没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
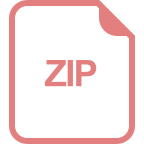
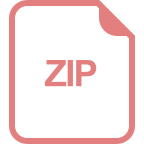
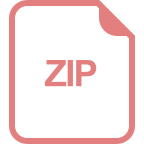
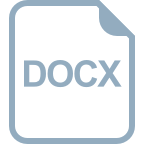
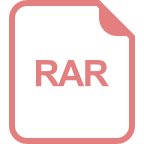
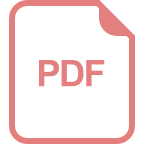
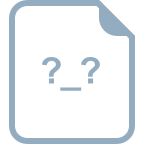
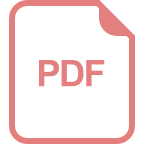
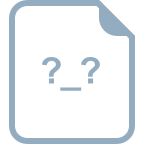
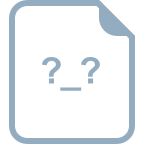
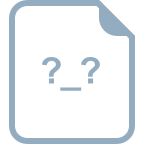
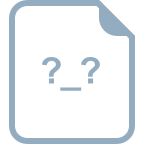
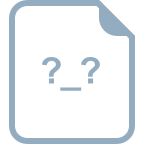
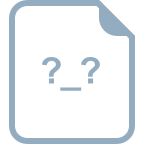
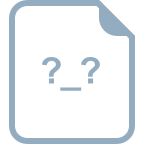
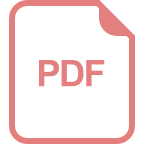
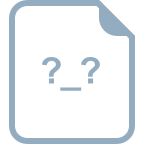
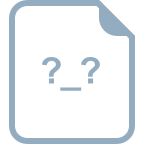
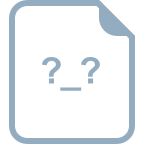
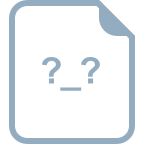
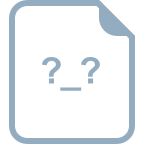
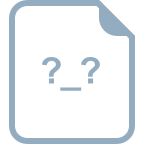
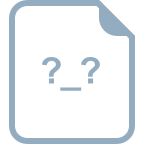
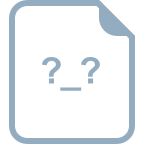
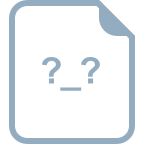
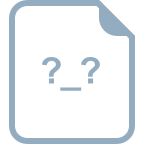
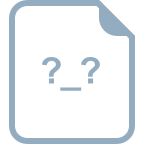
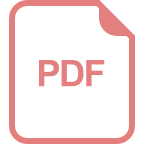
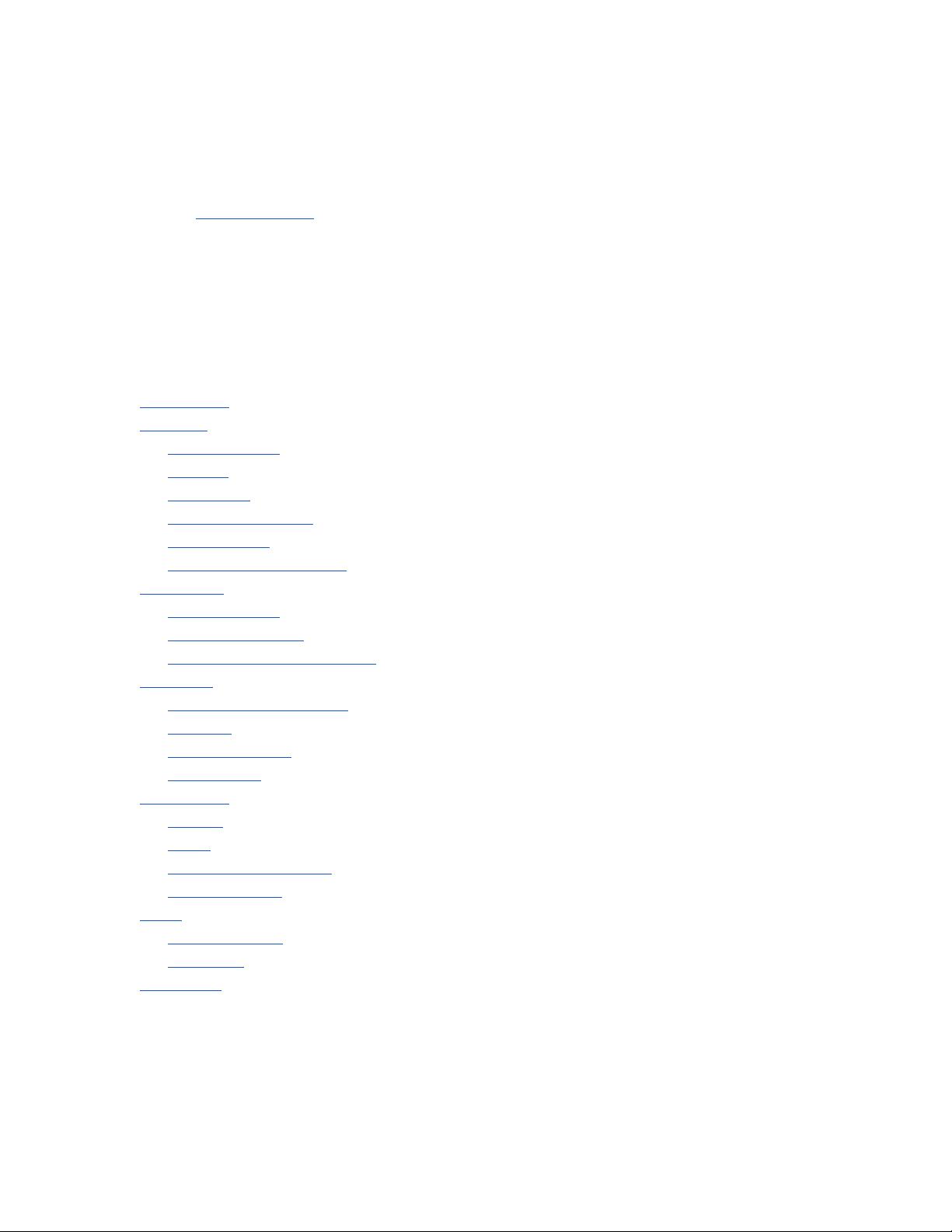
UsingProjectKotlinforAndroid
Statically-typed,highly-interoperableaugmentationtotheJavalanguage.
Author: @JakeWharton
Created: 2015-01-20
Updated: 2015-01-26
Note:
This is a copy (and slight edit) of a document that was presented internally at Square. It
advocates the use of the Kotlin language for developing Android apps.
Table of Contents
Introduction
Features
Interoperability
Lambas
Null Safety
Extension Methods
Data Classes
Other Awesome Things
Use Cases
Utility Methods
RxJava, Listeners
Mock Mode & Test Fixtures
Technical
Feature Implementation
Runtime
Build Integration
IDE Support
Alternatives
Groovy
Scala
JDK 8 + Retrolambda
Wait for Java 8
Risks
New Language
Jack & Jill
Resources
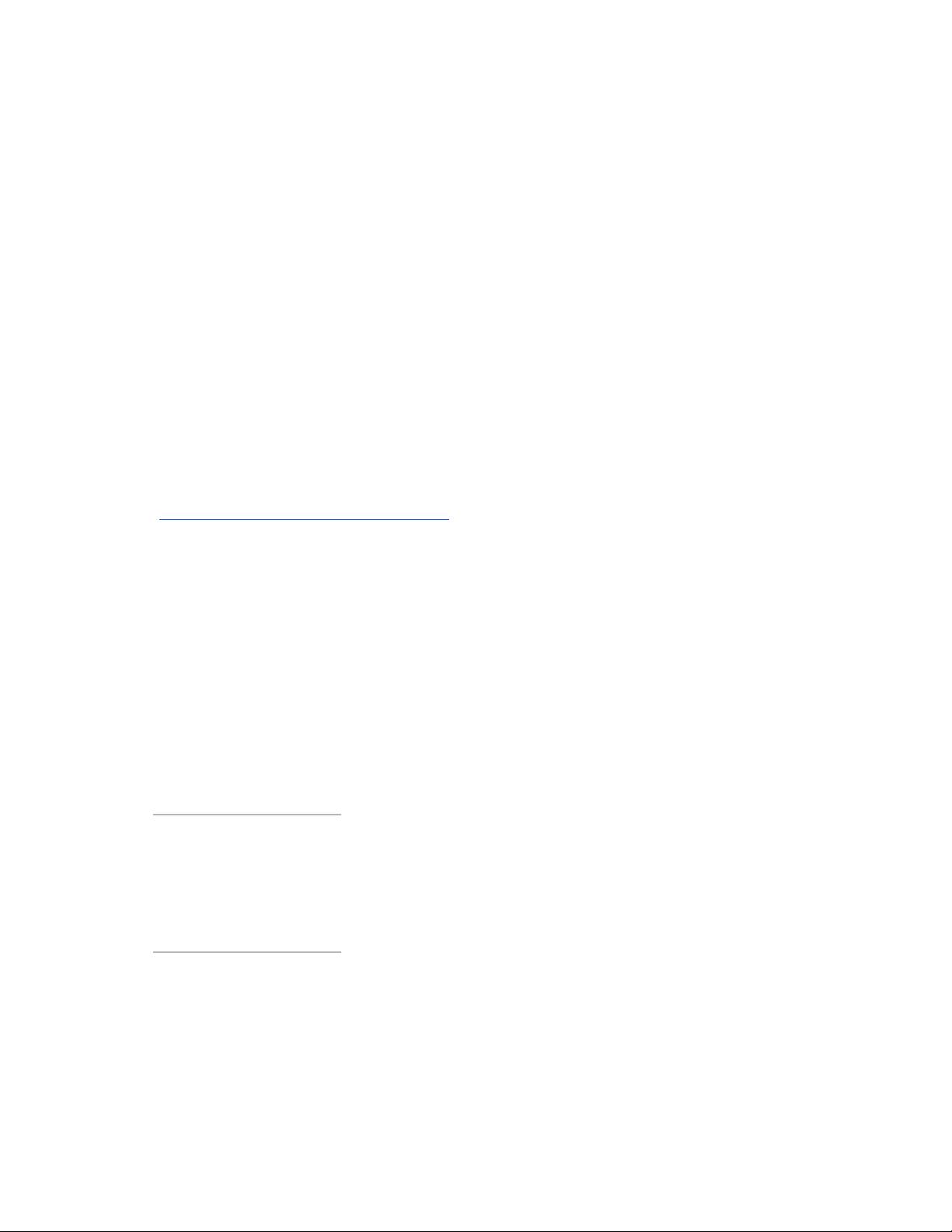
Introduction
Kotlin is a language by JetBrains, the company behind IntelliJ IDEA and other (sweet) tools, and
is purpose built for large-scale software projects to improve upon Java with a focus on
readability, correctness, and developer productivity.
The language was created in response to limitations in Java which were hindering development
of JetBrains' software products and after an evaluation of all other JVM languages proved
unsuitable. Since the goal of Kotlin was for use in improving their products, it focuses very
strongly on interop with Java code and the Java standard library.
Features
Below are the features which provide the largest value to Android and solve specific, incessant
problems that plague client application development. For a comprehensive list of features refer
to the official Kotlin reference documentation.
Interoperability
The most important feature of the Kotlin language and runtime is its core focus on
interoperability. Unlike other JVM alternative languages (most specifically: Scala), idiomatic
Kotlin should be able to easily call Java as well as have idiomatic Java easily call into Kotlin. In
fact, you should never know that you are crossing that boundary in either direction.
The runtime of Kotlin exists only to support the language features making it extremely lean. The
java standard library types, collections, etc. are all reused and ultimately are augmented for
greater utility through some of the subsequently mentioned features.
(See the Kotlin documentation for more.)
Lambas
'nuff said.
(See the Kotlin documentation for more.)
NullSafety
Our best friend null is a first-class citizen in Kotlin's type system. Types are aware of their
nullability and it receives special treatment in both flow control and dereferencing.
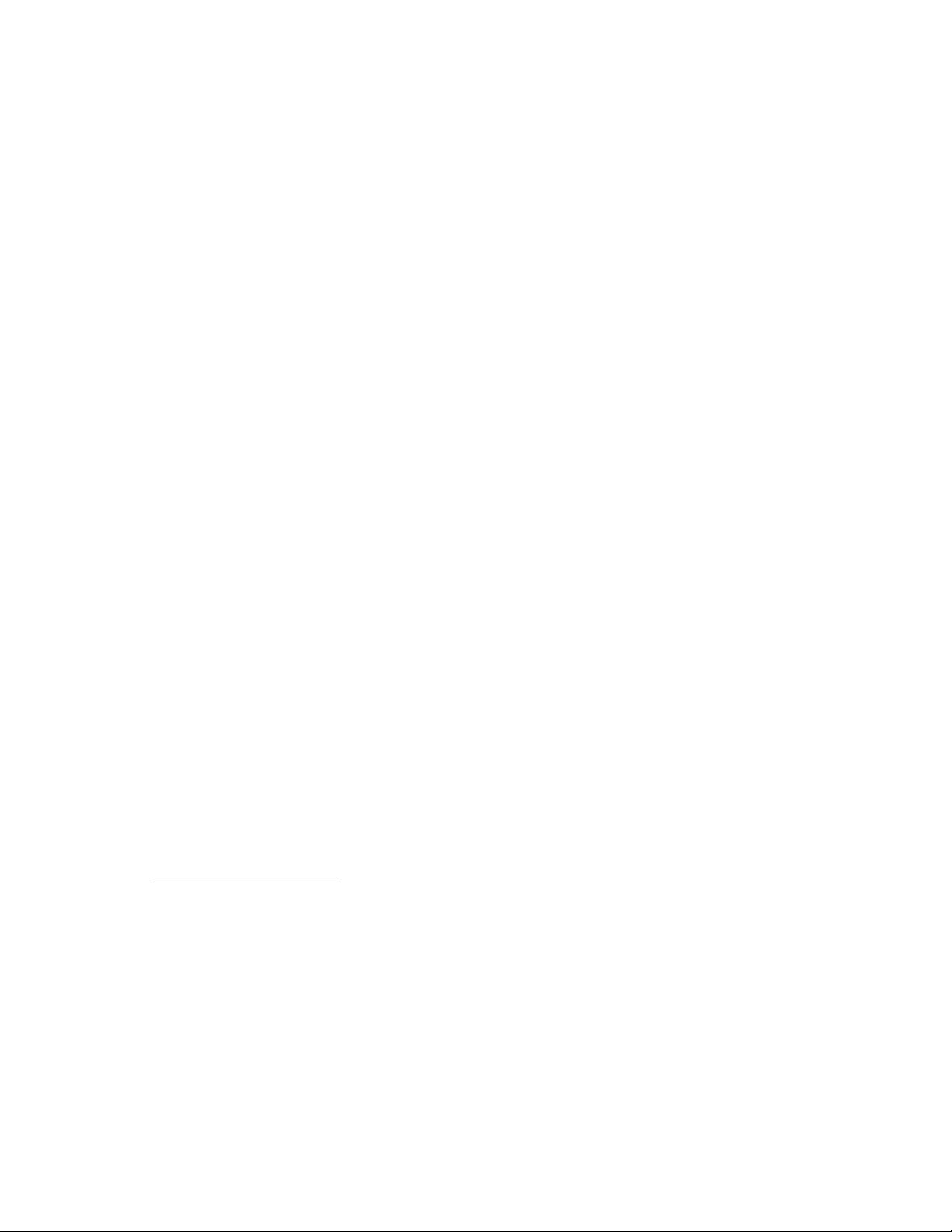
val x: String? = "Hi"
x.length // Does not compile.
val y: String = null // Does not compile.
Since null is unavoidable, there are many ways to deal with it.
if (x != null) {
x.length // Compiles! Not idiomatic just to get length!
}
// Same as above (IntelliJ auto-suggested the change).
x?.length
// Elvis operator.
val
len
=
x?.
length
?:
-
1
val
len
= x!!.
length
// Will throw if null. Rarely used.
Having null in the type system is vastly superior to nullability annotations as one would find in
Java. In this case both the IDE and the compiler are strictly enforcing contracts instead of just
suggesting.
By pushing nullability into the type system, traditional workarounds like optional are not needed.
This is especially important on Android whose heap and garbage collector are much more
sensitive to tiny and/or short-lived objects. Java 10 will push Optional onto the stack, but we'll
all be dead before Android sees that.
Due to the aforementioned heap and GC concerns, Android uses null a lot. Like a lot
a lot.
Since all references are nullable in Java, Android code is often littered with unneeded null
checks or experiences crashes due to dereferencing something which was not thought to be
null. Moving this into the type system will eliminate this ambiguity removing both the noisy,
needless checks and a large majority of inadvertent exceptions.
(See the Kotlin documentation for more.)
ExtensionMethods
In a way similar to C#, Kotlin allows the declaration of both static and instance methods into
types which you do not control–including those from the Java standard library. Unlike C#,
however, these method are statically resolved using imports which provides clarity on their
origin (just as a statically imported helper method on a utility class would).
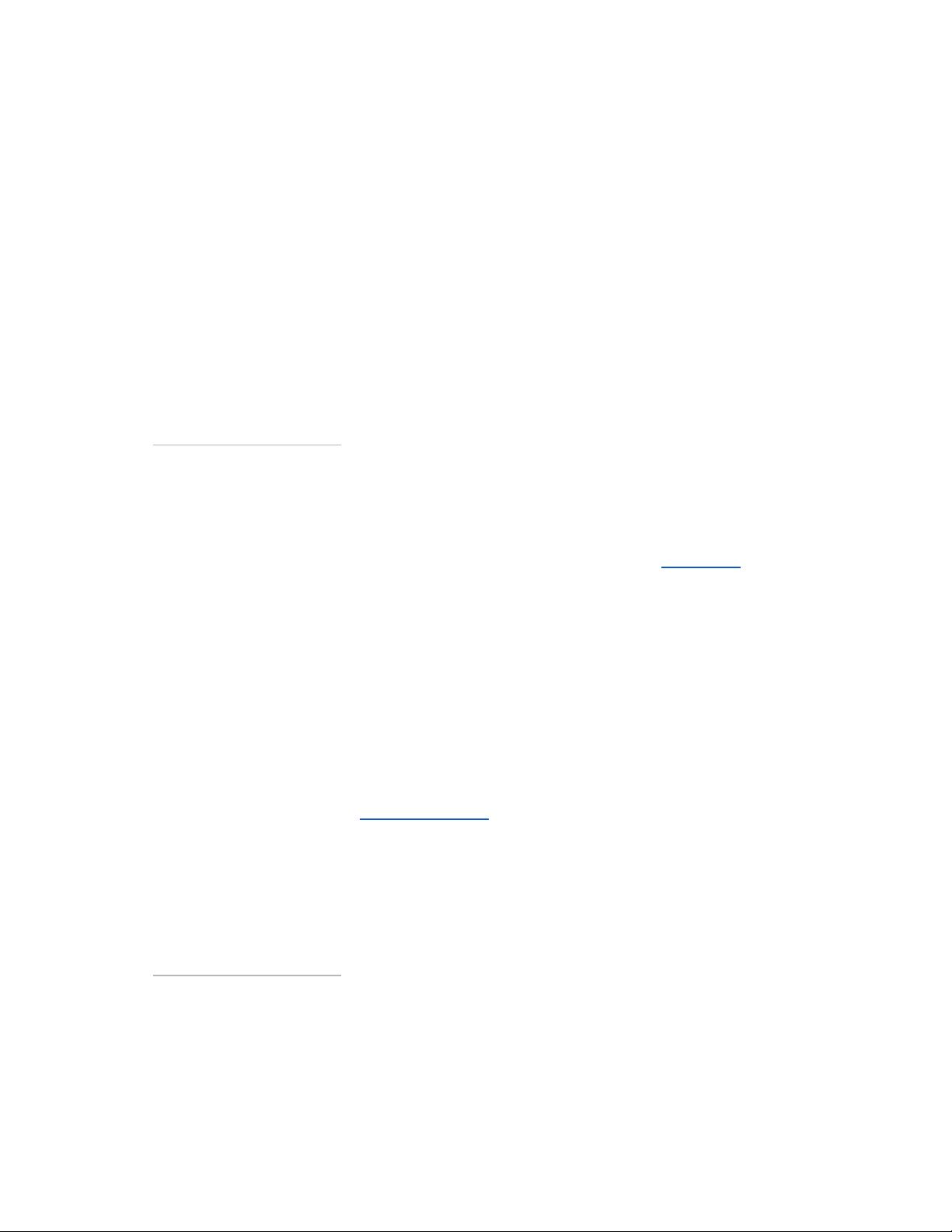
fun String.last() : Char {
return this[length - 1] // Using built-in extension for array indexing!
}
val x
= "Hey!"
println(x.last()) // Prints "!".
Half of Guava* and 2/3rds of the Cash app* is comprised of static utility methods. While this
wouldn't eliminate their presence, it would allow you to use them directly on the types for which
they were implemented. This is a big win for readability. Death to the plurals (Collections), the
numbereds (Collections2), and the 'util' suffixeds (StringUtils).
* Not even remotely a true statistic
(See the Kotlin documentation for more.)
DataClasses
Akin to what value types in Java 10 are hoping to accomplish, data classes are those which
exist only to group data in a semantic, immutable type. We currently use AutoValue to simulate
value types in an easy fashion.
data class Money(val currency: String, val amount: Int)
This class gives you equals, hashCode, toString for free. Each component is exposed by a
read-only property of the same name. There is also a copy method for changing values into a
new instance.
val money = Money("USD", 100)
val moreMoney = money.copy(amount = 200) // Oh hey named params!
Instances can be unpacked with multi-assignment. This is similar to Python's tuples and has
been shimmed into the standard library such as for Map.Entry when looping over a map.
for ((key, value) in map) {
println(key + ": " + value)
}
(See the Kotlin documentation for more.)
OtherAwesomeThings
● Classes and methods are final by default. You can declare them open if you want
extensibility.
剩余21页未读,继续阅读
资源评论
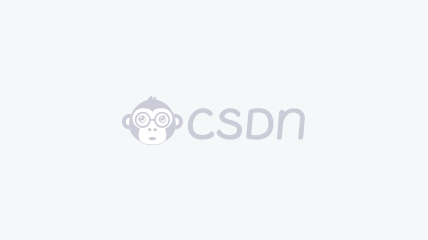

xgc82
- 粉丝: 15
- 资源: 114
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

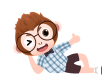
最新资源
- 机械设计螺母自动组装成方管并焊接机sw18全套设计资料100%好用.zip.zip
- 机械设计零件防错视觉检测机(sw18可编辑+工程图+BOM)全套设计资料100%好用.zip.zip
- 机械设计流水线机器人装盘机sw21全套设计资料100%好用.zip.zip
- 机械设计磨床输送机 磨削输送机sw21全套设计资料100%好用.zip.zip
- 机械设计螺丝装袋塑封机2018可编辑全套设计资料100%好用.zip.zip
- 机械设计铝条点胶贴合机sw21全套设计资料100%好用.zip.zip
- 机械设计内径公差测定器sw16可编辑全套设计资料100%好用.zip.zip
- 机械设计木勺的设备sw18全套设计资料100%好用.zip.zip
- 机械设计偏光镜贴合机sw21全套设计资料100%好用.zip.zip
- 机械设计乒乓球上打孔插入塞子机sw21全套设计资料100%好用.zip.zip
- 机械设计平板电脑自动贴双面胶带机sw14可编辑全套设计资料100%好用.zip.zip
- 联想M7450F打印机官方驱动安装程序
- 电热锅炉供暖系统的仿人智能控制器的设计与研究
- 基于ARM9的无线数据采集系统研究与设计
- 二相混合式步进电机细分控制技术研究及驱动器的设计
- 基于FPGA的多通道多速率信号传输研究与设计
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


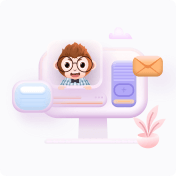
安全验证
文档复制为VIP权益,开通VIP直接复制
