import java.awt.event.*;
import java.awt.print.PageFormat;
import java.awt.print.PrinterJob;
import java.text.*;
import java.util.*;
import java.io.*;
import javax.swing.undo.*;
import javax.swing.*;
import javax.swing.event.*;
import javax.swing.text.StyledDocument;
import java.awt.BorderLayout;
import java.awt.Color;
import java.awt.Component;
import java.awt.Container;
import java.awt.Dimension;
import java.awt.FlowLayout;
import java.awt.Font;
import java.awt.Graphics;
import java.awt.GraphicsEnvironment;
import java.awt.GridLayout;
import java.awt.Image;
import java.awt.MenuItem;
import java.awt.PrintJob;
import java.awt.Rectangle;
import java.awt.Toolkit;
import java.awt.datatransfer.*;
import java.util.List;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import com.sxt.nodepad.util.TestLine;
public class Notepad4 extends JFrame implements ActionListener, DocumentListener {
JMenu mFile, mEdit, mMode, mView, mHelp;
// ---------------文件菜单
JMenuItem mFile_New, mFile_Open, mFile_Save, mFile_ASave, mFile_Print,mFile_Page, mFile_Exit;
// ---------------编辑菜单
JMenuItem mEdit_Undo, mEdit_Cut, mEdit_Copy, mEdit_Paste, mEdit_Del, mEdit_Search, mEdit_SearchNext, mEdit_Replace,
mEdit_Turnto, mEdit_SelectAll, mEdit_TimeDate,mEdit_InsertIcon;
// ---------------格式菜单
JCheckBoxMenuItem formatMenu_LineWrap;
JMenu formatMenu_Color;
JMenuItem formatMenu_Font, formatMenu_Color_FgColor, formatMenu_Color_BgColor;
JMenuItem formatMenu_ChangeToUp;//转换大写
JMenuItem formatMenu_ChangeToLow;//转换小写
// ---------------查看菜单
JCheckBoxMenuItem viewMenu_Status;
JMenuItem viewMenu_Count;
// ---------------帮助菜单
JMenuItem mHelp_HelpTopics, mHelp_About;
// ---------------弹出菜单级菜单项
JPopupMenu popupMenu;
JMenuItem popupMenu_Undo, popupMenu_Cut, popupMenu_Copy, popupMenu_Paste, popupMenu_Delete, popupMenu_SelectAll;
// ---------------工具栏按钮
JButton newButton, openButton, saveButton, saveAsButton, printButton, undoButton, redoButton, cutButton, copyButton,
pasteButton, deleteButton, searchButton, timeButton, fontButton, boldButton, italicButton, fgcolorButton,
bgcolorButton, helpButton;
// 文本编辑区域
static JTextArea Text;
// 状态栏标签
JLabel statusLabel1, statusLabel2, statusLabel3;
JToolBar statusBar;
// ---------------系统剪贴板
Toolkit toolKit = Toolkit.getDefaultToolkit();
Clipboard clipBoard = toolKit.getSystemClipboard();
// ---------------创建撤消操作管理器
protected UndoManager undo = new UndoManager();
protected UndoableEditListener undoHandler = new UndoHandler();
// ----------------其它变量
boolean isNewFile = true; // 是否新文件(未保存过的)
File currentFile; // 当前文件名
String oldValue; // 存放编辑区原来的内容,用于比较文本是否有改动
JButton fontOkButton; // 字体设置里的"确定"按钮
// ----------------设置编辑区默认字体
protected Font defaultFont = new Font("宋体", Font.PLAIN, 12);
GregorianCalendar time = new GregorianCalendar();
int hour = time.get(Calendar.HOUR_OF_DAY);
int min = time.get(Calendar.MINUTE);
int second = time.get(Calendar.SECOND);
File saveFileName = null, fileName = null;
PrintJob p=null;//声明一个要打印的对象
Graphics g=null;//要打印的对象
private Object textArea;
JFileChooser file=new JFileChooser();
private StyledDocument doc = null;
JTextPane text=new JTextPane();
private JTextArea TextArea;
private JScrollPane scrollPane;
public Notepad4() {
super("Note");
setDefaultCloseOperation(DO_NOTHING_ON_CLOSE);
Container container = getContentPane();
// System.out.println(Text.getDragEnabled()); //支持自动拖放
JScrollPane scroll = new JScrollPane(Text);
scroll.setHorizontalScrollBarPolicy(JScrollPane.HORIZONTAL_SCROLLBAR_ALWAYS);
scroll.setVerticalScrollBarPolicy(JScrollPane.VERTICAL_SCROLLBAR_ALWAYS);
Text.setWrapStyleWord(true); // 设置单词在一行不足容纳时换行
Text.setLineWrap(true);
Text.setFont(defaultFont); // 设置编辑区默认字体
Text.setBackground(Color.white); // 设置编辑区默认背景色
Text.setForeground(Color.black); // 设置编辑区默认前景色
oldValue = Text.getText(); // 获取原文本编辑区的内容
// --------------------------编辑区注册事件监听
Text.getDocument().addUndoableEditListener(undoHandler); // 添加负责通知任何更改的撤消侦听器
Text.getDocument().addDocumentListener(this); // 添加负责通知任何更改的文档侦听器
JMenuBar MenuBar = new JMenuBar();
mFile = new JMenu("文件(F)", true); // 创建菜单
mEdit = new JMenu("编辑(E)", true);
mMode = new JMenu("格式(O)", true);
mView = new JMenu("查看(V)", true);
mHelp = new JMenu("帮助(H)", true);
mEdit.addActionListener(new ActionListener() // 注册事件监听
{
public void actionPerformed(ActionEvent e) {
checkMenuItemEnabled(); // 设置剪切、复制、粘贴、删除等功能的可用性
}
});
mFile.setMnemonic('F');
mEdit.setMnemonic('E');
mMode.setMnemonic('O');
mView.setMnemonic('V');
mHelp.setMnemonic('H');
MenuBar.add(mFile);
MenuBar.add(mEdit);
MenuBar.add(mMode);
MenuBar.add(mView);
MenuBar.add(mHelp);
// --------------文件菜单
mFile_New = new JMenuItem("新建(N)", 'N');
mFile_New = new JMenuItem("新建(N)",new ImageIcon("Icons/new.gif"));
mFile_Open = new JMenuItem("打开(O)", 'O');
mFile_Open= new JMenuItem("打开(O)",new ImageIcon("Icons/open.gif"));
mFile_Save = new JMenuItem("保存(S)", 'S');
mFile_Save= new JMenuItem("保存(S)",new ImageIcon("Icons/save.gif"));
mFile_ASave = new JMenuItem("另存为(A)", 'A');
mFile_ASave = new JMenuItem("另存为(A)",new ImageIcon("Icons/save as.gif"));
mFile_Print = new JMenuItem("打印(P)", 'P');
mFile_Print= new JMenuItem("打印(P)",new ImageIcon("Icons/print.gif"));
mFile_Page = new JMenuItem("页面设置(U)",'U');
mFile_Page = new JMenuItem("页面设置(U)",new ImageIcon("Icons/page.gif"));
mFile_Exit = new JMenuItem("退出(X)", 'X');
mFile_Exit= new JMenuItem("退出(X)",new ImageIcon("Icons/exit.gif"));
mFile_New.setAccelerator(KeyStroke.getKeyStroke(KeyEvent.VK_N, InputEvent.CTRL_MASK));
mFile_Open.setAccelerator(KeyStroke.getKeyStroke(KeyEvent.VK_O, InputEvent.CTRL_MASK));
mFile_Save.setAccelerator(KeyStroke.getKeyStroke(KeyEvent.VK_S, InputEvent.CTRL_MASK));
mFile_Print.setAccelerator(KeyStroke.getKeyStroke(KeyEvent.VK_P, InputEvent.CTRL_MASK));
mFile_New.addActionListener(this); // 注册事件监听
mFile_Open.addActionListener(this);
mFile_Save.addActionListener(this);
mFile_ASave.addActionListener(this);
mFile_Print.addActionListener(this);
mFile_Page.addActionListener(this);
mFile_Exit.addActionListener(this);
mFile.add(mFile_New); // 添加菜单项
mFile.add(mFile_Open);
mFile.add(mFile_Save);
mFile.add(mFile_ASave);
mFile.addSeparator(); // 添加分割线
mFile.add(mFile_Print);
mFile.addSeparator(); // 添加分割线
mFile.add(mFile_Page);
mFile.addSeparator(); // 添加分割线
mFile.add(mFile_Exit);
// --------------编辑菜单
mEdit_Undo = new JMenuItem("撤消(U)", 'U');
mEdit_Undo= new JMenuItem("撤消(U)",new ImageIcon("Icons/undo.gif"));
mEdit_Cut = new JMenuItem("剪切(T)", 'T');
mEdit_Cut= new JMenuItem("剪切(T)",new ImageIcon("Icons/cut.gif"));
mEdit_Copy = new JMenuItem("复制(C)", 'C');
mEdit_Copy= new JMenuItem("复制(C)",new ImageIcon("Icons/copy.gif"));
mEdit_Paste = new JMenuItem("粘贴(P)", 'P');
mEdit_Paste= new JMenuItem("粘贴(P)",new ImageIcon("Icons/paste.gif"));
mEdit_Del = new JMenuItem("删除(L)", 'L');
mEdit_Del= new JMenuItem("删除(L)",new ImageIcon("Icons/delete.gif"));
mEdit_Search = new JMenuItem("查找(F)", 'F');
mEdit_Search= new JMenuItem("查找(F)",new ImageIcon("Icons/search.gif"));
mEdit_SearchNext = new JMenuItem("查找下一个(N)", 'N');
mEdit_SearchNext= new JMenuItem("查找下一个(N)",new ImageIcon("Icons/search.gif"));
mEdit_Replace
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
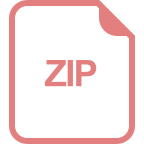
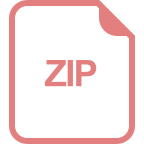
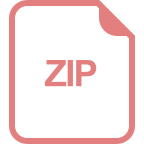
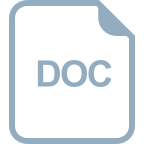
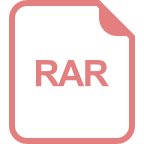
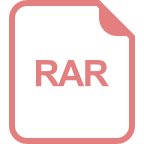
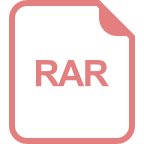
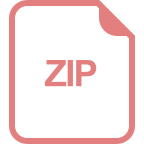
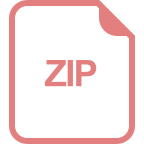
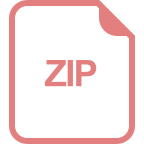
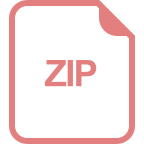
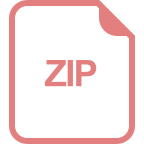
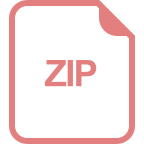
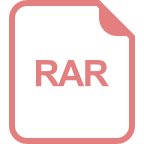
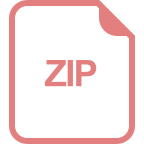
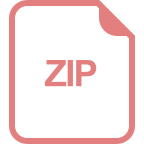
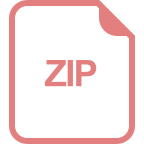
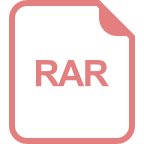
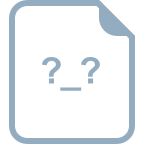
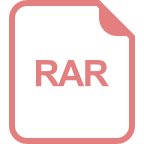
收起资源包目录



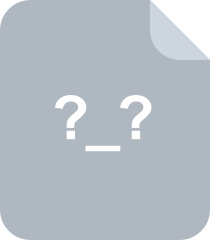

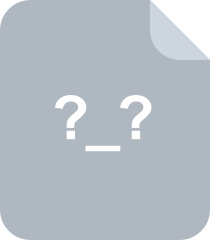
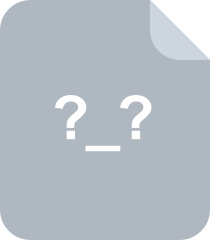
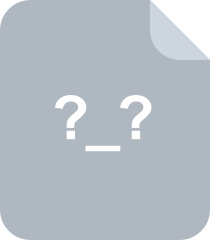
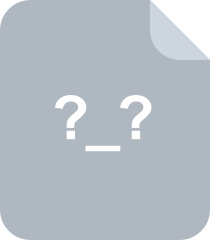




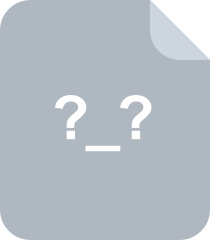
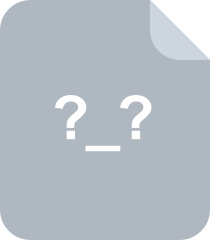
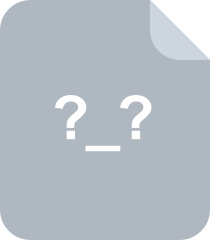
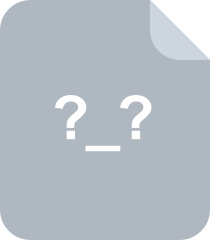
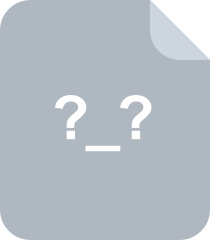
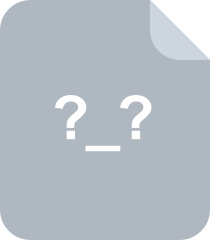
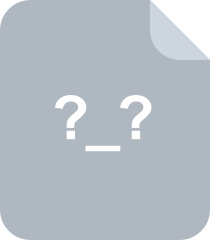
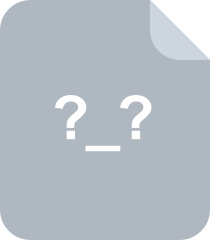
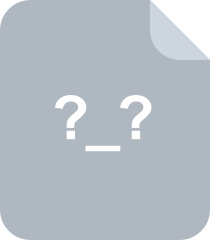
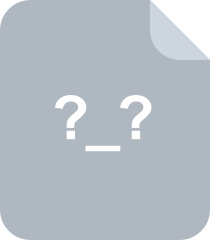
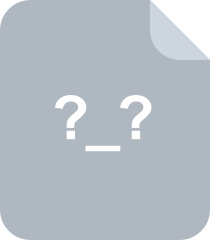
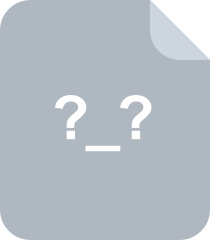
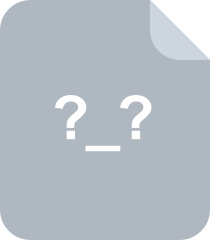
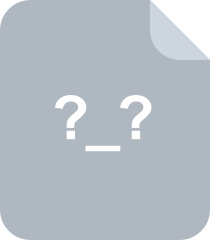
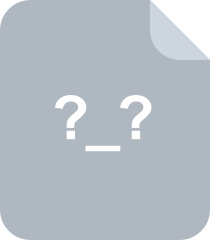

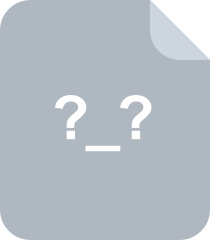

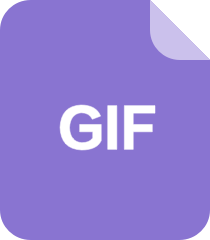
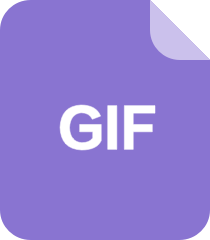
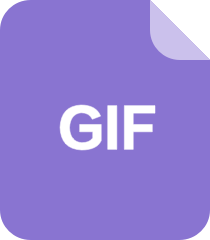
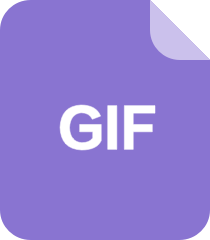
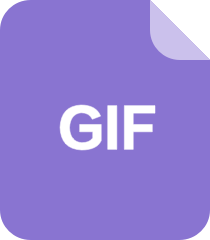
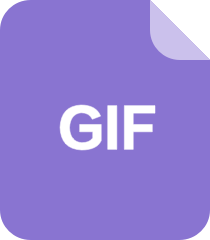
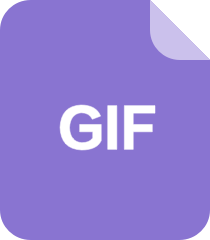
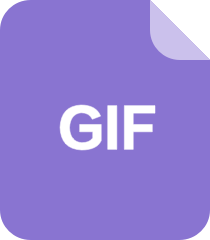
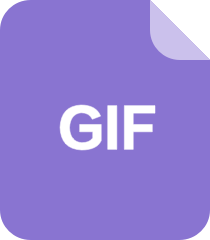
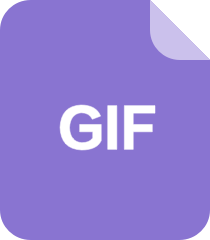
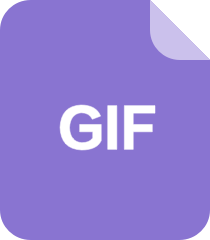
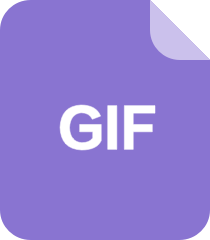
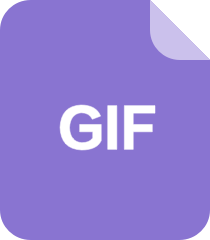
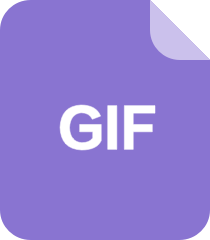
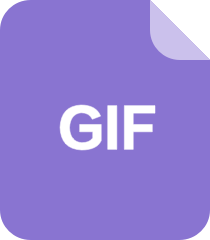
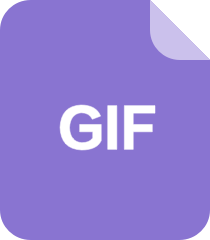
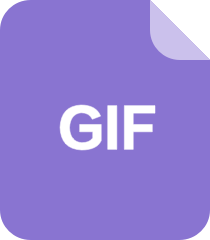
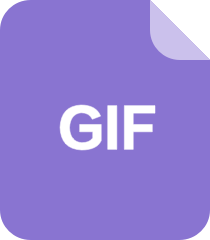
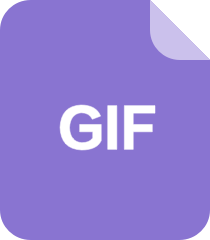
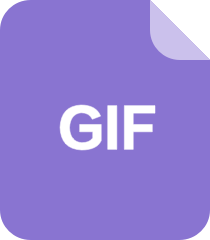
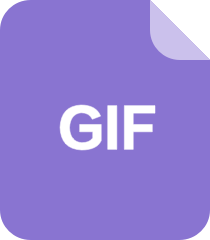
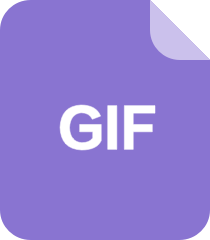
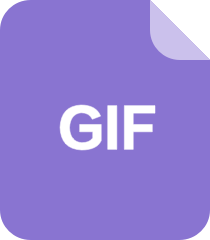
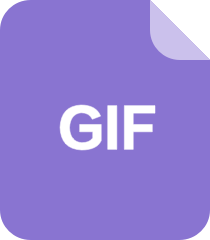
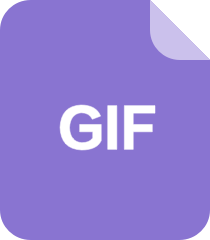
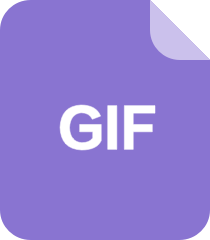
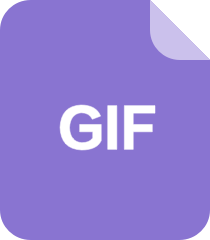
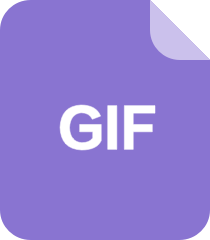
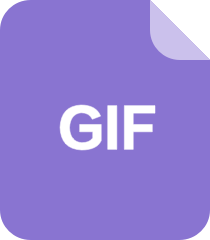
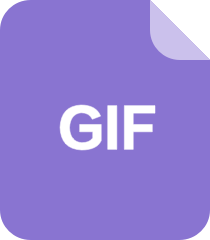
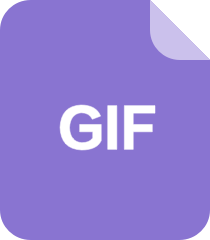
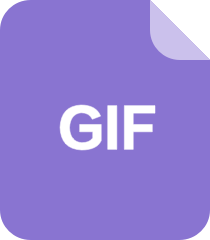
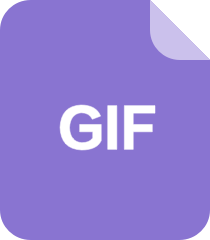

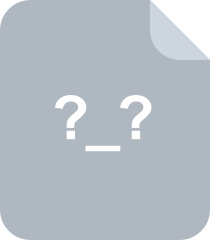
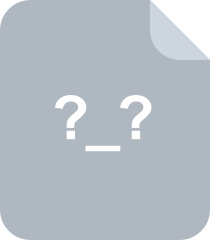
共 56 条
- 1
资源评论
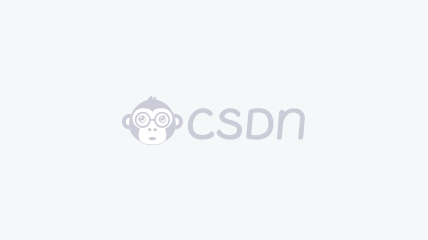
- qq_415237752019-12-30使用了什么非法什么的,不能运行啊

xcl490
- 粉丝: 0
- 资源: 1
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

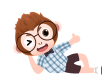
最新资源
- py-apple-controller-四足机器人
- py-apple-bldc-quadruped-robot-四足机器人
- 四足机器人-四足机器人
- asm-西电微机原理实验
- 四足机器人行走机制设计与应用解析
- 探索POINTS 1.5视觉思考模型:开启高效思考之门
- 支持 DELPHI 12.2的RXLIB 控件
- game_patch_1.29.13.13020.pak
- 4S店车辆管理系统.zip
- J2EE在在线项目管理与任务分配中的应用_411v2rh8_226-wx.zip
- “课件通”中小学教学课件共享平台.zip
- Java Web的租房管理系统(编号:22787207).zip
- Java大学生创新能力培养平台的设计与实现(编号:49116136).zip
- JavaWeb图书管理系统(编号:29027118)(1).zip
- springboot4S店车辆管理系统 LW PPT.zip
- spingboot茶文化推广系统(编号:3018432).zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


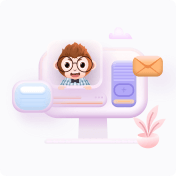
安全验证
文档复制为VIP权益,开通VIP直接复制
