package com.my.hadoop.mapreduce.util.opencv;
import java.awt.image.BufferedImage;
import java.awt.image.DataBufferByte;
import java.awt.image.Raster;
import java.awt.image.RenderedImage;
import java.awt.image.SampleModel;
import java.awt.image.WritableRaster;
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
import java.io.DataInputStream;
import java.io.DataOutputStream;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import javax.imageio.ImageIO;
import org.apache.hadoop.fs.FSDataInputStream;
import org.apache.hadoop.fs.Path;
import org.apache.hadoop.io.IOUtils;
import org.opencv.core.Core;
import org.opencv.core.CvType;
import org.opencv.core.Mat;
import org.opencv.core.MatOfRect;
import org.opencv.core.Point;
import org.opencv.core.Rect;
import org.opencv.core.Scalar;
import org.opencv.imgcodecs.Imgcodecs;
import org.opencv.imgproc.Imgproc;
import org.opencv.objdetect.CascadeClassifier;
//
// Detects faces in an image, draws boxes around them, and writes the results
// to "faceDetection.png".
//
public class DetectFaceDemo {
public static void main(String[] args) {
// Load the native library.
System.loadLibrary(Core.NATIVE_LIBRARY_NAME);
System.out
.println(new DetectFaceDemo()
.findface(
DetectFaceDemo.class.getResource("lbpcascade_frontalface.xml").getPath(),
DetectFaceDemo.class.getResource("lena.png").getPath()
));
// System.out
// .println(new DetectFaceDemo()
// .findfacenum(
// "/home/xxx/workspace/WordCount/src/com/my/hadoop/mapreduce/hdwithseqfile/demo/lbpcascade_frontalface.xml",
// "/home/xxx/workspace/WordCount/src/com/my/hadoop/mapreduce/hdwithseqfile/demo/lena.png"));
// new DetectFaceDemo().findfaces("/home/xxx/Desktop/tmp");
// new DetectFaceDemo()
// .findface(
// DetectFaceDemo.class.getResource("lbpcascade_frontalface.xml").getPath(),
// new byte[2],"1");
}
public DetectFaceDemo(){
System.loadLibrary(Core.NATIVE_LIBRARY_NAME);
}
public void findfaces(String parentPath) {
File parent = new File(parentPath);
File[] fs = parent.listFiles();
for (int i = 0; i < fs.length; i++) {
findfacenum(
"/home/xxx/workspace/WordCount/src/com/my/hadoop/mapreduce/hdwithseqfile/demo/lbpcascade_frontalface.xml",
"fs[i].getAbsolutePath()");
}
}
public static void printHexString(byte[] b) {
for (int i = 0; i < b.length; i++) {
String hex = Integer.toHexString(b[i] & 0xFF);
if (hex.length() == 1) {
hex = '0' + hex;
}
System.out.print(hex.toUpperCase());
}
}
public static BufferedImage mat2Img(Mat mat) {
BufferedImage image = new BufferedImage(mat.width(), mat.height(), BufferedImage.TYPE_3BYTE_BGR);
WritableRaster raster = image.getRaster();
DataBufferByte dataBuffer = (DataBufferByte) raster.getDataBuffer();
byte[] data = dataBuffer.getData();
mat.get(0, 0, data);
return image;
}
public static Mat img2Mat(BufferedImage image) {
byte[] data = ((DataBufferByte) image.getRaster().getDataBuffer())
.getData();
Mat mat = new Mat(image.getHeight(), image.getWidth(), CvType.CV_8UC3);
mat.put(0, 0, data);
return mat;
}
public boolean findface(String xmlfilePath, byte[] pic,String key) {
CascadeClassifier faceDetector = new CascadeClassifier(xmlfilePath);
//
InputStream in = new ByteArrayInputStream(pic);
BufferedImage bImageFromConvert = null;
try {
bImageFromConvert = ImageIO.read(in);
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
Mat image = img2Mat(bImageFromConvert);
// // Detect faces in the image.
// // MatOfRect is a special container class for Rect.
MatOfRect faceDetections = new MatOfRect();
// Imgcodecs.imwrite("/home/xxx/Desktop/tmp/down.jpg", newMat);
faceDetector.detectMultiScale(image, faceDetections);
int count = faceDetections.toArray().length;
if (count > 0) {
return true;
}
return false;
}
public boolean findface(String xmlfilePath, String picpath) {
// 注意:源程序的路径会多打印一个‘/’,因此总是出现如下错误
/*
* Detected 0 faces Writing faceDetection.png libpng warning: Image
* width is zero in IHDR libpng warning: Image height is zero in IHDR
* libpng error: Invalid IHDR data
*/
CascadeClassifier faceDetector = new CascadeClassifier(xmlfilePath);
Mat image = Imgcodecs.imread(picpath);
// Mat image = new Mat();
// Imgcodecs.
// Detect faces in the image.
// MatOfRect is a special container class for Rect.
MatOfRect faceDetections = new MatOfRect();
faceDetector.detectMultiScale(image, faceDetections);
int count = faceDetections.toArray().length;
if (count > 0) {
return true;
}
return false;
// Draw a bounding box around each face.
// for (Rect rect : faceDetections.toArray()) {
// Imgproc.rectangle(image, new Point(rect.x, rect.y), new Point(rect.x
// + rect.width, rect.y + rect.height), new Scalar(0, 255, 0));
// }
// Save the visualized detection.
// String filename = "faceDetection.png";
// System.out.println(String.format("Writing %s", filename));
// Imgcodecs.imwrite(filename, image);
}
public int findfacenum(String xmlfilePath, String picpath) {
CascadeClassifier faceDetector = new CascadeClassifier(xmlfilePath);
Mat image = Imgcodecs.imread(picpath);
// Detect faces in the image.
// MatOfRect is a special container class for Rect.
MatOfRect faceDetections = new MatOfRect();
faceDetector.detectMultiScale(image, faceDetections);
int count = faceDetections.toArray().length;
return count;
}
}
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
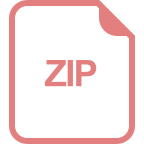
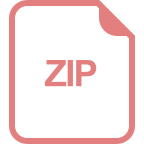
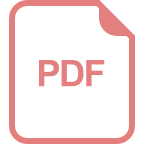
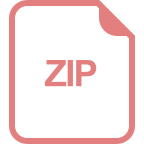
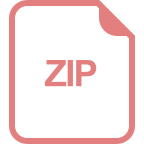
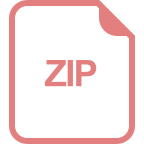
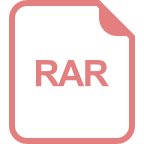
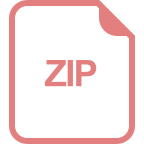
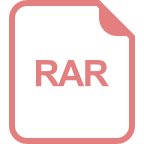
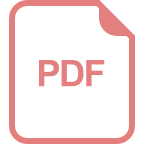
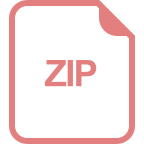
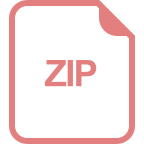
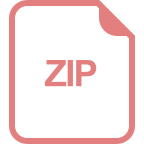
收起资源包目录


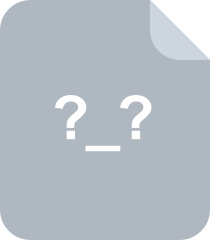

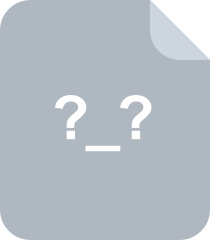






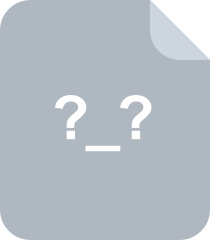
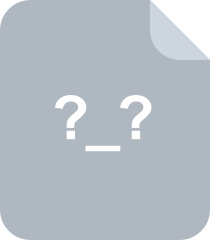
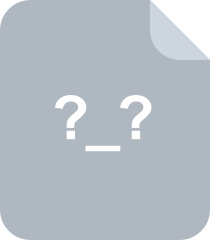
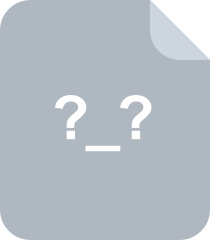
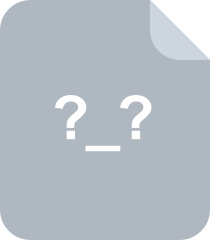
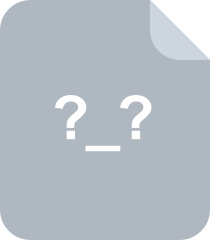
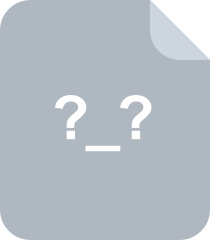
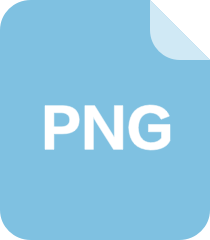


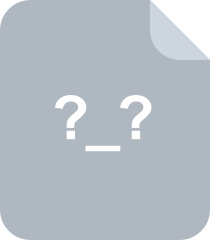
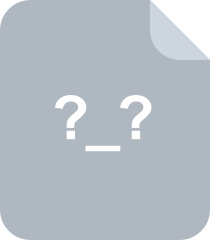
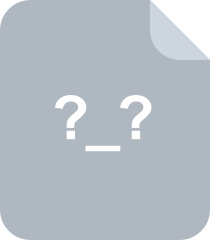
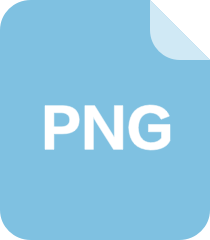

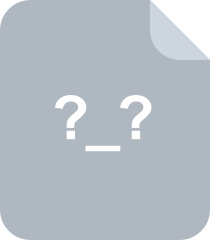






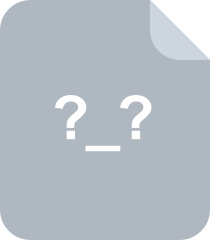
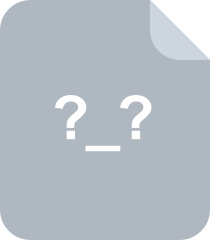
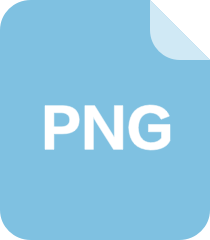
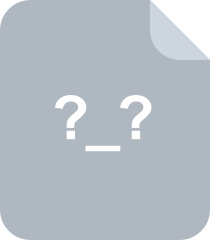


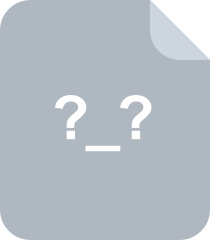
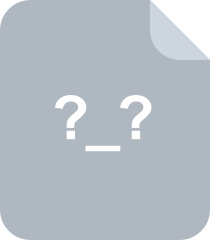
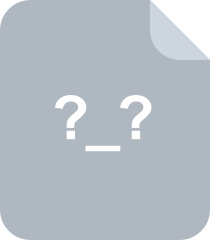
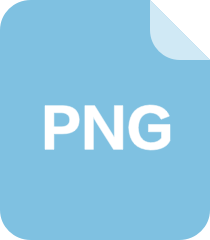
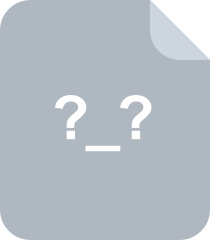

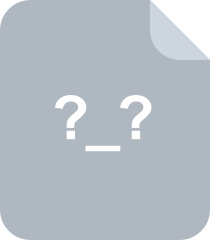
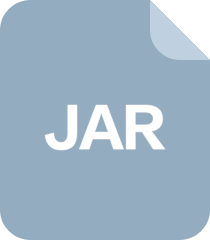
共 26 条
- 1
资源评论
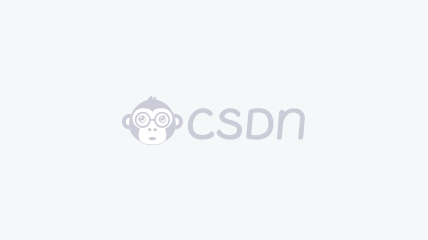
- liaobin6652018-04-21好资源,不错!

wzm112358
- 粉丝: 4
- 资源: 3
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

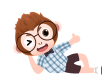
安全验证
文档复制为VIP权益,开通VIP直接复制
