Native Abstractions for Node.js
===============================
**A header file filled with macro and utility goodness for making addon development for Node.js easier across versions 0.8, 0.10 and 0.11, and eventually 0.12.**
***Current version: 0.3.2*** *(See [nan.h](https://github.com/rvagg/nan/blob/master/nan.h) for changelog)*
[](https://nodei.co/npm/nan/) [](https://nodei.co/npm/nan/)
Thanks to the crazy changes in V8 (and some in Node core), keeping native addons compiling happily across versions, particularly 0.10 to 0.11/0.12, is a minor nightmare. The goal of this project is to store all logic necessary to develop native Node.js addons without having to inspect `NODE_MODULE_VERSION` and get yourself into a macro-tangle.
This project also contains some helper utilities that make addon development a bit more pleasant.
* **[Usage](#usage)**
* **[Example](#example)**
* **[API](#api)**
<a name="usage"></a>
## Usage
Simply add **NAN** as a dependency in the *package.json* of your Node addon:
```js
"dependencies": {
...
"nan" : "~0.3.1"
...
}
```
Pull in the path to **NAN** in your *binding.gyp* so that you can use `#include "nan.h"` in your *.cpp*:
```js
"include_dirs" : [
...
"<!(node -p -e \"require('path').dirname(require.resolve('nan'))\")"
...
]
```
This works like a `-I<path-to-NAN>` when compiling your addon.
<a name="example"></a>
## Example
See **[LevelDOWN](https://github.com/rvagg/node-leveldown/pull/48)** for a full example of **NAN** in use.
For a simpler example, see the **[async pi estimation example](https://github.com/rvagg/nan/tree/master/examples/async_pi_estimate)** in the examples directory for full code and an explanation of what this Monte Carlo Pi estimation example does. Below are just some parts of the full example that illustrate the use of **NAN**.
Compare to the current 0.10 version of this example, found in the [node-addon-examples](https://github.com/rvagg/node-addon-examples/tree/master/9_async_work) repository and also a 0.11 version of the same found [here](https://github.com/kkoopa/node-addon-examples/tree/5c01f58fc993377a567812597e54a83af69686d7/9_async_work).
Note that there is no embedded version sniffing going on here and also the async work is made much simpler, see below for details on the `NanAsyncWorker` class.
```c++
// addon.cc
#include <node.h>
#include "nan.h"
// ...
using namespace v8;
void InitAll(Handle<Object> exports) {
exports->Set(NanSymbol("calculateSync"),
FunctionTemplate::New(CalculateSync)->GetFunction());
exports->Set(NanSymbol("calculateAsync"),
FunctionTemplate::New(CalculateAsync)->GetFunction());
}
NODE_MODULE(addon, InitAll)
```
```c++
// sync.h
#include <node.h>
#include "nan.h"
NAN_METHOD(CalculateSync);
```
```c++
// sync.cc
#include <node.h>
#include "nan.h"
#include "sync.h"
// ...
using namespace v8;
// Simple synchronous access to the `Estimate()` function
NAN_METHOD(CalculateSync) {
NanScope();
// expect a number as the first argument
int points = args[0]->Uint32Value();
double est = Estimate(points);
NanReturnValue(Number::New(est));
}
```
```c++
// async.cc
#include <node.h>
#include "nan.h"
#include "async.h"
// ...
using namespace v8;
class PiWorker : public NanAsyncWorker {
public:
PiWorker(NanCallback *callback, int points)
: NanAsyncWorker(callback), points(points) {}
~PiWorker() {}
// Executed inside the worker-thread.
// It is not safe to access V8, or V8 data structures
// here, so everything we need for input and output
// should go on `this`.
void Execute () {
estimate = Estimate(points);
}
// Executed when the async work is complete
// this function will be run inside the main event loop
// so it is safe to use V8 again
void HandleOKCallback () {
NanScope();
Local<Value> argv[] = {
Local<Value>::New(Null())
, Number::New(estimate)
};
callback->Call(2, argv);
};
private:
int points;
double estimate;
};
// Asynchronous access to the `Estimate()` function
NAN_METHOD(CalculateAsync) {
NanScope();
int points = args[0]->Uint32Value();
NanCallback *callback = new NanCallback(args[1].As<Function>());
NanAsyncQueueWorker(new PiWorker(callback, points));
NanReturnUndefined();
}
```
<a name="api"></a>
## API
* <a href="#api_nan_method"><b><code>NAN_METHOD</code></b></a>
* <a href="#api_nan_getter"><b><code>NAN_GETTER</code></b></a>
* <a href="#api_nan_setter"><b><code>NAN_SETTER</code></b></a>
* <a href="#api_nan_property_getter"><b><code>NAN_PROPERTY_GETTER</code></b></a>
* <a href="#api_nan_property_setter"><b><code>NAN_PROPERTY_SETTER</code></b></a>
* <a href="#api_nan_property_enumerator"><b><code>NAN_PROPERTY_ENUMERATOR</code></b></a>
* <a href="#api_nan_property_deleter"><b><code>NAN_PROPERTY_DELETER</code></b></a>
* <a href="#api_nan_property_query"><b><code>NAN_PROPERTY_QUERY</code></b></a>
* <a href="#api_nan_weak_callback"><b><code>NAN_WEAK_CALLBACK</code></b></a>
* <a href="#api_nan_return_value"><b><code>NanReturnValue</code></b></a>
* <a href="#api_nan_return_undefined"><b><code>NanReturnUndefined</code></b></a>
* <a href="#api_nan_return_null"><b><code>NanReturnNull</code></b></a>
* <a href="#api_nan_return_empty_string"><b><code>NanReturnEmptyString</code></b></a>
* <a href="#api_nan_scope"><b><code>NanScope</code></b></a>
* <a href="#api_nan_locker"><b><code>NanLocker</code></b></a>
* <a href="#api_nan_unlocker"><b><code>NanUnlocker</code></b></a>
* <a href="#api_nan_get_internal_field_pointer"><b><code>NanGetInternalFieldPointer</code></b></a>
* <a href="#api_nan_set_internal_field_pointer"><b><code>NanSetInternalFieldPointer</code></b></a>
* <a href="#api_nan_object_wrap_handle"><b><code>NanObjectWrapHandle</code></b></a>
* <a href="#api_nan_make_weak"><b><code>NanMakeWeak</code></b></a>
* <a href="#api_nan_symbol"><b><code>NanSymbol</code></b></a>
* <a href="#api_nan_get_pointer_safe"><b><code>NanGetPointerSafe</code></b></a>
* <a href="#api_nan_set_pointer_safe"><b><code>NanSetPointerSafe</code></b></a>
* <a href="#api_nan_from_v8_string"><b><code>NanFromV8String</code></b></a>
* <a href="#api_nan_boolean_option_value"><b><code>NanBooleanOptionValue</code></b></a>
* <a href="#api_nan_uint32_option_value"><b><code>NanUInt32OptionValue</code></b></a>
* <a href="#api_nan_throw_error"><b><code>NanThrowError</code></b>, <b><code>NanThrowTypeError</code></b>, <b><code>NanThrowRangeError</code></b>, <b><code>NanThrowError(Handle<Value>)</code></b>, <b><code>NanThrowError(Handle<Value>, int)</code></b></a>
* <a href="#api_nan_new_buffer_handle"><b><code>NanNewBufferHandle(char *, size_t, FreeCallback, void *)</code></b>, <b><code>NanNewBufferHandle(char *, uint32_t)</code></b>, <b><code>NanNewBufferHandle(uint32_t)</code></b></a>
* <a href="#api_nan_buffer_use"><b><code>NanBufferUse(char *, uint32_t)</code></b></a>
* <a href="#api_nan_new_context_handle"><b><code>NanNewContextHandle</code></b></a>
* <a href="#api_nan_has_instance"><b><code>NanHasInstance</code></b></a>
* <a href="#api_nan_persistent_to_local"><b><code>NanPersistentToLocal</code></b></a>
* <a href="#api_nan_dispose"><b><code>NanDispose</code></b></a>
* <a href="#api_nan_assign_persistent"><b><code>NanAssignPersistent</code></b></a>
* <a href="#api_nan_init_persistent"><b><code>NanInitPersistent</code></b></a>
* <a href="#api_nan_callback"><b><code>NanCallback</code></b></a>
* <a href="#api_nan_async_worker"><b><code>NanAsyncWorker</code></b></a>
* <a href="#api_nan_async_queue_worker"><b><code>NanAsyncQueueWorker</code></b></a>
<a name="api_nan_method"></a>
### NAN_METHOD(methodname)
Use `NAN_METHOD` to define your V8 accessible methods:
```c++
// .h:
class Foo : public node::ObjectWrap {
...
static NAN_METHOD(Bar);
static NAN_METHOD(Baz);
}
// .cc:
NAN_METHOD(Foo::Bar)
没有合适的资源?快使用搜索试试~ 我知道了~
《HTML 5网页开发实例详解》源码2
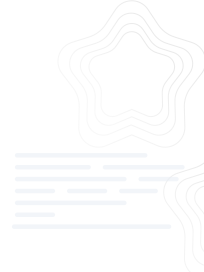
共1104个文件
js:400个
png:166个
as:112个

需积分: 13 24 下载量 138 浏览量
2015-12-06
02:23:44
上传
评论 1
收藏 51.43MB RAR 举报
温馨提示
由于上传大小权限原因,分两部分上传。详细介绍了html5语义化标签,图像操作,地理定位,本地离线存储,通信api,websocket……共14章,每章都用代码案例详细介绍了关于html5的所有高级新特性。
资源推荐
资源详情
资源评论
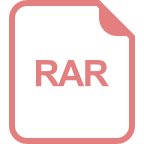
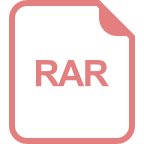
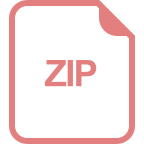
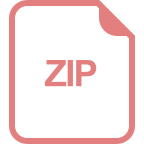
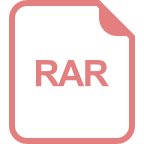
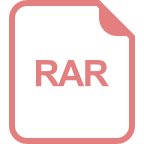
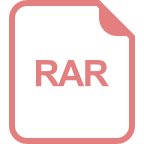
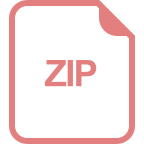
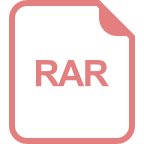
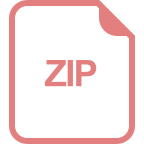
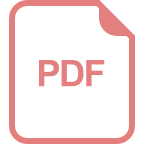
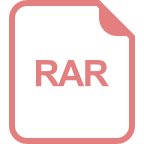
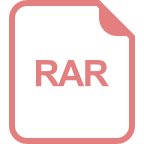
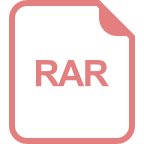
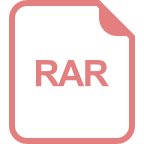
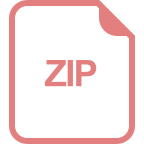
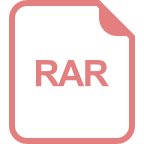
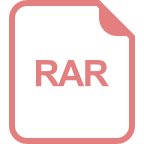
收起资源包目录

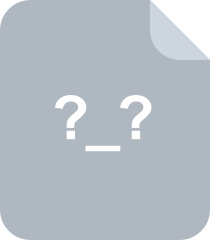
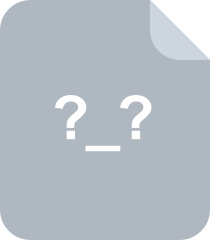
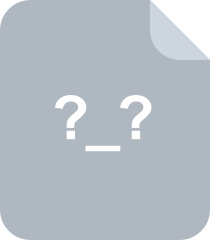
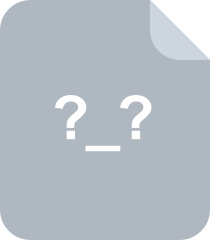
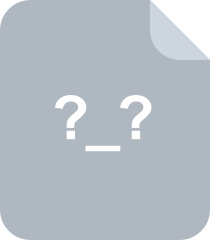
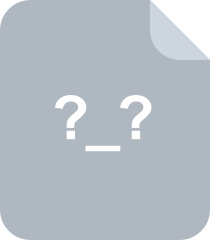
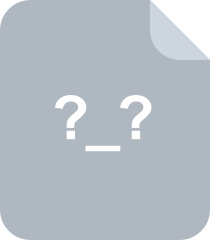
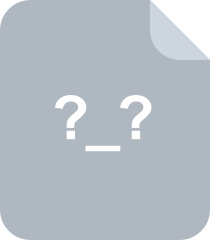
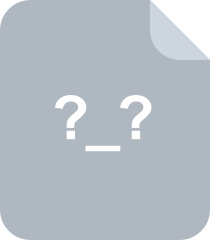
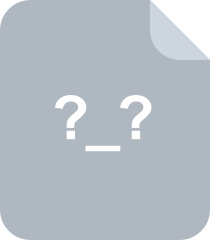
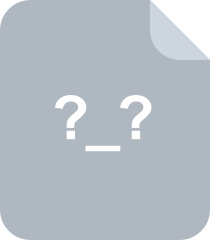
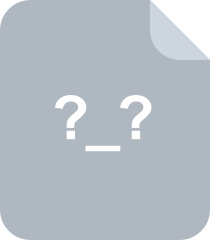
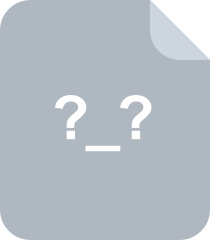
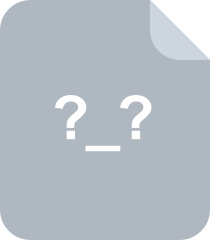
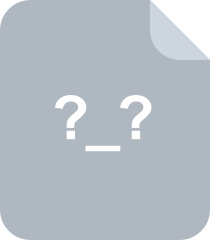
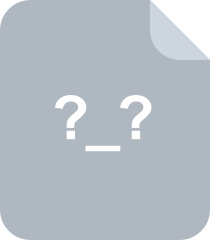
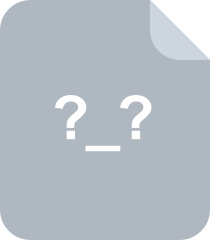
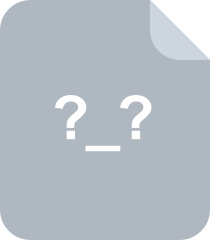
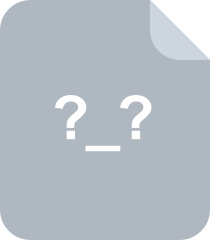
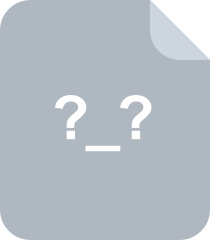
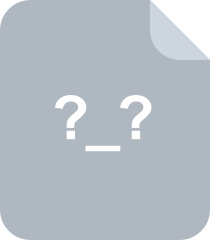
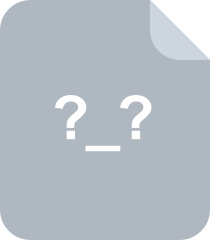
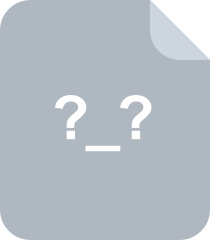
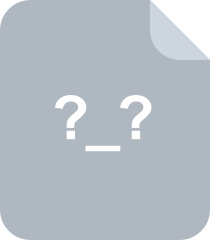
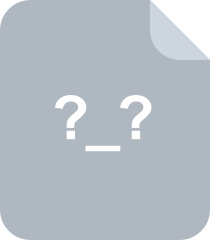
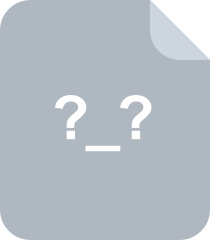
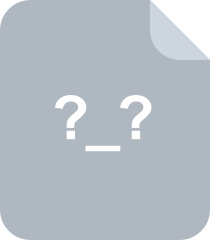
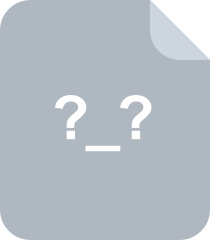
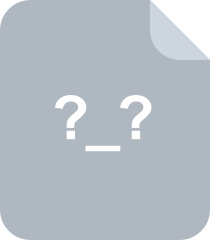
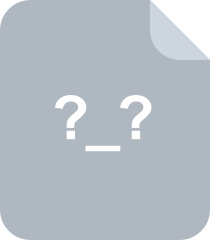
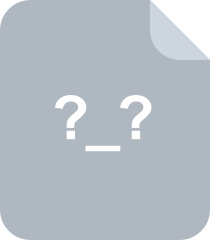
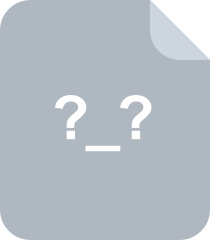
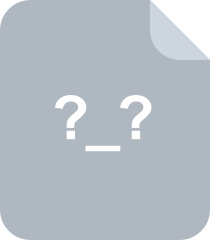
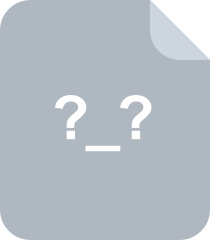
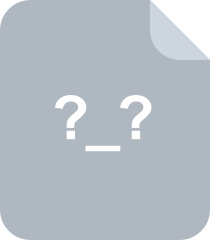
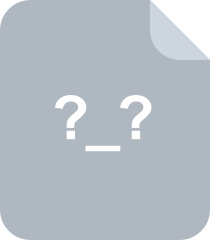
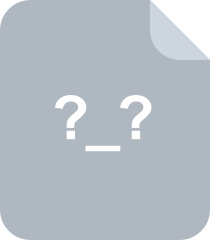
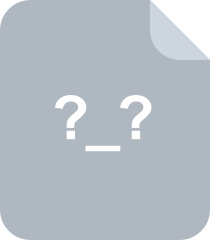
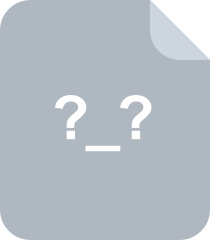
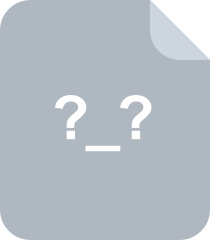
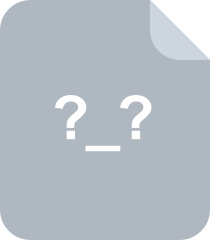
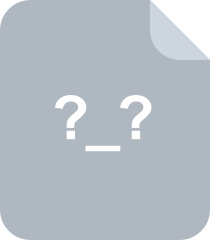
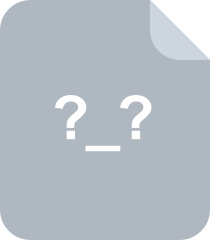
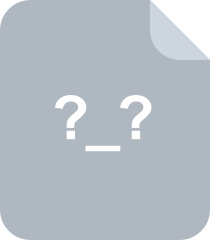
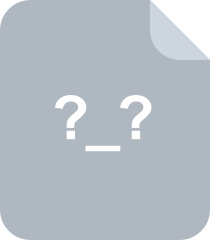
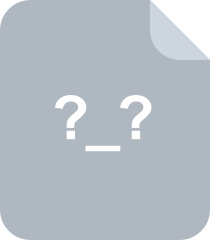
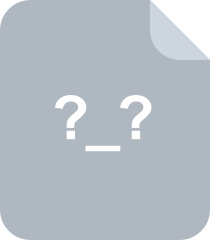
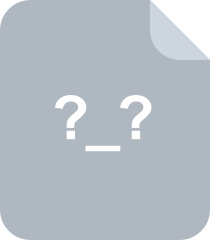
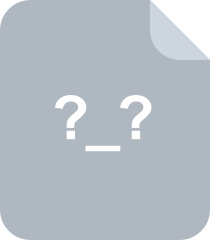
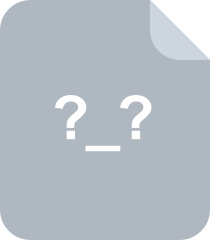
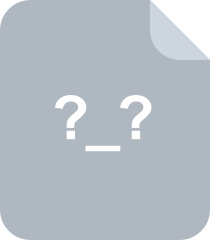
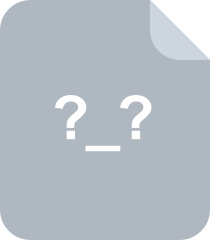
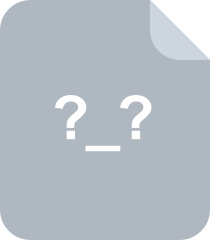
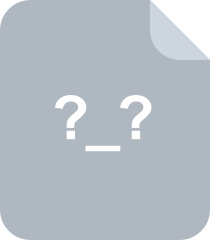
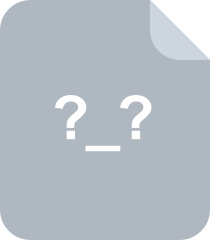
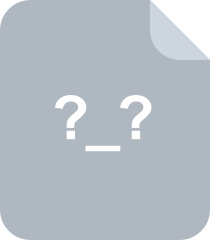
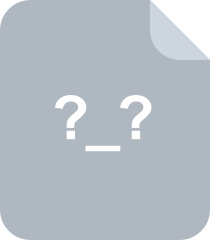
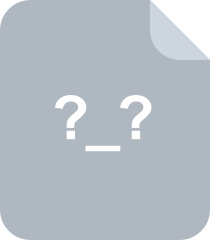
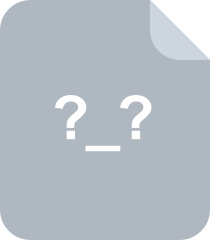
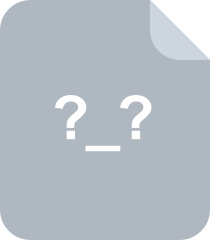
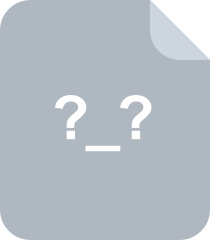
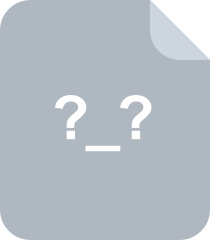
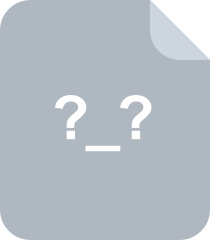
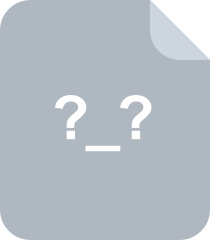
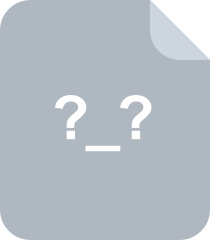
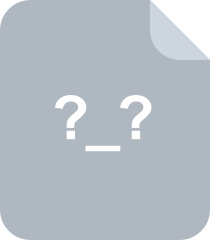
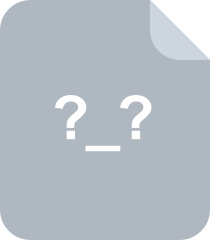
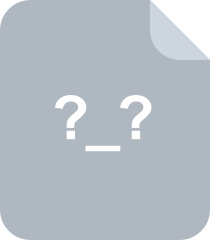
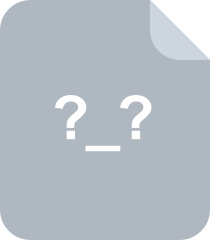
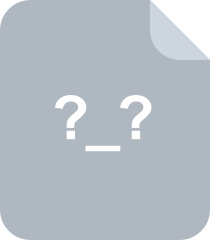
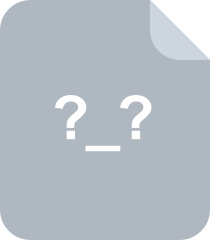
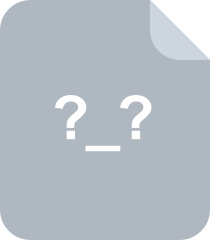
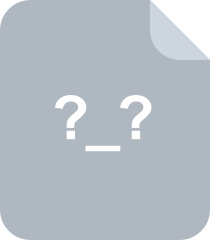
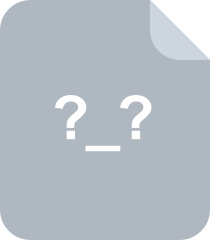
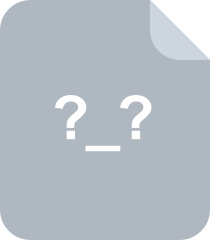
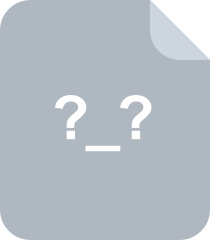
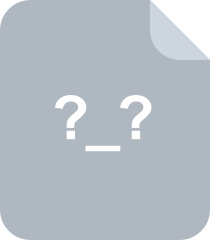
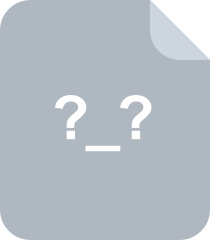
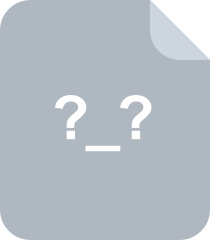
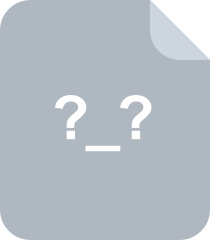
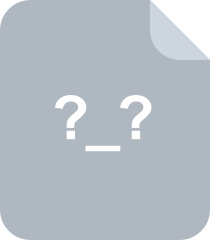
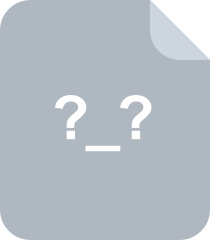
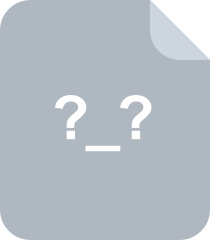
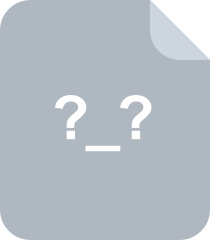
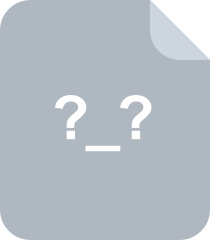
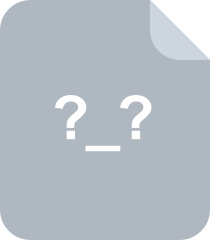
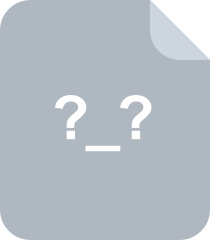
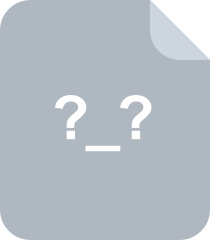
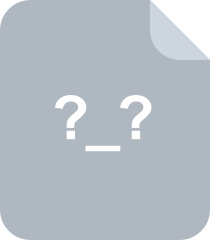
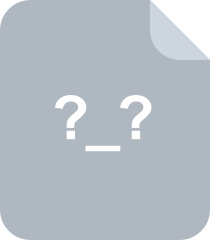
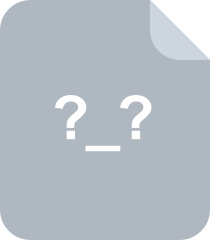
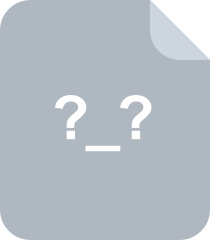
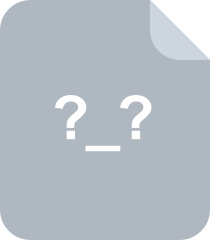
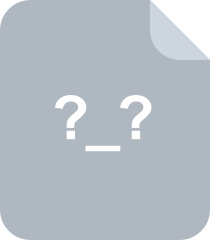
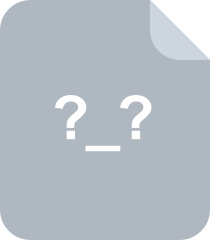
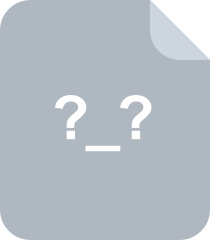
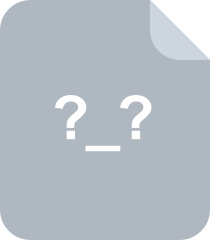
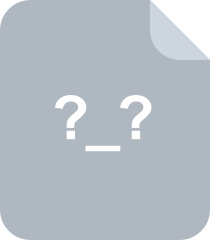
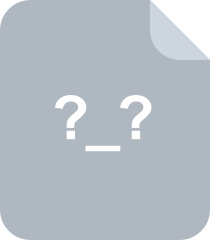
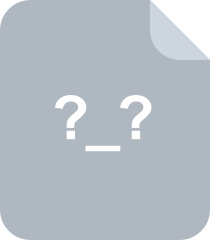
共 1104 条
- 1
- 2
- 3
- 4
- 5
- 6
- 12
资源评论
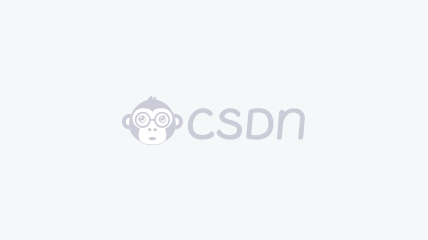

啊哈前端
- 粉丝: 73
- 资源: 7
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

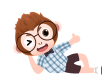
最新资源
- 学校课程软件工程常见10道题目以及答案demo
- javaweb新手开发中常见的目录结构讲解
- 新手小白的git使用的手册入门学习demo
- 基于Java观察者模式的info-express多对多广播通信框架设计源码
- 利用python爬取豆瓣电影评分简单案例demo
- 机器人开发中常见的几道问题以及答案demo
- 基于SpringBoot和layuimini的简洁美观后台权限管理系统设计源码
- 实验报告五六代码.zip
- hdw-dubbo-ui基于vue、element-ui构建开发,实现后台管理前端功能.zip
- (Grafana + Zabbix + ASP.NET Core 2.1 + ECharts + Dapper + Swagger + layuiAdmin)基于角色授权的权限体系.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


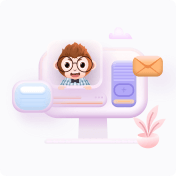
安全验证
文档复制为VIP权益,开通VIP直接复制
