windows .ini配制文件的读取
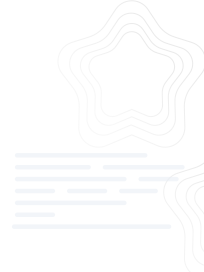

在Windows操作系统中,`.ini`文件是一种传统的配置文件格式,用于存储应用程序的设置和配置信息。这类文件以纯文本形式存在,结构清晰,易于编辑。本文将深入探讨`.ini`文件的结构、读取方法以及如何自定义一个类来处理这类文件。 `.ini`文件的结构通常包括三个主要部分:`[Section]`、`Key = Value`和注释。每个`[Section]`代表一类设置,键值对`Key = Value`则存储具体的配置信息。例如: ```ini [General] Language=Chinese Theme=Dark [Network] Server=192.168.1.1 Port=8080 ``` 在上述例子中,`[General]`和`[Network]`是两个不同的配置段,分别包含了语言和主题设置,以及服务器地址和端口号。 要读取`.ini`文件,Windows提供了API函数,如`GetPrivateProfileString`和`WritePrivateProfileString`等。但为了实现自定义类,我们可以使用C++的标准库`fstream`和字符串操作来进行读写。 我们需要创建一个名为`IniReader`的类,该类包含以下功能: 1. 打开和关闭`.ini`文件。 2. 读取特定`Section`下的`Key`值。 3. 写入或更新`Key`值。 4. 删除`Key`或整个`Section`。 以下是一个简单的`IniReader`类设计: ```cpp #include <fstream> #include <string> #include <unordered_map> class IniReader { public: IniReader(const std::string& filename) : filename_(filename) {} ~IniReader() {} bool Open() { file_.open(filename_, std::ios::in); return file_.is_open(); } void Close() { if (file_.is_open()) { file_.close(); } } std::string ReadValue(const std::string& section, const std::string& key) { // 实现读取`Key`值的逻辑 } void WriteValue(const std::string& section, const std::string& key, const std::string& value) { // 实现写入或更新`Key`值的逻辑 } void RemoveKey(const std::string& section, const std::string& key) { // 实现删除`Key`的逻辑 } void RemoveSection(const std::string& section) { // 实现删除`Section`的逻辑 } private: std::ifstream file_; std::string filename_; }; ``` 在`ReadValue`方法中,我们需要遍历文件,找到指定的`Section`,然后在该段内查找`Key`。对于`WriteValue`,我们需要检查`Key`是否已存在,如果不存在,则添加到对应`Section`;如果存在,则更新其值。`RemoveKey`和`RemoveSection`则需要删除相应的条目。 在实际开发中,我们还可以扩展这个类以支持更复杂的操作,比如支持多线程读写、错误处理和日志记录等。此外,为了提高性能,可以考虑使用缓存来存储已经读取的`Section`和`Key`值。 `.ini`文件是Windows系统中的经典配置文件格式,通过自定义类,我们可以方便地进行读写操作,实现应用程序的配置管理。理解并熟练运用此类文件,对于开发跨平台软件或与旧系统集成具有重要意义。
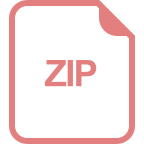
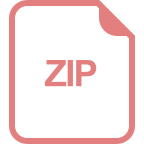
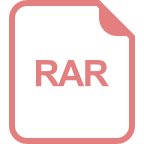
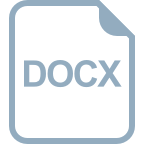
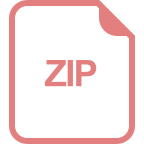
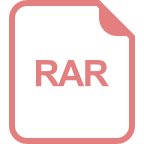
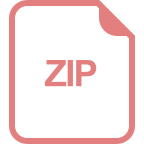
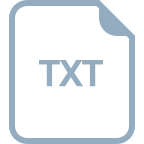
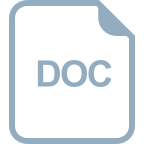
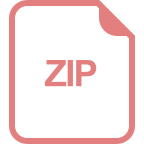
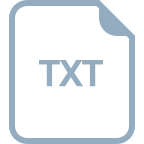
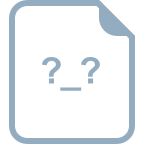
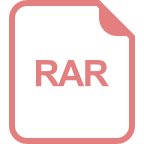
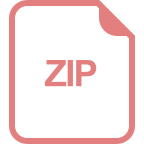
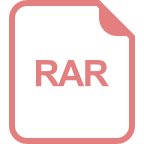


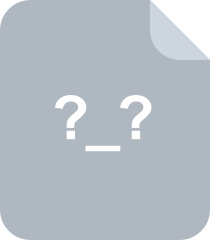
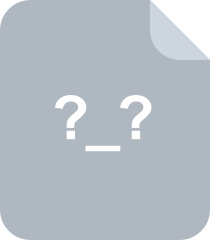
- 1
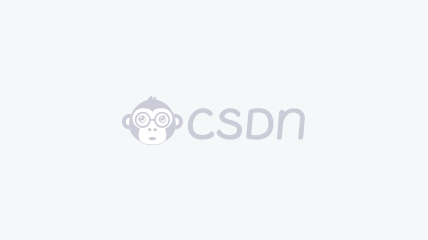

- 粉丝: 0
- 资源: 4
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

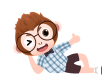
最新资源

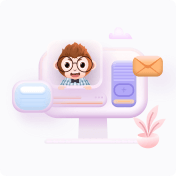
