[out] input_codec_context Codec context of opened file
* @return Error code (0 if successful)
*/
static int open_input_file(const char *filename,
AVFormatContext **input_format_context,
AVCodecContext **input_codec_context)
{
AVCodecContext *avctx;
const AVCodec *input_codec;
const AVStream *stream;
int error;
/* Open the input file to read from it. */
if ((error = avformat_open_input(input_format_context, filename, NULL,
NULL)) < 0) {
fprintf(stderr, "Could not open input file '%s' (error '%s')\n",
filename, av_err2str(error));
*input_format_context = NULL;
return error;
}
/* Get information on the input file (number of streams etc.). */
if ((error = avformat_find_stream_info(*input_format_context, NULL)) < 0) {
fprintf(stderr, "Could not open find stream info (error '%s')\n",
av_err2str(error));
avformat_close_input(input_format_context);
return error;
}
/* Make sure that there is only one stream in the input file. */
if ((*input_format_context)->nb_streams != 1) {
fprintf(stderr, "Expected one audio input stream, but found %d\n",
(*input_format_context)->nb_streams);
avformat_close_input(input_format_context);
return AVERROR_EXIT;
}
stream = (*input_format_context)->streams[0];
/* Find a decoder for the audio stream. */
if (!(input_codec = avcodec_find_decoder(stream->codecpar->codec_id))) {
fprintf(stderr, "Could not find input codec\n");
avformat_close_input(input_format_context);
return AVERROR_EXIT;
}
/* Allocate a new decoding context. */
avctx = avcodec_alloc_context3(input_codec);
if (!avctx) {
fprintf(stderr, "Could not allocate a decoding context\n");
avformat_close_input(input_format_context);
return AVERROR(ENOMEM);
}
/* Initialize the stream parameters with demuxer information. */
error = avcodec_parameters_to_context(avctx, stream->codecpar);
if (error < 0) {
avformat_close_input(input_format_context);
avcodec_free_context(&avctx);
return error;
}
/* Open the decoder for the audio stream to use it later. */
if ((error = avcodec_open2(avctx, input_codec, NULL)) < 0) {
fprintf(stderr, "Could not open input codec (error '%s')\n",
av_err2str(error));
avcodec_free_context(&avctx);
avformat_close_input(input_format_context);
return error;
}
/* Set the packet timebase for the decoder. */
avctx->pkt_timebase = stream->time_base;
/* Save the decoder context for easier access later. */
*input_codec_context = avctx;
return 0;
}
/**
* Open an output file and the required encoder.
* Also set some basic encoder parameters.
* Some of these parameters are based on the input file's parameters.
* @param filename File to be opened
* @param input_codec_context Codec context of input file
* @param[out] output_format_context Format context of output file
* @param[out] output_codec_context Codec context of output file
* @return Error code (0 if successful)
*/
static int open_output_file(const char *filename,
AVCodecContext *input_codec_context,
AVFormatContext **output_format_context,
AVCodecContext **output_codec_context)
{
AVCodecContext *avctx = NULL;
AVIOContext *output_io_context = NULL;
AVStream *stream = NULL;
const AVCodec *output_codec = NULL;
int error;
/* Open the output file to write to it. */
if ((error = avio_open(&output_io_context, filename,
AVIO_FLAG_WRITE)) < 0) {
fprintf(stderr, "Could not open output file '%s' (error '%s')\n",
filename, av_err2str(error));
return error;
}
/* Create a new format context for the output container format. */
if (!(*output_format_context = avformat_alloc_context())) {
fprintf(stderr, "Could not allocate output format context\n");
return AVERROR(ENOMEM);
}
/* Associate the output file (pointer) with the container format context. */
(*output_format_context)->pb = output_io_context;
/* Guess the desired container format based on the file extension. */
if (!((*output_format_context)->oformat = av_guess_format(NULL, filename,
NULL))) {
fprintf(stderr, "Could not find output file format\n");
goto cleanup;
}
if (!((*output_format_context)->url = av_strdup(filename))) {
fprintf(stderr, "Could not allocate url.\n");
error = AVERROR(ENOMEM);
goto cleanup;
}
/* Find the encoder to be used by its name. */
if (!(output_codec = avcodec_find_encoder(AV_CODEC_ID_AAC))) {
fprintf(stderr, "Could not find an AAC encoder.\n");
goto cleanup;
}
/* Create a new audio stream in the output file container. */
if (!(stream = avformat_new_stream(*output_format_context, NULL))) {
fprintf(stderr, "Could not create new stream\n");
error = AVERROR(ENOMEM);
goto cleanup;
}
avctx = avcodec_alloc_context3(output_codec);
if (!avctx) {
fprintf(stderr, "Could not allocate an encoding context\n");
error = AVERROR(ENOMEM);
goto cleanup;
}
/* Set the basic encoder parameters.
* The input file's sample rate is used to avoid a sample rate conversion. */
av_channel_layout_default(&avctx->ch_layout, OUTPUT_CHANNELS);
avctx->sample_rate = input_codec_context->sample_rate;
avctx->sample_fmt = output_codec->sample_fmts[0];
avctx->bit_rate = OUTPUT_BIT_RATE;
/* Set the sample rate for the container. */
stream->time_base.den = input_codec_context->sample_rate;
stream->time_base.num = 1;
/* Some container formats (like MP4) require global headers to be present.
* Mark the encoder so that it behaves accordingly. */
没有合适的资源?快使用搜索试试~ 我知道了~
ffmpeg-6.1.2-full-build-with-mingw32
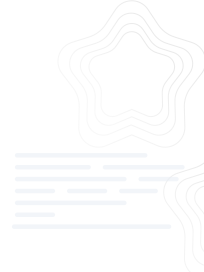
共303个文件
h:144个
html:31个
c:24个

需积分: 5 0 下载量 145 浏览量
2024-09-12
00:44:32
上传
评论
收藏 18.34MB 7Z 举报
温馨提示
ffmpeg-6.1.2编译支持x264和x265 x86版本 SDL2.dll avcodec-60.dll avcodec.lib avdevice-60.dll avdevice.lib avfilter-9.dll avfilter.lib avformat-60.dll avformat.lib avutil-58.dll avutil.lib ffmpeg.exe ffplay.exe ffprobe.exe libgcc_s_dw2-1.dll libx264-164.dll libx265-209.dll postproc-57.dll postproc.lib swresample-4.dll swresample.lib swscale-7.dll swscale.l
资源推荐
资源详情
资源评论
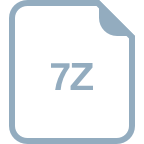
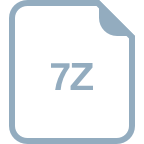
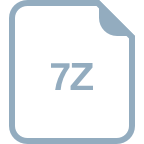
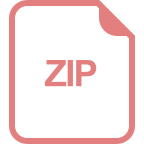
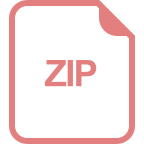
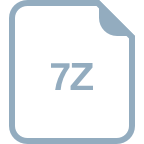
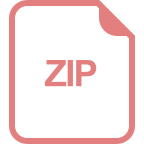
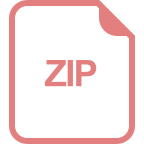
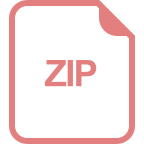
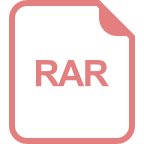
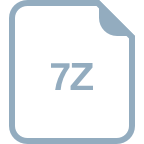
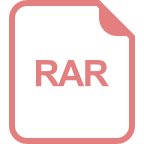
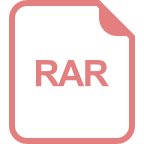
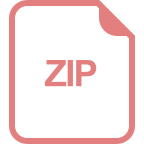
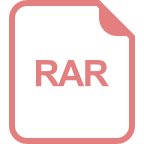
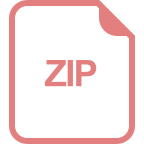
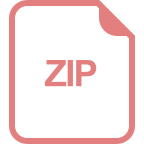
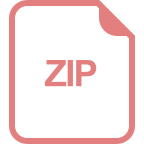
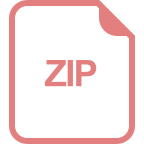
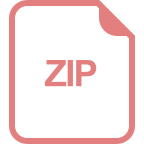
收起资源包目录

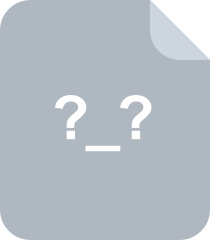
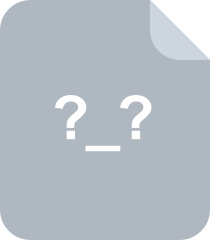
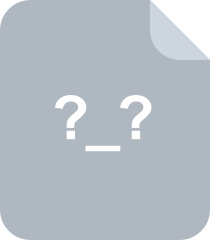
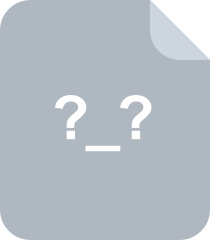
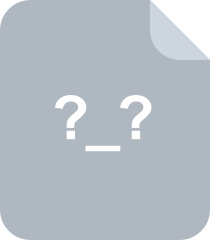
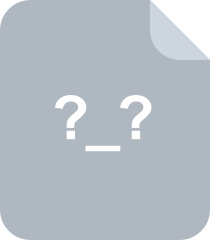
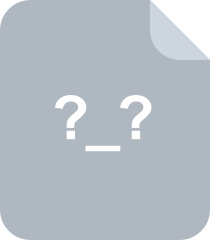
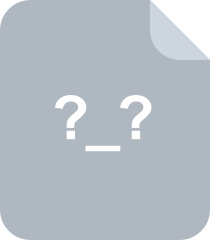
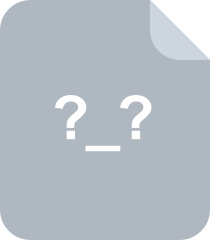
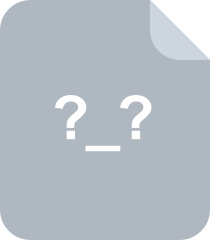
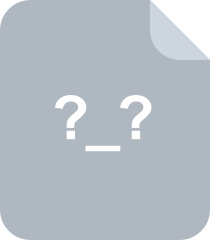
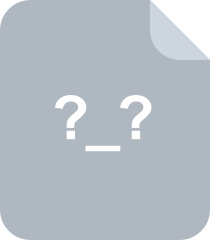
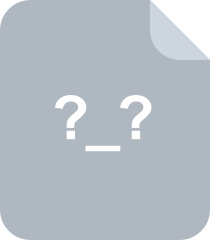
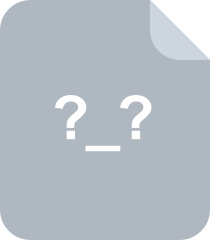
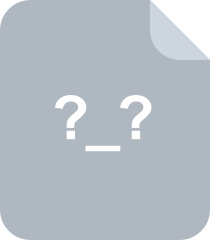
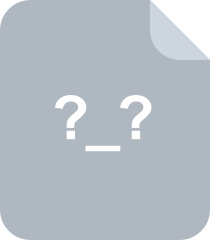
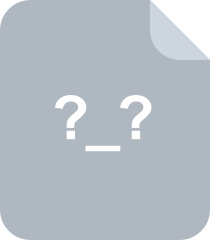
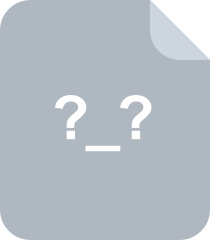
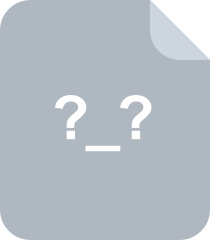
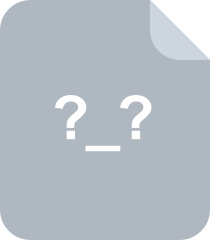
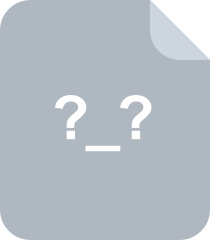
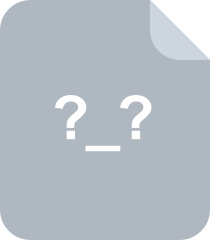
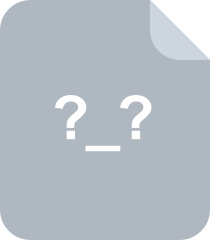
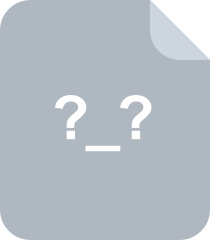
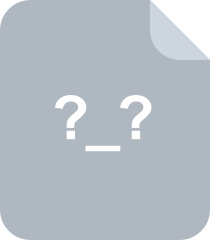
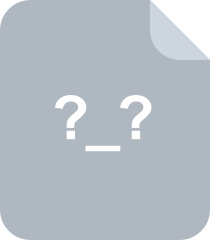
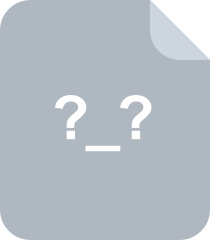
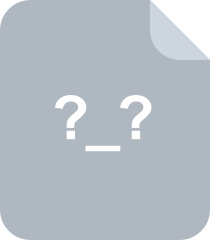
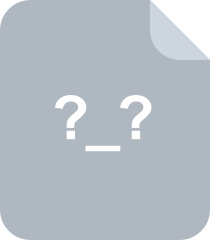
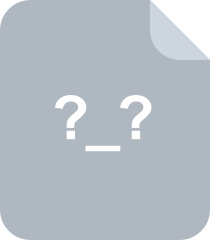
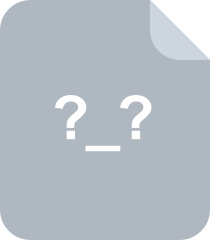
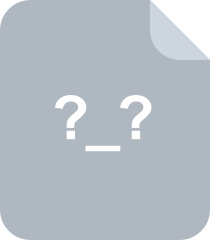
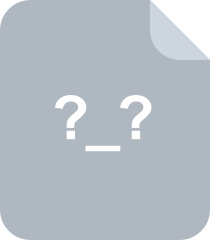
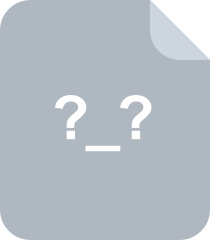
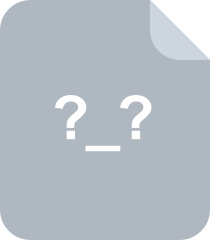
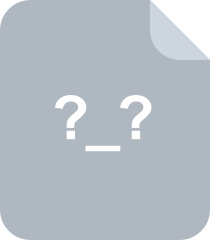
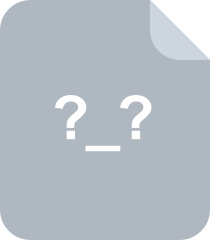
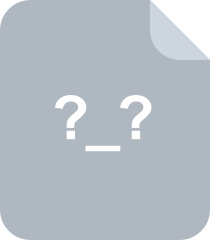
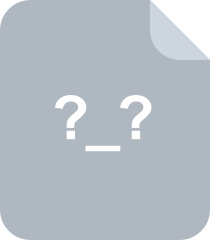
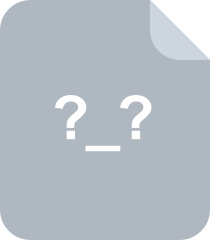
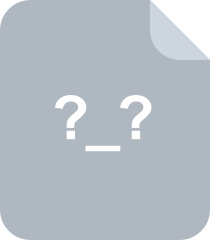
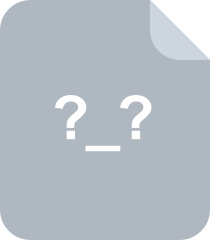
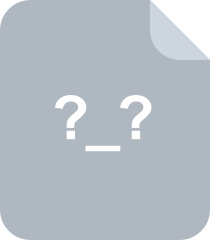
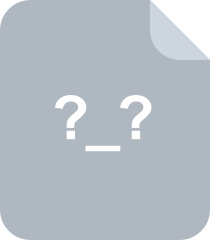
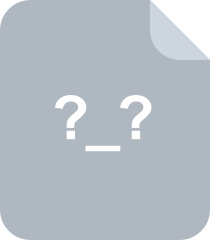
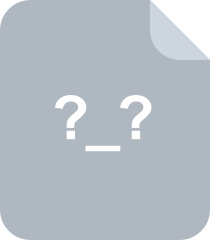
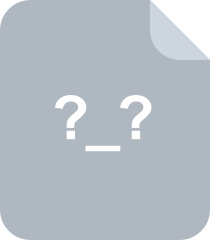
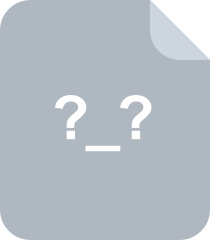
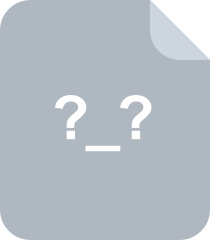
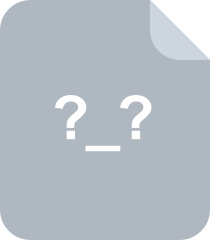
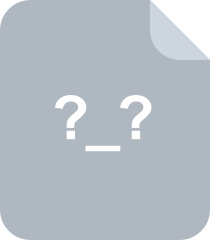
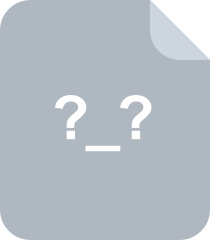
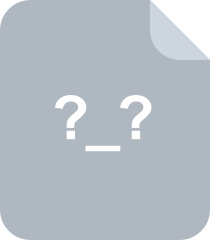
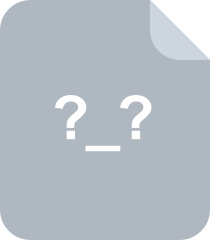
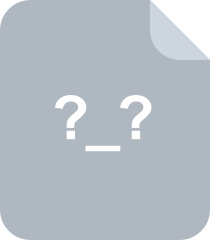
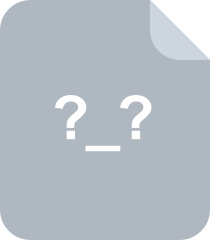
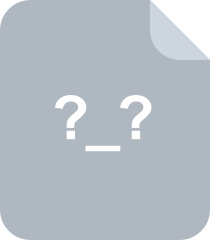
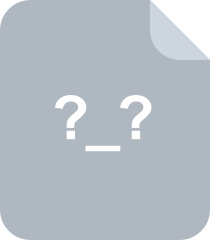
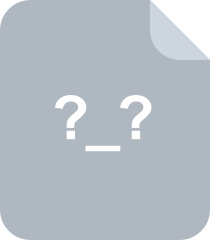
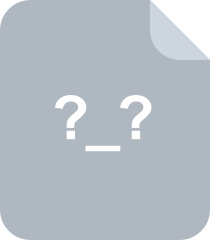
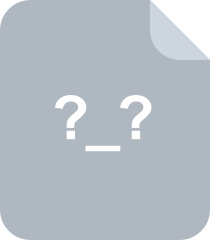
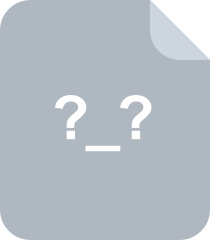
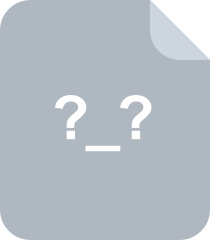
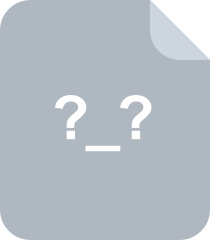
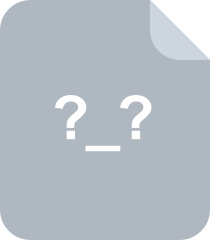
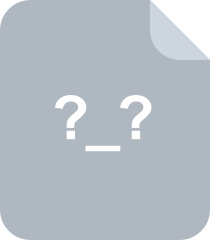
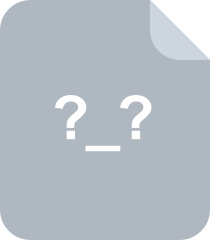
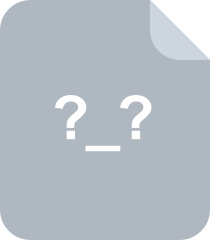
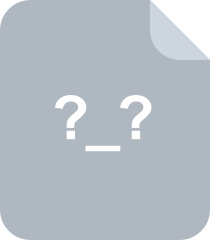
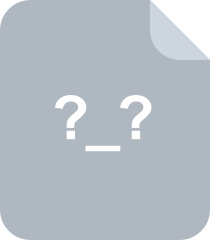
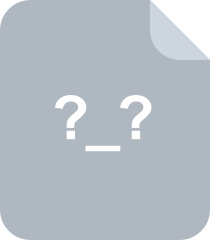
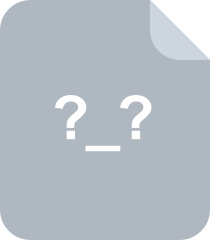
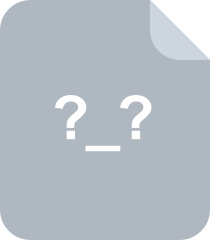
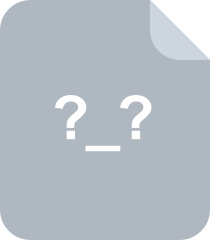
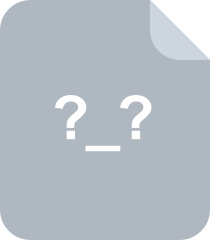
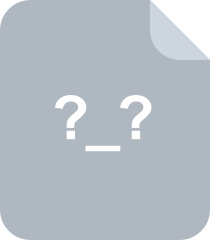
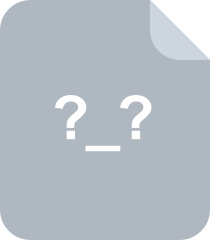
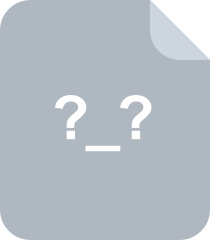
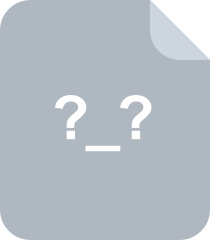
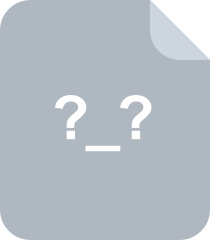
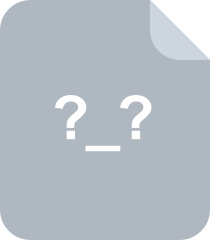
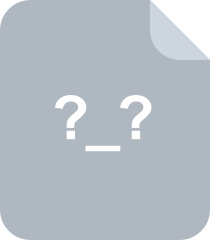
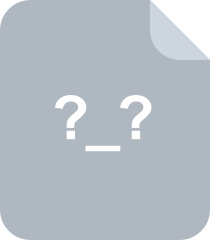
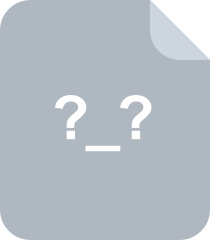
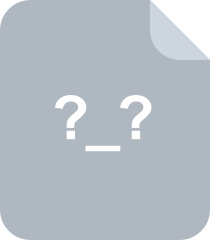
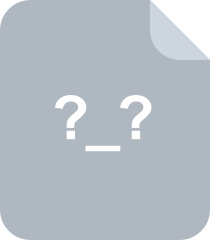
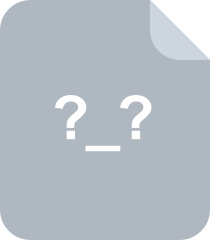
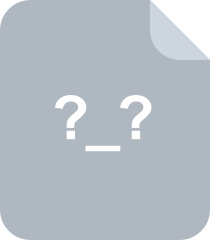
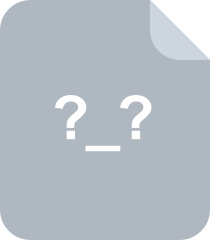
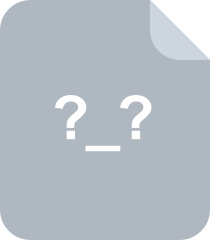
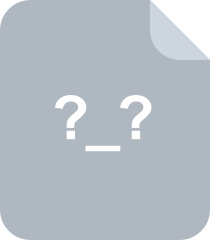
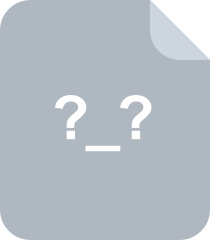
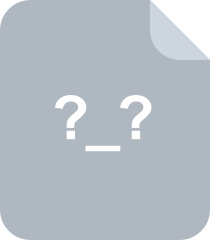
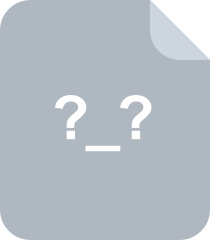
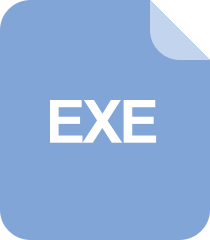
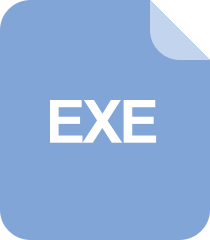
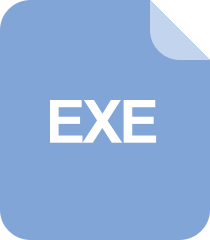
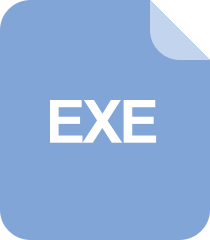
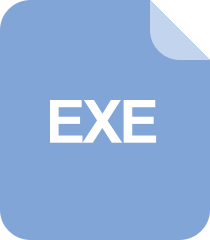
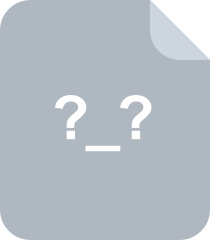
共 303 条
- 1
- 2
- 3
- 4
资源评论
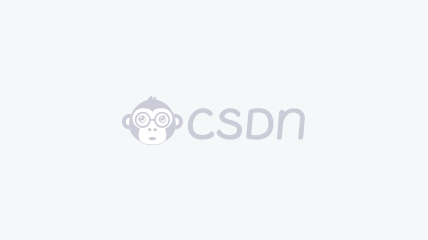

摸鱼儿v
- 粉丝: 5
- 资源: 44
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

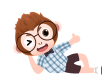
最新资源
- 基于Pygame库实现新年烟花效果的Python代码
- 浪漫节日代码 - 爱心代码、圣诞树代码
- 睡眠健康与生活方式数据集,睡眠和生活习惯关联分析()
- 国际象棋检测10-YOLO(v5至v9)、COCO、CreateML、Paligemma数据集合集.rar
- 100个情侣头像,唯美手绘情侣头像
- 自动驾驶不同工况避障模型(perscan、simulink、carsim联仿),能够避开预设的(静态)障碍物
- 使用Python和Pygame实现圣诞节动画效果
- 数据分析-49-客户细分-K-Means聚类分析
- 车辆轨迹自适应预瞄跟踪控制和自适应p反馈联合控制,自适应预苗模型和基于模糊p控制均在simulink中搭建 个人觉得跟踪效果相比模糊pid效果好很多,轨迹跟踪过程,转角控制平滑自然,车速在36到72
- 企业可持续发展性数据集,ESG数据集,公司可持续发展性数据(可用于多种企业可持续性研究场景)
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


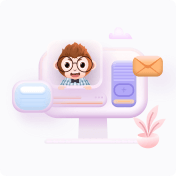
安全验证
文档复制为VIP权益,开通VIP直接复制
