### 做网站需要的技术经验和元素 在进行网站开发时,掌握一定的技术经验和元素是非常重要的。本文将基于提供的部分内容,详细介绍网站开发中的关键技术点:如何使用ASP.NET处理图像与SQL Server数据库之间的交互,并且简要提及如何在WinForm应用程序中实现类似的功能。 #### ASP.NET与SQL Server中的图像处理 **1. 上传图像到SQL Server** 在SQL Server数据库中创建一个表用于存储图像数据: ```sql CREATE TABLE Test ( Id INT IDENTITY (1, 1), FImage IMAGE ) ``` 接下来,创建一个存储过程`UpdateImage`,用于插入图像数据: ```sql CREATE PROCEDURE UpdateImage ( @UpdateImage Image ) AS INSERT INTO Test (FImage) VALUES (@UpdateImage) ``` 在ASP.NET页面中,通过以下步骤实现图像的上传: - 创建一个`<input type="file">`控件用于选择文件。 - 创建一个按钮控件,触发图像上传事件。 - 在`UpPhoto.aspx.cs`文件中编写`btnAdd_Click`方法来处理上传逻辑。 ```csharp private void btnAdd_Click(object sender, System.EventArgs e) { // 将上传的图像转换为byte数组 HttpPostedFile upPhoto = UpPhoto.PostedFile; int upPhotoLength = upPhoto.ContentLength; byte[] photoArray = new Byte[upPhotoLength]; Stream photoStream = upPhoto.InputStream; photoStream.Read(photoArray, 0, upPhotoLength); // 连接到数据库 SqlConnection conn = new SqlConnection("DataSource=localhost;Database=test;UserId=sa;Pwd=sa"); SqlCommand cmd = new SqlCommand("UpdateImage", conn); cmd.CommandType = CommandType.StoredProcedure; cmd.Parameters.Add("@UpdateImage", SqlDbType.Image); cmd.Parameters["@UpdateImage"].Value = photoArray; // 执行操作 conn.Open(); cmd.ExecuteNonQuery(); conn.Close(); } ``` **2. 从SQL Server读取并显示图像** 为了显示从数据库中获取的图像,可以在ASP.NET页面中添加一个`<asp:image>`控件,并设置其`ImageUrl`属性指向一个名为`ShowPhoto.aspx`的页面。 在`ShowPhoto.aspx`页面中编写代码来实现图像数据的读取和输出: ```csharp private void Page_Load(object sender, System.EventArgs e) { if (!Page.IsPostBack) { SqlConnection conn = new SqlConnection("DataSource=localhost;Database=test;UserId=sa;Pwd=sa"); string strSql = "SELECT * FROM Test WHERE Id = 2"; // 获取id为2的图像 SqlCommand cmd = new SqlCommand(strSql, conn); conn.Open(); SqlDataReader reader = cmd.ExecuteReader(); reader.Read(); Response.ContentType = "application/octet-stream"; Response.BinaryWrite((Byte[])reader["FImage"]); Response.End(); reader.Close(); } } ``` #### WinForm应用中的图像处理 对于WinForm应用程序而言,也可以实现类似的功能,即向SQL Server数据库上传并从其中读取图像数据。 **1. 使用WinForm上传图像** 创建一个`OpenFileDialog`控件来选择图像文件。然后,创建一个按钮控件来触发图像上传操作。具体步骤如下: - 在窗体上添加一个`OpenFileDialog`控件(例如命名为`ofdSelectPic`)。 - 添加一个按钮控件(例如命名为`btnUpload`),并在其点击事件中实现图像上传逻辑。 在`btnUpload_Click`事件中,可以使用类似ASP.NET中的方法来实现图像数据的处理和存储: ```csharp private void btnUpload_Click(object sender, EventArgs e) { if (ofdSelectPic.ShowDialog() == DialogResult.OK) { // 将选定的图像转换为byte数组 byte[] imageData = File.ReadAllBytes(ofdSelectPic.FileName); // 连接到数据库 using (SqlConnection conn = new SqlConnection("DataSource=localhost;Database=test;UserId=sa;Pwd=sa")) { // 创建或使用已有的存储过程 SqlCommand cmd = new SqlCommand("UpdateImage", conn); cmd.CommandType = CommandType.StoredProcedure; cmd.Parameters.Add("@UpdateImage", SqlDbType.Image).Value = imageData; conn.Open(); cmd.ExecuteNonQuery(); } } } ``` **2. 使用WinForm从SQL Server读取并显示图像** 为了在WinForm应用中显示从数据库读取的图像,可以使用`PictureBox`控件。具体步骤如下: - 创建一个`PictureBox`控件(例如命名为`pictureBox1`)。 - 编写一个方法来读取图像数据并显示在`PictureBox`中。 ```csharp private void LoadImageFromDatabase(int imageId) { using (SqlConnection conn = new SqlConnection("DataSource=localhost;Database=test;UserId=sa;Pwd=sa")) { string strSql = "SELECT FImage FROM Test WHERE Id = @Id"; SqlCommand cmd = new SqlCommand(strSql, conn); cmd.Parameters.AddWithValue("@Id", imageId); conn.Open(); byte[] imageData = (byte[])cmd.ExecuteScalar(); if (imageData != null) { pictureBox1.Image = Image.FromStream(new MemoryStream(imageData)); } } } ``` ### 结论 本文详细介绍了在网站开发中如何使用ASP.NET与SQL Server进行图像的上传和显示,并简要概述了在WinForm应用中实现类似功能的方法。这些技术是构建具有丰富多媒体内容的现代网站的基础。希望本文能帮助读者更好地理解和掌握网站开发中的关键技术和实践经验。
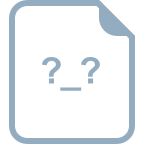
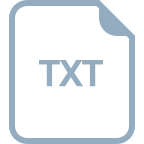
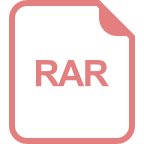
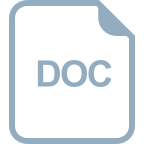
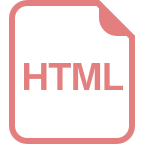
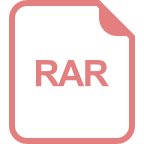
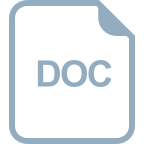
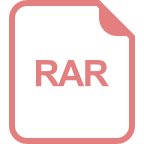
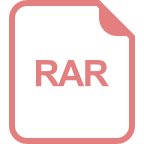
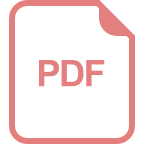
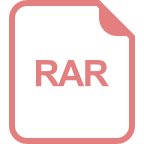
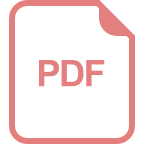
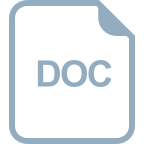
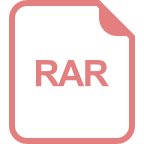
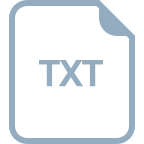
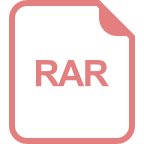
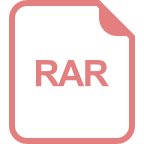
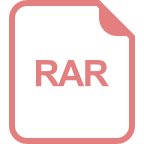
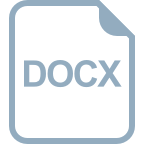
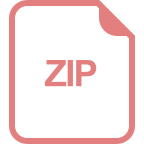
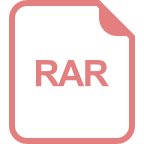
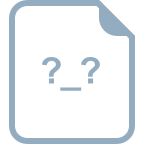
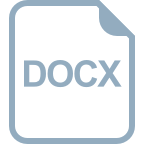
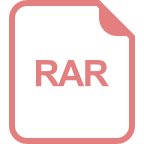
本文总结如何在.Net WinForm和.Net WebForm(asp.net)中将图片存入SQL Server中并读取显示的方法 。
1.使用asp.net将图片上传并存入SQL Server中,然后从SQL Server中读取并显示出来:
1)上传并存入SQL Server
数据库结构
create table test
{
id identity(1,1),
FImage image
}
相关的存储过程
Create proc UpdateImage
(
@UpdateImage Image
)
As
Insert Into test(FImage) values(@UpdateImage)
GO
在UpPhoto.aspx文件中添加如下:
<input id="UpPhoto" name="UpPhoto" runat="server" type="file">
<asp:Button id="btnAdd" name="btnAdd" runat="server" Text="上传"></asp:Button>
然后在后置代码文件UpPhoto.aspx.cs添加btnAdd按钮的单击事件处理代码:
private void btnAdd_Click(object sender, System.EventArgs e)
{
//获得图象并把图象转换为byte[]
HttpPostedFile upPhoto=UpPhoto.PostedFile;
int upPhotoLength=upPhoto.ContentLength;
byte[] PhotoArray=new Byte[upPhotoLength];
Stream PhotoStream=upPhoto.InputStream;
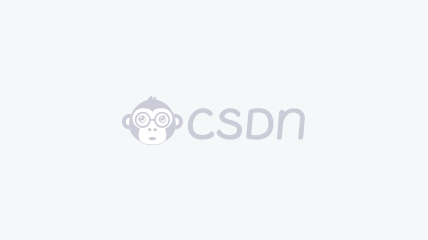

- 粉丝: 0
- 资源: 7
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

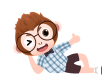
最新资源

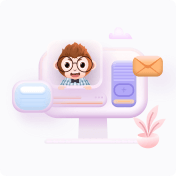
