package com.jewel.tag.pagination;
/**
* <p>Title: www.entel.com.cn</p>
* <p>Description: </p>
* <p>Copyright: Copyright (c) 2003</p>
* <p>Company: entel</p>
* @author chendesheng
* @version 1.0
*/
import java.lang.reflect.*;
import java.util.*;
import javax.servlet.jsp.*;
import javax.servlet.jsp.tagext.*;
import org.apache.commons.collections.*;
import org.apache.struts.util.*;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpSession;
public class PagerTag extends BodyTagSupport implements PagerInterface {
protected Iterator iterator = null;
protected int lengthCount = 0;
protected int lengthValue = 0;
protected MessageResources messages =
MessageResources.getMessageResources(
"org.apache.struts.taglib.logic.LocalStrings");
protected int offsetValue = 0;
protected boolean started = false;
protected Object collection = null;
public Object getCollection() {
return (this.collection);
}
public void setCollection(Object collection) {
this.collection = collection;
}
protected String id = null;
public String getId() {
return (this.id);
}
public void setId(String id) {
this.id = id;
}
public int getIndex() {
if (started) {
return (offsetValue + lengthCount - 1);
} else {
return (0);
}
}
protected String indexId = null;
public String getIndexId() {
return (this.indexId);
}
public void setIndexId(String indexId) {
this.indexId = indexId;
}
protected String length = null;
public String getLength() {
return (this.length);
}
public void setLength(String length) {
this.length = length;
}
protected String name = null;
public String getName() {
return (this.name);
}
public void setName(String name) {
this.name = name;
}
protected String offset = null;
public String getOffset() {
return (this.offset);
}
public void setOffset(String offset) {
this.offset = offset;
}
protected String property = null;
public String getProperty() {
return (this.property);
}
public void setProperty(String property) {
this.property = property;
}
protected String scope = null;
public String getScope() {
return (this.scope);
}
public void setScope(String scope) {
this.scope = scope;
}
protected String type = null;
public String getType() {
return (this.type);
}
public void setType(String type) {
this.type = type;
}
public int doStartTag() throws JspException {
Object collection = this.collection;
HttpServletRequest hsr = (HttpServletRequest) pageContext.getRequest();
HttpSession session = hsr.getSession(true);
String sessionID = session.getId();
int AllCountElement = 0;
if (collection == null) {
collection = RequestUtils.lookup(pageContext, name, property, scope);
}
if (collection == null) {
JspException e = new JspException(messages.getMessage(
"iterate.collection"));
RequestUtils.saveException(pageContext, e);
throw e;
}
if (collection.getClass().isArray()) {
try {
iterator = Arrays.asList( (Object[]) collection).iterator();
//Common.AllElementCount = Array.getLength(collection);
session.setAttribute(sessionID+"allcount",""+Array.getLength(collection));
AllCountElement = Array.getLength(collection);
} catch (ClassCastException e) {
int length = Array.getLength(collection);
//Common.AllElementCount = length;
session.setAttribute(sessionID+"allcount",""+length);
AllCountElement = length;
ArrayList c = new ArrayList(length);
for (int i = 0; i < length; i++) {
c.add(Array.get(collection, i));
}
iterator = c.iterator();
}
} else if (collection instanceof Collection) {
iterator = ( (Collection) collection).iterator();
//Common.AllElementCount = ( (Collection) collection).size();
session.setAttribute(sessionID+"allcount",""+( (Collection) collection).size());
AllCountElement = ( (Collection) collection).size();
} else if (collection instanceof Iterator) {
iterator = (Iterator) collection;
//Common.AllElementCount = Array.getLength(collection);
session.setAttribute(sessionID+"allcount",""+Array.getLength(collection));
AllCountElement = Array.getLength(collection);
} else if (collection instanceof Map) {
iterator = ( (Map) collection).entrySet().iterator();
//Common.AllElementCount = ( (Map) collection).size();
session.setAttribute(sessionID+"allcount",""+( (Map) collection).size());
AllCountElement = ( (Map) collection).size();
} else if (collection instanceof Enumeration) {
iterator = IteratorUtils.asIterator( (Enumeration) collection);
//Common.AllElementCount = Array.getLength(collection);
session.setAttribute(sessionID+"allcount",""+Array.getLength(collection));
AllCountElement = Array.getLength(collection);
} else {
JspException e = new JspException(messages.getMessage("iterate.iterator"));
RequestUtils.saveException(pageContext, e);
throw e;
}
// set Attribute of the "offset"
String tmpoffset = hsr.getParameter("offset");
if(tmpoffset != null && !tmpoffset.trim().equalsIgnoreCase("")){
setOffset(tmpoffset);
}else{
try{
String position = (String)session.getAttribute(sessionID+"offset");
if(position == null||position.equalsIgnoreCase(""))
position = "0";
setOffset(position);
}catch(Exception ex){
setOffset("0");
}
}
offsetValue = Integer.parseInt(getOffset());
if (offsetValue < 0 || offsetValue>AllCountElement ) {
offsetValue = 0;
}
// set out offset Value
//Common.offset = offsetValue;
session.setAttribute(sessionID+"offset",""+offsetValue);
// set Attribute of the "length"
String tmplength = hsr.getParameter("length");
if(tmplength != null && !tmplength.trim().equalsIgnoreCase(""))
length = tmplength;
if (length == null) {
lengthValue = 0;
} else {
try {
lengthValue = Integer.parseInt(length);
} catch (NumberFormatException e) {
Integer lengthObject = (Integer) RequestUtils.lookup(pageContext,
sessionID+"length", "session");
if (lengthObject == null) {
lengthValue = 0;
} else {
lengthValue = lengthObject.intValue();
}
}
}
if (lengthValue < 0) {
lengthValue = 0;
}
lengthCount = 0;
// set out the "length" attribute to Common.pageLength
//Common.pageLength = lengthValue;
session.setAttribute(sessionID+"length",""+lengthValue);
for (int i = 0; i < offsetValue; i++) {
if (iterator.hasNext()) {
iterator.next();
}
}
if (iterator.hasNext()) {
Object element = iterator.next();
if (element == null) {
pageContext.removeAttribute(id);
} else {
pageContext.setAttribute(id, element);
}
lengthCount++;
started = true;
if (indexId != null) {
pageContext.setAttribute(indexId, new Integer(getIndex()));
}
return (EVAL_BODY_AGAIN);
} else {
return (SKIP_BODY);
}
}
public int doAfterBody() throws JspException {
if (bodyContent != null) {
ResponseUtils.writePrevious(pageContext, bodyContent.getString());
bodyContent.clearBody();
}
if ( (lengthValue > 0) && (lengthCount >= lengthValue)) {
return (SKIP_BODY);
}
if (iterator.hasNext()) {
Object element = iterator.next();
if (element == null) {
没有合适的资源?快使用搜索试试~ 我知道了~
德生珠宝网网站源码
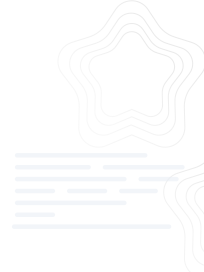
共58个文件
java:12个
root:9个
repository:9个


温馨提示
大家可以访问www.dolsen.net的网站,此网站的产品列表的分页使用自写的标签完成,非常灵活简单实用,<br>1 共12项 共1页 当前第1页 首页 前页 后页 未页 跳转,灵活定制,本作者特共享出来,希望大家互相学习;如果想要请发邮件给我。或到本网站论坛初级Java开发者下载。标题写明,分页tag源代码。dolsen.net@163.com 基于struts jsp java等技术
资源推荐
资源详情
资源评论
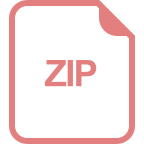
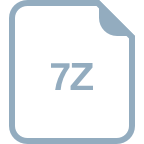
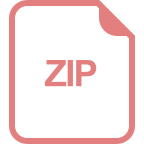
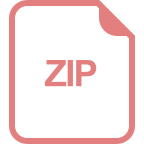
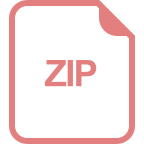
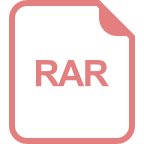
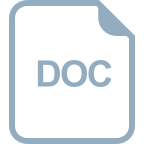
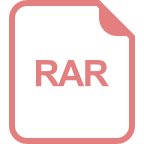
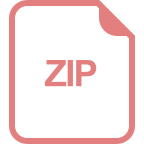
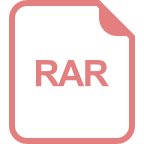
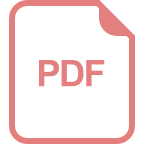
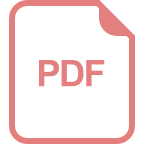
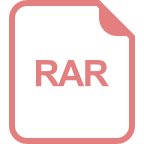
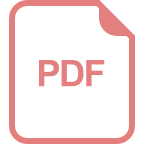
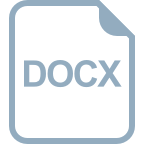
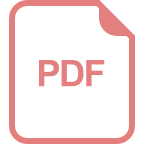
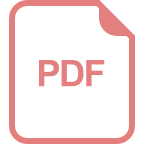
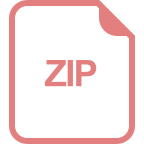
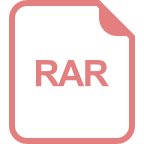
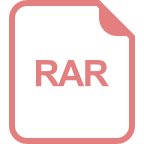
收起资源包目录



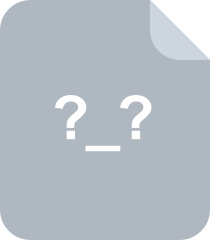
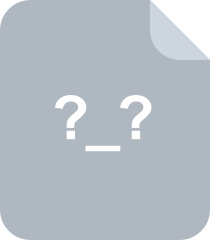
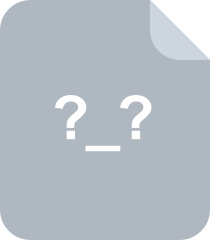
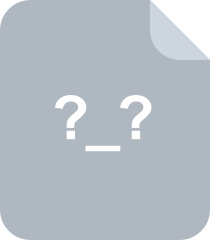
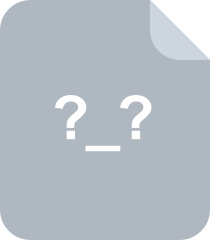
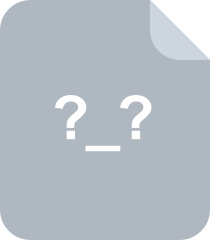
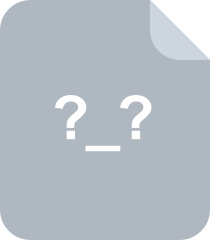
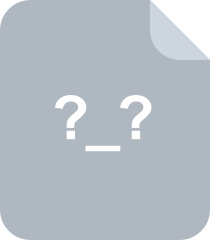
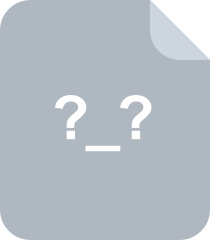
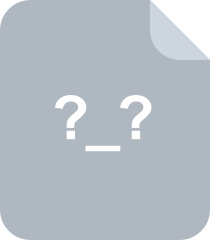
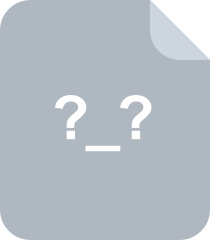
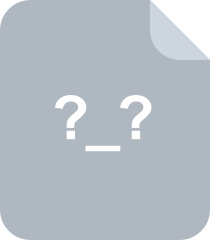
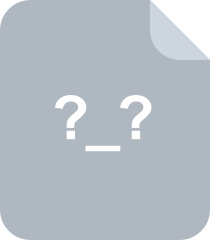
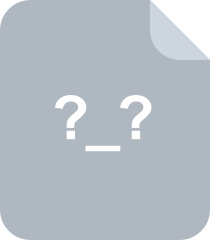
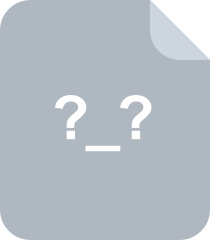



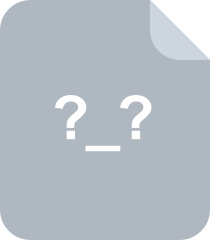


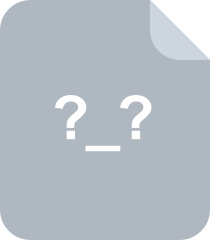
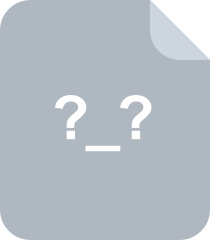
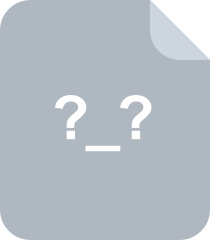


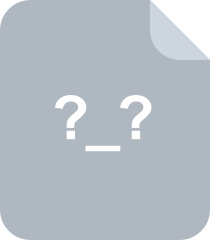
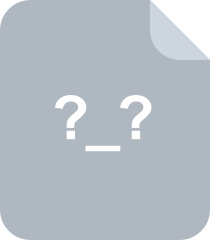
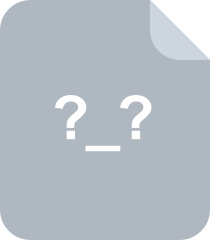

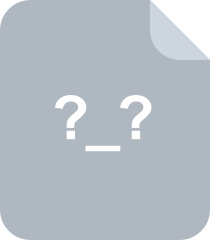

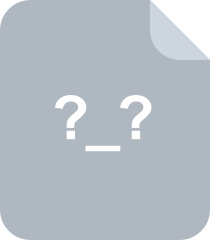
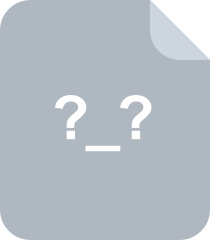


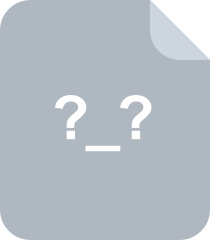
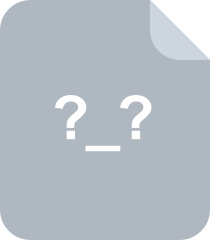
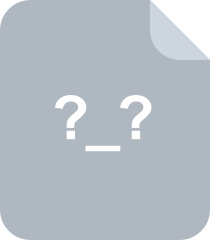


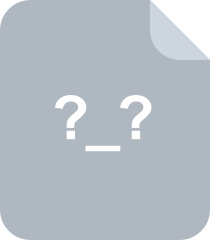
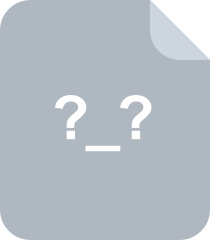
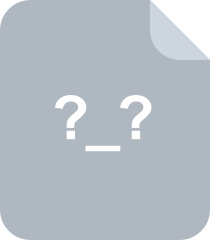



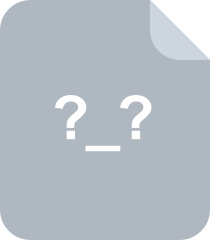
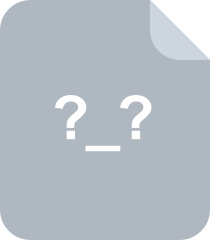
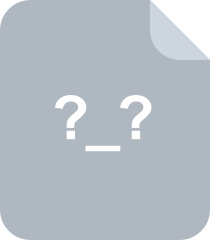


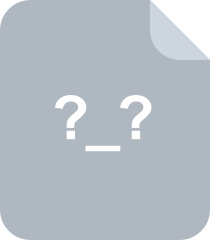
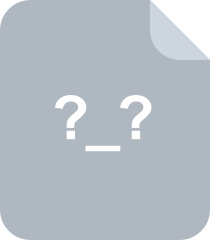
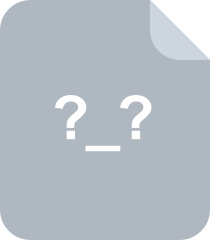

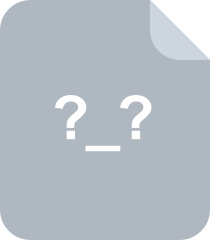
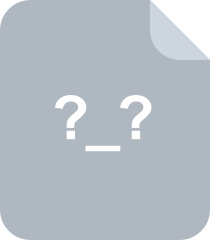
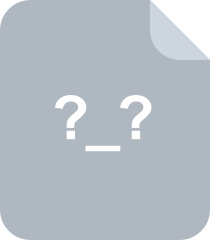
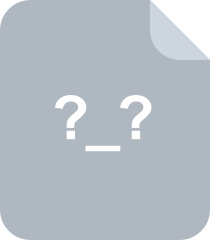

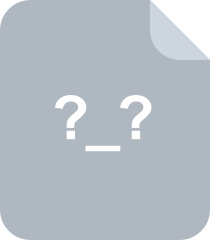
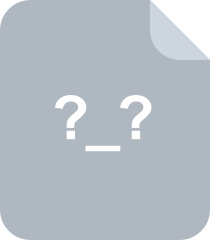
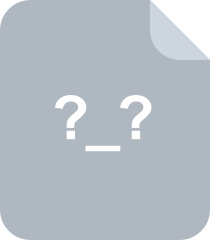
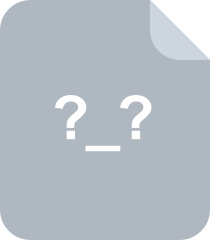
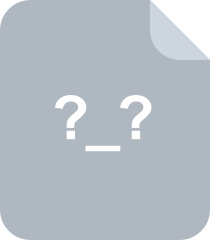
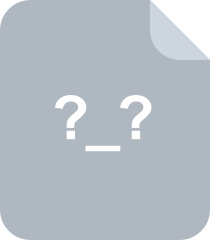
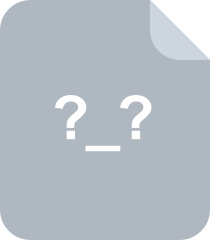
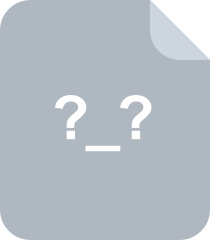
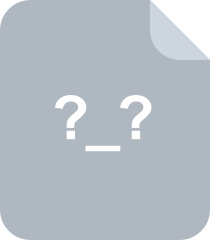
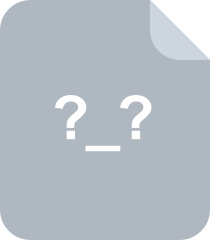
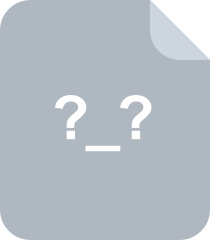
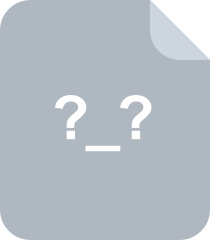


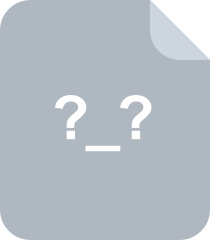
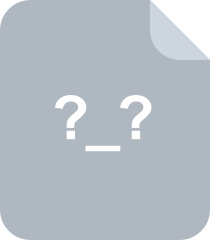
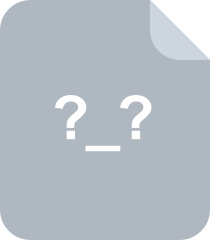
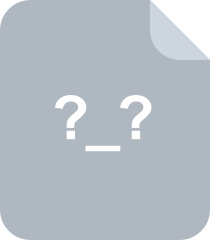
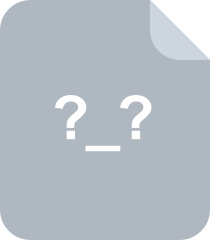
共 58 条
- 1
资源评论
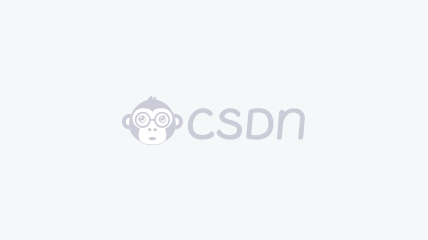
- xmys5212013-06-07不知道什么垃圾文件 一张图片都没有 36KB 根本没法用 难怪不用分
- sxdak472015-01-06没有什么用。
- qyzdmb2015-11-13还不错,学习一种新的行业形式
- 痞子纵横2013-06-01最近想做个珠宝类的网站,下载参考下,还可以~
- GJMLN2013-04-26下来看了,感觉还可以做得更好。

wwwdolsennet
- 粉丝: 1
- 资源: 1
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

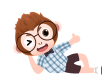
最新资源
- 一个由Java实现的游戏服务器端框架,可快速开发出易维护、高性能、高扩展能力的游戏服务器
- 生涯发展报告_编辑.pdf
- three.js开发的3D模型可视化编辑器 包含模型加载,模型文件导入导出,模型背景图,全景图,模型动画,模型灯光,模型定位,辅助线,模型辉光,模型拖拽,模型拆解, 模型材质等可视化操作编辑系统
- 全国330多个地级市一、二、三产业GDP和全国及各省土地流转和耕地面积数据-最新出炉.zip
- spring boot接口性能优化方案和spring cloud gateway网关限流实战
- 基于Netty实现的命令行斗地主游戏,新增癞子模式,德州扑克,增加超时机制,完美复现欢乐斗地主,欢迎体验在线版
- FIC7608-spec-brief-V1.1 - 20240419
- 惠普打印机(M233sdn)驱动下载
- 大飞哥本地离线AI智能抠图 1.0本地模型算法进行AI证件抠图支持单张和批量图片格式转换抠图软件
- 初学者Python入门指南:从安装到应用
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


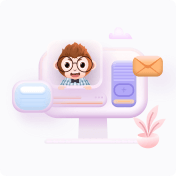
安全验证
文档复制为VIP权益,开通VIP直接复制
